while (cin >> *pchar) awaits further input after Ctrl-Z
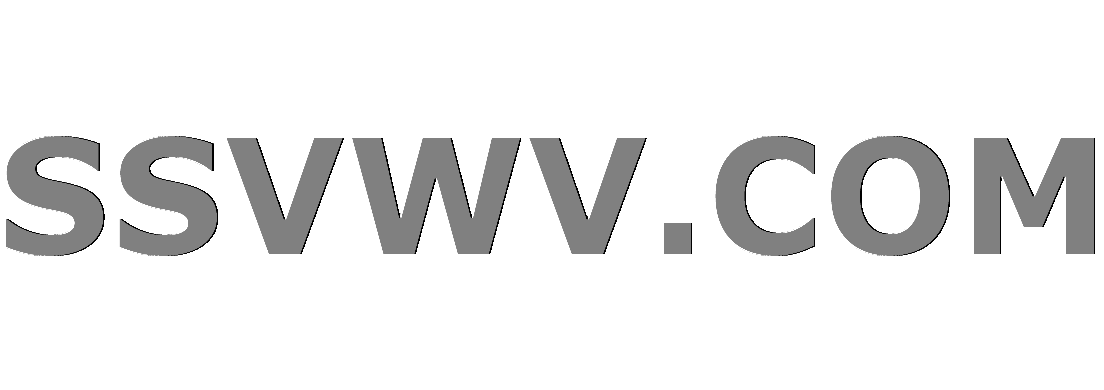
Multi tool use
up vote
0
down vote
favorite
In my Introduction to C++ classes, we were asked to write a function that returns the length of a string using pointers. The code that I wrote (see full code below) seems to work just fine, but here's the thing I don't understand.
I would think typing 'Yes' followed by Ctrl-Z ( I'm using Windows 10) in the console would stop the input. However, after pressing Ctrl-Z -> Enter the console still waits for further input. I have to start a new line after 'Yes', press Ctrl-Z and then hit Enter again to stop the input.
Why is this the case? Is there a way to stop the input after pressing just Ctrl-Z without any of the two new lines?
I read several posts on cin here, including this, this, and this, but they don't seem to answer my question.
#include "pch.h"
#include <iostream>
using namespace std;
unsigned strlen(const char *str)
{
int count = 0;
while (*str != '') { str++; count++; }
return count;
}
int main()
{
char str[100] = {};
char *pchar;
pchar = str;
while (cin >> *pchar) pchar++;
pchar = str;
cout << 'n' << strlen(pchar);
return 0;
}
c++ cin
New contributor
John Allison is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
|
show 7 more comments
up vote
0
down vote
favorite
In my Introduction to C++ classes, we were asked to write a function that returns the length of a string using pointers. The code that I wrote (see full code below) seems to work just fine, but here's the thing I don't understand.
I would think typing 'Yes' followed by Ctrl-Z ( I'm using Windows 10) in the console would stop the input. However, after pressing Ctrl-Z -> Enter the console still waits for further input. I have to start a new line after 'Yes', press Ctrl-Z and then hit Enter again to stop the input.
Why is this the case? Is there a way to stop the input after pressing just Ctrl-Z without any of the two new lines?
I read several posts on cin here, including this, this, and this, but they don't seem to answer my question.
#include "pch.h"
#include <iostream>
using namespace std;
unsigned strlen(const char *str)
{
int count = 0;
while (*str != '') { str++; count++; }
return count;
}
int main()
{
char str[100] = {};
char *pchar;
pchar = str;
while (cin >> *pchar) pchar++;
pchar = str;
cout << 'n' << strlen(pchar);
return 0;
}
c++ cin
New contributor
John Allison is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
4
Not trying to put too fine a point to it, but your C++ introduction course appears to teach C first... which is not a good idea. You shouldn't be doingchar *
in C++. Ever. Nor should you be doingstrlen()
by hand (a.k.a. "reinventing the wheel"). I know you probably cannot change any of that; I am just voicing my sadness that so many "Intro to C++" courses out there are still "C (with classes)". This is doing both you and C++'s reputation a disservice.
