How to conditionally convert a field to date if it's a string in MongoDB?
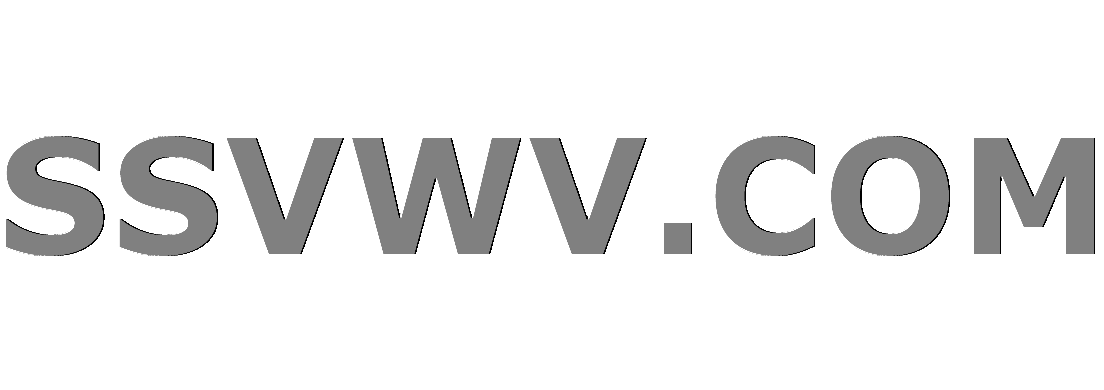
Multi tool use
While creating a query, I realized that the app I have inherited have a collection with a timestamp
field containing either a string
or an ISODate
value.
So, this aggregation stage :
{
"$addFields": {
"timestamp": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"minTimestamp": {
"$dateFromString": {
"dateString": "2016-01-01"
}
},
"maxTimestamp": {
"$dateFromString": {
"dateString": "2017-01-01"
}
}
}
}
produces the error : $dateFromString requires that 'dateString' be a string, found: date
on certain documents.
Obviously, the logical answer would be to convert all field values into ISODate
, however this behemoth of a system seem to save inconsistent values being set for that field, and it is not possible for me to guarantee the type in advance.
Is there a way to conditionally convert the field to ISODate
?
The app is running on MongoDB 3.6.4.
mongodb aggregation-framework
add a comment |
While creating a query, I realized that the app I have inherited have a collection with a timestamp
field containing either a string
or an ISODate
value.
So, this aggregation stage :
{
"$addFields": {
"timestamp": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"minTimestamp": {
"$dateFromString": {
"dateString": "2016-01-01"
}
},
"maxTimestamp": {
"$dateFromString": {
"dateString": "2017-01-01"
}
}
}
}
produces the error : $dateFromString requires that 'dateString' be a string, found: date
on certain documents.
Obviously, the logical answer would be to convert all field values into ISODate
, however this behemoth of a system seem to save inconsistent values being set for that field, and it is not possible for me to guarantee the type in advance.
Is there a way to conditionally convert the field to ISODate
?
The app is running on MongoDB 3.6.4.
mongodb aggregation-framework
add a comment |
While creating a query, I realized that the app I have inherited have a collection with a timestamp
field containing either a string
or an ISODate
value.
So, this aggregation stage :
{
"$addFields": {
"timestamp": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"minTimestamp": {
"$dateFromString": {
"dateString": "2016-01-01"
}
},
"maxTimestamp": {
"$dateFromString": {
"dateString": "2017-01-01"
}
}
}
}
produces the error : $dateFromString requires that 'dateString' be a string, found: date
on certain documents.
Obviously, the logical answer would be to convert all field values into ISODate
, however this behemoth of a system seem to save inconsistent values being set for that field, and it is not possible for me to guarantee the type in advance.
Is there a way to conditionally convert the field to ISODate
?
The app is running on MongoDB 3.6.4.
mongodb aggregation-framework
While creating a query, I realized that the app I have inherited have a collection with a timestamp
field containing either a string
or an ISODate
value.
