Spring Data REDIS with JEDIS connection terminated after few minutes
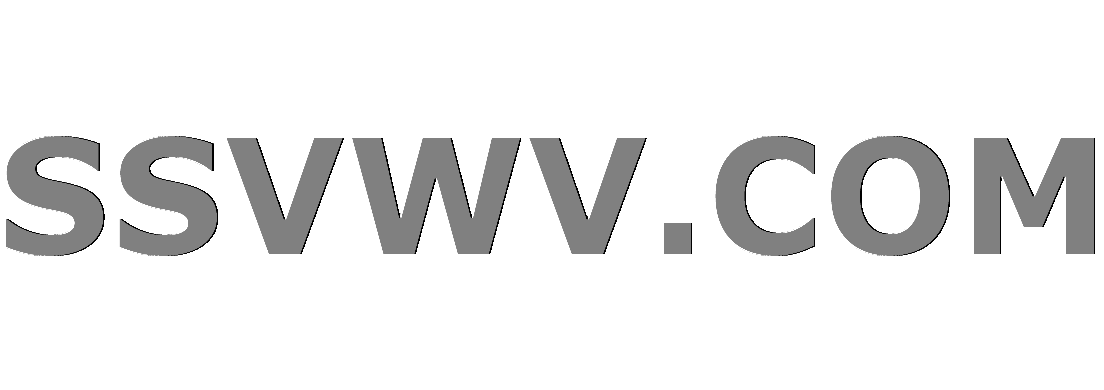
Multi tool use
I have web application Spring+Spring MVC in Eclipse (OS WIN 10).
I have the following mavenized setup:
<spring.version>5.1.2.RELEASE</spring.version>
<spring-data-redis.version>2.1.2.RELEASE</spring-data-redis.version>
<jedis.version>2.9.0</jedis.version>
I've created function:
@Override
public Map<String, Double> find(final String set, final double min, final double max) {
Set<Tuple> mostViewed = redisTemplate.execute(new RedisCallback<Set<Tuple>>() {
@Override
public Set<Tuple> doInRedis(RedisConnection con)
throws DataAccessException {
Set<Tuple> zRangeByScoreWithScore = con.zRangeByScoreWithScores(set.getBytes(), min, max);
return zRangeByScoreWithScore;
}
});
Map<String, Double> mScoreMap = new HashMap<String, Double>();
for (Tuple t : mostViewed) {
mScoreMap.put(new String(t.getValue()), t.getScore());
}
return mScoreMap;
}
My JEDIS configuration:
@Bean
public JedisPoolConfig jedisPoolConfig() {
JedisPoolConfig jedisPoolConfig = new JedisPoolConfig();
jedisPoolConfig.setMaxTotal(10);
jedisPoolConfig.setMaxIdle(5);
jedisPoolConfig.setMinIdle(2);
jedisPoolConfig.setTestOnBorrow(true);
jedisPoolConfig.setTestOnReturn(true);
jedisPoolConfig.setMaxWaitMillis(3000);
jedisPoolConfig.setTimeBetweenEvictionRunsMillis(60000);
return jedisPoolConfig;
}
@Bean
public JedisConnectionFactory jedisConnectionFactory() {
RedisStandaloneConfiguration redisStandaloneConfiguration = new RedisStandaloneConfiguration("localhost", 6379);
JedisClientConfigurationBuilder jedisClientConfiguration = JedisClientConfiguration.builder();
jedisClientConfiguration.connectTimeout(Duration.ofSeconds(60));// 60s connection timeout
jedisClientConfiguration.usePooling();
JedisConnectionFactory jedisConFactory = new JedisConnectionFactory(redisStandaloneConfiguration,
jedisClientConfiguration.build());
return jedisConFactory;
}
@Bean
public StringRedisSerializer stringRedisSerializer() {
StringRedisSerializer stringRedisSerializer = new StringRedisSerializer();
return stringRedisSerializer;
}
@Bean
RedisTemplate<String, String> redisTemplate() {
final RedisTemplate<String, String> redisTemplate = new RedisTemplate<String, String>();
redisTemplate.setConnectionFactory(jedisConnectionFactory());
redisTemplate.setDefaultSerializer(stringRedisSerializer());
redisTemplate.setKeySerializer(stringRedisSerializer());
redisTemplate.setHashKeySerializer(stringRedisSerializer());
redisTemplate.setEnableTransactionSupport(true);
return redisTemplate;
}
but, after about 1-2 minutes, my application freeze, with this message in log file (Eclipse log):
[http-nio-8080-exec-9] DEBUG o.s.d.r.core.RedisConnectionUtils - Opening RedisConnection
redis server log (refresh application in web browser has no effect, there are no new messages):
[984] 21 Nov 11:45:00.163 * 100 changes in 300 seconds. Saving...