– DevSolar
2 days ago
4
@JohnAllison: ...pointers, which you shouldn't have to touch at all, or at least not until you're doing the advanced course. You're being taught all the C stuff -- pointers, arrays,char *
-- all of which is unnecessary / bad C++ style, and which you will have to un-learn once you get to "real" C++. Instead of showing you<string>
and references and having you do real stuff instead of reinventing the (C) wheel. I feel this is wasting your time, and making things unnecessarily difficult for you (and the instructor).
– DevSolar
2 days ago
2
@JohnAllison: In C++ we havestd::string
and the corresponding functions operating on those. Just two counterquestions: What happens, in your code, if the user enters the 101st character without ending the input? What happens if, e.g. by input redirection, the user enters a null byte somewhere in his input? Also, you can't teach C strings a.k.a.char
without teaching pointers as well... which IMHO shouldn't be taught in an intro to C++, at all.
– DevSolar
2 days ago
1
@marvin: His course should have started with the C++ that makes buffer overflows a non-issue. And I know I am not answering. I am commenting.
– DevSolar
2 days ago
2
@JohnAllison: And I understand the "bottom up" approach and all that, but I disagree with its helpfulness. Students want to achieve results. Especially with C++ where everybody tells you how godawful hard it reputedly is (which it is only if you have to learn, then unlearn C first), I find it more helpful to go top-down, and dive right in using string, vector,<algorithm>
, polymorphy and templates to their full potential instead of worrying about what's under the hood. That's for the advanced course, "library development"...
– DevSolar
2 days ago
|
show 7 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
In my Introduction to C++ classes, we were asked to write a function that returns the length of a string using pointers. The code that I wrote (see full code below) seems to work just fine, but here's the thing I don't understand.
I would think typing 'Yes' followed by Ctrl-Z ( I'm using Windows 10) in the console would stop the input. However, after pressing Ctrl-Z -> Enter the console still waits for further input. I have to start a new line after 'Yes', press Ctrl-Z and then hit Enter again to stop the input.
Why is this the case? Is there a way to stop the input after pressing just Ctrl-Z without any of the two new lines?
I read several posts on cin here, including this, this, and this, but they don't seem to answer my question.
#include "pch.h"
#include <iostream>
using namespace std;
unsigned strlen(const char *str)
{
int count = 0;
while (*str != '') { str++; count++; }
return count;
}
int main()
{
char str[100] = {};
char *pchar;
pchar = str;
while (cin >> *pchar) pchar++;
pchar = str;
cout << 'n' << strlen(pchar);
return 0;
}
c++ cin
New contributor
John Allison is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
In my Introduction to C++ classes, we were asked to write a function that returns the length of a string using pointers. The code that I wrote (see full code below) seems to work just fine, but here's the thing I don't understand.
I would think typing 'Yes' followed by Ctrl-Z ( I'm using Windows 10) in the console would stop the input. However, after pressing Ctrl-Z -> Enter the console still waits for further input. I have to start a new line after 'Yes', press Ctrl-Z and then hit Enter again to stop the input.
Why is this the case? Is there a way to stop the input after pressing just Ctrl-Z without any of the two new lines?
I read several posts on cin here, including this, this, and this, but they don't seem to answer my question.
#include "pch.h"
#include <iostream>
using namespace std;
unsigned strlen(const char *str)
{
int count = 0;
while (*str != '') { str++; count++; }
return count;
}
int main()
{
char str[100] = {};
char *pchar;
pchar = str;
while (cin >> *pchar) pchar++;
pchar = str;
cout << 'n' << strlen(pchar);
return 0;
}
c++ cin
c++ cin
New contributor
John Allison is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
John Allison is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 2 days ago
New contributor
John Allison is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 2 days ago


John Allison
11211
11211
New contributor
John Allison is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
John Allison is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
John Allison is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
4
Not trying to put too fine a point to it, but your C++ introduction course appears to teach C first... which is not a good idea. You shouldn't be doingchar *
in C++. Ever. Nor should you be doingstrlen()
by hand (a.k.a. "reinventing the wheel"). I know you probably cannot change any of that; I am just voicing my sadness that so many "Intro to C++" courses out there are still "C (with classes)". This is doing both you and C++'s reputation a disservice.
