Improvise the code against test cases where code fails
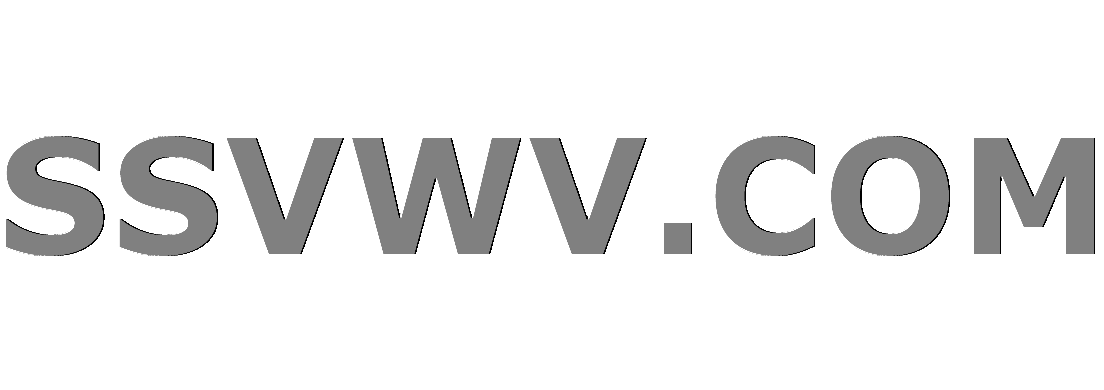
Multi tool use
$begingroup$
I wrote below code and i want to identify the test cases where my code fails.I recently participated in contest where my one test case is failed to pass out of 15 test cases, I have one more attempt left and I want to identify the possible test cases where my test case failed. so i will improvise the same, in addition I also want to enhance it, meaning,also suggest me the best practice to improve performance.
At last, In the below code I have one method returnFinalComplement(String reverseStr)
which return the complement, for calculating complement I have two Approaches, both are doing the same job, I am currenlty using approach-1
, but i want to know about approach-2
, which approach can pass all the test cases .
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.SortedSet;
import java.util.TreeSet;
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
*
* @author Ossu
*/
class DNAString {
private final static Character DATASET = {'A', 'C', 'G', 'T'};
private final static int MIN_RANGE = 0;
private final static int MAX_RANGE = 1000;
private final static String INVALID_CHAR = "Invalid input,not matched with dataset";
private final static String CHAR_LENGTH_EXCEEDS = "Input does not allow to be exceeds more than " + MAX_RANGE + " characters";
private static String returnFinalComplement(String reverseStr) {
//APPROACH-1
StringBuilder finalStr = new StringBuilder();
for (char c : reverseStr.toCharArray()) {
finalStr.append(
c == 'G' ? 'C'
: c == 'C' ? 'G'
: c == 'T' ? 'A'
: c == 'A' ? 'T'
: c
);
}
return finalStr.toString();
//APPROACH-2
char charlist = list.toCharArray();
for (int i = 0; i < charlist.length; i++) {
switch (charlist[i]) {
case 'A': {
charlist[i] = 'T';
}
break;
case 'T': {
charlist[i] = 'A';
}
break;
case 'G': {
charlist[i] = 'C';
}
break;
case 'C': {
charlist[i] = 'G';
}
break;
}
}
return new String(charlist);
}
public static boolean validateInput(String input) {
List<Character> chars = new ArrayList<>();
for (char c : input.toCharArray()) {
chars.add(c);
}
boolean result = false;
SortedSet<Character> mySet = new TreeSet<>(chars);
for (int i = 0; i <= mySet.size();) {
for (Character c : mySet) {
result = Objects.equals(c, DATASET[i]);
i++;
if (!result) {
break;
}
}
if (result) {
return result;
} else {
break;
}
}
return result;
}
public static String reverseIt(String source) {
int i, len = source.length();
StringBuilder dest = new StringBuilder(len);
for (i = (len - 1); i >= 0; i--) {
dest.append(source.charAt(i));
}
return dest.toString();
}
public static void main(String args) throws IOException {
BufferedReader readInput = new BufferedReader(new InputStreamReader(System.in));
String source = readInput.readLine();
if (source.length() > MIN_RANGE && source.length() <= MAX_RANGE) {
boolean validateInput = validateInput(source);
if (validateInput) {
// String reverseString = reverseIt(source);
String reverseString = reverseIt(source);
System.out.println(returnFinalComplement(reverseString));
// String revereStringComplement = returnFinalComplement(reverseString);
} else {
System.out.println(INVALID_CHAR);
}
} else {
System.out.println(CHAR_LENGTH_EXCEEDS);
}
}
}
java
$endgroup$
add a comment |
$begingroup$
I wrote below code and i want to identify the test cases where my code fails.I recently participated in contest where my one test case is failed to pass out of 15 test cases, I have one more attempt left and I want to identify the possible test cases where my test case failed. so i will improvise the same, in addition I also want to enhance it, meaning,also suggest me the best practice to improve performance.
