Styling not applied to vue web component during development
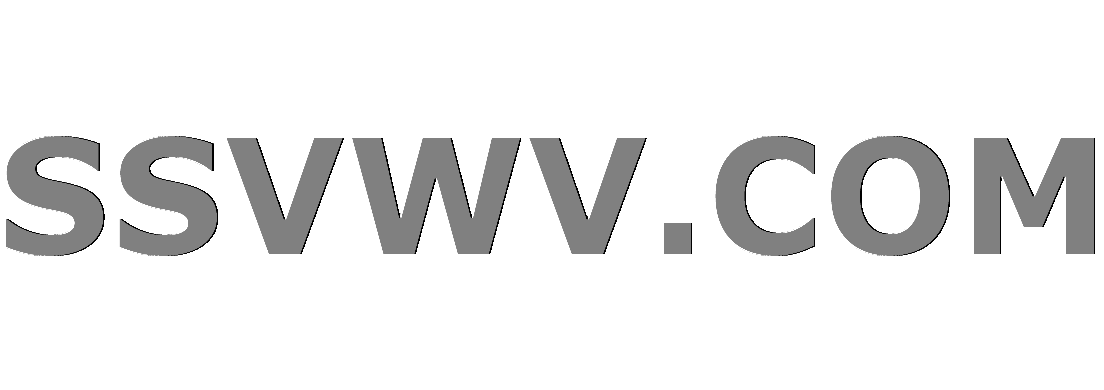
Multi tool use
While developing a Vue web component, the style
is not applied to the web component, but added to the head
of the document. This means that the style is ignored in the shadow DOM. Here is how I wrap the web component in main.js:
import Vue from 'vue';
import wrap from '@vue/web-component-wrapper';
import MyWebComponent from './components/MyWebComponent';
const WrappedElement = wrap(Vue, MyWebComponent);
window.customElements.define('my-web-component', WrappedElement);
Again, any CSS rules inside the style tags do not take effect.
When I build for production, the styles are added to the web component. I use the following command to do the wrapping:
vue-cli-service build --target wc --name my-web-component ./src/components/MyWebComponent.vue
Is there a way to achieve the same thing with vue-cli-service serve
?
edit: example repo here: https://github.com/snirp/vue-web-component
edit2: I have the feeling my problem is closely related to this issue. I cannot make much sense of the workarounds, and I would value a more basic solution.
css vue.js vuejs2 web-component
add a comment |
While developing a Vue web component, the style
is not applied to the web component, but added to the head
of the document. This means that the style is ignored in the shadow DOM. Here is how I wrap the web component in main.js:
import Vue from 'vue';
import wrap from '@vue/web-component-wrapper';
import MyWebComponent from './components/MyWebComponent';
const WrappedElement = wrap(Vue, MyWebComponent);
window.customElements.define('my-web-component', WrappedElement);
Again, any CSS rules inside the style tags do not take effect.
When I build for production, the styles are added to the web component. I use the following command to do the wrapping:
vue-cli-service build --target wc --name my-web-component ./src/components/MyWebComponent.vue
Is there a way to achieve the same thing with vue-cli-service serve
?
edit: example repo here: https://github.com/snirp/vue-web-component
edit2: I have the feeling my problem is closely related to this issue. I cannot make much sense of the workarounds, and I would value a more basic solution.
css vue.js vuejs2 web-component
Do you want to inline style??
– Tushar Kumawat
Nov 30 '18 at 15:47
No inline styles, I just want to add the <style> section of the Vue component added to the Shadow DOM of the web component. Feel free to check the Github repo.
– Roy Prins
Nov 30 '18 at 16:05
add a comment |
While developing a Vue web component, the style
is not applied to the web component, but added to the head
of the document. This means that the style is ignored in the shadow DOM. Here is how I wrap the web component in main.js:
import Vue from 'vue';
import wrap from '@vue/web-component-wrapper';
import MyWebComponent from './components/MyWebComponent';
const WrappedElement = wrap(Vue, MyWebComponent);
window.customElements.define('my-web-component', WrappedElement);
Again, any CSS rules inside the style tags do not take effect.