[984] 21 Nov 11:45:00.170 * Background saving started by pid 13692
[984] 21 Nov 11:45:00.270 # fork operation complete
[984] 21 Nov 11:45:00.270 * Background saving terminated with success
[984] 21 Nov 12:30:00.162 * 100 changes in 300 seconds. Saving...
[984] 21 Nov 12:30:00.169 * Background saving started by pid 11976
[984] 21 Nov 12:30:00.269 # fork operation complete
[984] 21 Nov 12:30:00.271 * Background saving terminated with success
when I stop and start server (Tomcat 8.0.41) in Eclipse everything is OK for 1-2 minutes. When I stop and start REDIS server has no effect.
spring redis jedis spring-data-redis
add a comment |
I have web application Spring+Spring MVC in Eclipse (OS WIN 10).
I have the following mavenized setup:
<spring.version>5.1.2.RELEASE</spring.version>
<spring-data-redis.version>2.1.2.RELEASE</spring-data-redis.version>
<jedis.version>2.9.0</jedis.version>
I've created function:
@Override
public Map<String, Double> find(final String set, final double min, final double max) {
Set<Tuple> mostViewed = redisTemplate.execute(new RedisCallback<Set<Tuple>>() {
@Override
public Set<Tuple> doInRedis(RedisConnection con)
throws DataAccessException {
Set<Tuple> zRangeByScoreWithScore = con.zRangeByScoreWithScores(set.getBytes(), min, max);
return zRangeByScoreWithScore;
}
});
Map<String, Double> mScoreMap = new HashMap<String, Double>();
for (Tuple t : mostViewed) {
mScoreMap.put(new String(t.getValue()), t.getScore());
}
return mScoreMap;
}
My JEDIS configuration:
@Bean
public JedisPoolConfig jedisPoolConfig() {
JedisPoolConfig jedisPoolConfig = new JedisPoolConfig();
jedisPoolConfig.setMaxTotal(10);
jedisPoolConfig.setMaxIdle(5);
jedisPoolConfig.setMinIdle(2);
jedisPoolConfig.setTestOnBorrow(true);
jedisPoolConfig.setTestOnReturn(true);
jedisPoolConfig.setMaxWaitMillis(3000);
jedisPoolConfig.setTimeBetweenEvictionRunsMillis(60000);
return jedisPoolConfig;
}
@Bean
public JedisConnectionFactory jedisConnectionFactory() {
RedisStandaloneConfiguration redisStandaloneConfiguration = new RedisStandaloneConfiguration("localhost", 6379);
JedisClientConfigurationBuilder jedisClientConfiguration = JedisClientConfiguration.builder();
jedisClientConfiguration.connectTimeout(Duration.ofSeconds(60));// 60s connection timeout
jedisClientConfiguration.usePooling();
JedisConnectionFactory jedisConFactory = new JedisConnectionFactory(redisStandaloneConfiguration,
jedisClientConfiguration.build());
return jedisConFactory;
}
@Bean
public StringRedisSerializer stringRedisSerializer() {
StringRedisSerializer stringRedisSerializer = new StringRedisSerializer();
return stringRedisSerializer;
}
@Bean
RedisTemplate<String, String> redisTemplate() {
final RedisTemplate<String, String> redisTemplate = new RedisTemplate<String, String>();
redisTemplate.setConnectionFactory(jedisConnectionFactory());
redisTemplate.setDefaultSerializer(stringRedisSerializer());
redisTemplate.setKeySerializer(stringRedisSerializer());
redisTemplate.setHashKeySerializer(stringRedisSerializer());
redisTemplate.setEnableTransactionSupport(true);
return redisTemplate;
}
but, after about 1-2 minutes, my application freeze, with this message in log file (Eclipse log):
[http-nio-8080-exec-9] DEBUG o.s.d.r.core.RedisConnectionUtils - Opening RedisConnection
redis server log (refresh application in web browser has no effect, there are no new messages):
[984] 21 Nov 11:45:00.163 * 100 changes in 300 seconds. Saving...
[984] 21 Nov 11:45:00.170 * Background saving started by pid 13692
[984] 21 Nov 11:45:00.270 # fork operation complete
[984] 21 Nov 11:45:00.270 * Background saving terminated with success
[984] 21 Nov 12:30:00.162 * 100 changes in 300 seconds. Saving...
