How to not repeat the same operations in RxJava?
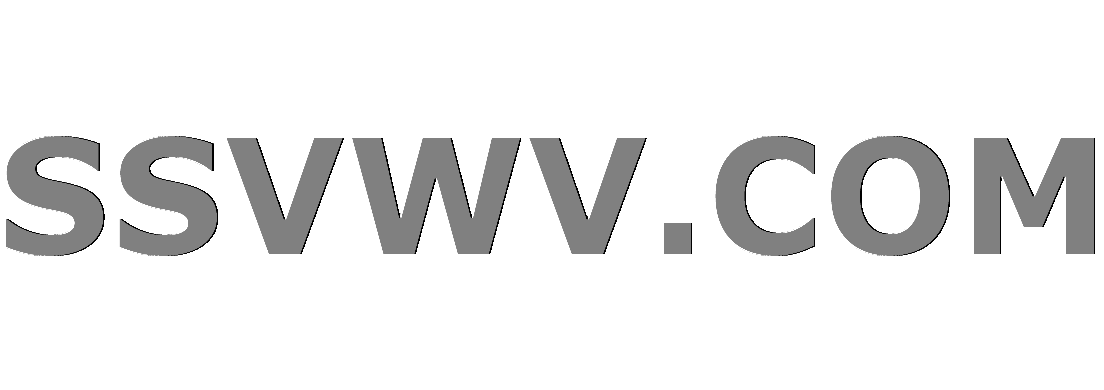
Multi tool use
up vote
1
down vote
favorite
I have a code like following:
authRepository.login(userName, password)
.doOnSubscribe(__ -> apiProgress.setValue(ApiProgress.start()))
.doFinally(() -> apiProgress.setValue(ApiProgress.stop()))
.subscribe(login -> loginData.setValue(login),
err -> apiError.setValue(ApiError.create(err)))
I need to repeat doOnSubscribe(..)
and doFinally
for all api calls.
Is there any way to achieve this thing ?
java

add a comment |
up vote
1
down vote
favorite
I have a code like following:
authRepository.login(userName, password)
.doOnSubscribe(__ -> apiProgress.setValue(ApiProgress.start()))
.doFinally(() -> apiProgress.setValue(ApiProgress.stop()))
.subscribe(login -> loginData.setValue(login),
err -> apiError.setValue(ApiError.create(err)))
I need to repeat doOnSubscribe(..)
and doFinally
for all api calls.
Is there any way to achieve this thing ?
java

add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have a code like following:
authRepository.login(userName, password)
.doOnSubscribe(__ -> apiProgress.setValue(ApiProgress.start()))
.doFinally(() -> apiProgress.setValue(ApiProgress.stop()))
.subscribe(login -> loginData.setValue(login),
err -> apiError.setValue(ApiError.create(err)))
I need to repeat doOnSubscribe(..)
and doFinally
for all api calls.
Is there any way to achieve this thing ?
java

I have a code like following:
authRepository.login(userName, password)
.doOnSubscribe(__ -> apiProgress.setValue(ApiProgress.start()))
.doFinally(() -> apiProgress.setValue(ApiProgress.stop()))
.subscribe(login -> loginData.setValue(login),
err -> apiError.setValue(ApiError.create(err)))
I need to repeat doOnSubscribe(..)
and doFinally
for all api calls.
