Fragment becomes empty after Notification
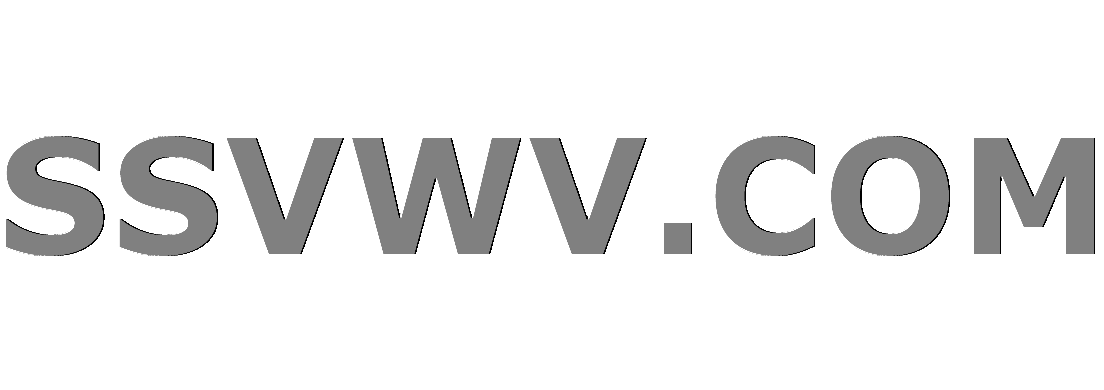
Multi tool use
I have an Activity
which loads a Fragment
using getFragmentManager()
. The fragment is working perfecty until an android notification appears. After that, the fragment becomes empty.
This is my application after the activity has loaded the fragment. The red square is the fragment.
Here, a external notification arrives to the mobile:
Only the fragment becomes empty:
Here my Activity
:
public class MainActivity extends AppCompatActivity implements NavigationView.OnNavigationItemSelectedListener, FragmentSettings.OnFragmentInteractionListener{
FragmentManager fragmentManager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
if (fragmentManager == null) {
fragmentManager = getSupportFragmentManager();
}
}
@SuppressWarnings("StatementWithEmptyBody")
@Override
public boolean onNavigationItemSelected(MenuItem item) {
if (id == R.id.nav_locker_room) {
fragmentNameSection = "FragmentLockerRoom";
fragment = FragmentLockerRoom.newInstance(user, "");
}
fragmentManager.beginTransaction().replace(R.id.flContent, fragment, fragmentNameSection).commit();
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
drawer.closeDrawer(GravityCompat.START);
}
@Override
public void onResume() {
super.onResume();
}
}
Here the activity_main.xml
:
<?xml version="1.0" encoding="utf-8"?>
<android.support.v4.widget.DrawerLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/drawer_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:openDrawer="start">
<include
layout="@layout/app_bar_main"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<android.support.design.widget.NavigationView
android:id="@+id/nav_view"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="start"
android:background="@drawable/side_nav_menu"
app:itemTextColor="@android:color/white"
app:itemIconTint="@android:color/white"
app:headerLayout="@layout/nav_header_main"
app:menu="@menu/activity_main_drawer" />
</android.support.v4.widget.DrawerLayout>
And finally this is my Fragment
:
public class FragmentSettings extends Fragment {
private static final String ARG_USER = "user";
private static final String ARG_PARAM2 = "param2";
private String mParam1;
private String mParam2;
getUser user;
View v;
DatabaseManager db;
private OnFragmentInteractionListener mListener;
ToggleButton notificationTrain, notificationNextMatch, liveSounds;
LayoutInflater inflater;
ViewGroup container;
FragmentSettings fragmentSettings;
public FragmentSettings() {
}
public static FragmentSettings newInstance(getUser user, String param2) {
FragmentSettings fragment = new FragmentSettings();
Bundle args = new Bundle();
args.putSerializable(ARG_USER, user);
args.putString(ARG_PARAM2, param2);
fragment.setArguments(args);
return fragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
user = (com.androidsrc.futbolin.communications.http.auth.get.getUser) getArguments().getSerializable(ARG_USER);
mParam2 = getArguments().getString(ARG_PARAM2);
}
this.fragmentSettings = this;
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
this.inflater = inflater;
this.container = container;
buildLayout();
return v;
}
// TODO: Rename method, update argument and hook method into UI event
public void onButtonPressed(Uri uri) {
if (mListener != null) {
mListener.onFragmentInteraction(uri);
}
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof OnFragmentInteractionListener) {
mListener = (OnFragmentInteractionListener) context;
} else {
throw new RuntimeException(context.toString()
+ " must implement OnFragmentInteractionListener");
}
}
@Override
public void onDetach() {
super.onDetach();
mListener = null;
}
/**
* This interface must be implemented by activities that contain this
* fragment to allow an interaction in this fragment to be communicated
* to the activity and potentially other fragments contained in that
* activity.
