Spring Data Rest POST response different from GET response
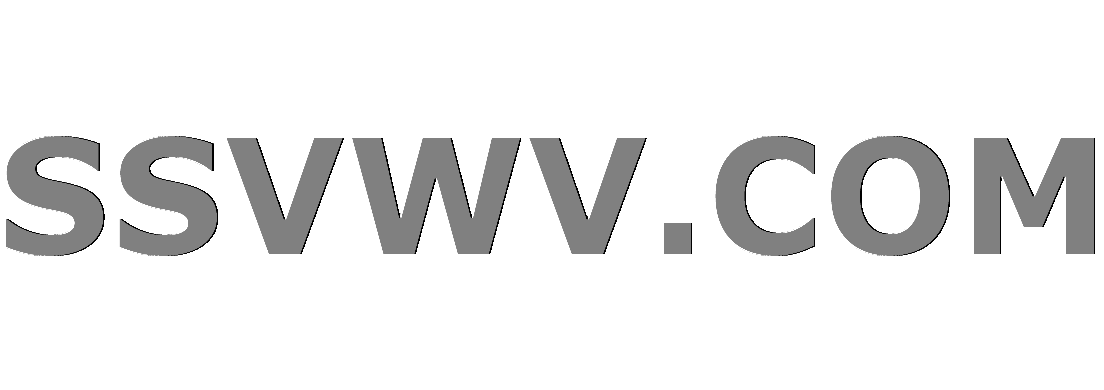
Multi tool use
On my Spring Data Rest project I have a Competition
entity that references an GeoLocation
entity:
public class Competition {
@Id
private String uname;
[...]
@NotNull
@OneToOne(cascade = CascadeType.ALL, orphanRemoval = true, fetch = FetchType.LAZY)
private GeoLocation geoLocation;
}
public class GeoLocation {
@Id private Long id;
private Double latitude;
private Double longitude;
}
Since every Competition
must have a GeoLocation
defined, the Competition
entity handles the creation via cascade
. When creating a new Competition
entity via POST, I get the following response:
{
"uname": "Some Competition",
"geoLocation": {
[content of geoLocation]
},
"_links": {
[...]
}
}
But when I call the newly created competition, the content of the GeoLocation
will be wrapped in a content
field.
{
"uname": "Some Competition",
"geoLocation": {
"content": {
[content of geoLocation]
}
},
"_links": {
[...]
}
}
I would expect that both requests would deliver the same response?
spring hibernate jpa spring-data-rest
add a comment |
On my Spring Data Rest project I have a Competition
entity that references an GeoLocation
entity:
public class Competition {
@Id
private String uname;
[...]
@NotNull
@OneToOne(cascade = CascadeType.ALL, orphanRemoval = true, fetch = FetchType.LAZY)
private GeoLocation geoLocation;
}
public class GeoLocation {
@Id private Long id;
private Double latitude;
private Double longitude;
}
Since every Competition
must have a GeoLocation
defined, the Competition
entity handles the creation via cascade
. When creating a new Competition
entity via POST, I get the following response:
{
"uname": "Some Competition",
"geoLocation": {
[content of geoLocation]
},
"_links": {
[...]
}
}
But when I call the newly created competition, the content of the GeoLocation
will be wrapped in a content
field.
{
"uname": "Some Competition",
"geoLocation": {
"content": {
[content of geoLocation]
}
},
"_links": {
[...]
}
}
I would expect that both requests would deliver the same response?
spring hibernate jpa spring-data-rest
Possibly same issue as I asked about here: stackoverflow.com/questions/42395831/…
– Alan Hay
Nov 26 '18 at 8:35
@AlanHay that looks almost identical, thank you.
– Sebastian Ullrich
Nov 26 '18 at 9:45
If so, making your relationship eager would probably solve.
– Alan Hay
Nov 26 '18 at 9:57
You're right again @AlanHay. Changing toEager
solved this issue.
– Sebastian Ullrich
Nov 26 '18 at 20:23
add a comment |
On my Spring Data Rest project I have a Competition
entity that references an GeoLocation
entity:
public class Competition {
@Id
private String uname;
[...]
@NotNull
@OneToOne(cascade = CascadeType.ALL, orphanRemoval = true, fetch = FetchType.LAZY)
private GeoLocation geoLocation;
}
public class GeoLocation {
@Id private Long id;
private Double latitude;
private Double longitude;
}
Since every Competition
must have a GeoLocation
defined, the Competition
entity handles the creation via cascade
. When creating a new Competition
entity via POST, I get the following response:
{
"uname": "Some Competition",
"geoLocation": {
[content of geoLocation]
},
"_links": {
[...]
}
}
But when I call the newly created competition, the content of the GeoLocation
will be wrapped in a content
field.
{
"uname": "Some Competition",
"geoLocation": {
"content": {
[content of geoLocation]
}
},
"_links": {
[...]
}
}
I would expect that both requests would deliver the same response?
spring hibernate jpa spring-data-rest
On my Spring Data Rest project I have a Competition
entity that references an GeoLocation
entity:
public class Competition {
@Id
private String uname;
[...]