[984] 21 Nov 12:30:00.169 * Background saving started by pid 11976
[984] 21 Nov 12:30:00.269 # fork operation complete
[984] 21 Nov 12:30:00.271 * Background saving terminated with success
when I stop and start server (Tomcat 8.0.41) in Eclipse everything is OK for 1-2 minutes. When I stop and start REDIS server has no effect.
spring redis jedis spring-data-redis
add a comment |
I have web application Spring+Spring MVC in Eclipse (OS WIN 10).
I have the following mavenized setup:
<spring.version>5.1.2.RELEASE</spring.version>
<spring-data-redis.version>2.1.2.RELEASE</spring-data-redis.version>
<jedis.version>2.9.0</jedis.version>
I've created function:
@Override
public Map<String, Double> find(final String set, final double min, final double max) {
Set<Tuple> mostViewed = redisTemplate.execute(new RedisCallback<Set<Tuple>>() {
@Override
public Set<Tuple> doInRedis(RedisConnection con)
throws DataAccessException {
Set<Tuple> zRangeByScoreWithScore = con.zRangeByScoreWithScores(set.getBytes(), min, max);
return zRangeByScoreWithScore;
}
});
Map<String, Double> mScoreMap = new HashMap<String, Double>();
for (Tuple t : mostViewed) {
mScoreMap.put(new String(t.getValue()), t.getScore());
}
return mScoreMap;
}
My JEDIS configuration:
@Bean
public JedisPoolConfig jedisPoolConfig() {
JedisPoolConfig jedisPoolConfig = new JedisPoolConfig();
jedisPoolConfig.setMaxTotal(10);
jedisPoolConfig.setMaxIdle(5);
jedisPoolConfig.setMinIdle(2);
jedisPoolConfig.setTestOnBorrow(true);
jedisPoolConfig.setTestOnReturn(true);
jedisPoolConfig.setMaxWaitMillis(3000);
jedisPoolConfig.setTimeBetweenEvictionRunsMillis(60000);
return jedisPoolConfig;
}
@Bean
public JedisConnectionFactory jedisConnectionFactory() {
RedisStandaloneConfiguration redisStandaloneConfiguration = new RedisStandaloneConfiguration("localhost", 6379);
JedisClientConfigurationBuilder jedisClientConfiguration = JedisClientConfiguration.builder();
jedisClientConfiguration.connectTimeout(Duration.ofSeconds(60));// 60s connection timeout
jedisClientConfiguration.usePooling();
JedisConnectionFactory jedisConFactory = new JedisConnectionFactory(redisStandaloneConfiguration,
jedisClientConfiguration.build());
return jedisConFactory;
}
@Bean
public StringRedisSerializer stringRedisSerializer() {
StringRedisSerializer stringRedisSerializer = new StringRedisSerializer();
return stringRedisSerializer;
}
@Bean
RedisTemplate<String, String> redisTemplate() {
final RedisTemplate<String, String> redisTemplate = new RedisTemplate<String, String>();
redisTemplate.setConnectionFactory(jedisConnectionFactory());
redisTemplate.setDefaultSerializer(stringRedisSerializer());
redisTemplate.setKeySerializer(stringRedisSerializer());
redisTemplate.setHashKeySerializer(stringRedisSerializer());
redisTemplate.setEnableTransactionSupport(true);
return redisTemplate;
}
but, after about 1-2 minutes, my application freeze, with this message in log file (Eclipse log):
[http-nio-8080-exec-9] DEBUG o.s.d.r.core.RedisConnectionUtils - Opening RedisConnection
redis server log (refresh application in web browser has no effect, there are no new messages):
[984] 21 Nov 11:45:00.163 * 100 changes in 300 seconds. Saving...
[984] 21 Nov 11:45:00.170 * Background saving started by pid 13692
[984] 21 Nov 11:45:00.270 # fork operation complete
[984] 21 Nov 11:45:00.270 * Background saving terminated with success
[984] 21 Nov 12:30:00.162 * 100 changes in 300 seconds. Saving...
[984] 21 Nov 12:30:00.169 * Background saving started by pid 11976
[984] 21 Nov 12:30:00.269 # fork operation complete
[984] 21 Nov 12:30:00.271 * Background saving terminated with success
when I stop and start server (Tomcat 8.0.41) in Eclipse everything is OK for 1-2 minutes. When I stop and start REDIS server has no effect.
spring redis jedis spring-data-redis
I have web application Spring+Spring MVC in Eclipse (OS WIN 10).
