Vuejs build checkbox dynamically from array
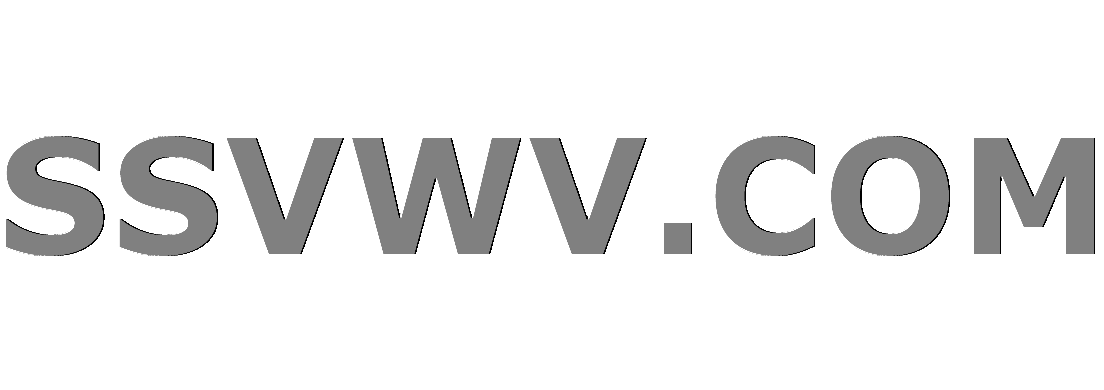
Multi tool use
up vote
0
down vote
favorite
I´m trying to build and checkbox from the setCheckbox method. The idea is to set dynamically the names to build the checkbox array.
<div id='example-3'>
<div v-for="(item, index) in names" :key="index">
<input type="checkbox" :id="item.name" v-model="item.checked">
<label :for="item.name">{{ item.name }}</label>
</div>
<span>Checked names: {{ checkedNames }}</span>
</div>
I removed the names array that supported the checkbox, in order to pass dynamically the new values.
new Vue({
el: '#example-3',
data() {
return {
names:
/* Below is how the names should receive the array to support the checkbox
[
{
name: '',
checked: false
}, {
name: '',
checked: false
}, {
name: '',
checked: false
}
]
*/
}
},
method: {
setCheckbox: function() {
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = value.match(regExp);
var arrCheckbox = ;
for (var i = 0; i < matches.length; i++) {
var str = matches[i];
arrCheckbox.push(str);
// set the names in the array
const names = arrCheckbox
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
})
Basically trying to pass array to names dynamically, method sets values require to build names.
https://jsfiddle.net/bernlt/aj6apozq/114/
javascript vue.js
add a comment |
up vote
0
down vote
favorite
I´m trying to build and checkbox from the setCheckbox method. The idea is to set dynamically the names to build the checkbox array.
<div id='example-3'>
<div v-for="(item, index) in names" :key="index">
<input type="checkbox" :id="item.name" v-model="item.checked">
<label :for="item.name">{{ item.name }}</label>
</div>
<span>Checked names: {{ checkedNames }}</span>
</div>
I removed the names array that supported the checkbox, in order to pass dynamically the new values.
new Vue({
el: '#example-3',
data() {
return {
names:
/* Below is how the names should receive the array to support the checkbox
[
{
name: '',
checked: false
}, {
name: '',
checked: false
}, {
name: '',
checked: false
}
]
*/
}
},
method: {
setCheckbox: function() {
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = value.match(regExp);
var arrCheckbox = ;
for (var i = 0; i < matches.length; i++) {
var str = matches[i];
arrCheckbox.push(str);
// set the names in the array
const names = arrCheckbox
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
})
Basically trying to pass array to names dynamically, method sets values require to build names.
https://jsfiddle.net/bernlt/aj6apozq/114/
javascript vue.js
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I´m trying to build and checkbox from the setCheckbox method. The idea is to set dynamically the names to build the checkbox array.
