How can I add a key/value pair to a JavaScript object?
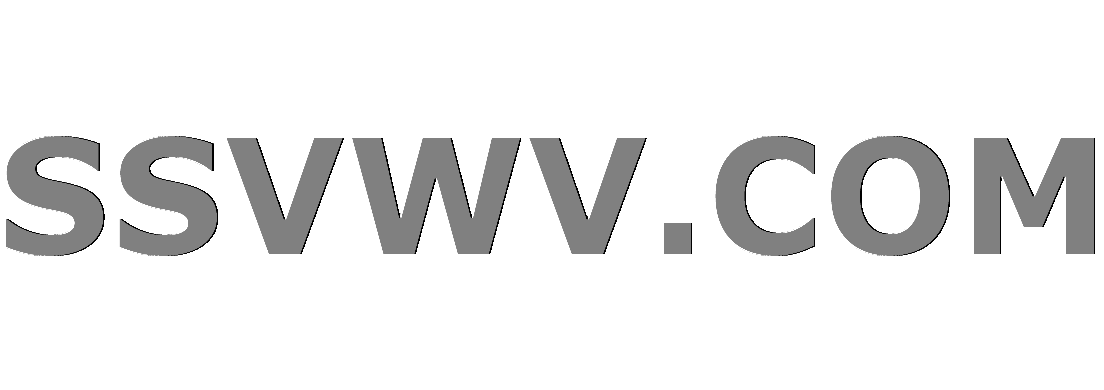
Multi tool use
up vote
1044
down vote
favorite
Here is my object literal:
var obj = {key1: value1, key2: value2};
How can I add {key3: value3}
to the object?
javascript object-literal
add a comment |
up vote
1044
down vote
favorite
Here is my object literal:
var obj = {key1: value1, key2: value2};
How can I add {key3: value3}
to the object?
javascript object-literal
1
Well, the whole issue of associative arrays in JS is weird, because you can do this... dreaminginjavascript.wordpress.com/2008/06/27/…
– Nosredna
Jul 22 '09 at 23:48
@Nosredna - the point is, there are no such things as associative arrays in javascript. In that article he is adding object properties to an array object, but these are not really part of the 'array'.
– UpTheCreek
Aug 30 '12 at 9:34
Is there a way to conditionally set key:value pairs in an object literal with future ES+ implementations?
– Con Antonakos
Apr 1 '16 at 19:00
add a comment |
up vote
1044
down vote
favorite
up vote
1044
down vote
favorite
Here is my object literal:
var obj = {key1: value1, key2: value2};
How can I add {key3: value3}
to the object?
javascript object-literal
Here is my object literal:
var obj = {key1: value1, key2: value2};
How can I add {key3: value3}
to the object?
javascript object-literal
javascript object-literal
edited Aug 14 '15 at 18:04
T.J. Crowder
668k11611801274
668k11611801274
asked Jul 22 '09 at 23:21
James Skidmore
24.1k3097133
24.1k3097133
1
Well, the whole issue of associative arrays in JS is weird, because you can do this... dreaminginjavascript.wordpress.com/2008/06/27/…
– Nosredna
Jul 22 '09 at 23:48
@Nosredna - the point is, there are no such things as associative arrays in javascript. In that article he is adding object properties to an array object, but these are not really part of the 'array'.
– UpTheCreek
Aug 30 '12 at 9:34
Is there a way to conditionally set key:value pairs in an object literal with future ES+ implementations?
– Con Antonakos
Apr 1 '16 at 19:00
add a comment |
1
Well, the whole issue of associative arrays in JS is weird, because you can do this... dreaminginjavascript.wordpress.com/2008/06/27/…
– Nosredna
Jul 22 '09 at 23:48
@Nosredna - the point is, there are no such things as associative arrays in javascript. In that article he is adding object properties to an array object, but these are not really part of the 'array'.
– UpTheCreek
Aug 30 '12 at 9:34
Is there a way to conditionally set key:value pairs in an object literal with future ES+ implementations?
– Con Antonakos
Apr 1 '16 at 19:00
1
1
Well, the whole issue of associative arrays in JS is weird, because you can do this... dreaminginjavascript.wordpress.com/2008/06/27/…
– Nosredna
Jul 22 '09 at 23:48
Well, the whole issue of associative arrays in JS is weird, because you can do this... dreaminginjavascript.wordpress.com/2008/06/27/…
– Nosredna
Jul 22 '09 at 23:48
@Nosredna - the point is, there are no such things as associative arrays in javascript. In that article he is adding object properties to an array object, but these are not really part of the 'array'.
– UpTheCreek
Aug 30 '12 at 9:34
@Nosredna - the point is, there are no such things as associative arrays in javascript. In that article he is adding object properties to an array object, but these are not really part of the 'array'.
– UpTheCreek
Aug 30 '12 at 9:34
Is there a way to conditionally set key:value pairs in an object literal with future ES+ implementations?
– Con Antonakos
Apr 1 '16 at 19:00
Is there a way to conditionally set key:value pairs in an object literal with future ES+ implementations?
– Con Antonakos
Apr 1 '16 at 19:00
add a comment |
23 Answers
23
active
oldest
votes
up vote
1746
down vote
accepted
There are two ways to add new properties to an object:
var obj = {
key1: value1,
key2: value2
};
Using dot notation:
obj.key3 = "value3";
Using square bracket notation:
obj["key3"] = "value3";
The first form is used when you know the name of the property. The second form is used when the name of the property is dynamically determined. Like in this example:
var getProperty = function (propertyName) {
return obj[propertyName];
};
getProperty("key1");
getProperty("key2");
getProperty("key3");
A real JavaScript array can be constructed using either:
The Array literal notation:
var arr = ;
The Array constructor notation:
var arr = new Array();
4
obj is an object. The part between (and including) the braces is the object literal. obj is not an object literal.
– Nosredna
Jul 22 '09 at 23:34
14
what if the key is a number? obj.123 = 456 doesn't work. obj[123] = 456 does work though
– axel freudiger
Nov 2 '12 at 10:39
4
@axelfreudiger indeed, anything that's not syntactically a valid variable identifier has to be used with bracket notation.
– Ionuț G. Stan
Nov 2 '12 at 10:42
1
@KyleKhalafObject.keys({"b": 1, "c": 3, "a": 2}).sort().forEach(console.log);
– Ionuț G. Stan
Jun 9 '17 at 6:50
2
@JohnSmith thelength
property isn't set because it's not an array, it's an object/map/dictionary.
– Ionuț G. Stan
Apr 17 at 6:09
|
show 11 more comments
up vote
139
down vote
Year 2017 answer: Object.assign()
Object.assign(dest, src1, src2, ...) merges objects.
It overwrites dest
with properties and values of (however many) source objects, then returns dest
.
The
Object.assign()
method is used to copy the values of all enumerable own properties from one or more source objects to a target object. It will return the target object.
Live example
var obj = {key1: "value1", key2: "value2"};
Object.assign(obj, {key3: "value3"});
document.body.innerHTML = JSON.stringify(obj);
Year 2018 answer: object spread operator {...}
obj = {...obj, ...pair};
From MDN:
It copies own enumerable properties from a provided object onto a new object.
Shallow-cloning (excluding prototype) or merging of objects is now possible using a shorter syntax than
Object.assign()
.
Note that
Object.assign()
triggers setters whereas spread syntax doesn’t.
Live example
It works in current Chrome and current Firefox. They say it doesn’t work in current Edge.
var obj = {key1: "value1", key2: "value2"};
var pair = {key3: "value3"};
obj = {...obj, ...pair};
document.body.innerHTML = JSON.stringify(obj);
Year 2019 answer
Object assignment operator +=
:
obj += {key3: "value3"};
Oops... I got carried away. Smuggling information from the future is illegal. Duly obscured!
9
obj+= sounds awesome
– Mehi Shokri
Jan 11 at 18:22
i am gonna love += :hungry: cool answer though!! doche
– Haroon Khan
Jan 31 at 12:58
2
This man is from the future. Best answer +1
– digiwebguy
Aug 16 at 13:14
phpstorm recommends usingconst
andlet
instead ofvar
, should that be the 2018 way?
– jim smith
Nov 12 at 10:31
add a comment |
up vote
78
down vote
I have grown fond of the LoDash / Underscore when writing larger projects.
Adding by obj['key']
or obj.key
are all solid pure JavaScript answers. However both of LoDash and Underscore libraries do provide many additional convenient functions when working with Objects and Arrays in general.
.push()
is for Arrays, not for objects.
Depending what you are looking for, there are two specific functions that may be nice to utilize and give functionality similar to the the feel of arr.push()
. For more info check the docs, they have some great examples there.
_.merge (Lodash only)
The second object will overwrite or add to the base object.
undefined
values are not copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
_.merge(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3"}
_.extend / _.assign
The second object will overwrite or add to the base object.
undefined
will be copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
_.extend(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3", key4: undefined}
_.defaults
The second object contains defaults that will be added to base object if they don't exist.
undefined
values will be copied if key already exists.
var obj = {key3: "value3", key5: "value5"};
var obj2 = {key1: "value1", key2:"value2", key3: "valueDefault", key4: "valueDefault", key5: undefined};
_.defaults(obj, obj2);
console.log(obj);
// → {key3: "value3", key5: "value5", key1: "value1", key2: "value2", key4: "valueDefault"}
$.extend
In addition, it may be worthwhile mentioning jQuery.extend, it functions similar to _.merge and may be a better option if you already are using jQuery.
The second object will overwrite or add to the base object.
undefined
values are not copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
$.extend(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3"}
Object.assign()
It may be worth mentioning the ES6/ ES2015 Object.assign, it functions similar to _.merge and may be the best option if you already are using an ES6/ES2015 polyfill like Babel if you want to polyfill yourself.
The second object will overwrite or add to the base object.
undefined
will be copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
Object.assign(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3", key4: undefined}
I believe _.merge is now _.extend(destination, others)
– A.D
Dec 8 '15 at 23:24
Ah, you're correct,_.extend
is a more universal alias since the underscore library is still usingextend
notmerge
. I'll update my answer.
– Sir.Nathan Stassen
Dec 9 '15 at 5:10
add a comment |
up vote
64
down vote
You could use either of these (provided key3 is the acutal key you want to use)
arr[ 'key3' ] = value3;
or
arr.key3 = value3;
If key3 is a variable, then you should do:
var key3 = 'a_key';
var value3 = 3;
arr[ key3 ] = value3;
After this, requesting arr.a_key
would return the value of value3
, a literal 3
.
7
This is not an array but an object. JS arrays are indexed only by integer. Try to do arr.length and it'll return 0. More reading about this: less-broken.com/blog/2010/12/…
– DevAntoine
Jul 18 '12 at 12:27
@DevAntoine's link is not accessible. If you want to get the "length" of this array type, use: Object.keys(your_array).length More reading about this problem, see: stackoverflow.com/questions/21356880/array-length-returns-0
– Thế Anh Nguyễn
Mar 14 at 5:01
One could also simple overwrite the length property of the array they're creating. Setting var myarray["length"] = numArrayFields solve this issue for me. Assuming you are keeping track of the number of fields your're adding to your array somehow that is.
– John Smith
Apr 17 at 5:31
add a comment |
up vote
27
down vote
arr.key3 = value3;
because your arr is not really an array... It's a prototype object. The real array would be:
var arr = [{key1: value1}, {key2: value2}];
but it's still not right. It should actually be:
var arr = [{key: key1, value: value1}, {key: key2, value: value2}];
add a comment |
up vote
23
down vote
var employees = ;
employees.push({id:100,name:'Yashwant',age:30});
employees.push({id:200,name:'Mahesh',age:35});
16
This is for arrays, not objects.
– Roly
Feb 28 '15 at 9:53
add a comment |
up vote
9
down vote
I know there is already an accepted answer for this but I thought I'd document my idea somewhere. Please [people] feel free to poke holes in this idea, as I'm not sure if it is the best solution... but I just put this together a few minutes ago:
Object.prototype.push = function( key, value ){
this[ key ] = value;
return this;
}
You would utilize it in this way:
var obj = {key1: value1, key2: value2};
obj.push( "key3", "value3" );
Since, the prototype function is returning this
you can continue to chain .push
's to the end of your obj
variable: obj.push(...).push(...).push(...);
Another feature is that you can pass an array or another object as the value in the push function arguments. See my fiddle for a working example: http://jsfiddle.net/7tEme/
maybe this isn't a good solution, I seem to be getting errors in jquery1.9:TypeError: 'undefined' is not a function (evaluating 'U[a].exec(s)')
which is weird because it works in jsfiddle even with jquery1.9
– sadmicrowave
Jun 26 '13 at 18:43
3
You should not extend Object.prototype; this breaks the "object-as-hashtables" feature of JavaScript (and subsequently a lot of libraries such as the Google Maps JS API). See discussion: stackoverflow.com/questions/1827458/…
– Justin R.
