Chaining APP_INITIALIZER functions in Angular 6
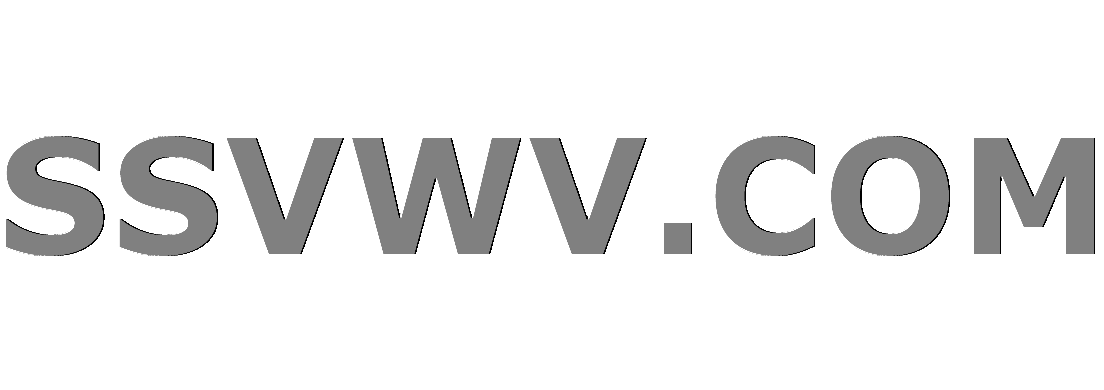
Multi tool use
up vote
0
down vote
favorite
I am trying to ensure that two items of config are loaded before my application starts while using Angular 6 and Typescript. I would like to retrieve an API endpoint from a config file, and then use that API endpoint to retrieve some permissions. The permissions then determine what should be shown on the screen, hence why I really want them to be loaded before the application.
I've read several posts about this including APP_INITALIZER won't delay initialization, Runtime configuration and Nested HTTP Requests
. However they all seem to require there to be one config.service.ts that handles all the config loading that is required. Is it not better practice to have one service to load the API endpoint (and other pieces of config), and another service to access the API?
I have put together a Plunker that demonstrates the two separate services to retrieve the information. The console is logging the various steps. The current output is:
going to load app settings
getting permissions
undefined (This is the api endpoint retrieved from the config file)
Angular is running in the development mode. Call enableProdMode() to enable the production mode.
got appsettings
I hope you can see that the code is trying to load the endpoint and trying to use it to retrieve the permissions simultaneously. The endpoint is actually retrieved after the application is loaded. I would like to have the api endpoint retrieved, THEN the permissions loaded and then the application loads. So the desired output is:
going to load app settings
got appsettings
getting permissions
https://webapi.endpoint/
Angular is running in the development mode. Call enableProdMode() to enable the production mode.
I'm new to Angular, so any help or pointers would be appreciated.
EDIT:
I've found this post but I don't understand the solution. It seems very related if anyone can help me unpick it?
angular rxjs
add a comment |
up vote
0
down vote
favorite
I am trying to ensure that two items of config are loaded before my application starts while using Angular 6 and Typescript. I would like to retrieve an API endpoint from a config file, and then use that API endpoint to retrieve some permissions. The permissions then determine what should be shown on the screen, hence why I really want them to be loaded before the application.
I've read several posts about this including APP_INITALIZER won't delay initialization, Runtime configuration and Nested HTTP Requests
. However they all seem to require there to be one config.service.ts that handles all the config loading that is required. Is it not better practice to have one service to load the API endpoint (and other pieces of config), and another service to access the API?
I have put together a Plunker that demonstrates the two separate services to retrieve the information. The console is logging the various steps. The current output is:
going to load app settings
getting permissions
undefined (This is the api endpoint retrieved from the config file)
Angular is running in the development mode. Call enableProdMode() to enable the production mode.
got appsettings
I hope you can see that the code is trying to load the endpoint and trying to use it to retrieve the permissions simultaneously. The endpoint is actually retrieved after the application is loaded. I would like to have the api endpoint retrieved, THEN the permissions loaded and then the application loads. So the desired output is:
going to load app settings
got appsettings
getting permissions
https://webapi.endpoint/
Angular is running in the development mode. Call enableProdMode() to enable the production mode.
I'm new to Angular, so any help or pointers would be appreciated.
EDIT:
I've found this post but I don't understand the solution. It seems very related if anyone can help me unpick it?
angular rxjs
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am trying to ensure that two items of config are loaded before my application starts while using Angular 6 and Typescript. I would like to retrieve an API endpoint from a config file, and then use that API endpoint to retrieve some permissions. The permissions then determine what should be shown on the screen, hence why I really want them to be loaded before the application.
I've read several posts about this including APP_INITALIZER won't delay initialization, Runtime configuration and Nested HTTP Requests
. However they all seem to require there to be one config.service.ts that handles all the config loading that is required. Is it not better practice to have one service to load the API endpoint (and other pieces of config), and another service to access the API?
I have put together a Plunker that demonstrates the two separate services to retrieve the information. The console is logging the various steps. The current output is:
going to load app settings
getting permissions
undefined (This is the api endpoint retrieved from the config file)
Angular is running in the development mode. Call enableProdMode() to enable the production mode.
got appsettings
I hope you can see that the code is trying to load the endpoint and trying to use it to retrieve the permissions simultaneously. The endpoint is actually retrieved after the application is loaded. I would like to have the api endpoint retrieved, THEN the permissions loaded and then the application loads. So the desired output is:
going to load app settings
got appsettings
getting permissions
https://webapi.endpoint/
Angular is running in the development mode. Call enableProdMode() to enable the production mode.
I'm new to Angular, so any help or pointers would be appreciated.
