How to find specific character to create array from string using php
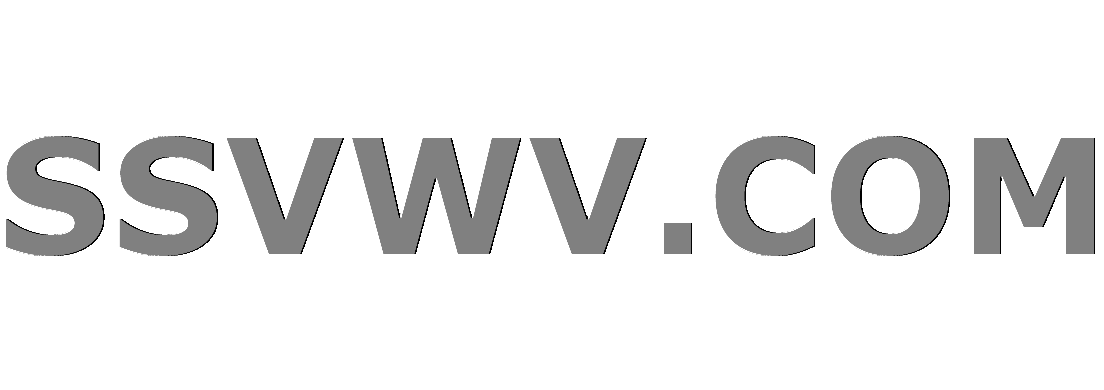
Multi tool use
I have some string like below:
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM',
I tried to find some character and to create array like
Expected Result:
Array
(
[0] => Array
(
[power] => 9.6W 24V
[temp] => 2.7
[degrees] => IP20
)
[1] => Array
(
[power] => 9.6W 24V
[temp] => 3
[degrees] => IP20
)
[2] => Array
(
[power] => HO 12.3 W 24V
[temp] => 3
[degrees] => IP65
)
)
but I cannot arrive right solution.
Explain: STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM
Here, W & V means power, K means Temp, IP means Degree
My tried Code:
<?php
$descriptions = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM',
];
$desc = ;
foreach($descriptions as $key=>$val){
$desc[$key]['power'] = strstr($val, 'V', true);
$desc[$key]['temp'] = strstr($val, 'K', true);
$desc[$key]['degrees'] = strstr($val, 'IP', true);
}
echo "<pre>";
print_r($desc).exit;
?>
I am seeing output like this:
Array
(
[0] => Array
(
[power] => STRIPLED 9,6W 24
[temp] => STRIPLED 9,6W 24V 2,7
[degrees] => STR
)
[1] => Array
(
[power] => STRIPLED 9,6W 24
[temp] => STRIPLED 9,6W 24V 3
[degrees] => STR
)
[2] => Array
(
[power] => STRIPLED H 6 W 24
[temp] => STRIPLED H 6 W 24V 4
[degrees] => STR
)
[3] => Array
(
[power] => STRIPLED HO 12,3 W 24
[temp] => STRIPLED HO 12,3 W 24V 3
[degrees] => STR
)
[4] => Array
(
[power] => STRIPLED HO 20W 24
[temp] => STRIPLED HO 20W 24V 2,7
[degrees] => STR
)
[5] => Array
(
[power] => STRIPLED 14,4W 24
[temp] => STRIPLED 14,4W 24V 2,7
[degrees] => STR
)
)
Can anybody give me a solution to create my expected result.
Thanks
php arrays php-7.2
add a comment |
I have some string like below:
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM',
I tried to find some character and to create array like
Expected Result:
Array
(
[0] => Array
(
[power] => 9.6W 24V
[temp] => 2.7
[degrees] => IP20
)
[1] => Array
(
[power] => 9.6W 24V
[temp] => 3
[degrees] => IP20
)
[2] => Array
(
[power] => HO 12.3 W 24V
[temp] => 3
[degrees] => IP65
)
)
but I cannot arrive right solution.
