Optimal Alphabet Stepping
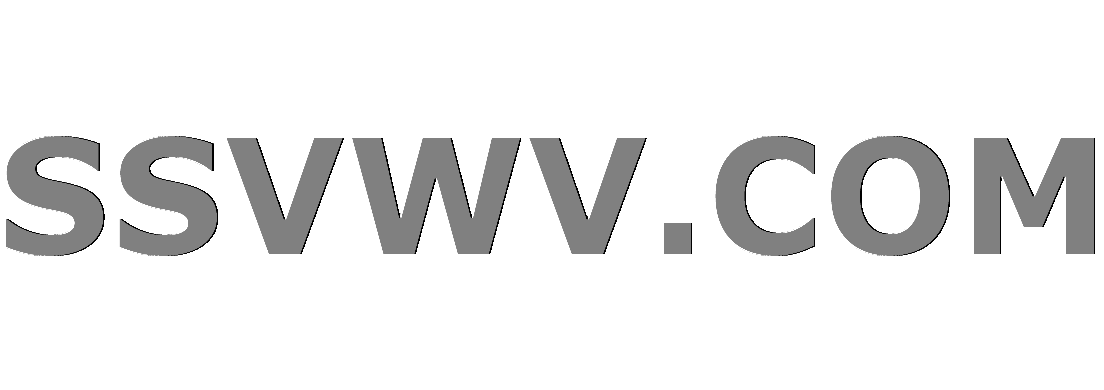
Multi tool use
up vote
6
down vote
favorite
Given an input string consisting of only letters, return the step-size that results in the minimum amount of steps that are needed to visit all the letters in order over a wrapping alphabet, starting at any letter.
For example, take the word, dog
. If we use a step-size of 1, we end up with:
defghijklmnopqrstuvwxyzabcdefg Alphabet
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
defghijklmnopqrstuvwxyzabcdefg Visited letters
d o g Needed letters
For a total of 30 steps.
However, if we use a step-size of 11, we get:
defghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdefg
^ ^ ^ ^ ^ ^
d o z k v g Visited letters
d o g Needed letters
For a total of 6 steps. This is the minimum amount of steps, so the return result for dog
is the step-size; 11
.
Test cases:
"dog" -> 11
"age" -> 6
"apple" -> 19
"alphabet" -> 9
"aaaaaaa" -> 0 for 0 indexed, 26 for 1 indexed
"abcdefga" -> 1 or 9
"aba" -> Any odd number except for 13
"ppcg" -> 15
"codegolf" -> 15
"testcase" -> 9
"z" -> Any number
"joking" -> 19
Rules
- Input will be a non-empty string consisting only of the letters
a
toz
(you can choose between uppercase or lowercase) - Output can be 0 indexed (i.e. the range
0-25
) or 1 indexed (1-26
) - If there's a tie, you can output any step-size or all of them
- This is code-golf, so the lowest amount of bytes for each language wins!
code-golf alphabet
add a comment |
up vote
6
down vote
favorite
Given an input string consisting of only letters, return the step-size that results in the minimum amount of steps that are needed to visit all the letters in order over a wrapping alphabet, starting at any letter.
For example, take the word, dog
. If we use a step-size of 1, we end up with:
defghijklmnopqrstuvwxyzabcdefg Alphabet
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
defghijklmnopqrstuvwxyzabcdefg Visited letters
d o g Needed letters
For a total of 30 steps.
However, if we use a step-size of 11, we get:
defghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdefg
^ ^ ^ ^ ^ ^
d o z k v g Visited letters
d o g Needed letters
For a total of 6 steps. This is the minimum amount of steps, so the return result for dog
is the step-size; 11
.
Test cases:
"dog" -> 11
"age" -> 6
"apple" -> 19
"alphabet" -> 9
"aaaaaaa" -> 0 for 0 indexed, 26 for 1 indexed
"abcdefga" -> 1 or 9
"aba" -> Any odd number except for 13
"ppcg" -> 15
"codegolf" -> 15
"testcase" -> 9
"z" -> Any number
"joking" -> 19
Rules
- Input will be a non-empty string consisting only of the letters
a
toz
(you can choose between uppercase or lowercase) - Output can be 0 indexed (i.e. the range
0-25
) or 1 indexed (1-26
) - If there's a tie, you can output any step-size or all of them
- This is code-golf, so the lowest amount of bytes for each language wins!
code-golf alphabet
Do we need to handle empty input?
– pizzapants184
2 hours ago
1
@pizzapants184 No. I've updated the question to specify the input will be non-empty
– Jo King
2 hours ago
1
@pizzapants184 Thanks, updating the question. Please let me know if any more of my test cases are wrong. I think that was left over from a previous iteration of the question
– Jo King
1 hour ago
Can we take input as an array of characters?
