Recall variable value using quosure
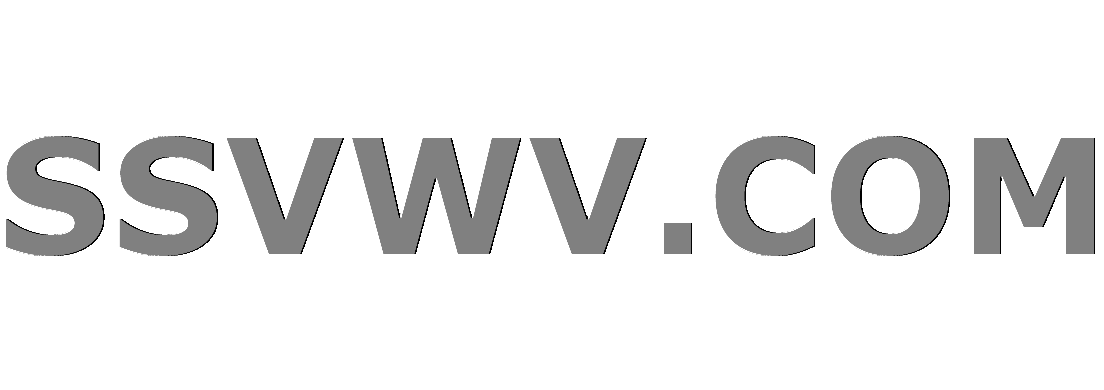
Multi tool use
I would like to recall the value of the variable dynamically in a loop. Here is an example of the problem. I am new to tidyverse and not great at quos and quosures.
library (tidyverse)
mtcars.df <- mtcars
mtcars.df <- mtcars.df %>% rownames_to_column( var = "Car")
temp.df <- mtcars %>% select(mpg, cyl, disp)
temp.df <- temp.df %>% rownames_to_column( var = "Car")
sample.name.temp <- "mpg_temp"
sample.name <- unlist(strsplit(as.character(sample.name.temp), '_', fixed = TRUE))[1]
names (temp.df)[2] <- paste0("DUM_", sample.name)
temp.name <- paste0("DUM_", sample.name)
mtcars.join <- full_join(mtcars.df, temp.df, by = c("cyl", "disp", "Car"))
This gives the desired output:
mtcars.join <- mtcars.join %>% mutate (DUM_mpg = case_when(
mpg <= 20 ~ 0,
TRUE ~ mpg
))
However, as noted above I am using this in a loop and need to recall the variable names: temp.name
and sample.name
dynamically within the loop. Below are my failed attempts.
mtcars.join <- mtcars.join %>% mutate (!!!temp.name = case_when(
!!!sample.name <= 20 ~ 0,
TRUE ~ !!!temp.name
))
mtcars.join <- mtcars.join %>% mutate (!!!rlang::syms(temp.name) = case_when(
!!!rlang::syms(sample.name) <= 20 ~ 0,
TRUE ~ !!!rlang::syms(temp.name)
))
mtcars.join <- mtcars.join %>% mutate (!!!rlang::syms(temp.name) = case_when(
!!!rlang::syms(sample.name) <= 20 ~ 0,
TRUE ~ !!!rlang::syms(temp.name)
))
mtcars.join <- mtcars.join %>% mutate (!!temp.name = case_when(
!!sample.name <= 20 ~ 0,
TRUE ~ !!temp.name
))
mtcars.join <- mtcars.join %>% mutate (mtcars.join[[temp.name]] = case_when(
mtcars.join [[sample.name]] <= 20 ~ 0,
TRUE ~ mtcars.join [[temp.name]]
))
Thanks for the help.
r loops dplyr tidyverse quosure
add a comment |
I would like to recall the value of the variable dynamically in a loop. Here is an example of the problem. I am new to tidyverse and not great at quos and quosures.
library (tidyverse)
mtcars.df <- mtcars
mtcars.df <- mtcars.df %>% rownames_to_column( var = "Car")
temp.df <- mtcars %>% select(mpg, cyl, disp)
temp.df <- temp.df %>% rownames_to_column( var = "Car")
sample.name.temp <- "mpg_temp"
sample.name <- unlist(strsplit(as.character(sample.name.temp), '_', fixed = TRUE))[1]
names (temp.df)[2] <- paste0("DUM_", sample.name)
temp.name <- paste0("DUM_", sample.name)
mtcars.join <- full_join(mtcars.df, temp.df, by = c("cyl", "disp", "Car"))
This gives the desired output:
mtcars.join <- mtcars.join %>% mutate (DUM_mpg = case_when(
mpg <= 20 ~ 0,
TRUE ~ mpg
))
However, as noted above I am using this in a loop and need to recall the variable names: temp.name
and sample.name
dynamically within the loop. Below are my failed attempts.
