What can I do in this code to prompt an error when a negative number is used?
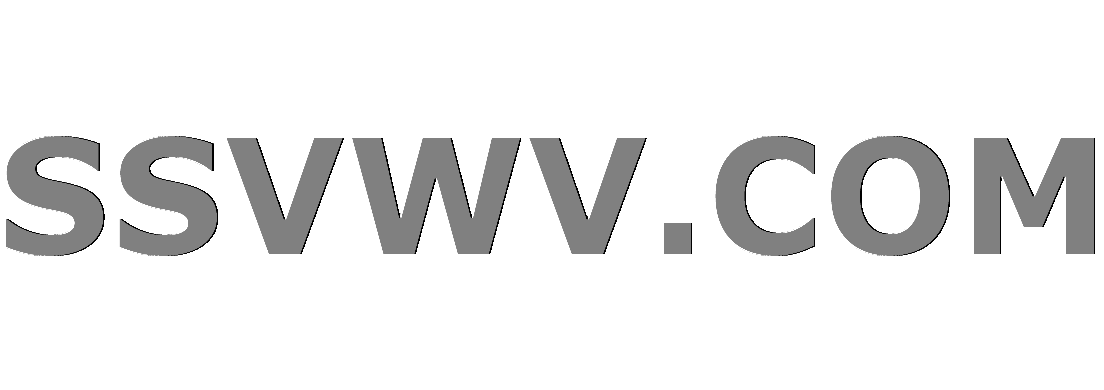
Multi tool use
up vote
3
down vote
favorite
i was wondering what can I do to prompt an error when I input a negative number. I used a for loop for this program. What this program does, or at least its supposed to do, is to output the factor of any given number (if positive). But I am not sure how to make my code to prompt an error or at least keep asking for a number if the number that input is less or equal to 0.
I used the variable n as the number to input by the user.
I am really new to programming and I am eager to finish this program as soon as possible. Can you please assist?
#include <iostream>
#include <math.h>
#include <stdlib.h>
using namespace std;
int main()
{
double n;
cout << "Welcome to this program... Hope you like it" << flush << endl;
do
{
int i, fact = 1, n;
cout << "Please enter a value for the variable " "n: ";
cin >> n;
for (i = 1; i <= n; i++) {
fact = fact * i;
}
cout << "Factorial of " << n << " is: " << fact << endl;
cout << " Thanks for using this program... hope you liked it!" << endl;
} while (n >= 0);
return 0;
}
c++ visual-studio-2010 visual-c++
add a comment |
up vote
3
down vote
favorite
i was wondering what can I do to prompt an error when I input a negative number. I used a for loop for this program. What this program does, or at least its supposed to do, is to output the factor of any given number (if positive). But I am not sure how to make my code to prompt an error or at least keep asking for a number if the number that input is less or equal to 0.
I used the variable n as the number to input by the user.
I am really new to programming and I am eager to finish this program as soon as possible. Can you please assist?
#include <iostream>
#include <math.h>
#include <stdlib.h>
using namespace std;
int main()
{
double n;
cout << "Welcome to this program... Hope you like it" << flush << endl;
do
{
int i, fact = 1, n;
cout << "Please enter a value for the variable " "n: ";
cin >> n;
for (i = 1; i <= n; i++) {
fact = fact * i;
}
cout << "Factorial of " << n << " is: " << fact << endl;
cout << " Thanks for using this program... hope you liked it!" << endl;
} while (n >= 0);
return 0;
}
c++ visual-studio-2010 visual-c++
6
Wait. You have 2n
s. Best not to do this. It's legal since they are in different scopes, but it's source of confusion.
– user4581301
Nov 20 at 0:41
Consider drawing a picture of what you want the code to do. Then draw a picture of what the code does. Them move the code around until the picture of what the code does matches what the code should do.
– user4581301
Nov 20 at 0:46
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
i was wondering what can I do to prompt an error when I input a negative number. I used a for loop for this program. What this program does, or at least its supposed to do, is to output the factor of any given number (if positive). But I am not sure how to make my code to prompt an error or at least keep asking for a number if the number that input is less or equal to 0.