* <p/>
* See the Android Training lesson <a href=
* "http://developer.android.com/training/basics/fragments/communicating.html"
* >Communicating with Other Fragments</a> for more information.
*/
public interface OnFragmentInteractionListener {
// TODO: Update argument type and name
void onFragmentInteraction(Uri uri);
}
public void buildLayout(){
db = new DatabaseManager(getActivity());
v = inflater.inflate(R.layout.fragment_fragment_settings, container, false);
notificationTrain = v.findViewById(R.id.fragment_settings_notification_trainment_toggle);
notificationNextMatch = v.findViewById(R.id.fragment_settings_notification_next_match_toggle);
liveSounds = v.findViewById(R.id.fragment_settings_notification_live_sounds_toggle);
notificationTrain.setChecked(db.findNotification().isTrainActive());
notificationNextMatch.setChecked(db.findNotification().isMatchActive());
liveSounds.setChecked(db.findNotification().isLiveSoundsActive());
Log.e("notif",db.findNotification().toString());
notificationTrain.setOnCheckedChangeListener( new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton toggleButton, boolean isChecked) {
if(db == null){
db = new DatabaseManager(getActivity());
}
Notification notification = db.findNotification();
notification.setTrainActive(isChecked);
db.saveNotification(notification);
}
}) ;
notificationNextMatch.setOnCheckedChangeListener( new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton toggleButton, boolean isChecked) {
if(db == null){
db = new DatabaseManager(getActivity());
}
Notification notification = db.findNotification();
notification.setMatchActive(isChecked);
db.saveNotification(notification);
}
}) ;
liveSounds.setOnCheckedChangeListener( new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton toggleButton, boolean isChecked) {
if(db == null){
db = new DatabaseManager(getActivity());
}
Notification notification = db.findNotification();
notification.setLiveSoundsActive(isChecked);
db.saveNotification(notification);
}
}) ;
}
}
And the layout of my Fragment
is:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<ScrollView
android:id="@+id/fragment_locker_room_scrollview"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
android:layout_marginBottom="60dp"
>
<androidx.constraintlayout.widget.ConstraintLayout
android:id="@+id/fragment_locker_room_total_constraint_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
>
<!--
REST OF VIEWS
-->
</androidx.constraintlayout.widget.ConstraintLayout>
</ScrollView>
</LinearLayout>


add a comment |
I have an Activity
which loads a Fragment
using getFragmentManager()
. The fragment is working perfecty until an android notification appears. After that, the fragment becomes empty.
This is my application after the activity has loaded the fragment. The red square is the fragment.
Here, a external notification arrives to the mobile:
Only the fragment becomes empty:
Here my Activity
:
public class MainActivity extends AppCompatActivity implements NavigationView.OnNavigationItemSelectedListener, FragmentSettings.OnFragmentInteractionListener{
FragmentManager fragmentManager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
if (fragmentManager == null) {
fragmentManager = getSupportFragmentManager();
}
}
@SuppressWarnings("StatementWithEmptyBody")
@Override
public boolean onNavigationItemSelected(MenuItem item) {
if (id == R.id.nav_locker_room) {
fragmentNameSection = "FragmentLockerRoom";
fragment = FragmentLockerRoom.newInstance(user, "");
}
fragmentManager.beginTransaction().replace(R.id.flContent, fragment, fragmentNameSection).commit();
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
drawer.closeDrawer(GravityCompat.START);
}
@Override
public void onResume() {
super.onResume();
}
}
Here the activity_main.xml
:
<?xml version="1.0" encoding="utf-8"?>
<android.support.v4.widget.DrawerLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/drawer_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:openDrawer="start">
<include
layout="@layout/app_bar_main"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<android.support.design.widget.NavigationView
android:id="@+id/nav_view"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="start"
android:background="@drawable/side_nav_menu"
app:itemTextColor="@android:color/white"
app:itemIconTint="@android:color/white"
app:headerLayout="@layout/nav_header_main"
app:menu="@menu/activity_main_drawer" />
</android.support.v4.widget.DrawerLayout>
And finally this is my Fragment
:
public class FragmentSettings extends Fragment {
