Method that returns any type in Java
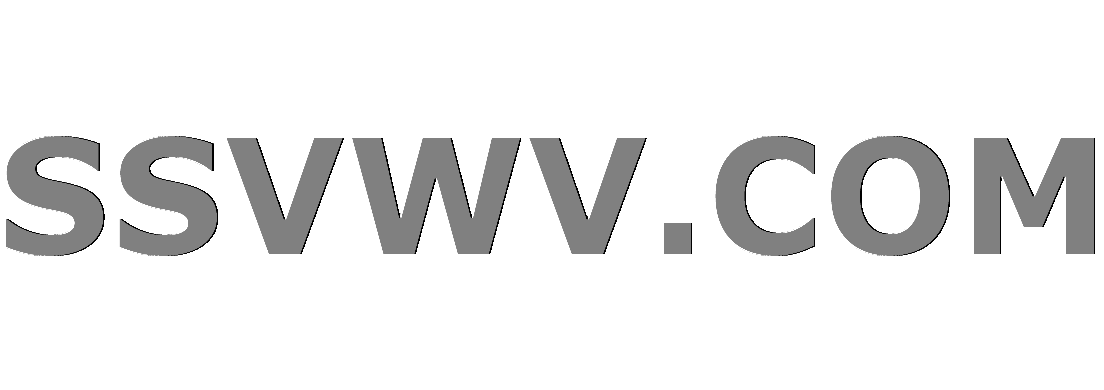
Multi tool use
I'm in a grade 11 computer science course and I'm trying to code a file that simplifies a lot of code. Right now I'm trying to merge input and output into one method;
public static void askln(String text, String type){
System.out.println(text);
if(type.equals("int"))
return getInt();
if(type.equals("char"))
return getChar();
if(type.equals("String"))
return getString();
if(type.equals("double"))
return getDouble();
if(type.equals("float"))
return getFloat();
if(type.equals("long"))
return getLong();
}
getInt()
is a method that gets an integer from the user. I'm assuming people will understand what the other getters do.
This code doesn't work because 'void' won't return anything. I was wondering if there was a return type that would allow me to return any value.
java return-type
|
show 1 more comment
I'm in a grade 11 computer science course and I'm trying to code a file that simplifies a lot of code. Right now I'm trying to merge input and output into one method;
public static void askln(String text, String type){
System.out.println(text);
if(type.equals("int"))
return getInt();
if(type.equals("char"))
return getChar();
if(type.equals("String"))
return getString();
if(type.equals("double"))
return getDouble();
if(type.equals("float"))
return getFloat();
if(type.equals("long"))
return getLong();
}
getInt()
is a method that gets an integer from the user. I'm assuming people will understand what the other getters do.
This code doesn't work because 'void' won't return anything. I was wondering if there was a return type that would allow me to return any value.
java return-type
3
public static Object
?
– Boris the Spider
Nov 22 '18 at 18:52
1
How do I compare strings in Java?
– luk2302
Nov 22 '18 at 18:53
1
Don't compare strings like this. In general, useequals
; but in this case, use a switch.
– Andy Turner
Nov 22 '18 at 18:53
1
I think you might do this with generics. Java is much stronger typed than other languages because having an unknown return type leads to bugs. Such a thing is frowned upon.
– K.Nicholas
Nov 22 '18 at 18:53
yeah perhaps make it a class or a struct with all these types and then return struct_name.value or class_object.value as it is hetrogenous (combination of types) hence we use object or struct_name as class identifiers to fetch concrete types
– Himanshu Ahuja
Nov 22 '18 at 18:57
|
show 1 more comment
I'm in a grade 11 computer science course and I'm trying to code a file that simplifies a lot of code. Right now I'm trying to merge input and output into one method;
public static void askln(String text, String type){
System.out.println(text);
if(type.equals("int"))
return getInt();
if(type.equals("char"))
return getChar();
if(type.equals("String"))
return getString();
if(type.equals("double"))
return getDouble();
if(type.equals("float"))
return getFloat();
if(type.equals("long"))
return getLong();
}
getInt()
is a method that gets an integer from the user. I'm assuming people will understand what the other getters do.
This code doesn't work because 'void' won't return anything. I was wondering if there was a return type that would allow me to return any value.
java return-type
I'm in a grade 11 computer science course and I'm trying to code a file that simplifies a lot of code. Right now I'm trying to merge input and output into one method;
public static void askln(String text, String type){
System.out.println(text);
if(type.equals("int"))
return getInt();
if(type.equals("char"))
return getChar();
if(type.equals("String"))
return getString();
if(type.equals("double"))
return getDouble();
if(type.equals("float"))
return getFloat();
if(type.equals("long"))
return getLong();
}
getInt()
is a method that gets an integer from the user. I'm assuming people will understand what the other getters do.
This code doesn't work because 'void' won't return anything. I was wondering if there was a return type that would allow me to return any value.
java return-type
java return-type
edited Nov 26 '18 at 18:16
Tim
asked Nov 22 '18 at 18:51


TimTim
92
92
3
public static Object
?
