Vuex add new array property to an object and push elements to that object inside mutation in a reactive...
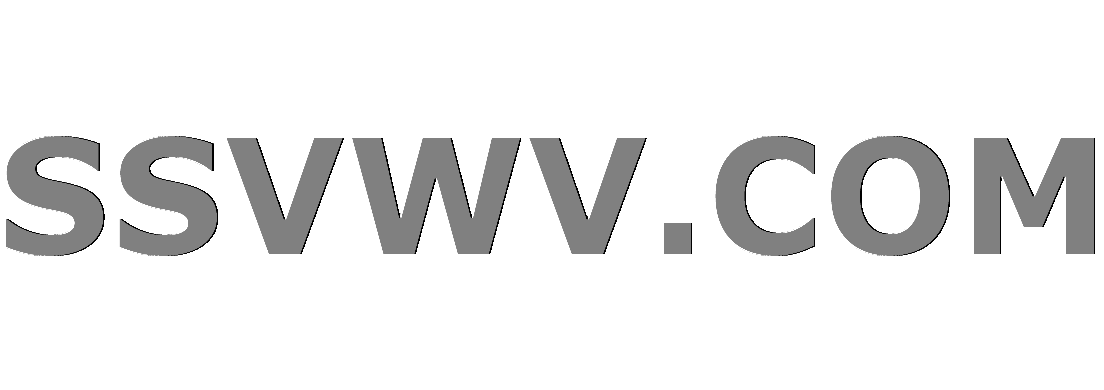
Multi tool use
According to the Official docs, vuex mutations has some limitation in reactivity.
When adding a new property to an object we have to do,
Vue.set(obj, 'newProp', 123)
This is fine. But how do we add new array property and push elements to that without breaking the reactivity?
This is what I have done up to now. This is working fine but the problem is this is not reactive. Getters
can't recognize the changes happen to the state.
set_add_ons(state, payload) {
try {
if (!state.tour_plan[state.tour_plan.length - 1].add_ons) {
state.tour_plan[state.tour_plan.length - 1].add_ons = ;
}
state.tour_plan[state.tour_plan.length - 1].add_ons.push(payload);
} catch (error) {
console.log("ERR", error)
}
},
How do I convert this to a code which is reactive?
vue.js vuejs2 vuex
add a comment |
According to the Official docs, vuex mutations has some limitation in reactivity.
When adding a new property to an object we have to do,
Vue.set(obj, 'newProp', 123)
This is fine. But how do we add new array property and push elements to that without breaking the reactivity?
This is what I have done up to now. This is working fine but the problem is this is not reactive. Getters
can't recognize the changes happen to the state.
set_add_ons(state, payload) {
try {
if (!state.tour_plan[state.tour_plan.length - 1].add_ons) {
state.tour_plan[state.tour_plan.length - 1].add_ons = ;
}
state.tour_plan[state.tour_plan.length - 1].add_ons.push(payload);
} catch (error) {
console.log("ERR", error)
}
},
How do I convert this to a code which is reactive?
vue.js vuejs2 vuex
add a comment |
According to the Official docs, vuex mutations has some limitation in reactivity.
When adding a new property to an object we have to do,
Vue.set(obj, 'newProp', 123)
This is fine. But how do we add new array property and push elements to that without breaking the reactivity?
This is what I have done up to now. This is working fine but the problem is this is not reactive. Getters
can't recognize the changes happen to the state.
set_add_ons(state, payload) {
try {
if (!state.tour_plan[state.tour_plan.length - 1].add_ons) {
state.tour_plan[state.tour_plan.length - 1].add_ons = ;
}
state.tour_plan[state.tour_plan.length - 1].add_ons.push(payload);
} catch (error) {
console.log("ERR", error)
}
},
How do I convert this to a code which is reactive?
vue.js vuejs2 vuex
According to the Official docs, vuex mutations has some limitation in reactivity.
When adding a new property to an object we have to do,
Vue.set(obj, 'newProp', 123)
This is fine. But how do we add new array property and push elements to that without breaking the reactivity?
This is what I have done up to now. This is working fine but the problem is this is not reactive. Getters
can't recognize the changes happen to the state.
set_add_ons(state, payload) {
try {
if (!state.tour_plan[state.tour_plan.length - 1].add_ons) {
state.tour_plan[state.tour_plan.length - 1].add_ons = ;
}
state.tour_plan[state.tour_plan.length - 1].add_ons.push(payload);
} catch (error) {
console.log("ERR", error)
}
},
How do I convert this to a code which is reactive?
