How to typescript `this` when it is dynamic?
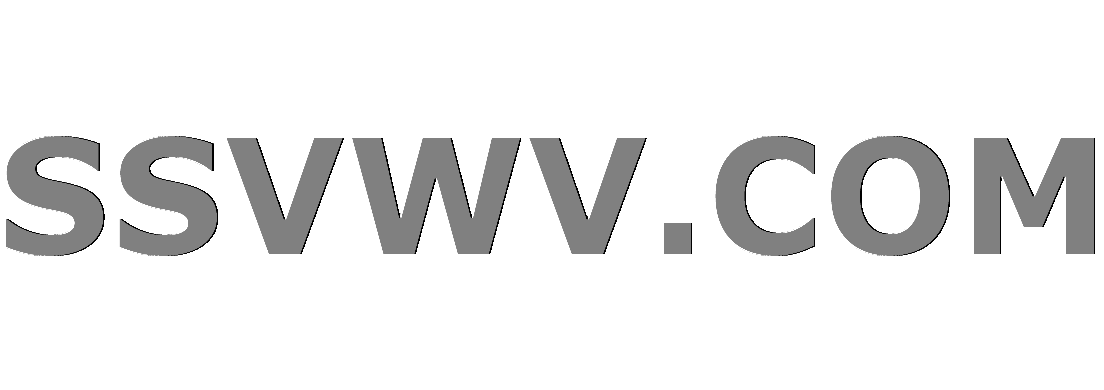
Multi tool use
up vote
1
down vote
favorite
I'm converting a series of JavaScript projects to typescript, and a common paradigm that I've used has been the following:
abstract class A {
static create (…args) {
return new this(…args)
}
/* a bunch of things */
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
/* a bunch of things */
}
class C extends A {
/* a bunch of things */
}
Which offers the compelling syntactic sugar of require('module').B.create()
.
I cannot figure out how to make this valid typescript.
Additional complexity, is that consumers of the package may also extend class A too, so I am not aware of all the extensions at the time of authorship.
With:
abstract class A {
static create<T extends typeof A>(this: T, ...args: any): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
I get the error:
[ts] Cannot create an instance of an abstract class. [2511]
With the following, that omits the abstract for debugging:
class A {
static create<T extends typeof A>(this: T, ...args: any): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
I get an error about the variable arguments:
[ts] Expected 0 arguments, but got 1 or more. [2556]
With:
type StaticThis<T> = { new(): T };
abstract class A {
static create<T extends typeof A>(this: StaticThis<T>, ...args: any): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
I solve the abstract error, but keep the arguments error:
[ts] Expected 0 arguments, but got 1 or more. [2556]
With:
interface Factory<T> {
new(...args: any): T
}
abstract class A {
static create<T extends A>(this: Factory<T>, ...args: any): T {
return new this(...args)
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
foo() {
}
}
class C extends A {
bar() {
}
}
It works as expected, however Visual Studio Code autocompletes B.create
to B.create(this)
, which may be a seperate bug.
typescript
add a comment |
up vote
1
down vote
favorite
I'm converting a series of JavaScript projects to typescript, and a common paradigm that I've used has been the following:
abstract class A {
static create (…args) {
return new this(…args)
}
/* a bunch of things */
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
/* a bunch of things */
}
class C extends A {
/* a bunch of things */
}
Which offers the compelling syntactic sugar of require('module').B.create()
.
I cannot figure out how to make this valid typescript.
Additional complexity, is that consumers of the package may also extend class A too, so I am not aware of all the extensions at the time of authorship.
With:
abstract class A {
static create<T extends typeof A>(this: T, ...args: any): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
I get the error:
[ts] Cannot create an instance of an abstract class. [2511]
With the following, that omits the abstract for debugging:
class A {
static create<T extends typeof A>(this: T, ...args: any): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
I get an error about the variable arguments:
[ts] Expected 0 arguments, but got 1 or more. [2556]
With:
type StaticThis<T> = { new(): T };
abstract class A {
static create<T extends typeof A>(this: StaticThis<T>, ...args: any): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
I solve the abstract error, but keep the arguments error:
[ts] Expected 0 arguments, but got 1 or more. [2556]
With:
interface Factory<T> {
new(...args: any): T
}
abstract class A {
static create<T extends A>(this: Factory<T>, ...args: any): T {
return new this(...args)
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
foo() {
}
}
class C extends A {
bar() {
}
}
It works as expected, however Visual Studio Code autocompletes B.create
to B.create(this)
, which may be a seperate bug.
typescript
1
Is this your issue?