At last, In the below code I have one method returnFinalComplement(String reverseStr)
which return the complement, for calculating complement I have two Approaches, both are doing the same job, I am currenlty using approach-1
, but i want to know about approach-2
, which approach can pass all the test cases .
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.SortedSet;
import java.util.TreeSet;
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
*
* @author Ossu
*/
class DNAString {
private final static Character DATASET = {'A', 'C', 'G', 'T'};
private final static int MIN_RANGE = 0;
private final static int MAX_RANGE = 1000;
private final static String INVALID_CHAR = "Invalid input,not matched with dataset";
private final static String CHAR_LENGTH_EXCEEDS = "Input does not allow to be exceeds more than " + MAX_RANGE + " characters";
private static String returnFinalComplement(String reverseStr) {
//APPROACH-1
StringBuilder finalStr = new StringBuilder();
for (char c : reverseStr.toCharArray()) {
finalStr.append(
c == 'G' ? 'C'
: c == 'C' ? 'G'
: c == 'T' ? 'A'
: c == 'A' ? 'T'
: c
);
}
return finalStr.toString();
//APPROACH-2
char charlist = list.toCharArray();
for (int i = 0; i < charlist.length; i++) {
switch (charlist[i]) {
case 'A': {
charlist[i] = 'T';
}
break;
case 'T': {
charlist[i] = 'A';
}
break;
case 'G': {
charlist[i] = 'C';
}
break;
case 'C': {
charlist[i] = 'G';
}
break;
}
}
return new String(charlist);
}
public static boolean validateInput(String input) {
List<Character> chars = new ArrayList<>();
for (char c : input.toCharArray()) {
chars.add(c);
}
boolean result = false;
SortedSet<Character> mySet = new TreeSet<>(chars);
for (int i = 0; i <= mySet.size();) {
for (Character c : mySet) {
result = Objects.equals(c, DATASET[i]);
i++;
if (!result) {
break;
}
}
if (result) {
return result;
} else {
break;
}
}
return result;
}
public static String reverseIt(String source) {
int i, len = source.length();
StringBuilder dest = new StringBuilder(len);
for (i = (len - 1); i >= 0; i--) {
dest.append(source.charAt(i));
}
return dest.toString();
}
public static void main(String args) throws IOException {
BufferedReader readInput = new BufferedReader(new InputStreamReader(System.in));
String source = readInput.readLine();
if (source.length() > MIN_RANGE && source.length() <= MAX_RANGE) {
boolean validateInput = validateInput(source);
if (validateInput) {
// String reverseString = reverseIt(source);
String reverseString = reverseIt(source);
System.out.println(returnFinalComplement(reverseString));
// String revereStringComplement = returnFinalComplement(reverseString);
} else {
System.out.println(INVALID_CHAR);
}
} else {
System.out.println(CHAR_LENGTH_EXCEEDS);
}
}
}
java
$endgroup$
2
$begingroup$
If this code is failing a test case, then it is not ready for review on this site.
$endgroup$
– Joe C
13 mins ago
$begingroup$
That's why i am here to identify and improvise, there is nothing wrong if someone tell me my mistake and I will learn from that, if you'are not interested than take the liberty of my words, just stay away from my post, if you're not able to taught some good things about coding standard apart from negative comments.