– DevSolar
2 days ago
4
@JohnAllison: ...pointers, which you shouldn't have to touch at all, or at least not until you're doing the advanced course. You're being taught all the C stuff -- pointers, arrays,char *
-- all of which is unnecessary / bad C++ style, and which you will have to un-learn once you get to "real" C++. Instead of showing you<string>
and references and having you do real stuff instead of reinventing the (C) wheel. I feel this is wasting your time, and making things unnecessarily difficult for you (and the instructor).
– DevSolar
2 days ago
2
@JohnAllison: In C++ we havestd::string
and the corresponding functions operating on those. Just two counterquestions: What happens, in your code, if the user enters the 101st character without ending the input? What happens if, e.g. by input redirection, the user enters a null byte somewhere in his input? Also, you can't teach C strings a.k.a.char
without teaching pointers as well... which IMHO shouldn't be taught in an intro to C++, at all.
– DevSolar
2 days ago
1
@marvin: His course should have started with the C++ that makes buffer overflows a non-issue. And I know I am not answering. I am commenting.
– DevSolar
2 days ago
2
@JohnAllison: And I understand the "bottom up" approach and all that, but I disagree with its helpfulness. Students want to achieve results. Especially with C++ where everybody tells you how godawful hard it reputedly is (which it is only if you have to learn, then unlearn C first), I find it more helpful to go top-down, and dive right in using string, vector,<algorithm>
, polymorphy and templates to their full potential instead of worrying about what's under the hood. That's for the advanced course, "library development"...
– DevSolar
2 days ago
|
show 7 more comments
4
Not trying to put too fine a point to it, but your C++ introduction course appears to teach C first... which is not a good idea. You shouldn't be doingchar *
in C++. Ever. Nor should you be doingstrlen()
by hand (a.k.a. "reinventing the wheel"). I know you probably cannot change any of that; I am just voicing my sadness that so many "Intro to C++" courses out there are still "C (with classes)". This is doing both you and C++'s reputation a disservice.
– DevSolar
2 days ago
4
@JohnAllison: ...pointers, which you shouldn't have to touch at all, or at least not until you're doing the advanced course. You're being taught all the C stuff -- pointers, arrays,char *
-- all of which is unnecessary / bad C++ style, and which you will have to un-learn once you get to "real" C++. Instead of showing you<string>
and references and having you do real stuff instead of reinventing the (C) wheel. I feel this is wasting your time, and making things unnecessarily difficult for you (and the instructor).
– DevSolar
2 days ago
2
@JohnAllison: In C++ we havestd::string
and the corresponding functions operating on those. Just two counterquestions: What happens, in your code, if the user enters the 101st character without ending the input? What happens if, e.g. by input redirection, the user enters a null byte somewhere in his input? Also, you can't teach C strings a.k.a.char
without teaching pointers as well... which IMHO shouldn't be taught in an intro to C++, at all.
– DevSolar
2 days ago
1
@marvin: His course should have started with the C++ that makes buffer overflows a non-issue. And I know I am not answering. I am commenting.
– DevSolar
2 days ago
2
@JohnAllison: And I understand the "bottom up" approach and all that, but I disagree with its helpfulness. Students want to achieve results. Especially with C++ where everybody tells you how godawful hard it reputedly is (which it is only if you have to learn, then unlearn C first), I find it more helpful to go top-down, and dive right in using string, vector,<algorithm>
, polymorphy and templates to their full potential instead of worrying about what's under the hood. That's for the advanced course, "library development"...