Oct 3 '13 at 16:54
add a comment |
up vote
8
down vote
You can create a class with the answer of @Ionuț G. Stan
function obj(){
obj=new Object();
this.add=function(key,value){
obj[""+key+""]=value;
}
this.obj=obj
}
Creating a new object with the last class:
my_obj=new obj();
my_obj.add('key1', 'value1');
my_obj.add('key2', 'value2');
my_obj.add('key3','value3');
Printing the object
console.log(my_obj.obj) // Return {key1: "value1", key2: "value2", key3: "value3"}
Printing a Key
console.log(my_obj.obj["key3"]) //Return value3
I'm newbie in javascript, comments are welcome. Works for me.
add a comment |
up vote
6
down vote
Your example shows an Object, not an Array. In that case, the preferred way to add a field to an Object is to just assign to it, like so:
arr.key3 = value3;
add a comment |
up vote
6
down vote
Two most used ways already mentioned in most answers
obj.key3 = "value3";
obj["key3"] = "value3";
One more way to define a property is using Object.defineProperty()
Object.defineProperty(obj, 'key3', {
value: "value3", // undefined by default
enumerable: true, // false by default
configurable: true, // false by default
writable: true // false by default
});
This method is useful when you want to have more control while defining property.
Property defined can be set as enumerable, configurable and writable by user.
add a comment |
up vote
5
down vote
In case you have multiple anonymous Object literals inside an Object and want to add another Object containing key/value pairs, do this:
Firebug' the Object:
console.log(Comicbook);
returns:
[Object { name="Spiderman", value="11"}, Object { name="Marsipulami",
value="18"}, Object { name="Garfield", value="2"}]
Code:
if (typeof Comicbook[3]=='undefined') {
private_formArray[3] = new Object();
private_formArray[3]["name"] = "Peanuts";
private_formArray[3]["value"] = "12";
}
will add Object {name="Peanuts", value="12"}
to the Comicbook Object
nicely & clearly explained other option which is more suitable to address objects with an id or name property then assigning it while adding , good one. specially when it has or could have in future more props same method will apply just put another coma and it's ready for change in plans
– Avia Afer
Mar 22 '16 at 7:44
add a comment |
up vote
5
down vote
Either obj['key3'] = value3
or obj.key3 = value3
will add the new pair to the obj
.
However, I know jQuery was not mentioned, but if you're using it, you can add the object through $.extend(obj,{key3: 'value3'})
. E.g.:
var obj = {key1: 'value1', key2: 'value2'};
$('#ini').append(JSON.stringify(obj));
$.extend(obj,{key3: 'value3'});
$('#ext').append(JSON.stringify(obj));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
jQuery.extend(target[,object1][,objectN]) merges the contents of two or more objects together into the first object.
And it also allows recursive adds/modifications with $.extend(true,object1,object2);
:
var object1 = {
apple: 0,
banana: { weight: 52, price: 100 },
cherry: 97
};
var object2 = {
banana: { price: 200 },
durian: 100
};
$("#ini").append(JSON.stringify(object1));
$.extend( true, object1, object2 );
$("#ext").append(JSON.stringify(object1));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
add a comment |
up vote
4
down vote
You can either add it this way:
arr['key3'] = value3;
or this way:
arr.key3 = value3;
The answers suggesting keying into the object with the variable key3
would only work if the value of key3
was 'key3'
.
add a comment |
up vote
4
down vote
According to Property Accessors defined in ECMA-262(http://www.ecma-international.org/publications/files/ECMA-ST/Ecma-262.pdf, P67), there are two ways you can do to add properties to a exists object. All these two way, the Javascript engine will treat them the same.
The first way is to use dot notation:
obj.key3 = value3;
But this way, you should use a IdentifierName after dot notation.
The second way is to use bracket notation:
obj["key3"] = value3;
and another form:
var key3 = "key3";
obj[key3] = value3;
This way, you could use a Expression (include IdentifierName) in the bracket notation.
add a comment |
up vote
3
down vote
A short and elegant way in next Javascript specification (candidate stage 3) is:
obj = { ... obj, ... { key3 : value3 } }
A deeper discussion can be found in Object spread vs Object.assign and on Dr. Axel Rauschmayers site.
It works already in node.js since release 8.6.0.
Vivaldi, Chrome, Opera, and Firefox in up to date releases know this feature also, but Mirosoft don't until today, neither in Internet Explorer nor in Edge.
add a comment |
up vote
3
down vote
var arrOfObj = [{name: 'eve'},{name:'john'},{name:'jane'}];
var injectObj = {isActive:true, timestamp:new Date()};
// function to inject key values in all object of json array
function injectKeyValueInArray (array, keyValues){
return new Promise((resolve, reject) => {
if (!array.length)
return resolve(array);
array.forEach((object) => {
for (let key in keyValues) {
object[key] = keyValues[key]
}
});
resolve(array);
})
};
//call function to inject json key value in all array object
injectKeyValueInArray(arrOfObj,injectObj).then((newArrOfObj)=>{
console.log(newArrOfObj);
});
Output like this:-
[ { name: 'eve',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z },
{ name: 'john',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z },
{ name: 'jane',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z } ]
add a comment |
up vote
2
down vote
We can do this in this way too.
var myMap = new Map();
myMap.set(0, 'my value1');
myMap.set(1, 'my value2');
for (var [key, value] of myMap) {
console.log(key + ' = ' + value);
}
add a comment |
up vote
1
down vote
Since its a question of the past but the problem of present. Would suggest one more solution: Just pass the key and values to the function and you will get a map object.
var map = {};
function addValueToMap(key, value) {
map[key] = map[key] || ;
map[key].push(value);
}
2
This doesn't seem to relate to the question that was asked at all.
– Quentin
Feb 16 at 11:15
add a comment |
up vote
0
down vote
In order to prepend a key-value pair to an object so the for in works with that element first do this:
var nwrow = {'newkey': 'value' };
for(var column in row){
nwrow[column] = row[column];
}
row = nwrow;
add a comment |
up vote
0
down vote
Best way to achieve same is stated below:
function getKey(key) {
return `${key}`;
}
var obj = {key1: "value1", key2: "value2", [getKey('key3')]: "value3"};
//console.log(obj);
add a comment |
up vote
0
down vote
Simply adding properties:
And we want to add prop2 : 2
to this object, these are the most convenient options:
- Dot operator:
object.prop2 = 2;
- square brackets:
object['prop2'] = 2;
So which one do we use then?
The dot operator is more clean syntax and should be used as a default (imo). However, the dot operator is not capable of adding dynamic keys to an object, which can be very useful in some cases. Here is an example:
const obj = {
prop1: 1
}
const key = Math.random() > 0.5 ? 'key1' : 'key2';
obj[key] = 'this value has a dynamic key';
console.log(obj);
Merging objects:
When we want to merge the properties of 2 objects these are the most convenient options:
Object.assign()
, takes a target object as an argument, and one or more source objects and will merge them together. For example:
const object1 = {
a: 1,
b: 2,
};
const object2 = Object.assign({
c: 3,
d: 4
}, object1);
console.log(object2);
- Object spread operator
...
const obj = {
prop1: 1,
prop2: 2
}
const newObj = {
...obj,
prop3: 3,
prop4: 4
}
console.log(newObj);
Which one do we use?
- The spread syntax is less verbose and has should be used as a default imo. Don't forgot to transpile this syntax to syntax which is supported by all browsers because it is relatively new.
Object.assign()
is more dynamic because we have access to all objects which are passed in as arguments and can manipulate them before they get assigned to the new Object.
1
At the top of your post: those aren't curly brackets but square brackets.
– Bram Vanroy
Aug 23 at 18:58
Thanks for pointing out ;)
– Willem van der Veen
Aug 23 at 20:01
add a comment |
up vote
0
down vote
supported by most of browsers, and it checks if object key available or not you want to add, if available it overides existing key value and it not available it add key with value
example 1
let my_object = {};
// now i want to add something in it
my_object.red = "this is red color";
// { red : "this is red color"}
example 2
let my_object = { inside_object : { car : "maruti" }}
// now i want to add something inside object of my object
my_object.inside_object.plane = "JetKing";
// { inside_object : { car : "maruti" , plane : "JetKing"} }
example 3
let my_object = { inside_object : { name : "abhishek" }}
// now i want to add something inside object with new keys birth , gender
my_object.inside_object.birth = "8 Aug";
my_object.inside_object.gender = "Male";
// { inside_object :
// { name : "abhishek",
// birth : "8 Aug",
// gender : "Male"
// }
// }
add a comment |
up vote
-17
down vote
arr.push({key3: value3});
4
This is wrong. That will add to an array, not to an object. You won't be able to reference the value using the key. Not directly, anyway.
– Matthew
Jan 26 '12 at 18:50
Wrong answer,push
is one ofArray
functions, notObject
.
– Afshin Mehrabani
Nov 21 '12 at 7:31
add a comment |
protected by Tushar Gupta Jul 27 '14 at 14:48
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
23 Answers
23
active
oldest
votes
23 Answers
23
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1746
down vote
accepted
There are two ways to add new properties to an object:
var obj = {
key1: value1,
key2: value2
};
Using dot notation:
obj.key3 = "value3";
Using square bracket notation:
obj["key3"] = "value3";
The first form is used when you know the name of the property. The second form is used when the name of the property is dynamically determined. Like in this example:
var getProperty = function (propertyName) {
return obj[propertyName];
};
getProperty("key1");
getProperty("key2");
getProperty("key3");
A real JavaScript array can be constructed using either:
The Array literal notation:
var arr = ;
The Array constructor notation:
var arr = new Array();
4
obj is an object. The part between (and including) the braces is the object literal. obj is not an object literal.
– Nosredna
Jul 22 '09 at 23:34
14
what if the key is a number? obj.123 = 456 doesn't work. obj[123] = 456 does work though
– axel freudiger
Nov 2 '12 at 10:39
4
@axelfreudiger indeed, anything that's not syntactically a valid variable identifier has to be used with bracket notation.
– Ionuț G. Stan
Nov 2 '12 at 10:42
1
@KyleKhalafObject.keys({"b": 1, "c": 3, "a": 2}).sort().forEach(console.log);
– Ionuț G. Stan
Jun 9 '17 at 6:50
2
@JohnSmith thelength
property isn't set because it's not an array, it's an object/map/dictionary.
– Ionuț G. Stan
Apr 17 at 6:09
|
show 11 more comments
up vote
1746
down vote
accepted
There are two ways to add new properties to an object:
var obj = {
key1: value1,
key2: value2
};
Using dot notation:
obj.key3 = "value3";
Using square bracket notation:
obj["key3"] = "value3";
The first form is used when you know the name of the property. The second form is used when the name of the property is dynamically determined. Like in this example:
var getProperty = function (propertyName) {
return obj[propertyName];
};
getProperty("key1");
getProperty("key2");
getProperty("key3");
A real JavaScript array can be constructed using either:
The Array literal notation:
var arr = ;
The Array constructor notation:
var arr = new Array();
4
obj is an object. The part between (and including) the braces is the object literal. obj is not an object literal.
– Nosredna
Jul 22 '09 at 23:34
14
what if the key is a number? obj.123 = 456 doesn't work. obj[123] = 456 does work though
– axel freudiger
Nov 2 '12 at 10:39
4
@axelfreudiger indeed, anything that's not syntactically a valid variable identifier has to be used with bracket notation.
– Ionuț G. Stan
Nov 2 '12 at 10:42
1
@KyleKhalafObject.keys({"b": 1, "c": 3, "a": 2}).sort().forEach(console.log);
– Ionuț G. Stan
Jun 9 '17 at 6:50
2
@JohnSmith thelength
property isn't set because it's not an array, it's an object/map/dictionary.
– Ionuț G. Stan
Apr 17 at 6:09
|
show 11 more comments
up vote
1746
down vote
accepted
up vote
1746
down vote
accepted
There are two ways to add new properties to an object:
var obj = {
key1: value1,
key2: value2
};
Using dot notation:
obj.key3 = "value3";
Using square bracket notation:
obj["key3"] = "value3";
The first form is used when you know the name of the property. The second form is used when the name of the property is dynamically determined. Like in this example:
var getProperty = function (propertyName) {
return obj[propertyName];
};
getProperty("key1");
getProperty("key2");
getProperty("key3");
A real JavaScript array can be constructed using either:
The Array literal notation:
var arr = ;
The Array constructor notation:
var arr = new Array();
There are two ways to add new properties to an object:
var obj = {
key1: value1,
key2: value2
};
Using dot notation:
obj.key3 = "value3";
Using square bracket notation:
obj["key3"] = "value3";
The first form is used when you know the name of the property. The second form is used when the name of the property is dynamically determined. Like in this example:
var getProperty = function (propertyName) {
return obj[propertyName];
};
getProperty("key1");
getProperty("key2");
getProperty("key3");
A real JavaScript array can be constructed using either:
The Array literal notation:
var arr = ;
The Array constructor notation:
var arr = new Array();
edited Nov 8 '11 at 16:36
pimvdb
111k54268334
111k54268334
answered Jul 22 '09 at 23:25
Ionuț G. Stan
130k18158186
130k18158186
4
obj is an object. The part between (and including) the braces is the object literal. obj is not an object literal.