mtcars.join <- mtcars.join %>% mutate (!!!temp.name = case_when(
!!!sample.name <= 20 ~ 0,
TRUE ~ !!!temp.name
))
mtcars.join <- mtcars.join %>% mutate (!!!rlang::syms(temp.name) = case_when(
!!!rlang::syms(sample.name) <= 20 ~ 0,
TRUE ~ !!!rlang::syms(temp.name)
))
mtcars.join <- mtcars.join %>% mutate (!!!rlang::syms(temp.name) = case_when(
!!!rlang::syms(sample.name) <= 20 ~ 0,
TRUE ~ !!!rlang::syms(temp.name)
))
mtcars.join <- mtcars.join %>% mutate (!!temp.name = case_when(
!!sample.name <= 20 ~ 0,
TRUE ~ !!temp.name
))
mtcars.join <- mtcars.join %>% mutate (mtcars.join[[temp.name]] = case_when(
mtcars.join [[sample.name]] <= 20 ~ 0,
TRUE ~ mtcars.join [[temp.name]]
))
Thanks for the help.
r loops dplyr tidyverse quosure
add a comment |
I would like to recall the value of the variable dynamically in a loop. Here is an example of the problem. I am new to tidyverse and not great at quos and quosures.
library (tidyverse)
mtcars.df <- mtcars
mtcars.df <- mtcars.df %>% rownames_to_column( var = "Car")
temp.df <- mtcars %>% select(mpg, cyl, disp)
temp.df <- temp.df %>% rownames_to_column( var = "Car")
sample.name.temp <- "mpg_temp"
sample.name <- unlist(strsplit(as.character(sample.name.temp), '_', fixed = TRUE))[1]
names (temp.df)[2] <- paste0("DUM_", sample.name)
temp.name <- paste0("DUM_", sample.name)
mtcars.join <- full_join(mtcars.df, temp.df, by = c("cyl", "disp", "Car"))
This gives the desired output:
mtcars.join <- mtcars.join %>% mutate (DUM_mpg = case_when(
mpg <= 20 ~ 0,
TRUE ~ mpg
))
However, as noted above I am using this in a loop and need to recall the variable names: temp.name
and sample.name
dynamically within the loop. Below are my failed attempts.
mtcars.join <- mtcars.join %>% mutate (!!!temp.name = case_when(
!!!sample.name <= 20 ~ 0,
TRUE ~ !!!temp.name
))
mtcars.join <- mtcars.join %>% mutate (!!!rlang::syms(temp.name) = case_when(
!!!rlang::syms(sample.name) <= 20 ~ 0,
TRUE ~ !!!rlang::syms(temp.name)
))
mtcars.join <- mtcars.join %>% mutate (!!!rlang::syms(temp.name) = case_when(
!!!rlang::syms(sample.name) <= 20 ~ 0,
TRUE ~ !!!rlang::syms(temp.name)
))
mtcars.join <- mtcars.join %>% mutate (!!temp.name = case_when(
!!sample.name <= 20 ~ 0,
TRUE ~ !!temp.name
))
mtcars.join <- mtcars.join %>% mutate (mtcars.join[[temp.name]] = case_when(
mtcars.join [[sample.name]] <= 20 ~ 0,
TRUE ~ mtcars.join [[temp.name]]
))
Thanks for the help.
r loops dplyr tidyverse quosure
I would like to recall the value of the variable dynamically in a loop. Here is an example of the problem. I am new to tidyverse and not great at quos and quosures.
library (tidyverse)
mtcars.df <- mtcars
mtcars.df <- mtcars.df %>% rownames_to_column( var = "Car")
temp.df <- mtcars %>% select(mpg, cyl, disp)
temp.df <- temp.df %>% rownames_to_column( var = "Car")
sample.name.temp <- "mpg_temp"
sample.name <- unlist(strsplit(as.character(sample.name.temp), '_', fixed = TRUE))[1]
names (temp.df)[2] <- paste0("DUM_", sample.name)
temp.name <- paste0("DUM_", sample.name)
mtcars.join <- full_join(mtcars.df, temp.df, by = c("cyl", "disp", "Car"))
This gives the desired output:
mtcars.join <- mtcars.join %>% mutate (DUM_mpg = case_when(
mpg <= 20 ~ 0,
TRUE ~ mpg
))
However, as noted above I am using this in a loop and need to recall the variable names: temp.name
and sample.name
dynamically within the loop. Below are my failed attempts.
