Toggling an array of filters
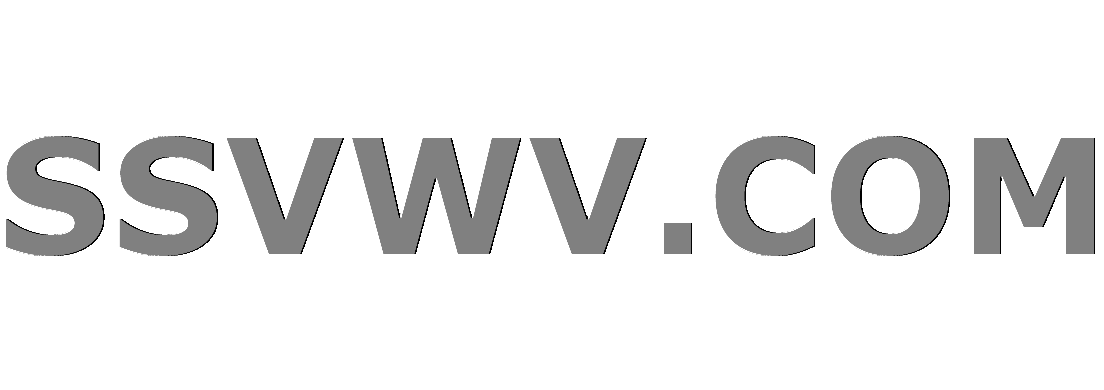
Multi tool use
up vote
1
down vote
favorite
What follows is a piece of code that essentially toggles an array of filters (if the filter doesnt exist it adds it, if it does, it removes it).
What would you suggest is the best way to write the following imperative approach declaratively?
var selectedFilters = [ {name:"SomeName"} , ... ]
var inputFilter = {name:"OtherName"};
var indexFound = -1;
for (let i = 0; i < selectedFilters.length; i++) {
if (selectedFilters[i].name === inputFilter.name) {
indexFound = i;
}
}
if (indexFound != -1) {
selectedFilters.splice(indexFound, 1);
} else {
selectedFilters.push(inputFilter);
}
An idea would be to use filter first to weed out the item if it exists by name, then if the resulting array is equal to the original, push. But it still doesnt feel right.
javascript
bumped to the homepage by Community♦ 14 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
add a comment |
up vote
1
down vote
favorite
What follows is a piece of code that essentially toggles an array of filters (if the filter doesnt exist it adds it, if it does, it removes it).
What would you suggest is the best way to write the following imperative approach declaratively?
var selectedFilters = [ {name:"SomeName"} , ... ]
var inputFilter = {name:"OtherName"};
var indexFound = -1;
for (let i = 0; i < selectedFilters.length; i++) {
if (selectedFilters[i].name === inputFilter.name) {
indexFound = i;
}
}
if (indexFound != -1) {
selectedFilters.splice(indexFound, 1);
} else {
selectedFilters.push(inputFilter);
}
An idea would be to use filter first to weed out the item if it exists by name, then if the resulting array is equal to the original, push. But it still doesnt feel right.
javascript
bumped to the homepage by Community♦ 14 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
What follows is a piece of code that essentially toggles an array of filters (if the filter doesnt exist it adds it, if it does, it removes it).
What would you suggest is the best way to write the following imperative approach declaratively?
var selectedFilters = [ {name:"SomeName"} , ... ]
var inputFilter = {name:"OtherName"};
var indexFound = -1;
for (let i = 0; i < selectedFilters.length; i++) {
if (selectedFilters[i].name === inputFilter.name) {
indexFound = i;
}
}
if (indexFound != -1) {
selectedFilters.splice(indexFound, 1);
} else {
selectedFilters.push(inputFilter);
}
An idea would be to use filter first to weed out the item if it exists by name, then if the resulting array is equal to the original, push. But it still doesnt feel right.
javascript
What follows is a piece of code that essentially toggles an array of filters (if the filter doesnt exist it adds it, if it does, it removes it).
