Get TextView data of fragment from Activity
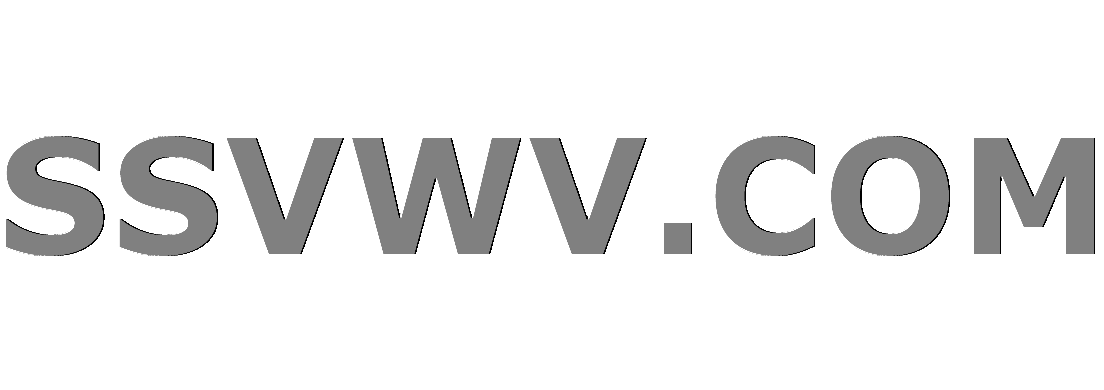
Multi tool use
up vote
0
down vote
favorite
I want pass data from textview fields of my fragment to activity method who insert data to sqlite data base.
This is my fragment with TextView:
public class BandasFragment extends Fragment {
private EditText et_codigo, et_nombre, et_genero, et_descripcion;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View vistaBandas = inflater.inflate(R.layout.fragment_bandas, container, false);
et_codigo = (EditText)vistaBandas.findViewById(R.id.txt_codigo);
et_nombre = (EditText)vistaBandas.findViewById(R.id.txt_nombre);
et_genero = (EditText)vistaBandas.findViewById(R.id.txt_genero);
et_descripcion = (EditText)vistaBandas.findViewById(R.id.txt_descripcion);
return vistaBandas;
}
}
This is method of my activity who insert data to SQlite database. So i need comunicate from this method to TextView fields of fragment or trasport data from fragment to this method.
public void Registrar(View view){
AdminSQLiteOpenHelper admin = new AdminSQLiteOpenHelper(this, "administracion", null, 1);
SQLiteDatabase BaseDeDatos = admin.getWritableDatabase();
String codigo = et_codigo.getText().toString();
String nombre = et_nombre.getText().toString();
String genero = et_genero.getText().toString();
String descripcion = et_descripcion.getText().toString();
if(!codigo.isEmpty()){
ContentValues registro = new ContentValues();
registro.put("codigo", codigo);
registro.put("nombre", nombre);
registro.put("genero", genero);
registro.put("descripcion", descripcion);
BaseDeDatos.insert("banda", null, registro);
BaseDeDatos.close();
et_codigo.setText("");
et_nombre.setText("");
et_genero.setText("");
et_descripcion.setText("");
Toast.makeText(this,"Registro exitoso", Toast.LENGTH_SHORT).show();
} else{
Toast.makeText(this,"Debes llenar todos los campos",Toast.LENGTH_SHORT).show();
}
}

add a comment |
up vote
0
down vote
favorite
I want pass data from textview fields of my fragment to activity method who insert data to sqlite data base.