Is there any way to achieve this thing ?
java

java

edited Nov 20 at 9:10
akarnokd
48.5k998146
48.5k998146
asked Nov 20 at 8:22


Vatish Sharma
167
167
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
Welcome to StackOverflow! https://stackoverflow.com/conduct
You can create something like this using Transformer
(http://reactivex.io/RxJava/javadoc/rx/Single.Transformer.html)
static <T> SingleTransformer<T, T> subscribeAndFinalTransformer() {
return new SingleTransformer<T, T>() {
@Override
public SingleSource<T> apply(Single<T> upstream) {
return upstream.doOnSubscribe(disposable -> {
// Your doOnSubscribe Block
}).doFinally(() -> {
// Your doFinally Block
});
}
};
}
and above reusable Transformer
can be attached for all the Single
using compose
method.
authRepository.login(userName, password).compose(subscribeAndFinalTransformer())
.subscribe()
authRepository.anotherApi().compose(subscribeAndFinalTransformer()).subscribe()
if you are using Observable
or Completable
, you should use equivalent Transformer
instead SingleTransformer
EDIT:
Above approach is convenient if you want to reuse some actions for only certain calls.
if you want to attach the actions to all of your API calls you can create Retrofit CallAdapter
class RxStreamAdapter implements CallAdapter {
private final Class rawType;
private final CallAdapter<Object, Object> nextAdapter;
private final Type returnType;
RxStreamAdapter(Class rawType,
Type returnType,
CallAdapter nextAdapter) {
this.rawType = rawType;
this.returnType = returnType;
this.nextAdapter = nextAdapter;
}
@Override
public Type responseType() {
return nextAdapter.responseType();
}
@Override
public Object adapt(Call call) {
if (rawType == Single.class) {
return ((Single) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
} else if (returnType == Completable.class) {
return ((Completable) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
} else {
// Observable
return ((Observable<Object>) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
}
}
@NotNull
private Consumer<Disposable> getDoOnSubscribe() {
return disposable -> {
};
}
@NotNull
private Action getDoFinally() {
return () -> {
};
}
}
And then add it while creating Retrofit Object (Before RxJava2CallAdapterFactory
)
RetrofitApi retrofitApi = new Retrofit
.Builder()
.addCallAdapterFactory(new CallAdapter.Factory() {
@Override
public CallAdapter<?, ?> get(Type returnType, Annotation annotations, Retrofit retrofit) {
CallAdapter<?, ?> nextAdapter = retrofit.nextCallAdapter(this, returnType, annotations);
Class<?> rawType = getRawType(returnType);
if (rawType == Single.class || rawType == Observable.class || rawType == Completable.class) {
return new RxStreamAdapter(getRawType(returnType), returnType, nextAdapter);
} else {
return nextAdapter;
}
}
})
.addCallAdapterFactory(RxJava2CallAdapterFactory.create())
.build()
You can also set hooks using RxJavaPlugins
. But you cannot differentiate b/w normal stream and Retrofit stream.
Hope it helps!
Thanks for answering. It does solve the problem at some extent but now I need to callcompose(subscribeAndFinalTransformer())
every time I will call an api. Is there any alternative ?
– Vatish Sharma
Nov 20 at 9:34
Btw, you provided the answer of asked question so I am accepting your solution :).
– Vatish Sharma
Nov 20 at 10:19
@VatishSharma I hope you are using retrofit. I have updated the answer. I will still go withcompose()
insteadCallAdapter
due to flexibility. Again there are some cases you had to go withAdapter
approach like when other developers might forget to attach the actions that needed for every call. In that case it would be helpful. Cheers !
– I Don't Exist
Nov 20 at 11:53
Great! Thanks, this is exactly what I want to achieve.
– Vatish Sharma
Nov 20 at 12:05
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Welcome to StackOverflow! https://stackoverflow.com/conduct
You can create something like this using Transformer
(http://reactivex.io/RxJava/javadoc/rx/Single.Transformer.html)
static <T> SingleTransformer<T, T> subscribeAndFinalTransformer() {
return new SingleTransformer<T, T>() {
@Override
public SingleSource<T> apply(Single<T> upstream) {
return upstream.doOnSubscribe(disposable -> {
// Your doOnSubscribe Block
}).doFinally(() -> {
// Your doFinally Block
});
}
};
}
and above reusable Transformer
can be attached for all the Single
using compose
method.