– Boris the Spider
Nov 22 '18 at 18:52
1
How do I compare strings in Java?
– luk2302
Nov 22 '18 at 18:53
1
Don't compare strings like this. In general, useequals
; but in this case, use a switch.
– Andy Turner
Nov 22 '18 at 18:53
1
I think you might do this with generics. Java is much stronger typed than other languages because having an unknown return type leads to bugs. Such a thing is frowned upon.
– K.Nicholas
Nov 22 '18 at 18:53
yeah perhaps make it a class or a struct with all these types and then return struct_name.value or class_object.value as it is hetrogenous (combination of types) hence we use object or struct_name as class identifiers to fetch concrete types
– Himanshu Ahuja
Nov 22 '18 at 18:57
|
show 1 more comment
3
public static Object
?
– Boris the Spider
Nov 22 '18 at 18:52
1
How do I compare strings in Java?
– luk2302
Nov 22 '18 at 18:53
1
Don't compare strings like this. In general, useequals
; but in this case, use a switch.
– Andy Turner
Nov 22 '18 at 18:53
1
I think you might do this with generics. Java is much stronger typed than other languages because having an unknown return type leads to bugs. Such a thing is frowned upon.
– K.Nicholas
Nov 22 '18 at 18:53
yeah perhaps make it a class or a struct with all these types and then return struct_name.value or class_object.value as it is hetrogenous (combination of types) hence we use object or struct_name as class identifiers to fetch concrete types
– Himanshu Ahuja
Nov 22 '18 at 18:57
3
3
public static Object
?– Boris the Spider
Nov 22 '18 at 18:52
public static Object
?– Boris the Spider
Nov 22 '18 at 18:52
1
1
How do I compare strings in Java?
– luk2302
Nov 22 '18 at 18:53
How do I compare strings in Java?
– luk2302
Nov 22 '18 at 18:53
1
1
Don't compare strings like this. In general, use
equals
; but in this case, use a switch.– Andy Turner
Nov 22 '18 at 18:53
Don't compare strings like this. In general, use
equals
; but in this case, use a switch.– Andy Turner
Nov 22 '18 at 18:53
1
1
I think you might do this with generics. Java is much stronger typed than other languages because having an unknown return type leads to bugs. Such a thing is frowned upon.
– K.Nicholas
Nov 22 '18 at 18:53
I think you might do this with generics. Java is much stronger typed than other languages because having an unknown return type leads to bugs. Such a thing is frowned upon.
– K.Nicholas
Nov 22 '18 at 18:53
yeah perhaps make it a class or a struct with all these types and then return struct_name.value or class_object.value as it is hetrogenous (combination of types) hence we use object or struct_name as class identifiers to fetch concrete types
– Himanshu Ahuja
Nov 22 '18 at 18:57
yeah perhaps make it a class or a struct with all these types and then return struct_name.value or class_object.value as it is hetrogenous (combination of types) hence we use object or struct_name as class identifiers to fetch concrete types
– Himanshu Ahuja
Nov 22 '18 at 18:57
|
show 1 more comment
2 Answers
2
active
oldest
votes
You should swap the parameter String type
to Class<T> type
and your return type to T
. You have to define a generic parameter right before the return value, so the signature will be as follows: public static <T> T askln(String text, Class<T> type)
.
To make your compiler happy, you probably should have the getXXX()
methods return Object and casting it while returning to T
.
Could that used with a primitive type (sayfloat
), or only with an object (sayFloat
)?
– handras
Nov 22 '18 at 19:16
this does not work with primitive types
– László Stahorszki
Nov 22 '18 at 19:21
add a comment |
Another way would be to have a function as a parameter to the method, this solution is based on that since you get the value from the user the input can be handle as a string and then we need a function that converts a string to the desired type.
public static <R> R askln(String text, Function<String, R> function){
System.out.print(text);
String str = getValue();
return function.apply(str);
}
An example for a double
Function<String, Double> f = (Double::parseDouble);
Double d = askln("Type a number ", f);
and an integer
Function<String, Integer> f2 = (Integer::parseInt);
Integer i = askln("Type an integer", f2);
You can then have different functions defined for converting to each supported type.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436707%2fmethod-that-returns-any-type-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You should swap the parameter String type
to Class<T> type
and your return type to T
. You have to define a generic parameter right before the return value, so the signature will be as follows: public static <T> T askln(String text, Class<T> type)
.
To make your compiler happy, you probably should have the getXXX()
methods return Object and casting it while returning to T
.
Could that used with a primitive type (sayfloat
), or only with an object (sayFloat
)?
– handras
Nov 22 '18 at 19:16
this does not work with primitive types
– László Stahorszki
Nov 22 '18 at 19:21
add a comment |
You should swap the parameter String type
to Class<T> type
and your return type to T
. You have to define a generic parameter right before the return value, so the signature will be as follows: public static <T> T askln(String text, Class<T> type)
.
To make your compiler happy, you probably should have the getXXX()
methods return Object and casting it while returning to T
.
