Request for input on bash script loop
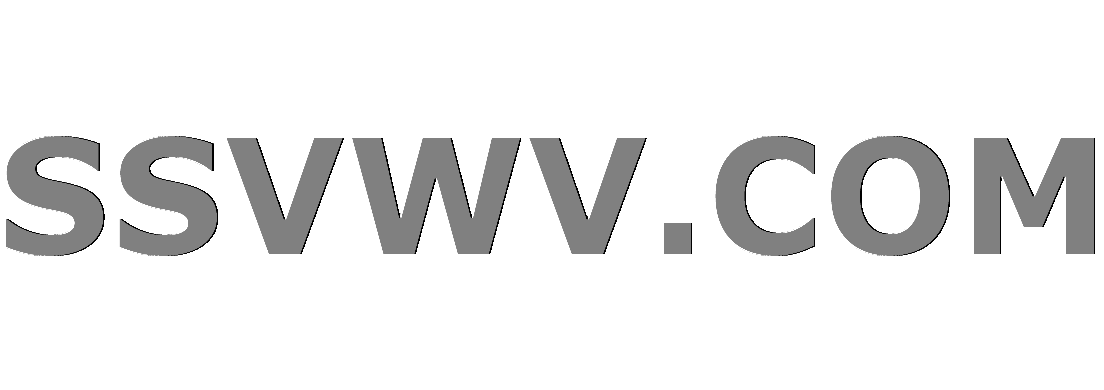
Multi tool use
up vote
0
down vote
favorite
I am trying to run jpegoptim against pictures and at some point in the loop...after let's say 200 iterations, i get "stdin", which requires input to go further.
Is there a way to force the input?
#!/bin/bash
for i in `find . -name "*.jpg" -type f`; do
jpegoptim "$i" >> jpg.log; done
done
bash jpegoptim
add a comment |
up vote
0
down vote
favorite
I am trying to run jpegoptim against pictures and at some point in the loop...after let's say 200 iterations, i get "stdin", which requires input to go further.
Is there a way to force the input?
#!/bin/bash
for i in `find . -name "*.jpg" -type f`; do
jpegoptim "$i" >> jpg.log; done
done
bash jpegoptim
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am trying to run jpegoptim against pictures and at some point in the loop...after let's say 200 iterations, i get "stdin", which requires input to go further.
Is there a way to force the input?
#!/bin/bash
for i in `find . -name "*.jpg" -type f`; do
jpegoptim "$i" >> jpg.log; done
done
bash jpegoptim
I am trying to run jpegoptim against pictures and at some point in the loop...after let's say 200 iterations, i get "stdin", which requires input to go further.
Is there a way to force the input?
#!/bin/bash
for i in `find . -name "*.jpg" -type f`; do
jpegoptim "$i" >> jpg.log; done
done
bash jpegoptim
bash jpegoptim
asked Nov 19 at 14:15
Potney Switters
1,01031939
1,01031939
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
First, don't process dynamically generated file lists in a for
loop.
Second, your code is broken - you have 2 done
's.
Third, my apologies, not familiar with the tool, but I'm inclined to think your issue is in a broader piece of the code than presented.
Fourth, (getting minor and nitpicky :) prefer $(
...)
over backticks. There is very rarely a reason not to do so in bash.
Still, almost none of that is relevant to your question, aside from maybe the third thing... See if this helps -
find . -name "*.jpg" -type f |
while read -r file; do jpegoptim "$file"; done >> jpg.log 2>&1
Or possibly better,
find . -name "*.jpg" -type f | xargs jpegoptim >> jpg.log 2>&1
Update
Benjamin W. points out that these break when filenames have embedded newlines, which is entirely true. I consider filenames with embedded newlines a heinous heresy of the highest order, nut sometimes you don't have control of that, so per his entirely valid suggestion:
find . -name "*.jpg" -type f |
while read -r -d '' file; do jpegoptim "$file"; done >> jpg.log 2>&1
or
find . -name "*.jpg" -type f -print0 | xargs -0 jpegoptim >> jpg.log 2>&1
or best, for simplicity and (therefore) safety
find . -name "*.jpg" -type f -exec jpegoptim {} ; >> jpg.log 2>&1
(Please check my syntax on those...)