I have the following mavenized setup:
<spring.version>5.1.2.RELEASE</spring.version>
<spring-data-redis.version>2.1.2.RELEASE</spring-data-redis.version>
<jedis.version>2.9.0</jedis.version>
I've created function:
@Override
public Map<String, Double> find(final String set, final double min, final double max) {
Set<Tuple> mostViewed = redisTemplate.execute(new RedisCallback<Set<Tuple>>() {
@Override
public Set<Tuple> doInRedis(RedisConnection con)
throws DataAccessException {
Set<Tuple> zRangeByScoreWithScore = con.zRangeByScoreWithScores(set.getBytes(), min, max);
return zRangeByScoreWithScore;
}
});
Map<String, Double> mScoreMap = new HashMap<String, Double>();
for (Tuple t : mostViewed) {
mScoreMap.put(new String(t.getValue()), t.getScore());
}
return mScoreMap;
}
My JEDIS configuration:
@Bean
public JedisPoolConfig jedisPoolConfig() {
JedisPoolConfig jedisPoolConfig = new JedisPoolConfig();
jedisPoolConfig.setMaxTotal(10);
jedisPoolConfig.setMaxIdle(5);
jedisPoolConfig.setMinIdle(2);
jedisPoolConfig.setTestOnBorrow(true);
jedisPoolConfig.setTestOnReturn(true);
jedisPoolConfig.setMaxWaitMillis(3000);
jedisPoolConfig.setTimeBetweenEvictionRunsMillis(60000);
return jedisPoolConfig;
}
@Bean
public JedisConnectionFactory jedisConnectionFactory() {
RedisStandaloneConfiguration redisStandaloneConfiguration = new RedisStandaloneConfiguration("localhost", 6379);
JedisClientConfigurationBuilder jedisClientConfiguration = JedisClientConfiguration.builder();
jedisClientConfiguration.connectTimeout(Duration.ofSeconds(60));// 60s connection timeout
jedisClientConfiguration.usePooling();
JedisConnectionFactory jedisConFactory = new JedisConnectionFactory(redisStandaloneConfiguration,
jedisClientConfiguration.build());
return jedisConFactory;
}
@Bean
public StringRedisSerializer stringRedisSerializer() {
StringRedisSerializer stringRedisSerializer = new StringRedisSerializer();
return stringRedisSerializer;
}
@Bean
RedisTemplate<String, String> redisTemplate() {
final RedisTemplate<String, String> redisTemplate = new RedisTemplate<String, String>();
redisTemplate.setConnectionFactory(jedisConnectionFactory());
redisTemplate.setDefaultSerializer(stringRedisSerializer());
redisTemplate.setKeySerializer(stringRedisSerializer());
redisTemplate.setHashKeySerializer(stringRedisSerializer());
redisTemplate.setEnableTransactionSupport(true);
return redisTemplate;
}
but, after about 1-2 minutes, my application freeze, with this message in log file (Eclipse log):
[http-nio-8080-exec-9] DEBUG o.s.d.r.core.RedisConnectionUtils - Opening RedisConnection
redis server log (refresh application in web browser has no effect, there are no new messages):
[984] 21 Nov 11:45:00.163 * 100 changes in 300 seconds. Saving...
[984] 21 Nov 11:45:00.170 * Background saving started by pid 13692
[984] 21 Nov 11:45:00.270 # fork operation complete
[984] 21 Nov 11:45:00.270 * Background saving terminated with success
[984] 21 Nov 12:30:00.162 * 100 changes in 300 seconds. Saving...
[984] 21 Nov 12:30:00.169 * Background saving started by pid 11976
[984] 21 Nov 12:30:00.269 # fork operation complete
[984] 21 Nov 12:30:00.271 * Background saving terminated with success
when I stop and start server (Tomcat 8.0.41) in Eclipse everything is OK for 1-2 minutes. When I stop and start REDIS server has no effect.
spring redis jedis spring-data-redis
spring redis jedis spring-data-redis
edited Nov 21 '18 at 22:33
asked Nov 21 '18 at 11:50
mary
61
61
add a comment |
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53411435%2fspring-data-redis-with-jedis-connection-terminated-after-few-minutes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53411435%2fspring-data-redis-with-jedis-connection-terminated-after-few-minutes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ArnIxXfrtirmox3O3UO0 H28NuoWooV8h2Nj3Q0wProZdYIkT3R,Y,Tczi8oi,uC98T,cMTVdqaPeHWUhs