Trouble getting Jest and enzyme to render a div test from my App.js
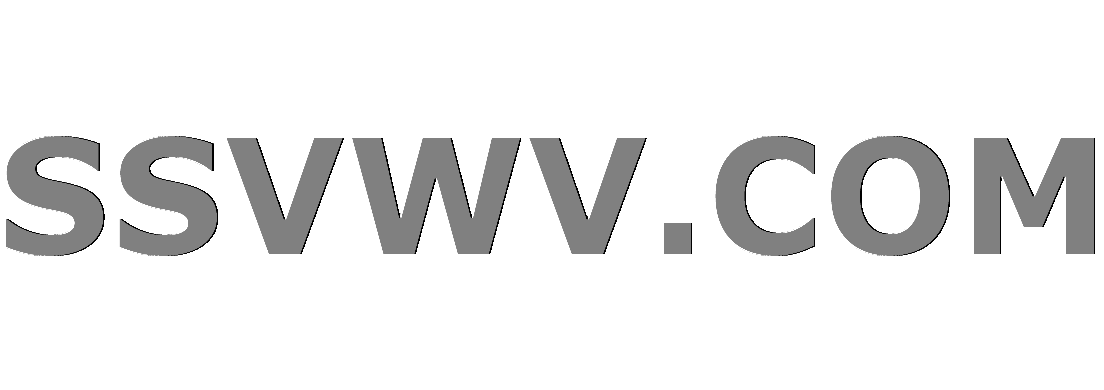
Multi tool use
I just started learning jest and enzyme for testing react components and i decided to build a MERN app to give it a go. I tried multiple times to get it to work but i am definitely doing something wrong. I am trying to get the div width to be at 400. is there also a way to write a test to make sure that the div content renders every time?
Here is my App.js file
import React, { Component } from 'react';
import gql from 'graphql-tag';
import { graphql, compose } from 'react-apollo';
import Paper from '@material-ui/core/Paper';
import List from '@material-ui/core/List';
import ListItem from '@material-ui/core/ListItem';
import ListItemSecondaryAction from '@material-ui/core/ListItemSecondaryAction';
import ListItemText from '@material-ui/core/ListItemText';
import Checkbox from '@material-ui/core/Checkbox';
import IconButton from '@material-ui/core/IconButton';
import CloseIcon from '@material-ui/icons/Close';
import Form from './Form'
// require enzyme
const Enzyme = require('enzyme');
const EnzymeAdapter = require('enzyme-adapter-react-16');
Enzyme.configure({ adapter: new EnzymeAdapter() });
const TodosQuery = gql`
{
todos {
id
text
complete
}
}
`;
const CreateMutation = gql`
mutation($text: String!) {
createTodo(text: $text) {
id
text
complete
}
}
`;
const UpdateMutation = gql`
mutation($id: ID!, $complete: Boolean!) {
updateTodo(id: $id, complete: $complete)
}
`;
const RemoveMutation = gql`
mutation($id: ID!) {
removeTodo(id: $id)
}
`;
class App extends Component {
//arrow functioning binds to 'this'
createTodo = async text => {
//create todo
await this.props.createTodo({
variables: {
text
},
update: (cache, {data: { createTodo }}) => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed (we also creating a copy of original array)
data.todos.unshift(createTodo)
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
updateTodo = async todo => {
//update todo
await this.props.updateTodo({
variables: {
id: todo.id,
complete: !todo.complete
},
update: cache => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed
data.todos = data.todos.map(x => x.id === todo.id ? { ...todo, complete: !todo.complete } : x );
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
removeTodo = async todo => {
//remove todo
await this.props.removeTodo({
variables: {
id: todo.id
},
update: cache => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed (we also creating a copy of original array)
data.todos = data.todos.filter(x => x.id !== todo.id)
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
render() {
console.log(this.props);
const { data: { loading, todos }} = this.props;
if (loading) {
return null;
}
return (
<div style={{ display: 'flex' }}>
<div style={{ margin: "auto", width: 400 }}>
<Paper elevation={1}>
<Form submit={this.createTodo} />
<List>
{todos.map(todo => (
<ListItem
key={todo.id}
role={undefined}
dense
button
onClick={() => this.updateTodo(todo)}
>
<Checkbox
//checked can also be 'true' (everything checked) or false (nothing gets checked):
checked={todo.complete}
tabIndex={-1}
disableRipple
/>
<ListItemText primary={todo.text} />
<ListItemSecondaryAction>
<IconButton onClick={() => this.removeTodo(todo)}>
<CloseIcon />
</IconButton>
</ListItemSecondaryAction>
</ListItem>
))}
</List>
</Paper>
</div>
</div>
)
}
}
//here, 'compose' squishes queries together and wraps around the 'App'
//we also pass props to queries
export default compose(
graphql(CreateMutation, {name: "createTodo"}),
graphql(RemoveMutation, {name: "removeTodo"}),
graphql(UpdateMutation, {name: "updateTodo"}),
graphql(TodosQuery)
)(App);
And here is the test that i have written in a app.test.js file
import React from 'react';
import ReactDOM from 'react-dom';
import App from '../App';
// shallow rendering to render a mock Dom
import { shallow, mount, render } from 'enzyme';
describe('div container', () => {
it('div width is always 400', () => {
expect(shallow(<App />).find('#style').width).toEqual(400)
})
})
Is there anything i am doing wrong or should be doing ?