– Nosredna
Jul 22 '09 at 23:34
14
what if the key is a number? obj.123 = 456 doesn't work. obj[123] = 456 does work though
– axel freudiger
Nov 2 '12 at 10:39
4
@axelfreudiger indeed, anything that's not syntactically a valid variable identifier has to be used with bracket notation.
– Ionuț G. Stan
Nov 2 '12 at 10:42
1
@KyleKhalafObject.keys({"b": 1, "c": 3, "a": 2}).sort().forEach(console.log);
– Ionuț G. Stan
Jun 9 '17 at 6:50
2
@JohnSmith thelength
property isn't set because it's not an array, it's an object/map/dictionary.
– Ionuț G. Stan
Apr 17 at 6:09
|
show 11 more comments
4
obj is an object. The part between (and including) the braces is the object literal. obj is not an object literal.
– Nosredna
Jul 22 '09 at 23:34
14
what if the key is a number? obj.123 = 456 doesn't work. obj[123] = 456 does work though
– axel freudiger
Nov 2 '12 at 10:39
4
@axelfreudiger indeed, anything that's not syntactically a valid variable identifier has to be used with bracket notation.
– Ionuț G. Stan
Nov 2 '12 at 10:42
1
@KyleKhalafObject.keys({"b": 1, "c": 3, "a": 2}).sort().forEach(console.log);
– Ionuț G. Stan
Jun 9 '17 at 6:50
2
@JohnSmith thelength
property isn't set because it's not an array, it's an object/map/dictionary.
– Ionuț G. Stan
Apr 17 at 6:09
4
4
obj is an object. The part between (and including) the braces is the object literal. obj is not an object literal.
– Nosredna
Jul 22 '09 at 23:34
obj is an object. The part between (and including) the braces is the object literal. obj is not an object literal.
– Nosredna
Jul 22 '09 at 23:34
14
14
what if the key is a number? obj.123 = 456 doesn't work. obj[123] = 456 does work though
– axel freudiger
Nov 2 '12 at 10:39
what if the key is a number? obj.123 = 456 doesn't work. obj[123] = 456 does work though
– axel freudiger
Nov 2 '12 at 10:39
4
4
@axelfreudiger indeed, anything that's not syntactically a valid variable identifier has to be used with bracket notation.
– Ionuț G. Stan
Nov 2 '12 at 10:42
@axelfreudiger indeed, anything that's not syntactically a valid variable identifier has to be used with bracket notation.
– Ionuț G. Stan
Nov 2 '12 at 10:42
1
1
@KyleKhalaf
Object.keys({"b": 1, "c": 3, "a": 2}).sort().forEach(console.log);
– Ionuț G. Stan
Jun 9 '17 at 6:50
@KyleKhalaf
Object.keys({"b": 1, "c": 3, "a": 2}).sort().forEach(console.log);
– Ionuț G. Stan
Jun 9 '17 at 6:50
2
2
@JohnSmith the
length
property isn't set because it's not an array, it's an object/map/dictionary.– Ionuț G. Stan
Apr 17 at 6:09
@JohnSmith the
length
property isn't set because it's not an array, it's an object/map/dictionary.– Ionuț G. Stan
Apr 17 at 6:09
|
show 11 more comments
up vote
139
down vote
Year 2017 answer: Object.assign()
Object.assign(dest, src1, src2, ...) merges objects.
It overwrites dest
with properties and values of (however many) source objects, then returns dest
.
The
Object.assign()
method is used to copy the values of all enumerable own properties from one or more source objects to a target object. It will return the target object.
Live example
var obj = {key1: "value1", key2: "value2"};
Object.assign(obj, {key3: "value3"});
document.body.innerHTML = JSON.stringify(obj);
Year 2018 answer: object spread operator {...}
obj = {...obj, ...pair};
From MDN:
It copies own enumerable properties from a provided object onto a new object.
Shallow-cloning (excluding prototype) or merging of objects is now possible using a shorter syntax than
Object.assign()
.
Note that
Object.assign()
triggers setters whereas spread syntax doesn’t.
Live example
It works in current Chrome and current Firefox. They say it doesn’t work in current Edge.
var obj = {key1: "value1", key2: "value2"};
var pair = {key3: "value3"};
obj = {...obj, ...pair};
document.body.innerHTML = JSON.stringify(obj);
Year 2019 answer
Object assignment operator +=
:
obj += {key3: "value3"};
Oops... I got carried away. Smuggling information from the future is illegal. Duly obscured!
9
obj+= sounds awesome
– Mehi Shokri
Jan 11 at 18:22
i am gonna love += :hungry: cool answer though!! doche
– Haroon Khan
Jan 31 at 12:58
2
This man is from the future. Best answer +1
– digiwebguy
Aug 16 at 13:14
phpstorm recommends usingconst
andlet
instead ofvar
, should that be the 2018 way?
– jim smith
Nov 12 at 10:31
add a comment |
up vote
139
down vote
Year 2017 answer: Object.assign()
Object.assign(dest, src1, src2, ...) merges objects.
It overwrites dest
with properties and values of (however many) source objects, then returns dest
.
The
Object.assign()
method is used to copy the values of all enumerable own properties from one or more source objects to a target object. It will return the target object.
Live example
var obj = {key1: "value1", key2: "value2"};
Object.assign(obj, {key3: "value3"});
document.body.innerHTML = JSON.stringify(obj);
Year 2018 answer: object spread operator {...}
obj = {...obj, ...pair};
From MDN:
It copies own enumerable properties from a provided object onto a new object.
Shallow-cloning (excluding prototype) or merging of objects is now possible using a shorter syntax than
Object.assign()
.
Note that
Object.assign()
triggers setters whereas spread syntax doesn’t.
Live example
It works in current Chrome and current Firefox. They say it doesn’t work in current Edge.
var obj = {key1: "value1", key2: "value2"};
var pair = {key3: "value3"};
obj = {...obj, ...pair};
document.body.innerHTML = JSON.stringify(obj);
Year 2019 answer
Object assignment operator +=
:
obj += {key3: "value3"};
Oops... I got carried away. Smuggling information from the future is illegal. Duly obscured!
9
obj+= sounds awesome
– Mehi Shokri
Jan 11 at 18:22
i am gonna love += :hungry: cool answer though!! doche
– Haroon Khan
Jan 31 at 12:58
2
This man is from the future. Best answer +1
– digiwebguy
Aug 16 at 13:14
phpstorm recommends usingconst
andlet
instead ofvar
, should that be the 2018 way?
– jim smith
Nov 12 at 10:31
add a comment |
up vote
139
down vote
up vote
139
down vote
Year 2017 answer: Object.assign()
Object.assign(dest, src1, src2, ...) merges objects.
It overwrites dest
with properties and values of (however many) source objects, then returns dest
.
The
Object.assign()
method is used to copy the values of all enumerable own properties from one or more source objects to a target object. It will return the target object.
Live example
var obj = {key1: "value1", key2: "value2"};
Object.assign(obj, {key3: "value3"});
document.body.innerHTML = JSON.stringify(obj);
Year 2018 answer: object spread operator {...}
obj = {...obj, ...pair};
From MDN:
It copies own enumerable properties from a provided object onto a new object.
Shallow-cloning (excluding prototype) or merging of objects is now possible using a shorter syntax than
Object.assign()
.
Note that
Object.assign()
triggers setters whereas spread syntax doesn’t.
Live example
It works in current Chrome and current Firefox. They say it doesn’t work in current Edge.
var obj = {key1: "value1", key2: "value2"};
var pair = {key3: "value3"};
obj = {...obj, ...pair};
document.body.innerHTML = JSON.stringify(obj);
Year 2019 answer
Object assignment operator +=
:
obj += {key3: "value3"};
Oops... I got carried away. Smuggling information from the future is illegal. Duly obscured!
Year 2017 answer: Object.assign()
Object.assign(dest, src1, src2, ...) merges objects.
It overwrites dest
with properties and values of (however many) source objects, then returns dest
.
The
Object.assign()
method is used to copy the values of all enumerable own properties from one or more source objects to a target object. It will return the target object.
Live example
var obj = {key1: "value1", key2: "value2"};
Object.assign(obj, {key3: "value3"});
document.body.innerHTML = JSON.stringify(obj);
Year 2018 answer: object spread operator {...}
obj = {...obj, ...pair};
From MDN:
It copies own enumerable properties from a provided object onto a new object.
Shallow-cloning (excluding prototype) or merging of objects is now possible using a shorter syntax than
Object.assign()
.
Note that
Object.assign()
triggers setters whereas spread syntax doesn’t.
Live example
It works in current Chrome and current Firefox. They say it doesn’t work in current Edge.
var obj = {key1: "value1", key2: "value2"};
var pair = {key3: "value3"};
obj = {...obj, ...pair};
document.body.innerHTML = JSON.stringify(obj);
Year 2019 answer
Object assignment operator +=
:
obj += {key3: "value3"};
Oops... I got carried away. Smuggling information from the future is illegal. Duly obscured!
var obj = {key1: "value1", key2: "value2"};
Object.assign(obj, {key3: "value3"});
document.body.innerHTML = JSON.stringify(obj);
var obj = {key1: "value1", key2: "value2"};
Object.assign(obj, {key3: "value3"});
document.body.innerHTML = JSON.stringify(obj);
var obj = {key1: "value1", key2: "value2"};
var pair = {key3: "value3"};
obj = {...obj, ...pair};
document.body.innerHTML = JSON.stringify(obj);
var obj = {key1: "value1", key2: "value2"};
var pair = {key3: "value3"};
obj = {...obj, ...pair};
document.body.innerHTML = JSON.stringify(obj);
edited Dec 30 '17 at 20:31
answered Nov 4 '17 at 23:51
7vujy0f0hy
2,10811119
2,10811119
9
obj+= sounds awesome
– Mehi Shokri
Jan 11 at 18:22
i am gonna love += :hungry: cool answer though!! doche
– Haroon Khan
Jan 31 at 12:58
2
This man is from the future. Best answer +1
– digiwebguy
Aug 16 at 13:14
phpstorm recommends usingconst
andlet
instead ofvar
, should that be the 2018 way?
– jim smith
Nov 12 at 10:31
add a comment |
9
obj+= sounds awesome
– Mehi Shokri
Jan 11 at 18:22
i am gonna love += :hungry: cool answer though!! doche
– Haroon Khan
Jan 31 at 12:58
2
This man is from the future. Best answer +1
– digiwebguy
Aug 16 at 13:14
phpstorm recommends usingconst
andlet
instead ofvar
, should that be the 2018 way?
– jim smith
Nov 12 at 10:31
9
9
obj+= sounds awesome
– Mehi Shokri
Jan 11 at 18:22
obj+= sounds awesome
– Mehi Shokri
Jan 11 at 18:22
i am gonna love += :hungry: cool answer though!! doche
– Haroon Khan
Jan 31 at 12:58
i am gonna love += :hungry: cool answer though!! doche
– Haroon Khan
Jan 31 at 12:58
2
2
This man is from the future. Best answer +1
– digiwebguy
Aug 16 at 13:14
This man is from the future. Best answer +1
– digiwebguy
Aug 16 at 13:14
phpstorm recommends using
const
and let
instead of var
, should that be the 2018 way?– jim smith
Nov 12 at 10:31
phpstorm recommends using
const
and let
instead of var
, should that be the 2018 way?– jim smith
Nov 12 at 10:31
add a comment |
up vote
78
down vote
I have grown fond of the LoDash / Underscore when writing larger projects.
Adding by obj['key']
or obj.key
are all solid pure JavaScript answers. However both of LoDash and Underscore libraries do provide many additional convenient functions when working with Objects and Arrays in general.
.push()
is for Arrays, not for objects.
Depending what you are looking for, there are two specific functions that may be nice to utilize and give functionality similar to the the feel of arr.push()
. For more info check the docs, they have some great examples there.
