Writing rapidjson document to a file using PrettyWriter
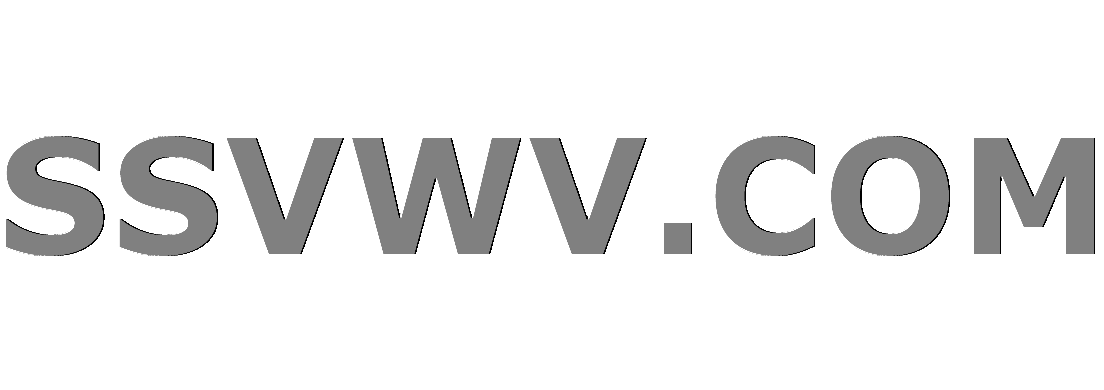
Multi tool use
up vote
0
down vote
favorite
I have been unable to find a direct answer to this question. After searching for some time, I've written the following code but I'm sure that there exists a simpler way of doing the same task.
int persistJSONChanges(rapidjson::Document& fa_cloneDoc, string jsonFilePath)
{
FILE* lp_file = fopen(jsonFilePath.c_str(), "w");
rapidjson::StringBuffer buffer;
rapidjson::PrettyWriter<rapidjson::StringBuffer> writer(buffer);
fa_cloneDoc.Accept(writer);
string temp=buffer.GetString();
unique_ptr<char>l_writeBuffer(new char[temp.size()]);
rapidjson::FileWriteStream l_writeStream(lp_file, l_writeBuffer.get(), temp.size());
rapidjson::PrettyWriter<rapidjson::FileWriteStream> l_writer(l_writeStream);
bool l_returnStatus=fa_cloneDoc.Accept(l_writer);
if(l_returnStatus==false)
{
cout<<endl<<"file update failed"<<endl;
return -1;
}
fclose(lp_file);
return 0;
}
rapidjson
add a comment |
up vote
0
down vote
favorite
I have been unable to find a direct answer to this question. After searching for some time, I've written the following code but I'm sure that there exists a simpler way of doing the same task.
int persistJSONChanges(rapidjson::Document& fa_cloneDoc, string jsonFilePath)
{
FILE* lp_file = fopen(jsonFilePath.c_str(), "w");
rapidjson::StringBuffer buffer;
rapidjson::PrettyWriter<rapidjson::StringBuffer> writer(buffer);
fa_cloneDoc.Accept(writer);
string temp=buffer.GetString();
unique_ptr<char>l_writeBuffer(new char[temp.size()]);
rapidjson::FileWriteStream l_writeStream(lp_file, l_writeBuffer.get(), temp.size());
rapidjson::PrettyWriter<rapidjson::FileWriteStream> l_writer(l_writeStream);
bool l_returnStatus=fa_cloneDoc.Accept(l_writer);
if(l_returnStatus==false)
{
cout<<endl<<"file update failed"<<endl;
return -1;
}
fclose(lp_file);
return 0;
}
rapidjson
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have been unable to find a direct answer to this question. After searching for some time, I've written the following code but I'm sure that there exists a simpler way of doing the same task.
int persistJSONChanges(rapidjson::Document& fa_cloneDoc, string jsonFilePath)
{
FILE* lp_file = fopen(jsonFilePath.c_str(), "w");
rapidjson::StringBuffer buffer;
rapidjson::PrettyWriter<rapidjson::StringBuffer> writer(buffer);
fa_cloneDoc.Accept(writer);
string temp=buffer.GetString();
unique_ptr<char>l_writeBuffer(new char[temp.size()]);
rapidjson::FileWriteStream l_writeStream(lp_file, l_writeBuffer.get(), temp.size());
rapidjson::PrettyWriter<rapidjson::FileWriteStream> l_writer(l_writeStream);
bool l_returnStatus=fa_cloneDoc.Accept(l_writer);
if(l_returnStatus==false)
{
cout<<endl<<"file update failed"<<endl;
return -1;
}
fclose(lp_file);
return 0;
}
rapidjson
I have been unable to find a direct answer to this question. After searching for some time, I've written the following code but I'm sure that there exists a simpler way of doing the same task.