<div id='example-3'>
<div v-for="(item, index) in names" :key="index">
<input type="checkbox" :id="item.name" v-model="item.checked">
<label :for="item.name">{{ item.name }}</label>
</div>
<span>Checked names: {{ checkedNames }}</span>
</div>
I removed the names array that supported the checkbox, in order to pass dynamically the new values.
new Vue({
el: '#example-3',
data() {
return {
names:
/* Below is how the names should receive the array to support the checkbox
[
{
name: '',
checked: false
}, {
name: '',
checked: false
}, {
name: '',
checked: false
}
]
*/
}
},
method: {
setCheckbox: function() {
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = value.match(regExp);
var arrCheckbox = ;
for (var i = 0; i < matches.length; i++) {
var str = matches[i];
arrCheckbox.push(str);
// set the names in the array
const names = arrCheckbox
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
})
Basically trying to pass array to names dynamically, method sets values require to build names.
https://jsfiddle.net/bernlt/aj6apozq/114/
javascript vue.js
I´m trying to build and checkbox from the setCheckbox method. The idea is to set dynamically the names to build the checkbox array.
<div id='example-3'>
<div v-for="(item, index) in names" :key="index">
<input type="checkbox" :id="item.name" v-model="item.checked">
<label :for="item.name">{{ item.name }}</label>
</div>
<span>Checked names: {{ checkedNames }}</span>
</div>
I removed the names array that supported the checkbox, in order to pass dynamically the new values.
new Vue({
el: '#example-3',
data() {
return {
names:
/* Below is how the names should receive the array to support the checkbox
[
{
name: '',
checked: false
}, {
name: '',
checked: false
}, {
name: '',
checked: false
}
]
*/
}
},
method: {
setCheckbox: function() {
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = value.match(regExp);
var arrCheckbox = ;
for (var i = 0; i < matches.length; i++) {
var str = matches[i];
arrCheckbox.push(str);
// set the names in the array
const names = arrCheckbox
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
})
Basically trying to pass array to names dynamically, method sets values require to build names.
https://jsfiddle.net/bernlt/aj6apozq/114/
javascript vue.js
javascript vue.js
edited Nov 19 at 20:00


ssc-hrep3
5,13321553
5,13321553
asked Nov 19 at 18:59
bernlt
1715
1715
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
accepted
You have several things you need to correct.
You called the methods attribute
method
.You don't call the
setCheckbox()
while initializing the component. You need to do this in eithercreated()
ormounted()
. If you want to dynamically watch the example string, you would also have to watch this string and the reset thenames
array.You don't save the names effectively. You just create a constant with the name
names
but you must assign it to the vue component (this
).You pushed a string into the array (the name), but you actually wanted to push an object containing a string and a boolean.
You could improve your code, if you split the logic of the loop and extracting the string from the checkbox view. You can do this by creating a component for the checkbox which is looped over multiple times. Then, you'd have a single state in a checkbox component with a name and a boolean.
The first four things should be fixed now in this snippet (I did not run it):
<div id='example-3'>
<div v-for="(item, index) in names" :key="index">
<input type="checkbox" :id="item.name" v-model="item.checked">
<label :for="item.name">{{ item.name }}</label>
</div>
<span>Checked names: {{ checkedNames }}</span>
</div>
I removed the names array that supported the checkbox, in order to pass dynamically the new values.
new Vue({
el: '#example-3',
data() {
return {
names:
}
},
methods: {
setCheckbox() {
this.names = ;
// you might want to extract this string and recall this function after it changes
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = value.match(regExp);
for (var i = 0; i < matches.length; i++) {
this.names.push({
name: matches[i],
checked: false
});
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
created() {
this.setCheckbox();
}
})
add a comment |
up vote
0
down vote
Several issues.
- you're not calling setCheckbox()
- several errors.
Here's something closer to what you're looking for:
new Vue({
el: '#example-3',
data() {
return {
names:
}
},
methods: {
setCheckbox: function() {
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = txt.match(regExp);
var arrCheckbox = ;
console.log(matches);
for (var i = 0; i < matches.length; i++) {
var str = matches[i].replace(/{(.*)}/, '$1');
this.names.push({name: str, checked: false});
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
mounted() {
this.setCheckbox();
}
})
Here's a working fiddle: https://jsfiddle.net/jmbldwn/3Lrbw56s/8/
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
You have several things you need to correct.
You called the methods attribute
method
.You don't call the
setCheckbox()
while initializing the component. You need to do this in eithercreated()
ormounted()
. If you want to dynamically watch the example string, you would also have to watch this string and the reset thenames
array.You don't save the names effectively. You just create a constant with the name
names
but you must assign it to the vue component (this
).You pushed a string into the array (the name), but you actually wanted to push an object containing a string and a boolean.