_.merge (Lodash only)
The second object will overwrite or add to the base object.
undefined
values are not copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
_.merge(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3"}
_.extend / _.assign
The second object will overwrite or add to the base object.
undefined
will be copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
_.extend(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3", key4: undefined}
_.defaults
The second object contains defaults that will be added to base object if they don't exist.
undefined
values will be copied if key already exists.
var obj = {key3: "value3", key5: "value5"};
var obj2 = {key1: "value1", key2:"value2", key3: "valueDefault", key4: "valueDefault", key5: undefined};
_.defaults(obj, obj2);
console.log(obj);
// → {key3: "value3", key5: "value5", key1: "value1", key2: "value2", key4: "valueDefault"}
$.extend
In addition, it may be worthwhile mentioning jQuery.extend, it functions similar to _.merge and may be a better option if you already are using jQuery.
The second object will overwrite or add to the base object.
undefined
values are not copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
$.extend(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3"}
Object.assign()
It may be worth mentioning the ES6/ ES2015 Object.assign, it functions similar to _.merge and may be the best option if you already are using an ES6/ES2015 polyfill like Babel if you want to polyfill yourself.
The second object will overwrite or add to the base object.
undefined
will be copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
Object.assign(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3", key4: undefined}
I believe _.merge is now _.extend(destination, others)
– A.D
Dec 8 '15 at 23:24
Ah, you're correct,_.extend
is a more universal alias since the underscore library is still usingextend
notmerge
. I'll update my answer.
– Sir.Nathan Stassen
Dec 9 '15 at 5:10
add a comment |
up vote
78
down vote
I have grown fond of the LoDash / Underscore when writing larger projects.
Adding by obj['key']
or obj.key
are all solid pure JavaScript answers. However both of LoDash and Underscore libraries do provide many additional convenient functions when working with Objects and Arrays in general.
.push()
is for Arrays, not for objects.
Depending what you are looking for, there are two specific functions that may be nice to utilize and give functionality similar to the the feel of arr.push()
. For more info check the docs, they have some great examples there.
_.merge (Lodash only)
The second object will overwrite or add to the base object.
undefined
values are not copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
_.merge(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3"}
_.extend / _.assign
The second object will overwrite or add to the base object.
undefined
will be copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
_.extend(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3", key4: undefined}
_.defaults
The second object contains defaults that will be added to base object if they don't exist.
undefined
values will be copied if key already exists.
var obj = {key3: "value3", key5: "value5"};
var obj2 = {key1: "value1", key2:"value2", key3: "valueDefault", key4: "valueDefault", key5: undefined};
_.defaults(obj, obj2);
console.log(obj);
// → {key3: "value3", key5: "value5", key1: "value1", key2: "value2", key4: "valueDefault"}
$.extend
In addition, it may be worthwhile mentioning jQuery.extend, it functions similar to _.merge and may be a better option if you already are using jQuery.
The second object will overwrite or add to the base object.
undefined
values are not copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
$.extend(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3"}
Object.assign()
It may be worth mentioning the ES6/ ES2015 Object.assign, it functions similar to _.merge and may be the best option if you already are using an ES6/ES2015 polyfill like Babel if you want to polyfill yourself.
The second object will overwrite or add to the base object.
undefined
will be copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
Object.assign(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3", key4: undefined}
I believe _.merge is now _.extend(destination, others)
– A.D
Dec 8 '15 at 23:24
Ah, you're correct,_.extend
is a more universal alias since the underscore library is still usingextend
notmerge
. I'll update my answer.
– Sir.Nathan Stassen
Dec 9 '15 at 5:10
add a comment |
up vote
78
down vote
up vote
78
down vote
I have grown fond of the LoDash / Underscore when writing larger projects.
Adding by obj['key']
or obj.key
are all solid pure JavaScript answers. However both of LoDash and Underscore libraries do provide many additional convenient functions when working with Objects and Arrays in general.
.push()
is for Arrays, not for objects.
Depending what you are looking for, there are two specific functions that may be nice to utilize and give functionality similar to the the feel of arr.push()
. For more info check the docs, they have some great examples there.
_.merge (Lodash only)
The second object will overwrite or add to the base object.
undefined
values are not copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
_.merge(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3"}
_.extend / _.assign
The second object will overwrite or add to the base object.
undefined
will be copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
_.extend(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3", key4: undefined}
_.defaults
The second object contains defaults that will be added to base object if they don't exist.
undefined
values will be copied if key already exists.
var obj = {key3: "value3", key5: "value5"};
var obj2 = {key1: "value1", key2:"value2", key3: "valueDefault", key4: "valueDefault", key5: undefined};
_.defaults(obj, obj2);
console.log(obj);
// → {key3: "value3", key5: "value5", key1: "value1", key2: "value2", key4: "valueDefault"}
$.extend
In addition, it may be worthwhile mentioning jQuery.extend, it functions similar to _.merge and may be a better option if you already are using jQuery.
The second object will overwrite or add to the base object.
undefined
values are not copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
$.extend(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3"}
Object.assign()
It may be worth mentioning the ES6/ ES2015 Object.assign, it functions similar to _.merge and may be the best option if you already are using an ES6/ES2015 polyfill like Babel if you want to polyfill yourself.
The second object will overwrite or add to the base object.
undefined
will be copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
Object.assign(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3", key4: undefined}
I have grown fond of the LoDash / Underscore when writing larger projects.
Adding by obj['key']
or obj.key
are all solid pure JavaScript answers. However both of LoDash and Underscore libraries do provide many additional convenient functions when working with Objects and Arrays in general.
.push()
is for Arrays, not for objects.
Depending what you are looking for, there are two specific functions that may be nice to utilize and give functionality similar to the the feel of arr.push()
. For more info check the docs, they have some great examples there.
_.merge (Lodash only)
The second object will overwrite or add to the base object.
undefined
values are not copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
_.merge(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3"}
_.extend / _.assign
The second object will overwrite or add to the base object.
undefined
will be copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
_.extend(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3", key4: undefined}
_.defaults
The second object contains defaults that will be added to base object if they don't exist.
undefined
values will be copied if key already exists.
var obj = {key3: "value3", key5: "value5"};
var obj2 = {key1: "value1", key2:"value2", key3: "valueDefault", key4: "valueDefault", key5: undefined};
_.defaults(obj, obj2);
console.log(obj);
// → {key3: "value3", key5: "value5", key1: "value1", key2: "value2", key4: "valueDefault"}
$.extend
In addition, it may be worthwhile mentioning jQuery.extend, it functions similar to _.merge and may be a better option if you already are using jQuery.
The second object will overwrite or add to the base object.
undefined
values are not copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
$.extend(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3"}
Object.assign()
It may be worth mentioning the ES6/ ES2015 Object.assign, it functions similar to _.merge and may be the best option if you already are using an ES6/ES2015 polyfill like Babel if you want to polyfill yourself.
The second object will overwrite or add to the base object.
undefined
will be copied.
var obj = {key1: "value1", key2: "value2"};
var obj2 = {key2:"value4", key3: "value3", key4: undefined};
Object.assign(obj, obj2);
console.log(obj);
// → {key1: "value1", key2: "value4", key3: "value3", key4: undefined}
edited Dec 28 '15 at 17:18
answered Nov 24 '14 at 18:11
Sir.Nathan Stassen
1,12011321
1,12011321
I believe _.merge is now _.extend(destination, others)
– A.D
Dec 8 '15 at 23:24
Ah, you're correct,_.extend
is a more universal alias since the underscore library is still usingextend
notmerge
. I'll update my answer.
– Sir.Nathan Stassen
Dec 9 '15 at 5:10
add a comment |
I believe _.merge is now _.extend(destination, others)
– A.D
Dec 8 '15 at 23:24
Ah, you're correct,_.extend
is a more universal alias since the underscore library is still usingextend
notmerge
. I'll update my answer.
– Sir.Nathan Stassen
Dec 9 '15 at 5:10
I believe _.merge is now _.extend(destination, others)
– A.D
Dec 8 '15 at 23:24
I believe _.merge is now _.extend(destination, others)
– A.D
Dec 8 '15 at 23:24
Ah, you're correct,
_.extend
is a more universal alias since the underscore library is still using extend
not merge
. I'll update my answer.– Sir.Nathan Stassen
Dec 9 '15 at 5:10
Ah, you're correct,
_.extend
is a more universal alias since the underscore library is still using extend
not merge
. I'll update my answer.– Sir.Nathan Stassen
Dec 9 '15 at 5:10
add a comment |
up vote
64
down vote
You could use either of these (provided key3 is the acutal key you want to use)
arr[ 'key3' ] = value3;
or
arr.key3 = value3;
If key3 is a variable, then you should do:
var key3 = 'a_key';
var value3 = 3;
arr[ key3 ] = value3;
After this, requesting arr.a_key
would return the value of value3
, a literal 3
.
7
This is not an array but an object. JS arrays are indexed only by integer. Try to do arr.length and it'll return 0. More reading about this: less-broken.com/blog/2010/12/…
– DevAntoine
Jul 18 '12 at 12:27
@DevAntoine's link is not accessible. If you want to get the "length" of this array type, use: Object.keys(your_array).length More reading about this problem, see: stackoverflow.com/questions/21356880/array-length-returns-0
– Thế Anh Nguyễn
Mar 14 at 5:01
One could also simple overwrite the length property of the array they're creating. Setting var myarray["length"] = numArrayFields solve this issue for me. Assuming you are keeping track of the number of fields your're adding to your array somehow that is.
– John Smith
Apr 17 at 5:31
add a comment |
up vote
64
down vote
You could use either of these (provided key3 is the acutal key you want to use)
arr[ 'key3' ] = value3;
or
arr.key3 = value3;
If key3 is a variable, then you should do:
var key3 = 'a_key';
var value3 = 3;
arr[ key3 ] = value3;
After this, requesting arr.a_key
would return the value of value3
, a literal 3
.
7
This is not an array but an object. JS arrays are indexed only by integer. Try to do arr.length and it'll return 0. More reading about this: less-broken.com/blog/2010/12/…
– DevAntoine
Jul 18 '12 at 12:27
@DevAntoine's link is not accessible. If you want to get the "length" of this array type, use: Object.keys(your_array).length More reading about this problem, see: stackoverflow.com/questions/21356880/array-length-returns-0
– Thế Anh Nguyễn
Mar 14 at 5:01
One could also simple overwrite the length property of the array they're creating. Setting var myarray["length"] = numArrayFields solve this issue for me. Assuming you are keeping track of the number of fields your're adding to your array somehow that is.
– John Smith
Apr 17 at 5:31
add a comment |
up vote
64
down vote
up vote
64
down vote
You could use either of these (provided key3 is the acutal key you want to use)
arr[ 'key3' ] = value3;
or
arr.key3 = value3;
If key3 is a variable, then you should do:
var key3 = 'a_key';
var value3 = 3;
arr[ key3 ] = value3;
After this, requesting arr.a_key
would return the value of value3
, a literal 3
.
You could use either of these (provided key3 is the acutal key you want to use)
arr[ 'key3' ] = value3;
or
arr.key3 = value3;
If key3 is a variable, then you should do:
var key3 = 'a_key';
var value3 = 3;
arr[ key3 ] = value3;
After this, requesting arr.a_key
would return the value of value3
, a literal 3
.
edited Mar 31 '12 at 0:03
ACK_stoverflow
1,65311628
1,65311628
answered Jul 22 '09 at 23:22
seth
30.7k75457
30.7k75457
7
This is not an array but an object. JS arrays are indexed only by integer. Try to do arr.length and it'll return 0. More reading about this: less-broken.com/blog/2010/12/…
– DevAntoine
Jul 18 '12 at 12:27
@DevAntoine's link is not accessible. If you want to get the "length" of this array type, use: Object.keys(your_array).length More reading about this problem, see: stackoverflow.com/questions/21356880/array-length-returns-0
– Thế Anh Nguyễn
Mar 14 at 5:01
One could also simple overwrite the length property of the array they're creating. Setting var myarray["length"] = numArrayFields solve this issue for me. Assuming you are keeping track of the number of fields your're adding to your array somehow that is.
– John Smith
Apr 17 at 5:31
add a comment |
7
This is not an array but an object. JS arrays are indexed only by integer. Try to do arr.length and it'll return 0. More reading about this: less-broken.com/blog/2010/12/…
– DevAntoine
Jul 18 '12 at 12:27
@DevAntoine's link is not accessible. If you want to get the "length" of this array type, use: Object.keys(your_array).length More reading about this problem, see: stackoverflow.com/questions/21356880/array-length-returns-0
– Thế Anh Nguyễn
Mar 14 at 5:01
One could also simple overwrite the length property of the array they're creating. Setting var myarray["length"] = numArrayFields solve this issue for me. Assuming you are keeping track of the number of fields your're adding to your array somehow that is.
