Comparing user id with user id from textfile
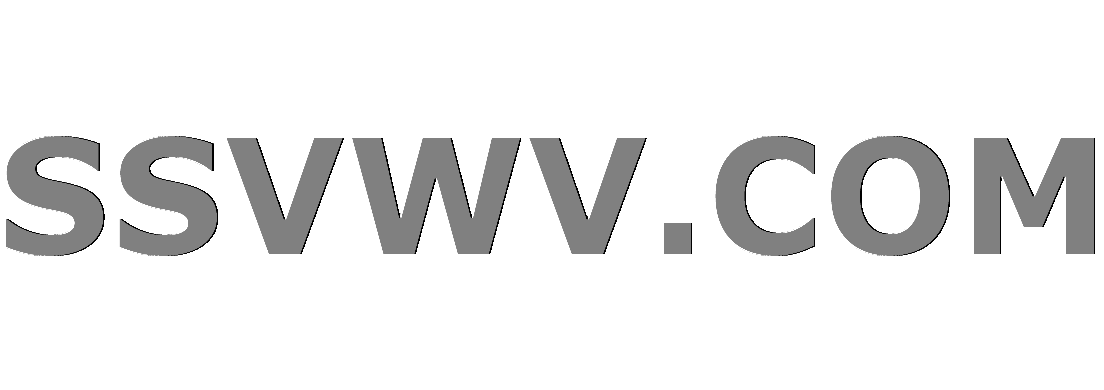
Multi tool use
I want to add user information and reject if user id already exists. Currently, my code displays "ID already Exists!" but still adds the user information.
this is my code:
String id = EditManagerID.getText();
String password = EditManagerPassword.getText();
String name = EditManagerName.getText();
String contact = EditManagerContact.getText();
String address = EditManagerAddress.getText();
String role = EditManagerRole.getText();
try
{
String reader;
BufferedReader br = new BufferedReader(new FileReader("ManagerDetails.txt"));
while ((reader = br.readLine())!=null)
{
String split = reader.split(",");
if (id.equals(split[0]))
{
JOptionPane.showMessageDialog(null, "ID already exists!", "Warning", JOptionPane.WARNING_MESSAGE);
}
}
}
catch(Exception e)
{
}
if(id.isEmpty() || password.isEmpty() || name.isEmpty() || contact.isEmpty() || address.isEmpty() || role.isEmpty())
{
JOptionPane.showMessageDialog(null, "Please Fill In Everything!", "Error", JOptionPane.ERROR_MESSAGE);
}
else
{
ClassUser u = new ClassUser (id, password, name, address, contact, role);
File f = new File("ManagerDetails.txt");
try(PrintWriter pw = new PrintWriter(new FileWriter(f, true))){
pw.println(u.toString()); //print into txtfile
JOptionPane.showMessageDialog(this, "Added Successfully!");
} catch(Exception e){
JOptionPane.showMessageDialog(null, "Invalid Input!", "Error", JOptionPane.ERROR_MESSAGE);
}
I tried to add an 'if-else' but not sure what to put as a statement.
java swing netbeans
add a comment |
I want to add user information and reject if user id already exists. Currently, my code displays "ID already Exists!" but still adds the user information.
this is my code:
String id = EditManagerID.getText();
String password = EditManagerPassword.getText();
String name = EditManagerName.getText();
String contact = EditManagerContact.getText();
String address = EditManagerAddress.getText();
String role = EditManagerRole.getText();
try
{
String reader;
BufferedReader br = new BufferedReader(new FileReader("ManagerDetails.txt"));
while ((reader = br.readLine())!=null)
{
String split = reader.split(",");
if (id.equals(split[0]))
{
JOptionPane.showMessageDialog(null, "ID already exists!", "Warning", JOptionPane.WARNING_MESSAGE);
}
}
}
catch(Exception e)
{
}
if(id.isEmpty() || password.isEmpty() || name.isEmpty() || contact.isEmpty() || address.isEmpty() || role.isEmpty())
{
JOptionPane.showMessageDialog(null, "Please Fill In Everything!", "Error", JOptionPane.ERROR_MESSAGE);
}
else
{
ClassUser u = new ClassUser (id, password, name, address, contact, role);
File f = new File("ManagerDetails.txt");
try(PrintWriter pw = new PrintWriter(new FileWriter(f, true))){
pw.println(u.toString()); //print into txtfile
JOptionPane.showMessageDialog(this, "Added Successfully!");
} catch(Exception e){
JOptionPane.showMessageDialog(null, "Invalid Input!", "Error", JOptionPane.ERROR_MESSAGE);
}
I tried to add an 'if-else' but not sure what to put as a statement.