When I build for production, the styles are added to the web component. I use the following command to do the wrapping:
vue-cli-service build --target wc --name my-web-component ./src/components/MyWebComponent.vue
Is there a way to achieve the same thing with vue-cli-service serve
?
edit: example repo here: https://github.com/snirp/vue-web-component
edit2: I have the feeling my problem is closely related to this issue. I cannot make much sense of the workarounds, and I would value a more basic solution.
css vue.js vuejs2 web-component
While developing a Vue web component, the style
is not applied to the web component, but added to the head
of the document. This means that the style is ignored in the shadow DOM. Here is how I wrap the web component in main.js:
import Vue from 'vue';
import wrap from '@vue/web-component-wrapper';
import MyWebComponent from './components/MyWebComponent';
const WrappedElement = wrap(Vue, MyWebComponent);
window.customElements.define('my-web-component', WrappedElement);
Again, any CSS rules inside the style tags do not take effect.
When I build for production, the styles are added to the web component. I use the following command to do the wrapping:
vue-cli-service build --target wc --name my-web-component ./src/components/MyWebComponent.vue
Is there a way to achieve the same thing with vue-cli-service serve
?
edit: example repo here: https://github.com/snirp/vue-web-component
edit2: I have the feeling my problem is closely related to this issue. I cannot make much sense of the workarounds, and I would value a more basic solution.
css vue.js vuejs2 web-component
css vue.js vuejs2 web-component
edited Dec 2 '18 at 6:50


tony19
23.5k44175
23.5k44175
asked Nov 22 '18 at 13:09
Roy PrinsRoy Prins
901927
901927
Do you want to inline style??
– Tushar Kumawat
Nov 30 '18 at 15:47
No inline styles, I just want to add the <style> section of the Vue component added to the Shadow DOM of the web component. Feel free to check the Github repo.
– Roy Prins
Nov 30 '18 at 16:05
add a comment |
Do you want to inline style??
– Tushar Kumawat
Nov 30 '18 at 15:47
No inline styles, I just want to add the <style> section of the Vue component added to the Shadow DOM of the web component. Feel free to check the Github repo.
– Roy Prins
Nov 30 '18 at 16:05
Do you want to inline style??
– Tushar Kumawat
Nov 30 '18 at 15:47
Do you want to inline style??
– Tushar Kumawat
Nov 30 '18 at 15:47
No inline styles, I just want to add the <style> section of the Vue component added to the Shadow DOM of the web component. Feel free to check the Github repo.
– Roy Prins
Nov 30 '18 at 16:05
No inline styles, I just want to add the <style> section of the Vue component added to the Shadow DOM of the web component. Feel free to check the Github repo.
– Roy Prins
Nov 30 '18 at 16:05
add a comment |
1 Answer
1
active
oldest
votes
Based on the GitHub issue you linked, the solution is to set the shadowMode
option in vue-loader
and vue-style-loader
. shadowMode
is false
by default in a Vue CLI project, but we can tweak that in vue.config.js
.