– John Smith
Apr 17 at 5:31
7
7
This is not an array but an object. JS arrays are indexed only by integer. Try to do arr.length and it'll return 0. More reading about this: less-broken.com/blog/2010/12/…
– DevAntoine
Jul 18 '12 at 12:27
This is not an array but an object. JS arrays are indexed only by integer. Try to do arr.length and it'll return 0. More reading about this: less-broken.com/blog/2010/12/…
– DevAntoine
Jul 18 '12 at 12:27
@DevAntoine's link is not accessible. If you want to get the "length" of this array type, use: Object.keys(your_array).length More reading about this problem, see: stackoverflow.com/questions/21356880/array-length-returns-0
– Thế Anh Nguyễn
Mar 14 at 5:01
@DevAntoine's link is not accessible. If you want to get the "length" of this array type, use: Object.keys(your_array).length More reading about this problem, see: stackoverflow.com/questions/21356880/array-length-returns-0
– Thế Anh Nguyễn
Mar 14 at 5:01
One could also simple overwrite the length property of the array they're creating. Setting var myarray["length"] = numArrayFields solve this issue for me. Assuming you are keeping track of the number of fields your're adding to your array somehow that is.
– John Smith
Apr 17 at 5:31
One could also simple overwrite the length property of the array they're creating. Setting var myarray["length"] = numArrayFields solve this issue for me. Assuming you are keeping track of the number of fields your're adding to your array somehow that is.
– John Smith
Apr 17 at 5:31
add a comment |
up vote
27
down vote
arr.key3 = value3;
because your arr is not really an array... It's a prototype object. The real array would be:
var arr = [{key1: value1}, {key2: value2}];
but it's still not right. It should actually be:
var arr = [{key: key1, value: value1}, {key: key2, value: value2}];
add a comment |
up vote
27
down vote
arr.key3 = value3;
because your arr is not really an array... It's a prototype object. The real array would be:
var arr = [{key1: value1}, {key2: value2}];
but it's still not right. It should actually be:
var arr = [{key: key1, value: value1}, {key: key2, value: value2}];
add a comment |
up vote
27
down vote
up vote
27
down vote
arr.key3 = value3;
because your arr is not really an array... It's a prototype object. The real array would be:
var arr = [{key1: value1}, {key2: value2}];
but it's still not right. It should actually be:
var arr = [{key: key1, value: value1}, {key: key2, value: value2}];
arr.key3 = value3;
because your arr is not really an array... It's a prototype object. The real array would be:
var arr = [{key1: value1}, {key2: value2}];
but it's still not right. It should actually be:
var arr = [{key: key1, value: value1}, {key: key2, value: value2}];
answered Jul 22 '09 at 23:25
Robert Koritnik
76.2k42237361
76.2k42237361
add a comment |
add a comment |
up vote
23
down vote
var employees = ;
employees.push({id:100,name:'Yashwant',age:30});
employees.push({id:200,name:'Mahesh',age:35});
16
This is for arrays, not objects.
– Roly
Feb 28 '15 at 9:53
add a comment |
up vote
23
down vote
var employees = ;
employees.push({id:100,name:'Yashwant',age:30});
employees.push({id:200,name:'Mahesh',age:35});
16
This is for arrays, not objects.
– Roly
Feb 28 '15 at 9:53
add a comment |
up vote
23
down vote
up vote
23
down vote
var employees = ;
employees.push({id:100,name:'Yashwant',age:30});
employees.push({id:200,name:'Mahesh',age:35});
var employees = ;
employees.push({id:100,name:'Yashwant',age:30});
employees.push({id:200,name:'Mahesh',age:35});
edited Sep 26 '17 at 8:46
Martijn Pieters♦
691k12923852231
691k12923852231
answered Oct 27 '13 at 17:49
Vicky
6,554136281
6,554136281
16
This is for arrays, not objects.
– Roly
Feb 28 '15 at 9:53
add a comment |
16
This is for arrays, not objects.
– Roly
Feb 28 '15 at 9:53
16
16
This is for arrays, not objects.
– Roly
Feb 28 '15 at 9:53
This is for arrays, not objects.
– Roly
Feb 28 '15 at 9:53
add a comment |
up vote
9
down vote
I know there is already an accepted answer for this but I thought I'd document my idea somewhere. Please [people] feel free to poke holes in this idea, as I'm not sure if it is the best solution... but I just put this together a few minutes ago:
Object.prototype.push = function( key, value ){
this[ key ] = value;
return this;
}
You would utilize it in this way:
var obj = {key1: value1, key2: value2};
obj.push( "key3", "value3" );
Since, the prototype function is returning this
you can continue to chain .push
's to the end of your obj
variable: obj.push(...).push(...).push(...);
Another feature is that you can pass an array or another object as the value in the push function arguments. See my fiddle for a working example: http://jsfiddle.net/7tEme/
maybe this isn't a good solution, I seem to be getting errors in jquery1.9:TypeError: 'undefined' is not a function (evaluating 'U[a].exec(s)')
which is weird because it works in jsfiddle even with jquery1.9
– sadmicrowave
Jun 26 '13 at 18:43
3
You should not extend Object.prototype; this breaks the "object-as-hashtables" feature of JavaScript (and subsequently a lot of libraries such as the Google Maps JS API). See discussion: stackoverflow.com/questions/1827458/…
– Justin R.
Oct 3 '13 at 16:54
add a comment |
up vote
9
down vote
I know there is already an accepted answer for this but I thought I'd document my idea somewhere. Please [people] feel free to poke holes in this idea, as I'm not sure if it is the best solution... but I just put this together a few minutes ago:
Object.prototype.push = function( key, value ){
this[ key ] = value;
return this;
}
You would utilize it in this way:
var obj = {key1: value1, key2: value2};
obj.push( "key3", "value3" );
Since, the prototype function is returning this
you can continue to chain .push
's to the end of your obj
variable: obj.push(...).push(...).push(...);
Another feature is that you can pass an array or another object as the value in the push function arguments. See my fiddle for a working example: http://jsfiddle.net/7tEme/
maybe this isn't a good solution, I seem to be getting errors in jquery1.9:TypeError: 'undefined' is not a function (evaluating 'U[a].exec(s)')
which is weird because it works in jsfiddle even with jquery1.9
– sadmicrowave
Jun 26 '13 at 18:43
3
You should not extend Object.prototype; this breaks the "object-as-hashtables" feature of JavaScript (and subsequently a lot of libraries such as the Google Maps JS API). See discussion: stackoverflow.com/questions/1827458/…
– Justin R.
Oct 3 '13 at 16:54
add a comment |
up vote
9
down vote
up vote
9
down vote
I know there is already an accepted answer for this but I thought I'd document my idea somewhere. Please [people] feel free to poke holes in this idea, as I'm not sure if it is the best solution... but I just put this together a few minutes ago:
Object.prototype.push = function( key, value ){
this[ key ] = value;
return this;
}
You would utilize it in this way:
var obj = {key1: value1, key2: value2};
obj.push( "key3", "value3" );
Since, the prototype function is returning this
you can continue to chain .push
's to the end of your obj
variable: obj.push(...).push(...).push(...);
Another feature is that you can pass an array or another object as the value in the push function arguments. See my fiddle for a working example: http://jsfiddle.net/7tEme/
I know there is already an accepted answer for this but I thought I'd document my idea somewhere. Please [people] feel free to poke holes in this idea, as I'm not sure if it is the best solution... but I just put this together a few minutes ago:
Object.prototype.push = function( key, value ){
this[ key ] = value;
return this;
}
You would utilize it in this way:
var obj = {key1: value1, key2: value2};
obj.push( "key3", "value3" );
Since, the prototype function is returning this
you can continue to chain .push
's to the end of your obj
variable: obj.push(...).push(...).push(...);
Another feature is that you can pass an array or another object as the value in the push function arguments. See my fiddle for a working example: http://jsfiddle.net/7tEme/
answered Jun 26 '13 at 18:34
sadmicrowave
14.5k3291152
14.5k3291152
maybe this isn't a good solution, I seem to be getting errors in jquery1.9:TypeError: 'undefined' is not a function (evaluating 'U[a].exec(s)')
which is weird because it works in jsfiddle even with jquery1.9
– sadmicrowave
Jun 26 '13 at 18:43
3
You should not extend Object.prototype; this breaks the "object-as-hashtables" feature of JavaScript (and subsequently a lot of libraries such as the Google Maps JS API). See discussion: stackoverflow.com/questions/1827458/…
– Justin R.
Oct 3 '13 at 16:54
add a comment |
maybe this isn't a good solution, I seem to be getting errors in jquery1.9:TypeError: 'undefined' is not a function (evaluating 'U[a].exec(s)')
which is weird because it works in jsfiddle even with jquery1.9
– sadmicrowave
Jun 26 '13 at 18:43
3
You should not extend Object.prototype; this breaks the "object-as-hashtables" feature of JavaScript (and subsequently a lot of libraries such as the Google Maps JS API). See discussion: stackoverflow.com/questions/1827458/…
– Justin R.
Oct 3 '13 at 16:54
maybe this isn't a good solution, I seem to be getting errors in jquery1.9:
TypeError: 'undefined' is not a function (evaluating 'U[a].exec(s)')
which is weird because it works in jsfiddle even with jquery1.9– sadmicrowave
Jun 26 '13 at 18:43
maybe this isn't a good solution, I seem to be getting errors in jquery1.9:
TypeError: 'undefined' is not a function (evaluating 'U[a].exec(s)')
which is weird because it works in jsfiddle even with jquery1.9– sadmicrowave
Jun 26 '13 at 18:43
3
3
You should not extend Object.prototype; this breaks the "object-as-hashtables" feature of JavaScript (and subsequently a lot of libraries such as the Google Maps JS API). See discussion: stackoverflow.com/questions/1827458/…
– Justin R.
Oct 3 '13 at 16:54
You should not extend Object.prototype; this breaks the "object-as-hashtables" feature of JavaScript (and subsequently a lot of libraries such as the Google Maps JS API). See discussion: stackoverflow.com/questions/1827458/…
– Justin R.
Oct 3 '13 at 16:54
add a comment |
up vote
8
down vote
You can create a class with the answer of @Ionuț G. Stan
function obj(){
obj=new Object();
this.add=function(key,value){
obj[""+key+""]=value;
}
this.obj=obj
}
Creating a new object with the last class:
my_obj=new obj();
my_obj.add('key1', 'value1');
my_obj.add('key2', 'value2');
my_obj.add('key3','value3');
Printing the object
console.log(my_obj.obj) // Return {key1: "value1", key2: "value2", key3: "value3"}
Printing a Key
console.log(my_obj.obj["key3"]) //Return value3
I'm newbie in javascript, comments are welcome. Works for me.
add a comment |
up vote
8
down vote
You can create a class with the answer of @Ionuț G. Stan
function obj(){
obj=new Object();
this.add=function(key,value){
obj[""+key+""]=value;
}
this.obj=obj
}
Creating a new object with the last class:
my_obj=new obj();
my_obj.add('key1', 'value1');
my_obj.add('key2', 'value2');
my_obj.add('key3','value3');
Printing the object
console.log(my_obj.obj) // Return {key1: "value1", key2: "value2", key3: "value3"}
Printing a Key
console.log(my_obj.obj["key3"]) //Return value3
I'm newbie in javascript, comments are welcome. Works for me.
add a comment |
up vote
8
down vote
up vote
8
down vote
You can create a class with the answer of @Ionuț G. Stan
function obj(){
obj=new Object();
this.add=function(key,value){
obj[""+key+""]=value;
}
this.obj=obj
}
Creating a new object with the last class:
my_obj=new obj();
my_obj.add('key1', 'value1');
my_obj.add('key2', 'value2');
my_obj.add('key3','value3');
Printing the object
console.log(my_obj.obj) // Return {key1: "value1", key2: "value2", key3: "value3"}
Printing a Key
console.log(my_obj.obj["key3"]) //Return value3
I'm newbie in javascript, comments are welcome. Works for me.
You can create a class with the answer of @Ionuț G. Stan
function obj(){
obj=new Object();
this.add=function(key,value){
obj[""+key+""]=value;
}
this.obj=obj
}
Creating a new object with the last class:
my_obj=new obj();
my_obj.add('key1', 'value1');
my_obj.add('key2', 'value2');
my_obj.add('key3','value3');
Printing the object
console.log(my_obj.obj) // Return {key1: "value1", key2: "value2", key3: "value3"}
Printing a Key
console.log(my_obj.obj["key3"]) //Return value3
I'm newbie in javascript, comments are welcome. Works for me.
edited May 23 '17 at 11:47
Community♦
11
11
answered Dec 28 '13 at 1:34
Eduen Sarceno
171126
171126
add a comment |
add a comment |
up vote
6
down vote
Your example shows an Object, not an Array. In that case, the preferred way to add a field to an Object is to just assign to it, like so:
arr.key3 = value3;
add a comment |
up vote
6
down vote
Your example shows an Object, not an Array. In that case, the preferred way to add a field to an Object is to just assign to it, like so:
arr.key3 = value3;
add a comment |
up vote
6
down vote
up vote
6
down vote
Your example shows an Object, not an Array. In that case, the preferred way to add a field to an Object is to just assign to it, like so:
arr.key3 = value3;
Your example shows an Object, not an Array. In that case, the preferred way to add a field to an Object is to just assign to it, like so:
arr.key3 = value3;
answered Jul 22 '09 at 23:25
bdukes
112k19126160
112k19126160
add a comment |
add a comment |
up vote
6
down vote
Two most used ways already mentioned in most answers
obj.key3 = "value3";
obj["key3"] = "value3";
One more way to define a property is using Object.defineProperty()
Object.defineProperty(obj, 'key3', {
value: "value3", // undefined by default
enumerable: true, // false by default
configurable: true, // false by default
writable: true // false by default
});
This method is useful when you want to have more control while defining property.