So, this aggregation stage :
{
"$addFields": {
"timestamp": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"minTimestamp": {
"$dateFromString": {
"dateString": "2016-01-01"
}
},
"maxTimestamp": {
"$dateFromString": {
"dateString": "2017-01-01"
}
}
}
}
produces the error : $dateFromString requires that 'dateString' be a string, found: date
on certain documents.
Obviously, the logical answer would be to convert all field values into ISODate
, however this behemoth of a system seem to save inconsistent values being set for that field, and it is not possible for me to guarantee the type in advance.
Is there a way to conditionally convert the field to ISODate
?
The app is running on MongoDB 3.6.4.
mongodb aggregation-framework
mongodb aggregation-framework
edited Nov 22 '18 at 20:28
Neil Lunn
97.7k23174184
97.7k23174184
asked Nov 22 '18 at 18:42
Yanick RochonYanick Rochon
34.1k1989158
34.1k1989158
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
If some of the fields are actually BSON Dates, then you probably want to leave them alone and output them as is. For this you can use $type
along with a $cond
expression:
{ "$addFields": {
"timestamp": {
"$cond": {
"if": { "$eq": [{ "$type": "$activity.timestamp" }, "string" ] },
"then": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"else": "$activity.timestamp"
}
}
}}
That's okay from MongoDB 3.4 and upwards which is when $type
was added.
Noting for users of MongoDB 4.0 and above, the $convert
operator actually has built in error handling branching:
{ "$addFields": {
"timestamp": {
"$convert": {
"input": "$activity.timestamp",
"to": "date",
"onError": "Neither date or string"
}
}
}}
The onError
can be any expression and is returned in the instances where the conversion was invalid. Finding a BSON Date is not actually an error and errors would only occur for an invalid numeric or string value or different type which did not support conversion.
If you know for certain that the data is always either a BSON Date or a valid string for conversion then there is the $toDate
helper which is basically a wrapper for $convert
without the onError
handling:
{ "$addFields": {
"timestamp": { "$toDate": "$activity.timestamp" }
}}
So some data scrubbing and/or query conditions can often be combined with that for a more streamlined coding experience.
A note on $dateToString usage
Within the question the $dateToString
is also being used to convert "static values" from strings into BSON Date. This is not a good idea.
Running functional code within a server expression which is more cleanly expressed in natural language code is not and never really was a good practice. As part of the general MongoDB philosophy, the parts that really should and can be expressed in that language should be done so that way.
For JavaScript simple Date
objects are serialized as BSON Date on submission to the server anyway:
"minTimestamp": new Date("2016-01-01")
Since the value of the "string" is external then it does not need server side expression to manipulate it. Just like issuing a query you cast such types before you submit to the server and not afterwards.
The same concept is true of all language implementations, as all languages have a "Date" type which the implemented driver understands and correctly serializes as a BSON Date anyway.
Conversion
With all of that said, the general "best practice" here is of course to actually convert the data. "Behemoth" or not, it's really only making matters worse to rely on conversion of the data at run time. This is even more so if your actual intention in such run-time conversion is to use the BSON Dates in further output processing, rather than just a pretty output.
Mileage varies on which approach works the best, but the basics are to either iterate the collection and update values in place, and the $type
"query" operator can help with selection here:
// Presuming date strings in"yyyy-mm-dd" format
var batch = ;
db.collection.find({ "activity.timestamp": { "$type": "string" } }).forEach(d => {
batch.push({
"updateOne": {
"filter": { "_id": d._id },
"update": { "$set": { "activity.timestamp": new Date(d.activity.timestamp) } }
}
});
if (batch.length >= 1000) {
db.collection.bulkWrite(batch);
batch = ;
}
})
if (batch.length > 0) {
db.collection.bulkWrite(batch);
batch = ;
}
Or run the aggregation with $out
to a new collection if constraints allow this:
{ "$addFields": {
"activity": {
"timestamp": {
"$cond": {
"if": { "$eq": [{ "$type": "$activity.timestamp" }, "string" ] },
"then": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"else": "$activity.timestamp"
}
}
}
}},
{ "$out": "newcollection" }
Any of the above aggregation methods shown can be used here where they are supported, but just showing this in example. Note also the $addFields
does allow the nested object syntax since output is "merged" into the existing document structure.