javascript node.js reactjs unit-testing jestjs
add a comment |
I just started learning jest and enzyme for testing react components and i decided to build a MERN app to give it a go. I tried multiple times to get it to work but i am definitely doing something wrong. I am trying to get the div width to be at 400. is there also a way to write a test to make sure that the div content renders every time?
Here is my App.js file
import React, { Component } from 'react';
import gql from 'graphql-tag';
import { graphql, compose } from 'react-apollo';
import Paper from '@material-ui/core/Paper';
import List from '@material-ui/core/List';
import ListItem from '@material-ui/core/ListItem';
import ListItemSecondaryAction from '@material-ui/core/ListItemSecondaryAction';
import ListItemText from '@material-ui/core/ListItemText';
import Checkbox from '@material-ui/core/Checkbox';
import IconButton from '@material-ui/core/IconButton';
import CloseIcon from '@material-ui/icons/Close';
import Form from './Form'
// require enzyme
const Enzyme = require('enzyme');
const EnzymeAdapter = require('enzyme-adapter-react-16');
Enzyme.configure({ adapter: new EnzymeAdapter() });
const TodosQuery = gql`
{
todos {
id
text
complete
}
}
`;
const CreateMutation = gql`
mutation($text: String!) {
createTodo(text: $text) {
id
text
complete
}
}
`;
const UpdateMutation = gql`
mutation($id: ID!, $complete: Boolean!) {
updateTodo(id: $id, complete: $complete)
}
`;
const RemoveMutation = gql`
mutation($id: ID!) {
removeTodo(id: $id)
}
`;
class App extends Component {
//arrow functioning binds to 'this'
createTodo = async text => {
//create todo
await this.props.createTodo({
variables: {
text
},
update: (cache, {data: { createTodo }}) => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed (we also creating a copy of original array)
data.todos.unshift(createTodo)
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
updateTodo = async todo => {
//update todo
await this.props.updateTodo({
variables: {
id: todo.id,
complete: !todo.complete
},
update: cache => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed
data.todos = data.todos.map(x => x.id === todo.id ? { ...todo, complete: !todo.complete } : x );
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
removeTodo = async todo => {
//remove todo
await this.props.removeTodo({
variables: {
id: todo.id
},
update: cache => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed (we also creating a copy of original array)
data.todos = data.todos.filter(x => x.id !== todo.id)
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
render() {
console.log(this.props);
const { data: { loading, todos }} = this.props;
if (loading) {
return null;
}
return (
<div style={{ display: 'flex' }}>
<div style={{ margin: "auto", width: 400 }}>
<Paper elevation={1}>
<Form submit={this.createTodo} />
<List>
{todos.map(todo => (
<ListItem
key={todo.id}
role={undefined}
dense
button
onClick={() => this.updateTodo(todo)}
>
<Checkbox
//checked can also be 'true' (everything checked) or false (nothing gets checked):
checked={todo.complete}
tabIndex={-1}
disableRipple
/>
<ListItemText primary={todo.text} />
<ListItemSecondaryAction>
<IconButton onClick={() => this.removeTodo(todo)}>
<CloseIcon />
</IconButton>
</ListItemSecondaryAction>
</ListItem>
))}
</List>
</Paper>
</div>
</div>
)
}
}
//here, 'compose' squishes queries together and wraps around the 'App'
//we also pass props to queries
export default compose(
graphql(CreateMutation, {name: "createTodo"}),
graphql(RemoveMutation, {name: "removeTodo"}),
graphql(UpdateMutation, {name: "updateTodo"}),
graphql(TodosQuery)
)(App);
And here is the test that i have written in a app.test.js file
import React from 'react';
import ReactDOM from 'react-dom';
import App from '../App';
// shallow rendering to render a mock Dom
import { shallow, mount, render } from 'enzyme';
describe('div container', () => {
it('div width is always 400', () => {
expect(shallow(<App />).find('#style').width).toEqual(400)
})
})
Is there anything i am doing wrong or should be doing ?