Explain: STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM
Here, W & V means power, K means Temp, IP means Degree
My tried Code:
<?php
$descriptions = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM',
];
$desc = ;
foreach($descriptions as $key=>$val){
$desc[$key]['power'] = strstr($val, 'V', true);
$desc[$key]['temp'] = strstr($val, 'K', true);
$desc[$key]['degrees'] = strstr($val, 'IP', true);
}
echo "<pre>";
print_r($desc).exit;
?>
I am seeing output like this:
Array
(
[0] => Array
(
[power] => STRIPLED 9,6W 24
[temp] => STRIPLED 9,6W 24V 2,7
[degrees] => STR
)
[1] => Array
(
[power] => STRIPLED 9,6W 24
[temp] => STRIPLED 9,6W 24V 3
[degrees] => STR
)
[2] => Array
(
[power] => STRIPLED H 6 W 24
[temp] => STRIPLED H 6 W 24V 4
[degrees] => STR
)
[3] => Array
(
[power] => STRIPLED HO 12,3 W 24
[temp] => STRIPLED HO 12,3 W 24V 3
[degrees] => STR
)
[4] => Array
(
[power] => STRIPLED HO 20W 24
[temp] => STRIPLED HO 20W 24V 2,7
[degrees] => STR
)
[5] => Array
(
[power] => STRIPLED 14,4W 24
[temp] => STRIPLED 14,4W 24V 2,7
[degrees] => STR
)
)
Can anybody give me a solution to create my expected result.
Thanks
php arrays php-7.2
you could simply do$desc[$key]['power'] = str_replace(',', '.', strstr($val, 'V', true));
this should do the trick
– user2749200
Nov 23 '18 at 5:15
add a comment |
I have some string like below:
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM',
I tried to find some character and to create array like
Expected Result:
Array
(
[0] => Array
(
[power] => 9.6W 24V
[temp] => 2.7
[degrees] => IP20
)
[1] => Array
(
[power] => 9.6W 24V
[temp] => 3
[degrees] => IP20
)
[2] => Array
(
[power] => HO 12.3 W 24V
[temp] => 3
[degrees] => IP65
)
)
but I cannot arrive right solution.
Explain: STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM
Here, W & V means power, K means Temp, IP means Degree
My tried Code:
<?php
$descriptions = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM',
];
$desc = ;
foreach($descriptions as $key=>$val){
$desc[$key]['power'] = strstr($val, 'V', true);
$desc[$key]['temp'] = strstr($val, 'K', true);
$desc[$key]['degrees'] = strstr($val, 'IP', true);
}
echo "<pre>";
print_r($desc).exit;
?>
I am seeing output like this:
Array
(
[0] => Array
(
[power] => STRIPLED 9,6W 24
[temp] => STRIPLED 9,6W 24V 2,7
[degrees] => STR
)
[1] => Array
(
[power] => STRIPLED 9,6W 24
[temp] => STRIPLED 9,6W 24V 3
[degrees] => STR
)
[2] => Array
(
[power] => STRIPLED H 6 W 24
[temp] => STRIPLED H 6 W 24V 4
[degrees] => STR
)
[3] => Array
(
[power] => STRIPLED HO 12,3 W 24
[temp] => STRIPLED HO 12,3 W 24V 3
[degrees] => STR
)
[4] => Array
(
[power] => STRIPLED HO 20W 24
[temp] => STRIPLED HO 20W 24V 2,7
[degrees] => STR
)
[5] => Array
(
[power] => STRIPLED 14,4W 24
[temp] => STRIPLED 14,4W 24V 2,7
[degrees] => STR
)
)
Can anybody give me a solution to create my expected result.
Thanks
php arrays php-7.2
I have some string like below:
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM',
I tried to find some character and to create array like
Expected Result:
Array
(
[0] => Array
(
[power] => 9.6W 24V
[temp] => 2.7
[degrees] => IP20
)
[1] => Array
(
[power] => 9.6W 24V
[temp] => 3
[degrees] => IP20
)
[2] => Array
(
[power] => HO 12.3 W 24V
[temp] => 3
[degrees] => IP65
)
)
but I cannot arrive right solution.