authRepository.login(userName, password).compose(subscribeAndFinalTransformer())
.subscribe()
authRepository.anotherApi().compose(subscribeAndFinalTransformer()).subscribe()
if you are using Observable
or Completable
, you should use equivalent Transformer
instead SingleTransformer
EDIT:
Above approach is convenient if you want to reuse some actions for only certain calls.
if you want to attach the actions to all of your API calls you can create Retrofit CallAdapter
class RxStreamAdapter implements CallAdapter {
private final Class rawType;
private final CallAdapter<Object, Object> nextAdapter;
private final Type returnType;
RxStreamAdapter(Class rawType,
Type returnType,
CallAdapter nextAdapter) {
this.rawType = rawType;
this.returnType = returnType;
this.nextAdapter = nextAdapter;
}
@Override
public Type responseType() {
return nextAdapter.responseType();
}
@Override
public Object adapt(Call call) {
if (rawType == Single.class) {
return ((Single) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
} else if (returnType == Completable.class) {
return ((Completable) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
} else {
// Observable
return ((Observable<Object>) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
}
}
@NotNull
private Consumer<Disposable> getDoOnSubscribe() {
return disposable -> {
};
}
@NotNull
private Action getDoFinally() {
return () -> {
};
}
}
And then add it while creating Retrofit Object (Before RxJava2CallAdapterFactory
)
RetrofitApi retrofitApi = new Retrofit
.Builder()
.addCallAdapterFactory(new CallAdapter.Factory() {
@Override
public CallAdapter<?, ?> get(Type returnType, Annotation annotations, Retrofit retrofit) {
CallAdapter<?, ?> nextAdapter = retrofit.nextCallAdapter(this, returnType, annotations);
Class<?> rawType = getRawType(returnType);
if (rawType == Single.class || rawType == Observable.class || rawType == Completable.class) {
return new RxStreamAdapter(getRawType(returnType), returnType, nextAdapter);
} else {
return nextAdapter;
}
}
})
.addCallAdapterFactory(RxJava2CallAdapterFactory.create())
.build()
You can also set hooks using RxJavaPlugins
. But you cannot differentiate b/w normal stream and Retrofit stream.
Hope it helps!
Thanks for answering. It does solve the problem at some extent but now I need to callcompose(subscribeAndFinalTransformer())
every time I will call an api. Is there any alternative ?
– Vatish Sharma
Nov 20 at 9:34
Btw, you provided the answer of asked question so I am accepting your solution :).
– Vatish Sharma
Nov 20 at 10:19
@VatishSharma I hope you are using retrofit. I have updated the answer. I will still go withcompose()
insteadCallAdapter
due to flexibility. Again there are some cases you had to go withAdapter
approach like when other developers might forget to attach the actions that needed for every call. In that case it would be helpful. Cheers !
– I Don't Exist
Nov 20 at 11:53
Great! Thanks, this is exactly what I want to achieve.
– Vatish Sharma
Nov 20 at 12:05
add a comment |
up vote
2
down vote
accepted
Welcome to StackOverflow! https://stackoverflow.com/conduct
You can create something like this using Transformer
(http://reactivex.io/RxJava/javadoc/rx/Single.Transformer.html)
static <T> SingleTransformer<T, T> subscribeAndFinalTransformer() {
return new SingleTransformer<T, T>() {
@Override
public SingleSource<T> apply(Single<T> upstream) {
return upstream.doOnSubscribe(disposable -> {
// Your doOnSubscribe Block
}).doFinally(() -> {
// Your doFinally Block
});
}
};
}
and above reusable Transformer
can be attached for all the Single
using compose
method.
authRepository.login(userName, password).compose(subscribeAndFinalTransformer())
.subscribe()
authRepository.anotherApi().compose(subscribeAndFinalTransformer()).subscribe()
if you are using Observable
or Completable
, you should use equivalent Transformer
instead SingleTransformer
EDIT:
Above approach is convenient if you want to reuse some actions for only certain calls.