EDIT:
I've found this post but I don't understand the solution. It seems very related if anyone can help me unpick it?
angular rxjs
I am trying to ensure that two items of config are loaded before my application starts while using Angular 6 and Typescript. I would like to retrieve an API endpoint from a config file, and then use that API endpoint to retrieve some permissions. The permissions then determine what should be shown on the screen, hence why I really want them to be loaded before the application.
I've read several posts about this including APP_INITALIZER won't delay initialization, Runtime configuration and Nested HTTP Requests
. However they all seem to require there to be one config.service.ts that handles all the config loading that is required. Is it not better practice to have one service to load the API endpoint (and other pieces of config), and another service to access the API?
I have put together a Plunker that demonstrates the two separate services to retrieve the information. The console is logging the various steps. The current output is:
going to load app settings
getting permissions
undefined (This is the api endpoint retrieved from the config file)
Angular is running in the development mode. Call enableProdMode() to enable the production mode.
got appsettings
I hope you can see that the code is trying to load the endpoint and trying to use it to retrieve the permissions simultaneously. The endpoint is actually retrieved after the application is loaded. I would like to have the api endpoint retrieved, THEN the permissions loaded and then the application loads. So the desired output is:
going to load app settings
got appsettings
getting permissions
https://webapi.endpoint/
Angular is running in the development mode. Call enableProdMode() to enable the production mode.
I'm new to Angular, so any help or pointers would be appreciated.
EDIT:
I've found this post but I don't understand the solution. It seems very related if anyone can help me unpick it?
angular rxjs
angular rxjs
edited Nov 22 at 8:17
asked Nov 20 at 9:13


Fletch
146
146
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
In the end I took inspiration from hackernoon and rearranged the way I called my promises. I kept my separate services, but called the permission service from the appsettings service.
app-settings.service.ts:
loadAppSettings() {
return (): Promise<any> => {
return new Promise((resolve, reject) => {
console.log('loadAppSettings:: inside promise');
setTimeout(() => {
console.log('loadAppSettings:: inside setTimeout');
this.permissionService.loadPermissions().then(x=> resolve());
}, 5000);
})
}
permissions.service.ts:
loadPermissions() {
return new Promise((resolve, reject) => {
console.log(`PermissionsService:: inside promise`);
setTimeout(() => {
console.log(`PermissionsService:: inside setTimeout`);
resolve();
}, 3000);
});
}
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
In the end I took inspiration from hackernoon and rearranged the way I called my promises. I kept my separate services, but called the permission service from the appsettings service.
app-settings.service.ts:
loadAppSettings() {
return (): Promise<any> => {
return new Promise((resolve, reject) => {
console.log('loadAppSettings:: inside promise');
setTimeout(() => {
console.log('loadAppSettings:: inside setTimeout');
this.permissionService.loadPermissions().then(x=> resolve());
}, 5000);
})
}
permissions.service.ts:
loadPermissions() {
return new Promise((resolve, reject) => {
console.log(`PermissionsService:: inside promise`);
setTimeout(() => {
console.log(`PermissionsService:: inside setTimeout`);
resolve();
}, 3000);
});
}
add a comment |
up vote
0
down vote
accepted
In the end I took inspiration from hackernoon and rearranged the way I called my promises. I kept my separate services, but called the permission service from the appsettings service.
app-settings.service.ts:
loadAppSettings() {
return (): Promise<any> => {
return new Promise((resolve, reject) => {
console.log('loadAppSettings:: inside promise');
setTimeout(() => {
console.log('loadAppSettings:: inside setTimeout');
this.permissionService.loadPermissions().then(x=> resolve());
}, 5000);
})
}
permissions.service.ts:
loadPermissions() {
return new Promise((resolve, reject) => {
console.log(`PermissionsService:: inside promise`);
setTimeout(() => {
console.log(`PermissionsService:: inside setTimeout`);
resolve();
}, 3000);
});
}
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
In the end I took inspiration from hackernoon and rearranged the way I called my promises. I kept my separate services, but called the permission service from the appsettings service.
app-settings.service.ts:
loadAppSettings() {
return (): Promise<any> => {
return new Promise((resolve, reject) => {
console.log('loadAppSettings:: inside promise');
setTimeout(() => {
console.log('loadAppSettings:: inside setTimeout');
this.permissionService.loadPermissions().then(x=> resolve());
}, 5000);
})
}
permissions.service.ts:
loadPermissions() {
return new Promise((resolve, reject) => {
console.log(`PermissionsService:: inside promise`);
setTimeout(() => {
console.log(`PermissionsService:: inside setTimeout`);
resolve();
}, 3000);
});
}
In the end I took inspiration from hackernoon and rearranged the way I called my promises. I kept my separate services, but called the permission service from the appsettings service.
app-settings.service.ts:
loadAppSettings() {
return (): Promise<any> => {
return new Promise((resolve, reject) => {
console.log('loadAppSettings:: inside promise');
setTimeout(() => {
console.log('loadAppSettings:: inside setTimeout');
this.permissionService.loadPermissions().then(x=> resolve());
}, 5000);
})
}
permissions.service.ts:
loadPermissions() {
return new Promise((resolve, reject) => {
console.log(`PermissionsService:: inside promise`);
setTimeout(() => {
console.log(`PermissionsService:: inside setTimeout`);
resolve();
}, 3000);
});
}
answered Nov 22 at 10:02


Fletch
146
146
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53389661%2fchaining-app-initializer-functions-in-angular-6%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7DV5Ar WT YCVGndoQfV,MHResNu6 zB9c,FxUe0PE72k1iE9lFPTE4ThrDFyM TdBuD cRqvg01U4OHVnsH5A9fRPxsG 4ifN ym moA