Explain: STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM
Here, W & V means power, K means Temp, IP means Degree
My tried Code:
<?php
$descriptions = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM',
];
$desc = ;
foreach($descriptions as $key=>$val){
$desc[$key]['power'] = strstr($val, 'V', true);
$desc[$key]['temp'] = strstr($val, 'K', true);
$desc[$key]['degrees'] = strstr($val, 'IP', true);
}
echo "<pre>";
print_r($desc).exit;
?>
I am seeing output like this:
Array
(
[0] => Array
(
[power] => STRIPLED 9,6W 24
[temp] => STRIPLED 9,6W 24V 2,7
[degrees] => STR
)
[1] => Array
(
[power] => STRIPLED 9,6W 24
[temp] => STRIPLED 9,6W 24V 3
[degrees] => STR
)
[2] => Array
(
[power] => STRIPLED H 6 W 24
[temp] => STRIPLED H 6 W 24V 4
[degrees] => STR
)
[3] => Array
(
[power] => STRIPLED HO 12,3 W 24
[temp] => STRIPLED HO 12,3 W 24V 3
[degrees] => STR
)
[4] => Array
(
[power] => STRIPLED HO 20W 24
[temp] => STRIPLED HO 20W 24V 2,7
[degrees] => STR
)
[5] => Array
(
[power] => STRIPLED 14,4W 24
[temp] => STRIPLED 14,4W 24V 2,7
[degrees] => STR
)
)
Can anybody give me a solution to create my expected result.
Thanks
php arrays php-7.2
php arrays php-7.2
edited Nov 23 '18 at 6:01
Nick
27.3k111941
27.3k111941
asked Nov 23 '18 at 4:53


Razib Al MamunRazib Al Mamun
2,25911020
2,25911020
you could simply do$desc[$key]['power'] = str_replace(',', '.', strstr($val, 'V', true));
this should do the trick
– user2749200
Nov 23 '18 at 5:15
add a comment |
you could simply do$desc[$key]['power'] = str_replace(',', '.', strstr($val, 'V', true));
this should do the trick
– user2749200
Nov 23 '18 at 5:15
you could simply do
$desc[$key]['power'] = str_replace(',', '.', strstr($val, 'V', true));
this should do the trick– user2749200
Nov 23 '18 at 5:15
you could simply do
$desc[$key]['power'] = str_replace(',', '.', strstr($val, 'V', true));
this should do the trick– user2749200
Nov 23 '18 at 5:15
add a comment |
2 Answers
2
active
oldest
votes
I think using preg_match
is probably the easiest way to extract the data you want. Based on your sample data, this regex should work:
^w+s+([^V]+V)s+([d.,]+)K.*?(IPd+)
This looks for a word, followed by some number of non V
characters (the "power" component), then a number before a K
(the "temperature" component), and finally a string matching IP
followed by some number of digits (the "degree" component). You could use this code (using array_map
) to process the array):
$descriptions = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM',
];
$desc = array_map(function ($v) {
preg_match('/^w+s+([^V]+V)s+([d.,]+)K.*?(IPd+)/', $v, $m);
array_shift($m);
return $m; }, $descriptions);
print_r($desc);
Output for your sample data:
Array
(
[0] => Array
(
[0] => 9.6W 24V
[1] => 2.7
[2] => IP20
)
[1] => Array
(
[0] => 9.6W 24V
[1] => 3
[2] => IP20
)
[2] => Array
(
[0] => H 6 W 24V
[1] => 4
[2] => IP20
)
[3] => Array
(
[0] => HO 12.3 W 24V
[1] => 3
[2] => IP65
)
[4] => Array
(
[0] => HO 20W 24V
[1] => 2.7
[2] => IP65
)
[5] => Array
(
[0] => 14.4W 24V
[1] => 2.7
[2] => IP65
)
)
Demo on 3v4l.org
Wow, excellent, speechless. It's working perfectly my side.
– Razib Al Mamun
Nov 23 '18 at 5:48
1
Cool. Glad I could help.