vue.js vuejs2 vuex
vue.js vuejs2 vuex
asked Nov 23 '18 at 4:56


Pathum SamararathnaPathum Samararathna
782723
782723
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You can use Vue.set
to create the add_ons
attribute to make it reactive; It should work for array too:
Vue.set(state.tour_plan[state.tour_plan.length - 1], 'add_ons', );
Demo:
new Vue({
el: '#app',
data: {
items: [{ id: 0 }]
},
methods: {
addArray () {
if (!this.items[this.items.length-1].add_on)
this.$set(this.items[this.items.length - 1], 'add_on', )
this.items[this.items.length - 1].add_on.push(1)
}
}
})
<script src="https://unpkg.com/vue"></script>
<div id="app">
<button v-on:click="addArray">
Add 1 to add_on create if not exists
</button>
<div v-for="item in items">add_on content: {{ item.add_on }}</div>
</div>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440846%2fvuex-add-new-array-property-to-an-object-and-push-elements-to-that-object-inside%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use Vue.set
to create the add_ons
attribute to make it reactive; It should work for array too:
Vue.set(state.tour_plan[state.tour_plan.length - 1], 'add_ons', );
Demo:
new Vue({
el: '#app',
data: {
items: [{ id: 0 }]
},
methods: {
addArray () {
if (!this.items[this.items.length-1].add_on)
this.$set(this.items[this.items.length - 1], 'add_on', )
this.items[this.items.length - 1].add_on.push(1)
}
}
})
<script src="https://unpkg.com/vue"></script>
<div id="app">
<button v-on:click="addArray">
Add 1 to add_on create if not exists
</button>
<div v-for="item in items">add_on content: {{ item.add_on }}</div>
</div>
add a comment |
You can use Vue.set
to create the add_ons
attribute to make it reactive; It should work for array too:
Vue.set(state.tour_plan[state.tour_plan.length - 1], 'add_ons', );
Demo:
new Vue({
el: '#app',
data: {
items: [{ id: 0 }]
},
methods: {
addArray () {
if (!this.items[this.items.length-1].add_on)
this.$set(this.items[this.items.length - 1], 'add_on', )
this.items[this.items.length - 1].add_on.push(1)
}
}
})
<script src="https://unpkg.com/vue"></script>
<div id="app">
<button v-on:click="addArray">
Add 1 to add_on create if not exists
</button>
<div v-for="item in items">add_on content: {{ item.add_on }}</div>
</div>
add a comment |
You can use Vue.set
to create the add_ons
attribute to make it reactive; It should work for array too:
Vue.set(state.tour_plan[state.tour_plan.length - 1], 'add_ons', );
Demo:
new Vue({
el: '#app',
data: {
items: [{ id: 0 }]
},
methods: {
addArray () {
if (!this.items[this.items.length-1].add_on)
this.$set(this.items[this.items.length - 1], 'add_on', )
this.items[this.items.length - 1].add_on.push(1)
}
}
})
<script src="https://unpkg.com/vue"></script>
<div id="app">
<button v-on:click="addArray">
Add 1 to add_on create if not exists
</button>
<div v-for="item in items">add_on content: {{ item.add_on }}</div>
</div>
You can use Vue.set
to create the add_ons
attribute to make it reactive; It should work for array too:
Vue.set(state.tour_plan[state.tour_plan.length - 1], 'add_ons', );
Demo:
new Vue({
el: '#app',
data: {
items: [{ id: 0 }]
},
methods: {
addArray () {
if (!this.items[this.items.length-1].add_on)
this.$set(this.items[this.items.length - 1], 'add_on', )
this.items[this.items.length - 1].add_on.push(1)
}
}
})
<script src="https://unpkg.com/vue"></script>
<div id="app">
<button v-on:click="addArray">
Add 1 to add_on create if not exists
</button>
<div v-for="item in items">add_on content: {{ item.add_on }}</div>
</div>
new Vue({
el: '#app',
data: {
items: [{ id: 0 }]
},
methods: {
addArray () {
if (!this.items[this.items.length-1].add_on)
this.$set(this.items[this.items.length - 1], 'add_on', )
this.items[this.items.length - 1].add_on.push(1)
}
}
})
<script src="https://unpkg.com/vue"></script>
<div id="app">
<button v-on:click="addArray">
Add 1 to add_on create if not exists
</button>
<div v-for="item in items">add_on content: {{ item.add_on }}</div>
</div>
new Vue({
el: '#app',
data: {
items: [{ id: 0 }]
},
methods: {
addArray () {
if (!this.items[this.items.length-1].add_on)
this.$set(this.items[this.items.length - 1], 'add_on', )
this.items[this.items.length - 1].add_on.push(1)
}
}
})
<script src="https://unpkg.com/vue"></script>
<div id="app">
<button v-on:click="addArray">
Add 1 to add_on create if not exists
</button>
<div v-for="item in items">add_on content: {{ item.add_on }}</div>
</div>
edited Nov 23 '18 at 5:19
answered Nov 23 '18 at 5:09


PsidomPsidom
124k1285127
124k1285127
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440846%2fvuex-add-new-array-property-to-an-object-and-push-elements-to-that-object-inside%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
u6oJQhv8iuOjlbPemXTjJ0SLo,KQSp3XwsfsHexmldu5PTLbsmrB5YJH3arydtVVcf6I1B4,DBUioVvL160CPB,VNm j1