OpenCV EAST Text detector implementation in Java
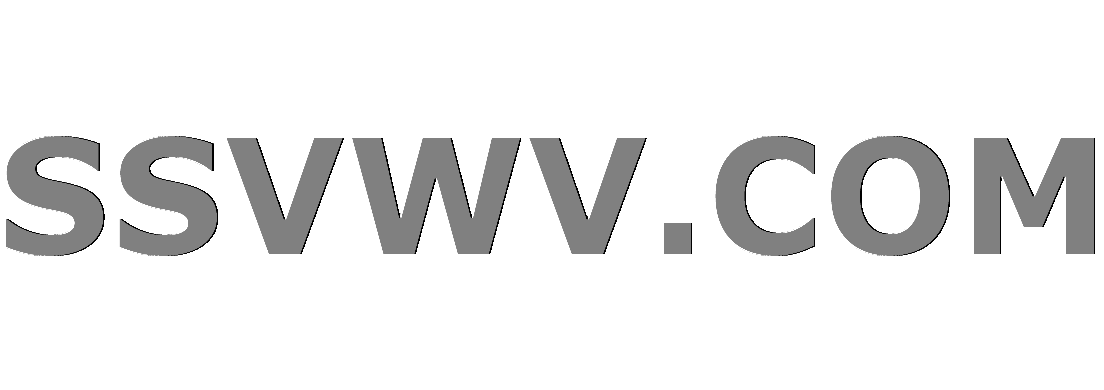
Multi tool use
I am trying to convert the text detection example from the below page in Java. The original code is in C++.
https://github.com/opencv/opencv/blob/master/samples/dnn/text_detection.cpp
But I am facing issues in converting the below lines (131-136 in the cpp file) in Java:
...
const float* scoresData = scores.ptr<float>(0, 0, y);
const float* x0_data = geometry.ptr<float>(0, 0, y);
const float* x1_data = geometry.ptr<float>(0, 1, y);
...
I tried using every method from the openCV Mat class but most of them throw exceptions!
My code till now is as below:
Net net = Dnn.readNet("C:\frozen_east_text_detection.pb");
Mat blob = Dnn.blobFromImage(resizedImg, 1.0, resizedImg.size(), new Scalar(123.68, 116.78, 103.94), true,
false);
net.setInput(blob);
List<Mat> outs = new ArrayList<>();
net.forward(outs, LAYER_NAMES);
Mat scores = outs.get(0);
Mat geometry = outs.get(1);
int numRows = scores.size(2);
int numCols = scores.size(3);
List<RotatedRect> boxes = new ArrayList<>();
List<Double> confidences = new ArrayList<>();
System.out.printf("numRows = %dn", scores.size(2));
System.out.printf("numCols = %dn", scores.size(3));
I am not very familiar with pointers, but what I could understand is the scores
native object seems to be a 4-D array, but it is a class in Java and there is no way in java to address a class with indices, like possible in C++ as shown, or in python as below (conversion of cpp program to python on another website):
for y in range(0, numRows):
scoresData = scores[0, 0, y]
xData0 = geometry[0, 0, y]
xData1 = geometry[0, 1, y]
xData2 = geometry[0, 2, y]
xData3 = geometry[0, 3, y]
anglesData = geometry[0, 4, y]
java opencv
|
show 1 more comment
I am trying to convert the text detection example from the below page in Java. The original code is in C++.
https://github.com/opencv/opencv/blob/master/samples/dnn/text_detection.cpp
But I am facing issues in converting the below lines (131-136 in the cpp file) in Java:
...
const float* scoresData = scores.ptr<float>(0, 0, y);
const float* x0_data = geometry.ptr<float>(0, 0, y);
const float* x1_data = geometry.ptr<float>(0, 1, y);
...
I tried using every method from the openCV Mat class but most of them throw exceptions!
