Update Product Quantity with Ajax
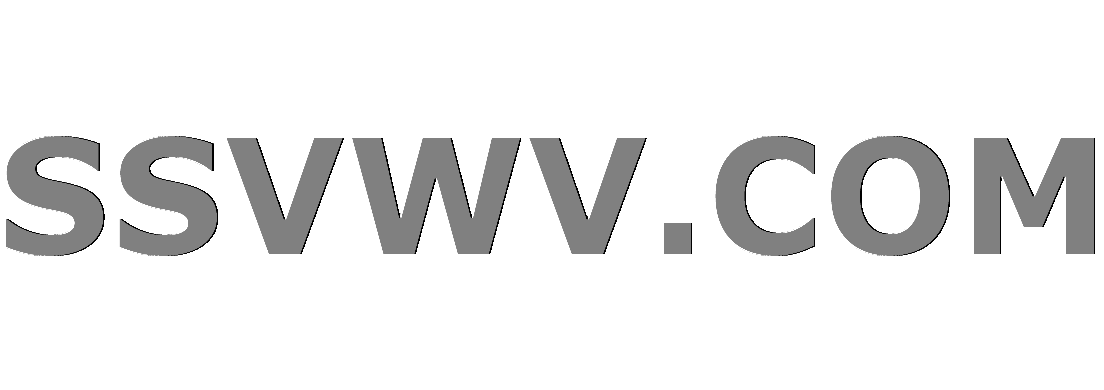
Multi tool use
up vote
3
down vote
favorite
So, on my Shopify site, I've got a plus/minus button to update the quantity of a product. Currently, it submits the form when clicking either of those buttons. Now I'm implementing a drawer cart using ajax though, so instead of just submitting the form and reloading the page when either of those buttons is clicked, I need to update the cart with Ajax, so it doesn't need a page to reload.
This is the code for my quantity buttons:
// This button will increment the value
$('.ajax-cart-form').on("click",'.qtyplus.qtyplus-2', function(){
// Stop acting like a button
// Get the field name
fieldName = $(this).attr('field');
// Get its current value
var currentVal = parseInt($('input[id='+fieldName+']').val());
// If is not undefined
if (!isNaN(currentVal)) {
// Increment
$('input[id='+fieldName+']').val(currentVal + 1);
} else {
// Otherwise put a 0 there
$('input[id='+fieldName+']').val(0);
}
$(this).closest('form').submit();
});
// This button will decrement the value till 0
$(".ajax-cart-form").on("click", '.qtyminus.qtyminus-2' ,function() {
// Stop acting like a button
// Get the field name
fieldName = $(this).attr('field');
// Get its current value
var currentVal = parseInt($('input[id='+fieldName+']').val());
// If it isn't undefined or its greater than 0
if (!isNaN(currentVal) && currentVal > 0) {
// Decrement one
$('input[id='+fieldName+']').val(currentVal - 1);
} else {
// Otherwise put a 0 there
$('input[id='+fieldName+']').val(0);
}
$(this).closest('form').submit();
});
And this is the append function to display my ajax cart:
$.getJSON(_config.shopifyAjaxCartURL, function(cart) {
$('.ajax-cart-form .cart-item').remove();
$('.ajax-subtotal .drawer-subtotal').remove();
$.each(cart.items, function(index, cartItem) {
var line= index +1;
var cents = "";
if (cartItem.price % 100 < 10) {
cents = "0";
}
var price = parseInt(cartItem.price/100) + "." + cents + cartItem.price % 100;
$('.ajax-cart-form').append("<div class='cart-item desktop-full mobile-full'><div class='cart-image desktop-5'><a href='"+ cartItem.url +"'title='"+cartItem.title+"'><img src='"+ cartItem.image +"'></a></div><div class='cart-title desktop-7'><p>"+ cartItem.title +"</p><div class='cart-item-actions'><span class='drawer-price'><strong>£ "+ price +" GBP</strong></span></div><div class='cart-quantity'><input type='button' value='-' class='qtyminus qtyminus-2 CartCount qty-btn' field='updates_"+ cartItem.id +"'/><input name='updates' id='updates_"+ cartItem.id +"' class='quantity CartCount' value='"+ cartItem.quantity +"' /><input type='button' value='+' class='qtyplus qtyplus-2 CartCount qty-btn' field='updates_"+ cartItem.id +"' /></div></div>");
});
$(function(index, cartItem) {
var line2= index +1;
var cents2 = "";
if (cart.original_total_price % 100 < 10) {
cents2 = "0";
}
var price2 = parseInt(cart.original_total_price/100) + "." + cents2 + cart.original_total_price % 100;
$('.ajax-subtotal').append("<div class='drawer-subtotal'><h3>Subtotal <span class='money'>£"+ price2 +" GBP</span></h3></div>");
});
});
I guess it'd be something like
jQuery.post('/cart/update.js', {updates: {794864053: 2, 794864233: 3}});
But not sure how to implement this with my plus/minus function. Any ideas on how to do this?
