JQuery preflight request CORS
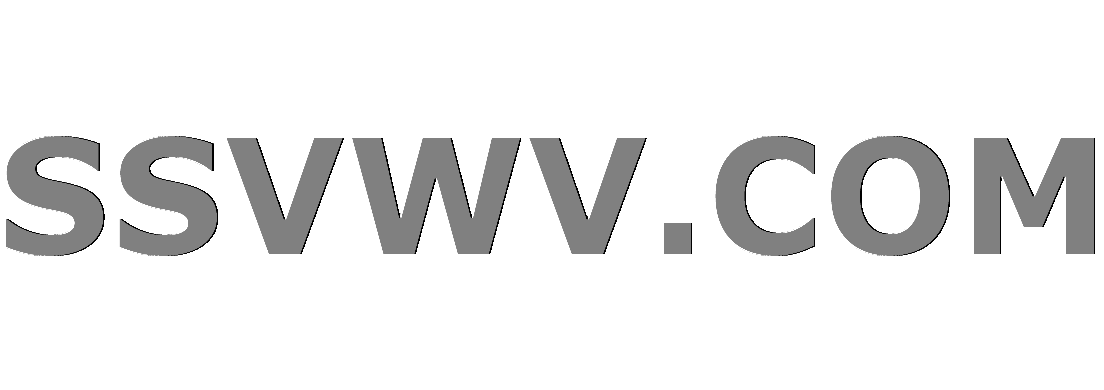
Multi tool use
up vote
0
down vote
favorite
I am writing a web map and try to get fetch a JSON from a web ressource I do have credentials for. This works great in Python running via a JuPyteR notebook:
import requests
header = { "Accept": "application/json, text/plain, */*",
"Accept-Language": "de-DE",
"Content-Type": "application/json",
"Pragma": "no-cache",
"Cache-Control": "no-cache",
"Origin": "https://myCoolServer.azurewebsites.net"}
}
dataJSON = json.dumps({ "username": "XXX",
"password": "XXX"}).encode('utf8')
url = "URL/v1/token"
r = requests.post(url, data=dataJSON, headers=header)
The request from python gives the following header:
'User-Agent': 'python-requests/2.19.1',
'Accept-Encoding': 'gzip, deflate',
'Accept': 'application/json, text/plain, */*',
'Connection': 'keep-alive',
'Accept-Language': 'de-DE',
'Content-Type': 'application/json',
'Pragma': 'no-cache',
'Cache-Control': 'no-cache',
'Content-Length': '67',
'Origin': 'https://myCoolServer.azurewebsites.net'
Now I am trying to recreate this in JavaScript to get data in my webmap:
$.ajax ({
method: 'POST',
data: JSON.stringify({ "username": name,
"password": pass}),
headers: {
"Accept": "application/json, text/plain, */*",
"Accept-Language": "de-DE",
"Content-Type": "application/json",
"Pragma": "no-cache",
"Cache-Control": "no-cache"
},
dataType:'json',
url:'URL/v1/token',
error: function() {
alert("Login failed. Check username/password!");
},
success: function(resp) {
token=resp.token;
}
The console shows the following header for the preflight Options call:
Host: "cool API"
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:63.0) Gecko/20100101 Firefox/63.0
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8
Accept-Language: de-DE
Accept-Encoding: gzip, deflate, br
Access-Control-Request-Method: POST
Access-Control-Request-Headers: cache-control,content-type,pragma
Origin: https://myCoolServer.azurewebsites.net
DNT: 1
Connection: keep-alive
Pragma: no-cache
Cache-Control: no-cache
The preflight Options response from the server is successfull in terms of a 200 response. Yet I don't get the POST finished as the console in FF and Chrome gives me:
Origin https://myCoolServer.azurewebsites.net not found in Access-Control-Allow-Origin header.