Though there are still efficiency considerations if you are processing a large number of files. One should also consider the possibility that the target program may or may not be able to multiprocess command-line arguments. Consider the difference between these:
$: find /tmp -type f -exec echo {} ;
/tmp/.mintty-version
/tmp/AdobeARM.log
/tmp/foo
. . .
$: find /tmp -type f | xargs echo
/tmp/.mintty-version /tmp/AdobeARM.log /tmp/foo ...
1
Both of these break for files with blanks or newlines in their names. Best practice is to use-print0 | xargs -0
, or-print0 | while read -r -d ''
. Or just usefind -exec
instead of either.
– Benjamin W.
Nov 19 at 14:41
add a comment |
up vote
2
down vote
You can use a counter then ask for user input using read
when the counter reaches 200
#!/bin/bash
count=0
for i in `find . -name "*.jpg" -type f`; do
jpegoptim "$i" >> jpg.log
[[ $((count++)) == 200 ]] && read -rp "Continue? [y/n] " resume
[[ "$resume" == 'n' ]] && break
done
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
First, don't process dynamically generated file lists in a for
loop.
Second, your code is broken - you have 2 done
's.
Third, my apologies, not familiar with the tool, but I'm inclined to think your issue is in a broader piece of the code than presented.
Fourth, (getting minor and nitpicky :) prefer $(
...)
over backticks. There is very rarely a reason not to do so in bash.
Still, almost none of that is relevant to your question, aside from maybe the third thing... See if this helps -
find . -name "*.jpg" -type f |
while read -r file; do jpegoptim "$file"; done >> jpg.log 2>&1
Or possibly better,
find . -name "*.jpg" -type f | xargs jpegoptim >> jpg.log 2>&1
Update
Benjamin W. points out that these break when filenames have embedded newlines, which is entirely true. I consider filenames with embedded newlines a heinous heresy of the highest order, nut sometimes you don't have control of that, so per his entirely valid suggestion:
find . -name "*.jpg" -type f |
while read -r -d '' file; do jpegoptim "$file"; done >> jpg.log 2>&1
or
find . -name "*.jpg" -type f -print0 | xargs -0 jpegoptim >> jpg.log 2>&1
or best, for simplicity and (therefore) safety
find . -name "*.jpg" -type f -exec jpegoptim {} ; >> jpg.log 2>&1
(Please check my syntax on those...)
Though there are still efficiency considerations if you are processing a large number of files. One should also consider the possibility that the target program may or may not be able to multiprocess command-line arguments. Consider the difference between these:
$: find /tmp -type f -exec echo {} ;
/tmp/.mintty-version
/tmp/AdobeARM.log
/tmp/foo
. . .
$: find /tmp -type f | xargs echo
/tmp/.mintty-version /tmp/AdobeARM.log /tmp/foo ...
1
Both of these break for files with blanks or newlines in their names. Best practice is to use-print0 | xargs -0
, or-print0 | while read -r -d ''
. Or just usefind -exec
instead of either.
– Benjamin W.
Nov 19 at 14:41
add a comment |
up vote
1
down vote
accepted
First, don't process dynamically generated file lists in a for
loop.
Second, your code is broken - you have 2 done
's.
Third, my apologies, not familiar with the tool, but I'm inclined to think your issue is in a broader piece of the code than presented.
Fourth, (getting minor and nitpicky :) prefer $(
...)
over backticks. There is very rarely a reason not to do so in bash.