This is my fragment with TextView:
public class BandasFragment extends Fragment {
private EditText et_codigo, et_nombre, et_genero, et_descripcion;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View vistaBandas = inflater.inflate(R.layout.fragment_bandas, container, false);
et_codigo = (EditText)vistaBandas.findViewById(R.id.txt_codigo);
et_nombre = (EditText)vistaBandas.findViewById(R.id.txt_nombre);
et_genero = (EditText)vistaBandas.findViewById(R.id.txt_genero);
et_descripcion = (EditText)vistaBandas.findViewById(R.id.txt_descripcion);
return vistaBandas;
}
}
This is method of my activity who insert data to SQlite database. So i need comunicate from this method to TextView fields of fragment or trasport data from fragment to this method.
public void Registrar(View view){
AdminSQLiteOpenHelper admin = new AdminSQLiteOpenHelper(this, "administracion", null, 1);
SQLiteDatabase BaseDeDatos = admin.getWritableDatabase();
String codigo = et_codigo.getText().toString();
String nombre = et_nombre.getText().toString();
String genero = et_genero.getText().toString();
String descripcion = et_descripcion.getText().toString();
if(!codigo.isEmpty()){
ContentValues registro = new ContentValues();
registro.put("codigo", codigo);
registro.put("nombre", nombre);
registro.put("genero", genero);
registro.put("descripcion", descripcion);
BaseDeDatos.insert("banda", null, registro);
BaseDeDatos.close();
et_codigo.setText("");
et_nombre.setText("");
et_genero.setText("");
et_descripcion.setText("");
Toast.makeText(this,"Registro exitoso", Toast.LENGTH_SHORT).show();
} else{
Toast.makeText(this,"Debes llenar todos los campos",Toast.LENGTH_SHORT).show();
}
}

2
Possible duplicate of Passing data between a fragment and its container activity
– Vadim Eksler
Nov 19 at 13:10
You can useBroadcastReceiver
, orInterface
. and have a look at This question also.
– Rumit Patel
Nov 19 at 13:12
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I want pass data from textview fields of my fragment to activity method who insert data to sqlite data base.
This is my fragment with TextView:
public class BandasFragment extends Fragment {
private EditText et_codigo, et_nombre, et_genero, et_descripcion;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View vistaBandas = inflater.inflate(R.layout.fragment_bandas, container, false);
et_codigo = (EditText)vistaBandas.findViewById(R.id.txt_codigo);
et_nombre = (EditText)vistaBandas.findViewById(R.id.txt_nombre);
et_genero = (EditText)vistaBandas.findViewById(R.id.txt_genero);
et_descripcion = (EditText)vistaBandas.findViewById(R.id.txt_descripcion);
return vistaBandas;
}
}
This is method of my activity who insert data to SQlite database. So i need comunicate from this method to TextView fields of fragment or trasport data from fragment to this method.
public void Registrar(View view){
AdminSQLiteOpenHelper admin = new AdminSQLiteOpenHelper(this, "administracion", null, 1);
SQLiteDatabase BaseDeDatos = admin.getWritableDatabase();
String codigo = et_codigo.getText().toString();
String nombre = et_nombre.getText().toString();
String genero = et_genero.getText().toString();
String descripcion = et_descripcion.getText().toString();
if(!codigo.isEmpty()){
ContentValues registro = new ContentValues();
registro.put("codigo", codigo);
registro.put("nombre", nombre);
registro.put("genero", genero);
registro.put("descripcion", descripcion);
BaseDeDatos.insert("banda", null, registro);
BaseDeDatos.close();
et_codigo.setText("");
et_nombre.setText("");
et_genero.setText("");
et_descripcion.setText("");
Toast.makeText(this,"Registro exitoso", Toast.LENGTH_SHORT).show();
} else{
Toast.makeText(this,"Debes llenar todos los campos",Toast.LENGTH_SHORT).show();
}
}

I want pass data from textview fields of my fragment to activity method who insert data to sqlite data base.
This is my fragment with TextView:
public class BandasFragment extends Fragment {
private EditText et_codigo, et_nombre, et_genero, et_descripcion;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View vistaBandas = inflater.inflate(R.layout.fragment_bandas, container, false);
et_codigo = (EditText)vistaBandas.findViewById(R.id.txt_codigo);
et_nombre = (EditText)vistaBandas.findViewById(R.id.txt_nombre);
et_genero = (EditText)vistaBandas.findViewById(R.id.txt_genero);
et_descripcion = (EditText)vistaBandas.findViewById(R.id.txt_descripcion);
return vistaBandas;
}
}
This is method of my activity who insert data to SQlite database. So i need comunicate from this method to TextView fields of fragment or trasport data from fragment to this method.