private static final String ARG_USER = "user";
private static final String ARG_PARAM2 = "param2";
private String mParam1;
private String mParam2;
getUser user;
View v;
DatabaseManager db;
private OnFragmentInteractionListener mListener;
ToggleButton notificationTrain, notificationNextMatch, liveSounds;
LayoutInflater inflater;
ViewGroup container;
FragmentSettings fragmentSettings;
public FragmentSettings() {
}
public static FragmentSettings newInstance(getUser user, String param2) {
FragmentSettings fragment = new FragmentSettings();
Bundle args = new Bundle();
args.putSerializable(ARG_USER, user);
args.putString(ARG_PARAM2, param2);
fragment.setArguments(args);
return fragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
user = (com.androidsrc.futbolin.communications.http.auth.get.getUser) getArguments().getSerializable(ARG_USER);
mParam2 = getArguments().getString(ARG_PARAM2);
}
this.fragmentSettings = this;
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
this.inflater = inflater;
this.container = container;
buildLayout();
return v;
}
// TODO: Rename method, update argument and hook method into UI event
public void onButtonPressed(Uri uri) {
if (mListener != null) {
mListener.onFragmentInteraction(uri);
}
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof OnFragmentInteractionListener) {
mListener = (OnFragmentInteractionListener) context;
} else {
throw new RuntimeException(context.toString()
+ " must implement OnFragmentInteractionListener");
}
}
@Override
public void onDetach() {
super.onDetach();
mListener = null;
}
/**
* This interface must be implemented by activities that contain this
* fragment to allow an interaction in this fragment to be communicated
* to the activity and potentially other fragments contained in that
* activity.
* <p/>
* See the Android Training lesson <a href=
* "http://developer.android.com/training/basics/fragments/communicating.html"
* >Communicating with Other Fragments</a> for more information.
*/
public interface OnFragmentInteractionListener {
// TODO: Update argument type and name
void onFragmentInteraction(Uri uri);
}
public void buildLayout(){
db = new DatabaseManager(getActivity());
v = inflater.inflate(R.layout.fragment_fragment_settings, container, false);
notificationTrain = v.findViewById(R.id.fragment_settings_notification_trainment_toggle);
notificationNextMatch = v.findViewById(R.id.fragment_settings_notification_next_match_toggle);
liveSounds = v.findViewById(R.id.fragment_settings_notification_live_sounds_toggle);
notificationTrain.setChecked(db.findNotification().isTrainActive());
notificationNextMatch.setChecked(db.findNotification().isMatchActive());
liveSounds.setChecked(db.findNotification().isLiveSoundsActive());
Log.e("notif",db.findNotification().toString());
notificationTrain.setOnCheckedChangeListener( new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton toggleButton, boolean isChecked) {
if(db == null){
db = new DatabaseManager(getActivity());
}
Notification notification = db.findNotification();
notification.setTrainActive(isChecked);
db.saveNotification(notification);
}
}) ;
notificationNextMatch.setOnCheckedChangeListener( new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton toggleButton, boolean isChecked) {
if(db == null){
db = new DatabaseManager(getActivity());
}
Notification notification = db.findNotification();
notification.setMatchActive(isChecked);
db.saveNotification(notification);
}
}) ;
liveSounds.setOnCheckedChangeListener( new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton toggleButton, boolean isChecked) {
if(db == null){
db = new DatabaseManager(getActivity());
}
Notification notification = db.findNotification();
notification.setLiveSoundsActive(isChecked);
db.saveNotification(notification);
}
}) ;
}
}
And the layout of my Fragment
is:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<ScrollView
android:id="@+id/fragment_locker_room_scrollview"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
android:layout_marginBottom="60dp"
>
<androidx.constraintlayout.widget.ConstraintLayout
android:id="@+id/fragment_locker_room_total_constraint_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
>
<!--
REST OF VIEWS
-->
</androidx.constraintlayout.widget.ConstraintLayout>
</ScrollView>
</LinearLayout>


add a comment |
I have an Activity
which loads a Fragment
using getFragmentManager()
. The fragment is working perfecty until an android notification appears. After that, the fragment becomes empty.