– DevSolar
2 days ago
4
4
Not trying to put too fine a point to it, but your C++ introduction course appears to teach C first... which is not a good idea. You shouldn't be doing
char *
in C++. Ever. Nor should you be doing strlen()
by hand (a.k.a. "reinventing the wheel"). I know you probably cannot change any of that; I am just voicing my sadness that so many "Intro to C++" courses out there are still "C (with classes)". This is doing both you and C++'s reputation a disservice.– DevSolar
2 days ago
Not trying to put too fine a point to it, but your C++ introduction course appears to teach C first... which is not a good idea. You shouldn't be doing
char *
in C++. Ever. Nor should you be doing strlen()
by hand (a.k.a. "reinventing the wheel"). I know you probably cannot change any of that; I am just voicing my sadness that so many "Intro to C++" courses out there are still "C (with classes)". This is doing both you and C++'s reputation a disservice.– DevSolar
2 days ago
4
4
@JohnAllison: ...pointers, which you shouldn't have to touch at all, or at least not until you're doing the advanced course. You're being taught all the C stuff -- pointers, arrays,
char *
-- all of which is unnecessary / bad C++ style, and which you will have to un-learn once you get to "real" C++. Instead of showing you <string>
and references and having you do real stuff instead of reinventing the (C) wheel. I feel this is wasting your time, and making things unnecessarily difficult for you (and the instructor).– DevSolar
2 days ago
@JohnAllison: ...pointers, which you shouldn't have to touch at all, or at least not until you're doing the advanced course. You're being taught all the C stuff -- pointers, arrays,
char *
-- all of which is unnecessary / bad C++ style, and which you will have to un-learn once you get to "real" C++. Instead of showing you <string>
and references and having you do real stuff instead of reinventing the (C) wheel. I feel this is wasting your time, and making things unnecessarily difficult for you (and the instructor).– DevSolar
2 days ago
2
2
@JohnAllison: In C++ we have
std::string
and the corresponding functions operating on those. Just two counterquestions: What happens, in your code, if the user enters the 101st character without ending the input? What happens if, e.g. by input redirection, the user enters a null byte somewhere in his input? Also, you can't teach C strings a.k.a. char
without teaching pointers as well... which IMHO shouldn't be taught in an intro to C++, at all.– DevSolar
2 days ago
@JohnAllison: In C++ we have
std::string
and the corresponding functions operating on those. Just two counterquestions: What happens, in your code, if the user enters the 101st character without ending the input? What happens if, e.g. by input redirection, the user enters a null byte somewhere in his input? Also, you can't teach C strings a.k.a. char
without teaching pointers as well... which IMHO shouldn't be taught in an intro to C++, at all.– DevSolar
2 days ago
1
1
@marvin: His course should have started with the C++ that makes buffer overflows a non-issue. And I know I am not answering. I am commenting.
– DevSolar
2 days ago
@marvin: His course should have started with the C++ that makes buffer overflows a non-issue. And I know I am not answering. I am commenting.
– DevSolar
2 days ago
2
2
@JohnAllison: And I understand the "bottom up" approach and all that, but I disagree with its helpfulness. Students want to achieve results. Especially with C++ where everybody tells you how godawful hard it reputedly is (which it is only if you have to learn, then unlearn C first), I find it more helpful to go top-down, and dive right in using string, vector,
<algorithm>
, polymorphy and templates to their full potential instead of worrying about what's under the hood. That's for the advanced course, "library development"...– DevSolar
2 days ago
@JohnAllison: And I understand the "bottom up" approach and all that, but I disagree with its helpfulness. Students want to achieve results. Especially with C++ where everybody tells you how godawful hard it reputedly is (which it is only if you have to learn, then unlearn C first), I find it more helpful to go top-down, and dive right in using string, vector,
<algorithm>
, polymorphy and templates to their full potential instead of worrying about what's under the hood. That's for the advanced course, "library development"...– DevSolar
2 days ago
|
show 7 more comments
2 Answers
2
active
oldest
votes
up vote
2
down vote
accepted
If you press Ctrl-Z after typing some other text, it flushes the line buffer (it does not set end-of-file condition).
You have to press it twice in a row; or press it after a newline; to cause the end-of-file condition to occur.
Your misunderstanding is to do with the windows console behaviour, not with C++ streams per se.
See also: Why do I require multiple EOF (CTRL+Z) characters?
This helped a lot, thanks!
– John Allison
2 days ago
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
2 days ago
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
2 days ago
add a comment |
up vote
1
down vote
Firstly we have to understand what you while loop actually does.
while (cin >> *pchar) pchar++;
says that continue getting input from the stdin
whilst cin
has not encountered an error (or to be specific whilst !cin.fail() == true
).
Note that cin
is basically an object of std::istream
.