javascript ajax
add a comment |
up vote
3
down vote
favorite
So, on my Shopify site, I've got a plus/minus button to update the quantity of a product. Currently, it submits the form when clicking either of those buttons. Now I'm implementing a drawer cart using ajax though, so instead of just submitting the form and reloading the page when either of those buttons is clicked, I need to update the cart with Ajax, so it doesn't need a page to reload.
This is the code for my quantity buttons:
// This button will increment the value
$('.ajax-cart-form').on("click",'.qtyplus.qtyplus-2', function(){
// Stop acting like a button
// Get the field name
fieldName = $(this).attr('field');
// Get its current value
var currentVal = parseInt($('input[id='+fieldName+']').val());
// If is not undefined
if (!isNaN(currentVal)) {
// Increment
$('input[id='+fieldName+']').val(currentVal + 1);
} else {
// Otherwise put a 0 there
$('input[id='+fieldName+']').val(0);
}
$(this).closest('form').submit();
});
// This button will decrement the value till 0
$(".ajax-cart-form").on("click", '.qtyminus.qtyminus-2' ,function() {
// Stop acting like a button
// Get the field name
fieldName = $(this).attr('field');
// Get its current value
var currentVal = parseInt($('input[id='+fieldName+']').val());
// If it isn't undefined or its greater than 0
if (!isNaN(currentVal) && currentVal > 0) {
// Decrement one
$('input[id='+fieldName+']').val(currentVal - 1);
} else {
// Otherwise put a 0 there
$('input[id='+fieldName+']').val(0);
}
$(this).closest('form').submit();
});
And this is the append function to display my ajax cart:
$.getJSON(_config.shopifyAjaxCartURL, function(cart) {
$('.ajax-cart-form .cart-item').remove();
$('.ajax-subtotal .drawer-subtotal').remove();
$.each(cart.items, function(index, cartItem) {
var line= index +1;
var cents = "";
if (cartItem.price % 100 < 10) {
cents = "0";
}
var price = parseInt(cartItem.price/100) + "." + cents + cartItem.price % 100;
$('.ajax-cart-form').append("<div class='cart-item desktop-full mobile-full'><div class='cart-image desktop-5'><a href='"+ cartItem.url +"'title='"+cartItem.title+"'><img src='"+ cartItem.image +"'></a></div><div class='cart-title desktop-7'><p>"+ cartItem.title +"</p><div class='cart-item-actions'><span class='drawer-price'><strong>£ "+ price +" GBP</strong></span></div><div class='cart-quantity'><input type='button' value='-' class='qtyminus qtyminus-2 CartCount qty-btn' field='updates_"+ cartItem.id +"'/><input name='updates' id='updates_"+ cartItem.id +"' class='quantity CartCount' value='"+ cartItem.quantity +"' /><input type='button' value='+' class='qtyplus qtyplus-2 CartCount qty-btn' field='updates_"+ cartItem.id +"' /></div></div>");
});
$(function(index, cartItem) {
var line2= index +1;
var cents2 = "";
if (cart.original_total_price % 100 < 10) {
cents2 = "0";
}
var price2 = parseInt(cart.original_total_price/100) + "." + cents2 + cart.original_total_price % 100;
$('.ajax-subtotal').append("<div class='drawer-subtotal'><h3>Subtotal <span class='money'>£"+ price2 +" GBP</span></h3></div>");
});
});
I guess it'd be something like
jQuery.post('/cart/update.js', {updates: {794864053: 2, 794864233: 3}});
But not sure how to implement this with my plus/minus function. Any ideas on how to do this?