mtcars.join <- mtcars.join %>% mutate (!!!temp.name = case_when(
!!!sample.name <= 20 ~ 0,
TRUE ~ !!!temp.name
))
mtcars.join <- mtcars.join %>% mutate (!!!rlang::syms(temp.name) = case_when(
!!!rlang::syms(sample.name) <= 20 ~ 0,
TRUE ~ !!!rlang::syms(temp.name)
))
mtcars.join <- mtcars.join %>% mutate (!!!rlang::syms(temp.name) = case_when(
!!!rlang::syms(sample.name) <= 20 ~ 0,
TRUE ~ !!!rlang::syms(temp.name)
))
mtcars.join <- mtcars.join %>% mutate (!!temp.name = case_when(
!!sample.name <= 20 ~ 0,
TRUE ~ !!temp.name
))
mtcars.join <- mtcars.join %>% mutate (mtcars.join[[temp.name]] = case_when(
mtcars.join [[sample.name]] <= 20 ~ 0,
TRUE ~ mtcars.join [[temp.name]]
))
Thanks for the help.
r loops dplyr tidyverse quosure
r loops dplyr tidyverse quosure
asked Nov 23 '18 at 16:25


KP1KP1
525
525
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
We need to use :=
. Also, if there is a single element, evaluation can be done with !!
mtcars.join %>%
mutate(!! temp.name := case_when(!! rlang::sym(sample.name) <= 20 ~ 0,
TRUE ~ !! rlang::sym(temp.name)))
# Car mpg cyl disp hp drat wt qsec vs am gear carb DUM_mpg
#1 Mazda RX4 21.0 6 160.0 110 3.90 2.620 16.46 0 1 4 4 21.0
#2 Mazda RX4 Wag 21.0 6 160.0 110 3.90 2.875 17.02 0 1 4 4 21.0
#3 Datsun 710 22.8 4 108.0 93 3.85 2.320 18.61 1 1 4 1 22.8
#4 Hornet 4 Drive 21.4 6 258.0 110 3.08 3.215 19.44 1 0 3 1 21.4
#5 Hornet Sportabout 18.7 8 360.0 175 3.15 3.440 17.02 0 0 3 2 0.0
#6 Valiant 18.1 6 225.0 105 2.76 3.460 20.22 1 0 3 1 0.0
#7 Duster 360 14.3 8 360.0 245 3.21 3.570 15.84 0 0 3 4 0.0
#...
#...
1
Thanks. Will have to check out!!
– KP1
Nov 23 '18 at 17:11
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450080%2frecall-variable-value-using-quosure%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
We need to use :=
. Also, if there is a single element, evaluation can be done with !!
mtcars.join %>%
mutate(!! temp.name := case_when(!! rlang::sym(sample.name) <= 20 ~ 0,
TRUE ~ !! rlang::sym(temp.name)))
# Car mpg cyl disp hp drat wt qsec vs am gear carb DUM_mpg
#1 Mazda RX4 21.0 6 160.0 110 3.90 2.620 16.46 0 1 4 4 21.0
#2 Mazda RX4 Wag 21.0 6 160.0 110 3.90 2.875 17.02 0 1 4 4 21.0
#3 Datsun 710 22.8 4 108.0 93 3.85 2.320 18.61 1 1 4 1 22.8
#4 Hornet 4 Drive 21.4 6 258.0 110 3.08 3.215 19.44 1 0 3 1 21.4
#5 Hornet Sportabout 18.7 8 360.0 175 3.15 3.440 17.02 0 0 3 2 0.0
#6 Valiant 18.1 6 225.0 105 2.76 3.460 20.22 1 0 3 1 0.0
#7 Duster 360 14.3 8 360.0 245 3.21 3.570 15.84 0 0 3 4 0.0
#...
#...
1
Thanks. Will have to check out!!