The response header from the Server gives me:
HTTP/1.1 200 OK
Date: Mon, 19 Nov 2018 08:07:30 GMT
Server: Apache/2.4.18 (Ubuntu)
Cache-Control: no-cache, private
Access-Control-Allow-Credentials: true
Access-Control-Allow-Methods: POST, PUT, GET, DELETE, OPTIONS
Access-Control-Allow-Headers: cache-control,content-type,pragma
Access-Control-Max-Age: 3600
Access-Control-Allow-Origin: null
Content-Length: 0
Keep-Alive: timeout=5, max=100
Connection: Keep-Alive
Content-Type: text/html; charset=UTF-8
As the whole idea works in Python I hope I can "mimicri" the behaviour in Javascript/Jquery as well. So I am looking for your remarks/hints.
Note: The whole thing works in JS when running on Chrome using a CORS plugin...
javascript python jquery cors
|
show 6 more comments
up vote
0
down vote
favorite
I am writing a web map and try to get fetch a JSON from a web ressource I do have credentials for. This works great in Python running via a JuPyteR notebook:
import requests
header = { "Accept": "application/json, text/plain, */*",
"Accept-Language": "de-DE",
"Content-Type": "application/json",
"Pragma": "no-cache",
"Cache-Control": "no-cache",
"Origin": "https://myCoolServer.azurewebsites.net"}
}
dataJSON = json.dumps({ "username": "XXX",
"password": "XXX"}).encode('utf8')
url = "URL/v1/token"
r = requests.post(url, data=dataJSON, headers=header)
The request from python gives the following header:
'User-Agent': 'python-requests/2.19.1',
'Accept-Encoding': 'gzip, deflate',
'Accept': 'application/json, text/plain, */*',
'Connection': 'keep-alive',
'Accept-Language': 'de-DE',
'Content-Type': 'application/json',
'Pragma': 'no-cache',
'Cache-Control': 'no-cache',
'Content-Length': '67',
'Origin': 'https://myCoolServer.azurewebsites.net'
Now I am trying to recreate this in JavaScript to get data in my webmap:
$.ajax ({
method: 'POST',
data: JSON.stringify({ "username": name,
"password": pass}),
headers: {
"Accept": "application/json, text/plain, */*",
"Accept-Language": "de-DE",
"Content-Type": "application/json",
"Pragma": "no-cache",
"Cache-Control": "no-cache"
},
dataType:'json',
url:'URL/v1/token',
error: function() {
alert("Login failed. Check username/password!");
},
success: function(resp) {
token=resp.token;
}
The console shows the following header for the preflight Options call:
Host: "cool API"
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:63.0) Gecko/20100101 Firefox/63.0
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8
Accept-Language: de-DE
Accept-Encoding: gzip, deflate, br
Access-Control-Request-Method: POST
Access-Control-Request-Headers: cache-control,content-type,pragma
Origin: https://myCoolServer.azurewebsites.net
DNT: 1
Connection: keep-alive
Pragma: no-cache
Cache-Control: no-cache
The preflight Options response from the server is successfull in terms of a 200 response. Yet I don't get the POST finished as the console in FF and Chrome gives me:
Origin https://myCoolServer.azurewebsites.net not found in Access-Control-Allow-Origin header.
The response header from the Server gives me:
HTTP/1.1 200 OK
Date: Mon, 19 Nov 2018 08:07:30 GMT
Server: Apache/2.4.18 (Ubuntu)
Cache-Control: no-cache, private
Access-Control-Allow-Credentials: true
Access-Control-Allow-Methods: POST, PUT, GET, DELETE, OPTIONS
Access-Control-Allow-Headers: cache-control,content-type,pragma
Access-Control-Max-Age: 3600
Access-Control-Allow-Origin: null
Content-Length: 0
Keep-Alive: timeout=5, max=100
Connection: Keep-Alive
Content-Type: text/html; charset=UTF-8
As the whole idea works in Python I hope I can "mimicri" the behaviour in Javascript/Jquery as well. So I am looking for your remarks/hints.
