How do I plot the integral of e^(-t^2) from x=0 to x=3 in Python?
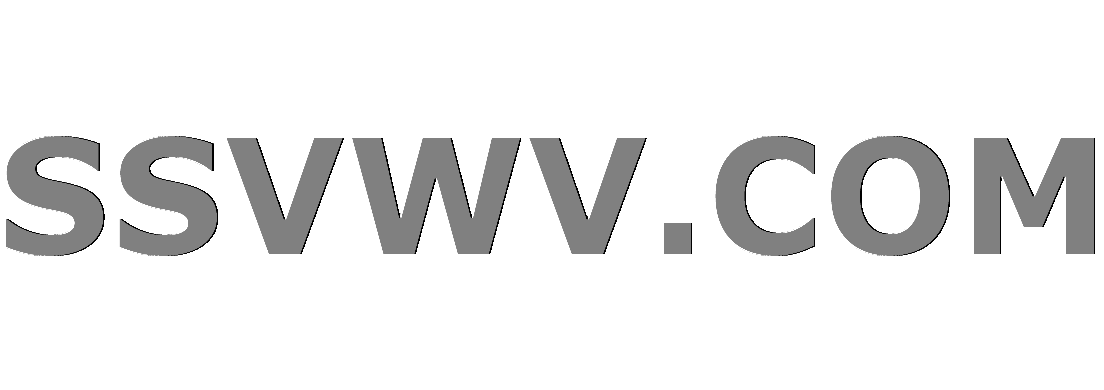
Multi tool use
I need to both calculate and plot the integral below in Python:
integral of the function e^(-t^2) from x=0 to x=3
So far I've managed to calculate the integral using Simpson's rule. The next bit which I'm struggling with is plotting the integral of e^(-t^2) vs x from x=0 to x=3 (see the image above).
Here's the code I've written to calculate the integral -
from math import exp
def f(t):
return exp(-(t**2))
a = 0
b = 3
h = 0.1
N = int((b-a)/h)
s_even = 0
s_odd = 0
for k in range(1,N,2):
s_odd += f(a+k*h)
for k in range(2,N,2):
s_even += f(a+k*h)
s = f(a) + f(b) + 4*s_odd + 2*s_even
Integral = h*s/3
print(Integral)
How do I then create a graph of this integral?
python math plot physics integral
add a comment |
I need to both calculate and plot the integral below in Python:
integral of the function e^(-t^2) from x=0 to x=3
So far I've managed to calculate the integral using Simpson's rule. The next bit which I'm struggling with is plotting the integral of e^(-t^2) vs x from x=0 to x=3 (see the image above).
Here's the code I've written to calculate the integral -
from math import exp
def f(t):
return exp(-(t**2))
a = 0
b = 3
h = 0.1
N = int((b-a)/h)
s_even = 0
s_odd = 0
for k in range(1,N,2):
s_odd += f(a+k*h)
for k in range(2,N,2):
s_even += f(a+k*h)
s = f(a) + f(b) + 4*s_odd + 2*s_even
Integral = h*s/3
print(Integral)
How do I then create a graph of this integral?
python math plot physics integral
Please try to find a more concrete title. What exactly are you struggeling with? Can you formulate a single question, so that the answer would help you?
– Martin Thoma
Nov 24 '18 at 6:14
If you are using version 3.2 or later then you can use math.erf for this; see en.wikipedia.org/wiki/Error_function for example
– dmuir
Nov 24 '18 at 12:58
add a comment |
I need to both calculate and plot the integral below in Python:
integral of the function e^(-t^2) from x=0 to x=3
So far I've managed to calculate the integral using Simpson's rule. The next bit which I'm struggling with is plotting the integral of e^(-t^2) vs x from x=0 to x=3 (see the image above).
Here's the code I've written to calculate the integral -
from math import exp
def f(t):
return exp(-(t**2))
a = 0
b = 3
h = 0.1
N = int((b-a)/h)
s_even = 0
s_odd = 0
for k in range(1,N,2):
s_odd += f(a+k*h)
for k in range(2,N,2):
s_even += f(a+k*h)
s = f(a) + f(b) + 4*s_odd + 2*s_even
Integral = h*s/3
print(Integral)
How do I then create a graph of this integral?
python math plot physics integral
I need to both calculate and plot the integral below in Python:
integral of the function e^(-t^2) from x=0 to x=3
So far I've managed to calculate the integral using Simpson's rule. The next bit which I'm struggling with is plotting the integral of e^(-t^2) vs x from x=0 to x=3 (see the image above).