public void Registrar(View view){
AdminSQLiteOpenHelper admin = new AdminSQLiteOpenHelper(this, "administracion", null, 1);
SQLiteDatabase BaseDeDatos = admin.getWritableDatabase();
String codigo = et_codigo.getText().toString();
String nombre = et_nombre.getText().toString();
String genero = et_genero.getText().toString();
String descripcion = et_descripcion.getText().toString();
if(!codigo.isEmpty()){
ContentValues registro = new ContentValues();
registro.put("codigo", codigo);
registro.put("nombre", nombre);
registro.put("genero", genero);
registro.put("descripcion", descripcion);
BaseDeDatos.insert("banda", null, registro);
BaseDeDatos.close();
et_codigo.setText("");
et_nombre.setText("");
et_genero.setText("");
et_descripcion.setText("");
Toast.makeText(this,"Registro exitoso", Toast.LENGTH_SHORT).show();
} else{
Toast.makeText(this,"Debes llenar todos los campos",Toast.LENGTH_SHORT).show();
}
}


edited Nov 20 at 9:10


Lekr0
1689
1689
asked Nov 19 at 13:06
Oren Diaz
1014
1014
2
Possible duplicate of Passing data between a fragment and its container activity
– Vadim Eksler
Nov 19 at 13:10
You can useBroadcastReceiver
, orInterface
. and have a look at This question also.
– Rumit Patel
Nov 19 at 13:12
add a comment |
2
Possible duplicate of Passing data between a fragment and its container activity
– Vadim Eksler
Nov 19 at 13:10
You can useBroadcastReceiver
, orInterface
. and have a look at This question also.
– Rumit Patel
Nov 19 at 13:12
2
2
Possible duplicate of Passing data between a fragment and its container activity
– Vadim Eksler
Nov 19 at 13:10
Possible duplicate of Passing data between a fragment and its container activity
– Vadim Eksler
Nov 19 at 13:10
You can use
BroadcastReceiver
, or Interface
. and have a look at This question also.– Rumit Patel
Nov 19 at 13:12
You can use
BroadcastReceiver
, or Interface
. and have a look at This question also.– Rumit Patel
Nov 19 at 13:12
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
We can access data from fragment textview by creating interface which is mediator bw activity and fragment
First you need to create an interface with method.(DataFromFragment.java)
public interface TestListener {
public String listener(String result;
}
2.in this step you need to override onAttach Method in Fragment in that method we will get parent activity context. so we can direct get interface method.
public void onAttach(Context context) {
super.onAttach(context);
try {
mListener = (TestListener) context;
} catch (ClassCastException e) {
throw new ClassCastException(context.toString() + e.getMessage());
}
}
3.in this step you need implement interface in activity.
public void listener(String result) {
Toast.makeText(this, result, Toast.LENGTH_SHORT).show();
}
- in this step you call interface method from fragment method.