– Nick
Nov 23 '18 at 5:49
1
Excellent Answer
– Tamil Selvan C
Nov 23 '18 at 6:01
add a comment |
You can also try this:
<?php
$arrays = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM'
];
foreach ($arrays as $array){
//remove STRIPLED
$result = ;
$removedStripled = str_replace('STRIPLED ', "", $array);
$arr = strtok($removedStripled, 'V');
$result['power'] = $arr.'V';
$temp = str_replace($arr.'V', "", $removedStripled);
$arr = strtok($temp, 'K');
$result['temp'] = $arr;
preg_match("/IP.*[0-9s]/", $removedStripled, $match);
$degrees = explode(' ', $match[0]);
$result['degress'] = $degrees[0];
echo "<pre>";
print_r($result);
echo "</pre>";
}
Thanks for your good answer.
– Razib Al Mamun
Nov 23 '18 at 10:25
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440819%2fhow-to-find-specific-character-to-create-array-from-string-using-php%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think using preg_match
is probably the easiest way to extract the data you want. Based on your sample data, this regex should work:
^w+s+([^V]+V)s+([d.,]+)K.*?(IPd+)
This looks for a word, followed by some number of non V
characters (the "power" component), then a number before a K
(the "temperature" component), and finally a string matching IP
followed by some number of digits (the "degree" component). You could use this code (using array_map
) to process the array):
$descriptions = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM',
];
$desc = array_map(function ($v) {
preg_match('/^w+s+([^V]+V)s+([d.,]+)K.*?(IPd+)/', $v, $m);
array_shift($m);
return $m; }, $descriptions);
print_r($desc);
Output for your sample data:
Array
(
[0] => Array
(
[0] => 9.6W 24V
[1] => 2.7
[2] => IP20
)
[1] => Array
(
[0] => 9.6W 24V
[1] => 3
[2] => IP20
)
[2] => Array
(
[0] => H 6 W 24V
[1] => 4
[2] => IP20
)
[3] => Array
(
[0] => HO 12.3 W 24V
[1] => 3
[2] => IP65
)
[4] => Array
(
[0] => HO 20W 24V
[1] => 2.7
[2] => IP65
)
[5] => Array
(
[0] => 14.4W 24V
[1] => 2.7
[2] => IP65
)
)
Demo on 3v4l.org
Wow, excellent, speechless. It's working perfectly my side.
– Razib Al Mamun
Nov 23 '18 at 5:48
1
Cool. Glad I could help.
– Nick
Nov 23 '18 at 5:49
1
Excellent Answer
– Tamil Selvan C
Nov 23 '18 at 6:01
add a comment |
I think using preg_match
is probably the easiest way to extract the data you want. Based on your sample data, this regex should work:
^w+s+([^V]+V)s+([d.,]+)K.*?(IPd+)
This looks for a word, followed by some number of non V
characters (the "power" component), then a number before a K
(the "temperature" component), and finally a string matching IP
followed by some number of digits (the "degree" component). You could use this code (using array_map
) to process the array):
$descriptions = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM',
];
$desc = array_map(function ($v) {
preg_match('/^w+s+([^V]+V)s+([d.,]+)K.*?(IPd+)/', $v, $m);
array_shift($m);
return $m; }, $descriptions);
print_r($desc);
Output for your sample data:
Array
(
[0] => Array
(
[0] => 9.6W 24V
[1] => 2.7
[2] => IP20
)
[1] => Array
(
[0] => 9.6W 24V
[1] => 3
[2] => IP20
)
[2] => Array
(
[0] => H 6 W 24V
[1] => 4
[2] => IP20
)
[3] => Array
(
[0] => HO 12.3 W 24V
[1] => 3
[2] => IP65
)
[4] => Array
(
[0] => HO 20W 24V
[1] => 2.7
[2] => IP65
)
[5] => Array
(
[0] => 14.4W 24V
[1] => 2.7
[2] => IP65
)
)
Demo on 3v4l.org
Wow, excellent, speechless. It's working perfectly my side.
– Razib Al Mamun
Nov 23 '18 at 5:48
1
Cool. Glad I could help.