Even on a production system you could always output to a new collection and then drop and rename with minimal downtime. The main constraint here would actually be index rebuilding, which would take significantly longer than a collection rename.
Thank you for the thorough explanation!
– Yanick Rochon
Nov 22 '18 at 23:26
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436629%2fhow-to-conditionally-convert-a-field-to-date-if-its-a-string-in-mongodb%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If some of the fields are actually BSON Dates, then you probably want to leave them alone and output them as is. For this you can use $type
along with a $cond
expression:
{ "$addFields": {
"timestamp": {
"$cond": {
"if": { "$eq": [{ "$type": "$activity.timestamp" }, "string" ] },
"then": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"else": "$activity.timestamp"
}
}
}}
That's okay from MongoDB 3.4 and upwards which is when $type
was added.
Noting for users of MongoDB 4.0 and above, the $convert
operator actually has built in error handling branching:
{ "$addFields": {
"timestamp": {
"$convert": {
"input": "$activity.timestamp",
"to": "date",
"onError": "Neither date or string"
}
}
}}
The onError
can be any expression and is returned in the instances where the conversion was invalid. Finding a BSON Date is not actually an error and errors would only occur for an invalid numeric or string value or different type which did not support conversion.
If you know for certain that the data is always either a BSON Date or a valid string for conversion then there is the $toDate
helper which is basically a wrapper for $convert
without the onError
handling:
{ "$addFields": {
"timestamp": { "$toDate": "$activity.timestamp" }
}}
So some data scrubbing and/or query conditions can often be combined with that for a more streamlined coding experience.
A note on $dateToString usage
Within the question the $dateToString
is also being used to convert "static values" from strings into BSON Date. This is not a good idea.
Running functional code within a server expression which is more cleanly expressed in natural language code is not and never really was a good practice. As part of the general MongoDB philosophy, the parts that really should and can be expressed in that language should be done so that way.
For JavaScript simple Date
objects are serialized as BSON Date on submission to the server anyway:
"minTimestamp": new Date("2016-01-01")
Since the value of the "string" is external then it does not need server side expression to manipulate it. Just like issuing a query you cast such types before you submit to the server and not afterwards.
The same concept is true of all language implementations, as all languages have a "Date" type which the implemented driver understands and correctly serializes as a BSON Date anyway.
Conversion
With all of that said, the general "best practice" here is of course to actually convert the data. "Behemoth" or not, it's really only making matters worse to rely on conversion of the data at run time. This is even more so if your actual intention in such run-time conversion is to use the BSON Dates in further output processing, rather than just a pretty output.
Mileage varies on which approach works the best, but the basics are to either iterate the collection and update values in place, and the $type
"query" operator can help with selection here:
// Presuming date strings in"yyyy-mm-dd" format
var batch = ;
db.collection.find({ "activity.timestamp": { "$type": "string" } }).forEach(d => {
batch.push({
"updateOne": {
"filter": { "_id": d._id },
"update": { "$set": { "activity.timestamp": new Date(d.activity.timestamp) } }
}
});
if (batch.length >= 1000) {
db.collection.bulkWrite(batch);
batch = ;
}
})
if (batch.length > 0) {
db.collection.bulkWrite(batch);
batch = ;
}
Or run the aggregation with $out
to a new collection if constraints allow this:
{ "$addFields": {
"activity": {
"timestamp": {
"$cond": {
"if": { "$eq": [{ "$type": "$activity.timestamp" }, "string" ] },
"then": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"else": "$activity.timestamp"
}
}
}
}},
{ "$out": "newcollection" }
Any of the above aggregation methods shown can be used here where they are supported, but just showing this in example. Note also the $addFields
does allow the nested object syntax since output is "merged" into the existing document structure.