if you want to attach the actions to all of your API calls you can create Retrofit CallAdapter
class RxStreamAdapter implements CallAdapter {
private final Class rawType;
private final CallAdapter<Object, Object> nextAdapter;
private final Type returnType;
RxStreamAdapter(Class rawType,
Type returnType,
CallAdapter nextAdapter) {
this.rawType = rawType;
this.returnType = returnType;
this.nextAdapter = nextAdapter;
}
@Override
public Type responseType() {
return nextAdapter.responseType();
}
@Override
public Object adapt(Call call) {
if (rawType == Single.class) {
return ((Single) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
} else if (returnType == Completable.class) {
return ((Completable) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
} else {
// Observable
return ((Observable<Object>) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
}
}
@NotNull
private Consumer<Disposable> getDoOnSubscribe() {
return disposable -> {
};
}
@NotNull
private Action getDoFinally() {
return () -> {
};
}
}
And then add it while creating Retrofit Object (Before RxJava2CallAdapterFactory
)
RetrofitApi retrofitApi = new Retrofit
.Builder()
.addCallAdapterFactory(new CallAdapter.Factory() {
@Override
public CallAdapter<?, ?> get(Type returnType, Annotation annotations, Retrofit retrofit) {
CallAdapter<?, ?> nextAdapter = retrofit.nextCallAdapter(this, returnType, annotations);
Class<?> rawType = getRawType(returnType);
if (rawType == Single.class || rawType == Observable.class || rawType == Completable.class) {
return new RxStreamAdapter(getRawType(returnType), returnType, nextAdapter);
} else {
return nextAdapter;
}
}
})
.addCallAdapterFactory(RxJava2CallAdapterFactory.create())
.build()
You can also set hooks using RxJavaPlugins
. But you cannot differentiate b/w normal stream and Retrofit stream.
Hope it helps!
Thanks for answering. It does solve the problem at some extent but now I need to callcompose(subscribeAndFinalTransformer())
every time I will call an api. Is there any alternative ?
– Vatish Sharma
Nov 20 at 9:34
Btw, you provided the answer of asked question so I am accepting your solution :).
– Vatish Sharma
Nov 20 at 10:19
@VatishSharma I hope you are using retrofit. I have updated the answer. I will still go withcompose()
insteadCallAdapter
due to flexibility. Again there are some cases you had to go withAdapter
approach like when other developers might forget to attach the actions that needed for every call. In that case it would be helpful. Cheers !
– I Don't Exist
Nov 20 at 11:53
Great! Thanks, this is exactly what I want to achieve.
– Vatish Sharma
Nov 20 at 12:05
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Welcome to StackOverflow! https://stackoverflow.com/conduct
You can create something like this using Transformer
(http://reactivex.io/RxJava/javadoc/rx/Single.Transformer.html)
static <T> SingleTransformer<T, T> subscribeAndFinalTransformer() {
return new SingleTransformer<T, T>() {
@Override
public SingleSource<T> apply(Single<T> upstream) {
return upstream.doOnSubscribe(disposable -> {
// Your doOnSubscribe Block
}).doFinally(() -> {
// Your doFinally Block
});
}
};
}
and above reusable Transformer
can be attached for all the Single
using compose
method.
authRepository.login(userName, password).compose(subscribeAndFinalTransformer())
.subscribe()
authRepository.anotherApi().compose(subscribeAndFinalTransformer()).subscribe()
if you are using Observable
or Completable
, you should use equivalent Transformer
instead SingleTransformer
EDIT:
Above approach is convenient if you want to reuse some actions for only certain calls.
