Can't cast MockUnit to String with mockneat
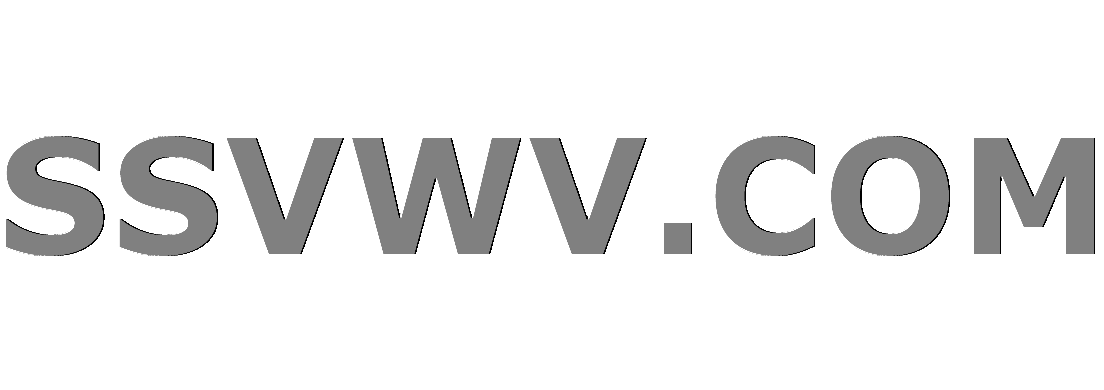
Multi tool use
Try to use my own Enum
like mock
with help of library mockneat
. But the problem is that I can't take string
value from MockUnit
object for my right working of switch loop.
It's my Enum
:
public enum SubType {
ROUTER("router"),
SERVER("server"),
private final String value;
SubType(final String newValue) {
value = newValue;
}
public String getValue() {
return value;
}
}
And it's my Build
class, where I try to use custom values from SubType
:
public class Build {
private MockNeat mockNeat = MockNeat.threadLocal();
private ArrayList<String> group = new ArrayList<>();
public void addToList() {
group.add("All Devices");
group.add("All Groups");
for (i = 0; i < 10; i++) {
MockUnit subType = mockNeat.from(SubType.class).map(SubType::getValue);
//EDITED:
//For example there are sout(subType) = "router"
Gson gson = new GsonBuilder().setPrettyPrinting().create();
String json = mockNeat.reflect(Build.class)
.field("group", constructGroupField(subType, group))
.map(gson::toJson)
.val();
System.out.println(json);
}
}
private ArrayList<String> constructGroupField(MockUnit subType, ArrayList<String> group) {
//System.out.println(subType);
//Output: net.andreinc.mockneat.abstraction.MockUnit$$Lambda$22/902830499@740773a3
//But how I can get there my string like "router" or ""server"
//How I can cast MockUnit to String value?
//EDITED:
// And there it can be already sout(subType)= "server"
switch (subType.toString()) {
case "router":
group.add("All AAA");
break;
case "server":
group.add("All BBB");
break;
}
return group;
}
}
The main goal is to, depending on which string be in the subtype
field, output the corresponding json
file
UPDATED:
The final Json
file, that I want to produce:
{
"product": "a",
"group": "router"
}
Another situation:
private String someMethod() {
MockUnitInt localId = mockNeat.ints().range(5000, 35_000);
Gson gson = new GsonBuilder().setPrettyPrinting().create();
return mockNeat.reflect(someEntity.class)
.field("deviceLocalID", localId.mapToString())
.field("localID", localId.mapToString())
.map(gson::toJson)
.val();
}
Even the localId
was a final
field in class, all time when something refer to it, it's give random value each time. Can't understand what wrong with that..
java string casting data-generation random-data
add a comment |
Try to use my own Enum
like mock
with help of library mockneat
. But the problem is that I can't take string
value from MockUnit
object for my right working of switch loop.
It's my Enum
:
public enum SubType {
ROUTER("router"),
SERVER("server"),
private final String value;
SubType(final String newValue) {
value = newValue;
}
public String getValue() {
return value;
}
}
And it's my Build
class, where I try to use custom values from SubType
:
public class Build {
private MockNeat mockNeat = MockNeat.threadLocal();
private ArrayList<String> group = new ArrayList<>();
public void addToList() {
group.add("All Devices");
group.add("All Groups");
for (i = 0; i < 10; i++) {
MockUnit subType = mockNeat.from(SubType.class).map(SubType::getValue);
//EDITED:
//For example there are sout(subType) = "router"
Gson gson = new GsonBuilder().setPrettyPrinting().create();
String json = mockNeat.reflect(Build.class)
.field("group", constructGroupField(subType, group))
.map(gson::toJson)
.val();
System.out.println(json);
}
}
private ArrayList<String> constructGroupField(MockUnit subType, ArrayList<String> group) {
//System.out.println(subType);
//Output: net.andreinc.mockneat.abstraction.MockUnit$$Lambda$22/902830499@740773a3
//But how I can get there my string like "router" or ""server"
//How I can cast MockUnit to String value?