int persistJSONChanges(rapidjson::Document& fa_cloneDoc, string jsonFilePath)
{
FILE* lp_file = fopen(jsonFilePath.c_str(), "w");
rapidjson::StringBuffer buffer;
rapidjson::PrettyWriter<rapidjson::StringBuffer> writer(buffer);
fa_cloneDoc.Accept(writer);
string temp=buffer.GetString();
unique_ptr<char>l_writeBuffer(new char[temp.size()]);
rapidjson::FileWriteStream l_writeStream(lp_file, l_writeBuffer.get(), temp.size());
rapidjson::PrettyWriter<rapidjson::FileWriteStream> l_writer(l_writeStream);
bool l_returnStatus=fa_cloneDoc.Accept(l_writer);
if(l_returnStatus==false)
{
cout<<endl<<"file update failed"<<endl;
return -1;
}
fclose(lp_file);
return 0;
}
rapidjson
rapidjson
asked Nov 20 at 8:22
Vishal Sharma
353320
353320
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
I think you misused the FileWriteStream
. It just needs a buffer of arbitrary size.
You simply needs:
FILE* fp = fopen(...);
char buffer[1024];
FileWriteStream fs(fp, buffer, sizeof(buffer));
PrettyWriter<FileWriteStream> writer(fs);
document.Accept(writer);
fclose(fp);
Is the size of the buffer really arbitrary? Can I use a really small value, regardless of the size of the document?
– Vishal Sharma
Nov 30 at 7:23
1
@VishalSharma Yes. This design is due to performance consideration. The size of buffer can be smaller than the JSON. You may check the source code of FileWriteStream and it is very simple.
– Milo Yip
Dec 1 at 15:54
add a comment |
up vote
0
down vote
I have tried the following which worked for me:
int persistJSONChanges(rapidjson::Document& fa_cloneDoc, string jsonFilePath)
{
rapidjson::StringBuffer buffer;
rapidjson::PrettyWriter<rapidjson::StringBuffer> writer(buffer);
bool l_returnStatus=fa_cloneDoc.Accept(writer);
if(l_returnStatus==false)
{
fprintf(stdout,"n[%s::%d] JSON File update failedn",__FILE__,__LINE__);
return -1;
}
string temp=buffer.GetString();
ofstream out(jsonFilePath.c_str(),std::ofstream::trunc);
out<<temp;
return 0;
}
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
I think you misused the FileWriteStream
. It just needs a buffer of arbitrary size.
You simply needs:
FILE* fp = fopen(...);
char buffer[1024];
FileWriteStream fs(fp, buffer, sizeof(buffer));
PrettyWriter<FileWriteStream> writer(fs);
document.Accept(writer);
fclose(fp);
Is the size of the buffer really arbitrary? Can I use a really small value, regardless of the size of the document?
– Vishal Sharma
Nov 30 at 7:23
1
@VishalSharma Yes. This design is due to performance consideration. The size of buffer can be smaller than the JSON. You may check the source code of FileWriteStream and it is very simple.
– Milo Yip
Dec 1 at 15:54
add a comment |
up vote
1
down vote
accepted
I think you misused the FileWriteStream
. It just needs a buffer of arbitrary size.
You simply needs:
FILE* fp = fopen(...);
char buffer[1024];
FileWriteStream fs(fp, buffer, sizeof(buffer));
PrettyWriter<FileWriteStream> writer(fs);
document.Accept(writer);
fclose(fp);
Is the size of the buffer really arbitrary? Can I use a really small value, regardless of the size of the document?
– Vishal Sharma
Nov 30 at 7:23
1
@VishalSharma Yes. This design is due to performance consideration. The size of buffer can be smaller than the JSON. You may check the source code of FileWriteStream and it is very simple.
– Milo Yip
Dec 1 at 15:54
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
I think you misused the FileWriteStream
. It just needs a buffer of arbitrary size.
You simply needs:
FILE* fp = fopen(...);
char buffer[1024];
FileWriteStream fs(fp, buffer, sizeof(buffer));
PrettyWriter<FileWriteStream> writer(fs);
document.Accept(writer);
fclose(fp);
I think you misused the FileWriteStream
. It just needs a buffer of arbitrary size.