public void returnData() {
mListener.listener("Ashok");
}
finally call returndata() method from activity to get result from fragment
FragmentManager fm = getFragmentManager();
BlankFragment f2 = (BlankFragment) fm.findFragmentById(R.id.fragment);
f2.returnData();
DataFromFragment' is abstract; cannot be instantiated - in step 3
– Oren Diaz
Nov 19 at 13:51
Please check this edit code working fine. keep vote
– Ashok Reddy M
Nov 20 at 7:31
add a comment |
up vote
0
down vote
If your fragment is under the same activity where you want to send data then just make a new method or update your Registrar method in your activity and your fragment is part of this activity already so just access this method in fragment and pass your value via method. your BandasFragment will be like this:
public class BandasFragment extends Fragment {
private EditText et_codigo, et_nombre, et_genero, et_descripcion;
RegistrarActivity registrarActivity;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View vistaBandas = inflater.inflate(R.layout.fragment_bandas, container, false);
et_codigo = (EditText)vistaBandas.findViewById(R.id.txt_codigo);
et_nombre = (EditText)vistaBandas.findViewById(R.id.txt_nombre);
et_genero = (EditText)vistaBandas.findViewById(R.id.txt_genero);
et_descripcion = (EditText)vistaBandas.findViewById(R.id.txt_descripcion);
registrarActivity = (RegistrarActivity) getActivity();
registrarActivity.getData(et_codigo.getText().toString(),
et_nombre.getText().toString(),
et_genero.getText().toString(),
et_descripcion.getText().toString());
return vistaBandas;
}
and your class will look like this:
public class RegistrarActivity extends AppCompatActivity {
String codigo,nombre,genero,descripcion;
public void Registrar(View view){
AdminSQLiteOpenHelper admin = new AdminSQLiteOpenHelper(this, "administracion", null, 1);
SQLiteDatabase BaseDeDatos = admin.getWritableDatabase();
if(!codigo.isEmpty()){
ContentValues registro = new ContentValues();
registro.put("codigo", codigo);
registro.put("nombre", nombre);
registro.put("genero", genero);
registro.put("descripcion", descripcion);
BaseDeDatos.insert("banda", null, registro);
BaseDeDatos.close();
et_codigo.setText("");
et_nombre.setText("");
et_genero.setText("");
et_descripcion.setText("");
Toast.makeText(this,"Registro exitoso", Toast.LENGTH_SHORT).show();
} else{
Toast.makeText(this,"Debes llenar todos los campos",Toast.LENGTH_SHORT).show();
}
}
public void getData(String codigo,String nombre,String genero,String descripcion){
this.codigo = codigo;
this.nombre = nombre;
this.genero = genero;
this.descripcion = descripcion;
}
}
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
We can access data from fragment textview by creating interface which is mediator bw activity and fragment
First you need to create an interface with method.(DataFromFragment.java)
public interface TestListener {
public String listener(String result;
}
2.in this step you need to override onAttach Method in Fragment in that method we will get parent activity context. so we can direct get interface method.
public void onAttach(Context context) {
super.onAttach(context);
try {
mListener = (TestListener) context;
} catch (ClassCastException e) {
throw new ClassCastException(context.toString() + e.getMessage());
}
}
3.in this step you need implement interface in activity.
public void listener(String result) {
Toast.makeText(this, result, Toast.LENGTH_SHORT).show();
}
- in this step you call interface method from fragment method.
public void returnData() {
mListener.listener("Ashok");
}
finally call returndata() method from activity to get result from fragment
FragmentManager fm = getFragmentManager();
BlankFragment f2 = (BlankFragment) fm.findFragmentById(R.id.fragment);
f2.returnData();
DataFromFragment' is abstract; cannot be instantiated - in step 3
– Oren Diaz
Nov 19 at 13:51
Please check this edit code working fine. keep vote
– Ashok Reddy M
Nov 20 at 7:31
add a comment |
up vote
1
down vote
We can access data from fragment textview by creating interface which is mediator bw activity and fragment
First you need to create an interface with method.(DataFromFragment.java)
public interface TestListener {
public String listener(String result;
}
2.in this step you need to override onAttach Method in Fragment in that method we will get parent activity context. so we can direct get interface method.
public void onAttach(Context context) {
super.onAttach(context);
try {
mListener = (TestListener) context;
} catch (ClassCastException e) {
throw new ClassCastException(context.toString() + e.getMessage());
}
}
3.in this step you need implement interface in activity.
public void listener(String result) {
Toast.makeText(this, result, Toast.LENGTH_SHORT).show();
}
- in this step you call interface method from fragment method.
public void returnData() {
mListener.listener("Ashok");
}
finally call returndata() method from activity to get result from fragment
FragmentManager fm = getFragmentManager();
BlankFragment f2 = (BlankFragment) fm.findFragmentById(R.id.fragment);
f2.returnData();
DataFromFragment' is abstract; cannot be instantiated - in step 3
– Oren Diaz
Nov 19 at 13:51
Please check this edit code working fine. keep vote
– Ashok Reddy M
Nov 20 at 7:31
add a comment |
up vote
1
down vote
up vote
1
down vote
We can access data from fragment textview by creating interface which is mediator bw activity and fragment
First you need to create an interface with method.(DataFromFragment.java)
public interface TestListener {
public String listener(String result;
}
2.in this step you need to override onAttach Method in Fragment in that method we will get parent activity context. so we can direct get interface method.