java swing netbeans
add a comment |
I want to add user information and reject if user id already exists. Currently, my code displays "ID already Exists!" but still adds the user information.
this is my code:
String id = EditManagerID.getText();
String password = EditManagerPassword.getText();
String name = EditManagerName.getText();
String contact = EditManagerContact.getText();
String address = EditManagerAddress.getText();
String role = EditManagerRole.getText();
try
{
String reader;
BufferedReader br = new BufferedReader(new FileReader("ManagerDetails.txt"));
while ((reader = br.readLine())!=null)
{
String split = reader.split(",");
if (id.equals(split[0]))
{
JOptionPane.showMessageDialog(null, "ID already exists!", "Warning", JOptionPane.WARNING_MESSAGE);
}
}
}
catch(Exception e)
{
}
if(id.isEmpty() || password.isEmpty() || name.isEmpty() || contact.isEmpty() || address.isEmpty() || role.isEmpty())
{
JOptionPane.showMessageDialog(null, "Please Fill In Everything!", "Error", JOptionPane.ERROR_MESSAGE);
}
else
{
ClassUser u = new ClassUser (id, password, name, address, contact, role);
File f = new File("ManagerDetails.txt");
try(PrintWriter pw = new PrintWriter(new FileWriter(f, true))){
pw.println(u.toString()); //print into txtfile
JOptionPane.showMessageDialog(this, "Added Successfully!");
} catch(Exception e){
JOptionPane.showMessageDialog(null, "Invalid Input!", "Error", JOptionPane.ERROR_MESSAGE);
}
I tried to add an 'if-else' but not sure what to put as a statement.
java swing netbeans
I want to add user information and reject if user id already exists. Currently, my code displays "ID already Exists!" but still adds the user information.
this is my code:
String id = EditManagerID.getText();
String password = EditManagerPassword.getText();
String name = EditManagerName.getText();
String contact = EditManagerContact.getText();
String address = EditManagerAddress.getText();
String role = EditManagerRole.getText();
try
{
String reader;
BufferedReader br = new BufferedReader(new FileReader("ManagerDetails.txt"));
while ((reader = br.readLine())!=null)
{
String split = reader.split(",");
if (id.equals(split[0]))
{
JOptionPane.showMessageDialog(null, "ID already exists!", "Warning", JOptionPane.WARNING_MESSAGE);
}
}
}
catch(Exception e)
{
}
if(id.isEmpty() || password.isEmpty() || name.isEmpty() || contact.isEmpty() || address.isEmpty() || role.isEmpty())
{
JOptionPane.showMessageDialog(null, "Please Fill In Everything!", "Error", JOptionPane.ERROR_MESSAGE);
}
else
{
ClassUser u = new ClassUser (id, password, name, address, contact, role);
File f = new File("ManagerDetails.txt");
try(PrintWriter pw = new PrintWriter(new FileWriter(f, true))){
pw.println(u.toString()); //print into txtfile
JOptionPane.showMessageDialog(this, "Added Successfully!");
} catch(Exception e){
JOptionPane.showMessageDialog(null, "Invalid Input!", "Error", JOptionPane.ERROR_MESSAGE);
}
I tried to add an 'if-else' but not sure what to put as a statement.
java swing netbeans
java swing netbeans
edited Nov 23 '18 at 17:04
Tobias Wilfert
7492920
7492920
asked Nov 23 '18 at 16:59
bk23bk23
103
103
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
In your code you 're saying if id already exists show message "ID already exists!" and then you continue adding users info anyway.
In your if id already exists statement set a boolean variable found to true and then make one more if statement and check if found is true or false.
boolean found = false;
// ...
if (id.equals(split[0]))
{
JOptionPane.showMessageDialog(null, "ID already exists!", "Warning",
JOptionPane.WARNING_MESSAGE);
found = true;
//stop the while
break;
}
// ...
//check if id already exists or not. if not then add users info
if(!found){
//put your adding users info code here
}
I've tried this, but it just adds the user infinitely.
– bk23
Nov 23 '18 at 17:19
ohh, my bad. sorry! wait im editing my answer
– oskozach
Nov 23 '18 at 17:27
no problem, take your time!
– bk23
Nov 23 '18 at 17:32
thank you so much zach! i've been looking at my code for the past hour hhaha
– bk23
Nov 23 '18 at 17:41
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450525%2fcomparing-user-id-with-user-id-from-textfile%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In your code you 're saying if id already exists show message "ID already exists!" and then you continue adding users info anyway.