Note: The whole thing works in JS when running on Chrome using a CORS plugin...
javascript python jquery cors
1
Show your server CORS configuration - it looks like there is problem (inAccess-Control-Allow-Origin: null
)
– Kamil Kiełczewski
20 hours ago
then it shouldn't work in python on my local as well, should it?
– Riccardo
19 hours ago
it depends... on what is your origin for request's from python - the difference must be there
– Kamil Kiełczewski
19 hours ago
changed post for clarity
– Riccardo
19 hours ago
you show your ajax RESPONSE headers, but show your ajax REQUEST headers (chrome> console> networks> your request)
– Kamil Kiełczewski
19 hours ago
|
show 6 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am writing a web map and try to get fetch a JSON from a web ressource I do have credentials for. This works great in Python running via a JuPyteR notebook:
import requests
header = { "Accept": "application/json, text/plain, */*",
"Accept-Language": "de-DE",
"Content-Type": "application/json",
"Pragma": "no-cache",
"Cache-Control": "no-cache",
"Origin": "https://myCoolServer.azurewebsites.net"}
}
dataJSON = json.dumps({ "username": "XXX",
"password": "XXX"}).encode('utf8')
url = "URL/v1/token"
r = requests.post(url, data=dataJSON, headers=header)
The request from python gives the following header:
'User-Agent': 'python-requests/2.19.1',
'Accept-Encoding': 'gzip, deflate',
'Accept': 'application/json, text/plain, */*',
'Connection': 'keep-alive',
'Accept-Language': 'de-DE',
'Content-Type': 'application/json',
'Pragma': 'no-cache',
'Cache-Control': 'no-cache',
'Content-Length': '67',
'Origin': 'https://myCoolServer.azurewebsites.net'
Now I am trying to recreate this in JavaScript to get data in my webmap:
$.ajax ({
method: 'POST',
data: JSON.stringify({ "username": name,
"password": pass}),
headers: {
"Accept": "application/json, text/plain, */*",
"Accept-Language": "de-DE",
"Content-Type": "application/json",
"Pragma": "no-cache",
"Cache-Control": "no-cache"
},
dataType:'json',
url:'URL/v1/token',
error: function() {
alert("Login failed. Check username/password!");
},
success: function(resp) {
token=resp.token;
}
The console shows the following header for the preflight Options call:
Host: "cool API"
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:63.0) Gecko/20100101 Firefox/63.0
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8
Accept-Language: de-DE
Accept-Encoding: gzip, deflate, br
Access-Control-Request-Method: POST
Access-Control-Request-Headers: cache-control,content-type,pragma
Origin: https://myCoolServer.azurewebsites.net
DNT: 1
Connection: keep-alive
Pragma: no-cache
Cache-Control: no-cache
The preflight Options response from the server is successfull in terms of a 200 response. Yet I don't get the POST finished as the console in FF and Chrome gives me:
Origin https://myCoolServer.azurewebsites.net not found in Access-Control-Allow-Origin header.
The response header from the Server gives me:
HTTP/1.1 200 OK
Date: Mon, 19 Nov 2018 08:07:30 GMT
Server: Apache/2.4.18 (Ubuntu)
Cache-Control: no-cache, private
Access-Control-Allow-Credentials: true
Access-Control-Allow-Methods: POST, PUT, GET, DELETE, OPTIONS
Access-Control-Allow-Headers: cache-control,content-type,pragma
Access-Control-Max-Age: 3600
Access-Control-Allow-Origin: null
Content-Length: 0
Keep-Alive: timeout=5, max=100
Connection: Keep-Alive
Content-Type: text/html; charset=UTF-8
As the whole idea works in Python I hope I can "mimicri" the behaviour in Javascript/Jquery as well. So I am looking for your remarks/hints.