Property defined can be set as enumerable, configurable and writable by user.
add a comment |
up vote
6
down vote
Two most used ways already mentioned in most answers
obj.key3 = "value3";
obj["key3"] = "value3";
One more way to define a property is using Object.defineProperty()
Object.defineProperty(obj, 'key3', {
value: "value3", // undefined by default
enumerable: true, // false by default
configurable: true, // false by default
writable: true // false by default
});
This method is useful when you want to have more control while defining property.
Property defined can be set as enumerable, configurable and writable by user.
add a comment |
up vote
6
down vote
up vote
6
down vote
Two most used ways already mentioned in most answers
obj.key3 = "value3";
obj["key3"] = "value3";
One more way to define a property is using Object.defineProperty()
Object.defineProperty(obj, 'key3', {
value: "value3", // undefined by default
enumerable: true, // false by default
configurable: true, // false by default
writable: true // false by default
});
This method is useful when you want to have more control while defining property.
Property defined can be set as enumerable, configurable and writable by user.
Two most used ways already mentioned in most answers
obj.key3 = "value3";
obj["key3"] = "value3";
One more way to define a property is using Object.defineProperty()
Object.defineProperty(obj, 'key3', {
value: "value3", // undefined by default
enumerable: true, // false by default
configurable: true, // false by default
writable: true // false by default
});
This method is useful when you want to have more control while defining property.
Property defined can be set as enumerable, configurable and writable by user.
answered Jan 13 '17 at 6:14


Sanchay Kumar
40248
40248
add a comment |
add a comment |
up vote
5
down vote
In case you have multiple anonymous Object literals inside an Object and want to add another Object containing key/value pairs, do this:
Firebug' the Object:
console.log(Comicbook);
returns:
[Object { name="Spiderman", value="11"}, Object { name="Marsipulami",
value="18"}, Object { name="Garfield", value="2"}]
Code:
if (typeof Comicbook[3]=='undefined') {
private_formArray[3] = new Object();
private_formArray[3]["name"] = "Peanuts";
private_formArray[3]["value"] = "12";
}
will add Object {name="Peanuts", value="12"}
to the Comicbook Object
nicely & clearly explained other option which is more suitable to address objects with an id or name property then assigning it while adding , good one. specially when it has or could have in future more props same method will apply just put another coma and it's ready for change in plans
– Avia Afer
Mar 22 '16 at 7:44
add a comment |
up vote
5
down vote
In case you have multiple anonymous Object literals inside an Object and want to add another Object containing key/value pairs, do this:
Firebug' the Object:
console.log(Comicbook);
returns:
[Object { name="Spiderman", value="11"}, Object { name="Marsipulami",
value="18"}, Object { name="Garfield", value="2"}]
Code:
if (typeof Comicbook[3]=='undefined') {
private_formArray[3] = new Object();
private_formArray[3]["name"] = "Peanuts";
private_formArray[3]["value"] = "12";
}
will add Object {name="Peanuts", value="12"}
to the Comicbook Object
nicely & clearly explained other option which is more suitable to address objects with an id or name property then assigning it while adding , good one. specially when it has or could have in future more props same method will apply just put another coma and it's ready for change in plans
– Avia Afer
Mar 22 '16 at 7:44
add a comment |
up vote
5
down vote
up vote
5
down vote
In case you have multiple anonymous Object literals inside an Object and want to add another Object containing key/value pairs, do this:
Firebug' the Object:
console.log(Comicbook);
returns:
[Object { name="Spiderman", value="11"}, Object { name="Marsipulami",
value="18"}, Object { name="Garfield", value="2"}]
Code:
if (typeof Comicbook[3]=='undefined') {
private_formArray[3] = new Object();
private_formArray[3]["name"] = "Peanuts";
private_formArray[3]["value"] = "12";
}
will add Object {name="Peanuts", value="12"}
to the Comicbook Object
In case you have multiple anonymous Object literals inside an Object and want to add another Object containing key/value pairs, do this:
Firebug' the Object:
console.log(Comicbook);
returns:
[Object { name="Spiderman", value="11"}, Object { name="Marsipulami",
value="18"}, Object { name="Garfield", value="2"}]
Code:
if (typeof Comicbook[3]=='undefined') {
private_formArray[3] = new Object();
private_formArray[3]["name"] = "Peanuts";
private_formArray[3]["value"] = "12";
}
will add Object {name="Peanuts", value="12"}
to the Comicbook Object
answered Aug 1 '12 at 14:28


nottinhill
7,01854499
7,01854499
nicely & clearly explained other option which is more suitable to address objects with an id or name property then assigning it while adding , good one. specially when it has or could have in future more props same method will apply just put another coma and it's ready for change in plans
– Avia Afer
Mar 22 '16 at 7:44
add a comment |
nicely & clearly explained other option which is more suitable to address objects with an id or name property then assigning it while adding , good one. specially when it has or could have in future more props same method will apply just put another coma and it's ready for change in plans
– Avia Afer
Mar 22 '16 at 7:44
nicely & clearly explained other option which is more suitable to address objects with an id or name property then assigning it while adding , good one. specially when it has or could have in future more props same method will apply just put another coma and it's ready for change in plans
– Avia Afer
Mar 22 '16 at 7:44
nicely & clearly explained other option which is more suitable to address objects with an id or name property then assigning it while adding , good one. specially when it has or could have in future more props same method will apply just put another coma and it's ready for change in plans
– Avia Afer
Mar 22 '16 at 7:44
add a comment |
up vote
5
down vote
Either obj['key3'] = value3
or obj.key3 = value3
will add the new pair to the obj
.
However, I know jQuery was not mentioned, but if you're using it, you can add the object through $.extend(obj,{key3: 'value3'})
. E.g.:
var obj = {key1: 'value1', key2: 'value2'};
$('#ini').append(JSON.stringify(obj));
$.extend(obj,{key3: 'value3'});
$('#ext').append(JSON.stringify(obj));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
jQuery.extend(target[,object1][,objectN]) merges the contents of two or more objects together into the first object.
And it also allows recursive adds/modifications with $.extend(true,object1,object2);
:
var object1 = {
apple: 0,
banana: { weight: 52, price: 100 },
cherry: 97
};
var object2 = {
banana: { price: 200 },
durian: 100
};
$("#ini").append(JSON.stringify(object1));
$.extend( true, object1, object2 );
$("#ext").append(JSON.stringify(object1));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
add a comment |
up vote
5
down vote
Either obj['key3'] = value3
or obj.key3 = value3
will add the new pair to the obj
.
However, I know jQuery was not mentioned, but if you're using it, you can add the object through $.extend(obj,{key3: 'value3'})
. E.g.:
var obj = {key1: 'value1', key2: 'value2'};
$('#ini').append(JSON.stringify(obj));
$.extend(obj,{key3: 'value3'});
$('#ext').append(JSON.stringify(obj));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
jQuery.extend(target[,object1][,objectN]) merges the contents of two or more objects together into the first object.
And it also allows recursive adds/modifications with $.extend(true,object1,object2);
:
var object1 = {
apple: 0,
banana: { weight: 52, price: 100 },
cherry: 97
};
var object2 = {
banana: { price: 200 },
durian: 100
};
$("#ini").append(JSON.stringify(object1));
$.extend( true, object1, object2 );
$("#ext").append(JSON.stringify(object1));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
add a comment |
up vote
5
down vote
up vote
5
down vote
Either obj['key3'] = value3
or obj.key3 = value3
will add the new pair to the obj
.
However, I know jQuery was not mentioned, but if you're using it, you can add the object through $.extend(obj,{key3: 'value3'})
. E.g.:
var obj = {key1: 'value1', key2: 'value2'};
$('#ini').append(JSON.stringify(obj));
$.extend(obj,{key3: 'value3'});
$('#ext').append(JSON.stringify(obj));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
jQuery.extend(target[,object1][,objectN]) merges the contents of two or more objects together into the first object.
And it also allows recursive adds/modifications with $.extend(true,object1,object2);
:
var object1 = {
apple: 0,
banana: { weight: 52, price: 100 },
cherry: 97
};
var object2 = {
banana: { price: 200 },
durian: 100
};
$("#ini").append(JSON.stringify(object1));
$.extend( true, object1, object2 );
$("#ext").append(JSON.stringify(object1));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
Either obj['key3'] = value3
or obj.key3 = value3
will add the new pair to the obj
.
However, I know jQuery was not mentioned, but if you're using it, you can add the object through $.extend(obj,{key3: 'value3'})
. E.g.:
var obj = {key1: 'value1', key2: 'value2'};
$('#ini').append(JSON.stringify(obj));
$.extend(obj,{key3: 'value3'});
$('#ext').append(JSON.stringify(obj));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
jQuery.extend(target[,object1][,objectN]) merges the contents of two or more objects together into the first object.
And it also allows recursive adds/modifications with $.extend(true,object1,object2);
:
var object1 = {
apple: 0,
banana: { weight: 52, price: 100 },
cherry: 97
};
var object2 = {
banana: { price: 200 },
durian: 100
};
$("#ini").append(JSON.stringify(object1));
$.extend( true, object1, object2 );
$("#ext").append(JSON.stringify(object1));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
var obj = {key1: 'value1', key2: 'value2'};
$('#ini').append(JSON.stringify(obj));
$.extend(obj,{key3: 'value3'});
$('#ext').append(JSON.stringify(obj));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
var obj = {key1: 'value1', key2: 'value2'};
$('#ini').append(JSON.stringify(obj));
$.extend(obj,{key3: 'value3'});
$('#ext').append(JSON.stringify(obj));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
var object1 = {
apple: 0,
banana: { weight: 52, price: 100 },
cherry: 97
};
var object2 = {
banana: { price: 200 },
durian: 100
};
$("#ini").append(JSON.stringify(object1));
$.extend( true, object1, object2 );
$("#ext").append(JSON.stringify(object1));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
var object1 = {
apple: 0,
banana: { weight: 52, price: 100 },
cherry: 97
};
var object2 = {
banana: { price: 200 },
durian: 100
};
$("#ini").append(JSON.stringify(object1));
$.extend( true, object1, object2 );
$("#ext").append(JSON.stringify(object1));
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<p id="ini">Initial: </p>
<p id="ext">Extended: </p>
edited Jun 8 '15 at 17:47
answered Jun 8 '15 at 17:17


Armfoot
3,06412949
3,06412949
add a comment |
add a comment |
up vote
4
down vote
You can either add it this way:
arr['key3'] = value3;
or this way:
arr.key3 = value3;
The answers suggesting keying into the object with the variable key3
would only work if the value of key3
was 'key3'
.
add a comment |
up vote
4
down vote
You can either add it this way:
arr['key3'] = value3;
or this way:
arr.key3 = value3;
The answers suggesting keying into the object with the variable key3
would only work if the value of key3
was 'key3'
.
add a comment |
up vote
4
down vote
up vote
4
down vote
You can either add it this way:
arr['key3'] = value3;
or this way:
arr.key3 = value3;
The answers suggesting keying into the object with the variable key3
would only work if the value of key3
was 'key3'
.
You can either add it this way:
arr['key3'] = value3;
or this way:
arr.key3 = value3;
The answers suggesting keying into the object with the variable key3
would only work if the value of key3
was 'key3'
.
answered Jul 22 '09 at 23:33
wombleton
7,71312229
7,71312229
add a comment |
add a comment |
up vote
4
down vote
According to Property Accessors defined in ECMA-262(http://www.ecma-international.org/publications/files/ECMA-ST/Ecma-262.pdf, P67), there are two ways you can do to add properties to a exists object. All these two way, the Javascript engine will treat them the same.
The first way is to use dot notation:
obj.key3 = value3;
But this way, you should use a IdentifierName after dot notation.