javascript ajax
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
So, on my Shopify site, I've got a plus/minus button to update the quantity of a product. Currently, it submits the form when clicking either of those buttons. Now I'm implementing a drawer cart using ajax though, so instead of just submitting the form and reloading the page when either of those buttons is clicked, I need to update the cart with Ajax, so it doesn't need a page to reload.
This is the code for my quantity buttons:
// This button will increment the value
$('.ajax-cart-form').on("click",'.qtyplus.qtyplus-2', function(){
// Stop acting like a button
// Get the field name
fieldName = $(this).attr('field');
// Get its current value
var currentVal = parseInt($('input[id='+fieldName+']').val());
// If is not undefined
if (!isNaN(currentVal)) {
// Increment
$('input[id='+fieldName+']').val(currentVal + 1);
} else {
// Otherwise put a 0 there
$('input[id='+fieldName+']').val(0);
}
$(this).closest('form').submit();
});
// This button will decrement the value till 0
$(".ajax-cart-form").on("click", '.qtyminus.qtyminus-2' ,function() {
// Stop acting like a button
// Get the field name
fieldName = $(this).attr('field');
// Get its current value
var currentVal = parseInt($('input[id='+fieldName+']').val());
// If it isn't undefined or its greater than 0
if (!isNaN(currentVal) && currentVal > 0) {
// Decrement one
$('input[id='+fieldName+']').val(currentVal - 1);
} else {
// Otherwise put a 0 there
$('input[id='+fieldName+']').val(0);
}
$(this).closest('form').submit();
});
And this is the append function to display my ajax cart:
$.getJSON(_config.shopifyAjaxCartURL, function(cart) {
$('.ajax-cart-form .cart-item').remove();
$('.ajax-subtotal .drawer-subtotal').remove();
$.each(cart.items, function(index, cartItem) {
var line= index +1;
var cents = "";
if (cartItem.price % 100 < 10) {
cents = "0";
}
var price = parseInt(cartItem.price/100) + "." + cents + cartItem.price % 100;
$('.ajax-cart-form').append("<div class='cart-item desktop-full mobile-full'><div class='cart-image desktop-5'><a href='"+ cartItem.url +"'title='"+cartItem.title+"'><img src='"+ cartItem.image +"'></a></div><div class='cart-title desktop-7'><p>"+ cartItem.title +"</p><div class='cart-item-actions'><span class='drawer-price'><strong>£ "+ price +" GBP</strong></span></div><div class='cart-quantity'><input type='button' value='-' class='qtyminus qtyminus-2 CartCount qty-btn' field='updates_"+ cartItem.id +"'/><input name='updates' id='updates_"+ cartItem.id +"' class='quantity CartCount' value='"+ cartItem.quantity +"' /><input type='button' value='+' class='qtyplus qtyplus-2 CartCount qty-btn' field='updates_"+ cartItem.id +"' /></div></div>");
});
$(function(index, cartItem) {
var line2= index +1;
var cents2 = "";
if (cart.original_total_price % 100 < 10) {
cents2 = "0";
}
var price2 = parseInt(cart.original_total_price/100) + "." + cents2 + cart.original_total_price % 100;
$('.ajax-subtotal').append("<div class='drawer-subtotal'><h3>Subtotal <span class='money'>£"+ price2 +" GBP</span></h3></div>");
});
});
I guess it'd be something like
jQuery.post('/cart/update.js', {updates: {794864053: 2, 794864233: 3}});
But not sure how to implement this with my plus/minus function. Any ideas on how to do this?
javascript ajax
So, on my Shopify site, I've got a plus/minus button to update the quantity of a product. Currently, it submits the form when clicking either of those buttons. Now I'm implementing a drawer cart using ajax though, so instead of just submitting the form and reloading the page when either of those buttons is clicked, I need to update the cart with Ajax, so it doesn't need a page to reload.