if you want to attach the actions to all of your API calls you can create Retrofit CallAdapter
class RxStreamAdapter implements CallAdapter {
private final Class rawType;
private final CallAdapter<Object, Object> nextAdapter;
private final Type returnType;
RxStreamAdapter(Class rawType,
Type returnType,
CallAdapter nextAdapter) {
this.rawType = rawType;
this.returnType = returnType;
this.nextAdapter = nextAdapter;
}
@Override
public Type responseType() {
return nextAdapter.responseType();
}
@Override
public Object adapt(Call call) {
if (rawType == Single.class) {
return ((Single) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
} else if (returnType == Completable.class) {
return ((Completable) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
} else {
// Observable
return ((Observable<Object>) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
}
}
@NotNull
private Consumer<Disposable> getDoOnSubscribe() {
return disposable -> {
};
}
@NotNull
private Action getDoFinally() {
return () -> {
};
}
}
And then add it while creating Retrofit Object (Before RxJava2CallAdapterFactory
)
RetrofitApi retrofitApi = new Retrofit
.Builder()
.addCallAdapterFactory(new CallAdapter.Factory() {
@Override
public CallAdapter<?, ?> get(Type returnType, Annotation annotations, Retrofit retrofit) {
CallAdapter<?, ?> nextAdapter = retrofit.nextCallAdapter(this, returnType, annotations);
Class<?> rawType = getRawType(returnType);
if (rawType == Single.class || rawType == Observable.class || rawType == Completable.class) {
return new RxStreamAdapter(getRawType(returnType), returnType, nextAdapter);
} else {
return nextAdapter;
}
}
})
.addCallAdapterFactory(RxJava2CallAdapterFactory.create())
.build()
You can also set hooks using RxJavaPlugins
. But you cannot differentiate b/w normal stream and Retrofit stream.
Hope it helps!
Welcome to StackOverflow! https://stackoverflow.com/conduct
You can create something like this using Transformer
(http://reactivex.io/RxJava/javadoc/rx/Single.Transformer.html)
static <T> SingleTransformer<T, T> subscribeAndFinalTransformer() {
return new SingleTransformer<T, T>() {
@Override
public SingleSource<T> apply(Single<T> upstream) {
return upstream.doOnSubscribe(disposable -> {
// Your doOnSubscribe Block
}).doFinally(() -> {
// Your doFinally Block
});
}
};
}
and above reusable Transformer
can be attached for all the Single
using compose
method.
authRepository.login(userName, password).compose(subscribeAndFinalTransformer())
.subscribe()
authRepository.anotherApi().compose(subscribeAndFinalTransformer()).subscribe()
if you are using Observable
or Completable
, you should use equivalent Transformer
instead SingleTransformer
EDIT:
Above approach is convenient if you want to reuse some actions for only certain calls.
if you want to attach the actions to all of your API calls you can create Retrofit CallAdapter
class RxStreamAdapter implements CallAdapter {
private final Class rawType;
private final CallAdapter<Object, Object> nextAdapter;
private final Type returnType;
RxStreamAdapter(Class rawType,
Type returnType,
CallAdapter nextAdapter) {
this.rawType = rawType;
this.returnType = returnType;
this.nextAdapter = nextAdapter;
}
@Override
public Type responseType() {
return nextAdapter.responseType();
}
@Override
public Object adapt(Call call) {
if (rawType == Single.class) {
return ((Single) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
} else if (returnType == Completable.class) {
return ((Completable) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
} else {
// Observable
return ((Observable<Object>) nextAdapter.adapt(call))
.doOnSubscribe(getDoOnSubscribe())
.doFinally(getDoFinally());
}
}
@NotNull
private Consumer<Disposable> getDoOnSubscribe() {
return disposable -> {
};
}
@NotNull
private Action getDoFinally() {
return () -> {
};
}
}
And then add it while creating Retrofit Object (Before RxJava2CallAdapterFactory
)
RetrofitApi retrofitApi = new Retrofit
.Builder()
.addCallAdapterFactory(new CallAdapter.Factory() {
@Override
public CallAdapter<?, ?> get(Type returnType, Annotation annotations, Retrofit retrofit) {
CallAdapter<?, ?> nextAdapter = retrofit.nextCallAdapter(this, returnType, annotations);
Class<?> rawType = getRawType(returnType);
if (rawType == Single.class || rawType == Observable.class || rawType == Completable.class) {
return new RxStreamAdapter(getRawType(returnType), returnType, nextAdapter);
} else {
return nextAdapter;
}
}
})
.addCallAdapterFactory(RxJava2CallAdapterFactory.create())
.build()
You can also set hooks using RxJavaPlugins
. But you cannot differentiate b/w normal stream and Retrofit stream.