Then secondly we have to understand what causes std::istream::fail
to return true
.
Here they are saying that std::istream::fail
returns true
if the badbit
or failbit
flags are set (and/or also if any non EOF error occurs).
Having said that, Ctrl-Z is actually EOF (end of file). And based on what I have said above, std::istream::fail
will return true
if any none EOF error occurs, and of course return false
if an EOF occurs.
So in short, EOF
/Ctrl-Z does not cause std::istream::fail
to return true
hence the loop will continue running.
If you really want to have your program cease executing when EOF
/Ctrl-Z gets hit, then adjust your loop to something like this...
while ((cin >> *pchar) && !cin.eof()) pchar++;
Now the loop above reads, whilst std::istream::fail() == false
and no EOF
/Ctrl-Z character has been entered, continue looping. Else cease the loop.
I hope that fully answers you.
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causescin.fail()
get totrue
?
– John Allison
2 days ago
that sequence worked with you old code?
– marvinIsSacul
2 days ago
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.
– John Allison
2 days ago
ifcin >> *pchar
setseofbit
, it will also setfailbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of!cin.eof()
is poor
– M.M
2 days ago
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
2 days ago
|
show 10 more comments
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
If you press Ctrl-Z after typing some other text, it flushes the line buffer (it does not set end-of-file condition).
You have to press it twice in a row; or press it after a newline; to cause the end-of-file condition to occur.
Your misunderstanding is to do with the windows console behaviour, not with C++ streams per se.
See also: Why do I require multiple EOF (CTRL+Z) characters?
This helped a lot, thanks!
– John Allison
2 days ago
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
2 days ago
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
2 days ago
add a comment |
up vote
2
down vote
accepted
If you press Ctrl-Z after typing some other text, it flushes the line buffer (it does not set end-of-file condition).
You have to press it twice in a row; or press it after a newline; to cause the end-of-file condition to occur.
Your misunderstanding is to do with the windows console behaviour, not with C++ streams per se.
See also: Why do I require multiple EOF (CTRL+Z) characters?
This helped a lot, thanks!
– John Allison
2 days ago
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
2 days ago
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
2 days ago
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
If you press Ctrl-Z after typing some other text, it flushes the line buffer (it does not set end-of-file condition).
You have to press it twice in a row; or press it after a newline; to cause the end-of-file condition to occur.
Your misunderstanding is to do with the windows console behaviour, not with C++ streams per se.
See also: Why do I require multiple EOF (CTRL+Z) characters?
If you press Ctrl-Z after typing some other text, it flushes the line buffer (it does not set end-of-file condition).
You have to press it twice in a row; or press it after a newline; to cause the end-of-file condition to occur.
Your misunderstanding is to do with the windows console behaviour, not with C++ streams per se.
See also: Why do I require multiple EOF (CTRL+Z) characters?
answered 2 days ago
M.M
103k11108228
103k11108228
This helped a lot, thanks!
– John Allison
2 days ago
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
2 days ago
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
2 days ago
add a comment |
This helped a lot, thanks!
– John Allison
2 days ago
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
2 days ago
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
2 days ago
This helped a lot, thanks!
– John Allison
2 days ago
This helped a lot, thanks!
– John Allison
2 days ago
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
2 days ago
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
2 days ago
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
2 days ago
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
2 days ago
add a comment |
up vote
1
down vote
Firstly we have to understand what you while loop actually does.
while (cin >> *pchar) pchar++;
says that continue getting input from the stdin
whilst cin
has not encountered an error (or to be specific whilst !cin.fail() == true
).
Note that cin
is basically an object of std::istream
.
Then secondly we have to understand what causes std::istream::fail
to return true
.
Here they are saying that std::istream::fail
returns true
if the badbit
or failbit
flags are set (and/or also if any non EOF error occurs).
Having said that, Ctrl-Z is actually EOF (end of file). And based on what I have said above, std::istream::fail
will return true
if any none EOF error occurs, and of course return false
if an EOF occurs.
So in short, EOF
/Ctrl-Z does not cause std::istream::fail
to return true
hence the loop will continue running.