You could improve your code, if you split the logic of the loop and extracting the string from the checkbox view. You can do this by creating a component for the checkbox which is looped over multiple times. Then, you'd have a single state in a checkbox component with a name and a boolean.
The first four things should be fixed now in this snippet (I did not run it):
<div id='example-3'>
<div v-for="(item, index) in names" :key="index">
<input type="checkbox" :id="item.name" v-model="item.checked">
<label :for="item.name">{{ item.name }}</label>
</div>
<span>Checked names: {{ checkedNames }}</span>
</div>
I removed the names array that supported the checkbox, in order to pass dynamically the new values.
new Vue({
el: '#example-3',
data() {
return {
names:
}
},
methods: {
setCheckbox() {
this.names = ;
// you might want to extract this string and recall this function after it changes
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = value.match(regExp);
for (var i = 0; i < matches.length; i++) {
this.names.push({
name: matches[i],
checked: false
});
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
created() {
this.setCheckbox();
}
})
add a comment |
up vote
0
down vote
accepted
You have several things you need to correct.
You called the methods attribute
method
.You don't call the
setCheckbox()
while initializing the component. You need to do this in eithercreated()
ormounted()
. If you want to dynamically watch the example string, you would also have to watch this string and the reset thenames
array.You don't save the names effectively. You just create a constant with the name
names
but you must assign it to the vue component (this
).You pushed a string into the array (the name), but you actually wanted to push an object containing a string and a boolean.
You could improve your code, if you split the logic of the loop and extracting the string from the checkbox view. You can do this by creating a component for the checkbox which is looped over multiple times. Then, you'd have a single state in a checkbox component with a name and a boolean.
The first four things should be fixed now in this snippet (I did not run it):
<div id='example-3'>
<div v-for="(item, index) in names" :key="index">
<input type="checkbox" :id="item.name" v-model="item.checked">
<label :for="item.name">{{ item.name }}</label>
</div>
<span>Checked names: {{ checkedNames }}</span>
</div>
I removed the names array that supported the checkbox, in order to pass dynamically the new values.
new Vue({
el: '#example-3',
data() {
return {
names:
}
},
methods: {
setCheckbox() {
this.names = ;
// you might want to extract this string and recall this function after it changes
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = value.match(regExp);
for (var i = 0; i < matches.length; i++) {
this.names.push({
name: matches[i],
checked: false
});
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
created() {
this.setCheckbox();
}
})
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
You have several things you need to correct.
You called the methods attribute
method
.You don't call the
setCheckbox()
while initializing the component. You need to do this in eithercreated()
ormounted()
. If you want to dynamically watch the example string, you would also have to watch this string and the reset thenames
array.You don't save the names effectively. You just create a constant with the name
names
but you must assign it to the vue component (this
).You pushed a string into the array (the name), but you actually wanted to push an object containing a string and a boolean.
You could improve your code, if you split the logic of the loop and extracting the string from the checkbox view. You can do this by creating a component for the checkbox which is looped over multiple times. Then, you'd have a single state in a checkbox component with a name and a boolean.
The first four things should be fixed now in this snippet (I did not run it):
<div id='example-3'>
<div v-for="(item, index) in names" :key="index">
<input type="checkbox" :id="item.name" v-model="item.checked">
<label :for="item.name">{{ item.name }}</label>
</div>
<span>Checked names: {{ checkedNames }}</span>
</div>
I removed the names array that supported the checkbox, in order to pass dynamically the new values.
new Vue({
el: '#example-3',
data() {
return {
names:
}
},
methods: {
setCheckbox() {
this.names = ;
// you might want to extract this string and recall this function after it changes
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = value.match(regExp);
for (var i = 0; i < matches.length; i++) {
this.names.push({
name: matches[i],
checked: false
});
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
created() {
this.setCheckbox();
}
})
You have several things you need to correct.
You called the methods attribute
method
.You don't call the
setCheckbox()
while initializing the component. You need to do this in eithercreated()
ormounted()
. If you want to dynamically watch the example string, you would also have to watch this string and the reset thenames
array.You don't save the names effectively. You just create a constant with the name
names
but you must assign it to the vue component (this
).You pushed a string into the array (the name), but you actually wanted to push an object containing a string and a boolean.