Could that used with a primitive type (sayfloat
), or only with an object (sayFloat
)?
– handras
Nov 22 '18 at 19:16
this does not work with primitive types
– László Stahorszki
Nov 22 '18 at 19:21
add a comment |
You should swap the parameter String type
to Class<T> type
and your return type to T
. You have to define a generic parameter right before the return value, so the signature will be as follows: public static <T> T askln(String text, Class<T> type)
.
To make your compiler happy, you probably should have the getXXX()
methods return Object and casting it while returning to T
.
You should swap the parameter String type
to Class<T> type
and your return type to T
. You have to define a generic parameter right before the return value, so the signature will be as follows: public static <T> T askln(String text, Class<T> type)
.
To make your compiler happy, you probably should have the getXXX()
methods return Object and casting it while returning to T
.
answered Nov 22 '18 at 18:57


László StahorszkiLászló Stahorszki
351211
351211
Could that used with a primitive type (sayfloat
), or only with an object (sayFloat
)?
– handras
Nov 22 '18 at 19:16
this does not work with primitive types
– László Stahorszki
Nov 22 '18 at 19:21
add a comment |
Could that used with a primitive type (sayfloat
), or only with an object (sayFloat
)?
– handras
Nov 22 '18 at 19:16
this does not work with primitive types
– László Stahorszki
Nov 22 '18 at 19:21
Could that used with a primitive type (say
float
), or only with an object (say Float
)?– handras
Nov 22 '18 at 19:16
Could that used with a primitive type (say
float
), or only with an object (say Float
)?– handras
Nov 22 '18 at 19:16
this does not work with primitive types
– László Stahorszki
Nov 22 '18 at 19:21
this does not work with primitive types
– László Stahorszki
Nov 22 '18 at 19:21
add a comment |
Another way would be to have a function as a parameter to the method, this solution is based on that since you get the value from the user the input can be handle as a string and then we need a function that converts a string to the desired type.
public static <R> R askln(String text, Function<String, R> function){
System.out.print(text);
String str = getValue();
return function.apply(str);
}
An example for a double
Function<String, Double> f = (Double::parseDouble);
Double d = askln("Type a number ", f);
and an integer
Function<String, Integer> f2 = (Integer::parseInt);
Integer i = askln("Type an integer", f2);
You can then have different functions defined for converting to each supported type.
add a comment |
Another way would be to have a function as a parameter to the method, this solution is based on that since you get the value from the user the input can be handle as a string and then we need a function that converts a string to the desired type.
public static <R> R askln(String text, Function<String, R> function){
System.out.print(text);
String str = getValue();
return function.apply(str);
}
An example for a double
Function<String, Double> f = (Double::parseDouble);
Double d = askln("Type a number ", f);
and an integer
Function<String, Integer> f2 = (Integer::parseInt);
Integer i = askln("Type an integer", f2);
You can then have different functions defined for converting to each supported type.
add a comment |
Another way would be to have a function as a parameter to the method, this solution is based on that since you get the value from the user the input can be handle as a string and then we need a function that converts a string to the desired type.
public static <R> R askln(String text, Function<String, R> function){
System.out.print(text);
String str = getValue();
return function.apply(str);
}
An example for a double
Function<String, Double> f = (Double::parseDouble);
Double d = askln("Type a number ", f);
and an integer
Function<String, Integer> f2 = (Integer::parseInt);
Integer i = askln("Type an integer", f2);
You can then have different functions defined for converting to each supported type.
Another way would be to have a function as a parameter to the method, this solution is based on that since you get the value from the user the input can be handle as a string and then we need a function that converts a string to the desired type.
public static <R> R askln(String text, Function<String, R> function){
System.out.print(text);
String str = getValue();
return function.apply(str);
}
An example for a double
Function<String, Double> f = (Double::parseDouble);
Double d = askln("Type a number ", f);
and an integer
Function<String, Integer> f2 = (Integer::parseInt);
Integer i = askln("Type an integer", f2);
You can then have different functions defined for converting to each supported type.
answered Nov 22 '18 at 19:45
Joakim DanielsonJoakim Danielson
7,9343724
7,9343724
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436707%2fmethod-that-returns-any-type-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6VZd,e1 khFvL 2RShftfn9RyTB,fJrrk,ZcsR0bFBvu47j
3
public static Object
?– Boris the Spider
Nov 22 '18 at 18:52
1
How do I compare strings in Java?
– luk2302
Nov 22 '18 at 18:53
1
Don't compare strings like this. In general, use
equals
; but in this case, use a switch.– Andy Turner
Nov 22 '18 at 18:53
1
I think you might do this with generics. Java is much stronger typed than other languages because having an unknown return type leads to bugs. Such a thing is frowned upon.
– K.Nicholas
Nov 22 '18 at 18:53
yeah perhaps make it a class or a struct with all these types and then return struct_name.value or class_object.value as it is hetrogenous (combination of types) hence we use object or struct_name as class identifiers to fetch concrete types
– Himanshu Ahuja
Nov 22 '18 at 18:57