Even on a production system you could always output to a new collection and then drop and rename with minimal downtime. The main constraint here would actually be index rebuilding, which would take significantly longer than a collection rename.
Thank you for the thorough explanation!
– Yanick Rochon
Nov 22 '18 at 23:26
add a comment |
If some of the fields are actually BSON Dates, then you probably want to leave them alone and output them as is. For this you can use $type
along with a $cond
expression:
{ "$addFields": {
"timestamp": {
"$cond": {
"if": { "$eq": [{ "$type": "$activity.timestamp" }, "string" ] },
"then": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"else": "$activity.timestamp"
}
}
}}
That's okay from MongoDB 3.4 and upwards which is when $type
was added.
Noting for users of MongoDB 4.0 and above, the $convert
operator actually has built in error handling branching:
{ "$addFields": {
"timestamp": {
"$convert": {
"input": "$activity.timestamp",
"to": "date",
"onError": "Neither date or string"
}
}
}}
The onError
can be any expression and is returned in the instances where the conversion was invalid. Finding a BSON Date is not actually an error and errors would only occur for an invalid numeric or string value or different type which did not support conversion.
If you know for certain that the data is always either a BSON Date or a valid string for conversion then there is the $toDate
helper which is basically a wrapper for $convert
without the onError
handling:
{ "$addFields": {
"timestamp": { "$toDate": "$activity.timestamp" }
}}
So some data scrubbing and/or query conditions can often be combined with that for a more streamlined coding experience.
A note on $dateToString usage
Within the question the $dateToString
is also being used to convert "static values" from strings into BSON Date. This is not a good idea.
Running functional code within a server expression which is more cleanly expressed in natural language code is not and never really was a good practice. As part of the general MongoDB philosophy, the parts that really should and can be expressed in that language should be done so that way.
For JavaScript simple Date
objects are serialized as BSON Date on submission to the server anyway:
"minTimestamp": new Date("2016-01-01")
Since the value of the "string" is external then it does not need server side expression to manipulate it. Just like issuing a query you cast such types before you submit to the server and not afterwards.
The same concept is true of all language implementations, as all languages have a "Date" type which the implemented driver understands and correctly serializes as a BSON Date anyway.
Conversion
With all of that said, the general "best practice" here is of course to actually convert the data. "Behemoth" or not, it's really only making matters worse to rely on conversion of the data at run time. This is even more so if your actual intention in such run-time conversion is to use the BSON Dates in further output processing, rather than just a pretty output.
Mileage varies on which approach works the best, but the basics are to either iterate the collection and update values in place, and the $type
"query" operator can help with selection here:
// Presuming date strings in"yyyy-mm-dd" format
var batch = ;
db.collection.find({ "activity.timestamp": { "$type": "string" } }).forEach(d => {
batch.push({
"updateOne": {
"filter": { "_id": d._id },
"update": { "$set": { "activity.timestamp": new Date(d.activity.timestamp) } }
}
});
if (batch.length >= 1000) {
db.collection.bulkWrite(batch);
batch = ;
}
})
if (batch.length > 0) {
db.collection.bulkWrite(batch);
batch = ;
}
Or run the aggregation with $out
to a new collection if constraints allow this:
{ "$addFields": {
"activity": {
"timestamp": {
"$cond": {
"if": { "$eq": [{ "$type": "$activity.timestamp" }, "string" ] },
"then": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"else": "$activity.timestamp"
}
}
}
}},
{ "$out": "newcollection" }
Any of the above aggregation methods shown can be used here where they are supported, but just showing this in example. Note also the $addFields
does allow the nested object syntax since output is "merged" into the existing document structure.
Even on a production system you could always output to a new collection and then drop and rename with minimal downtime. The main constraint here would actually be index rebuilding, which would take significantly longer than a collection rename.
Thank you for the thorough explanation!