First, we'd inspect the Webpack config to determine which loaders to change:
# run at project root
vue inspect
The command output reveals several loader configs with shadowMode: false
:
/* config.module.rule('css') */
{
test: /.css$/,
oneOf: [
/* config.module.rule('css').oneOf('vue-modules') */
{
resourceQuery: /module/,
use: [
/* config.module.rule('css').oneOf('vue-modules').use('vue-style-loader') */
{
loader: 'vue-style-loader',
options: {
sourceMap: false,
shadowMode: false // <---
}
},
/* ... */
]
},
/* ... */
full list of Webpack loader configs with shadowMode: false
:
config.module.rule('vue').use('vue-loader')
config.module.rule('css').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('css').oneOf('vue').use('vue-style-loader')
config.module.rule('css').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('css').oneOf('normal').use('vue-style-loader')
config.module.rule('postcss').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('postcss').oneOf('vue').use('vue-style-loader')
config.module.rule('postcss').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('postcss').oneOf('normal').use('vue-style-loader')
config.module.rule('scss').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('scss').oneOf('vue').use('vue-style-loader')
config.module.rule('scss').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('scss').oneOf('normal').use('vue-style-loader')
config.module.rule('sass').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('sass').oneOf('vue').use('vue-style-loader')
config.module.rule('sass').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('sass').oneOf('normal').use('vue-style-loader')
config.module.rule('less').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('less').oneOf('vue').use('vue-style-loader')
config.module.rule('less').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('less').oneOf('normal').use('vue-style-loader')
config.module.rule('stylus').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('stylus').oneOf('vue').use('vue-style-loader')
config.module.rule('stylus').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('stylus').oneOf('normal').use('vue-style-loader')
So, we can set shadowMode: true
for those configs in vue.config.js
with this snippet:
function enableShadowCss(config) {
const configs = [
config.module.rule('vue').use('vue-loader'),
config.module.rule('css').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('css').oneOf('vue').use('vue-style-loader'),
config.module.rule('css').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('css').oneOf('normal').use('vue-style-loader'),
config.module.rule('postcss').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('postcss').oneOf('vue').use('vue-style-loader'),
config.module.rule('postcss').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('postcss').oneOf('normal').use('vue-style-loader'),
config.module.rule('scss').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('scss').oneOf('vue').use('vue-style-loader'),
config.module.rule('scss').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('scss').oneOf('normal').use('vue-style-loader'),
config.module.rule('sass').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('sass').oneOf('vue').use('vue-style-loader'),
config.module.rule('sass').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('sass').oneOf('normal').use('vue-style-loader'),
config.module.rule('less').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('less').oneOf('vue').use('vue-style-loader'),
config.module.rule('less').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('less').oneOf('normal').use('vue-style-loader'),
config.module.rule('stylus').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('stylus').oneOf('vue').use('vue-style-loader'),
config.module.rule('stylus').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('stylus').oneOf('normal').use('vue-style-loader'),
];
configs.forEach(c => c.tap(options => {
options.shadowMode = true;
return options;
}));
}
module.exports = {
// https://cli.vuejs.org/guide/webpack.html#chaining-advanced
chainWebpack: config => {
enableShadowCss(config);
}
}
Creating <projectroot>/vue.config.js
with the snippet above enables Shadow CSS in development mode in your project. See https://github.com/snirp/vue-web-component/pull/1.
Thanks a bundle, that works like a charm. I will try to brush upenableShadowConfig()
to recursively scan the config forshadowMode = false
values. That should make it a bit more resilient. Would you agree that there should be preferably be a single (default) config setting for shadowMode?
– Roy Prins
Dec 2 '18 at 10:05
Ps: I plan to do a short blog post on Vue web components. (How) can I credit you for the input?
– Roy Prins
Dec 2 '18 at 10:09
@RoyPrins No problem :) Yes, I agreeshadowMode
should typically be the same value throughout: either allfalse
(the default) or alltrue
. But perhaps there's an interesting use case for having only a subset of components use Shadow CSS.
– tony19
Dec 2 '18 at 10:53
@RoyPrins Sure, credit tony19@gmail.com (email if more info needed). I'm looking forward to the read.
– tony19
Dec 2 '18 at 10:55
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53431754%2fstyling-not-applied-to-vue-web-component-during-development%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Based on the GitHub issue you linked, the solution is to set the shadowMode
option in vue-loader
and vue-style-loader
. shadowMode
is false
by default in a Vue CLI project, but we can tweak that in vue.config.js
.
