phpmailer form validation in a single page is not working properly. How to display error messages in the same...
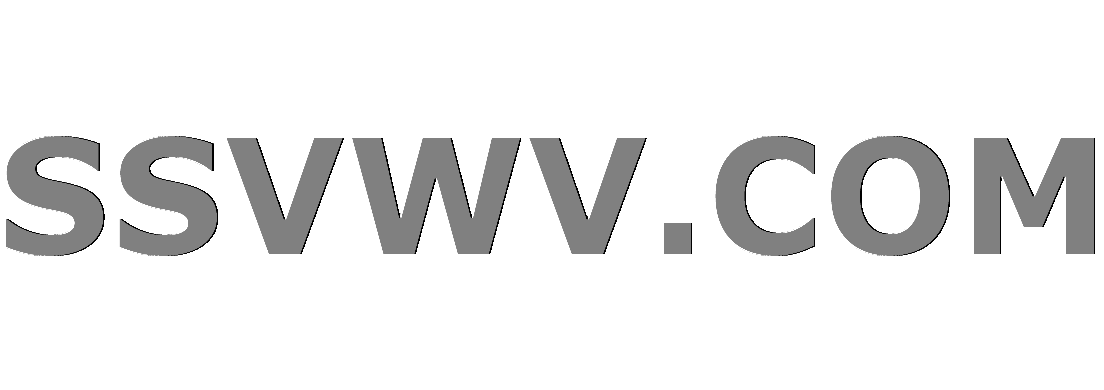
Multi tool use
I'm trying to display error messages. If I use exit()
function instead of writing all the errors in a message variable then the code works fine but still the error messages are not being displayed on the same page, it is taking me to another page.
I want errors to be displayed on the same page whenever submit button is clicked.
This the index.php page
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Reset Password</title>
</head>
<body>
<a href="request-reset.php">Forgot password?</a>
</body>
</html>
This is the request-reset.php page
<?php
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require 'PHPMailer/src/Exception.php';
require 'PHPMailer/src/PHPMailer.php';
require 'PHPMailer/src/SMTP.php';
require 'db.php';
if(isset($_POST['email']) && $_POST['email'] != "") {
$emailTo = $_POST['email'];
if(!filter_var($emailTo, FILTER_VALIDATE_EMAIL)) {
echo "ERROR: Email is invalid";
exit();
}
$code = uniqid(true);
$emailCheck = mysqli_query($connection, "SELECT email FROM users WHERE email = '$emailTo'");
if(mysqli_num_rows($emailCheck) > 0) {
$query = mysqli_query($connection, "INSERT INTO reset_passwords(code, email) VALUES('$code', '$emailTo')");
if(!$query) {
exit("Error");
}
}
else {
exit("Email does not exist in our database!");
}
$mail = new PHPMailer(true); // Passing `true` enables exceptions
try {
//Server settings
$mail->isSMTP(); // Set mailer to use SMTP
$mail->Host = 'smtp.gmail.com'; // Specify main and backup SMTP servers
$mail->SMTPAuth = true; // Enable SMTP authentication
$mail->Username = 'ramanath781@gmail.com'; // SMTP username
$mail->Password = '11111111111'; // SMTP password
$mail->SMTPSecure = 'tls'; // Enable TLS encryption, `ssl` also accepted
$mail->Port = 587; // TCP port to connect to
//Recipients
$mail->setFrom('ramanath784@gmail.com', 'Music Online');
$mail->addAddress($emailTo); // Add a recipient
$mail->addReplyTo('no-reply@ramanath.com', 'No Reply');
//Content
$url = "https://" . $_SERVER["HTTP_HOST"] . dirname($_SERVER["PHP_SELF"]) . "/reset-password.php?code=$code";
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = 'Request For Password Change';
$mail->Body = "<h1>You requested a password reset</h1>
Click <a href='$url'>this link</a> to change the password";
$mail->AltBody = 'This is the body in plain text for non-HTML mail clients';
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) {
echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo;
}
exit();
}
?>
<?php
include("header.php");
include("forgot-password-form.php");
include("footer.php");
?>
This is the reset-password.php page
<?php
include("db.php");
if(!isset($_GET['code'])) {
exit("Can't find page!");
}
$message = null;
$code = $_GET['code'];
$getEmailQuery = mysqli_query($connection, "SELECT email FROM reset_passwords WHERE code = '$code'");
if(mysqli_num_rows($getEmailQuery) == 0) {
exit("<h1>Can't find page!</h1>");
}
if(isset($_POST['submit'])) {
$newPassword1 = mysqli_real_escape_string($connection, $_POST['newPassword1']);
$newPassword2 = mysqli_real_escape_string($connection, $_POST['newPassword2']);
if(!isset($_POST['newPassword1']) || !isset($_POST['newPassword2'])) {
$message = "ERROR: Not all passwords have been set";
}
if($_POST['newPassword1'] == "" || $_POST['newPassword2'] == "") {
$message = "ERROR: Please fill in all fields";
}
$newPassword1 = $_POST['newPassword1'];
$newPassword2 = $_POST['newPassword2'];
if($newPassword1 != $newPassword2) {
$message = "ERROR: Your new passwords do not match";
}
if(preg_match("/[^A-Za-z0-9]/", $newPassword1)) {