Here's the code I've written to calculate the integral -
from math import exp
def f(t):
return exp(-(t**2))
a = 0
b = 3
h = 0.1
N = int((b-a)/h)
s_even = 0
s_odd = 0
for k in range(1,N,2):
s_odd += f(a+k*h)
for k in range(2,N,2):
s_even += f(a+k*h)
s = f(a) + f(b) + 4*s_odd + 2*s_even
Integral = h*s/3
print(Integral)
How do I then create a graph of this integral?
python math plot physics integral
python math plot physics integral
edited Nov 24 '18 at 7:14


eyllanesc
77.9k103156
77.9k103156
asked Nov 24 '18 at 6:11


CameronCameron
94
94
Please try to find a more concrete title. What exactly are you struggeling with? Can you formulate a single question, so that the answer would help you?
– Martin Thoma
Nov 24 '18 at 6:14
If you are using version 3.2 or later then you can use math.erf for this; see en.wikipedia.org/wiki/Error_function for example
– dmuir
Nov 24 '18 at 12:58
add a comment |
Please try to find a more concrete title. What exactly are you struggeling with? Can you formulate a single question, so that the answer would help you?
– Martin Thoma
Nov 24 '18 at 6:14
If you are using version 3.2 or later then you can use math.erf for this; see en.wikipedia.org/wiki/Error_function for example
– dmuir
Nov 24 '18 at 12:58
Please try to find a more concrete title. What exactly are you struggeling with? Can you formulate a single question, so that the answer would help you?
– Martin Thoma
Nov 24 '18 at 6:14
Please try to find a more concrete title. What exactly are you struggeling with? Can you formulate a single question, so that the answer would help you?
– Martin Thoma
Nov 24 '18 at 6:14
If you are using version 3.2 or later then you can use math.erf for this; see en.wikipedia.org/wiki/Error_function for example
– dmuir
Nov 24 '18 at 12:58
If you are using version 3.2 or later then you can use math.erf for this; see en.wikipedia.org/wiki/Error_function for example
– dmuir
Nov 24 '18 at 12:58
add a comment |
2 Answers
2
active
oldest
votes
Here's a script I wrote that performs your calculation and plots it using PyQtGraph:
from pyqtgraph.Qt import QtGui, QtCore
import pyqtgraph as pg
from math import exp
class I:
def f(self,t):
return exp(-(t**2))
def __init__(self, a = 0, b = 3, h = 0.1):
N = int((b-a)/h)
s_even = s_odd = 0
for k in range(1,N,2):
s_odd += self.f(a+k*h)
for k in range(2,N,2):
s_even += self.f(a+k*h)
s = self.f(a) + self.f(b) + 4*s_odd + 2*s_even
self.I = h*s/3
def __str__(self):
return "I: %s" % self.I
def plot(array):
app = QtGui.QApplication()
win = pg.GraphicsWindow(title="Basic plotting examples")
win.resize(1000,600)
win.setWindowTitle('pyqtgraph example: Plotting')
# Enable antialiasing for prettier plots
pg.setConfigOptions(antialias=True)
p1 = win.addPlot(title="Basic array plotting", y=array)
QtGui.QApplication.instance().exec_()
def main():
a=0
b=a+0.001
points=
while(a<3):
points.append(I(a,b).I)
a=b
b=a+0.001
plot(points)
## Start Qt event loop unless running in interactive mode or using pyside.
if __name__ == '__main__':
main()
Here is the graph it draws:
1
From your graph it looks like you are plotting something like the exponential function itselfe^(-x**2)
rather than its integral.
– Rory Daulton
Nov 24 '18 at 10:29
I am just demonstrating how one can create a graph in Python by plotting what the OP states to be the integral. If it is not an integral hopefully the OP will see your comment and realize his mistake.