First, we'd inspect the Webpack config to determine which loaders to change:
# run at project root
vue inspect
The command output reveals several loader configs with shadowMode: false
:
/* config.module.rule('css') */
{
test: /.css$/,
oneOf: [
/* config.module.rule('css').oneOf('vue-modules') */
{
resourceQuery: /module/,
use: [
/* config.module.rule('css').oneOf('vue-modules').use('vue-style-loader') */
{
loader: 'vue-style-loader',
options: {
sourceMap: false,
shadowMode: false // <---
}
},
/* ... */
]
},
/* ... */
full list of Webpack loader configs with shadowMode: false
:
config.module.rule('vue').use('vue-loader')
config.module.rule('css').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('css').oneOf('vue').use('vue-style-loader')
config.module.rule('css').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('css').oneOf('normal').use('vue-style-loader')
config.module.rule('postcss').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('postcss').oneOf('vue').use('vue-style-loader')
config.module.rule('postcss').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('postcss').oneOf('normal').use('vue-style-loader')
config.module.rule('scss').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('scss').oneOf('vue').use('vue-style-loader')
config.module.rule('scss').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('scss').oneOf('normal').use('vue-style-loader')
config.module.rule('sass').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('sass').oneOf('vue').use('vue-style-loader')
config.module.rule('sass').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('sass').oneOf('normal').use('vue-style-loader')
config.module.rule('less').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('less').oneOf('vue').use('vue-style-loader')
config.module.rule('less').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('less').oneOf('normal').use('vue-style-loader')
config.module.rule('stylus').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('stylus').oneOf('vue').use('vue-style-loader')
config.module.rule('stylus').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('stylus').oneOf('normal').use('vue-style-loader')
So, we can set shadowMode: true
for those configs in vue.config.js
with this snippet:
function enableShadowCss(config) {
const configs = [
config.module.rule('vue').use('vue-loader'),
config.module.rule('css').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('css').oneOf('vue').use('vue-style-loader'),
config.module.rule('css').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('css').oneOf('normal').use('vue-style-loader'),
config.module.rule('postcss').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('postcss').oneOf('vue').use('vue-style-loader'),
config.module.rule('postcss').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('postcss').oneOf('normal').use('vue-style-loader'),
config.module.rule('scss').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('scss').oneOf('vue').use('vue-style-loader'),
config.module.rule('scss').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('scss').oneOf('normal').use('vue-style-loader'),
config.module.rule('sass').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('sass').oneOf('vue').use('vue-style-loader'),
config.module.rule('sass').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('sass').oneOf('normal').use('vue-style-loader'),
config.module.rule('less').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('less').oneOf('vue').use('vue-style-loader'),
config.module.rule('less').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('less').oneOf('normal').use('vue-style-loader'),
config.module.rule('stylus').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('stylus').oneOf('vue').use('vue-style-loader'),
config.module.rule('stylus').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('stylus').oneOf('normal').use('vue-style-loader'),
];
configs.forEach(c => c.tap(options => {
options.shadowMode = true;
return options;
}));
}
module.exports = {
// https://cli.vuejs.org/guide/webpack.html#chaining-advanced
chainWebpack: config => {
enableShadowCss(config);
}
}
Creating <projectroot>/vue.config.js
with the snippet above enables Shadow CSS in development mode in your project. See https://github.com/snirp/vue-web-component/pull/1.
Thanks a bundle, that works like a charm. I will try to brush upenableShadowConfig()
to recursively scan the config forshadowMode = false
values. That should make it a bit more resilient. Would you agree that there should be preferably be a single (default) config setting for shadowMode?
– Roy Prins
Dec 2 '18 at 10:05
Ps: I plan to do a short blog post on Vue web components. (How) can I credit you for the input?
– Roy Prins
Dec 2 '18 at 10:09
@RoyPrins No problem :) Yes, I agreeshadowMode
should typically be the same value throughout: either allfalse
(the default) or alltrue
. But perhaps there's an interesting use case for having only a subset of components use Shadow CSS.
– tony19
Dec 2 '18 at 10:53
@RoyPrins Sure, credit tony19@gmail.com (email if more info needed). I'm looking forward to the read.