If you really want to have your program cease executing when EOF
/Ctrl-Z gets hit, then adjust your loop to something like this...
while ((cin >> *pchar) && !cin.eof()) pchar++;
Now the loop above reads, whilst std::istream::fail() == false
and no EOF
/Ctrl-Z character has been entered, continue looping. Else cease the loop.
I hope that fully answers you.
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causescin.fail()
get totrue
?
– John Allison
2 days ago
that sequence worked with you old code?
– marvinIsSacul
2 days ago
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.
– John Allison
2 days ago
ifcin >> *pchar
setseofbit
, it will also setfailbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of!cin.eof()
is poor
– M.M
2 days ago
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
2 days ago
|
show 10 more comments
up vote
1
down vote
Firstly we have to understand what you while loop actually does.
while (cin >> *pchar) pchar++;
says that continue getting input from the stdin
whilst cin
has not encountered an error (or to be specific whilst !cin.fail() == true
).
Note that cin
is basically an object of std::istream
.
Then secondly we have to understand what causes std::istream::fail
to return true
.
Here they are saying that std::istream::fail
returns true
if the badbit
or failbit
flags are set (and/or also if any non EOF error occurs).
Having said that, Ctrl-Z is actually EOF (end of file). And based on what I have said above, std::istream::fail
will return true
if any none EOF error occurs, and of course return false
if an EOF occurs.
So in short, EOF
/Ctrl-Z does not cause std::istream::fail
to return true
hence the loop will continue running.
If you really want to have your program cease executing when EOF
/Ctrl-Z gets hit, then adjust your loop to something like this...
while ((cin >> *pchar) && !cin.eof()) pchar++;
Now the loop above reads, whilst std::istream::fail() == false
and no EOF
/Ctrl-Z character has been entered, continue looping. Else cease the loop.
I hope that fully answers you.
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causescin.fail()
get totrue
?
– John Allison
2 days ago
that sequence worked with you old code?
– marvinIsSacul
2 days ago
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.
– John Allison
2 days ago
ifcin >> *pchar
setseofbit
, it will also setfailbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of!cin.eof()
is poor
– M.M
2 days ago
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
2 days ago
|
show 10 more comments
up vote
1
down vote
up vote
1
down vote
Firstly we have to understand what you while loop actually does.
while (cin >> *pchar) pchar++;
says that continue getting input from the stdin
whilst cin
has not encountered an error (or to be specific whilst !cin.fail() == true
).
Note that cin
is basically an object of std::istream
.
Then secondly we have to understand what causes std::istream::fail
to return true
.
Here they are saying that std::istream::fail
returns true
if the badbit
or failbit
flags are set (and/or also if any non EOF error occurs).
Having said that, Ctrl-Z is actually EOF (end of file). And based on what I have said above, std::istream::fail
will return true
if any none EOF error occurs, and of course return false
if an EOF occurs.
So in short, EOF
/Ctrl-Z does not cause std::istream::fail
to return true
hence the loop will continue running.
If you really want to have your program cease executing when EOF
/Ctrl-Z gets hit, then adjust your loop to something like this...
while ((cin >> *pchar) && !cin.eof()) pchar++;
Now the loop above reads, whilst std::istream::fail() == false
and no EOF
/Ctrl-Z character has been entered, continue looping. Else cease the loop.
I hope that fully answers you.
Firstly we have to understand what you while loop actually does.
while (cin >> *pchar) pchar++;
says that continue getting input from the stdin
whilst cin
has not encountered an error (or to be specific whilst !cin.fail() == true
).
Note that cin
is basically an object of std::istream
.
Then secondly we have to understand what causes std::istream::fail
to return true
.
Here they are saying that std::istream::fail
returns true
if the badbit
or failbit
flags are set (and/or also if any non EOF error occurs).
Having said that, Ctrl-Z is actually EOF (end of file). And based on what I have said above, std::istream::fail
will return true
if any none EOF error occurs, and of course return false
if an EOF occurs.
So in short, EOF
/Ctrl-Z does not cause std::istream::fail
to return true
hence the loop will continue running.
If you really want to have your program cease executing when EOF
/Ctrl-Z gets hit, then adjust your loop to something like this...
while ((cin >> *pchar) && !cin.eof()) pchar++;
Now the loop above reads, whilst std::istream::fail() == false
and no EOF
/Ctrl-Z character has been entered, continue looping. Else cease the loop.