– jcalz
Nov 20 at 1:13
@jcalz thank you, it seems so, want to post the typescript compatible code snippet in an answer so I can mark it as such.
– balupton
Nov 20 at 1:15
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm converting a series of JavaScript projects to typescript, and a common paradigm that I've used has been the following:
abstract class A {
static create (…args) {
return new this(…args)
}
/* a bunch of things */
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
/* a bunch of things */
}
class C extends A {
/* a bunch of things */
}
Which offers the compelling syntactic sugar of require('module').B.create()
.
I cannot figure out how to make this valid typescript.
Additional complexity, is that consumers of the package may also extend class A too, so I am not aware of all the extensions at the time of authorship.
With:
abstract class A {
static create<T extends typeof A>(this: T, ...args: any): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
I get the error:
[ts] Cannot create an instance of an abstract class. [2511]
With the following, that omits the abstract for debugging:
class A {
static create<T extends typeof A>(this: T, ...args: any): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
I get an error about the variable arguments:
[ts] Expected 0 arguments, but got 1 or more. [2556]
With:
type StaticThis<T> = { new(): T };
abstract class A {
static create<T extends typeof A>(this: StaticThis<T>, ...args: any): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
I solve the abstract error, but keep the arguments error:
[ts] Expected 0 arguments, but got 1 or more. [2556]
With:
interface Factory<T> {
new(...args: any): T
}
abstract class A {
static create<T extends A>(this: Factory<T>, ...args: any): T {
return new this(...args)
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
foo() {
}
}
class C extends A {
bar() {
}
}
It works as expected, however Visual Studio Code autocompletes B.create
to B.create(this)
, which may be a seperate bug.
typescript
I'm converting a series of JavaScript projects to typescript, and a common paradigm that I've used has been the following:
abstract class A {
static create (…args) {
return new this(…args)
}
/* a bunch of things */
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
/* a bunch of things */
}
class C extends A {
/* a bunch of things */
}
Which offers the compelling syntactic sugar of require('module').B.create()
.
I cannot figure out how to make this valid typescript.
Additional complexity, is that consumers of the package may also extend class A too, so I am not aware of all the extensions at the time of authorship.
With:
abstract class A {
static create<T extends typeof A>(this: T, ...args: any): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
I get the error:
[ts] Cannot create an instance of an abstract class. [2511]
With the following, that omits the abstract for debugging:
class A {
static create<T extends typeof A>(this: T, ...args: any): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
I get an error about the variable arguments:
[ts] Expected 0 arguments, but got 1 or more. [2556]
With:
type StaticThis<T> = { new(): T };
abstract class A {
static create<T extends typeof A>(this: StaticThis<T>, ...args: any): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
I solve the abstract error, but keep the arguments error:
[ts] Expected 0 arguments, but got 1 or more. [2556]
With:
interface Factory<T> {
new(...args: any): T
}
abstract class A {
static create<T extends A>(this: Factory<T>, ...args: any): T {
return new this(...args)
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
foo() {
}
}
class C extends A {
bar() {
}
}
It works as expected, however Visual Studio Code autocompletes B.create
to B.create(this)
, which may be a seperate bug.
typescript
typescript
edited Nov 20 at 1:50
asked Nov 20 at 1:07
balupton
30.8k23104153
30.8k23104153
1
Is this your issue?
– jcalz
Nov 20 at 1:13
@jcalz thank you, it seems so, want to post the typescript compatible code snippet in an answer so I can mark it as such.
– balupton
Nov 20 at 1:15
add a comment |
1
Is this your issue?
– jcalz
Nov 20 at 1:13
@jcalz thank you, it seems so, want to post the typescript compatible code snippet in an answer so I can mark it as such.
– balupton
Nov 20 at 1:15
1
1
Is this your issue?
– jcalz
Nov 20 at 1:13
Is this your issue?
– jcalz
Nov 20 at 1:13
@jcalz thank you, it seems so, want to post the typescript compatible code snippet in an answer so I can mark it as such.
– balupton
Nov 20 at 1:15
@jcalz thank you, it seems so, want to post the typescript compatible code snippet in an answer so I can mark it as such.
– balupton
Nov 20 at 1:15
add a comment |
2 Answers
2
active
oldest
votes
up vote
2
down vote
accepted
I think a minor modification to the code alluded to in the relevant issue will also give you the proper constructor argument checking for each subclass, at least since TypeScript 3.0 introduced generic rest parameters:
type StaticThis<Args extends any, T> = { new(...args: Args): T };
abstract class A {
public static create<Args extends any, T extends A>(
this: StaticThis<Args, T>,
...args: Args
): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
const b = B.create(1, "b"); // okay
const c = C.create(); // okay
const badB = B.create(2, 3); // error, type '3' not assignable to type 'string';
const badC = C.create(4); // error, expected 0 arguments
const badA = A.create(); // error, can't use abstract class here
I'm kind of not sure about why you'd rather jump through these hoops instead of just using new
on the subclasses, but you probably have your reasons.