– Shaggy
8 mins ago
add a comment |
up vote
6
down vote
favorite
up vote
6
down vote
favorite
Given an input string consisting of only letters, return the step-size that results in the minimum amount of steps that are needed to visit all the letters in order over a wrapping alphabet, starting at any letter.
For example, take the word, dog
. If we use a step-size of 1, we end up with:
defghijklmnopqrstuvwxyzabcdefg Alphabet
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
defghijklmnopqrstuvwxyzabcdefg Visited letters
d o g Needed letters
For a total of 30 steps.
However, if we use a step-size of 11, we get:
defghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdefg
^ ^ ^ ^ ^ ^
d o z k v g Visited letters
d o g Needed letters
For a total of 6 steps. This is the minimum amount of steps, so the return result for dog
is the step-size; 11
.
Test cases:
"dog" -> 11
"age" -> 6
"apple" -> 19
"alphabet" -> 9
"aaaaaaa" -> 0 for 0 indexed, 26 for 1 indexed
"abcdefga" -> 1 or 9
"aba" -> Any odd number except for 13
"ppcg" -> 15
"codegolf" -> 15
"testcase" -> 9
"z" -> Any number
"joking" -> 19
Rules
- Input will be a non-empty string consisting only of the letters
a
toz
(you can choose between uppercase or lowercase) - Output can be 0 indexed (i.e. the range
0-25
) or 1 indexed (1-26
) - If there's a tie, you can output any step-size or all of them
- This is code-golf, so the lowest amount of bytes for each language wins!
code-golf alphabet
Given an input string consisting of only letters, return the step-size that results in the minimum amount of steps that are needed to visit all the letters in order over a wrapping alphabet, starting at any letter.
For example, take the word, dog
. If we use a step-size of 1, we end up with:
defghijklmnopqrstuvwxyzabcdefg Alphabet
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
defghijklmnopqrstuvwxyzabcdefg Visited letters
d o g Needed letters
For a total of 30 steps.
However, if we use a step-size of 11, we get:
defghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdefg
^ ^ ^ ^ ^ ^
d o z k v g Visited letters
d o g Needed letters
For a total of 6 steps. This is the minimum amount of steps, so the return result for dog
is the step-size; 11
.
Test cases:
"dog" -> 11
"age" -> 6
"apple" -> 19
"alphabet" -> 9
"aaaaaaa" -> 0 for 0 indexed, 26 for 1 indexed
"abcdefga" -> 1 or 9
"aba" -> Any odd number except for 13
"ppcg" -> 15
"codegolf" -> 15
"testcase" -> 9
"z" -> Any number
"joking" -> 19
Rules
- Input will be a non-empty string consisting only of the letters
a
toz
(you can choose between uppercase or lowercase) - Output can be 0 indexed (i.e. the range
0-25
) or 1 indexed (1-26
) - If there's a tie, you can output any step-size or all of them
- This is code-golf, so the lowest amount of bytes for each language wins!
code-golf alphabet
code-golf alphabet
edited 1 hour ago
asked 3 hours ago
Jo King
20.2k245106
20.2k245106
Do we need to handle empty input?
– pizzapants184
2 hours ago
1
@pizzapants184 No. I've updated the question to specify the input will be non-empty
– Jo King
2 hours ago
1
@pizzapants184 Thanks, updating the question. Please let me know if any more of my test cases are wrong. I think that was left over from a previous iteration of the question
– Jo King
1 hour ago
Can we take input as an array of characters?
– Shaggy
8 mins ago
add a comment |
Do we need to handle empty input?
– pizzapants184
2 hours ago
1
@pizzapants184 No. I've updated the question to specify the input will be non-empty
– Jo King
2 hours ago
1
@pizzapants184 Thanks, updating the question. Please let me know if any more of my test cases are wrong. I think that was left over from a previous iteration of the question
– Jo King
1 hour ago
Can we take input as an array of characters?
– Shaggy
8 mins ago
Do we need to handle empty input?
– pizzapants184
2 hours ago
Do we need to handle empty input?
– pizzapants184
2 hours ago
1
1
@pizzapants184 No. I've updated the question to specify the input will be non-empty
– Jo King
2 hours ago
@pizzapants184 No. I've updated the question to specify the input will be non-empty
– Jo King
2 hours ago
1
1
@pizzapants184 Thanks, updating the question. Please let me know if any more of my test cases are wrong. I think that was left over from a previous iteration of the question
– Jo King
1 hour ago
@pizzapants184 Thanks, updating the question. Please let me know if any more of my test cases are wrong. I think that was left over from a previous iteration of the question
– Jo King
1 hour ago
Can we take input as an array of characters?
– Shaggy
8 mins ago
Can we take input as an array of characters?