Note: The whole thing works in JS when running on Chrome using a CORS plugin...
javascript python jquery cors
I am writing a web map and try to get fetch a JSON from a web ressource I do have credentials for. This works great in Python running via a JuPyteR notebook:
import requests
header = { "Accept": "application/json, text/plain, */*",
"Accept-Language": "de-DE",
"Content-Type": "application/json",
"Pragma": "no-cache",
"Cache-Control": "no-cache",
"Origin": "https://myCoolServer.azurewebsites.net"}
}
dataJSON = json.dumps({ "username": "XXX",
"password": "XXX"}).encode('utf8')
url = "URL/v1/token"
r = requests.post(url, data=dataJSON, headers=header)
The request from python gives the following header:
'User-Agent': 'python-requests/2.19.1',
'Accept-Encoding': 'gzip, deflate',
'Accept': 'application/json, text/plain, */*',
'Connection': 'keep-alive',
'Accept-Language': 'de-DE',
'Content-Type': 'application/json',
'Pragma': 'no-cache',
'Cache-Control': 'no-cache',
'Content-Length': '67',
'Origin': 'https://myCoolServer.azurewebsites.net'
Now I am trying to recreate this in JavaScript to get data in my webmap:
$.ajax ({
method: 'POST',
data: JSON.stringify({ "username": name,
"password": pass}),
headers: {
"Accept": "application/json, text/plain, */*",
"Accept-Language": "de-DE",
"Content-Type": "application/json",
"Pragma": "no-cache",
"Cache-Control": "no-cache"
},
dataType:'json',
url:'URL/v1/token',
error: function() {
alert("Login failed. Check username/password!");
},
success: function(resp) {
token=resp.token;
}
The console shows the following header for the preflight Options call:
Host: "cool API"
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:63.0) Gecko/20100101 Firefox/63.0
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8
Accept-Language: de-DE
Accept-Encoding: gzip, deflate, br
Access-Control-Request-Method: POST
Access-Control-Request-Headers: cache-control,content-type,pragma
Origin: https://myCoolServer.azurewebsites.net
DNT: 1
Connection: keep-alive
Pragma: no-cache
Cache-Control: no-cache
The preflight Options response from the server is successfull in terms of a 200 response. Yet I don't get the POST finished as the console in FF and Chrome gives me:
Origin https://myCoolServer.azurewebsites.net not found in Access-Control-Allow-Origin header.
The response header from the Server gives me:
HTTP/1.1 200 OK
Date: Mon, 19 Nov 2018 08:07:30 GMT
Server: Apache/2.4.18 (Ubuntu)
Cache-Control: no-cache, private
Access-Control-Allow-Credentials: true
Access-Control-Allow-Methods: POST, PUT, GET, DELETE, OPTIONS
Access-Control-Allow-Headers: cache-control,content-type,pragma
Access-Control-Max-Age: 3600
Access-Control-Allow-Origin: null
Content-Length: 0
Keep-Alive: timeout=5, max=100
Connection: Keep-Alive
Content-Type: text/html; charset=UTF-8
As the whole idea works in Python I hope I can "mimicri" the behaviour in Javascript/Jquery as well. So I am looking for your remarks/hints.
Note: The whole thing works in JS when running on Chrome using a CORS plugin...
javascript python jquery cors
javascript python jquery cors
edited 19 hours ago
asked 20 hours ago


Riccardo
1066
1066
1
Show your server CORS configuration - it looks like there is problem (inAccess-Control-Allow-Origin: null
)
– Kamil Kiełczewski
20 hours ago
then it shouldn't work in python on my local as well, should it?
– Riccardo
19 hours ago
it depends... on what is your origin for request's from python - the difference must be there
– Kamil Kiełczewski
19 hours ago
changed post for clarity
– Riccardo
19 hours ago
you show your ajax RESPONSE headers, but show your ajax REQUEST headers (chrome> console> networks> your request)
– Kamil Kiełczewski
19 hours ago
|
show 6 more comments
1
Show your server CORS configuration - it looks like there is problem (inAccess-Control-Allow-Origin: null
)
– Kamil Kiełczewski
20 hours ago
then it shouldn't work in python on my local as well, should it?