– Nick
Nov 23 '18 at 5:49
1
Excellent Answer
– Tamil Selvan C
Nov 23 '18 at 6:01
add a comment |
I think using preg_match
is probably the easiest way to extract the data you want. Based on your sample data, this regex should work:
^w+s+([^V]+V)s+([d.,]+)K.*?(IPd+)
This looks for a word, followed by some number of non V
characters (the "power" component), then a number before a K
(the "temperature" component), and finally a string matching IP
followed by some number of digits (the "degree" component). You could use this code (using array_map
) to process the array):
$descriptions = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM',
];
$desc = array_map(function ($v) {
preg_match('/^w+s+([^V]+V)s+([d.,]+)K.*?(IPd+)/', $v, $m);
array_shift($m);
return $m; }, $descriptions);
print_r($desc);
Output for your sample data:
Array
(
[0] => Array
(
[0] => 9.6W 24V
[1] => 2.7
[2] => IP20
)
[1] => Array
(
[0] => 9.6W 24V
[1] => 3
[2] => IP20
)
[2] => Array
(
[0] => H 6 W 24V
[1] => 4
[2] => IP20
)
[3] => Array
(
[0] => HO 12.3 W 24V
[1] => 3
[2] => IP65
)
[4] => Array
(
[0] => HO 20W 24V
[1] => 2.7
[2] => IP65
)
[5] => Array
(
[0] => 14.4W 24V
[1] => 2.7
[2] => IP65
)
)
Demo on 3v4l.org
I think using preg_match
is probably the easiest way to extract the data you want. Based on your sample data, this regex should work:
^w+s+([^V]+V)s+([d.,]+)K.*?(IPd+)
This looks for a word, followed by some number of non V
characters (the "power" component), then a number before a K
(the "temperature" component), and finally a string matching IP
followed by some number of digits (the "degree" component). You could use this code (using array_map
) to process the array):
$descriptions = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM',
];
$desc = array_map(function ($v) {
preg_match('/^w+s+([^V]+V)s+([d.,]+)K.*?(IPd+)/', $v, $m);
array_shift($m);
return $m; }, $descriptions);
print_r($desc);
Output for your sample data:
Array
(
[0] => Array
(
[0] => 9.6W 24V
[1] => 2.7
[2] => IP20
)
[1] => Array
(
[0] => 9.6W 24V
[1] => 3
[2] => IP20
)
[2] => Array
(
[0] => H 6 W 24V
[1] => 4
[2] => IP20
)
[3] => Array
(
[0] => HO 12.3 W 24V
[1] => 3
[2] => IP65
)
[4] => Array
(
[0] => HO 20W 24V
[1] => 2.7
[2] => IP65
)
[5] => Array
(
[0] => 14.4W 24V
[1] => 2.7
[2] => IP65
)
)
Demo on 3v4l.org
edited Nov 23 '18 at 10:58
answered Nov 23 '18 at 5:30
NickNick
27.3k111941
27.3k111941
Wow, excellent, speechless. It's working perfectly my side.
– Razib Al Mamun
Nov 23 '18 at 5:48
1
Cool. Glad I could help.
– Nick
Nov 23 '18 at 5:49
1
Excellent Answer
– Tamil Selvan C
Nov 23 '18 at 6:01
add a comment |
Wow, excellent, speechless. It's working perfectly my side.
– Razib Al Mamun
Nov 23 '18 at 5:48
1
Cool. Glad I could help.
– Nick
Nov 23 '18 at 5:49
1
Excellent Answer
– Tamil Selvan C
Nov 23 '18 at 6:01
Wow, excellent, speechless. It's working perfectly my side.
– Razib Al Mamun
Nov 23 '18 at 5:48
Wow, excellent, speechless. It's working perfectly my side.
– Razib Al Mamun
Nov 23 '18 at 5:48
1
1
Cool. Glad I could help.
– Nick
Nov 23 '18 at 5:49
Cool. Glad I could help.