This is my application after the activity has loaded the fragment. The red square is the fragment.
Here, a external notification arrives to the mobile:
Only the fragment becomes empty:
Here my Activity
:
public class MainActivity extends AppCompatActivity implements NavigationView.OnNavigationItemSelectedListener, FragmentSettings.OnFragmentInteractionListener{
FragmentManager fragmentManager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
if (fragmentManager == null) {
fragmentManager = getSupportFragmentManager();
}
}
@SuppressWarnings("StatementWithEmptyBody")
@Override
public boolean onNavigationItemSelected(MenuItem item) {
if (id == R.id.nav_locker_room) {
fragmentNameSection = "FragmentLockerRoom";
fragment = FragmentLockerRoom.newInstance(user, "");
}
fragmentManager.beginTransaction().replace(R.id.flContent, fragment, fragmentNameSection).commit();
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
drawer.closeDrawer(GravityCompat.START);
}
@Override
public void onResume() {
super.onResume();
}
}
Here the activity_main.xml
:
<?xml version="1.0" encoding="utf-8"?>
<android.support.v4.widget.DrawerLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/drawer_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:openDrawer="start">
<include
layout="@layout/app_bar_main"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<android.support.design.widget.NavigationView
android:id="@+id/nav_view"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="start"
android:background="@drawable/side_nav_menu"
app:itemTextColor="@android:color/white"
app:itemIconTint="@android:color/white"
app:headerLayout="@layout/nav_header_main"
app:menu="@menu/activity_main_drawer" />
</android.support.v4.widget.DrawerLayout>
And finally this is my Fragment
:
public class FragmentSettings extends Fragment {
private static final String ARG_USER = "user";
private static final String ARG_PARAM2 = "param2";
private String mParam1;
private String mParam2;
getUser user;
View v;
DatabaseManager db;
private OnFragmentInteractionListener mListener;
ToggleButton notificationTrain, notificationNextMatch, liveSounds;
LayoutInflater inflater;
ViewGroup container;
FragmentSettings fragmentSettings;
public FragmentSettings() {
}
public static FragmentSettings newInstance(getUser user, String param2) {
FragmentSettings fragment = new FragmentSettings();
Bundle args = new Bundle();
args.putSerializable(ARG_USER, user);
args.putString(ARG_PARAM2, param2);
fragment.setArguments(args);
return fragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
user = (com.androidsrc.futbolin.communications.http.auth.get.getUser) getArguments().getSerializable(ARG_USER);
mParam2 = getArguments().getString(ARG_PARAM2);
}
this.fragmentSettings = this;
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
this.inflater = inflater;
this.container = container;
buildLayout();
return v;
}
// TODO: Rename method, update argument and hook method into UI event
public void onButtonPressed(Uri uri) {
if (mListener != null) {
mListener.onFragmentInteraction(uri);
}
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof OnFragmentInteractionListener) {
mListener = (OnFragmentInteractionListener) context;
} else {
throw new RuntimeException(context.toString()
+ " must implement OnFragmentInteractionListener");
}
}
@Override
public void onDetach() {
super.onDetach();
mListener = null;
}
/**
* This interface must be implemented by activities that contain this
* fragment to allow an interaction in this fragment to be communicated
* to the activity and potentially other fragments contained in that
* activity.
* <p/>
* See the Android Training lesson <a href=
* "http://developer.android.com/training/basics/fragments/communicating.html"
* >Communicating with Other Fragments</a> for more information.