$message = "ERROR: Your password must only contain alphanumeric characters";
}
if(strlen($newPassword1) > 20 || strlen($newPassword1) < 8) {
$message = "ERROR: Your password must be between 8 and 20 characters";
}
$pw = $_POST['newPassword1'];
$pw = md5($pw);
$row = mysqli_fetch_array($getEmailQuery);
$email = $row['email'];
$query = mysqli_query($connection, "UPDATE users SET password = '$pw' WHERE email = '$email'");
if($query) {
$query = mysqli_query($connection, "DELETE FROM reset_passwords WHERE code = '$code'");
$message = "Password updated";
}
else {
$message = "Something went wrong!";
}
}
?>
<?php
include("header.php");
?>
<form id="register-form" role="form" autocomplete="off" class="form" method="post">
<div class="form-group">
<div class="input-group">
<span class="input-group-addon"><i class="glyphicon glyphicon-lock"></i></span>
<input id="password" name="newPassword1" placeholder="New password" class="form-control" type="password">
</div>
<span><?php echo $message; ?></span>
</div>
<div class="form-group">
<div class="input-group">
<span class="input-group-addon"><i class="glyphicon glyphicon-lock"></i></span>
<input id="password" name="newPassword2" placeholder="Confirm password" class="form-control" type="password">
</div>
<span><?php echo $message; ?></span>
</div>
<div class="form-group">
<input name="submit" class="btn btn-lg btn-primary btn-block" value="Reset Password" type="submit">
</div>
<input type="hidden" class="hide" name="token" id="token" value="">
</form>
<?php
include("footer.php");
?>
php phpmyadmin
add a comment |
I'm trying to display error messages. If I use exit()
function instead of writing all the errors in a message variable then the code works fine but still the error messages are not being displayed on the same page, it is taking me to another page.
I want errors to be displayed on the same page whenever submit button is clicked.
This the index.php page
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Reset Password</title>
</head>
<body>
<a href="request-reset.php">Forgot password?</a>
</body>
</html>
This is the request-reset.php page
<?php
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require 'PHPMailer/src/Exception.php';
require 'PHPMailer/src/PHPMailer.php';
require 'PHPMailer/src/SMTP.php';
require 'db.php';
if(isset($_POST['email']) && $_POST['email'] != "") {
$emailTo = $_POST['email'];
if(!filter_var($emailTo, FILTER_VALIDATE_EMAIL)) {
echo "ERROR: Email is invalid";
exit();
}
$code = uniqid(true);
$emailCheck = mysqli_query($connection, "SELECT email FROM users WHERE email = '$emailTo'");
if(mysqli_num_rows($emailCheck) > 0) {
$query = mysqli_query($connection, "INSERT INTO reset_passwords(code, email) VALUES('$code', '$emailTo')");
if(!$query) {
exit("Error");
}
}
else {
exit("Email does not exist in our database!");
}
$mail = new PHPMailer(true); // Passing `true` enables exceptions
try {
//Server settings
$mail->isSMTP(); // Set mailer to use SMTP
$mail->Host = 'smtp.gmail.com'; // Specify main and backup SMTP servers
$mail->SMTPAuth = true; // Enable SMTP authentication
$mail->Username = 'ramanath781@gmail.com'; // SMTP username
$mail->Password = '11111111111'; // SMTP password
$mail->SMTPSecure = 'tls'; // Enable TLS encryption, `ssl` also accepted
$mail->Port = 587; // TCP port to connect to
//Recipients
$mail->setFrom('ramanath784@gmail.com', 'Music Online');
$mail->addAddress($emailTo); // Add a recipient
$mail->addReplyTo('no-reply@ramanath.com', 'No Reply');
//Content
$url = "https://" . $_SERVER["HTTP_HOST"] . dirname($_SERVER["PHP_SELF"]) . "/reset-password.php?code=$code";
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = 'Request For Password Change';
