How to test DOM methods in embed js script library?
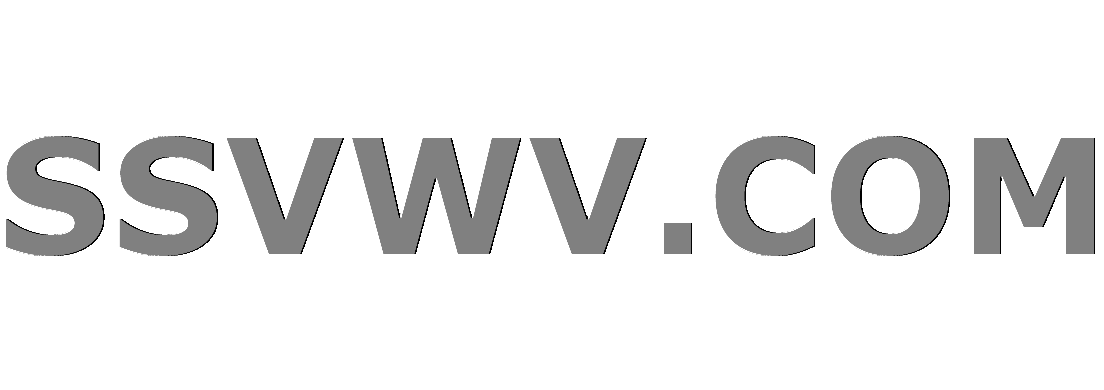
Multi tool use
up vote
2
down vote
favorite
I am working in a Javascript library to embed in the browser.
The thing is that I was started the project from https://github.com/krasimir/webpack-library-starter and I would to test my library but I have a problem with the DOM
because my proyect hasn't got html so, I don't know how I should to test the DOM
methods.
The library is to embed in to our clients websites, the idea is to make a embed script like google maps, analytics sdk, etc.
I am using chai
and mocha
to test but maybe I should to change to ava
or something like.
I know that in React for example you have tools to simulate the DOM
but it is in vanilla JS so... help please.
Example of my library class:
export class MyClass {
constructor(htmlElement) {
this.clientWebsiteHTMLElement = htmlElement;
this.addChild();
}
addChild() {
let child = document.createElement('div');
this.clientWebsiteHTMLElement.appendChild(child);
}
}
Example of my-lib.js
import { MyClass } from './my-class.js'
if (window && document) {
(function (window, document){
let container = document.getElementById('container');
let myClass = new MyClass(container);
window.myLib = myClass;
})(window, document)
}
Example of entry point (In to the client website, is not in the same project):
<html>
<head></head>
<body>
<div id="container"></div>
<script src="http://myserver.com/to/serve/embed/scripts/myLib-bundle.js"></script>
</body>
<html>
Really is more complex than this, but the solution are the same.
How I can test that for example?
Thanks! :D
javascript unit-testing testing dom embedded-resource
add a comment |
up vote
2
down vote
favorite
I am working in a Javascript library to embed in the browser.
The thing is that I was started the project from https://github.com/krasimir/webpack-library-starter and I would to test my library but I have a problem with the DOM
because my proyect hasn't got html so, I don't know how I should to test the DOM
methods.
The library is to embed in to our clients websites, the idea is to make a embed script like google maps, analytics sdk, etc.
I am using chai
and mocha
to test but maybe I should to change to ava
or something like.
I know that in React for example you have tools to simulate the DOM
but it is in vanilla JS so... help please.
Example of my library class:
export class MyClass {
constructor(htmlElement) {
this.clientWebsiteHTMLElement = htmlElement;
this.addChild();
}
addChild() {
let child = document.createElement('div');
this.clientWebsiteHTMLElement.appendChild(child);
}
}
Example of my-lib.js
import { MyClass } from './my-class.js'
if (window && document) {
(function (window, document){
let container = document.getElementById('container');
let myClass = new MyClass(container);
window.myLib = myClass;
})(window, document)
}
Example of entry point (In to the client website, is not in the same project):
<html>
<head></head>
<body>
<div id="container"></div>
<script src="http://myserver.com/to/serve/embed/scripts/myLib-bundle.js"></script>
</body>
<html>
Really is more complex than this, but the solution are the same.
How I can test that for example?
Thanks! :D
javascript unit-testing testing dom embedded-resource
The most commonly used tool for this is JSDOM: github.com/jsdom/jsdom
– Patrick Hund
Nov 19 at 19:50
Oh great, can you write a more specific example to test this?(Using with chai or whatever testing suite)
– Rubén Soler
Nov 20 at 5:38
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I am working in a Javascript library to embed in the browser.
