Using PowerShell Parser for console application
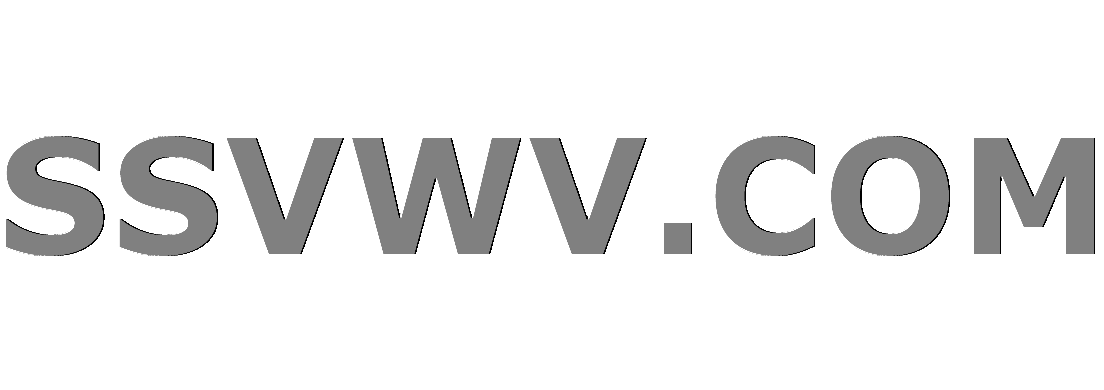
Multi tool use
My larger PowerShell/console application requires OOP, etc. for easier development.
I know about parsers such as NDesk.Options and CommandLineParser but I want something to simulate cmdlet parsing as close as possible. E.g. Support parsing for:
MyProject.exe do-thing ("computer1", "computer2") -p "parameter"
MyProject.exe do-thing -cn ("computer1", "computer2") -p "parameter" -verbose
Is it possible to use System.Management.Automation.Language.Parser or a another tool to simulate PowerShell parsing? Are there any examples and pitfalls to watch out for?
powershell parsing automation console console-application
add a comment |
My larger PowerShell/console application requires OOP, etc. for easier development.
I know about parsers such as NDesk.Options and CommandLineParser but I want something to simulate cmdlet parsing as close as possible. E.g. Support parsing for:
MyProject.exe do-thing ("computer1", "computer2") -p "parameter"
MyProject.exe do-thing -cn ("computer1", "computer2") -p "parameter" -verbose
Is it possible to use System.Management.Automation.Language.Parser or a another tool to simulate PowerShell parsing? Are there any examples and pitfalls to watch out for?
powershell parsing automation console console-application
add a comment |
My larger PowerShell/console application requires OOP, etc. for easier development.
I know about parsers such as NDesk.Options and CommandLineParser but I want something to simulate cmdlet parsing as close as possible. E.g. Support parsing for:
MyProject.exe do-thing ("computer1", "computer2") -p "parameter"
MyProject.exe do-thing -cn ("computer1", "computer2") -p "parameter" -verbose
Is it possible to use System.Management.Automation.Language.Parser or a another tool to simulate PowerShell parsing? Are there any examples and pitfalls to watch out for?
powershell parsing automation console console-application
My larger PowerShell/console application requires OOP, etc. for easier development.
I know about parsers such as NDesk.Options and CommandLineParser but I want something to simulate cmdlet parsing as close as possible. E.g. Support parsing for:
MyProject.exe do-thing ("computer1", "computer2") -p "parameter"
MyProject.exe do-thing -cn ("computer1", "computer2") -p "parameter" -verbose
Is it possible to use System.Management.Automation.Language.Parser or a another tool to simulate PowerShell parsing? Are there any examples and pitfalls to watch out for?
powershell parsing automation console console-application
powershell parsing automation console console-application
asked Nov 24 '18 at 5:54


JPtheK9JPtheK9
146110
146110
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Sure, you could use Parser.ParseInput()
to parse the args and then extract the individual elements from the resulting CommandAst
:
using System.Management.Automation.Language;
public class Program
{
public static void Main(string args)
{
Token tokens;
ParseError errors;
Ast topAst = Parser.ParseInput(String.Join(" ", args), out tokens, out errors);
// Find the CommandAst object
CommandAst parsedCommand = topAst.Find(ast => ast is CommandAst, true);
// Grab the command name
string commandName = ((StringConstantExpressionAst)parsedCommand.CommandElements[0]).Value;
// Grab the remaining command arguments from CommandAst.CommandElements
}
}
I'd would probably store the arguments in a Dictionary<string,string>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53455554%2fusing-powershell-parser-for-console-application%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sure, you could use Parser.ParseInput()
to parse the args and then extract the individual elements from the resulting CommandAst
:
using System.Management.Automation.Language;
public class Program
{
public static void Main(string args)
{
Token tokens;
ParseError errors;
Ast topAst = Parser.ParseInput(String.Join(" ", args), out tokens, out errors);
// Find the CommandAst object
CommandAst parsedCommand = topAst.Find(ast => ast is CommandAst, true);
// Grab the command name
string commandName = ((StringConstantExpressionAst)parsedCommand.CommandElements[0]).Value;
// Grab the remaining command arguments from CommandAst.CommandElements
}
}
I'd would probably store the arguments in a Dictionary<string,string>
add a comment |
Sure, you could use Parser.ParseInput()
to parse the args and then extract the individual elements from the resulting CommandAst
:
using System.Management.Automation.Language;
public class Program
{
public static void Main(string args)
{
Token tokens;
ParseError errors;
Ast topAst = Parser.ParseInput(String.Join(" ", args), out tokens, out errors);
// Find the CommandAst object
CommandAst parsedCommand = topAst.Find(ast => ast is CommandAst, true);
// Grab the command name
string commandName = ((StringConstantExpressionAst)parsedCommand.CommandElements[0]).Value;
// Grab the remaining command arguments from CommandAst.CommandElements
}
}
I'd would probably store the arguments in a Dictionary<string,string>
add a comment |
Sure, you could use Parser.ParseInput()
to parse the args and then extract the individual elements from the resulting CommandAst
:
using System.Management.Automation.Language;
public class Program
{
public static void Main(string args)
{
Token tokens;
ParseError errors;
Ast topAst = Parser.ParseInput(String.Join(" ", args), out tokens, out errors);
// Find the CommandAst object
CommandAst parsedCommand = topAst.Find(ast => ast is CommandAst, true);
// Grab the command name
string commandName = ((StringConstantExpressionAst)parsedCommand.CommandElements[0]).Value;
// Grab the remaining command arguments from CommandAst.CommandElements
}
}
I'd would probably store the arguments in a Dictionary<string,string>
Sure, you could use Parser.ParseInput()
to parse the args and then extract the individual elements from the resulting CommandAst
:
using System.Management.Automation.Language;
public class Program
{
public static void Main(string args)
{
Token tokens;
ParseError errors;
Ast topAst = Parser.ParseInput(String.Join(" ", args), out tokens, out errors);
// Find the CommandAst object
CommandAst parsedCommand = topAst.Find(ast => ast is CommandAst, true);
// Grab the command name
string commandName = ((StringConstantExpressionAst)parsedCommand.CommandElements[0]).Value;
// Grab the remaining command arguments from CommandAst.CommandElements
}
}
I'd would probably store the arguments in a Dictionary<string,string>
answered Nov 24 '18 at 15:20


Mathias R. JessenMathias R. Jessen
57.6k460105
57.6k460105
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53455554%2fusing-powershell-parser-for-console-application%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LWRcaxoifJ