$mail->Body = "<h1>You requested a password reset</h1>
Click <a href='$url'>this link</a> to change the password";
$mail->AltBody = 'This is the body in plain text for non-HTML mail clients';
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) {
echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo;
}
exit();
}
?>
<?php
include("header.php");
include("forgot-password-form.php");
include("footer.php");
?>
This is the reset-password.php page
<?php
include("db.php");
if(!isset($_GET['code'])) {
exit("Can't find page!");
}
$message = null;
$code = $_GET['code'];
$getEmailQuery = mysqli_query($connection, "SELECT email FROM reset_passwords WHERE code = '$code'");
if(mysqli_num_rows($getEmailQuery) == 0) {
exit("<h1>Can't find page!</h1>");
}
if(isset($_POST['submit'])) {
$newPassword1 = mysqli_real_escape_string($connection, $_POST['newPassword1']);
$newPassword2 = mysqli_real_escape_string($connection, $_POST['newPassword2']);
if(!isset($_POST['newPassword1']) || !isset($_POST['newPassword2'])) {
$message = "ERROR: Not all passwords have been set";
}
if($_POST['newPassword1'] == "" || $_POST['newPassword2'] == "") {
$message = "ERROR: Please fill in all fields";
}
$newPassword1 = $_POST['newPassword1'];
$newPassword2 = $_POST['newPassword2'];
if($newPassword1 != $newPassword2) {
$message = "ERROR: Your new passwords do not match";
}
if(preg_match("/[^A-Za-z0-9]/", $newPassword1)) {
$message = "ERROR: Your password must only contain alphanumeric characters";
}
if(strlen($newPassword1) > 20 || strlen($newPassword1) < 8) {
$message = "ERROR: Your password must be between 8 and 20 characters";
}
$pw = $_POST['newPassword1'];
$pw = md5($pw);
$row = mysqli_fetch_array($getEmailQuery);
$email = $row['email'];
$query = mysqli_query($connection, "UPDATE users SET password = '$pw' WHERE email = '$email'");
if($query) {
$query = mysqli_query($connection, "DELETE FROM reset_passwords WHERE code = '$code'");
$message = "Password updated";
}
else {
$message = "Something went wrong!";
}
}
?>
<?php
include("header.php");
?>
<form id="register-form" role="form" autocomplete="off" class="form" method="post">
<div class="form-group">
<div class="input-group">
<span class="input-group-addon"><i class="glyphicon glyphicon-lock"></i></span>
<input id="password" name="newPassword1" placeholder="New password" class="form-control" type="password">
</div>
<span><?php echo $message; ?></span>
</div>
<div class="form-group">
<div class="input-group">
<span class="input-group-addon"><i class="glyphicon glyphicon-lock"></i></span>
<input id="password" name="newPassword2" placeholder="Confirm password" class="form-control" type="password">
</div>
<span><?php echo $message; ?></span>
</div>
<div class="form-group">
<input name="submit" class="btn btn-lg btn-primary btn-block" value="Reset Password" type="submit">
</div>
<input type="hidden" class="hide" name="token" id="token" value="">
</form>
<?php
include("footer.php");
?>
php phpmyadmin
There is no code given that might trigger a redirect - is this the whole code? Additionally, what have you tried to spot the error on your own?
– Nico Haase
Nov 21 '18 at 19:56
Nope that was not the whole code ..I have shared all the whole code. can you solve this problem now?
– Ramanath
Nov 21 '18 at 20:17
Sorry, but that is a lot of code. What have you tried to find the error?
– Nico Haase
Nov 21 '18 at 20:26
add a comment |
I'm trying to display error messages. If I use exit()
function instead of writing all the errors in a message variable then the code works fine but still the error messages are not being displayed on the same page, it is taking me to another page.
I want errors to be displayed on the same page whenever submit button is clicked.