– Red Cricket
Nov 25 '18 at 18:36
add a comment |
Thanks for your help Red Cricket. It looks like you may have graphed the function e^(-t^2) rather than the integral of that function. Nonetheless, I think I've worked it out; I've discovered scipy has an integrate function:
from math import exp
from numpy import arange
from scipy import integrate
def f(t):
return exp(-(t**2))
a = 0
b = 3
h = 0.1
N = int((b-a)/h)
s_even = 0
s_odd = 0
for k in range(1,N,2):
s_odd += f(a+k*h)
for k in range(2,N,2):
s_even += f(a+k*h)
s = f(a) + f(b) + 4*s_odd + 2*s_even
I = h*s/3
function =
x =
for t in arange(0,4,h):
function.append(f(t))
for i in arange(0,4,h):
x.append(i)
function_int = integrate.cumtrapz(function,x,initial=0)
plot(x,function_int)
show()
print(I)
This produces a graph of the integral and prints the final value of the integral itself. Hooray!
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53455648%2fhow-do-i-plot-the-integral-of-e-t2-from-x-0-to-x-3-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Here's a script I wrote that performs your calculation and plots it using PyQtGraph:
from pyqtgraph.Qt import QtGui, QtCore
import pyqtgraph as pg
from math import exp
class I:
def f(self,t):
return exp(-(t**2))
def __init__(self, a = 0, b = 3, h = 0.1):
N = int((b-a)/h)
s_even = s_odd = 0
for k in range(1,N,2):
s_odd += self.f(a+k*h)
for k in range(2,N,2):
s_even += self.f(a+k*h)
s = self.f(a) + self.f(b) + 4*s_odd + 2*s_even
self.I = h*s/3
def __str__(self):
return "I: %s" % self.I
def plot(array):
app = QtGui.QApplication()
win = pg.GraphicsWindow(title="Basic plotting examples")
win.resize(1000,600)
win.setWindowTitle('pyqtgraph example: Plotting')
# Enable antialiasing for prettier plots
pg.setConfigOptions(antialias=True)
p1 = win.addPlot(title="Basic array plotting", y=array)
QtGui.QApplication.instance().exec_()
def main():
a=0
b=a+0.001
points=
while(a<3):
points.append(I(a,b).I)
a=b
b=a+0.001
plot(points)
## Start Qt event loop unless running in interactive mode or using pyside.
if __name__ == '__main__':
main()
Here is the graph it draws:
1
From your graph it looks like you are plotting something like the exponential function itselfe^(-x**2)
rather than its integral.
– Rory Daulton
Nov 24 '18 at 10:29
I am just demonstrating how one can create a graph in Python by plotting what the OP states to be the integral. If it is not an integral hopefully the OP will see your comment and realize his mistake.
– Red Cricket
Nov 25 '18 at 18:36
add a comment |
Here's a script I wrote that performs your calculation and plots it using PyQtGraph:
from pyqtgraph.Qt import QtGui, QtCore
import pyqtgraph as pg
from math import exp
class I:
def f(self,t):
return exp(-(t**2))
def __init__(self, a = 0, b = 3, h = 0.1):
N = int((b-a)/h)
s_even = s_odd = 0
for k in range(1,N,2):
s_odd += self.f(a+k*h)
for k in range(2,N,2):
s_even += self.f(a+k*h)
s = self.f(a) + self.f(b) + 4*s_odd + 2*s_even
self.I = h*s/3
def __str__(self):
return "I: %s" % self.I
def plot(array):
app = QtGui.QApplication()
win = pg.GraphicsWindow(title="Basic plotting examples")
win.resize(1000,600)
win.setWindowTitle('pyqtgraph example: Plotting')
# Enable antialiasing for prettier plots
pg.setConfigOptions(antialias=True)
p1 = win.addPlot(title="Basic array plotting", y=array)
QtGui.QApplication.instance().exec_()
def main():
a=0
b=a+0.001
points=
while(a<3):
points.append(I(a,b).I)
a=b
b=a+0.001
plot(points)
## Start Qt event loop unless running in interactive mode or using pyside.
if __name__ == '__main__':
main()
Here is the graph it draws:
1
From your graph it looks like you are plotting something like the exponential function itselfe^(-x**2)
rather than its integral.
– Rory Daulton
Nov 24 '18 at 10:29
I am just demonstrating how one can create a graph in Python by plotting what the OP states to be the integral. If it is not an integral hopefully the OP will see your comment and realize his mistake.