What would you suggest is the best way to write the following imperative approach declaratively?
var selectedFilters = [ {name:"SomeName"} , ... ]
var inputFilter = {name:"OtherName"};
var indexFound = -1;
for (let i = 0; i < selectedFilters.length; i++) {
if (selectedFilters[i].name === inputFilter.name) {
indexFound = i;
}
}
if (indexFound != -1) {
selectedFilters.splice(indexFound, 1);
} else {
selectedFilters.push(inputFilter);
}
An idea would be to use filter first to weed out the item if it exists by name, then if the resulting array is equal to the original, push. But it still doesnt feel right.
javascript
javascript
edited Mar 27 at 15:10


200_success
127k15148411
127k15148411
asked Mar 27 at 11:16
George Avgoustis
1062
1062
bumped to the homepage by Community♦ 14 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
bumped to the homepage by Community♦ 14 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
up vote
0
down vote
Why not just filter with selectedFilters.filter(predName(inputFilter))
with a predicate predName = ({name: filterName}) => ({name}) => name === filterName
.
add a comment |
up vote
0
down vote
Pure V State
There are two ways you can do this.
Pure
The first functional pure method first copies the array, then checks if the item to toggle exists then depending on that result adds or removes the item. Making sure that the added item is a copy, not a reference. It has no side effects but requires additional memory and CPU cycles.
const toggleItem = (itemDesc, items, prop = "name") => {
items = [...items];
const index = items.findIndex(item => itemDesc[prop] === item[prop]);
index > -1 ? items.splice(index, 1) : items.push({...itemDesc});
return items;
}
State
The second does not create a new array and keeps all references It is "functionally" impure and ensures that the changed state is available to all references to the original. It is considerably quicker and uses less memory.
const toggleItem = (itemDesc, items, prop = "name") => {
const index = items.findIndex(item => itemDesc[prop] === item[prop]);
index > -1 ? items.splice(index, 1) : items.push(itemDesc);
return items;
}
What's the point initems = [...items]
? Making arrays generally immutable and each time producing a new sequence? Shouldn't it be implemented in a more structure-sharing manner?
– bipll
Mar 27 at 13:38
@bipll The array needs to be copied to prevent side effects and keep the function pure. I do not code like that (its crazily inefficient and makes coding so much harder) but for many functional is the bee's knees and it is a requirement of the functional programming paradigm.
– Blindman67
Mar 27 at 14:09
add a comment |
up vote
0
down vote
Here is a functional version. Javascript has quite a lot functional type functions that can help you out here. It could probably made to be even nicer, but this was my first approach
function updateFilters(currentFilters, newFilter) {
const hasName = filter => filter.name === newFilter.name;
const foundIndex = currentFilters.findIndex(hasName);
return foundIndex === -1
? currentFilters.concat([newFilter])
: currentFilters.splice(foundIndex, 1);
}
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Why not just filter with selectedFilters.filter(predName(inputFilter))
with a predicate predName = ({name: filterName}) => ({name}) => name === filterName
.
add a comment |
up vote
0
down vote
Why not just filter with selectedFilters.filter(predName(inputFilter))
with a predicate predName = ({name: filterName}) => ({name}) => name === filterName
.
add a comment |
up vote
0
down vote
up vote
0
down vote
Why not just filter with selectedFilters.filter(predName(inputFilter))
with a predicate predName = ({name: filterName}) => ({name}) => name === filterName
.
Why not just filter with selectedFilters.filter(predName(inputFilter))
with a predicate predName = ({name: filterName}) => ({name}) => name === filterName
.
answered Mar 27 at 12:43
morbusg
21636
21636
add a comment |
add a comment |
up vote
0
down vote
Pure V State
There are two ways you can do this.