This the index.php page
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Reset Password</title>
</head>
<body>
<a href="request-reset.php">Forgot password?</a>
</body>
</html>
This is the request-reset.php page
<?php
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require 'PHPMailer/src/Exception.php';
require 'PHPMailer/src/PHPMailer.php';
require 'PHPMailer/src/SMTP.php';
require 'db.php';
if(isset($_POST['email']) && $_POST['email'] != "") {
$emailTo = $_POST['email'];
if(!filter_var($emailTo, FILTER_VALIDATE_EMAIL)) {
echo "ERROR: Email is invalid";
exit();
}
$code = uniqid(true);
$emailCheck = mysqli_query($connection, "SELECT email FROM users WHERE email = '$emailTo'");
if(mysqli_num_rows($emailCheck) > 0) {
$query = mysqli_query($connection, "INSERT INTO reset_passwords(code, email) VALUES('$code', '$emailTo')");
if(!$query) {
exit("Error");
}
}
else {
exit("Email does not exist in our database!");
}
$mail = new PHPMailer(true); // Passing `true` enables exceptions
try {
//Server settings
$mail->isSMTP(); // Set mailer to use SMTP
$mail->Host = 'smtp.gmail.com'; // Specify main and backup SMTP servers
$mail->SMTPAuth = true; // Enable SMTP authentication
$mail->Username = 'ramanath781@gmail.com'; // SMTP username
$mail->Password = '11111111111'; // SMTP password
$mail->SMTPSecure = 'tls'; // Enable TLS encryption, `ssl` also accepted
$mail->Port = 587; // TCP port to connect to
//Recipients
$mail->setFrom('ramanath784@gmail.com', 'Music Online');
$mail->addAddress($emailTo); // Add a recipient
$mail->addReplyTo('no-reply@ramanath.com', 'No Reply');
//Content
$url = "https://" . $_SERVER["HTTP_HOST"] . dirname($_SERVER["PHP_SELF"]) . "/reset-password.php?code=$code";
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = 'Request For Password Change';
$mail->Body = "<h1>You requested a password reset</h1>
Click <a href='$url'>this link</a> to change the password";
$mail->AltBody = 'This is the body in plain text for non-HTML mail clients';
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) {
echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo;
}
exit();
}
?>
<?php
include("header.php");
include("forgot-password-form.php");
include("footer.php");
?>
This is the reset-password.php page
<?php
include("db.php");
if(!isset($_GET['code'])) {
exit("Can't find page!");
}
$message = null;
$code = $_GET['code'];
$getEmailQuery = mysqli_query($connection, "SELECT email FROM reset_passwords WHERE code = '$code'");
if(mysqli_num_rows($getEmailQuery) == 0) {
exit("<h1>Can't find page!</h1>");
}
if(isset($_POST['submit'])) {
$newPassword1 = mysqli_real_escape_string($connection, $_POST['newPassword1']);
$newPassword2 = mysqli_real_escape_string($connection, $_POST['newPassword2']);
if(!isset($_POST['newPassword1']) || !isset($_POST['newPassword2'])) {
$message = "ERROR: Not all passwords have been set";
}
if($_POST['newPassword1'] == "" || $_POST['newPassword2'] == "") {
$message = "ERROR: Please fill in all fields";
}
$newPassword1 = $_POST['newPassword1'];
$newPassword2 = $_POST['newPassword2'];
if($newPassword1 != $newPassword2) {
$message = "ERROR: Your new passwords do not match";
}
if(preg_match("/[^A-Za-z0-9]/", $newPassword1)) {
$message = "ERROR: Your password must only contain alphanumeric characters";
}
if(strlen($newPassword1) > 20 || strlen($newPassword1) < 8) {
$message = "ERROR: Your password must be between 8 and 20 characters";
}
$pw = $_POST['newPassword1'];
$pw = md5($pw);
$row = mysqli_fetch_array($getEmailQuery);
$email = $row['email'];
$query = mysqli_query($connection, "UPDATE users SET password = '$pw' WHERE email = '$email'");
if($query) {
$query = mysqli_query($connection, "DELETE FROM reset_passwords WHERE code = '$code'");
$message = "Password updated";
}
else {
$message = "Something went wrong!";
}
}
?>
<?php
include("header.php");
?>
<form id="register-form" role="form" autocomplete="off" class="form" method="post">
<div class="form-group">
<div class="input-group">
<span class="input-group-addon"><i class="glyphicon glyphicon-lock"></i></span>
<input id="password" name="newPassword1" placeholder="New password" class="form-control" type="password">
</div>
<span><?php echo $message; ?></span>
</div>
<div class="form-group">
<div class="input-group">
<span class="input-group-addon"><i class="glyphicon glyphicon-lock"></i></span>
<input id="password" name="newPassword2" placeholder="Confirm password" class="form-control" type="password">
</div>
<span><?php echo $message; ?></span>
</div>
<div class="form-group">
<input name="submit" class="btn btn-lg btn-primary btn-block" value="Reset Password" type="submit">
</div>
<input type="hidden" class="hide" name="token" id="token" value="">
</form>
<?php
include("footer.php");
?>
php phpmyadmin
I'm trying to display error messages. If I use exit()
function instead of writing all the errors in a message variable then the code works fine but still the error messages are not being displayed on the same page, it is taking me to another page.