– Riccardo
19 hours ago
it depends... on what is your origin for request's from python - the difference must be there
– Kamil Kiełczewski
19 hours ago
changed post for clarity
– Riccardo
19 hours ago
you show your ajax RESPONSE headers, but show your ajax REQUEST headers (chrome> console> networks> your request)
– Kamil Kiełczewski
19 hours ago
1
1
Show your server CORS configuration - it looks like there is problem (in
Access-Control-Allow-Origin: null
)– Kamil Kiełczewski
20 hours ago
Show your server CORS configuration - it looks like there is problem (in
Access-Control-Allow-Origin: null
)– Kamil Kiełczewski
20 hours ago
then it shouldn't work in python on my local as well, should it?
– Riccardo
19 hours ago
then it shouldn't work in python on my local as well, should it?
– Riccardo
19 hours ago
it depends... on what is your origin for request's from python - the difference must be there
– Kamil Kiełczewski
19 hours ago
it depends... on what is your origin for request's from python - the difference must be there
– Kamil Kiełczewski
19 hours ago
changed post for clarity
– Riccardo
19 hours ago
changed post for clarity
– Riccardo
19 hours ago
you show your ajax RESPONSE headers, but show your ajax REQUEST headers (chrome> console> networks> your request)
– Kamil Kiełczewski
19 hours ago
you show your ajax RESPONSE headers, but show your ajax REQUEST headers (chrome> console> networks> your request)
– Kamil Kiełczewski
19 hours ago
|
show 6 more comments
2 Answers
2
active
oldest
votes
up vote
2
down vote
Python does not check CORS and the browser does to avoid security issues like XSS,
You need to make the server respond the header "Access-Control-Allow-Origin" with the domain from what the endpoint is being called ("https://myCoolServer.azurewebsites.net" ?)
If you want the server to allow being called for any domain use:
"Access-Control-Allow-Origin: *"
( currently is "Access-Control-Allow-Origin: null" )
This is needed because the browser needs to know if that server expect to be called from that domain.
add a comment |
up vote
2
down vote
CORS rules are primarily enforced by the client, not by the server. You python script does not implement these rules, hence the request succeeds, but your browser does implement them and blocks the request.
Now the server is supposed to provide information to the client about whether to allow the CORS request. The preflight request is just there to obtain this information (since your request is not a "simple request", cf this doc). In your case, clearly the server is not well configured:
Access-Control-Allow-Origin: null
You should have "*" instead of null, or at least "https://myCoolServer.azurewebsites.net".
New contributor
Rolvernew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
Python does not check CORS and the browser does to avoid security issues like XSS,
You need to make the server respond the header "Access-Control-Allow-Origin" with the domain from what the endpoint is being called ("https://myCoolServer.azurewebsites.net" ?)
If you want the server to allow being called for any domain use:
"Access-Control-Allow-Origin: *"
( currently is "Access-Control-Allow-Origin: null" )
This is needed because the browser needs to know if that server expect to be called from that domain.
add a comment |
up vote
2
down vote
Python does not check CORS and the browser does to avoid security issues like XSS,
You need to make the server respond the header "Access-Control-Allow-Origin" with the domain from what the endpoint is being called ("https://myCoolServer.azurewebsites.net" ?)
If you want the server to allow being called for any domain use:
"Access-Control-Allow-Origin: *"
( currently is "Access-Control-Allow-Origin: null" )
This is needed because the browser needs to know if that server expect to be called from that domain.
add a comment |
up vote
2
down vote
up vote
2
down vote
Python does not check CORS and the browser does to avoid security issues like XSS,
You need to make the server respond the header "Access-Control-Allow-Origin" with the domain from what the endpoint is being called ("https://myCoolServer.azurewebsites.net" ?)