The second way is to use bracket notation:
obj["key3"] = value3;
and another form:
var key3 = "key3";
obj[key3] = value3;
This way, you could use a Expression (include IdentifierName) in the bracket notation.
add a comment |
up vote
4
down vote
According to Property Accessors defined in ECMA-262(http://www.ecma-international.org/publications/files/ECMA-ST/Ecma-262.pdf, P67), there are two ways you can do to add properties to a exists object. All these two way, the Javascript engine will treat them the same.
The first way is to use dot notation:
obj.key3 = value3;
But this way, you should use a IdentifierName after dot notation.
The second way is to use bracket notation:
obj["key3"] = value3;
and another form:
var key3 = "key3";
obj[key3] = value3;
This way, you could use a Expression (include IdentifierName) in the bracket notation.
add a comment |
up vote
4
down vote
up vote
4
down vote
According to Property Accessors defined in ECMA-262(http://www.ecma-international.org/publications/files/ECMA-ST/Ecma-262.pdf, P67), there are two ways you can do to add properties to a exists object. All these two way, the Javascript engine will treat them the same.
The first way is to use dot notation:
obj.key3 = value3;
But this way, you should use a IdentifierName after dot notation.
The second way is to use bracket notation:
obj["key3"] = value3;
and another form:
var key3 = "key3";
obj[key3] = value3;
This way, you could use a Expression (include IdentifierName) in the bracket notation.
According to Property Accessors defined in ECMA-262(http://www.ecma-international.org/publications/files/ECMA-ST/Ecma-262.pdf, P67), there are two ways you can do to add properties to a exists object. All these two way, the Javascript engine will treat them the same.
The first way is to use dot notation:
obj.key3 = value3;
But this way, you should use a IdentifierName after dot notation.
The second way is to use bracket notation:
obj["key3"] = value3;
and another form:
var key3 = "key3";
obj[key3] = value3;
This way, you could use a Expression (include IdentifierName) in the bracket notation.
answered Aug 2 '13 at 3:51
Chen Yang
15911
15911
add a comment |
add a comment |
up vote
3
down vote
A short and elegant way in next Javascript specification (candidate stage 3) is:
obj = { ... obj, ... { key3 : value3 } }
A deeper discussion can be found in Object spread vs Object.assign and on Dr. Axel Rauschmayers site.
It works already in node.js since release 8.6.0.
Vivaldi, Chrome, Opera, and Firefox in up to date releases know this feature also, but Mirosoft don't until today, neither in Internet Explorer nor in Edge.
add a comment |
up vote
3
down vote
A short and elegant way in next Javascript specification (candidate stage 3) is:
obj = { ... obj, ... { key3 : value3 } }
A deeper discussion can be found in Object spread vs Object.assign and on Dr. Axel Rauschmayers site.
It works already in node.js since release 8.6.0.
Vivaldi, Chrome, Opera, and Firefox in up to date releases know this feature also, but Mirosoft don't until today, neither in Internet Explorer nor in Edge.
add a comment |
up vote
3
down vote
up vote
3
down vote
A short and elegant way in next Javascript specification (candidate stage 3) is:
obj = { ... obj, ... { key3 : value3 } }
A deeper discussion can be found in Object spread vs Object.assign and on Dr. Axel Rauschmayers site.
It works already in node.js since release 8.6.0.
Vivaldi, Chrome, Opera, and Firefox in up to date releases know this feature also, but Mirosoft don't until today, neither in Internet Explorer nor in Edge.
A short and elegant way in next Javascript specification (candidate stage 3) is:
obj = { ... obj, ... { key3 : value3 } }
A deeper discussion can be found in Object spread vs Object.assign and on Dr. Axel Rauschmayers site.
It works already in node.js since release 8.6.0.
Vivaldi, Chrome, Opera, and Firefox in up to date releases know this feature also, but Mirosoft don't until today, neither in Internet Explorer nor in Edge.
edited Dec 4 '17 at 19:00
answered Nov 25 '17 at 10:17


xyxyber
515
515
add a comment |
add a comment |
up vote
3
down vote
var arrOfObj = [{name: 'eve'},{name:'john'},{name:'jane'}];
var injectObj = {isActive:true, timestamp:new Date()};
// function to inject key values in all object of json array
function injectKeyValueInArray (array, keyValues){
return new Promise((resolve, reject) => {
if (!array.length)
return resolve(array);
array.forEach((object) => {
for (let key in keyValues) {
object[key] = keyValues[key]
}
});
resolve(array);
})
};
//call function to inject json key value in all array object
injectKeyValueInArray(arrOfObj,injectObj).then((newArrOfObj)=>{
console.log(newArrOfObj);
});
Output like this:-
[ { name: 'eve',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z },
{ name: 'john',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z },
{ name: 'jane',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z } ]
add a comment |
up vote
3
down vote
var arrOfObj = [{name: 'eve'},{name:'john'},{name:'jane'}];
var injectObj = {isActive:true, timestamp:new Date()};
// function to inject key values in all object of json array
function injectKeyValueInArray (array, keyValues){
return new Promise((resolve, reject) => {
if (!array.length)
return resolve(array);
array.forEach((object) => {
for (let key in keyValues) {
object[key] = keyValues[key]
}
});
resolve(array);
})
};
//call function to inject json key value in all array object
injectKeyValueInArray(arrOfObj,injectObj).then((newArrOfObj)=>{
console.log(newArrOfObj);
});
Output like this:-
[ { name: 'eve',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z },
{ name: 'john',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z },
{ name: 'jane',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z } ]
add a comment |
up vote
3
down vote
up vote
3
down vote
var arrOfObj = [{name: 'eve'},{name:'john'},{name:'jane'}];
var injectObj = {isActive:true, timestamp:new Date()};
// function to inject key values in all object of json array
function injectKeyValueInArray (array, keyValues){
return new Promise((resolve, reject) => {
if (!array.length)
return resolve(array);
array.forEach((object) => {
for (let key in keyValues) {
object[key] = keyValues[key]
}
});
resolve(array);
})
};
//call function to inject json key value in all array object
injectKeyValueInArray(arrOfObj,injectObj).then((newArrOfObj)=>{
console.log(newArrOfObj);
});
Output like this:-
[ { name: 'eve',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z },
{ name: 'john',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z },
{ name: 'jane',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z } ]
var arrOfObj = [{name: 'eve'},{name:'john'},{name:'jane'}];
var injectObj = {isActive:true, timestamp:new Date()};
// function to inject key values in all object of json array
function injectKeyValueInArray (array, keyValues){
return new Promise((resolve, reject) => {
if (!array.length)
return resolve(array);
array.forEach((object) => {
for (let key in keyValues) {
object[key] = keyValues[key]
}
});
resolve(array);
})
};
//call function to inject json key value in all array object
injectKeyValueInArray(arrOfObj,injectObj).then((newArrOfObj)=>{
console.log(newArrOfObj);
});
Output like this:-
[ { name: 'eve',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z },
{ name: 'john',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z },
{ name: 'jane',
isActive: true,
timestamp: 2017-12-16T16:03:53.083Z } ]
answered Dec 16 '17 at 16:10
Sayed Tauseef Haider Naqvi
128311
128311
add a comment |
add a comment |
up vote
2
down vote
We can do this in this way too.
var myMap = new Map();
myMap.set(0, 'my value1');
myMap.set(1, 'my value2');
for (var [key, value] of myMap) {
console.log(key + ' = ' + value);
}
add a comment |
up vote
2
down vote
We can do this in this way too.
var myMap = new Map();
myMap.set(0, 'my value1');
myMap.set(1, 'my value2');
for (var [key, value] of myMap) {
console.log(key + ' = ' + value);
}
add a comment |
up vote
2
down vote
up vote
2
down vote
We can do this in this way too.
var myMap = new Map();
myMap.set(0, 'my value1');
myMap.set(1, 'my value2');
for (var [key, value] of myMap) {
console.log(key + ' = ' + value);
}
We can do this in this way too.
var myMap = new Map();
myMap.set(0, 'my value1');
myMap.set(1, 'my value2');
for (var [key, value] of myMap) {
console.log(key + ' = ' + value);
}
answered Sep 7 '17 at 3:36


Miraj Hamid
163313
163313
add a comment |
add a comment |
up vote
1
down vote
Since its a question of the past but the problem of present. Would suggest one more solution: Just pass the key and values to the function and you will get a map object.
var map = {};
function addValueToMap(key, value) {
map[key] = map[key] || ;
map[key].push(value);
}
2
This doesn't seem to relate to the question that was asked at all.
– Quentin
Feb 16 at 11:15
add a comment |
up vote
1
down vote
Since its a question of the past but the problem of present. Would suggest one more solution: Just pass the key and values to the function and you will get a map object.
var map = {};
function addValueToMap(key, value) {
map[key] = map[key] || ;
map[key].push(value);
}
2
This doesn't seem to relate to the question that was asked at all.
– Quentin
Feb 16 at 11:15
add a comment |
up vote
1
down vote
up vote
1
down vote
Since its a question of the past but the problem of present. Would suggest one more solution: Just pass the key and values to the function and you will get a map object.
var map = {};
function addValueToMap(key, value) {
map[key] = map[key] || ;
map[key].push(value);
}
Since its a question of the past but the problem of present. Would suggest one more solution: Just pass the key and values to the function and you will get a map object.
var map = {};
function addValueToMap(key, value) {
map[key] = map[key] || ;
map[key].push(value);
}
edited Feb 16 at 11:42
answered Feb 16 at 11:10
PPing
495
495
2
This doesn't seem to relate to the question that was asked at all.
– Quentin
Feb 16 at 11:15
add a comment |
2
This doesn't seem to relate to the question that was asked at all.
– Quentin
Feb 16 at 11:15
2
2
This doesn't seem to relate to the question that was asked at all.
– Quentin
Feb 16 at 11:15
This doesn't seem to relate to the question that was asked at all.
– Quentin
Feb 16 at 11:15
add a comment |
up vote
0
down vote
In order to prepend a key-value pair to an object so the for in works with that element first do this:
var nwrow = {'newkey': 'value' };
for(var column in row){
nwrow[column] = row[column];
}
row = nwrow;
add a comment |
up vote
0
down vote
In order to prepend a key-value pair to an object so the for in works with that element first do this:
var nwrow = {'newkey': 'value' };
for(var column in row){
nwrow[column] = row[column];
}
row = nwrow;
add a comment |
up vote
0
down vote
up vote
0
down vote
In order to prepend a key-value pair to an object so the for in works with that element first do this:
var nwrow = {'newkey': 'value' };
for(var column in row){
nwrow[column] = row[column];
}
row = nwrow;
In order to prepend a key-value pair to an object so the for in works with that element first do this:
var nwrow = {'newkey': 'value' };
for(var column in row){
nwrow[column] = row[column];
}
row = nwrow;
answered Dec 8 '16 at 22:24
relipse
65511019
65511019
add a comment |
add a comment |
up vote
0
down vote
Best way to achieve same is stated below:
function getKey(key) {
return `${key}`;
}
var obj = {key1: "value1", key2: "value2", [getKey('key3')]: "value3"};
//console.log(obj);
add a comment |
up vote
0
down vote
Best way to achieve same is stated below:
function getKey(key) {
return `${key}`;
}
var obj = {key1: "value1", key2: "value2", [getKey('key3')]: "value3"};
//console.log(obj);
add a comment |
up vote
0
down vote
up vote
0
down vote
Best way to achieve same is stated below:
function getKey(key) {
return `${key}`;
}
var obj = {key1: "value1", key2: "value2", [getKey('key3')]: "value3"};
//console.log(obj);
Best way to achieve same is stated below:
function getKey(key) {
return `${key}`;
}
var obj = {key1: "value1", key2: "value2", [getKey('key3')]: "value3"};
//console.log(obj);
answered Nov 21 '17 at 4:19


sgajera
1009
1009
add a comment |
add a comment |
up vote
0
down vote
Simply adding properties:
And we want to add prop2 : 2
to this object, these are the most convenient options:
- Dot operator:
object.prop2 = 2;
- square brackets:
object['prop2'] = 2;
So which one do we use then?
The dot operator is more clean syntax and should be used as a default (imo). However, the dot operator is not capable of adding dynamic keys to an object, which can be very useful in some cases. Here is an example:
const obj = {
prop1: 1
}
const key = Math.random() > 0.5 ? 'key1' : 'key2';
obj[key] = 'this value has a dynamic key';
console.log(obj);
Merging objects:
When we want to merge the properties of 2 objects these are the most convenient options:
Object.assign()
, takes a target object as an argument, and one or more source objects and will merge them together. For example:
const object1 = {
a: 1,
b: 2,
};
const object2 = Object.assign({
c: 3,
d: 4
}, object1);
console.log(object2);
- Object spread operator
...
const obj = {
prop1: 1,
prop2: 2
}
const newObj = {
...obj,
prop3: 3,
prop4: 4
}
console.log(newObj);
Which one do we use?