$endgroup$
– user10753505
5 mins ago
$begingroup$
I'm just saying that this question is off-topic on this site. It might be on-topic on our sister site Stack Overflow, but you should review their help center before posting there. (In particular, they will want you to shorten your code significantly, to no more than 15-20 lines, before posting there.)
$endgroup$
– Joe C
3 mins ago
$begingroup$
@JoeC Not just shortened, but turned into a Minimum, Complete, Verifiable Example. Since this is code for a programming-challenge, I'd recommend against that and try debugging the program (starting from scratch might be in order) instead.
$endgroup$
– Mast
11 secs ago
add a comment |
$begingroup$
I wrote below code and i want to identify the test cases where my code fails.I recently participated in contest where my one test case is failed to pass out of 15 test cases, I have one more attempt left and I want to identify the possible test cases where my test case failed. so i will improvise the same, in addition I also want to enhance it, meaning,also suggest me the best practice to improve performance.
At last, In the below code I have one method returnFinalComplement(String reverseStr)
which return the complement, for calculating complement I have two Approaches, both are doing the same job, I am currenlty using approach-1
, but i want to know about approach-2
, which approach can pass all the test cases .
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.SortedSet;
import java.util.TreeSet;
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
*
* @author Ossu
*/
class DNAString {
private final static Character DATASET = {'A', 'C', 'G', 'T'};
private final static int MIN_RANGE = 0;
private final static int MAX_RANGE = 1000;
private final static String INVALID_CHAR = "Invalid input,not matched with dataset";
private final static String CHAR_LENGTH_EXCEEDS = "Input does not allow to be exceeds more than " + MAX_RANGE + " characters";
private static String returnFinalComplement(String reverseStr) {
//APPROACH-1
StringBuilder finalStr = new StringBuilder();
for (char c : reverseStr.toCharArray()) {
finalStr.append(
c == 'G' ? 'C'
: c == 'C' ? 'G'
: c == 'T' ? 'A'
: c == 'A' ? 'T'
: c
);
}
return finalStr.toString();
//APPROACH-2
char charlist = list.toCharArray();
for (int i = 0; i < charlist.length; i++) {
switch (charlist[i]) {
case 'A': {
charlist[i] = 'T';
}
break;
case 'T': {
charlist[i] = 'A';
}
break;
case 'G': {
charlist[i] = 'C';
}
break;
case 'C': {
charlist[i] = 'G';
}
break;
}
}
return new String(charlist);
}
public static boolean validateInput(String input) {
List<Character> chars = new ArrayList<>();
for (char c : input.toCharArray()) {
chars.add(c);
}
boolean result = false;
SortedSet<Character> mySet = new TreeSet<>(chars);
for (int i = 0; i <= mySet.size();) {
for (Character c : mySet) {
result = Objects.equals(c, DATASET[i]);
i++;
if (!result) {
break;
}
}
if (result) {
return result;
} else {
break;
}
}
return result;
}
public static String reverseIt(String source) {
int i, len = source.length();
StringBuilder dest = new StringBuilder(len);
for (i = (len - 1); i >= 0; i--) {
dest.append(source.charAt(i));
}
return dest.toString();
}
public static void main(String args) throws IOException {
BufferedReader readInput = new BufferedReader(new InputStreamReader(System.in));
String source = readInput.readLine();
if (source.length() > MIN_RANGE && source.length() <= MAX_RANGE) {
boolean validateInput = validateInput(source);
if (validateInput) {
// String reverseString = reverseIt(source);
String reverseString = reverseIt(source);
System.out.println(returnFinalComplement(reverseString));
// String revereStringComplement = returnFinalComplement(reverseString);
} else {
System.out.println(INVALID_CHAR);
}
} else {
System.out.println(CHAR_LENGTH_EXCEEDS);
}
}
}
java
$endgroup$
I wrote below code and i want to identify the test cases where my code fails.I recently participated in contest where my one test case is failed to pass out of 15 test cases, I have one more attempt left and I want to identify the possible test cases where my test case failed. so i will improvise the same, in addition I also want to enhance it, meaning,also suggest me the best practice to improve performance.