public void onAttach(Context context) {
super.onAttach(context);
try {
mListener = (TestListener) context;
} catch (ClassCastException e) {
throw new ClassCastException(context.toString() + e.getMessage());
}
}
3.in this step you need implement interface in activity.
public void listener(String result) {
Toast.makeText(this, result, Toast.LENGTH_SHORT).show();
}
- in this step you call interface method from fragment method.
public void returnData() {
mListener.listener("Ashok");
}
finally call returndata() method from activity to get result from fragment
FragmentManager fm = getFragmentManager();
BlankFragment f2 = (BlankFragment) fm.findFragmentById(R.id.fragment);
f2.returnData();
We can access data from fragment textview by creating interface which is mediator bw activity and fragment
First you need to create an interface with method.(DataFromFragment.java)
public interface TestListener {
public String listener(String result;
}
2.in this step you need to override onAttach Method in Fragment in that method we will get parent activity context. so we can direct get interface method.
public void onAttach(Context context) {
super.onAttach(context);
try {
mListener = (TestListener) context;
} catch (ClassCastException e) {
throw new ClassCastException(context.toString() + e.getMessage());
}
}
3.in this step you need implement interface in activity.
public void listener(String result) {
Toast.makeText(this, result, Toast.LENGTH_SHORT).show();
}
- in this step you call interface method from fragment method.
public void returnData() {
mListener.listener("Ashok");
}
finally call returndata() method from activity to get result from fragment
FragmentManager fm = getFragmentManager();
BlankFragment f2 = (BlankFragment) fm.findFragmentById(R.id.fragment);
f2.returnData();
edited Nov 20 at 7:30
answered Nov 19 at 13:33
Ashok Reddy M
10914
10914
DataFromFragment' is abstract; cannot be instantiated - in step 3
– Oren Diaz
Nov 19 at 13:51
Please check this edit code working fine. keep vote
– Ashok Reddy M
Nov 20 at 7:31
add a comment |
DataFromFragment' is abstract; cannot be instantiated - in step 3
– Oren Diaz
Nov 19 at 13:51
Please check this edit code working fine. keep vote
– Ashok Reddy M
Nov 20 at 7:31
DataFromFragment' is abstract; cannot be instantiated - in step 3
– Oren Diaz
Nov 19 at 13:51
DataFromFragment' is abstract; cannot be instantiated - in step 3
– Oren Diaz
Nov 19 at 13:51
Please check this edit code working fine. keep vote
– Ashok Reddy M
Nov 20 at 7:31
Please check this edit code working fine. keep vote
– Ashok Reddy M
Nov 20 at 7:31
add a comment |
up vote
0
down vote
If your fragment is under the same activity where you want to send data then just make a new method or update your Registrar method in your activity and your fragment is part of this activity already so just access this method in fragment and pass your value via method. your BandasFragment will be like this:
public class BandasFragment extends Fragment {
private EditText et_codigo, et_nombre, et_genero, et_descripcion;
RegistrarActivity registrarActivity;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View vistaBandas = inflater.inflate(R.layout.fragment_bandas, container, false);
et_codigo = (EditText)vistaBandas.findViewById(R.id.txt_codigo);
et_nombre = (EditText)vistaBandas.findViewById(R.id.txt_nombre);
et_genero = (EditText)vistaBandas.findViewById(R.id.txt_genero);
et_descripcion = (EditText)vistaBandas.findViewById(R.id.txt_descripcion);
registrarActivity = (RegistrarActivity) getActivity();
registrarActivity.getData(et_codigo.getText().toString(),
et_nombre.getText().toString(),
et_genero.getText().toString(),
et_descripcion.getText().toString());
return vistaBandas;
}
and your class will look like this:
public class RegistrarActivity extends AppCompatActivity {
String codigo,nombre,genero,descripcion;
public void Registrar(View view){