– Red Cricket
Nov 25 '18 at 18:36
add a comment |
Here's a script I wrote that performs your calculation and plots it using PyQtGraph:
from pyqtgraph.Qt import QtGui, QtCore
import pyqtgraph as pg
from math import exp
class I:
def f(self,t):
return exp(-(t**2))
def __init__(self, a = 0, b = 3, h = 0.1):
N = int((b-a)/h)
s_even = s_odd = 0
for k in range(1,N,2):
s_odd += self.f(a+k*h)
for k in range(2,N,2):
s_even += self.f(a+k*h)
s = self.f(a) + self.f(b) + 4*s_odd + 2*s_even
self.I = h*s/3
def __str__(self):
return "I: %s" % self.I
def plot(array):
app = QtGui.QApplication()
win = pg.GraphicsWindow(title="Basic plotting examples")
win.resize(1000,600)
win.setWindowTitle('pyqtgraph example: Plotting')
# Enable antialiasing for prettier plots
pg.setConfigOptions(antialias=True)
p1 = win.addPlot(title="Basic array plotting", y=array)
QtGui.QApplication.instance().exec_()
def main():
a=0
b=a+0.001
points=
while(a<3):
points.append(I(a,b).I)
a=b
b=a+0.001
plot(points)
## Start Qt event loop unless running in interactive mode or using pyside.
if __name__ == '__main__':
main()
Here is the graph it draws:
Here's a script I wrote that performs your calculation and plots it using PyQtGraph:
from pyqtgraph.Qt import QtGui, QtCore
import pyqtgraph as pg
from math import exp
class I:
def f(self,t):
return exp(-(t**2))
def __init__(self, a = 0, b = 3, h = 0.1):
N = int((b-a)/h)
s_even = s_odd = 0
for k in range(1,N,2):
s_odd += self.f(a+k*h)
for k in range(2,N,2):
s_even += self.f(a+k*h)
s = self.f(a) + self.f(b) + 4*s_odd + 2*s_even
self.I = h*s/3
def __str__(self):
return "I: %s" % self.I
def plot(array):
app = QtGui.QApplication()
win = pg.GraphicsWindow(title="Basic plotting examples")
win.resize(1000,600)
win.setWindowTitle('pyqtgraph example: Plotting')
# Enable antialiasing for prettier plots
pg.setConfigOptions(antialias=True)
p1 = win.addPlot(title="Basic array plotting", y=array)
QtGui.QApplication.instance().exec_()
def main():
a=0
b=a+0.001
points=
while(a<3):
points.append(I(a,b).I)
a=b
b=a+0.001
plot(points)
## Start Qt event loop unless running in interactive mode or using pyside.
if __name__ == '__main__':
main()
Here is the graph it draws:
edited Nov 24 '18 at 7:09
answered Nov 24 '18 at 6:46


Red CricketRed Cricket
4,454103386
4,454103386
1
From your graph it looks like you are plotting something like the exponential function itselfe^(-x**2)
rather than its integral.
– Rory Daulton
Nov 24 '18 at 10:29
I am just demonstrating how one can create a graph in Python by plotting what the OP states to be the integral. If it is not an integral hopefully the OP will see your comment and realize his mistake.
– Red Cricket
Nov 25 '18 at 18:36
add a comment |
1
From your graph it looks like you are plotting something like the exponential function itselfe^(-x**2)
rather than its integral.
– Rory Daulton
Nov 24 '18 at 10:29
I am just demonstrating how one can create a graph in Python by plotting what the OP states to be the integral. If it is not an integral hopefully the OP will see your comment and realize his mistake.
– Red Cricket
Nov 25 '18 at 18:36
1
1
From your graph it looks like you are plotting something like the exponential function itself
e^(-x**2)
rather than its integral.– Rory Daulton
Nov 24 '18 at 10:29
From your graph it looks like you are plotting something like the exponential function itself
e^(-x**2)
rather than its integral.– Rory Daulton
Nov 24 '18 at 10:29
I am just demonstrating how one can create a graph in Python by plotting what the OP states to be the integral. If it is not an integral hopefully the OP will see your comment and realize his mistake.
– Red Cricket
Nov 25 '18 at 18:36
I am just demonstrating how one can create a graph in Python by plotting what the OP states to be the integral. If it is not an integral hopefully the OP will see your comment and realize his mistake.