*/
public interface OnFragmentInteractionListener {
// TODO: Update argument type and name
void onFragmentInteraction(Uri uri);
}
public void buildLayout(){
db = new DatabaseManager(getActivity());
v = inflater.inflate(R.layout.fragment_fragment_settings, container, false);
notificationTrain = v.findViewById(R.id.fragment_settings_notification_trainment_toggle);
notificationNextMatch = v.findViewById(R.id.fragment_settings_notification_next_match_toggle);
liveSounds = v.findViewById(R.id.fragment_settings_notification_live_sounds_toggle);
notificationTrain.setChecked(db.findNotification().isTrainActive());
notificationNextMatch.setChecked(db.findNotification().isMatchActive());
liveSounds.setChecked(db.findNotification().isLiveSoundsActive());
Log.e("notif",db.findNotification().toString());
notificationTrain.setOnCheckedChangeListener( new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton toggleButton, boolean isChecked) {
if(db == null){
db = new DatabaseManager(getActivity());
}
Notification notification = db.findNotification();
notification.setTrainActive(isChecked);
db.saveNotification(notification);
}
}) ;
notificationNextMatch.setOnCheckedChangeListener( new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton toggleButton, boolean isChecked) {
if(db == null){
db = new DatabaseManager(getActivity());
}
Notification notification = db.findNotification();
notification.setMatchActive(isChecked);
db.saveNotification(notification);
}
}) ;
liveSounds.setOnCheckedChangeListener( new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton toggleButton, boolean isChecked) {
if(db == null){
db = new DatabaseManager(getActivity());
}
Notification notification = db.findNotification();
notification.setLiveSoundsActive(isChecked);
db.saveNotification(notification);
}
}) ;
}
}
And the layout of my Fragment
is:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<ScrollView
android:id="@+id/fragment_locker_room_scrollview"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
android:layout_marginBottom="60dp"
>
<androidx.constraintlayout.widget.ConstraintLayout
android:id="@+id/fragment_locker_room_total_constraint_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
>
<!--
REST OF VIEWS
-->
</androidx.constraintlayout.widget.ConstraintLayout>
</ScrollView>
</LinearLayout>


I have an Activity
which loads a Fragment
using getFragmentManager()
. The fragment is working perfecty until an android notification appears. After that, the fragment becomes empty.
This is my application after the activity has loaded the fragment. The red square is the fragment.
Here, a external notification arrives to the mobile:
Only the fragment becomes empty:
Here my Activity
:
public class MainActivity extends AppCompatActivity implements NavigationView.OnNavigationItemSelectedListener, FragmentSettings.OnFragmentInteractionListener{
FragmentManager fragmentManager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
if (fragmentManager == null) {
fragmentManager = getSupportFragmentManager();
}
}
@SuppressWarnings("StatementWithEmptyBody")
@Override
public boolean onNavigationItemSelected(MenuItem item) {
if (id == R.id.nav_locker_room) {
fragmentNameSection = "FragmentLockerRoom";
fragment = FragmentLockerRoom.newInstance(user, "");
}
fragmentManager.beginTransaction().replace(R.id.flContent, fragment, fragmentNameSection).commit();
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
drawer.closeDrawer(GravityCompat.START);
}
@Override
public void onResume() {
super.onResume();
}
}
Here the activity_main.xml
:
<?xml version="1.0" encoding="utf-8"?>
<android.support.v4.widget.DrawerLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/drawer_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:openDrawer="start">
<include
layout="@layout/app_bar_main"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<android.support.design.widget.NavigationView
android:id="@+id/nav_view"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="start"
android:background="@drawable/side_nav_menu"
app:itemTextColor="@android:color/white"
app:itemIconTint="@android:color/white"
app:headerLayout="@layout/nav_header_main"
app:menu="@menu/activity_main_drawer" />
</android.support.v4.widget.DrawerLayout>
And finally this is my Fragment
:
public class FragmentSettings extends Fragment {
private static final String ARG_USER = "user";
private static final String ARG_PARAM2 = "param2";
private String mParam1;
private String mParam2;
getUser user;
View v;
DatabaseManager db;
private OnFragmentInteractionListener mListener;
ToggleButton notificationTrain, notificationNextMatch, liveSounds;
LayoutInflater inflater;
ViewGroup container;
FragmentSettings fragmentSettings;
public FragmentSettings() {
}
public static FragmentSettings newInstance(getUser user, String param2) {
FragmentSettings fragment = new FragmentSettings();
Bundle args = new Bundle();
args.putSerializable(ARG_USER, user);
args.putString(ARG_PARAM2, param2);
fragment.setArguments(args);
return fragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
user = (com.androidsrc.futbolin.communications.http.auth.get.getUser) getArguments().getSerializable(ARG_USER);
mParam2 = getArguments().getString(ARG_PARAM2);
}
this.fragmentSettings = this;
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
this.inflater = inflater;
this.container = container;
buildLayout();
return v;
}
// TODO: Rename method, update argument and hook method into UI event
public void onButtonPressed(Uri uri) {
if (mListener != null) {
mListener.onFragmentInteraction(uri);
}
}
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof OnFragmentInteractionListener) {
mListener = (OnFragmentInteractionListener) context;
} else {
throw new RuntimeException(context.toString()
+ " must implement OnFragmentInteractionListener");
}
}
@Override
public void onDetach() {
super.onDetach();
mListener = null;
}
/**
* This interface must be implemented by activities that contain this
* fragment to allow an interaction in this fragment to be communicated
* to the activity and potentially other fragments contained in that
* activity.