I want errors to be displayed on the same page whenever submit button is clicked.
This the index.php page
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Reset Password</title>
</head>
<body>
<a href="request-reset.php">Forgot password?</a>
</body>
</html>
This is the request-reset.php page
<?php
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require 'PHPMailer/src/Exception.php';
require 'PHPMailer/src/PHPMailer.php';
require 'PHPMailer/src/SMTP.php';
require 'db.php';
if(isset($_POST['email']) && $_POST['email'] != "") {
$emailTo = $_POST['email'];
if(!filter_var($emailTo, FILTER_VALIDATE_EMAIL)) {
echo "ERROR: Email is invalid";
exit();
}
$code = uniqid(true);
$emailCheck = mysqli_query($connection, "SELECT email FROM users WHERE email = '$emailTo'");
if(mysqli_num_rows($emailCheck) > 0) {
$query = mysqli_query($connection, "INSERT INTO reset_passwords(code, email) VALUES('$code', '$emailTo')");
if(!$query) {
exit("Error");
}
}
else {
exit("Email does not exist in our database!");
}
$mail = new PHPMailer(true); // Passing `true` enables exceptions
try {
//Server settings
$mail->isSMTP(); // Set mailer to use SMTP
$mail->Host = 'smtp.gmail.com'; // Specify main and backup SMTP servers
$mail->SMTPAuth = true; // Enable SMTP authentication
$mail->Username = 'ramanath781@gmail.com'; // SMTP username
$mail->Password = '11111111111'; // SMTP password
$mail->SMTPSecure = 'tls'; // Enable TLS encryption, `ssl` also accepted
$mail->Port = 587; // TCP port to connect to
//Recipients
$mail->setFrom('ramanath784@gmail.com', 'Music Online');
$mail->addAddress($emailTo); // Add a recipient
$mail->addReplyTo('no-reply@ramanath.com', 'No Reply');
//Content
$url = "https://" . $_SERVER["HTTP_HOST"] . dirname($_SERVER["PHP_SELF"]) . "/reset-password.php?code=$code";
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = 'Request For Password Change';
$mail->Body = "<h1>You requested a password reset</h1>
Click <a href='$url'>this link</a> to change the password";
$mail->AltBody = 'This is the body in plain text for non-HTML mail clients';
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) {
echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo;
}
exit();
}
?>
<?php
include("header.php");
include("forgot-password-form.php");
include("footer.php");
?>
This is the reset-password.php page
<?php
include("db.php");
if(!isset($_GET['code'])) {
exit("Can't find page!");
}
$message = null;
$code = $_GET['code'];
$getEmailQuery = mysqli_query($connection, "SELECT email FROM reset_passwords WHERE code = '$code'");
if(mysqli_num_rows($getEmailQuery) == 0) {
exit("<h1>Can't find page!</h1>");
}
if(isset($_POST['submit'])) {
$newPassword1 = mysqli_real_escape_string($connection, $_POST['newPassword1']);
$newPassword2 = mysqli_real_escape_string($connection, $_POST['newPassword2']);
if(!isset($_POST['newPassword1']) || !isset($_POST['newPassword2'])) {
$message = "ERROR: Not all passwords have been set";
}
if($_POST['newPassword1'] == "" || $_POST['newPassword2'] == "") {
$message = "ERROR: Please fill in all fields";
}
$newPassword1 = $_POST['newPassword1'];
$newPassword2 = $_POST['newPassword2'];
if($newPassword1 != $newPassword2) {
$message = "ERROR: Your new passwords do not match";
}
if(preg_match("/[^A-Za-z0-9]/", $newPassword1)) {
$message = "ERROR: Your password must only contain alphanumeric characters";
}
if(strlen($newPassword1) > 20 || strlen($newPassword1) < 8) {
$message = "ERROR: Your password must be between 8 and 20 characters";
}
$pw = $_POST['newPassword1'];
$pw = md5($pw);
$row = mysqli_fetch_array($getEmailQuery);
$email = $row['email'];
$query = mysqli_query($connection, "UPDATE users SET password = '$pw' WHERE email = '$email'");
if($query) {