This is the code for my quantity buttons:
// This button will increment the value
$('.ajax-cart-form').on("click",'.qtyplus.qtyplus-2', function(){
// Stop acting like a button
// Get the field name
fieldName = $(this).attr('field');
// Get its current value
var currentVal = parseInt($('input[id='+fieldName+']').val());
// If is not undefined
if (!isNaN(currentVal)) {
// Increment
$('input[id='+fieldName+']').val(currentVal + 1);
} else {
// Otherwise put a 0 there
$('input[id='+fieldName+']').val(0);
}
$(this).closest('form').submit();
});
// This button will decrement the value till 0
$(".ajax-cart-form").on("click", '.qtyminus.qtyminus-2' ,function() {
// Stop acting like a button
// Get the field name
fieldName = $(this).attr('field');
// Get its current value
var currentVal = parseInt($('input[id='+fieldName+']').val());
// If it isn't undefined or its greater than 0
if (!isNaN(currentVal) && currentVal > 0) {
// Decrement one
$('input[id='+fieldName+']').val(currentVal - 1);
} else {
// Otherwise put a 0 there
$('input[id='+fieldName+']').val(0);
}
$(this).closest('form').submit();
});
And this is the append function to display my ajax cart:
$.getJSON(_config.shopifyAjaxCartURL, function(cart) {
$('.ajax-cart-form .cart-item').remove();
$('.ajax-subtotal .drawer-subtotal').remove();
$.each(cart.items, function(index, cartItem) {
var line= index +1;
var cents = "";
if (cartItem.price % 100 < 10) {
cents = "0";
}
var price = parseInt(cartItem.price/100) + "." + cents + cartItem.price % 100;
$('.ajax-cart-form').append("<div class='cart-item desktop-full mobile-full'><div class='cart-image desktop-5'><a href='"+ cartItem.url +"'title='"+cartItem.title+"'><img src='"+ cartItem.image +"'></a></div><div class='cart-title desktop-7'><p>"+ cartItem.title +"</p><div class='cart-item-actions'><span class='drawer-price'><strong>£ "+ price +" GBP</strong></span></div><div class='cart-quantity'><input type='button' value='-' class='qtyminus qtyminus-2 CartCount qty-btn' field='updates_"+ cartItem.id +"'/><input name='updates' id='updates_"+ cartItem.id +"' class='quantity CartCount' value='"+ cartItem.quantity +"' /><input type='button' value='+' class='qtyplus qtyplus-2 CartCount qty-btn' field='updates_"+ cartItem.id +"' /></div></div>");
});
$(function(index, cartItem) {
var line2= index +1;
var cents2 = "";
if (cart.original_total_price % 100 < 10) {
cents2 = "0";
}
var price2 = parseInt(cart.original_total_price/100) + "." + cents2 + cart.original_total_price % 100;
$('.ajax-subtotal').append("<div class='drawer-subtotal'><h3>Subtotal <span class='money'>£"+ price2 +" GBP</span></h3></div>");
});
});
I guess it'd be something like
jQuery.post('/cart/update.js', {updates: {794864053: 2, 794864233: 3}});
But not sure how to implement this with my plus/minus function. Any ideas on how to do this?
javascript ajax
javascript ajax
edited 2 days ago
Ab Majeed
172
172
asked 2 days ago
MariaL
326222
326222
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53372222%2fupdate-product-quantity-with-ajax%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
f74,t4m3eYjIeDGLU4Cj4QeEvjPieX