AdminSQLiteOpenHelper admin = new AdminSQLiteOpenHelper(this, "administracion", null, 1);
SQLiteDatabase BaseDeDatos = admin.getWritableDatabase();
if(!codigo.isEmpty()){
ContentValues registro = new ContentValues();
registro.put("codigo", codigo);
registro.put("nombre", nombre);
registro.put("genero", genero);
registro.put("descripcion", descripcion);
BaseDeDatos.insert("banda", null, registro);
BaseDeDatos.close();
et_codigo.setText("");
et_nombre.setText("");
et_genero.setText("");
et_descripcion.setText("");
Toast.makeText(this,"Registro exitoso", Toast.LENGTH_SHORT).show();
} else{
Toast.makeText(this,"Debes llenar todos los campos",Toast.LENGTH_SHORT).show();
}
}
public void getData(String codigo,String nombre,String genero,String descripcion){
this.codigo = codigo;
this.nombre = nombre;
this.genero = genero;
this.descripcion = descripcion;
}
}
add a comment |
up vote
0
down vote
If your fragment is under the same activity where you want to send data then just make a new method or update your Registrar method in your activity and your fragment is part of this activity already so just access this method in fragment and pass your value via method. your BandasFragment will be like this:
public class BandasFragment extends Fragment {
private EditText et_codigo, et_nombre, et_genero, et_descripcion;
RegistrarActivity registrarActivity;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View vistaBandas = inflater.inflate(R.layout.fragment_bandas, container, false);
et_codigo = (EditText)vistaBandas.findViewById(R.id.txt_codigo);
et_nombre = (EditText)vistaBandas.findViewById(R.id.txt_nombre);
et_genero = (EditText)vistaBandas.findViewById(R.id.txt_genero);
et_descripcion = (EditText)vistaBandas.findViewById(R.id.txt_descripcion);
registrarActivity = (RegistrarActivity) getActivity();
registrarActivity.getData(et_codigo.getText().toString(),
et_nombre.getText().toString(),
et_genero.getText().toString(),
et_descripcion.getText().toString());
return vistaBandas;
}
and your class will look like this:
public class RegistrarActivity extends AppCompatActivity {
String codigo,nombre,genero,descripcion;
public void Registrar(View view){
AdminSQLiteOpenHelper admin = new AdminSQLiteOpenHelper(this, "administracion", null, 1);
SQLiteDatabase BaseDeDatos = admin.getWritableDatabase();
if(!codigo.isEmpty()){
ContentValues registro = new ContentValues();
registro.put("codigo", codigo);
registro.put("nombre", nombre);
registro.put("genero", genero);
registro.put("descripcion", descripcion);
BaseDeDatos.insert("banda", null, registro);
BaseDeDatos.close();
et_codigo.setText("");
et_nombre.setText("");
et_genero.setText("");
et_descripcion.setText("");
Toast.makeText(this,"Registro exitoso", Toast.LENGTH_SHORT).show();
} else{
Toast.makeText(this,"Debes llenar todos los campos",Toast.LENGTH_SHORT).show();
}
}
public void getData(String codigo,String nombre,String genero,String descripcion){
this.codigo = codigo;
this.nombre = nombre;
this.genero = genero;
this.descripcion = descripcion;
}
}
add a comment |
up vote
0
down vote
up vote
0
down vote
If your fragment is under the same activity where you want to send data then just make a new method or update your Registrar method in your activity and your fragment is part of this activity already so just access this method in fragment and pass your value via method. your BandasFragment will be like this:
public class BandasFragment extends Fragment {
private EditText et_codigo, et_nombre, et_genero, et_descripcion;
RegistrarActivity registrarActivity;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View vistaBandas = inflater.inflate(R.layout.fragment_bandas, container, false);
et_codigo = (EditText)vistaBandas.findViewById(R.id.txt_codigo);
et_nombre = (EditText)vistaBandas.findViewById(R.id.txt_nombre);
et_genero = (EditText)vistaBandas.findViewById(R.id.txt_genero);
et_descripcion = (EditText)vistaBandas.findViewById(R.id.txt_descripcion);