javascript node.js reactjs unit-testing jestjs
add a comment |
I just started learning jest and enzyme for testing react components and i decided to build a MERN app to give it a go. I tried multiple times to get it to work but i am definitely doing something wrong. I am trying to get the div width to be at 400. is there also a way to write a test to make sure that the div content renders every time?
Here is my App.js file
import React, { Component } from 'react';
import gql from 'graphql-tag';
import { graphql, compose } from 'react-apollo';
import Paper from '@material-ui/core/Paper';
import List from '@material-ui/core/List';
import ListItem from '@material-ui/core/ListItem';
import ListItemSecondaryAction from '@material-ui/core/ListItemSecondaryAction';
import ListItemText from '@material-ui/core/ListItemText';
import Checkbox from '@material-ui/core/Checkbox';
import IconButton from '@material-ui/core/IconButton';
import CloseIcon from '@material-ui/icons/Close';
import Form from './Form'
// require enzyme
const Enzyme = require('enzyme');
const EnzymeAdapter = require('enzyme-adapter-react-16');
Enzyme.configure({ adapter: new EnzymeAdapter() });
const TodosQuery = gql`
{
todos {
id
text
complete
}
}
`;
const CreateMutation = gql`
mutation($text: String!) {
createTodo(text: $text) {
id
text
complete
}
}
`;
const UpdateMutation = gql`
mutation($id: ID!, $complete: Boolean!) {
updateTodo(id: $id, complete: $complete)
}
`;
const RemoveMutation = gql`
mutation($id: ID!) {
removeTodo(id: $id)
}
`;
class App extends Component {
//arrow functioning binds to 'this'
createTodo = async text => {
//create todo
await this.props.createTodo({
variables: {
text
},
update: (cache, {data: { createTodo }}) => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed (we also creating a copy of original array)
data.todos.unshift(createTodo)
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
updateTodo = async todo => {
//update todo
await this.props.updateTodo({
variables: {
id: todo.id,
complete: !todo.complete
},
update: cache => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed
data.todos = data.todos.map(x => x.id === todo.id ? { ...todo, complete: !todo.complete } : x );
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
removeTodo = async todo => {
//remove todo
await this.props.removeTodo({
variables: {
id: todo.id
},
update: cache => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed (we also creating a copy of original array)
data.todos = data.todos.filter(x => x.id !== todo.id)
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
render() {
console.log(this.props);
const { data: { loading, todos }} = this.props;
if (loading) {
return null;
}
return (
<div style={{ display: 'flex' }}>
<div style={{ margin: "auto", width: 400 }}>
<Paper elevation={1}>
<Form submit={this.createTodo} />
<List>
{todos.map(todo => (
<ListItem
key={todo.id}
role={undefined}
dense
button
onClick={() => this.updateTodo(todo)}
>
<Checkbox
//checked can also be 'true' (everything checked) or false (nothing gets checked):
checked={todo.complete}
tabIndex={-1}
disableRipple
/>
<ListItemText primary={todo.text} />
<ListItemSecondaryAction>
<IconButton onClick={() => this.removeTodo(todo)}>
<CloseIcon />
</IconButton>
</ListItemSecondaryAction>
</ListItem>
))}
</List>
</Paper>
</div>
</div>
)
}
}
//here, 'compose' squishes queries together and wraps around the 'App'
//we also pass props to queries
export default compose(
graphql(CreateMutation, {name: "createTodo"}),
graphql(RemoveMutation, {name: "removeTodo"}),
graphql(UpdateMutation, {name: "updateTodo"}),
graphql(TodosQuery)
)(App);
And here is the test that i have written in a app.test.js file
import React from 'react';
import ReactDOM from 'react-dom';
import App from '../App';
// shallow rendering to render a mock Dom
import { shallow, mount, render } from 'enzyme';
describe('div container', () => {
it('div width is always 400', () => {
expect(shallow(<App />).find('#style').width).toEqual(400)
})
})
Is there anything i am doing wrong or should be doing ?