– Yanick Rochon
Nov 22 '18 at 23:26
add a comment |
If some of the fields are actually BSON Dates, then you probably want to leave them alone and output them as is. For this you can use $type
along with a $cond
expression:
{ "$addFields": {
"timestamp": {
"$cond": {
"if": { "$eq": [{ "$type": "$activity.timestamp" }, "string" ] },
"then": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"else": "$activity.timestamp"
}
}
}}
That's okay from MongoDB 3.4 and upwards which is when $type
was added.
Noting for users of MongoDB 4.0 and above, the $convert
operator actually has built in error handling branching:
{ "$addFields": {
"timestamp": {
"$convert": {
"input": "$activity.timestamp",
"to": "date",
"onError": "Neither date or string"
}
}
}}
The onError
can be any expression and is returned in the instances where the conversion was invalid. Finding a BSON Date is not actually an error and errors would only occur for an invalid numeric or string value or different type which did not support conversion.
If you know for certain that the data is always either a BSON Date or a valid string for conversion then there is the $toDate
helper which is basically a wrapper for $convert
without the onError
handling:
{ "$addFields": {
"timestamp": { "$toDate": "$activity.timestamp" }
}}
So some data scrubbing and/or query conditions can often be combined with that for a more streamlined coding experience.
A note on $dateToString usage
Within the question the $dateToString
is also being used to convert "static values" from strings into BSON Date. This is not a good idea.
Running functional code within a server expression which is more cleanly expressed in natural language code is not and never really was a good practice. As part of the general MongoDB philosophy, the parts that really should and can be expressed in that language should be done so that way.
For JavaScript simple Date
objects are serialized as BSON Date on submission to the server anyway:
"minTimestamp": new Date("2016-01-01")
Since the value of the "string" is external then it does not need server side expression to manipulate it. Just like issuing a query you cast such types before you submit to the server and not afterwards.
The same concept is true of all language implementations, as all languages have a "Date" type which the implemented driver understands and correctly serializes as a BSON Date anyway.
Conversion
With all of that said, the general "best practice" here is of course to actually convert the data. "Behemoth" or not, it's really only making matters worse to rely on conversion of the data at run time. This is even more so if your actual intention in such run-time conversion is to use the BSON Dates in further output processing, rather than just a pretty output.
Mileage varies on which approach works the best, but the basics are to either iterate the collection and update values in place, and the $type
"query" operator can help with selection here:
// Presuming date strings in"yyyy-mm-dd" format
var batch = ;
db.collection.find({ "activity.timestamp": { "$type": "string" } }).forEach(d => {
batch.push({
"updateOne": {
"filter": { "_id": d._id },
"update": { "$set": { "activity.timestamp": new Date(d.activity.timestamp) } }
}
});
if (batch.length >= 1000) {
db.collection.bulkWrite(batch);
batch = ;
}
})
if (batch.length > 0) {
db.collection.bulkWrite(batch);
batch = ;
}
Or run the aggregation with $out
to a new collection if constraints allow this:
{ "$addFields": {
"activity": {
"timestamp": {
"$cond": {
"if": { "$eq": [{ "$type": "$activity.timestamp" }, "string" ] },
"then": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"else": "$activity.timestamp"
}
}
}
}},
{ "$out": "newcollection" }
Any of the above aggregation methods shown can be used here where they are supported, but just showing this in example. Note also the $addFields
does allow the nested object syntax since output is "merged" into the existing document structure.
Even on a production system you could always output to a new collection and then drop and rename with minimal downtime. The main constraint here would actually be index rebuilding, which would take significantly longer than a collection rename.
If some of the fields are actually BSON Dates, then you probably want to leave them alone and output them as is. For this you can use $type
along with a $cond
expression:
{ "$addFields": {
"timestamp": {
"$cond": {
"if": { "$eq": [{ "$type": "$activity.timestamp" }, "string" ] },
"then": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"else": "$activity.timestamp"
}
}
}}
That's okay from MongoDB 3.4 and upwards which is when $type
was added.