– tony19
Dec 2 '18 at 10:55
add a comment |
Based on the GitHub issue you linked, the solution is to set the shadowMode
option in vue-loader
and vue-style-loader
. shadowMode
is false
by default in a Vue CLI project, but we can tweak that in vue.config.js
.
First, we'd inspect the Webpack config to determine which loaders to change:
# run at project root
vue inspect
The command output reveals several loader configs with shadowMode: false
:
/* config.module.rule('css') */
{
test: /.css$/,
oneOf: [
/* config.module.rule('css').oneOf('vue-modules') */
{
resourceQuery: /module/,
use: [
/* config.module.rule('css').oneOf('vue-modules').use('vue-style-loader') */
{
loader: 'vue-style-loader',
options: {
sourceMap: false,
shadowMode: false // <---
}
},
/* ... */
]
},
/* ... */
full list of Webpack loader configs with shadowMode: false
:
config.module.rule('vue').use('vue-loader')
config.module.rule('css').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('css').oneOf('vue').use('vue-style-loader')
config.module.rule('css').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('css').oneOf('normal').use('vue-style-loader')
config.module.rule('postcss').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('postcss').oneOf('vue').use('vue-style-loader')
config.module.rule('postcss').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('postcss').oneOf('normal').use('vue-style-loader')
config.module.rule('scss').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('scss').oneOf('vue').use('vue-style-loader')
config.module.rule('scss').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('scss').oneOf('normal').use('vue-style-loader')
config.module.rule('sass').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('sass').oneOf('vue').use('vue-style-loader')
config.module.rule('sass').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('sass').oneOf('normal').use('vue-style-loader')
config.module.rule('less').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('less').oneOf('vue').use('vue-style-loader')
config.module.rule('less').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('less').oneOf('normal').use('vue-style-loader')
config.module.rule('stylus').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('stylus').oneOf('vue').use('vue-style-loader')
config.module.rule('stylus').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('stylus').oneOf('normal').use('vue-style-loader')
So, we can set shadowMode: true
for those configs in vue.config.js
with this snippet:
function enableShadowCss(config) {
const configs = [
config.module.rule('vue').use('vue-loader'),
config.module.rule('css').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('css').oneOf('vue').use('vue-style-loader'),
config.module.rule('css').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('css').oneOf('normal').use('vue-style-loader'),
config.module.rule('postcss').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('postcss').oneOf('vue').use('vue-style-loader'),
config.module.rule('postcss').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('postcss').oneOf('normal').use('vue-style-loader'),
config.module.rule('scss').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('scss').oneOf('vue').use('vue-style-loader'),
config.module.rule('scss').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('scss').oneOf('normal').use('vue-style-loader'),
config.module.rule('sass').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('sass').oneOf('vue').use('vue-style-loader'),
config.module.rule('sass').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('sass').oneOf('normal').use('vue-style-loader'),
config.module.rule('less').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('less').oneOf('vue').use('vue-style-loader'),
config.module.rule('less').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('less').oneOf('normal').use('vue-style-loader'),
config.module.rule('stylus').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('stylus').oneOf('vue').use('vue-style-loader'),
config.module.rule('stylus').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('stylus').oneOf('normal').use('vue-style-loader'),
];
configs.forEach(c => c.tap(options => {
options.shadowMode = true;
return options;
}));
}
module.exports = {
// https://cli.vuejs.org/guide/webpack.html#chaining-advanced
chainWebpack: config => {
enableShadowCss(config);
}
}
Creating <projectroot>/vue.config.js
with the snippet above enables Shadow CSS in development mode in your project. See https://github.com/snirp/vue-web-component/pull/1.
Thanks a bundle, that works like a charm. I will try to brush upenableShadowConfig()
to recursively scan the config forshadowMode = false
values. That should make it a bit more resilient. Would you agree that there should be preferably be a single (default) config setting for shadowMode?
– Roy Prins
Dec 2 '18 at 10:05
Ps: I plan to do a short blog post on Vue web components. (How) can I credit you for the input?