You simply needs:
FILE* fp = fopen(...);
char buffer[1024];
FileWriteStream fs(fp, buffer, sizeof(buffer));
PrettyWriter<FileWriteStream> writer(fs);
document.Accept(writer);
fclose(fp);
answered Nov 30 at 5:12
Milo Yip
3,3791620
3,3791620
Is the size of the buffer really arbitrary? Can I use a really small value, regardless of the size of the document?
– Vishal Sharma
Nov 30 at 7:23
1
@VishalSharma Yes. This design is due to performance consideration. The size of buffer can be smaller than the JSON. You may check the source code of FileWriteStream and it is very simple.
– Milo Yip
Dec 1 at 15:54
add a comment |
Is the size of the buffer really arbitrary? Can I use a really small value, regardless of the size of the document?
– Vishal Sharma
Nov 30 at 7:23
1
@VishalSharma Yes. This design is due to performance consideration. The size of buffer can be smaller than the JSON. You may check the source code of FileWriteStream and it is very simple.
– Milo Yip
Dec 1 at 15:54
Is the size of the buffer really arbitrary? Can I use a really small value, regardless of the size of the document?
– Vishal Sharma
Nov 30 at 7:23
Is the size of the buffer really arbitrary? Can I use a really small value, regardless of the size of the document?
– Vishal Sharma
Nov 30 at 7:23
1
1
@VishalSharma Yes. This design is due to performance consideration. The size of buffer can be smaller than the JSON. You may check the source code of FileWriteStream and it is very simple.
– Milo Yip
Dec 1 at 15:54
@VishalSharma Yes. This design is due to performance consideration. The size of buffer can be smaller than the JSON. You may check the source code of FileWriteStream and it is very simple.
– Milo Yip
Dec 1 at 15:54
add a comment |
up vote
0
down vote
I have tried the following which worked for me:
int persistJSONChanges(rapidjson::Document& fa_cloneDoc, string jsonFilePath)
{
rapidjson::StringBuffer buffer;
rapidjson::PrettyWriter<rapidjson::StringBuffer> writer(buffer);
bool l_returnStatus=fa_cloneDoc.Accept(writer);
if(l_returnStatus==false)
{
fprintf(stdout,"n[%s::%d] JSON File update failedn",__FILE__,__LINE__);
return -1;
}
string temp=buffer.GetString();
ofstream out(jsonFilePath.c_str(),std::ofstream::trunc);
out<<temp;
return 0;
}
add a comment |
up vote
0
down vote
I have tried the following which worked for me:
int persistJSONChanges(rapidjson::Document& fa_cloneDoc, string jsonFilePath)
{
rapidjson::StringBuffer buffer;
rapidjson::PrettyWriter<rapidjson::StringBuffer> writer(buffer);
bool l_returnStatus=fa_cloneDoc.Accept(writer);
if(l_returnStatus==false)
{
fprintf(stdout,"n[%s::%d] JSON File update failedn",__FILE__,__LINE__);
return -1;
}
string temp=buffer.GetString();
ofstream out(jsonFilePath.c_str(),std::ofstream::trunc);
out<<temp;
return 0;
}
add a comment |
up vote
0
down vote
up vote
0
down vote
I have tried the following which worked for me:
int persistJSONChanges(rapidjson::Document& fa_cloneDoc, string jsonFilePath)
{
rapidjson::StringBuffer buffer;
rapidjson::PrettyWriter<rapidjson::StringBuffer> writer(buffer);
bool l_returnStatus=fa_cloneDoc.Accept(writer);
if(l_returnStatus==false)
{
fprintf(stdout,"n[%s::%d] JSON File update failedn",__FILE__,__LINE__);
return -1;
}
string temp=buffer.GetString();
ofstream out(jsonFilePath.c_str(),std::ofstream::trunc);
out<<temp;
return 0;
}
I have tried the following which worked for me:
int persistJSONChanges(rapidjson::Document& fa_cloneDoc, string jsonFilePath)
{
rapidjson::StringBuffer buffer;
rapidjson::PrettyWriter<rapidjson::StringBuffer> writer(buffer);
bool l_returnStatus=fa_cloneDoc.Accept(writer);
if(l_returnStatus==false)
{
fprintf(stdout,"n[%s::%d] JSON File update failedn",__FILE__,__LINE__);
return -1;
}
string temp=buffer.GetString();
ofstream out(jsonFilePath.c_str(),std::ofstream::trunc);
out<<temp;
return 0;
}
answered Nov 30 at 7:18
Vishal Sharma
353320
353320
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53388845%2fwriting-rapidjson-document-to-a-file-using-prettywriter%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
90C40g,h2uKzrN,x,1i3z