– KP1
Nov 23 '18 at 17:11
add a comment |
We need to use :=
. Also, if there is a single element, evaluation can be done with !!
mtcars.join %>%
mutate(!! temp.name := case_when(!! rlang::sym(sample.name) <= 20 ~ 0,
TRUE ~ !! rlang::sym(temp.name)))
# Car mpg cyl disp hp drat wt qsec vs am gear carb DUM_mpg
#1 Mazda RX4 21.0 6 160.0 110 3.90 2.620 16.46 0 1 4 4 21.0
#2 Mazda RX4 Wag 21.0 6 160.0 110 3.90 2.875 17.02 0 1 4 4 21.0
#3 Datsun 710 22.8 4 108.0 93 3.85 2.320 18.61 1 1 4 1 22.8
#4 Hornet 4 Drive 21.4 6 258.0 110 3.08 3.215 19.44 1 0 3 1 21.4
#5 Hornet Sportabout 18.7 8 360.0 175 3.15 3.440 17.02 0 0 3 2 0.0
#6 Valiant 18.1 6 225.0 105 2.76 3.460 20.22 1 0 3 1 0.0
#7 Duster 360 14.3 8 360.0 245 3.21 3.570 15.84 0 0 3 4 0.0
#...
#...
1
Thanks. Will have to check out!!
– KP1
Nov 23 '18 at 17:11
add a comment |
We need to use :=
. Also, if there is a single element, evaluation can be done with !!
mtcars.join %>%
mutate(!! temp.name := case_when(!! rlang::sym(sample.name) <= 20 ~ 0,
TRUE ~ !! rlang::sym(temp.name)))
# Car mpg cyl disp hp drat wt qsec vs am gear carb DUM_mpg
#1 Mazda RX4 21.0 6 160.0 110 3.90 2.620 16.46 0 1 4 4 21.0
#2 Mazda RX4 Wag 21.0 6 160.0 110 3.90 2.875 17.02 0 1 4 4 21.0
#3 Datsun 710 22.8 4 108.0 93 3.85 2.320 18.61 1 1 4 1 22.8
#4 Hornet 4 Drive 21.4 6 258.0 110 3.08 3.215 19.44 1 0 3 1 21.4
#5 Hornet Sportabout 18.7 8 360.0 175 3.15 3.440 17.02 0 0 3 2 0.0
#6 Valiant 18.1 6 225.0 105 2.76 3.460 20.22 1 0 3 1 0.0
#7 Duster 360 14.3 8 360.0 245 3.21 3.570 15.84 0 0 3 4 0.0
#...
#...
We need to use :=
. Also, if there is a single element, evaluation can be done with !!
mtcars.join %>%
mutate(!! temp.name := case_when(!! rlang::sym(sample.name) <= 20 ~ 0,
TRUE ~ !! rlang::sym(temp.name)))
# Car mpg cyl disp hp drat wt qsec vs am gear carb DUM_mpg
#1 Mazda RX4 21.0 6 160.0 110 3.90 2.620 16.46 0 1 4 4 21.0
#2 Mazda RX4 Wag 21.0 6 160.0 110 3.90 2.875 17.02 0 1 4 4 21.0
#3 Datsun 710 22.8 4 108.0 93 3.85 2.320 18.61 1 1 4 1 22.8
#4 Hornet 4 Drive 21.4 6 258.0 110 3.08 3.215 19.44 1 0 3 1 21.4
#5 Hornet Sportabout 18.7 8 360.0 175 3.15 3.440 17.02 0 0 3 2 0.0
#6 Valiant 18.1 6 225.0 105 2.76 3.460 20.22 1 0 3 1 0.0
#7 Duster 360 14.3 8 360.0 245 3.21 3.570 15.84 0 0 3 4 0.0
#...
#...
edited Nov 23 '18 at 16:44
answered Nov 23 '18 at 16:28
akrunakrun
406k13197272
406k13197272
1
Thanks. Will have to check out!!
– KP1
Nov 23 '18 at 17:11
add a comment |
1
Thanks. Will have to check out!!
– KP1
Nov 23 '18 at 17:11
1
1
Thanks. Will have to check out
!!
– KP1
Nov 23 '18 at 17:11
Thanks. Will have to check out
!!
– KP1
Nov 23 '18 at 17:11
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450080%2frecall-variable-value-using-quosure%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sMEcbI4k70eog X H5Kt k9jPgs CuG5DI8AZD Rm93Udebu1PFa0U576 C1q,vp,tnohx4xmYXkbf2ZCvpXGda Onz,MJV,v677