Noting for users of MongoDB 4.0 and above, the $convert
operator actually has built in error handling branching:
{ "$addFields": {
"timestamp": {
"$convert": {
"input": "$activity.timestamp",
"to": "date",
"onError": "Neither date or string"
}
}
}}
The onError
can be any expression and is returned in the instances where the conversion was invalid. Finding a BSON Date is not actually an error and errors would only occur for an invalid numeric or string value or different type which did not support conversion.
If you know for certain that the data is always either a BSON Date or a valid string for conversion then there is the $toDate
helper which is basically a wrapper for $convert
without the onError
handling:
{ "$addFields": {
"timestamp": { "$toDate": "$activity.timestamp" }
}}
So some data scrubbing and/or query conditions can often be combined with that for a more streamlined coding experience.
A note on $dateToString usage
Within the question the $dateToString
is also being used to convert "static values" from strings into BSON Date. This is not a good idea.
Running functional code within a server expression which is more cleanly expressed in natural language code is not and never really was a good practice. As part of the general MongoDB philosophy, the parts that really should and can be expressed in that language should be done so that way.
For JavaScript simple Date
objects are serialized as BSON Date on submission to the server anyway:
"minTimestamp": new Date("2016-01-01")
Since the value of the "string" is external then it does not need server side expression to manipulate it. Just like issuing a query you cast such types before you submit to the server and not afterwards.
The same concept is true of all language implementations, as all languages have a "Date" type which the implemented driver understands and correctly serializes as a BSON Date anyway.
Conversion
With all of that said, the general "best practice" here is of course to actually convert the data. "Behemoth" or not, it's really only making matters worse to rely on conversion of the data at run time. This is even more so if your actual intention in such run-time conversion is to use the BSON Dates in further output processing, rather than just a pretty output.
Mileage varies on which approach works the best, but the basics are to either iterate the collection and update values in place, and the $type
"query" operator can help with selection here:
// Presuming date strings in"yyyy-mm-dd" format
var batch = ;
db.collection.find({ "activity.timestamp": { "$type": "string" } }).forEach(d => {
batch.push({
"updateOne": {
"filter": { "_id": d._id },
"update": { "$set": { "activity.timestamp": new Date(d.activity.timestamp) } }
}
});
if (batch.length >= 1000) {
db.collection.bulkWrite(batch);
batch = ;
}
})
if (batch.length > 0) {
db.collection.bulkWrite(batch);
batch = ;
}
Or run the aggregation with $out
to a new collection if constraints allow this:
{ "$addFields": {
"activity": {
"timestamp": {
"$cond": {
"if": { "$eq": [{ "$type": "$activity.timestamp" }, "string" ] },
"then": {
"$dateFromString": {
"dateString": "$activity.timestamp"
}
},
"else": "$activity.timestamp"
}
}
}
}},
{ "$out": "newcollection" }
Any of the above aggregation methods shown can be used here where they are supported, but just showing this in example. Note also the $addFields
does allow the nested object syntax since output is "merged" into the existing document structure.
Even on a production system you could always output to a new collection and then drop and rename with minimal downtime. The main constraint here would actually be index rebuilding, which would take significantly longer than a collection rename.
edited Nov 22 '18 at 21:30
answered Nov 22 '18 at 20:28
Neil LunnNeil Lunn
97.7k23174184
97.7k23174184
Thank you for the thorough explanation!
– Yanick Rochon
Nov 22 '18 at 23:26
add a comment |
Thank you for the thorough explanation!
– Yanick Rochon
Nov 22 '18 at 23:26
Thank you for the thorough explanation!
– Yanick Rochon
Nov 22 '18 at 23:26
Thank you for the thorough explanation!
– Yanick Rochon
Nov 22 '18 at 23:26
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436629%2fhow-to-conditionally-convert-a-field-to-date-if-its-a-string-in-mongodb%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NhU8jP6gw viefrb