javascript node.js reactjs unit-testing jestjs
I just started learning jest and enzyme for testing react components and i decided to build a MERN app to give it a go. I tried multiple times to get it to work but i am definitely doing something wrong. I am trying to get the div width to be at 400. is there also a way to write a test to make sure that the div content renders every time?
Here is my App.js file
import React, { Component } from 'react';
import gql from 'graphql-tag';
import { graphql, compose } from 'react-apollo';
import Paper from '@material-ui/core/Paper';
import List from '@material-ui/core/List';
import ListItem from '@material-ui/core/ListItem';
import ListItemSecondaryAction from '@material-ui/core/ListItemSecondaryAction';
import ListItemText from '@material-ui/core/ListItemText';
import Checkbox from '@material-ui/core/Checkbox';
import IconButton from '@material-ui/core/IconButton';
import CloseIcon from '@material-ui/icons/Close';
import Form from './Form'
// require enzyme
const Enzyme = require('enzyme');
const EnzymeAdapter = require('enzyme-adapter-react-16');
Enzyme.configure({ adapter: new EnzymeAdapter() });
const TodosQuery = gql`
{
todos {
id
text
complete
}
}
`;
const CreateMutation = gql`
mutation($text: String!) {
createTodo(text: $text) {
id
text
complete
}
}
`;
const UpdateMutation = gql`
mutation($id: ID!, $complete: Boolean!) {
updateTodo(id: $id, complete: $complete)
}
`;
const RemoveMutation = gql`
mutation($id: ID!) {
removeTodo(id: $id)
}
`;
class App extends Component {
//arrow functioning binds to 'this'
createTodo = async text => {
//create todo
await this.props.createTodo({
variables: {
text
},
update: (cache, {data: { createTodo }}) => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed (we also creating a copy of original array)
data.todos.unshift(createTodo)
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
updateTodo = async todo => {
//update todo
await this.props.updateTodo({
variables: {
id: todo.id,
complete: !todo.complete
},
update: cache => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed
data.todos = data.todos.map(x => x.id === todo.id ? { ...todo, complete: !todo.complete } : x );
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
removeTodo = async todo => {
//remove todo
await this.props.removeTodo({
variables: {
id: todo.id
},
update: cache => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed (we also creating a copy of original array)
data.todos = data.todos.filter(x => x.id !== todo.id)
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
render() {
console.log(this.props);
const { data: { loading, todos }} = this.props;
if (loading) {
return null;
}
return (
<div style={{ display: 'flex' }}>
<div style={{ margin: "auto", width: 400 }}>
<Paper elevation={1}>
<Form submit={this.createTodo} />
<List>
{todos.map(todo => (
<ListItem
key={todo.id}
role={undefined}
dense
button
onClick={() => this.updateTodo(todo)}
>
<Checkbox
//checked can also be 'true' (everything checked) or false (nothing gets checked):
checked={todo.complete}
tabIndex={-1}
disableRipple
/>
<ListItemText primary={todo.text} />
<ListItemSecondaryAction>
<IconButton onClick={() => this.removeTodo(todo)}>
<CloseIcon />
</IconButton>
</ListItemSecondaryAction>
</ListItem>
))}
</List>
</Paper>
</div>
</div>
)
}
}
//here, 'compose' squishes queries together and wraps around the 'App'
//we also pass props to queries
export default compose(
graphql(CreateMutation, {name: "createTodo"}),
graphql(RemoveMutation, {name: "removeTodo"}),
graphql(UpdateMutation, {name: "updateTodo"}),
graphql(TodosQuery)
)(App);
And here is the test that i have written in a app.test.js file
import React from 'react';
import ReactDOM from 'react-dom';
import App from '../App';
// shallow rendering to render a mock Dom
import { shallow, mount, render } from 'enzyme';
describe('div container', () => {
it('div width is always 400', () => {
expect(shallow(<App />).find('#style').width).toEqual(400)
})
})
Is there anything i am doing wrong or should be doing ?