My code till now is as below:
Net net = Dnn.readNet("C:\frozen_east_text_detection.pb");
Mat blob = Dnn.blobFromImage(resizedImg, 1.0, resizedImg.size(), new Scalar(123.68, 116.78, 103.94), true,
false);
net.setInput(blob);
List<Mat> outs = new ArrayList<>();
net.forward(outs, LAYER_NAMES);
Mat scores = outs.get(0);
Mat geometry = outs.get(1);
int numRows = scores.size(2);
int numCols = scores.size(3);
List<RotatedRect> boxes = new ArrayList<>();
List<Double> confidences = new ArrayList<>();
System.out.printf("numRows = %dn", scores.size(2));
System.out.printf("numCols = %dn", scores.size(3));
I am not very familiar with pointers, but what I could understand is the scores
native object seems to be a 4-D array, but it is a class in Java and there is no way in java to address a class with indices, like possible in C++ as shown, or in python as below (conversion of cpp program to python on another website):
for y in range(0, numRows):
scoresData = scores[0, 0, y]
xData0 = geometry[0, 0, y]
xData1 = geometry[0, 1, y]
xData2 = geometry[0, 2, y]
xData3 = geometry[0, 3, y]
anglesData = geometry[0, 4, y]
java opencv
1
Maybe you couldreshape
thoseMat
s it to get rid of the first two dimensions? Then it would be just some simple arithmetics to calculate the right position to read from. | It does seem that the Java bindings are somewhat limited regardingMat
s with more than 2 dimensions.
– Dan Mašek
Nov 20 at 22:22
Can you guide me a little? I am out of my depth here. What are channels, and what exactly does reshaping do?
– inquizitive
Nov 21 at 0:14
Channels, speaking about regularMat
instances, would refer to the third (implicit) dimension. For example, an RGB image is considered a 2dMat
with 3 channels. The two dimensions are width and height, and channels corresponding to the red, green and white components.
– Dan Mašek
Nov 21 at 0:34
Reshaping... the data of aMat
is stored in a linear array (the details of the layout are in the C++ documentation). There's a header which contains the information about the "shape" (size of all the dimensions) which determines how coordinates map to the underlying data array. Best to make an example... imagine you have a RGB (3 channel) image with 10 rows and 10 columns. You can reshape this into a 1 channelMat
with 100 rows and 3 columns. The result has each pixel from the original per row, first column is Red and so on.
– Dan Mašek
Nov 21 at 0:43
1
It's a 100 mb file that I got from the below URL: bitbucket.org/tomhoag/opencv-text-detection/src/… . I uploaded it here just in case: gofile.io/?c=JkdZkN
– inquizitive
Nov 21 at 2:09
|
show 1 more comment
I am trying to convert the text detection example from the below page in Java. The original code is in C++.
https://github.com/opencv/opencv/blob/master/samples/dnn/text_detection.cpp
But I am facing issues in converting the below lines (131-136 in the cpp file) in Java:
...
const float* scoresData = scores.ptr<float>(0, 0, y);
const float* x0_data = geometry.ptr<float>(0, 0, y);
const float* x1_data = geometry.ptr<float>(0, 1, y);
...
I tried using every method from the openCV Mat class but most of them throw exceptions!
My code till now is as below:
Net net = Dnn.readNet("C:\frozen_east_text_detection.pb");
Mat blob = Dnn.blobFromImage(resizedImg, 1.0, resizedImg.size(), new Scalar(123.68, 116.78, 103.94), true,
false);
net.setInput(blob);
List<Mat> outs = new ArrayList<>();
net.forward(outs, LAYER_NAMES);
Mat scores = outs.get(0);
Mat geometry = outs.get(1);
int numRows = scores.size(2);
int numCols = scores.size(3);
List<RotatedRect> boxes = new ArrayList<>();
List<Double> confidences = new ArrayList<>();
System.out.printf("numRows = %dn", scores.size(2));
System.out.printf("numCols = %dn", scores.size(3));
I am not very familiar with pointers, but what I could understand is the scores
native object seems to be a 4-D array, but it is a class in Java and there is no way in java to address a class with indices, like possible in C++ as shown, or in python as below (conversion of cpp program to python on another website):
for y in range(0, numRows):
scoresData = scores[0, 0, y]
xData0 = geometry[0, 0, y]
xData1 = geometry[0, 1, y]
xData2 = geometry[0, 2, y]
xData3 = geometry[0, 3, y]
anglesData = geometry[0, 4, y]
java opencv
I am trying to convert the text detection example from the below page in Java. The original code is in C++.
https://github.com/opencv/opencv/blob/master/samples/dnn/text_detection.cpp
But I am facing issues in converting the below lines (131-136 in the cpp file) in Java:
...
const float* scoresData = scores.ptr<float>(0, 0, y);
const float* x0_data = geometry.ptr<float>(0, 0, y);
const float* x1_data = geometry.ptr<float>(0, 1, y);
...