In your if id already exists statement set a boolean variable found to true and then make one more if statement and check if found is true or false.
boolean found = false;
// ...
if (id.equals(split[0]))
{
JOptionPane.showMessageDialog(null, "ID already exists!", "Warning",
JOptionPane.WARNING_MESSAGE);
found = true;
//stop the while
break;
}
// ...
//check if id already exists or not. if not then add users info
if(!found){
//put your adding users info code here
}
I've tried this, but it just adds the user infinitely.
– bk23
Nov 23 '18 at 17:19
ohh, my bad. sorry! wait im editing my answer
– oskozach
Nov 23 '18 at 17:27
no problem, take your time!
– bk23
Nov 23 '18 at 17:32
thank you so much zach! i've been looking at my code for the past hour hhaha
– bk23
Nov 23 '18 at 17:41
add a comment |
In your code you 're saying if id already exists show message "ID already exists!" and then you continue adding users info anyway.
In your if id already exists statement set a boolean variable found to true and then make one more if statement and check if found is true or false.
boolean found = false;
// ...
if (id.equals(split[0]))
{
JOptionPane.showMessageDialog(null, "ID already exists!", "Warning",
JOptionPane.WARNING_MESSAGE);
found = true;
//stop the while
break;
}
// ...
//check if id already exists or not. if not then add users info
if(!found){
//put your adding users info code here
}
I've tried this, but it just adds the user infinitely.
– bk23
Nov 23 '18 at 17:19
ohh, my bad. sorry! wait im editing my answer
– oskozach
Nov 23 '18 at 17:27
no problem, take your time!
– bk23
Nov 23 '18 at 17:32
thank you so much zach! i've been looking at my code for the past hour hhaha
– bk23
Nov 23 '18 at 17:41
add a comment |
In your code you 're saying if id already exists show message "ID already exists!" and then you continue adding users info anyway.
In your if id already exists statement set a boolean variable found to true and then make one more if statement and check if found is true or false.
boolean found = false;
// ...
if (id.equals(split[0]))
{
JOptionPane.showMessageDialog(null, "ID already exists!", "Warning",
JOptionPane.WARNING_MESSAGE);
found = true;
//stop the while
break;
}
// ...
//check if id already exists or not. if not then add users info
if(!found){
//put your adding users info code here
}
In your code you 're saying if id already exists show message "ID already exists!" and then you continue adding users info anyway.
In your if id already exists statement set a boolean variable found to true and then make one more if statement and check if found is true or false.
boolean found = false;
// ...
if (id.equals(split[0]))
{
JOptionPane.showMessageDialog(null, "ID already exists!", "Warning",
JOptionPane.WARNING_MESSAGE);
found = true;
//stop the while
break;
}
// ...
//check if id already exists or not. if not then add users info
if(!found){
//put your adding users info code here
}
edited Nov 23 '18 at 17:37
answered Nov 23 '18 at 17:13


oskozachoskozach
6615
6615
I've tried this, but it just adds the user infinitely.
– bk23
Nov 23 '18 at 17:19
ohh, my bad. sorry! wait im editing my answer
– oskozach
Nov 23 '18 at 17:27
no problem, take your time!
– bk23
Nov 23 '18 at 17:32
thank you so much zach! i've been looking at my code for the past hour hhaha
– bk23
Nov 23 '18 at 17:41
add a comment |
I've tried this, but it just adds the user infinitely.
– bk23
Nov 23 '18 at 17:19
ohh, my bad. sorry! wait im editing my answer
– oskozach
Nov 23 '18 at 17:27
no problem, take your time!
– bk23
Nov 23 '18 at 17:32
thank you so much zach! i've been looking at my code for the past hour hhaha
– bk23
Nov 23 '18 at 17:41
I've tried this, but it just adds the user infinitely.
– bk23
Nov 23 '18 at 17:19
I've tried this, but it just adds the user infinitely.
– bk23
Nov 23 '18 at 17:19
ohh, my bad. sorry! wait im editing my answer
– oskozach
Nov 23 '18 at 17:27
ohh, my bad. sorry! wait im editing my answer
– oskozach
Nov 23 '18 at 17:27
no problem, take your time!
– bk23
Nov 23 '18 at 17:32
no problem, take your time!
– bk23
Nov 23 '18 at 17:32
thank you so much zach! i've been looking at my code for the past hour hhaha
– bk23
Nov 23 '18 at 17:41
thank you so much zach! i've been looking at my code for the past hour hhaha
– bk23
Nov 23 '18 at 17:41
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450525%2fcomparing-user-id-with-user-id-from-textfile%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
x3tNXu45,k 0iyIurgGm PlHXI07G