import React, { Component } from 'react';
import gql from 'graphql-tag';
import { graphql, compose } from 'react-apollo';
import Paper from '@material-ui/core/Paper';
import List from '@material-ui/core/List';
import ListItem from '@material-ui/core/ListItem';
import ListItemSecondaryAction from '@material-ui/core/ListItemSecondaryAction';
import ListItemText from '@material-ui/core/ListItemText';
import Checkbox from '@material-ui/core/Checkbox';
import IconButton from '@material-ui/core/IconButton';
import CloseIcon from '@material-ui/icons/Close';
import Form from './Form'
// require enzyme
const Enzyme = require('enzyme');
const EnzymeAdapter = require('enzyme-adapter-react-16');
Enzyme.configure({ adapter: new EnzymeAdapter() });
const TodosQuery = gql`
{
todos {
id
text
complete
}
}
`;
const CreateMutation = gql`
mutation($text: String!) {
createTodo(text: $text) {
id
text
complete
}
}
`;
const UpdateMutation = gql`
mutation($id: ID!, $complete: Boolean!) {
updateTodo(id: $id, complete: $complete)
}
`;
const RemoveMutation = gql`
mutation($id: ID!) {
removeTodo(id: $id)
}
`;
class App extends Component {
//arrow functioning binds to 'this'
createTodo = async text => {
//create todo
await this.props.createTodo({
variables: {
text
},
update: (cache, {data: { createTodo }}) => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed (we also creating a copy of original array)
data.todos.unshift(createTodo)
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
updateTodo = async todo => {
//update todo
await this.props.updateTodo({
variables: {
id: todo.id,
complete: !todo.complete
},
update: cache => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed
data.todos = data.todos.map(x => x.id === todo.id ? { ...todo, complete: !todo.complete } : x );
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
removeTodo = async todo => {
//remove todo
await this.props.removeTodo({
variables: {
id: todo.id
},
update: cache => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed (we also creating a copy of original array)
data.todos = data.todos.filter(x => x.id !== todo.id)
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
render() {
console.log(this.props);
const { data: { loading, todos }} = this.props;
if (loading) {
return null;
}
return (
<div style={{ display: 'flex' }}>
<div style={{ margin: "auto", width: 400 }}>
<Paper elevation={1}>
<Form submit={this.createTodo} />
<List>
{todos.map(todo => (
<ListItem
key={todo.id}
role={undefined}
dense
button
onClick={() => this.updateTodo(todo)}
>
<Checkbox
//checked can also be 'true' (everything checked) or false (nothing gets checked):
checked={todo.complete}
tabIndex={-1}
disableRipple
/>
<ListItemText primary={todo.text} />
<ListItemSecondaryAction>
<IconButton onClick={() => this.removeTodo(todo)}>
<CloseIcon />
</IconButton>
</ListItemSecondaryAction>
</ListItem>
))}
</List>
</Paper>
</div>
</div>
)
}
}
//here, 'compose' squishes queries together and wraps around the 'App'
//we also pass props to queries
export default compose(
graphql(CreateMutation, {name: "createTodo"}),
graphql(RemoveMutation, {name: "removeTodo"}),
graphql(UpdateMutation, {name: "updateTodo"}),
graphql(TodosQuery)
)(App);
import React, { Component } from 'react';
import gql from 'graphql-tag';
import { graphql, compose } from 'react-apollo';
import Paper from '@material-ui/core/Paper';
import List from '@material-ui/core/List';
import ListItem from '@material-ui/core/ListItem';
import ListItemSecondaryAction from '@material-ui/core/ListItemSecondaryAction';
import ListItemText from '@material-ui/core/ListItemText';
import Checkbox from '@material-ui/core/Checkbox';
import IconButton from '@material-ui/core/IconButton';
import CloseIcon from '@material-ui/icons/Close';
import Form from './Form'
// require enzyme
const Enzyme = require('enzyme');
const EnzymeAdapter = require('enzyme-adapter-react-16');