At last, In the below code I have one method returnFinalComplement(String reverseStr)
which return the complement, for calculating complement I have two Approaches, both are doing the same job, I am currenlty using approach-1
, but i want to know about approach-2
, which approach can pass all the test cases .
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.SortedSet;
import java.util.TreeSet;
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
*
* @author Ossu
*/
class DNAString {
private final static Character DATASET = {'A', 'C', 'G', 'T'};
private final static int MIN_RANGE = 0;
private final static int MAX_RANGE = 1000;
private final static String INVALID_CHAR = "Invalid input,not matched with dataset";
private final static String CHAR_LENGTH_EXCEEDS = "Input does not allow to be exceeds more than " + MAX_RANGE + " characters";
private static String returnFinalComplement(String reverseStr) {
//APPROACH-1
StringBuilder finalStr = new StringBuilder();
for (char c : reverseStr.toCharArray()) {
finalStr.append(
c == 'G' ? 'C'
: c == 'C' ? 'G'
: c == 'T' ? 'A'
: c == 'A' ? 'T'
: c
);
}
return finalStr.toString();
//APPROACH-2
char charlist = list.toCharArray();
for (int i = 0; i < charlist.length; i++) {
switch (charlist[i]) {
case 'A': {
charlist[i] = 'T';
}
break;
case 'T': {
charlist[i] = 'A';
}
break;
case 'G': {
charlist[i] = 'C';
}
break;
case 'C': {
charlist[i] = 'G';
}
break;
}
}
return new String(charlist);
}
public static boolean validateInput(String input) {
List<Character> chars = new ArrayList<>();
for (char c : input.toCharArray()) {
chars.add(c);
}
boolean result = false;
SortedSet<Character> mySet = new TreeSet<>(chars);
for (int i = 0; i <= mySet.size();) {
for (Character c : mySet) {
result = Objects.equals(c, DATASET[i]);
i++;
if (!result) {
break;
}
}
if (result) {
return result;
} else {
break;
}
}
return result;
}
public static String reverseIt(String source) {
int i, len = source.length();
StringBuilder dest = new StringBuilder(len);
for (i = (len - 1); i >= 0; i--) {
dest.append(source.charAt(i));
}
return dest.toString();
}
public static void main(String args) throws IOException {
BufferedReader readInput = new BufferedReader(new InputStreamReader(System.in));
String source = readInput.readLine();
if (source.length() > MIN_RANGE && source.length() <= MAX_RANGE) {
boolean validateInput = validateInput(source);
if (validateInput) {
// String reverseString = reverseIt(source);
String reverseString = reverseIt(source);
System.out.println(returnFinalComplement(reverseString));
// String revereStringComplement = returnFinalComplement(reverseString);
} else {
System.out.println(INVALID_CHAR);
}
} else {
System.out.println(CHAR_LENGTH_EXCEEDS);
}
}
}
java
java
edited 8 mins ago
user10753505
asked 15 mins ago
user10753505user10753505
53
53
2
$begingroup$
If this code is failing a test case, then it is not ready for review on this site.
$endgroup$
– Joe C
13 mins ago
$begingroup$
That's why i am here to identify and improvise, there is nothing wrong if someone tell me my mistake and I will learn from that, if you'are not interested than take the liberty of my words, just stay away from my post, if you're not able to taught some good things about coding standard apart from negative comments.
$endgroup$
– user10753505
5 mins ago
$begingroup$
I'm just saying that this question is off-topic on this site. It might be on-topic on our sister site Stack Overflow, but you should review their help center before posting there. (In particular, they will want you to shorten your code significantly, to no more than 15-20 lines, before posting there.)
$endgroup$
– Joe C
3 mins ago
$begingroup$
@JoeC Not just shortened, but turned into a Minimum, Complete, Verifiable Example. Since this is code for a programming-challenge, I'd recommend against that and try debugging the program (starting from scratch might be in order) instead.
$endgroup$
– Mast
11 secs ago
add a comment |
2
$begingroup$
If this code is failing a test case, then it is not ready for review on this site.