I tried using every method from the openCV Mat class but most of them throw exceptions!
My code till now is as below:
Net net = Dnn.readNet("C:\frozen_east_text_detection.pb");
Mat blob = Dnn.blobFromImage(resizedImg, 1.0, resizedImg.size(), new Scalar(123.68, 116.78, 103.94), true,
false);
net.setInput(blob);
List<Mat> outs = new ArrayList<>();
net.forward(outs, LAYER_NAMES);
Mat scores = outs.get(0);
Mat geometry = outs.get(1);
int numRows = scores.size(2);
int numCols = scores.size(3);
List<RotatedRect> boxes = new ArrayList<>();
List<Double> confidences = new ArrayList<>();
System.out.printf("numRows = %dn", scores.size(2));
System.out.printf("numCols = %dn", scores.size(3));
I am not very familiar with pointers, but what I could understand is the scores
native object seems to be a 4-D array, but it is a class in Java and there is no way in java to address a class with indices, like possible in C++ as shown, or in python as below (conversion of cpp program to python on another website):
for y in range(0, numRows):
scoresData = scores[0, 0, y]
xData0 = geometry[0, 0, y]
xData1 = geometry[0, 1, y]
xData2 = geometry[0, 2, y]
xData3 = geometry[0, 3, y]
anglesData = geometry[0, 4, y]
java opencv
java opencv
asked Nov 20 at 21:46
inquizitive
359116
359116
1
Maybe you couldreshape
thoseMat
s it to get rid of the first two dimensions? Then it would be just some simple arithmetics to calculate the right position to read from. | It does seem that the Java bindings are somewhat limited regardingMat
s with more than 2 dimensions.
– Dan Mašek
Nov 20 at 22:22
Can you guide me a little? I am out of my depth here. What are channels, and what exactly does reshaping do?
– inquizitive
Nov 21 at 0:14
Channels, speaking about regularMat
instances, would refer to the third (implicit) dimension. For example, an RGB image is considered a 2dMat
with 3 channels. The two dimensions are width and height, and channels corresponding to the red, green and white components.
– Dan Mašek
Nov 21 at 0:34
Reshaping... the data of aMat
is stored in a linear array (the details of the layout are in the C++ documentation). There's a header which contains the information about the "shape" (size of all the dimensions) which determines how coordinates map to the underlying data array. Best to make an example... imagine you have a RGB (3 channel) image with 10 rows and 10 columns. You can reshape this into a 1 channelMat
with 100 rows and 3 columns. The result has each pixel from the original per row, first column is Red and so on.
– Dan Mašek
Nov 21 at 0:43
1
It's a 100 mb file that I got from the below URL: bitbucket.org/tomhoag/opencv-text-detection/src/… . I uploaded it here just in case: gofile.io/?c=JkdZkN
– inquizitive
Nov 21 at 2:09
|
show 1 more comment
1
Maybe you couldreshape
thoseMat
s it to get rid of the first two dimensions? Then it would be just some simple arithmetics to calculate the right position to read from. | It does seem that the Java bindings are somewhat limited regardingMat
s with more than 2 dimensions.
– Dan Mašek
Nov 20 at 22:22
Can you guide me a little? I am out of my depth here. What are channels, and what exactly does reshaping do?
– inquizitive
Nov 21 at 0:14
Channels, speaking about regularMat
instances, would refer to the third (implicit) dimension. For example, an RGB image is considered a 2dMat
with 3 channels. The two dimensions are width and height, and channels corresponding to the red, green and white components.
– Dan Mašek
Nov 21 at 0:34
Reshaping... the data of aMat
is stored in a linear array (the details of the layout are in the C++ documentation). There's a header which contains the information about the "shape" (size of all the dimensions) which determines how coordinates map to the underlying data array. Best to make an example... imagine you have a RGB (3 channel) image with 10 rows and 10 columns. You can reshape this into a 1 channelMat
with 100 rows and 3 columns. The result has each pixel from the original per row, first column is Red and so on.