Still, almost none of that is relevant to your question, aside from maybe the third thing... See if this helps -
find . -name "*.jpg" -type f |
while read -r file; do jpegoptim "$file"; done >> jpg.log 2>&1
Or possibly better,
find . -name "*.jpg" -type f | xargs jpegoptim >> jpg.log 2>&1
Update
Benjamin W. points out that these break when filenames have embedded newlines, which is entirely true. I consider filenames with embedded newlines a heinous heresy of the highest order, nut sometimes you don't have control of that, so per his entirely valid suggestion:
find . -name "*.jpg" -type f |
while read -r -d '' file; do jpegoptim "$file"; done >> jpg.log 2>&1
or
find . -name "*.jpg" -type f -print0 | xargs -0 jpegoptim >> jpg.log 2>&1
or best, for simplicity and (therefore) safety
find . -name "*.jpg" -type f -exec jpegoptim {} ; >> jpg.log 2>&1
(Please check my syntax on those...)
Though there are still efficiency considerations if you are processing a large number of files. One should also consider the possibility that the target program may or may not be able to multiprocess command-line arguments. Consider the difference between these:
$: find /tmp -type f -exec echo {} ;
/tmp/.mintty-version
/tmp/AdobeARM.log
/tmp/foo
. . .
$: find /tmp -type f | xargs echo
/tmp/.mintty-version /tmp/AdobeARM.log /tmp/foo ...
1
Both of these break for files with blanks or newlines in their names. Best practice is to use-print0 | xargs -0
, or-print0 | while read -r -d ''
. Or just usefind -exec
instead of either.
– Benjamin W.
Nov 19 at 14:41
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
First, don't process dynamically generated file lists in a for
loop.
Second, your code is broken - you have 2 done
's.
Third, my apologies, not familiar with the tool, but I'm inclined to think your issue is in a broader piece of the code than presented.
Fourth, (getting minor and nitpicky :) prefer $(
...)
over backticks. There is very rarely a reason not to do so in bash.
Still, almost none of that is relevant to your question, aside from maybe the third thing... See if this helps -
find . -name "*.jpg" -type f |
while read -r file; do jpegoptim "$file"; done >> jpg.log 2>&1
Or possibly better,
find . -name "*.jpg" -type f | xargs jpegoptim >> jpg.log 2>&1
Update
Benjamin W. points out that these break when filenames have embedded newlines, which is entirely true. I consider filenames with embedded newlines a heinous heresy of the highest order, nut sometimes you don't have control of that, so per his entirely valid suggestion:
find . -name "*.jpg" -type f |
while read -r -d '' file; do jpegoptim "$file"; done >> jpg.log 2>&1
or
find . -name "*.jpg" -type f -print0 | xargs -0 jpegoptim >> jpg.log 2>&1
or best, for simplicity and (therefore) safety
find . -name "*.jpg" -type f -exec jpegoptim {} ; >> jpg.log 2>&1
(Please check my syntax on those...)
Though there are still efficiency considerations if you are processing a large number of files. One should also consider the possibility that the target program may or may not be able to multiprocess command-line arguments. Consider the difference between these:
$: find /tmp -type f -exec echo {} ;
/tmp/.mintty-version
/tmp/AdobeARM.log
/tmp/foo
. . .
$: find /tmp -type f | xargs echo
/tmp/.mintty-version /tmp/AdobeARM.log /tmp/foo ...
First, don't process dynamically generated file lists in a for
loop.
Second, your code is broken - you have 2 done
's.
Third, my apologies, not familiar with the tool, but I'm inclined to think your issue is in a broader piece of the code than presented.
Fourth, (getting minor and nitpicky :) prefer $(
...)
over backticks. There is very rarely a reason not to do so in bash.