@NotNull
@OneToOne(cascade = CascadeType.ALL, orphanRemoval = true, fetch = FetchType.LAZY)
private GeoLocation geoLocation;
}
public class GeoLocation {
@Id private Long id;
private Double latitude;
private Double longitude;
}
Since every Competition
must have a GeoLocation
defined, the Competition
entity handles the creation via cascade
. When creating a new Competition
entity via POST, I get the following response:
{
"uname": "Some Competition",
"geoLocation": {
[content of geoLocation]
},
"_links": {
[...]
}
}
But when I call the newly created competition, the content of the GeoLocation
will be wrapped in a content
field.
{
"uname": "Some Competition",
"geoLocation": {
"content": {
[content of geoLocation]
}
},
"_links": {
[...]
}
}
I would expect that both requests would deliver the same response?
spring hibernate jpa spring-data-rest
spring hibernate jpa spring-data-rest
asked Nov 25 '18 at 22:41
Sebastian UllrichSebastian Ullrich
31219
31219
Possibly same issue as I asked about here: stackoverflow.com/questions/42395831/…
– Alan Hay
Nov 26 '18 at 8:35
@AlanHay that looks almost identical, thank you.
– Sebastian Ullrich
Nov 26 '18 at 9:45
If so, making your relationship eager would probably solve.
– Alan Hay
Nov 26 '18 at 9:57
You're right again @AlanHay. Changing toEager
solved this issue.
– Sebastian Ullrich
Nov 26 '18 at 20:23
add a comment |
Possibly same issue as I asked about here: stackoverflow.com/questions/42395831/…
– Alan Hay
Nov 26 '18 at 8:35
@AlanHay that looks almost identical, thank you.
– Sebastian Ullrich
Nov 26 '18 at 9:45
If so, making your relationship eager would probably solve.
– Alan Hay
Nov 26 '18 at 9:57
You're right again @AlanHay. Changing toEager
solved this issue.
– Sebastian Ullrich
Nov 26 '18 at 20:23
Possibly same issue as I asked about here: stackoverflow.com/questions/42395831/…
– Alan Hay
Nov 26 '18 at 8:35
Possibly same issue as I asked about here: stackoverflow.com/questions/42395831/…
– Alan Hay
Nov 26 '18 at 8:35
@AlanHay that looks almost identical, thank you.
– Sebastian Ullrich
Nov 26 '18 at 9:45
@AlanHay that looks almost identical, thank you.
– Sebastian Ullrich
Nov 26 '18 at 9:45
If so, making your relationship eager would probably solve.
– Alan Hay
Nov 26 '18 at 9:57
If so, making your relationship eager would probably solve.
– Alan Hay
Nov 26 '18 at 9:57
You're right again @AlanHay. Changing to
Eager
solved this issue.– Sebastian Ullrich
Nov 26 '18 at 20:23
You're right again @AlanHay. Changing to
Eager
solved this issue.– Sebastian Ullrich
Nov 26 '18 at 20:23
add a comment |
1 Answer
1
active
oldest
votes
@JsonUnwrapped
solved this issue for me:
public class Competition {
@Id
private String uname;
[...]
@NotNull
@JsonUnwrapped
@OneToOne(cascade = CascadeType.ALL, orphanRemoval = true, fetch = FetchType.LAZY)
private GeoLocation geoLocation;
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472735%2fspring-data-rest-post-response-different-from-get-response%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
@JsonUnwrapped
solved this issue for me:
public class Competition {
@Id
private String uname;
[...]
@NotNull
@JsonUnwrapped
@OneToOne(cascade = CascadeType.ALL, orphanRemoval = true, fetch = FetchType.LAZY)
private GeoLocation geoLocation;
}
add a comment |
@JsonUnwrapped
solved this issue for me:
public class Competition {
@Id
private String uname;
[...]
@NotNull
@JsonUnwrapped
@OneToOne(cascade = CascadeType.ALL, orphanRemoval = true, fetch = FetchType.LAZY)
private GeoLocation geoLocation;
}
add a comment |
@JsonUnwrapped
solved this issue for me:
public class Competition {
@Id
private String uname;
[...]
@NotNull
@JsonUnwrapped
@OneToOne(cascade = CascadeType.ALL, orphanRemoval = true, fetch = FetchType.LAZY)
private GeoLocation geoLocation;
}
@JsonUnwrapped
solved this issue for me:
public class Competition {
@Id
private String uname;
[...]
@NotNull
@JsonUnwrapped
@OneToOne(cascade = CascadeType.ALL, orphanRemoval = true, fetch = FetchType.LAZY)
private GeoLocation geoLocation;
}
answered Dec 4 '18 at 23:06
Sebastian UllrichSebastian Ullrich
31219
31219
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472735%2fspring-data-rest-post-response-different-from-get-response%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
GPXMe7tgzZcscHd69OEgm1aHxsf2mRcY0Pgi95 zD4cpnT2q,5,6,L7LHoo2 PQ
Possibly same issue as I asked about here: stackoverflow.com/questions/42395831/…
– Alan Hay
Nov 26 '18 at 8:35
@AlanHay that looks almost identical, thank you.
– Sebastian Ullrich
Nov 26 '18 at 9:45
If so, making your relationship eager would probably solve.
– Alan Hay
Nov 26 '18 at 9:57
You're right again @AlanHay. Changing to
Eager
solved this issue.– Sebastian Ullrich
Nov 26 '18 at 20:23