registrarActivity = (RegistrarActivity) getActivity();
registrarActivity.getData(et_codigo.getText().toString(),
et_nombre.getText().toString(),
et_genero.getText().toString(),
et_descripcion.getText().toString());
return vistaBandas;
}
and your class will look like this:
public class RegistrarActivity extends AppCompatActivity {
String codigo,nombre,genero,descripcion;
public void Registrar(View view){
AdminSQLiteOpenHelper admin = new AdminSQLiteOpenHelper(this, "administracion", null, 1);
SQLiteDatabase BaseDeDatos = admin.getWritableDatabase();
if(!codigo.isEmpty()){
ContentValues registro = new ContentValues();
registro.put("codigo", codigo);
registro.put("nombre", nombre);
registro.put("genero", genero);
registro.put("descripcion", descripcion);
BaseDeDatos.insert("banda", null, registro);
BaseDeDatos.close();
et_codigo.setText("");
et_nombre.setText("");
et_genero.setText("");
et_descripcion.setText("");
Toast.makeText(this,"Registro exitoso", Toast.LENGTH_SHORT).show();
} else{
Toast.makeText(this,"Debes llenar todos los campos",Toast.LENGTH_SHORT).show();
}
}
public void getData(String codigo,String nombre,String genero,String descripcion){
this.codigo = codigo;
this.nombre = nombre;
this.genero = genero;
this.descripcion = descripcion;
}
}
If your fragment is under the same activity where you want to send data then just make a new method or update your Registrar method in your activity and your fragment is part of this activity already so just access this method in fragment and pass your value via method. your BandasFragment will be like this:
public class BandasFragment extends Fragment {
private EditText et_codigo, et_nombre, et_genero, et_descripcion;
RegistrarActivity registrarActivity;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View vistaBandas = inflater.inflate(R.layout.fragment_bandas, container, false);
et_codigo = (EditText)vistaBandas.findViewById(R.id.txt_codigo);
et_nombre = (EditText)vistaBandas.findViewById(R.id.txt_nombre);
et_genero = (EditText)vistaBandas.findViewById(R.id.txt_genero);
et_descripcion = (EditText)vistaBandas.findViewById(R.id.txt_descripcion);
registrarActivity = (RegistrarActivity) getActivity();
registrarActivity.getData(et_codigo.getText().toString(),
et_nombre.getText().toString(),
et_genero.getText().toString(),
et_descripcion.getText().toString());
return vistaBandas;
}
and your class will look like this:
public class RegistrarActivity extends AppCompatActivity {
String codigo,nombre,genero,descripcion;
public void Registrar(View view){
AdminSQLiteOpenHelper admin = new AdminSQLiteOpenHelper(this, "administracion", null, 1);
SQLiteDatabase BaseDeDatos = admin.getWritableDatabase();
if(!codigo.isEmpty()){
ContentValues registro = new ContentValues();
registro.put("codigo", codigo);
registro.put("nombre", nombre);
registro.put("genero", genero);
registro.put("descripcion", descripcion);
BaseDeDatos.insert("banda", null, registro);
BaseDeDatos.close();
et_codigo.setText("");
et_nombre.setText("");
et_genero.setText("");
et_descripcion.setText("");
Toast.makeText(this,"Registro exitoso", Toast.LENGTH_SHORT).show();
} else{
Toast.makeText(this,"Debes llenar todos los campos",Toast.LENGTH_SHORT).show();
}
}
public void getData(String codigo,String nombre,String genero,String descripcion){
this.codigo = codigo;
this.nombre = nombre;
this.genero = genero;
this.descripcion = descripcion;
}
}
answered Nov 19 at 13:27
Taha wakeel
896
896
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53375324%2fget-textview-data-of-fragment-from-activity%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6jyVo0jXP2sPcdTgCY2xN8HQeWl,gRDEmUox 2uq ytCHIZdykNu7sQa,5pHY96i0b
2
Possible duplicate of Passing data between a fragment and its container activity
– Vadim Eksler
Nov 19 at 13:10
You can use
BroadcastReceiver
, orInterface
. and have a look at This question also.– Rumit Patel
Nov 19 at 13:12