If you want the server to allow being called for any domain use:
"Access-Control-Allow-Origin: *"
( currently is "Access-Control-Allow-Origin: null" )
This is needed because the browser needs to know if that server expect to be called from that domain.
Python does not check CORS and the browser does to avoid security issues like XSS,
You need to make the server respond the header "Access-Control-Allow-Origin" with the domain from what the endpoint is being called ("https://myCoolServer.azurewebsites.net" ?)
If you want the server to allow being called for any domain use:
"Access-Control-Allow-Origin: *"
( currently is "Access-Control-Allow-Origin: null" )
This is needed because the browser needs to know if that server expect to be called from that domain.
answered 19 hours ago
Sebastián Espinosa
1,329816
1,329816
add a comment |
add a comment |
up vote
2
down vote
CORS rules are primarily enforced by the client, not by the server. You python script does not implement these rules, hence the request succeeds, but your browser does implement them and blocks the request.
Now the server is supposed to provide information to the client about whether to allow the CORS request. The preflight request is just there to obtain this information (since your request is not a "simple request", cf this doc). In your case, clearly the server is not well configured:
Access-Control-Allow-Origin: null
You should have "*" instead of null, or at least "https://myCoolServer.azurewebsites.net".
New contributor
Rolvernew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
2
down vote
CORS rules are primarily enforced by the client, not by the server. You python script does not implement these rules, hence the request succeeds, but your browser does implement them and blocks the request.
Now the server is supposed to provide information to the client about whether to allow the CORS request. The preflight request is just there to obtain this information (since your request is not a "simple request", cf this doc). In your case, clearly the server is not well configured:
Access-Control-Allow-Origin: null
You should have "*" instead of null, or at least "https://myCoolServer.azurewebsites.net".
New contributor
Rolvernew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
2
down vote
up vote
2
down vote
CORS rules are primarily enforced by the client, not by the server. You python script does not implement these rules, hence the request succeeds, but your browser does implement them and blocks the request.
Now the server is supposed to provide information to the client about whether to allow the CORS request. The preflight request is just there to obtain this information (since your request is not a "simple request", cf this doc). In your case, clearly the server is not well configured:
Access-Control-Allow-Origin: null
You should have "*" instead of null, or at least "https://myCoolServer.azurewebsites.net".
New contributor
Rolvernew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
CORS rules are primarily enforced by the client, not by the server. You python script does not implement these rules, hence the request succeeds, but your browser does implement them and blocks the request.
Now the server is supposed to provide information to the client about whether to allow the CORS request. The preflight request is just there to obtain this information (since your request is not a "simple request", cf this doc). In your case, clearly the server is not well configured:
Access-Control-Allow-Origin: null
You should have "*" instead of null, or at least "https://myCoolServer.azurewebsites.net".
New contributor
Rolvernew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Rolvernew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 19 hours ago


Rolvernew
663
663
New contributor
Rolvernew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Rolvernew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Rolvernew is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53370860%2fjquery-preflight-request-cors%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ak,nXvMuuf3OE4IRoFapXQRWgI6 k5 2Vp6wOPzdgBb B2j PvYDL N eVx7,zm,Xk27WVNW Ew3FUh3Ke4 8Rz2
1
Show your server CORS configuration - it looks like there is problem (in
Access-Control-Allow-Origin: null
)– Kamil Kiełczewski
20 hours ago
then it shouldn't work in python on my local as well, should it?
– Riccardo
19 hours ago
it depends... on what is your origin for request's from python - the difference must be there
– Kamil Kiełczewski
19 hours ago
changed post for clarity
– Riccardo
19 hours ago
you show your ajax RESPONSE headers, but show your ajax REQUEST headers (chrome> console> networks> your request)
– Kamil Kiełczewski
19 hours ago