Enzyme.configure({ adapter: new EnzymeAdapter() });
const TodosQuery = gql`
{
todos {
id
text
complete
}
}
`;
const CreateMutation = gql`
mutation($text: String!) {
createTodo(text: $text) {
id
text
complete
}
}
`;
const UpdateMutation = gql`
mutation($id: ID!, $complete: Boolean!) {
updateTodo(id: $id, complete: $complete)
}
`;
const RemoveMutation = gql`
mutation($id: ID!) {
removeTodo(id: $id)
}
`;
class App extends Component {
//arrow functioning binds to 'this'
createTodo = async text => {
//create todo
await this.props.createTodo({
variables: {
text
},
update: (cache, {data: { createTodo }}) => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed (we also creating a copy of original array)
data.todos.unshift(createTodo)
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
updateTodo = async todo => {
//update todo
await this.props.updateTodo({
variables: {
id: todo.id,
complete: !todo.complete
},
update: cache => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed
data.todos = data.todos.map(x => x.id === todo.id ? { ...todo, complete: !todo.complete } : x );
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
removeTodo = async todo => {
//remove todo
await this.props.removeTodo({
variables: {
id: todo.id
},
update: cache => {
//read the data from our cache for this query
const data = cache.readQuery({ query: TodosQuery });
//query returns 'data' with 'todos', then do the front end changes needed (we also creating a copy of original array)
data.todos = data.todos.filter(x => x.id !== todo.id)
//write data back to the cache
cache.writeQuery({ query: TodosQuery, data });
}
});
};
render() {
console.log(this.props);
const { data: { loading, todos }} = this.props;
if (loading) {
return null;
}
return (
<div style={{ display: 'flex' }}>
<div style={{ margin: "auto", width: 400 }}>
<Paper elevation={1}>
<Form submit={this.createTodo} />
<List>
{todos.map(todo => (
<ListItem
key={todo.id}
role={undefined}
dense
button
onClick={() => this.updateTodo(todo)}
>
<Checkbox
//checked can also be 'true' (everything checked) or false (nothing gets checked):
checked={todo.complete}
tabIndex={-1}
disableRipple
/>
<ListItemText primary={todo.text} />
<ListItemSecondaryAction>
<IconButton onClick={() => this.removeTodo(todo)}>
<CloseIcon />
</IconButton>
</ListItemSecondaryAction>
</ListItem>
))}
</List>
</Paper>
</div>
</div>
)
}
}
//here, 'compose' squishes queries together and wraps around the 'App'
//we also pass props to queries
export default compose(
graphql(CreateMutation, {name: "createTodo"}),
graphql(RemoveMutation, {name: "removeTodo"}),
graphql(UpdateMutation, {name: "updateTodo"}),
graphql(TodosQuery)
)(App);
import React from 'react';
import ReactDOM from 'react-dom';
import App from '../App';
// shallow rendering to render a mock Dom
import { shallow, mount, render } from 'enzyme';
describe('div container', () => {
it('div width is always 400', () => {
expect(shallow(<App />).find('#style').width).toEqual(400)
})
})
import React from 'react';
import ReactDOM from 'react-dom';
import App from '../App';
// shallow rendering to render a mock Dom
import { shallow, mount, render } from 'enzyme';
describe('div container', () => {
it('div width is always 400', () => {
expect(shallow(<App />).find('#style').width).toEqual(400)
})
})
javascript node.js reactjs unit-testing jestjs
javascript node.js reactjs unit-testing jestjs
edited Nov 26 '18 at 21:11
skyboyer
3,94311230
3,94311230
asked Nov 25 '18 at 22:21
maryanntmaryannt
62
62
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472591%2ftrouble-getting-jest-and-enzyme-to-render-a-div-test-from-my-app-js%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472591%2ftrouble-getting-jest-and-enzyme-to-render-a-div-test-from-my-app-js%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8yPIDdt,qE,Rob,FJ0c4uwWdWcn9Wtws8vRaH l ZJKTsyAamswkucUstK5VPVg3pVkqO