– Red Cricket
Nov 25 '18 at 18:36
add a comment |
Thanks for your help Red Cricket. It looks like you may have graphed the function e^(-t^2) rather than the integral of that function. Nonetheless, I think I've worked it out; I've discovered scipy has an integrate function:
from math import exp
from numpy import arange
from scipy import integrate
def f(t):
return exp(-(t**2))
a = 0
b = 3
h = 0.1
N = int((b-a)/h)
s_even = 0
s_odd = 0
for k in range(1,N,2):
s_odd += f(a+k*h)
for k in range(2,N,2):
s_even += f(a+k*h)
s = f(a) + f(b) + 4*s_odd + 2*s_even
I = h*s/3
function =
x =
for t in arange(0,4,h):
function.append(f(t))
for i in arange(0,4,h):
x.append(i)
function_int = integrate.cumtrapz(function,x,initial=0)
plot(x,function_int)
show()
print(I)
This produces a graph of the integral and prints the final value of the integral itself. Hooray!
add a comment |
Thanks for your help Red Cricket. It looks like you may have graphed the function e^(-t^2) rather than the integral of that function. Nonetheless, I think I've worked it out; I've discovered scipy has an integrate function:
from math import exp
from numpy import arange
from scipy import integrate
def f(t):
return exp(-(t**2))
a = 0
b = 3
h = 0.1
N = int((b-a)/h)
s_even = 0
s_odd = 0
for k in range(1,N,2):
s_odd += f(a+k*h)
for k in range(2,N,2):
s_even += f(a+k*h)
s = f(a) + f(b) + 4*s_odd + 2*s_even
I = h*s/3
function =
x =
for t in arange(0,4,h):
function.append(f(t))
for i in arange(0,4,h):
x.append(i)
function_int = integrate.cumtrapz(function,x,initial=0)
plot(x,function_int)
show()
print(I)
This produces a graph of the integral and prints the final value of the integral itself. Hooray!
add a comment |
Thanks for your help Red Cricket. It looks like you may have graphed the function e^(-t^2) rather than the integral of that function. Nonetheless, I think I've worked it out; I've discovered scipy has an integrate function:
from math import exp
from numpy import arange
from scipy import integrate
def f(t):
return exp(-(t**2))
a = 0
b = 3
h = 0.1
N = int((b-a)/h)
s_even = 0
s_odd = 0
for k in range(1,N,2):
s_odd += f(a+k*h)
for k in range(2,N,2):
s_even += f(a+k*h)
s = f(a) + f(b) + 4*s_odd + 2*s_even
I = h*s/3
function =
x =
for t in arange(0,4,h):
function.append(f(t))
for i in arange(0,4,h):
x.append(i)
function_int = integrate.cumtrapz(function,x,initial=0)
plot(x,function_int)
show()
print(I)
This produces a graph of the integral and prints the final value of the integral itself. Hooray!
Thanks for your help Red Cricket. It looks like you may have graphed the function e^(-t^2) rather than the integral of that function. Nonetheless, I think I've worked it out; I've discovered scipy has an integrate function:
from math import exp
from numpy import arange
from scipy import integrate
def f(t):
return exp(-(t**2))
a = 0
b = 3
h = 0.1
N = int((b-a)/h)
s_even = 0
s_odd = 0
for k in range(1,N,2):
s_odd += f(a+k*h)
for k in range(2,N,2):
s_even += f(a+k*h)
s = f(a) + f(b) + 4*s_odd + 2*s_even
I = h*s/3
function =
x =
for t in arange(0,4,h):
function.append(f(t))
for i in arange(0,4,h):
x.append(i)
function_int = integrate.cumtrapz(function,x,initial=0)
plot(x,function_int)
show()
print(I)
This produces a graph of the integral and prints the final value of the integral itself. Hooray!
answered Nov 27 '18 at 11:19


CameronCameron
94
94
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53455648%2fhow-do-i-plot-the-integral-of-e-t2-from-x-0-to-x-3-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
t,xTaQF,Lft,4 KhBmUCvra9 p 1URLBzmVwb,2rfdU,X9FDUumyG Yb
Please try to find a more concrete title. What exactly are you struggeling with? Can you formulate a single question, so that the answer would help you?
– Martin Thoma
Nov 24 '18 at 6:14
If you are using version 3.2 or later then you can use math.erf for this; see en.wikipedia.org/wiki/Error_function for example
– dmuir
Nov 24 '18 at 12:58