– Roy Prins
Dec 2 '18 at 10:09
@RoyPrins No problem :) Yes, I agreeshadowMode
should typically be the same value throughout: either allfalse
(the default) or alltrue
. But perhaps there's an interesting use case for having only a subset of components use Shadow CSS.
– tony19
Dec 2 '18 at 10:53
@RoyPrins Sure, credit tony19@gmail.com (email if more info needed). I'm looking forward to the read.
– tony19
Dec 2 '18 at 10:55
add a comment |
Based on the GitHub issue you linked, the solution is to set the shadowMode
option in vue-loader
and vue-style-loader
. shadowMode
is false
by default in a Vue CLI project, but we can tweak that in vue.config.js
.
First, we'd inspect the Webpack config to determine which loaders to change:
# run at project root
vue inspect
The command output reveals several loader configs with shadowMode: false
:
/* config.module.rule('css') */
{
test: /.css$/,
oneOf: [
/* config.module.rule('css').oneOf('vue-modules') */
{
resourceQuery: /module/,
use: [
/* config.module.rule('css').oneOf('vue-modules').use('vue-style-loader') */
{
loader: 'vue-style-loader',
options: {
sourceMap: false,
shadowMode: false // <---
}
},
/* ... */
]
},
/* ... */
full list of Webpack loader configs with shadowMode: false
:
config.module.rule('vue').use('vue-loader')
config.module.rule('css').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('css').oneOf('vue').use('vue-style-loader')
config.module.rule('css').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('css').oneOf('normal').use('vue-style-loader')
config.module.rule('postcss').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('postcss').oneOf('vue').use('vue-style-loader')
config.module.rule('postcss').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('postcss').oneOf('normal').use('vue-style-loader')
config.module.rule('scss').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('scss').oneOf('vue').use('vue-style-loader')
config.module.rule('scss').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('scss').oneOf('normal').use('vue-style-loader')
config.module.rule('sass').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('sass').oneOf('vue').use('vue-style-loader')
config.module.rule('sass').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('sass').oneOf('normal').use('vue-style-loader')
config.module.rule('less').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('less').oneOf('vue').use('vue-style-loader')
config.module.rule('less').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('less').oneOf('normal').use('vue-style-loader')
config.module.rule('stylus').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('stylus').oneOf('vue').use('vue-style-loader')
config.module.rule('stylus').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('stylus').oneOf('normal').use('vue-style-loader')
So, we can set shadowMode: true
for those configs in vue.config.js
with this snippet:
function enableShadowCss(config) {
const configs = [
config.module.rule('vue').use('vue-loader'),
config.module.rule('css').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('css').oneOf('vue').use('vue-style-loader'),
config.module.rule('css').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('css').oneOf('normal').use('vue-style-loader'),
config.module.rule('postcss').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('postcss').oneOf('vue').use('vue-style-loader'),
config.module.rule('postcss').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('postcss').oneOf('normal').use('vue-style-loader'),
config.module.rule('scss').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('scss').oneOf('vue').use('vue-style-loader'),
config.module.rule('scss').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('scss').oneOf('normal').use('vue-style-loader'),
config.module.rule('sass').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('sass').oneOf('vue').use('vue-style-loader'),
config.module.rule('sass').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('sass').oneOf('normal').use('vue-style-loader'),
config.module.rule('less').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('less').oneOf('vue').use('vue-style-loader'),
config.module.rule('less').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('less').oneOf('normal').use('vue-style-loader'),
config.module.rule('stylus').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('stylus').oneOf('vue').use('vue-style-loader'),
config.module.rule('stylus').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('stylus').oneOf('normal').use('vue-style-loader'),
];
configs.forEach(c => c.tap(options => {
options.shadowMode = true;
return options;
}));
}
module.exports = {
// https://cli.vuejs.org/guide/webpack.html#chaining-advanced
chainWebpack: config => {
enableShadowCss(config);
}
}
Creating <projectroot>/vue.config.js
with the snippet above enables Shadow CSS in development mode in your project. See https://github.com/snirp/vue-web-component/pull/1.