Pure
The first functional pure method first copies the array, then checks if the item to toggle exists then depending on that result adds or removes the item. Making sure that the added item is a copy, not a reference. It has no side effects but requires additional memory and CPU cycles.
const toggleItem = (itemDesc, items, prop = "name") => {
items = [...items];
const index = items.findIndex(item => itemDesc[prop] === item[prop]);
index > -1 ? items.splice(index, 1) : items.push({...itemDesc});
return items;
}
State
The second does not create a new array and keeps all references It is "functionally" impure and ensures that the changed state is available to all references to the original. It is considerably quicker and uses less memory.
const toggleItem = (itemDesc, items, prop = "name") => {
const index = items.findIndex(item => itemDesc[prop] === item[prop]);
index > -1 ? items.splice(index, 1) : items.push(itemDesc);
return items;
}
What's the point initems = [...items]
? Making arrays generally immutable and each time producing a new sequence? Shouldn't it be implemented in a more structure-sharing manner?
– bipll
Mar 27 at 13:38
@bipll The array needs to be copied to prevent side effects and keep the function pure. I do not code like that (its crazily inefficient and makes coding so much harder) but for many functional is the bee's knees and it is a requirement of the functional programming paradigm.
– Blindman67
Mar 27 at 14:09
add a comment |
up vote
0
down vote
Pure V State
There are two ways you can do this.
Pure
The first functional pure method first copies the array, then checks if the item to toggle exists then depending on that result adds or removes the item. Making sure that the added item is a copy, not a reference. It has no side effects but requires additional memory and CPU cycles.
const toggleItem = (itemDesc, items, prop = "name") => {
items = [...items];
const index = items.findIndex(item => itemDesc[prop] === item[prop]);
index > -1 ? items.splice(index, 1) : items.push({...itemDesc});
return items;
}
State
The second does not create a new array and keeps all references It is "functionally" impure and ensures that the changed state is available to all references to the original. It is considerably quicker and uses less memory.
const toggleItem = (itemDesc, items, prop = "name") => {
const index = items.findIndex(item => itemDesc[prop] === item[prop]);
index > -1 ? items.splice(index, 1) : items.push(itemDesc);
return items;
}
What's the point initems = [...items]
? Making arrays generally immutable and each time producing a new sequence? Shouldn't it be implemented in a more structure-sharing manner?
– bipll
Mar 27 at 13:38
@bipll The array needs to be copied to prevent side effects and keep the function pure. I do not code like that (its crazily inefficient and makes coding so much harder) but for many functional is the bee's knees and it is a requirement of the functional programming paradigm.
– Blindman67
Mar 27 at 14:09
add a comment |
up vote
0
down vote
up vote
0
down vote
Pure V State
There are two ways you can do this.
Pure
The first functional pure method first copies the array, then checks if the item to toggle exists then depending on that result adds or removes the item. Making sure that the added item is a copy, not a reference. It has no side effects but requires additional memory and CPU cycles.
const toggleItem = (itemDesc, items, prop = "name") => {
items = [...items];
const index = items.findIndex(item => itemDesc[prop] === item[prop]);
index > -1 ? items.splice(index, 1) : items.push({...itemDesc});
return items;
}
State
The second does not create a new array and keeps all references It is "functionally" impure and ensures that the changed state is available to all references to the original. It is considerably quicker and uses less memory.
const toggleItem = (itemDesc, items, prop = "name") => {
const index = items.findIndex(item => itemDesc[prop] === item[prop]);
index > -1 ? items.splice(index, 1) : items.push(itemDesc);
return items;
}
Pure V State
There are two ways you can do this.
Pure
The first functional pure method first copies the array, then checks if the item to toggle exists then depending on that result adds or removes the item. Making sure that the added item is a copy, not a reference. It has no side effects but requires additional memory and CPU cycles.
const toggleItem = (itemDesc, items, prop = "name") => {
items = [...items];
const index = items.findIndex(item => itemDesc[prop] === item[prop]);
index > -1 ? items.splice(index, 1) : items.push({...itemDesc});
return items;
}
State
The second does not create a new array and keeps all references It is "functionally" impure and ensures that the changed state is available to all references to the original. It is considerably quicker and uses less memory.
const toggleItem = (itemDesc, items, prop = "name") => {
const index = items.findIndex(item => itemDesc[prop] === item[prop]);
index > -1 ? items.splice(index, 1) : items.push(itemDesc);
return items;
}
answered Mar 27 at 13:27


Blindman67
6,5241521
6,5241521
What's the point initems = [...items]
? Making arrays generally immutable and each time producing a new sequence? Shouldn't it be implemented in a more structure-sharing manner?