– Dan Mašek
Nov 21 at 0:43
1
It's a 100 mb file that I got from the below URL: bitbucket.org/tomhoag/opencv-text-detection/src/… . I uploaded it here just in case: gofile.io/?c=JkdZkN
– inquizitive
Nov 21 at 2:09
1
1
Maybe you could
reshape
those Mat
s it to get rid of the first two dimensions? Then it would be just some simple arithmetics to calculate the right position to read from. | It does seem that the Java bindings are somewhat limited regarding Mat
s with more than 2 dimensions.– Dan Mašek
Nov 20 at 22:22
Maybe you could
reshape
those Mat
s it to get rid of the first two dimensions? Then it would be just some simple arithmetics to calculate the right position to read from. | It does seem that the Java bindings are somewhat limited regarding Mat
s with more than 2 dimensions.– Dan Mašek
Nov 20 at 22:22
Can you guide me a little? I am out of my depth here. What are channels, and what exactly does reshaping do?
– inquizitive
Nov 21 at 0:14
Can you guide me a little? I am out of my depth here. What are channels, and what exactly does reshaping do?
– inquizitive
Nov 21 at 0:14
Channels, speaking about regular
Mat
instances, would refer to the third (implicit) dimension. For example, an RGB image is considered a 2d Mat
with 3 channels. The two dimensions are width and height, and channels corresponding to the red, green and white components.– Dan Mašek
Nov 21 at 0:34
Channels, speaking about regular
Mat
instances, would refer to the third (implicit) dimension. For example, an RGB image is considered a 2d Mat
with 3 channels. The two dimensions are width and height, and channels corresponding to the red, green and white components.– Dan Mašek
Nov 21 at 0:34
Reshaping... the data of a
Mat
is stored in a linear array (the details of the layout are in the C++ documentation). There's a header which contains the information about the "shape" (size of all the dimensions) which determines how coordinates map to the underlying data array. Best to make an example... imagine you have a RGB (3 channel) image with 10 rows and 10 columns. You can reshape this into a 1 channel Mat
with 100 rows and 3 columns. The result has each pixel from the original per row, first column is Red and so on.– Dan Mašek
Nov 21 at 0:43
Reshaping... the data of a
Mat
is stored in a linear array (the details of the layout are in the C++ documentation). There's a header which contains the information about the "shape" (size of all the dimensions) which determines how coordinates map to the underlying data array. Best to make an example... imagine you have a RGB (3 channel) image with 10 rows and 10 columns. You can reshape this into a 1 channel Mat
with 100 rows and 3 columns. The result has each pixel from the original per row, first column is Red and so on.– Dan Mašek
Nov 21 at 0:43
1
1
It's a 100 mb file that I got from the below URL: bitbucket.org/tomhoag/opencv-text-detection/src/… . I uploaded it here just in case: gofile.io/?c=JkdZkN
– inquizitive
Nov 21 at 2:09
It's a 100 mb file that I got from the below URL: bitbucket.org/tomhoag/opencv-text-detection/src/… . I uploaded it here just in case: gofile.io/?c=JkdZkN
– inquizitive
Nov 21 at 2:09
|
show 1 more comment
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402064%2fopencv-east-text-detector-implementation-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402064%2fopencv-east-text-detector-implementation-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
4ghCMOBqOP,qs,E
1
Maybe you could
reshape
thoseMat
s it to get rid of the first two dimensions? Then it would be just some simple arithmetics to calculate the right position to read from. | It does seem that the Java bindings are somewhat limited regardingMat
s with more than 2 dimensions.– Dan Mašek
Nov 20 at 22:22
Can you guide me a little? I am out of my depth here. What are channels, and what exactly does reshaping do?
– inquizitive
Nov 21 at 0:14
Channels, speaking about regular
Mat
instances, would refer to the third (implicit) dimension. For example, an RGB image is considered a 2dMat
with 3 channels. The two dimensions are width and height, and channels corresponding to the red, green and white components.– Dan Mašek
Nov 21 at 0:34
Reshaping... the data of a
Mat
is stored in a linear array (the details of the layout are in the C++ documentation). There's a header which contains the information about the "shape" (size of all the dimensions) which determines how coordinates map to the underlying data array. Best to make an example... imagine you have a RGB (3 channel) image with 10 rows and 10 columns. You can reshape this into a 1 channelMat
with 100 rows and 3 columns. The result has each pixel from the original per row, first column is Red and so on.– Dan Mašek
Nov 21 at 0:43
1
It's a 100 mb file that I got from the below URL: bitbucket.org/tomhoag/opencv-text-detection/src/… . I uploaded it here just in case: gofile.io/?c=JkdZkN
– inquizitive
Nov 21 at 2:09