Based on the GitHub issue you linked, the solution is to set the shadowMode
option in vue-loader
and vue-style-loader
. shadowMode
is false
by default in a Vue CLI project, but we can tweak that in vue.config.js
.
First, we'd inspect the Webpack config to determine which loaders to change:
# run at project root
vue inspect
The command output reveals several loader configs with shadowMode: false
:
/* config.module.rule('css') */
{
test: /.css$/,
oneOf: [
/* config.module.rule('css').oneOf('vue-modules') */
{
resourceQuery: /module/,
use: [
/* config.module.rule('css').oneOf('vue-modules').use('vue-style-loader') */
{
loader: 'vue-style-loader',
options: {
sourceMap: false,
shadowMode: false // <---
}
},
/* ... */
]
},
/* ... */
full list of Webpack loader configs with shadowMode: false
:
config.module.rule('vue').use('vue-loader')
config.module.rule('css').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('css').oneOf('vue').use('vue-style-loader')
config.module.rule('css').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('css').oneOf('normal').use('vue-style-loader')
config.module.rule('postcss').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('postcss').oneOf('vue').use('vue-style-loader')
config.module.rule('postcss').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('postcss').oneOf('normal').use('vue-style-loader')
config.module.rule('scss').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('scss').oneOf('vue').use('vue-style-loader')
config.module.rule('scss').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('scss').oneOf('normal').use('vue-style-loader')
config.module.rule('sass').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('sass').oneOf('vue').use('vue-style-loader')
config.module.rule('sass').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('sass').oneOf('normal').use('vue-style-loader')
config.module.rule('less').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('less').oneOf('vue').use('vue-style-loader')
config.module.rule('less').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('less').oneOf('normal').use('vue-style-loader')
config.module.rule('stylus').oneOf('vue-modules').use('vue-style-loader')
config.module.rule('stylus').oneOf('vue').use('vue-style-loader')
config.module.rule('stylus').oneOf('normal-modules').use('vue-style-loader')
config.module.rule('stylus').oneOf('normal').use('vue-style-loader')
So, we can set shadowMode: true
for those configs in vue.config.js
with this snippet:
function enableShadowCss(config) {
const configs = [
config.module.rule('vue').use('vue-loader'),
config.module.rule('css').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('css').oneOf('vue').use('vue-style-loader'),
config.module.rule('css').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('css').oneOf('normal').use('vue-style-loader'),
config.module.rule('postcss').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('postcss').oneOf('vue').use('vue-style-loader'),
config.module.rule('postcss').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('postcss').oneOf('normal').use('vue-style-loader'),
config.module.rule('scss').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('scss').oneOf('vue').use('vue-style-loader'),
config.module.rule('scss').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('scss').oneOf('normal').use('vue-style-loader'),
config.module.rule('sass').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('sass').oneOf('vue').use('vue-style-loader'),
config.module.rule('sass').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('sass').oneOf('normal').use('vue-style-loader'),
config.module.rule('less').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('less').oneOf('vue').use('vue-style-loader'),
config.module.rule('less').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('less').oneOf('normal').use('vue-style-loader'),
config.module.rule('stylus').oneOf('vue-modules').use('vue-style-loader'),
config.module.rule('stylus').oneOf('vue').use('vue-style-loader'),
config.module.rule('stylus').oneOf('normal-modules').use('vue-style-loader'),
config.module.rule('stylus').oneOf('normal').use('vue-style-loader'),
];
configs.forEach(c => c.tap(options => {
options.shadowMode = true;
return options;
}));
}
module.exports = {
// https://cli.vuejs.org/guide/webpack.html#chaining-advanced
chainWebpack: config => {
enableShadowCss(config);
}
}
Creating <projectroot>/vue.config.js
with the snippet above enables Shadow CSS in development mode in your project. See https://github.com/snirp/vue-web-component/pull/1.
edited Dec 2 '18 at 9:08
answered Dec 2 '18 at 6:42


tony19tony19
23.5k44175
23.5k44175
Thanks a bundle, that works like a charm. I will try to brush upenableShadowConfig()
to recursively scan the config forshadowMode = false
values. That should make it a bit more resilient. Would you agree that there should be preferably be a single (default) config setting for shadowMode?