– bipll
Mar 27 at 13:38
@bipll The array needs to be copied to prevent side effects and keep the function pure. I do not code like that (its crazily inefficient and makes coding so much harder) but for many functional is the bee's knees and it is a requirement of the functional programming paradigm.
– Blindman67
Mar 27 at 14:09
add a comment |
What's the point initems = [...items]
? Making arrays generally immutable and each time producing a new sequence? Shouldn't it be implemented in a more structure-sharing manner?
– bipll
Mar 27 at 13:38
@bipll The array needs to be copied to prevent side effects and keep the function pure. I do not code like that (its crazily inefficient and makes coding so much harder) but for many functional is the bee's knees and it is a requirement of the functional programming paradigm.
– Blindman67
Mar 27 at 14:09
What's the point in
items = [...items]
? Making arrays generally immutable and each time producing a new sequence? Shouldn't it be implemented in a more structure-sharing manner?– bipll
Mar 27 at 13:38
What's the point in
items = [...items]
? Making arrays generally immutable and each time producing a new sequence? Shouldn't it be implemented in a more structure-sharing manner?– bipll
Mar 27 at 13:38
@bipll The array needs to be copied to prevent side effects and keep the function pure. I do not code like that (its crazily inefficient and makes coding so much harder) but for many functional is the bee's knees and it is a requirement of the functional programming paradigm.
– Blindman67
Mar 27 at 14:09
@bipll The array needs to be copied to prevent side effects and keep the function pure. I do not code like that (its crazily inefficient and makes coding so much harder) but for many functional is the bee's knees and it is a requirement of the functional programming paradigm.
– Blindman67
Mar 27 at 14:09
add a comment |
up vote
0
down vote
Here is a functional version. Javascript has quite a lot functional type functions that can help you out here. It could probably made to be even nicer, but this was my first approach
function updateFilters(currentFilters, newFilter) {
const hasName = filter => filter.name === newFilter.name;
const foundIndex = currentFilters.findIndex(hasName);
return foundIndex === -1
? currentFilters.concat([newFilter])
: currentFilters.splice(foundIndex, 1);
}
add a comment |
up vote
0
down vote
Here is a functional version. Javascript has quite a lot functional type functions that can help you out here. It could probably made to be even nicer, but this was my first approach
function updateFilters(currentFilters, newFilter) {
const hasName = filter => filter.name === newFilter.name;
const foundIndex = currentFilters.findIndex(hasName);
return foundIndex === -1
? currentFilters.concat([newFilter])
: currentFilters.splice(foundIndex, 1);
}
add a comment |
up vote
0
down vote
up vote
0
down vote
Here is a functional version. Javascript has quite a lot functional type functions that can help you out here. It could probably made to be even nicer, but this was my first approach
function updateFilters(currentFilters, newFilter) {
const hasName = filter => filter.name === newFilter.name;
const foundIndex = currentFilters.findIndex(hasName);
return foundIndex === -1
? currentFilters.concat([newFilter])
: currentFilters.splice(foundIndex, 1);
}
Here is a functional version. Javascript has quite a lot functional type functions that can help you out here. It could probably made to be even nicer, but this was my first approach
function updateFilters(currentFilters, newFilter) {
const hasName = filter => filter.name === newFilter.name;
const foundIndex = currentFilters.findIndex(hasName);
return foundIndex === -1
? currentFilters.concat([newFilter])
: currentFilters.splice(foundIndex, 1);
}
edited Mar 27 at 16:10


Sᴀᴍ Onᴇᴌᴀ
7,73061748
7,73061748
answered Mar 27 at 11:38
August
1658
1658
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f190572%2ftoggling-an-array-of-filters%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LLUzouXjJsV9X5 189fmLRhRGqhTPXDU4KKFzeRs2eX7HLe lfBBh2CGzQrXDKzTs4zLQ2spuLE AX,7CP