The thing is that I was started the project from https://github.com/krasimir/webpack-library-starter and I would to test my library but I have a problem with the DOM
because my proyect hasn't got html so, I don't know how I should to test the DOM
methods.
The library is to embed in to our clients websites, the idea is to make a embed script like google maps, analytics sdk, etc.
I am using chai
and mocha
to test but maybe I should to change to ava
or something like.
I know that in React for example you have tools to simulate the DOM
but it is in vanilla JS so... help please.
Example of my library class:
export class MyClass {
constructor(htmlElement) {
this.clientWebsiteHTMLElement = htmlElement;
this.addChild();
}
addChild() {
let child = document.createElement('div');
this.clientWebsiteHTMLElement.appendChild(child);
}
}
Example of my-lib.js
import { MyClass } from './my-class.js'
if (window && document) {
(function (window, document){
let container = document.getElementById('container');
let myClass = new MyClass(container);
window.myLib = myClass;
})(window, document)
}
Example of entry point (In to the client website, is not in the same project):
<html>
<head></head>
<body>
<div id="container"></div>
<script src="http://myserver.com/to/serve/embed/scripts/myLib-bundle.js"></script>
</body>
<html>
Really is more complex than this, but the solution are the same.
How I can test that for example?
Thanks! :D
javascript unit-testing testing dom embedded-resource
I am working in a Javascript library to embed in the browser.
The thing is that I was started the project from https://github.com/krasimir/webpack-library-starter and I would to test my library but I have a problem with the DOM
because my proyect hasn't got html so, I don't know how I should to test the DOM
methods.
The library is to embed in to our clients websites, the idea is to make a embed script like google maps, analytics sdk, etc.
I am using chai
and mocha
to test but maybe I should to change to ava
or something like.
I know that in React for example you have tools to simulate the DOM
but it is in vanilla JS so... help please.
Example of my library class:
export class MyClass {
constructor(htmlElement) {
this.clientWebsiteHTMLElement = htmlElement;
this.addChild();
}
addChild() {
let child = document.createElement('div');
this.clientWebsiteHTMLElement.appendChild(child);
}
}
Example of my-lib.js
import { MyClass } from './my-class.js'
if (window && document) {
(function (window, document){
let container = document.getElementById('container');
let myClass = new MyClass(container);
window.myLib = myClass;
})(window, document)
}
Example of entry point (In to the client website, is not in the same project):
<html>
<head></head>
<body>
<div id="container"></div>
<script src="http://myserver.com/to/serve/embed/scripts/myLib-bundle.js"></script>
</body>
<html>
Really is more complex than this, but the solution are the same.
How I can test that for example?
Thanks! :D
javascript unit-testing testing dom embedded-resource
javascript unit-testing testing dom embedded-resource
edited Nov 19 at 19:31
asked Nov 19 at 19:01


Rubén Soler
916217
916217
The most commonly used tool for this is JSDOM: github.com/jsdom/jsdom
– Patrick Hund
Nov 19 at 19:50
Oh great, can you write a more specific example to test this?(Using with chai or whatever testing suite)
– Rubén Soler
Nov 20 at 5:38
add a comment |
The most commonly used tool for this is JSDOM: github.com/jsdom/jsdom
– Patrick Hund
Nov 19 at 19:50
Oh great, can you write a more specific example to test this?(Using with chai or whatever testing suite)
– Rubén Soler
Nov 20 at 5:38
The most commonly used tool for this is JSDOM: github.com/jsdom/jsdom
– Patrick Hund
Nov 19 at 19:50
The most commonly used tool for this is JSDOM: github.com/jsdom/jsdom
– Patrick Hund
Nov 19 at 19:50
Oh great, can you write a more specific example to test this?(Using with chai or whatever testing suite)
– Rubén Soler
Nov 20 at 5:38
Oh great, can you write a more specific example to test this?(Using with chai or whatever testing suite)
– Rubén Soler
Nov 20 at 5:38
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
The problem is that Mocha tests are executed with Node.js, meaning by default, there is no browser available that can provide a DOM that provides APIs like document or window.
A popular tool to provide a DOM for these tests is JSDOM.
Here's a simple example how you could test your class MyClass
:
import { MyClass } from '../modules/MyClass';
import { JSDOM } from 'jsdom';
const dom = new JSDOM(
'<!DOCTYPE html><html><head></head><body><div id="root"></div></body></html>'
);
global.document = dom.window.document;
describe('My class', () => {
it('adds an element to the HTML element passed to its constructur', () => {
const htmlElement = document.getElementById('root');
const myClass = new MyClass(htmlElement);
expect(htmlElement.children.length).toBe(1);
});
});
Note: Since you're already considering switching your testing library, may I suggest switching to Jest? It comes with JSDom pre-installed.