//EDITED:
// And there it can be already sout(subType)= "server"
switch (subType.toString()) {
case "router":
group.add("All AAA");
break;
case "server":
group.add("All BBB");
break;
}
return group;
}
}
The main goal is to, depending on which string be in the subtype
field, output the corresponding json
file
UPDATED:
The final Json
file, that I want to produce:
{
"product": "a",
"group": "router"
}
Another situation:
private String someMethod() {
MockUnitInt localId = mockNeat.ints().range(5000, 35_000);
Gson gson = new GsonBuilder().setPrettyPrinting().create();
return mockNeat.reflect(someEntity.class)
.field("deviceLocalID", localId.mapToString())
.field("localID", localId.mapToString())
.map(gson::toJson)
.val();
}
Even the localId
was a final
field in class, all time when something refer to it, it's give random value each time. Can't understand what wrong with that..
java string casting data-generation random-data
add a comment |
Try to use my own Enum
like mock
with help of library mockneat
. But the problem is that I can't take string
value from MockUnit
object for my right working of switch loop.
It's my Enum
:
public enum SubType {
ROUTER("router"),
SERVER("server"),
private final String value;
SubType(final String newValue) {
value = newValue;
}
public String getValue() {
return value;
}
}
And it's my Build
class, where I try to use custom values from SubType
:
public class Build {
private MockNeat mockNeat = MockNeat.threadLocal();
private ArrayList<String> group = new ArrayList<>();
public void addToList() {
group.add("All Devices");
group.add("All Groups");
for (i = 0; i < 10; i++) {
MockUnit subType = mockNeat.from(SubType.class).map(SubType::getValue);
//EDITED:
//For example there are sout(subType) = "router"
Gson gson = new GsonBuilder().setPrettyPrinting().create();
String json = mockNeat.reflect(Build.class)
.field("group", constructGroupField(subType, group))
.map(gson::toJson)
.val();
System.out.println(json);
}
}
private ArrayList<String> constructGroupField(MockUnit subType, ArrayList<String> group) {
//System.out.println(subType);
//Output: net.andreinc.mockneat.abstraction.MockUnit$$Lambda$22/902830499@740773a3
//But how I can get there my string like "router" or ""server"
//How I can cast MockUnit to String value?
//EDITED:
// And there it can be already sout(subType)= "server"
switch (subType.toString()) {
case "router":
group.add("All AAA");
break;
case "server":
group.add("All BBB");
break;
}
return group;
}
}
The main goal is to, depending on which string be in the subtype
field, output the corresponding json
file
UPDATED:
The final Json
file, that I want to produce:
{
"product": "a",
"group": "router"
}
Another situation:
private String someMethod() {
MockUnitInt localId = mockNeat.ints().range(5000, 35_000);
Gson gson = new GsonBuilder().setPrettyPrinting().create();
return mockNeat.reflect(someEntity.class)
.field("deviceLocalID", localId.mapToString())
.field("localID", localId.mapToString())
.map(gson::toJson)
.val();
}
Even the localId
was a final
field in class, all time when something refer to it, it's give random value each time. Can't understand what wrong with that..
java string casting data-generation random-data
Try to use my own Enum
like mock
with help of library mockneat
. But the problem is that I can't take string
value from MockUnit
object for my right working of switch loop.
It's my Enum
:
public enum SubType {
ROUTER("router"),
SERVER("server"),
private final String value;
SubType(final String newValue) {
value = newValue;
}
public String getValue() {
return value;
}
}
And it's my Build
class, where I try to use custom values from SubType
:
public class Build {
private MockNeat mockNeat = MockNeat.threadLocal();
private ArrayList<String> group = new ArrayList<>();
public void addToList() {
group.add("All Devices");
group.add("All Groups");
for (i = 0; i < 10; i++) {
MockUnit subType = mockNeat.from(SubType.class).map(SubType::getValue);
//EDITED:
//For example there are sout(subType) = "router"
Gson gson = new GsonBuilder().setPrettyPrinting().create();
String json = mockNeat.reflect(Build.class)
.field("group", constructGroupField(subType, group))
.map(gson::toJson)
.val();
System.out.println(json);
}
}
private ArrayList<String> constructGroupField(MockUnit subType, ArrayList<String> group) {
//System.out.println(subType);
//Output: net.andreinc.mockneat.abstraction.MockUnit$$Lambda$22/902830499@740773a3
//But how I can get there my string like "router" or ""server"
//How I can cast MockUnit to String value?