I used the variable n as the number to input by the user.
I am really new to programming and I am eager to finish this program as soon as possible. Can you please assist?
#include <iostream>
#include <math.h>
#include <stdlib.h>
using namespace std;
int main()
{
double n;
cout << "Welcome to this program... Hope you like it" << flush << endl;
do
{
int i, fact = 1, n;
cout << "Please enter a value for the variable " "n: ";
cin >> n;
for (i = 1; i <= n; i++) {
fact = fact * i;
}
cout << "Factorial of " << n << " is: " << fact << endl;
cout << " Thanks for using this program... hope you liked it!" << endl;
} while (n >= 0);
return 0;
}
c++ visual-studio-2010 visual-c++
i was wondering what can I do to prompt an error when I input a negative number. I used a for loop for this program. What this program does, or at least its supposed to do, is to output the factor of any given number (if positive). But I am not sure how to make my code to prompt an error or at least keep asking for a number if the number that input is less or equal to 0.
I used the variable n as the number to input by the user.
I am really new to programming and I am eager to finish this program as soon as possible. Can you please assist?
#include <iostream>
#include <math.h>
#include <stdlib.h>
using namespace std;
int main()
{
double n;
cout << "Welcome to this program... Hope you like it" << flush << endl;
do
{
int i, fact = 1, n;
cout << "Please enter a value for the variable " "n: ";
cin >> n;
for (i = 1; i <= n; i++) {
fact = fact * i;
}
cout << "Factorial of " << n << " is: " << fact << endl;
cout << " Thanks for using this program... hope you liked it!" << endl;
} while (n >= 0);
return 0;
}
c++ visual-studio-2010 visual-c++
c++ visual-studio-2010 visual-c++
asked Nov 20 at 0:35


Diego Montilla
161
161
6
Wait. You have 2n
s. Best not to do this. It's legal since they are in different scopes, but it's source of confusion.
– user4581301
Nov 20 at 0:41
Consider drawing a picture of what you want the code to do. Then draw a picture of what the code does. Them move the code around until the picture of what the code does matches what the code should do.
– user4581301
Nov 20 at 0:46
add a comment |
6
Wait. You have 2n
s. Best not to do this. It's legal since they are in different scopes, but it's source of confusion.
– user4581301
Nov 20 at 0:41
Consider drawing a picture of what you want the code to do. Then draw a picture of what the code does. Them move the code around until the picture of what the code does matches what the code should do.
– user4581301
Nov 20 at 0:46
6
6
Wait. You have 2
n
s. Best not to do this. It's legal since they are in different scopes, but it's source of confusion.– user4581301
Nov 20 at 0:41
Wait. You have 2
n
s. Best not to do this. It's legal since they are in different scopes, but it's source of confusion.– user4581301
Nov 20 at 0:41
Consider drawing a picture of what you want the code to do. Then draw a picture of what the code does. Them move the code around until the picture of what the code does matches what the code should do.
– user4581301
Nov 20 at 0:46
Consider drawing a picture of what you want the code to do. Then draw a picture of what the code does. Them move the code around until the picture of what the code does matches what the code should do.
– user4581301
Nov 20 at 0:46
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
Commens in the code:
#include <iostream>
// it's best not to do using namespace std. It's a huge namespace
int main()
{
std::cout << "Welcome to this program... Hope you like itn";
do
{
int i, fact = 1, n;
std::cout << "Please enter a value for the variable " "n: ";
std::cin >> n;
// if std::cin is in a failed state then break out of the loop.
if (std::cin.fail()) break;
// added check if n<0. if n<0 ptint error on stderr
if (n<0) {
std::clog << "Error: you must supply a number equal to or greater than zeron";
} else { // if n>=0
for (i = 1; i <= n; i++) {
fact = fact * i;
}
std::cout << "Factorial of " << n << " is: " << fact << "n";
}
} while (true); // <- now always true
std::cout << "nThanks for using this program... hope you liked it!n";
return 0;
}
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Commens in the code:
#include <iostream>
// it's best not to do using namespace std. It's a huge namespace
int main()
{
std::cout << "Welcome to this program... Hope you like itn";
do
{
int i, fact = 1, n;
std::cout << "Please enter a value for the variable " "n: ";
std::cin >> n;
// if std::cin is in a failed state then break out of the loop.