You could improve your code, if you split the logic of the loop and extracting the string from the checkbox view. You can do this by creating a component for the checkbox which is looped over multiple times. Then, you'd have a single state in a checkbox component with a name and a boolean.
The first four things should be fixed now in this snippet (I did not run it):
<div id='example-3'>
<div v-for="(item, index) in names" :key="index">
<input type="checkbox" :id="item.name" v-model="item.checked">
<label :for="item.name">{{ item.name }}</label>
</div>
<span>Checked names: {{ checkedNames }}</span>
</div>
I removed the names array that supported the checkbox, in order to pass dynamically the new values.
new Vue({
el: '#example-3',
data() {
return {
names:
}
},
methods: {
setCheckbox() {
this.names = ;
// you might want to extract this string and recall this function after it changes
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = value.match(regExp);
for (var i = 0; i < matches.length; i++) {
this.names.push({
name: matches[i],
checked: false
});
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
created() {
this.setCheckbox();
}
})
answered Nov 19 at 20:17


ssc-hrep3
5,13321553
5,13321553
add a comment |
add a comment |
up vote
0
down vote
Several issues.
- you're not calling setCheckbox()
- several errors.
Here's something closer to what you're looking for:
new Vue({
el: '#example-3',
data() {
return {
names:
}
},
methods: {
setCheckbox: function() {
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = txt.match(regExp);
var arrCheckbox = ;
console.log(matches);
for (var i = 0; i < matches.length; i++) {
var str = matches[i].replace(/{(.*)}/, '$1');
this.names.push({name: str, checked: false});
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
mounted() {
this.setCheckbox();
}
})
Here's a working fiddle: https://jsfiddle.net/jmbldwn/3Lrbw56s/8/
add a comment |
up vote
0
down vote
Several issues.
- you're not calling setCheckbox()
- several errors.
Here's something closer to what you're looking for:
new Vue({
el: '#example-3',
data() {
return {
names:
}
},
methods: {
setCheckbox: function() {
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = txt.match(regExp);
var arrCheckbox = ;
console.log(matches);
for (var i = 0; i < matches.length; i++) {
var str = matches[i].replace(/{(.*)}/, '$1');
this.names.push({name: str, checked: false});
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
mounted() {
this.setCheckbox();
}
})
Here's a working fiddle: https://jsfiddle.net/jmbldwn/3Lrbw56s/8/
add a comment |
up vote
0
down vote
up vote
0
down vote
Several issues.
- you're not calling setCheckbox()
- several errors.
Here's something closer to what you're looking for:
new Vue({
el: '#example-3',
data() {
return {
names:
}
},
methods: {
setCheckbox: function() {
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = txt.match(regExp);
var arrCheckbox = ;
console.log(matches);
for (var i = 0; i < matches.length; i++) {
var str = matches[i].replace(/{(.*)}/, '$1');
this.names.push({name: str, checked: false});
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
mounted() {
this.setCheckbox();
}
})
Here's a working fiddle: https://jsfiddle.net/jmbldwn/3Lrbw56s/8/
Several issues.
- you're not calling setCheckbox()
- several errors.
Here's something closer to what you're looking for:
new Vue({
el: '#example-3',
data() {
return {
names:
}
},
methods: {
setCheckbox: function() {
var txt = "These arethe names for checkbox {Rob} is 20, {Carlos} is 22 and {Mike} is 19."
var regExp = /{([^}]*)}/g;
var matches = txt.match(regExp);
var arrCheckbox = ;
console.log(matches);
for (var i = 0; i < matches.length; i++) {
var str = matches[i].replace(/{(.*)}/, '$1');
this.names.push({name: str, checked: false});
}
}
},
computed: {
checkedNames() {
return this.names.filter(item => item.checked).map(name => name.name)
}
},
mounted() {
this.setCheckbox();
}
})
Here's a working fiddle: https://jsfiddle.net/jmbldwn/3Lrbw56s/8/
answered Nov 19 at 20:20
Jim B.
2,4261928
2,4261928
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53381014%2fvuejs-build-checkbox-dynamically-from-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BnwDrIs,0P,QGtL,IKvwlMr473CyQYCyEn b1EbQvN,jOVkdn,UErH0,SSeWwGkFetbJ42nY Jm,3vsfJ6hM