– Shaggy
8 mins ago
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
Python 2, 222 bytes
A=map(chr,range(65,91))
def t(s,l,S=0):
a=i=A.index(s[0])
for c in s[1:]:
while a!=A.index(c):
S+=1;a+=l;a%=26
if a==i:return float('inf')
i=a
return S
def f(s):T=[t(s,l)for l in range(26)];return T.index(min(T))
Try it online!
-8 bytes from Jo King
This would be shorter in a language with a prime number of letters (wouldn't need the Actually, this submission would still need that for handling strings like "aaa".float('inf')
handling of infinite loops).
This submission is 0-indexed (returns values from 0 to 25 inclusive).
f
takes a(n uppercase) string and returns the Optimal Alphabet Stepping
t
is a helper function that takes the string and an alphabet stepping and returns the number of hops needed to finish the string (or float('inf')
if impossible).
1
216 bytes
– Jo King
1 hour ago
Usewhile a!=A(c)and S<len(s)*26:
and you may removeif a==i:return float('inf')
, sincelen(s)*26
is upper bound of any answer.
– tsh
22 mins ago
add a comment |
up vote
1
down vote
JavaScript, 153 bytes
w=>[...Array(26)].map((_,i)=>i).map((_,s,a)=>~[...w].map(c=>(t+=a.find(v=>!p|(u(c,36)+~v*s-u(p,36))%26==0),p=c),p=t=0,u=parseInt)+t<m&&(m=t,n=s),m=1/0)|n
Try it online!
144 bytes
– Shaggy
7 mins ago
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
Python 2, 222 bytes
A=map(chr,range(65,91))
def t(s,l,S=0):
a=i=A.index(s[0])
for c in s[1:]:
while a!=A.index(c):
S+=1;a+=l;a%=26
if a==i:return float('inf')
i=a
return S
def f(s):T=[t(s,l)for l in range(26)];return T.index(min(T))
Try it online!
-8 bytes from Jo King
This would be shorter in a language with a prime number of letters (wouldn't need the Actually, this submission would still need that for handling strings like "aaa".float('inf')
handling of infinite loops).
This submission is 0-indexed (returns values from 0 to 25 inclusive).
f
takes a(n uppercase) string and returns the Optimal Alphabet Stepping
t
is a helper function that takes the string and an alphabet stepping and returns the number of hops needed to finish the string (or float('inf')
if impossible).
1
216 bytes
– Jo King
1 hour ago
Usewhile a!=A(c)and S<len(s)*26:
and you may removeif a==i:return float('inf')
, sincelen(s)*26
is upper bound of any answer.
– tsh
22 mins ago
add a comment |
up vote
1
down vote
Python 2, 222 bytes
A=map(chr,range(65,91))
def t(s,l,S=0):
a=i=A.index(s[0])
for c in s[1:]:
while a!=A.index(c):
S+=1;a+=l;a%=26
if a==i:return float('inf')
i=a
return S
def f(s):T=[t(s,l)for l in range(26)];return T.index(min(T))
Try it online!
-8 bytes from Jo King
This would be shorter in a language with a prime number of letters (wouldn't need the Actually, this submission would still need that for handling strings like "aaa".float('inf')
handling of infinite loops).
This submission is 0-indexed (returns values from 0 to 25 inclusive).
f
takes a(n uppercase) string and returns the Optimal Alphabet Stepping
t
is a helper function that takes the string and an alphabet stepping and returns the number of hops needed to finish the string (or float('inf')
if impossible).
1
216 bytes
– Jo King
1 hour ago
Usewhile a!=A(c)and S<len(s)*26:
and you may removeif a==i:return float('inf')
, sincelen(s)*26
is upper bound of any answer.
– tsh
22 mins ago
add a comment |
up vote
1
down vote
up vote
1
down vote
Python 2, 222 bytes
A=map(chr,range(65,91))
def t(s,l,S=0):
a=i=A.index(s[0])
for c in s[1:]:
while a!=A.index(c):
S+=1;a+=l;a%=26
if a==i:return float('inf')
i=a
return S
def f(s):T=[t(s,l)for l in range(26)];return T.index(min(T))
Try it online!
-8 bytes from Jo King
This would be shorter in a language with a prime number of letters (wouldn't need the Actually, this submission would still need that for handling strings like "aaa".float('inf')
handling of infinite loops).
This submission is 0-indexed (returns values from 0 to 25 inclusive).
f
takes a(n uppercase) string and returns the Optimal Alphabet Stepping
t
is a helper function that takes the string and an alphabet stepping and returns the number of hops needed to finish the string (or float('inf')
if impossible).
Python 2, 222 bytes
A=map(chr,range(65,91))
def t(s,l,S=0):
a=i=A.index(s[0])
for c in s[1:]:
while a!=A.index(c):
S+=1;a+=l;a%=26
if a==i:return float('inf')
i=a
return S
def f(s):T=[t(s,l)for l in range(26)];return T.index(min(T))
Try it online!