– Nick
Nov 23 '18 at 5:49
1
1
Excellent Answer
– Tamil Selvan C
Nov 23 '18 at 6:01
Excellent Answer
– Tamil Selvan C
Nov 23 '18 at 6:01
add a comment |
You can also try this:
<?php
$arrays = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM'
];
foreach ($arrays as $array){
//remove STRIPLED
$result = ;
$removedStripled = str_replace('STRIPLED ', "", $array);
$arr = strtok($removedStripled, 'V');
$result['power'] = $arr.'V';
$temp = str_replace($arr.'V', "", $removedStripled);
$arr = strtok($temp, 'K');
$result['temp'] = $arr;
preg_match("/IP.*[0-9s]/", $removedStripled, $match);
$degrees = explode(' ', $match[0]);
$result['degress'] = $degrees[0];
echo "<pre>";
print_r($result);
echo "</pre>";
}
Thanks for your good answer.
– Razib Al Mamun
Nov 23 '18 at 10:25
add a comment |
You can also try this:
<?php
$arrays = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM'
];
foreach ($arrays as $array){
//remove STRIPLED
$result = ;
$removedStripled = str_replace('STRIPLED ', "", $array);
$arr = strtok($removedStripled, 'V');
$result['power'] = $arr.'V';
$temp = str_replace($arr.'V', "", $removedStripled);
$arr = strtok($temp, 'K');
$result['temp'] = $arr;
preg_match("/IP.*[0-9s]/", $removedStripled, $match);
$degrees = explode(' ', $match[0]);
$result['degress'] = $degrees[0];
echo "<pre>";
print_r($result);
echo "</pre>";
}
Thanks for your good answer.
– Razib Al Mamun
Nov 23 '18 at 10:25
add a comment |
You can also try this:
<?php
$arrays = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM'
];
foreach ($arrays as $array){
//remove STRIPLED
$result = ;
$removedStripled = str_replace('STRIPLED ', "", $array);
$arr = strtok($removedStripled, 'V');
$result['power'] = $arr.'V';
$temp = str_replace($arr.'V', "", $removedStripled);
$arr = strtok($temp, 'K');
$result['temp'] = $arr;
preg_match("/IP.*[0-9s]/", $removedStripled, $match);
$degrees = explode(' ', $match[0]);
$result['degress'] = $degrees[0];
echo "<pre>";
print_r($result);
echo "</pre>";
}
You can also try this:
<?php
$arrays = [
'STRIPLED 9.6W 24V 2.7K 120LED IP20 1CM',
'STRIPLED 9.6W 24V 3K 120LED IP20 1CM',
'STRIPLED H 6 W 24V 4K 140 LED IP20 1CM',
'STRIPLED HO 12.3 W 24V 3K 128 LED IP65 1CM',
'STRIPLED HO 20W 24V 2.7K 280 LED IP65 1CM',
'STRIPLED 14.4W 24V 2.7K 180 LED IP65 1 CM'
];
foreach ($arrays as $array){
//remove STRIPLED
$result = ;
$removedStripled = str_replace('STRIPLED ', "", $array);
$arr = strtok($removedStripled, 'V');
$result['power'] = $arr.'V';
$temp = str_replace($arr.'V', "", $removedStripled);
$arr = strtok($temp, 'K');
$result['temp'] = $arr;
preg_match("/IP.*[0-9s]/", $removedStripled, $match);
$degrees = explode(' ', $match[0]);
$result['degress'] = $degrees[0];
echo "<pre>";
print_r($result);
echo "</pre>";
}
answered Nov 23 '18 at 10:20


Aourongajab AbirAourongajab Abir
111
111
Thanks for your good answer.
– Razib Al Mamun
Nov 23 '18 at 10:25
add a comment |
Thanks for your good answer.
– Razib Al Mamun
Nov 23 '18 at 10:25
Thanks for your good answer.
– Razib Al Mamun
Nov 23 '18 at 10:25
Thanks for your good answer.
– Razib Al Mamun
Nov 23 '18 at 10:25
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440819%2fhow-to-find-specific-character-to-create-array-from-string-using-php%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
CHiXoqKRO s1mTitupIIsT4LR8xyX4imfqlhF3YsmEI3,BR 0GXGGW143MiGzR3,5G,J,Egvo6 Cd
you could simply do
$desc[$key]['power'] = str_replace(',', '.', strstr($val, 'V', true));
this should do the trick– user2749200
Nov 23 '18 at 5:15