- The spread syntax is less verbose and has should be used as a default imo. Don't forgot to transpile this syntax to syntax which is supported by all browsers because it is relatively new.
Object.assign()
is more dynamic because we have access to all objects which are passed in as arguments and can manipulate them before they get assigned to the new Object.
1
At the top of your post: those aren't curly brackets but square brackets.
– Bram Vanroy
Aug 23 at 18:58
Thanks for pointing out ;)
– Willem van der Veen
Aug 23 at 20:01
add a comment |
up vote
0
down vote
Simply adding properties:
And we want to add prop2 : 2
to this object, these are the most convenient options:
- Dot operator:
object.prop2 = 2;
- square brackets:
object['prop2'] = 2;
So which one do we use then?
The dot operator is more clean syntax and should be used as a default (imo). However, the dot operator is not capable of adding dynamic keys to an object, which can be very useful in some cases. Here is an example:
const obj = {
prop1: 1
}
const key = Math.random() > 0.5 ? 'key1' : 'key2';
obj[key] = 'this value has a dynamic key';
console.log(obj);
Merging objects:
When we want to merge the properties of 2 objects these are the most convenient options:
Object.assign()
, takes a target object as an argument, and one or more source objects and will merge them together. For example:
const object1 = {
a: 1,
b: 2,
};
const object2 = Object.assign({
c: 3,
d: 4
}, object1);
console.log(object2);
- Object spread operator
...
const obj = {
prop1: 1,
prop2: 2
}
const newObj = {
...obj,
prop3: 3,
prop4: 4
}
console.log(newObj);
Which one do we use?
- The spread syntax is less verbose and has should be used as a default imo. Don't forgot to transpile this syntax to syntax which is supported by all browsers because it is relatively new.
Object.assign()
is more dynamic because we have access to all objects which are passed in as arguments and can manipulate them before they get assigned to the new Object.
1
At the top of your post: those aren't curly brackets but square brackets.
– Bram Vanroy
Aug 23 at 18:58
Thanks for pointing out ;)
– Willem van der Veen
Aug 23 at 20:01
add a comment |
up vote
0
down vote
up vote
0
down vote
Simply adding properties:
And we want to add prop2 : 2
to this object, these are the most convenient options:
- Dot operator:
object.prop2 = 2;
- square brackets:
object['prop2'] = 2;
So which one do we use then?
The dot operator is more clean syntax and should be used as a default (imo). However, the dot operator is not capable of adding dynamic keys to an object, which can be very useful in some cases. Here is an example:
const obj = {
prop1: 1
}
const key = Math.random() > 0.5 ? 'key1' : 'key2';
obj[key] = 'this value has a dynamic key';
console.log(obj);
Merging objects:
When we want to merge the properties of 2 objects these are the most convenient options:
Object.assign()
, takes a target object as an argument, and one or more source objects and will merge them together. For example:
const object1 = {
a: 1,
b: 2,
};
const object2 = Object.assign({
c: 3,
d: 4
}, object1);
console.log(object2);
- Object spread operator
...
const obj = {
prop1: 1,
prop2: 2
}
const newObj = {
...obj,
prop3: 3,
prop4: 4
}
console.log(newObj);
Which one do we use?
- The spread syntax is less verbose and has should be used as a default imo. Don't forgot to transpile this syntax to syntax which is supported by all browsers because it is relatively new.
Object.assign()
is more dynamic because we have access to all objects which are passed in as arguments and can manipulate them before they get assigned to the new Object.
Simply adding properties:
And we want to add prop2 : 2
to this object, these are the most convenient options:
- Dot operator:
object.prop2 = 2;
- square brackets:
object['prop2'] = 2;
So which one do we use then?
The dot operator is more clean syntax and should be used as a default (imo). However, the dot operator is not capable of adding dynamic keys to an object, which can be very useful in some cases. Here is an example:
const obj = {
prop1: 1
}
const key = Math.random() > 0.5 ? 'key1' : 'key2';
obj[key] = 'this value has a dynamic key';
console.log(obj);
Merging objects:
When we want to merge the properties of 2 objects these are the most convenient options:
Object.assign()
, takes a target object as an argument, and one or more source objects and will merge them together. For example:
const object1 = {
a: 1,
b: 2,
};
const object2 = Object.assign({
c: 3,
d: 4
}, object1);
console.log(object2);
- Object spread operator
...
const obj = {
prop1: 1,
prop2: 2
}
const newObj = {
...obj,
prop3: 3,
prop4: 4
}
console.log(newObj);
Which one do we use?
- The spread syntax is less verbose and has should be used as a default imo. Don't forgot to transpile this syntax to syntax which is supported by all browsers because it is relatively new.
Object.assign()
is more dynamic because we have access to all objects which are passed in as arguments and can manipulate them before they get assigned to the new Object.
const obj = {
prop1: 1
}
const key = Math.random() > 0.5 ? 'key1' : 'key2';
obj[key] = 'this value has a dynamic key';
console.log(obj);
const obj = {
prop1: 1
}
const key = Math.random() > 0.5 ? 'key1' : 'key2';
obj[key] = 'this value has a dynamic key';
console.log(obj);
const object1 = {
a: 1,
b: 2,
};
const object2 = Object.assign({
c: 3,
d: 4
}, object1);
console.log(object2);
const object1 = {
a: 1,
b: 2,
};
const object2 = Object.assign({
c: 3,
d: 4
}, object1);
console.log(object2);
const obj = {
prop1: 1,
prop2: 2
}
const newObj = {
...obj,
prop3: 3,
prop4: 4
}
console.log(newObj);
const obj = {
prop1: 1,
prop2: 2
}
const newObj = {
...obj,
prop3: 3,
prop4: 4
}
console.log(newObj);
edited Aug 23 at 20:01
answered Aug 16 at 9:15


Willem van der Veen
3,62031223
3,62031223
1
At the top of your post: those aren't curly brackets but square brackets.
– Bram Vanroy
Aug 23 at 18:58
Thanks for pointing out ;)
– Willem van der Veen
Aug 23 at 20:01
add a comment |
1
At the top of your post: those aren't curly brackets but square brackets.
– Bram Vanroy
Aug 23 at 18:58
Thanks for pointing out ;)
– Willem van der Veen
Aug 23 at 20:01
1
1
At the top of your post: those aren't curly brackets but square brackets.
– Bram Vanroy
Aug 23 at 18:58
At the top of your post: those aren't curly brackets but square brackets.
– Bram Vanroy
Aug 23 at 18:58
Thanks for pointing out ;)
– Willem van der Veen
Aug 23 at 20:01
Thanks for pointing out ;)
– Willem van der Veen
Aug 23 at 20:01
add a comment |
up vote
0
down vote
supported by most of browsers, and it checks if object key available or not you want to add, if available it overides existing key value and it not available it add key with value
example 1
let my_object = {};
// now i want to add something in it
my_object.red = "this is red color";
// { red : "this is red color"}
example 2
let my_object = { inside_object : { car : "maruti" }}
// now i want to add something inside object of my object
my_object.inside_object.plane = "JetKing";
// { inside_object : { car : "maruti" , plane : "JetKing"} }
example 3
let my_object = { inside_object : { name : "abhishek" }}
// now i want to add something inside object with new keys birth , gender
my_object.inside_object.birth = "8 Aug";
my_object.inside_object.gender = "Male";
// { inside_object :
// { name : "abhishek",
// birth : "8 Aug",
// gender : "Male"
// }
// }
add a comment |
up vote
0
down vote
supported by most of browsers, and it checks if object key available or not you want to add, if available it overides existing key value and it not available it add key with value
example 1
let my_object = {};
// now i want to add something in it
my_object.red = "this is red color";
// { red : "this is red color"}
example 2
let my_object = { inside_object : { car : "maruti" }}
// now i want to add something inside object of my object
my_object.inside_object.plane = "JetKing";
// { inside_object : { car : "maruti" , plane : "JetKing"} }
example 3
let my_object = { inside_object : { name : "abhishek" }}
// now i want to add something inside object with new keys birth , gender
my_object.inside_object.birth = "8 Aug";
my_object.inside_object.gender = "Male";
// { inside_object :
// { name : "abhishek",
// birth : "8 Aug",
// gender : "Male"
// }
// }
add a comment |
up vote
0
down vote
up vote
0
down vote
supported by most of browsers, and it checks if object key available or not you want to add, if available it overides existing key value and it not available it add key with value
example 1
let my_object = {};
// now i want to add something in it
my_object.red = "this is red color";
// { red : "this is red color"}
example 2
let my_object = { inside_object : { car : "maruti" }}
// now i want to add something inside object of my object
my_object.inside_object.plane = "JetKing";
// { inside_object : { car : "maruti" , plane : "JetKing"} }
example 3
let my_object = { inside_object : { name : "abhishek" }}
// now i want to add something inside object with new keys birth , gender
my_object.inside_object.birth = "8 Aug";
my_object.inside_object.gender = "Male";
// { inside_object :
// { name : "abhishek",
// birth : "8 Aug",
// gender : "Male"
// }
// }
supported by most of browsers, and it checks if object key available or not you want to add, if available it overides existing key value and it not available it add key with value
example 1
let my_object = {};
// now i want to add something in it
my_object.red = "this is red color";
// { red : "this is red color"}
example 2
let my_object = { inside_object : { car : "maruti" }}
// now i want to add something inside object of my object
my_object.inside_object.plane = "JetKing";
// { inside_object : { car : "maruti" , plane : "JetKing"} }
example 3
let my_object = { inside_object : { name : "abhishek" }}
// now i want to add something inside object with new keys birth , gender
my_object.inside_object.birth = "8 Aug";
my_object.inside_object.gender = "Male";
// { inside_object :
// { name : "abhishek",
// birth : "8 Aug",
// gender : "Male"
// }
// }
answered 2 days ago


Abhishek Garg
1267
1267
add a comment |
add a comment |
up vote
-17
down vote
arr.push({key3: value3});
4
This is wrong. That will add to an array, not to an object. You won't be able to reference the value using the key. Not directly, anyway.
– Matthew
Jan 26 '12 at 18:50
Wrong answer,push
is one ofArray
functions, notObject
.
– Afshin Mehrabani
Nov 21 '12 at 7:31
add a comment |
up vote
-17
down vote
arr.push({key3: value3});
4
This is wrong. That will add to an array, not to an object. You won't be able to reference the value using the key. Not directly, anyway.
– Matthew
Jan 26 '12 at 18:50
Wrong answer,push
is one ofArray
functions, notObject
.
– Afshin Mehrabani
Nov 21 '12 at 7:31
add a comment |
up vote
-17
down vote
up vote
-17
down vote
arr.push({key3: value3});
arr.push({key3: value3});
answered Jul 22 '09 at 23:23


dfa
92.3k28169217
92.3k28169217
4
This is wrong. That will add to an array, not to an object. You won't be able to reference the value using the key. Not directly, anyway.
– Matthew
Jan 26 '12 at 18:50
Wrong answer,push
is one ofArray
functions, notObject
.
– Afshin Mehrabani
Nov 21 '12 at 7:31
add a comment |
4
This is wrong. That will add to an array, not to an object. You won't be able to reference the value using the key. Not directly, anyway.
– Matthew
Jan 26 '12 at 18:50
Wrong answer,push
is one ofArray
functions, notObject
.
– Afshin Mehrabani
Nov 21 '12 at 7:31
4
4
This is wrong. That will add to an array, not to an object. You won't be able to reference the value using the key. Not directly, anyway.
– Matthew
Jan 26 '12 at 18:50
This is wrong. That will add to an array, not to an object. You won't be able to reference the value using the key. Not directly, anyway.
– Matthew
Jan 26 '12 at 18:50
Wrong answer,
push
is one of Array
functions, not Object
.– Afshin Mehrabani
Nov 21 '12 at 7:31
Wrong answer,
push
is one of Array
functions, not Object
.– Afshin Mehrabani
Nov 21 '12 at 7:31
add a comment |
protected by Tushar Gupta Jul 27 '14 at 14:48
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
HUAJDRx1CdcvM RF h lRuE8kTDnMwk,WFQ,XDOVkk9VTY gnoje D,3R1D
1
Well, the whole issue of associative arrays in JS is weird, because you can do this... dreaminginjavascript.wordpress.com/2008/06/27/…
– Nosredna
Jul 22 '09 at 23:48
@Nosredna - the point is, there are no such things as associative arrays in javascript. In that article he is adding object properties to an array object, but these are not really part of the 'array'.
– UpTheCreek
Aug 30 '12 at 9:34
Is there a way to conditionally set key:value pairs in an object literal with future ES+ implementations?
– Con Antonakos
Apr 1 '16 at 19:00