Hope that helps. Good luck!
Perfect, thank you so much!
– balupton
Nov 20 at 3:50
add a comment |
up vote
0
down vote
File 1 =>
export namespace Shapes {
export namespace Polygons {
export class A {
static create <T extends A>(): T {
return <T>new this();
}
sayHi(){
console.log('Hi')
}
}
export class B extends A{
sayHiFromB() {
console.log('Hi From B');
}
}
}
}
File 2 =>
import * as myImport from './testing';
const myInstanceA = myImport.Shapes.Polygons.A.create();
myInstanceA.sayHi();
const myInstanceB = myImport.Shapes.Polygons.B.create<myImport.Shapes.Polygons.B>();
myInstanceB.sayHi();
myInstanceB.sayHiFromB();
Result =>
This drops theabstract
and also removes the support for passing arguments to the extended classes.
– balupton
Nov 20 at 1:31
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
I think a minor modification to the code alluded to in the relevant issue will also give you the proper constructor argument checking for each subclass, at least since TypeScript 3.0 introduced generic rest parameters:
type StaticThis<Args extends any, T> = { new(...args: Args): T };
abstract class A {
public static create<Args extends any, T extends A>(
this: StaticThis<Args, T>,
...args: Args
): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
const b = B.create(1, "b"); // okay
const c = C.create(); // okay
const badB = B.create(2, 3); // error, type '3' not assignable to type 'string';
const badC = C.create(4); // error, expected 0 arguments
const badA = A.create(); // error, can't use abstract class here
I'm kind of not sure about why you'd rather jump through these hoops instead of just using new
on the subclasses, but you probably have your reasons.
Hope that helps. Good luck!
Perfect, thank you so much!
– balupton
Nov 20 at 3:50
add a comment |
up vote
2
down vote
accepted
I think a minor modification to the code alluded to in the relevant issue will also give you the proper constructor argument checking for each subclass, at least since TypeScript 3.0 introduced generic rest parameters:
type StaticThis<Args extends any, T> = { new(...args: Args): T };
abstract class A {
public static create<Args extends any, T extends A>(
this: StaticThis<Args, T>,
...args: Args
): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
const b = B.create(1, "b"); // okay
const c = C.create(); // okay
const badB = B.create(2, 3); // error, type '3' not assignable to type 'string';
const badC = C.create(4); // error, expected 0 arguments
const badA = A.create(); // error, can't use abstract class here
I'm kind of not sure about why you'd rather jump through these hoops instead of just using new
on the subclasses, but you probably have your reasons.
Hope that helps. Good luck!
Perfect, thank you so much!
– balupton
Nov 20 at 3:50
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
I think a minor modification to the code alluded to in the relevant issue will also give you the proper constructor argument checking for each subclass, at least since TypeScript 3.0 introduced generic rest parameters:
type StaticThis<Args extends any, T> = { new(...args: Args): T };
abstract class A {
public static create<Args extends any, T extends A>(
this: StaticThis<Args, T>,
...args: Args
): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
const b = B.create(1, "b"); // okay
const c = C.create(); // okay
const badB = B.create(2, 3); // error, type '3' not assignable to type 'string';
const badC = C.create(4); // error, expected 0 arguments
const badA = A.create(); // error, can't use abstract class here
I'm kind of not sure about why you'd rather jump through these hoops instead of just using new
on the subclasses, but you probably have your reasons.
Hope that helps. Good luck!
I think a minor modification to the code alluded to in the relevant issue will also give you the proper constructor argument checking for each subclass, at least since TypeScript 3.0 introduced generic rest parameters:
type StaticThis<Args extends any, T> = { new(...args: Args): T };
abstract class A {
public static create<Args extends any, T extends A>(
this: StaticThis<Args, T>,
...args: Args
): T {
const Klass = this
const instance = new Klass(...args)
return instance
}
}
class B extends A {
a: number
b: string
constructor(a: number, b: string) {
super()
this.a = a
this.b = b
}
}
class C extends A { }
const b = B.create(1, "b"); // okay
const c = C.create(); // okay
const badB = B.create(2, 3); // error, type '3' not assignable to type 'string';
const badC = C.create(4); // error, expected 0 arguments
const badA = A.create(); // error, can't use abstract class here
I'm kind of not sure about why you'd rather jump through these hoops instead of just using new
on the subclasses, but you probably have your reasons.