I hope that fully answers you.
edited 2 days ago
answered 2 days ago


marvinIsSacul
27116
27116
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causescin.fail()
get totrue
?
– John Allison
2 days ago
that sequence worked with you old code?
– marvinIsSacul
2 days ago
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.
– John Allison
2 days ago
ifcin >> *pchar
setseofbit
, it will also setfailbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of!cin.eof()
is poor
– M.M
2 days ago
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
2 days ago
|
show 10 more comments
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causescin.fail()
get totrue
?
– John Allison
2 days ago
that sequence worked with you old code?
– marvinIsSacul
2 days ago
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.
– John Allison
2 days ago
ifcin >> *pchar
setseofbit
, it will also setfailbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of!cin.eof()
is poor
– M.M
2 days ago
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
2 days ago
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causes
cin.fail()
get to true
?– John Allison
2 days ago
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causes
cin.fail()
get to true
?– John Allison
2 days ago
that sequence worked with you old code?
– marvinIsSacul
2 days ago
that sequence worked with you old code?
– marvinIsSacul
2 days ago
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding
!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.– John Allison
2 days ago
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding
!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.– John Allison
2 days ago
if
cin >> *pchar
sets eofbit
, it will also set failbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of !cin.eof()
is poor– M.M
2 days ago
if
cin >> *pchar
sets eofbit
, it will also set failbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of !cin.eof()
is poor– M.M
2 days ago
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
2 days ago
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
2 days ago
|
show 10 more comments
John Allison is a new contributor. Be nice, and check out our Code of Conduct.
John Allison is a new contributor. Be nice, and check out our Code of Conduct.
John Allison is a new contributor. Be nice, and check out our Code of Conduct.
John Allison is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53371127%2fwhile-cin-pchar-awaits-further-input-after-ctrl-z%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
g45bHRZrsBc4X1 kb,F6I,kapq9uGfIdOE6XWfF1dEXvrUCte oUTObofphfRu3,57LPyd UJhF1x99zF7wd5EKSOXD,5VMU eTTTaP
4
Not trying to put too fine a point to it, but your C++ introduction course appears to teach C first... which is not a good idea. You shouldn't be doing
char *
in C++. Ever. Nor should you be doingstrlen()
by hand (a.k.a. "reinventing the wheel"). I know you probably cannot change any of that; I am just voicing my sadness that so many "Intro to C++" courses out there are still "C (with classes)". This is doing both you and C++'s reputation a disservice.– DevSolar
2 days ago
4
@JohnAllison: ...pointers, which you shouldn't have to touch at all, or at least not until you're doing the advanced course. You're being taught all the C stuff -- pointers, arrays,
char *
-- all of which is unnecessary / bad C++ style, and which you will have to un-learn once you get to "real" C++. Instead of showing you<string>
and references and having you do real stuff instead of reinventing the (C) wheel. I feel this is wasting your time, and making things unnecessarily difficult for you (and the instructor).– DevSolar
2 days ago
2
@JohnAllison: In C++ we have
std::string
and the corresponding functions operating on those. Just two counterquestions: What happens, in your code, if the user enters the 101st character without ending the input? What happens if, e.g. by input redirection, the user enters a null byte somewhere in his input? Also, you can't teach C strings a.k.a.char
without teaching pointers as well... which IMHO shouldn't be taught in an intro to C++, at all.– DevSolar
2 days ago
1
@marvin: His course should have started with the C++ that makes buffer overflows a non-issue. And I know I am not answering. I am commenting.
– DevSolar
2 days ago
2
@JohnAllison: And I understand the "bottom up" approach and all that, but I disagree with its helpfulness. Students want to achieve results. Especially with C++ where everybody tells you how godawful hard it reputedly is (which it is only if you have to learn, then unlearn C first), I find it more helpful to go top-down, and dive right in using string, vector,
<algorithm>
, polymorphy and templates to their full potential instead of worrying about what's under the hood. That's for the advanced course, "library development"...– DevSolar
2 days ago