-8 bytes from Jo King
This would be shorter in a language with a prime number of letters (wouldn't need the Actually, this submission would still need that for handling strings like "aaa".float('inf')
handling of infinite loops).
This submission is 0-indexed (returns values from 0 to 25 inclusive).
f
takes a(n uppercase) string and returns the Optimal Alphabet Stepping
t
is a helper function that takes the string and an alphabet stepping and returns the number of hops needed to finish the string (or float('inf')
if impossible).
edited 58 mins ago
answered 1 hour ago
pizzapants184
2,594716
2,594716
1
216 bytes
– Jo King
1 hour ago
Usewhile a!=A(c)and S<len(s)*26:
and you may removeif a==i:return float('inf')
, sincelen(s)*26
is upper bound of any answer.
– tsh
22 mins ago
add a comment |
1
216 bytes
– Jo King
1 hour ago
Usewhile a!=A(c)and S<len(s)*26:
and you may removeif a==i:return float('inf')
, sincelen(s)*26
is upper bound of any answer.
– tsh
22 mins ago
1
1
216 bytes
– Jo King
1 hour ago
216 bytes
– Jo King
1 hour ago
Use
while a!=A(c)and S<len(s)*26:
and you may remove if a==i:return float('inf')
, since len(s)*26
is upper bound of any answer.– tsh
22 mins ago
Use
while a!=A(c)and S<len(s)*26:
and you may remove if a==i:return float('inf')
, since len(s)*26
is upper bound of any answer.– tsh
22 mins ago
add a comment |
up vote
1
down vote
JavaScript, 153 bytes
w=>[...Array(26)].map((_,i)=>i).map((_,s,a)=>~[...w].map(c=>(t+=a.find(v=>!p|(u(c,36)+~v*s-u(p,36))%26==0),p=c),p=t=0,u=parseInt)+t<m&&(m=t,n=s),m=1/0)|n
Try it online!
144 bytes
– Shaggy
7 mins ago
add a comment |
up vote
1
down vote
JavaScript, 153 bytes
w=>[...Array(26)].map((_,i)=>i).map((_,s,a)=>~[...w].map(c=>(t+=a.find(v=>!p|(u(c,36)+~v*s-u(p,36))%26==0),p=c),p=t=0,u=parseInt)+t<m&&(m=t,n=s),m=1/0)|n
Try it online!
144 bytes
– Shaggy
7 mins ago
add a comment |
up vote
1
down vote
up vote
1
down vote
JavaScript, 153 bytes
w=>[...Array(26)].map((_,i)=>i).map((_,s,a)=>~[...w].map(c=>(t+=a.find(v=>!p|(u(c,36)+~v*s-u(p,36))%26==0),p=c),p=t=0,u=parseInt)+t<m&&(m=t,n=s),m=1/0)|n
Try it online!
JavaScript, 153 bytes
w=>[...Array(26)].map((_,i)=>i).map((_,s,a)=>~[...w].map(c=>(t+=a.find(v=>!p|(u(c,36)+~v*s-u(p,36))%26==0),p=c),p=t=0,u=parseInt)+t<m&&(m=t,n=s),m=1/0)|n
Try it online!
answered 37 mins ago
tsh
8,15511546
8,15511546
144 bytes
– Shaggy
7 mins ago
add a comment |
144 bytes
– Shaggy
7 mins ago
144 bytes
– Shaggy
7 mins ago
144 bytes
– Shaggy
7 mins ago
add a comment |
If this is an answer to a challenge…
…Be sure to follow the challenge specification. However, please refrain from exploiting obvious loopholes. Answers abusing any of the standard loopholes are considered invalid. If you think a specification is unclear or underspecified, comment on the question instead.
…Try to optimize your score. For instance, answers to code-golf challenges should attempt to be as short as possible. You can always include a readable version of the code in addition to the competitive one.
Explanations of your answer make it more interesting to read and are very much encouraged.…Include a short header which indicates the language(s) of your code and its score, as defined by the challenge.
More generally…
…Please make sure to answer the question and provide sufficient detail.
…Avoid asking for help, clarification or responding to other answers (use comments instead).
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f177404%2foptimal-alphabet-stepping%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZSJbeph,i8EaevlGhYolQ7KFkWIfJ 7Qf1,VwDC1h5ojy s9teI,ydwegU5 I1
Do we need to handle empty input?
– pizzapants184
2 hours ago
1
@pizzapants184 No. I've updated the question to specify the input will be non-empty
– Jo King
2 hours ago
1
@pizzapants184 Thanks, updating the question. Please let me know if any more of my test cases are wrong. I think that was left over from a previous iteration of the question
– Jo King
1 hour ago
Can we take input as an array of characters?
– Shaggy
8 mins ago