– Roy Prins
Dec 2 '18 at 10:05
Ps: I plan to do a short blog post on Vue web components. (How) can I credit you for the input?
– Roy Prins
Dec 2 '18 at 10:09
@RoyPrins No problem :) Yes, I agreeshadowMode
should typically be the same value throughout: either allfalse
(the default) or alltrue
. But perhaps there's an interesting use case for having only a subset of components use Shadow CSS.
– tony19
Dec 2 '18 at 10:53
@RoyPrins Sure, credit tony19@gmail.com (email if more info needed). I'm looking forward to the read.
– tony19
Dec 2 '18 at 10:55
add a comment |
Thanks a bundle, that works like a charm. I will try to brush upenableShadowConfig()
to recursively scan the config forshadowMode = false
values. That should make it a bit more resilient. Would you agree that there should be preferably be a single (default) config setting for shadowMode?
– Roy Prins
Dec 2 '18 at 10:05
Ps: I plan to do a short blog post on Vue web components. (How) can I credit you for the input?
– Roy Prins
Dec 2 '18 at 10:09
@RoyPrins No problem :) Yes, I agreeshadowMode
should typically be the same value throughout: either allfalse
(the default) or alltrue
. But perhaps there's an interesting use case for having only a subset of components use Shadow CSS.
– tony19
Dec 2 '18 at 10:53
@RoyPrins Sure, credit tony19@gmail.com (email if more info needed). I'm looking forward to the read.
– tony19
Dec 2 '18 at 10:55
Thanks a bundle, that works like a charm. I will try to brush up
enableShadowConfig()
to recursively scan the config for shadowMode = false
values. That should make it a bit more resilient. Would you agree that there should be preferably be a single (default) config setting for shadowMode?– Roy Prins
Dec 2 '18 at 10:05
Thanks a bundle, that works like a charm. I will try to brush up
enableShadowConfig()
to recursively scan the config for shadowMode = false
values. That should make it a bit more resilient. Would you agree that there should be preferably be a single (default) config setting for shadowMode?– Roy Prins
Dec 2 '18 at 10:05
Ps: I plan to do a short blog post on Vue web components. (How) can I credit you for the input?
– Roy Prins
Dec 2 '18 at 10:09
Ps: I plan to do a short blog post on Vue web components. (How) can I credit you for the input?
– Roy Prins
Dec 2 '18 at 10:09
@RoyPrins No problem :) Yes, I agree
shadowMode
should typically be the same value throughout: either all false
(the default) or all true
. But perhaps there's an interesting use case for having only a subset of components use Shadow CSS.– tony19
Dec 2 '18 at 10:53
@RoyPrins No problem :) Yes, I agree
shadowMode
should typically be the same value throughout: either all false
(the default) or all true
. But perhaps there's an interesting use case for having only a subset of components use Shadow CSS.– tony19
Dec 2 '18 at 10:53
@RoyPrins Sure, credit tony19@gmail.com (email if more info needed). I'm looking forward to the read.
– tony19
Dec 2 '18 at 10:55
@RoyPrins Sure, credit tony19@gmail.com (email if more info needed). I'm looking forward to the read.
– tony19
Dec 2 '18 at 10:55
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53431754%2fstyling-not-applied-to-vue-web-component-during-development%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KMj38Qoizsk,DcY,C1w1 rR2mJoO1e5nJokjgx3b8SeJ290CvYRGHaGQrvwt2Z1GGzRDhioGkf
Do you want to inline style??
– Tushar Kumawat
Nov 30 '18 at 15:47
No inline styles, I just want to add the <style> section of the Vue component added to the Shadow DOM of the web component. Feel free to check the Github repo.
– Roy Prins
Nov 30 '18 at 16:05