Great, very thanks! :)
– Rubén Soler
Nov 20 at 9:54
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
The problem is that Mocha tests are executed with Node.js, meaning by default, there is no browser available that can provide a DOM that provides APIs like document or window.
A popular tool to provide a DOM for these tests is JSDOM.
Here's a simple example how you could test your class MyClass
:
import { MyClass } from '../modules/MyClass';
import { JSDOM } from 'jsdom';
const dom = new JSDOM(
'<!DOCTYPE html><html><head></head><body><div id="root"></div></body></html>'
);
global.document = dom.window.document;
describe('My class', () => {
it('adds an element to the HTML element passed to its constructur', () => {
const htmlElement = document.getElementById('root');
const myClass = new MyClass(htmlElement);
expect(htmlElement.children.length).toBe(1);
});
});
Note: Since you're already considering switching your testing library, may I suggest switching to Jest? It comes with JSDom pre-installed.
Great, very thanks! :)
– Rubén Soler
Nov 20 at 9:54
add a comment |
up vote
1
down vote
accepted
The problem is that Mocha tests are executed with Node.js, meaning by default, there is no browser available that can provide a DOM that provides APIs like document or window.
A popular tool to provide a DOM for these tests is JSDOM.
Here's a simple example how you could test your class MyClass
:
import { MyClass } from '../modules/MyClass';
import { JSDOM } from 'jsdom';
const dom = new JSDOM(
'<!DOCTYPE html><html><head></head><body><div id="root"></div></body></html>'
);
global.document = dom.window.document;
describe('My class', () => {
it('adds an element to the HTML element passed to its constructur', () => {
const htmlElement = document.getElementById('root');
const myClass = new MyClass(htmlElement);
expect(htmlElement.children.length).toBe(1);
});
});
Note: Since you're already considering switching your testing library, may I suggest switching to Jest? It comes with JSDom pre-installed.
Great, very thanks! :)
– Rubén Soler
Nov 20 at 9:54
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
The problem is that Mocha tests are executed with Node.js, meaning by default, there is no browser available that can provide a DOM that provides APIs like document or window.
A popular tool to provide a DOM for these tests is JSDOM.
Here's a simple example how you could test your class MyClass
:
import { MyClass } from '../modules/MyClass';
import { JSDOM } from 'jsdom';
const dom = new JSDOM(
'<!DOCTYPE html><html><head></head><body><div id="root"></div></body></html>'
);
global.document = dom.window.document;
describe('My class', () => {
it('adds an element to the HTML element passed to its constructur', () => {
const htmlElement = document.getElementById('root');
const myClass = new MyClass(htmlElement);
expect(htmlElement.children.length).toBe(1);
});
});
Note: Since you're already considering switching your testing library, may I suggest switching to Jest? It comes with JSDom pre-installed.
The problem is that Mocha tests are executed with Node.js, meaning by default, there is no browser available that can provide a DOM that provides APIs like document or window.
A popular tool to provide a DOM for these tests is JSDOM.
Here's a simple example how you could test your class MyClass
:
import { MyClass } from '../modules/MyClass';
import { JSDOM } from 'jsdom';
const dom = new JSDOM(
'<!DOCTYPE html><html><head></head><body><div id="root"></div></body></html>'
);
global.document = dom.window.document;
describe('My class', () => {
it('adds an element to the HTML element passed to its constructur', () => {
const htmlElement = document.getElementById('root');
const myClass = new MyClass(htmlElement);
expect(htmlElement.children.length).toBe(1);
});
});
Note: Since you're already considering switching your testing library, may I suggest switching to Jest? It comes with JSDom pre-installed.
answered Nov 20 at 9:43
Patrick Hund
6,69852147
6,69852147
Great, very thanks! :)
– Rubén Soler
Nov 20 at 9:54
add a comment |
Great, very thanks! :)
– Rubén Soler
Nov 20 at 9:54
Great, very thanks! :)
– Rubén Soler
Nov 20 at 9:54
Great, very thanks! :)
– Rubén Soler
Nov 20 at 9:54
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53381039%2fhow-to-test-dom-methods-in-embed-js-script-library%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VPFiSMKGKI4NhyxKG11o,y4126GIau4
The most commonly used tool for this is JSDOM: github.com/jsdom/jsdom
– Patrick Hund
Nov 19 at 19:50
Oh great, can you write a more specific example to test this?(Using with chai or whatever testing suite)
– Rubén Soler
Nov 20 at 5:38