$query = mysqli_query($connection, "DELETE FROM reset_passwords WHERE code = '$code'");
$message = "Password updated";
}
else {
$message = "Something went wrong!";
}
}
?>
<?php
include("header.php");
?>
<form id="register-form" role="form" autocomplete="off" class="form" method="post">
<div class="form-group">
<div class="input-group">
<span class="input-group-addon"><i class="glyphicon glyphicon-lock"></i></span>
<input id="password" name="newPassword1" placeholder="New password" class="form-control" type="password">
</div>
<span><?php echo $message; ?></span>
</div>
<div class="form-group">
<div class="input-group">
<span class="input-group-addon"><i class="glyphicon glyphicon-lock"></i></span>
<input id="password" name="newPassword2" placeholder="Confirm password" class="form-control" type="password">
</div>
<span><?php echo $message; ?></span>
</div>
<div class="form-group">
<input name="submit" class="btn btn-lg btn-primary btn-block" value="Reset Password" type="submit">
</div>
<input type="hidden" class="hide" name="token" id="token" value="">
</form>
<?php
include("footer.php");
?>
php phpmyadmin
php phpmyadmin
edited Nov 23 '18 at 20:19
user6910411
32.8k96995
32.8k96995
asked Nov 21 '18 at 19:37
RamanathRamanath
11
11
There is no code given that might trigger a redirect - is this the whole code? Additionally, what have you tried to spot the error on your own?
– Nico Haase
Nov 21 '18 at 19:56
Nope that was not the whole code ..I have shared all the whole code. can you solve this problem now?
– Ramanath
Nov 21 '18 at 20:17
Sorry, but that is a lot of code. What have you tried to find the error?
– Nico Haase
Nov 21 '18 at 20:26
add a comment |
There is no code given that might trigger a redirect - is this the whole code? Additionally, what have you tried to spot the error on your own?
– Nico Haase
Nov 21 '18 at 19:56
Nope that was not the whole code ..I have shared all the whole code. can you solve this problem now?
– Ramanath
Nov 21 '18 at 20:17
Sorry, but that is a lot of code. What have you tried to find the error?
– Nico Haase
Nov 21 '18 at 20:26
There is no code given that might trigger a redirect - is this the whole code? Additionally, what have you tried to spot the error on your own?
– Nico Haase
Nov 21 '18 at 19:56
There is no code given that might trigger a redirect - is this the whole code? Additionally, what have you tried to spot the error on your own?
– Nico Haase
Nov 21 '18 at 19:56
Nope that was not the whole code ..I have shared all the whole code. can you solve this problem now?
– Ramanath
Nov 21 '18 at 20:17
Nope that was not the whole code ..I have shared all the whole code. can you solve this problem now?
– Ramanath
Nov 21 '18 at 20:17
Sorry, but that is a lot of code. What have you tried to find the error?
– Nico Haase
Nov 21 '18 at 20:26
Sorry, but that is a lot of code. What have you tried to find the error?
– Nico Haase
Nov 21 '18 at 20:26
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53419402%2fphpmailer-form-validation-in-a-single-page-is-not-working-properly-how-to-displ%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53419402%2fphpmailer-form-validation-in-a-single-page-is-not-working-properly-how-to-displ%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
GLtBZiL R2d8gZ6M,5JvWDrw xqhlvywCbkg0q,s 3iZ2F
There is no code given that might trigger a redirect - is this the whole code? Additionally, what have you tried to spot the error on your own?
– Nico Haase
Nov 21 '18 at 19:56
Nope that was not the whole code ..I have shared all the whole code. can you solve this problem now?
– Ramanath
Nov 21 '18 at 20:17
Sorry, but that is a lot of code. What have you tried to find the error?
– Nico Haase
Nov 21 '18 at 20:26