* <p/>
* See the Android Training lesson <a href=
* "http://developer.android.com/training/basics/fragments/communicating.html"
* >Communicating with Other Fragments</a> for more information.
*/
public interface OnFragmentInteractionListener {
// TODO: Update argument type and name
void onFragmentInteraction(Uri uri);
}
public void buildLayout(){
db = new DatabaseManager(getActivity());
v = inflater.inflate(R.layout.fragment_fragment_settings, container, false);
notificationTrain = v.findViewById(R.id.fragment_settings_notification_trainment_toggle);
notificationNextMatch = v.findViewById(R.id.fragment_settings_notification_next_match_toggle);
liveSounds = v.findViewById(R.id.fragment_settings_notification_live_sounds_toggle);
notificationTrain.setChecked(db.findNotification().isTrainActive());
notificationNextMatch.setChecked(db.findNotification().isMatchActive());
liveSounds.setChecked(db.findNotification().isLiveSoundsActive());
Log.e("notif",db.findNotification().toString());
notificationTrain.setOnCheckedChangeListener( new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton toggleButton, boolean isChecked) {
if(db == null){
db = new DatabaseManager(getActivity());
}
Notification notification = db.findNotification();
notification.setTrainActive(isChecked);
db.saveNotification(notification);
}
}) ;
notificationNextMatch.setOnCheckedChangeListener( new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton toggleButton, boolean isChecked) {
if(db == null){
db = new DatabaseManager(getActivity());
}
Notification notification = db.findNotification();
notification.setMatchActive(isChecked);
db.saveNotification(notification);
}
}) ;
liveSounds.setOnCheckedChangeListener( new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton toggleButton, boolean isChecked) {
if(db == null){
db = new DatabaseManager(getActivity());
}
Notification notification = db.findNotification();
notification.setLiveSoundsActive(isChecked);
db.saveNotification(notification);
}
}) ;
}
}
And the layout of my Fragment
is:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<ScrollView
android:id="@+id/fragment_locker_room_scrollview"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
android:layout_marginBottom="60dp"
>
<androidx.constraintlayout.widget.ConstraintLayout
android:id="@+id/fragment_locker_room_total_constraint_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
>
<!--
REST OF VIEWS
-->
</androidx.constraintlayout.widget.ConstraintLayout>
</ScrollView>
</LinearLayout>




edited Nov 22 '18 at 23:37
Alberto Crespo
asked Jul 6 '18 at 16:39
Alberto CrespoAlberto Crespo
1,08451435
1,08451435
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Finally I found the solution. And ScrollView
that has a layout_height="match_parent"
when a notification is received changes his layout_height
to 0.
Changing layout_height="match_parent"
to layout_height="wrap_content"
in ScrollView
solves the issue.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f51214596%2ffragment-becomes-empty-after-notification%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Finally I found the solution. And ScrollView
that has a layout_height="match_parent"
when a notification is received changes his layout_height
to 0.
Changing layout_height="match_parent"
to layout_height="wrap_content"
in ScrollView
solves the issue.
add a comment |
Finally I found the solution. And ScrollView
that has a layout_height="match_parent"
when a notification is received changes his layout_height
to 0.
Changing layout_height="match_parent"
to layout_height="wrap_content"
in ScrollView
solves the issue.
add a comment |
Finally I found the solution. And ScrollView
that has a layout_height="match_parent"
when a notification is received changes his layout_height
to 0.
Changing layout_height="match_parent"
to layout_height="wrap_content"
in ScrollView
solves the issue.
Finally I found the solution. And ScrollView
that has a layout_height="match_parent"
when a notification is received changes his layout_height
to 0.
Changing layout_height="match_parent"
to layout_height="wrap_content"
in ScrollView
solves the issue.
answered Nov 22 '18 at 23:41
Alberto CrespoAlberto Crespo
1,08451435
1,08451435
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f51214596%2ffragment-becomes-empty-after-notification%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
mC W4VbzoL,um,d9IDeJQ5,pJ KhnkCz8M nz67CvlmcUIrW8d4yU88qu1u4mn NlqnBVRxw67msdklxGbp8JBEwLVhEjZatZDAv