if (std::cin.fail()) break;
// added check if n<0. if n<0 ptint error on stderr
if (n<0) {
std::clog << "Error: you must supply a number equal to or greater than zeron";
} else { // if n>=0
for (i = 1; i <= n; i++) {
fact = fact * i;
}
std::cout << "Factorial of " << n << " is: " << fact << "n";
}
} while (true); // <- now always true
std::cout << "nThanks for using this program... hope you liked it!n";
return 0;
}
add a comment |
up vote
0
down vote
Commens in the code:
#include <iostream>
// it's best not to do using namespace std. It's a huge namespace
int main()
{
std::cout << "Welcome to this program... Hope you like itn";
do
{
int i, fact = 1, n;
std::cout << "Please enter a value for the variable " "n: ";
std::cin >> n;
// if std::cin is in a failed state then break out of the loop.
if (std::cin.fail()) break;
// added check if n<0. if n<0 ptint error on stderr
if (n<0) {
std::clog << "Error: you must supply a number equal to or greater than zeron";
} else { // if n>=0
for (i = 1; i <= n; i++) {
fact = fact * i;
}
std::cout << "Factorial of " << n << " is: " << fact << "n";
}
} while (true); // <- now always true
std::cout << "nThanks for using this program... hope you liked it!n";
return 0;
}
add a comment |
up vote
0
down vote
up vote
0
down vote
Commens in the code:
#include <iostream>
// it's best not to do using namespace std. It's a huge namespace
int main()
{
std::cout << "Welcome to this program... Hope you like itn";
do
{
int i, fact = 1, n;
std::cout << "Please enter a value for the variable " "n: ";
std::cin >> n;
// if std::cin is in a failed state then break out of the loop.
if (std::cin.fail()) break;
// added check if n<0. if n<0 ptint error on stderr
if (n<0) {
std::clog << "Error: you must supply a number equal to or greater than zeron";
} else { // if n>=0
for (i = 1; i <= n; i++) {
fact = fact * i;
}
std::cout << "Factorial of " << n << " is: " << fact << "n";
}
} while (true); // <- now always true
std::cout << "nThanks for using this program... hope you liked it!n";
return 0;
}
Commens in the code:
#include <iostream>
// it's best not to do using namespace std. It's a huge namespace
int main()
{
std::cout << "Welcome to this program... Hope you like itn";
do
{
int i, fact = 1, n;
std::cout << "Please enter a value for the variable " "n: ";
std::cin >> n;
// if std::cin is in a failed state then break out of the loop.
if (std::cin.fail()) break;
// added check if n<0. if n<0 ptint error on stderr
if (n<0) {
std::clog << "Error: you must supply a number equal to or greater than zeron";
} else { // if n>=0
for (i = 1; i <= n; i++) {
fact = fact * i;
}
std::cout << "Factorial of " << n << " is: " << fact << "n";
}
} while (true); // <- now always true
std::cout << "nThanks for using this program... hope you liked it!n";
return 0;
}
edited Nov 20 at 6:30
answered Nov 20 at 0:53


Ted Lyngmo
1,368214
1,368214
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384606%2fwhat-can-i-do-in-this-code-to-prompt-an-error-when-a-negative-number-is-used%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
lPUxwG92no wTZRWF,4 AyBXAz38R,F,Xj7P,kJup7
6
Wait. You have 2
n
s. Best not to do this. It's legal since they are in different scopes, but it's source of confusion.– user4581301
Nov 20 at 0:41
Consider drawing a picture of what you want the code to do. Then draw a picture of what the code does. Them move the code around until the picture of what the code does matches what the code should do.
– user4581301
Nov 20 at 0:46