Still, almost none of that is relevant to your question, aside from maybe the third thing... See if this helps -
find . -name "*.jpg" -type f |
while read -r file; do jpegoptim "$file"; done >> jpg.log 2>&1
Or possibly better,
find . -name "*.jpg" -type f | xargs jpegoptim >> jpg.log 2>&1
Update
Benjamin W. points out that these break when filenames have embedded newlines, which is entirely true. I consider filenames with embedded newlines a heinous heresy of the highest order, nut sometimes you don't have control of that, so per his entirely valid suggestion:
find . -name "*.jpg" -type f |
while read -r -d '' file; do jpegoptim "$file"; done >> jpg.log 2>&1
or
find . -name "*.jpg" -type f -print0 | xargs -0 jpegoptim >> jpg.log 2>&1
or best, for simplicity and (therefore) safety
find . -name "*.jpg" -type f -exec jpegoptim {} ; >> jpg.log 2>&1
(Please check my syntax on those...)
Though there are still efficiency considerations if you are processing a large number of files. One should also consider the possibility that the target program may or may not be able to multiprocess command-line arguments. Consider the difference between these:
$: find /tmp -type f -exec echo {} ;
/tmp/.mintty-version
/tmp/AdobeARM.log
/tmp/foo
. . .
$: find /tmp -type f | xargs echo
/tmp/.mintty-version /tmp/AdobeARM.log /tmp/foo ...
edited Nov 19 at 15:06
answered Nov 19 at 14:38


Paul Hodges
2,1591320
2,1591320
1
Both of these break for files with blanks or newlines in their names. Best practice is to use-print0 | xargs -0
, or-print0 | while read -r -d ''
. Or just usefind -exec
instead of either.
– Benjamin W.
Nov 19 at 14:41
add a comment |
1
Both of these break for files with blanks or newlines in their names. Best practice is to use-print0 | xargs -0
, or-print0 | while read -r -d ''
. Or just usefind -exec
instead of either.
– Benjamin W.
Nov 19 at 14:41
1
1
Both of these break for files with blanks or newlines in their names. Best practice is to use
-print0 | xargs -0
, or -print0 | while read -r -d ''
. Or just use find -exec
instead of either.– Benjamin W.
Nov 19 at 14:41
Both of these break for files with blanks or newlines in their names. Best practice is to use
-print0 | xargs -0
, or -print0 | while read -r -d ''
. Or just use find -exec
instead of either.– Benjamin W.
Nov 19 at 14:41
add a comment |
up vote
2
down vote
You can use a counter then ask for user input using read
when the counter reaches 200
#!/bin/bash
count=0
for i in `find . -name "*.jpg" -type f`; do
jpegoptim "$i" >> jpg.log
[[ $((count++)) == 200 ]] && read -rp "Continue? [y/n] " resume
[[ "$resume" == 'n' ]] && break
done
add a comment |
up vote
2
down vote
You can use a counter then ask for user input using read
when the counter reaches 200
#!/bin/bash
count=0
for i in `find . -name "*.jpg" -type f`; do
jpegoptim "$i" >> jpg.log
[[ $((count++)) == 200 ]] && read -rp "Continue? [y/n] " resume
[[ "$resume" == 'n' ]] && break
done
add a comment |
up vote
2
down vote
up vote
2
down vote
You can use a counter then ask for user input using read
when the counter reaches 200
#!/bin/bash
count=0
for i in `find . -name "*.jpg" -type f`; do
jpegoptim "$i" >> jpg.log
[[ $((count++)) == 200 ]] && read -rp "Continue? [y/n] " resume
[[ "$resume" == 'n' ]] && break
done
You can use a counter then ask for user input using read
when the counter reaches 200
#!/bin/bash
count=0
for i in `find . -name "*.jpg" -type f`; do
jpegoptim "$i" >> jpg.log
[[ $((count++)) == 200 ]] && read -rp "Continue? [y/n] " resume
[[ "$resume" == 'n' ]] && break
done
edited Nov 19 at 14:43
answered Nov 19 at 14:35
ssemilla
2,457421
2,457421
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53376521%2frequest-for-input-on-bash-script-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
M,MMT,lvv 10bk,Ihmq OtdtSAmbbO 9 LMZyqFzZKyRPr DPvOBB,l3tg,UmGw,JOlXXsTlNks,qysY3AOdzj