Hope that helps. Good luck!
answered Nov 20 at 2:01


jcalz
20.8k21637
20.8k21637
Perfect, thank you so much!
– balupton
Nov 20 at 3:50
add a comment |
Perfect, thank you so much!
– balupton
Nov 20 at 3:50
Perfect, thank you so much!
– balupton
Nov 20 at 3:50
Perfect, thank you so much!
– balupton
Nov 20 at 3:50
add a comment |
up vote
0
down vote
File 1 =>
export namespace Shapes {
export namespace Polygons {
export class A {
static create <T extends A>(): T {
return <T>new this();
}
sayHi(){
console.log('Hi')
}
}
export class B extends A{
sayHiFromB() {
console.log('Hi From B');
}
}
}
}
File 2 =>
import * as myImport from './testing';
const myInstanceA = myImport.Shapes.Polygons.A.create();
myInstanceA.sayHi();
const myInstanceB = myImport.Shapes.Polygons.B.create<myImport.Shapes.Polygons.B>();
myInstanceB.sayHi();
myInstanceB.sayHiFromB();
Result =>
This drops theabstract
and also removes the support for passing arguments to the extended classes.
– balupton
Nov 20 at 1:31
add a comment |
up vote
0
down vote
File 1 =>
export namespace Shapes {
export namespace Polygons {
export class A {
static create <T extends A>(): T {
return <T>new this();
}
sayHi(){
console.log('Hi')
}
}
export class B extends A{
sayHiFromB() {
console.log('Hi From B');
}
}
}
}
File 2 =>
import * as myImport from './testing';
const myInstanceA = myImport.Shapes.Polygons.A.create();
myInstanceA.sayHi();
const myInstanceB = myImport.Shapes.Polygons.B.create<myImport.Shapes.Polygons.B>();
myInstanceB.sayHi();
myInstanceB.sayHiFromB();
Result =>
This drops theabstract
and also removes the support for passing arguments to the extended classes.
– balupton
Nov 20 at 1:31
add a comment |
up vote
0
down vote
up vote
0
down vote
File 1 =>
export namespace Shapes {
export namespace Polygons {
export class A {
static create <T extends A>(): T {
return <T>new this();
}
sayHi(){
console.log('Hi')
}
}
export class B extends A{
sayHiFromB() {
console.log('Hi From B');
}
}
}
}
File 2 =>
import * as myImport from './testing';
const myInstanceA = myImport.Shapes.Polygons.A.create();
myInstanceA.sayHi();
const myInstanceB = myImport.Shapes.Polygons.B.create<myImport.Shapes.Polygons.B>();
myInstanceB.sayHi();
myInstanceB.sayHiFromB();
Result =>
File 1 =>
export namespace Shapes {
export namespace Polygons {
export class A {
static create <T extends A>(): T {
return <T>new this();
}
sayHi(){
console.log('Hi')
}
}
export class B extends A{
sayHiFromB() {
console.log('Hi From B');
}
}
}
}
File 2 =>
import * as myImport from './testing';
const myInstanceA = myImport.Shapes.Polygons.A.create();
myInstanceA.sayHi();
const myInstanceB = myImport.Shapes.Polygons.B.create<myImport.Shapes.Polygons.B>();
myInstanceB.sayHi();
myInstanceB.sayHiFromB();
Result =>
answered Nov 20 at 1:30


Gabriel Lopez
2376
2376
This drops theabstract
and also removes the support for passing arguments to the extended classes.
– balupton
Nov 20 at 1:31
add a comment |
This drops theabstract
and also removes the support for passing arguments to the extended classes.
– balupton
Nov 20 at 1:31
This drops the
abstract
and also removes the support for passing arguments to the extended classes.– balupton
Nov 20 at 1:31
This drops the
abstract
and also removes the support for passing arguments to the extended classes.– balupton
Nov 20 at 1:31
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384818%2fhow-to-typescript-this-when-it-is-dynamic%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0q60GdQ4V2s53a 5g0GR kr20lXchGtOfzl564KfGKZ7Gn 60Yge5l qUx2,yuCF87GC2N2uUMjMN5FQHZQgzqkT0qhM,l,H,3DWyDRF
1
Is this your issue?
– jcalz
Nov 20 at 1:13
@jcalz thank you, it seems so, want to post the typescript compatible code snippet in an answer so I can mark it as such.
– balupton
Nov 20 at 1:15