Hope it helps!
edited Nov 20 at 11:50
answered Nov 20 at 9:01
I Don't Exist
2,33311418
2,33311418
Thanks for answering. It does solve the problem at some extent but now I need to callcompose(subscribeAndFinalTransformer())
every time I will call an api. Is there any alternative ?
– Vatish Sharma
Nov 20 at 9:34
Btw, you provided the answer of asked question so I am accepting your solution :).
– Vatish Sharma
Nov 20 at 10:19
@VatishSharma I hope you are using retrofit. I have updated the answer. I will still go withcompose()
insteadCallAdapter
due to flexibility. Again there are some cases you had to go withAdapter
approach like when other developers might forget to attach the actions that needed for every call. In that case it would be helpful. Cheers !
– I Don't Exist
Nov 20 at 11:53
Great! Thanks, this is exactly what I want to achieve.
– Vatish Sharma
Nov 20 at 12:05
add a comment |
Thanks for answering. It does solve the problem at some extent but now I need to callcompose(subscribeAndFinalTransformer())
every time I will call an api. Is there any alternative ?
– Vatish Sharma
Nov 20 at 9:34
Btw, you provided the answer of asked question so I am accepting your solution :).
– Vatish Sharma
Nov 20 at 10:19
@VatishSharma I hope you are using retrofit. I have updated the answer. I will still go withcompose()
insteadCallAdapter
due to flexibility. Again there are some cases you had to go withAdapter
approach like when other developers might forget to attach the actions that needed for every call. In that case it would be helpful. Cheers !
– I Don't Exist
Nov 20 at 11:53
Great! Thanks, this is exactly what I want to achieve.
– Vatish Sharma
Nov 20 at 12:05
Thanks for answering. It does solve the problem at some extent but now I need to call
compose(subscribeAndFinalTransformer())
every time I will call an api. Is there any alternative ?– Vatish Sharma
Nov 20 at 9:34
Thanks for answering. It does solve the problem at some extent but now I need to call
compose(subscribeAndFinalTransformer())
every time I will call an api. Is there any alternative ?– Vatish Sharma
Nov 20 at 9:34
Btw, you provided the answer of asked question so I am accepting your solution :).
– Vatish Sharma
Nov 20 at 10:19
Btw, you provided the answer of asked question so I am accepting your solution :).
– Vatish Sharma
Nov 20 at 10:19
@VatishSharma I hope you are using retrofit. I have updated the answer. I will still go with
compose()
instead CallAdapter
due to flexibility. Again there are some cases you had to go with Adapter
approach like when other developers might forget to attach the actions that needed for every call. In that case it would be helpful. Cheers !– I Don't Exist
Nov 20 at 11:53
@VatishSharma I hope you are using retrofit. I have updated the answer. I will still go with
compose()
instead CallAdapter
due to flexibility. Again there are some cases you had to go with Adapter
approach like when other developers might forget to attach the actions that needed for every call. In that case it would be helpful. Cheers !– I Don't Exist
Nov 20 at 11:53
Great! Thanks, this is exactly what I want to achieve.
– Vatish Sharma
Nov 20 at 12:05
Great! Thanks, this is exactly what I want to achieve.
– Vatish Sharma
Nov 20 at 12:05
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53388850%2fhow-to-not-repeat-the-same-operations-in-rxjava%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
RnKZMokCCkw8zGQVZMp7wnQwE U39RMHGmcKKK