//EDITED:
// And there it can be already sout(subType)= "server"
switch (subType.toString()) {
case "router":
group.add("All AAA");
break;
case "server":
group.add("All BBB");
break;
}
return group;
}
}
The main goal is to, depending on which string be in the subtype
field, output the corresponding json
file
UPDATED:
The final Json
file, that I want to produce:
{
"product": "a",
"group": "router"
}
Another situation:
private String someMethod() {
MockUnitInt localId = mockNeat.ints().range(5000, 35_000);
Gson gson = new GsonBuilder().setPrettyPrinting().create();
return mockNeat.reflect(someEntity.class)
.field("deviceLocalID", localId.mapToString())
.field("localID", localId.mapToString())
.map(gson::toJson)
.val();
}
Even the localId
was a final
field in class, all time when something refer to it, it's give random value each time. Can't understand what wrong with that..
java string casting data-generation random-data
java string casting data-generation random-data
edited Nov 26 '18 at 15:16
Spike Johnson
asked Nov 22 '18 at 14:54


Spike JohnsonSpike Johnson
11518
11518
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I think the answer is to change the switch line to:
switch (subType.val().toString())
The idea is that any MockUnit
is not returning any value until you explicitly call val()
on it.
But it's still not clear for me what you want to achieve? How do you want your final json to look like ?
PS: I might help, I am the author of MockNeat.
Thank you, with usingval()
method, I could takeString
value, from my var. But the problem is still in value of MockUnit object. I edited the answer.
– Spike Johnson
Nov 26 '18 at 12:27
@SpikeJohnson Show me how your end JSON will look like in the end (a mock) and I might be able to help you more. Only from the code, I am not sure I understand in what direction you are going.
– Andrei Ciobanu
Nov 26 '18 at 14:53
I edited the question, did you ask about that? In other project, i watching the same, even a variable, which I want make random, is a final, all time, when I appeal to it - the new random result..
– Spike Johnson
Nov 26 '18 at 15:04
I solved the problem with good oldRandom
, but you library could help me with my task more elegantly.
– Spike Johnson
Nov 26 '18 at 15:07
I'm added the same other situation.
– Spike Johnson
Nov 26 '18 at 15:16
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53433557%2fcant-cast-mockunit-to-string-with-mockneat%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think the answer is to change the switch line to:
switch (subType.val().toString())
The idea is that any MockUnit
is not returning any value until you explicitly call val()
on it.
But it's still not clear for me what you want to achieve? How do you want your final json to look like ?
PS: I might help, I am the author of MockNeat.
Thank you, with usingval()
method, I could takeString
value, from my var. But the problem is still in value of MockUnit object. I edited the answer.
– Spike Johnson
Nov 26 '18 at 12:27
@SpikeJohnson Show me how your end JSON will look like in the end (a mock) and I might be able to help you more. Only from the code, I am not sure I understand in what direction you are going.
– Andrei Ciobanu
Nov 26 '18 at 14:53
I edited the question, did you ask about that? In other project, i watching the same, even a variable, which I want make random, is a final, all time, when I appeal to it - the new random result..
– Spike Johnson
Nov 26 '18 at 15:04
I solved the problem with good oldRandom
, but you library could help me with my task more elegantly.
– Spike Johnson
Nov 26 '18 at 15:07
I'm added the same other situation.
– Spike Johnson
Nov 26 '18 at 15:16
add a comment |
I think the answer is to change the switch line to:
switch (subType.val().toString())
The idea is that any MockUnit
is not returning any value until you explicitly call val()
on it.
But it's still not clear for me what you want to achieve? How do you want your final json to look like ?
PS: I might help, I am the author of MockNeat.
Thank you, with usingval()
method, I could takeString
value, from my var. But the problem is still in value of MockUnit object. I edited the answer.
– Spike Johnson
Nov 26 '18 at 12:27
@SpikeJohnson Show me how your end JSON will look like in the end (a mock) and I might be able to help you more. Only from the code, I am not sure I understand in what direction you are going.