$endgroup$
– Joe C
13 mins ago
$begingroup$
That's why i am here to identify and improvise, there is nothing wrong if someone tell me my mistake and I will learn from that, if you'are not interested than take the liberty of my words, just stay away from my post, if you're not able to taught some good things about coding standard apart from negative comments.
$endgroup$
– user10753505
5 mins ago
$begingroup$
I'm just saying that this question is off-topic on this site. It might be on-topic on our sister site Stack Overflow, but you should review their help center before posting there. (In particular, they will want you to shorten your code significantly, to no more than 15-20 lines, before posting there.)
$endgroup$
– Joe C
3 mins ago
$begingroup$
@JoeC Not just shortened, but turned into a Minimum, Complete, Verifiable Example. Since this is code for a programming-challenge, I'd recommend against that and try debugging the program (starting from scratch might be in order) instead.
$endgroup$
– Mast
11 secs ago
2
2
$begingroup$
If this code is failing a test case, then it is not ready for review on this site.
$endgroup$
– Joe C
13 mins ago
$begingroup$
If this code is failing a test case, then it is not ready for review on this site.
$endgroup$
– Joe C
13 mins ago
$begingroup$
That's why i am here to identify and improvise, there is nothing wrong if someone tell me my mistake and I will learn from that, if you'are not interested than take the liberty of my words, just stay away from my post, if you're not able to taught some good things about coding standard apart from negative comments.
$endgroup$
– user10753505
5 mins ago
$begingroup$
That's why i am here to identify and improvise, there is nothing wrong if someone tell me my mistake and I will learn from that, if you'are not interested than take the liberty of my words, just stay away from my post, if you're not able to taught some good things about coding standard apart from negative comments.
$endgroup$
– user10753505
5 mins ago
$begingroup$
I'm just saying that this question is off-topic on this site. It might be on-topic on our sister site Stack Overflow, but you should review their help center before posting there. (In particular, they will want you to shorten your code significantly, to no more than 15-20 lines, before posting there.)
$endgroup$
– Joe C
3 mins ago
$begingroup$
I'm just saying that this question is off-topic on this site. It might be on-topic on our sister site Stack Overflow, but you should review their help center before posting there. (In particular, they will want you to shorten your code significantly, to no more than 15-20 lines, before posting there.)
$endgroup$
– Joe C
3 mins ago
$begingroup$
@JoeC Not just shortened, but turned into a Minimum, Complete, Verifiable Example. Since this is code for a programming-challenge, I'd recommend against that and try debugging the program (starting from scratch might be in order) instead.
$endgroup$
– Mast
11 secs ago
$begingroup$
@JoeC Not just shortened, but turned into a Minimum, Complete, Verifiable Example. Since this is code for a programming-challenge, I'd recommend against that and try debugging the program (starting from scratch might be in order) instead.
$endgroup$
– Mast
11 secs ago
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f211871%2fimprovise-the-code-against-test-cases-where-code-fails%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f211871%2fimprovise-the-code-against-test-cases-where-code-fails%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
dhF,jSh o
2
$begingroup$
If this code is failing a test case, then it is not ready for review on this site.
$endgroup$
– Joe C
13 mins ago
$begingroup$
That's why i am here to identify and improvise, there is nothing wrong if someone tell me my mistake and I will learn from that, if you'are not interested than take the liberty of my words, just stay away from my post, if you're not able to taught some good things about coding standard apart from negative comments.
$endgroup$
– user10753505
5 mins ago
$begingroup$
I'm just saying that this question is off-topic on this site. It might be on-topic on our sister site Stack Overflow, but you should review their help center before posting there. (In particular, they will want you to shorten your code significantly, to no more than 15-20 lines, before posting there.)
$endgroup$
– Joe C
3 mins ago
$begingroup$
@JoeC Not just shortened, but turned into a Minimum, Complete, Verifiable Example. Since this is code for a programming-challenge, I'd recommend against that and try debugging the program (starting from scratch might be in order) instead.
$endgroup$
– Mast
11 secs ago