– Andrei Ciobanu
Nov 26 '18 at 14:53
I edited the question, did you ask about that? In other project, i watching the same, even a variable, which I want make random, is a final, all time, when I appeal to it - the new random result..
– Spike Johnson
Nov 26 '18 at 15:04
I solved the problem with good oldRandom
, but you library could help me with my task more elegantly.
– Spike Johnson
Nov 26 '18 at 15:07
I'm added the same other situation.
– Spike Johnson
Nov 26 '18 at 15:16
add a comment |
I think the answer is to change the switch line to:
switch (subType.val().toString())
The idea is that any MockUnit
is not returning any value until you explicitly call val()
on it.
But it's still not clear for me what you want to achieve? How do you want your final json to look like ?
PS: I might help, I am the author of MockNeat.
I think the answer is to change the switch line to:
switch (subType.val().toString())
The idea is that any MockUnit
is not returning any value until you explicitly call val()
on it.
But it's still not clear for me what you want to achieve? How do you want your final json to look like ?
PS: I might help, I am the author of MockNeat.
answered Nov 25 '18 at 9:59
Andrei CiobanuAndrei Ciobanu
5,7291862110
5,7291862110
Thank you, with usingval()
method, I could takeString
value, from my var. But the problem is still in value of MockUnit object. I edited the answer.
– Spike Johnson
Nov 26 '18 at 12:27
@SpikeJohnson Show me how your end JSON will look like in the end (a mock) and I might be able to help you more. Only from the code, I am not sure I understand in what direction you are going.
– Andrei Ciobanu
Nov 26 '18 at 14:53
I edited the question, did you ask about that? In other project, i watching the same, even a variable, which I want make random, is a final, all time, when I appeal to it - the new random result..
– Spike Johnson
Nov 26 '18 at 15:04
I solved the problem with good oldRandom
, but you library could help me with my task more elegantly.
– Spike Johnson
Nov 26 '18 at 15:07
I'm added the same other situation.
– Spike Johnson
Nov 26 '18 at 15:16
add a comment |
Thank you, with usingval()
method, I could takeString
value, from my var. But the problem is still in value of MockUnit object. I edited the answer.
– Spike Johnson
Nov 26 '18 at 12:27
@SpikeJohnson Show me how your end JSON will look like in the end (a mock) and I might be able to help you more. Only from the code, I am not sure I understand in what direction you are going.
– Andrei Ciobanu
Nov 26 '18 at 14:53
I edited the question, did you ask about that? In other project, i watching the same, even a variable, which I want make random, is a final, all time, when I appeal to it - the new random result..
– Spike Johnson
Nov 26 '18 at 15:04
I solved the problem with good oldRandom
, but you library could help me with my task more elegantly.
– Spike Johnson
Nov 26 '18 at 15:07
I'm added the same other situation.
– Spike Johnson
Nov 26 '18 at 15:16
Thank you, with using
val()
method, I could take String
value, from my var. But the problem is still in value of MockUnit object. I edited the answer.– Spike Johnson
Nov 26 '18 at 12:27
Thank you, with using
val()
method, I could take String
value, from my var. But the problem is still in value of MockUnit object. I edited the answer.– Spike Johnson
Nov 26 '18 at 12:27
@SpikeJohnson Show me how your end JSON will look like in the end (a mock) and I might be able to help you more. Only from the code, I am not sure I understand in what direction you are going.
– Andrei Ciobanu
Nov 26 '18 at 14:53
@SpikeJohnson Show me how your end JSON will look like in the end (a mock) and I might be able to help you more. Only from the code, I am not sure I understand in what direction you are going.
– Andrei Ciobanu
Nov 26 '18 at 14:53
I edited the question, did you ask about that? In other project, i watching the same, even a variable, which I want make random, is a final, all time, when I appeal to it - the new random result..
– Spike Johnson
Nov 26 '18 at 15:04
I edited the question, did you ask about that? In other project, i watching the same, even a variable, which I want make random, is a final, all time, when I appeal to it - the new random result..
– Spike Johnson
Nov 26 '18 at 15:04
I solved the problem with good old
Random
, but you library could help me with my task more elegantly.– Spike Johnson
Nov 26 '18 at 15:07
I solved the problem with good old
Random
, but you library could help me with my task more elegantly.– Spike Johnson
Nov 26 '18 at 15:07
I'm added the same other situation.
– Spike Johnson
Nov 26 '18 at 15:16
I'm added the same other situation.
– Spike Johnson
Nov 26 '18 at 15:16
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53433557%2fcant-cast-mockunit-to-string-with-mockneat%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pG q9Lpts3