why do we use entrySet() method and use the returned set to iterate a map?
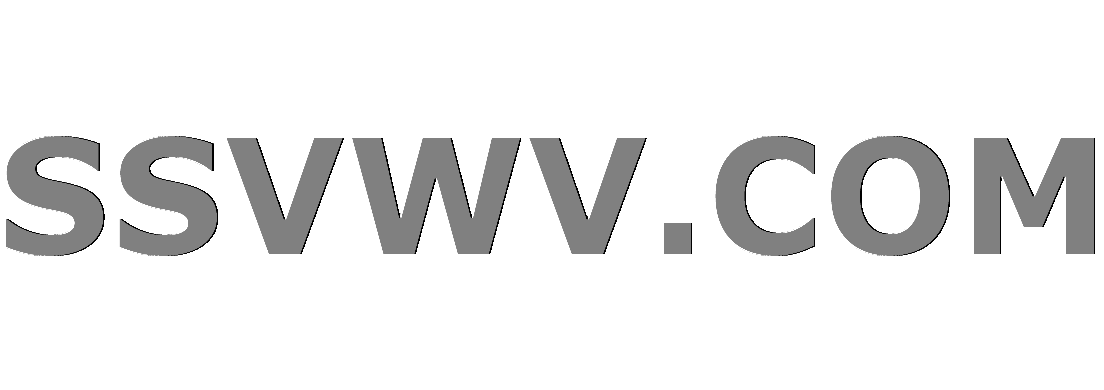
Multi tool use
Usually we write this to get the keys and values from a map.
Map m=new HashMap();
Set s=map.entrySet();
Iterator i=s.iterator()
while(s.hasNext()){
Map.Entry m= (map.Entry) s.next();
System.out.println(""+m.getKey()+""+ m.getValue());
}
Why do we iterate using a set why not directly map?
java dictionary collections iterator
add a comment |
Usually we write this to get the keys and values from a map.
Map m=new HashMap();
Set s=map.entrySet();
Iterator i=s.iterator()
while(s.hasNext()){
Map.Entry m= (map.Entry) s.next();
System.out.println(""+m.getKey()+""+ m.getValue());
}
Why do we iterate using a set why not directly map?
java dictionary collections iterator
add a comment |
Usually we write this to get the keys and values from a map.
Map m=new HashMap();
Set s=map.entrySet();
Iterator i=s.iterator()
while(s.hasNext()){
Map.Entry m= (map.Entry) s.next();
System.out.println(""+m.getKey()+""+ m.getValue());
}
Why do we iterate using a set why not directly map?
java dictionary collections iterator
Usually we write this to get the keys and values from a map.
Map m=new HashMap();
Set s=map.entrySet();
Iterator i=s.iterator()
while(s.hasNext()){
Map.Entry m= (map.Entry) s.next();
System.out.println(""+m.getKey()+""+ m.getValue());
}
Why do we iterate using a set why not directly map?
java dictionary collections iterator
java dictionary collections iterator
edited Nov 26 '18 at 0:20


Chris Halcrow
10.9k46890
10.9k46890
asked Mar 25 '11 at 5:33
priyapriya
1,65261815
1,65261815
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
This is as close to iterating over the map as we can because you have to say whether you want just the keys, just the values or the whole key/value entry. For Sets and Lists, there is only one option so, no need to have a separate method to do this.
BTW: This is how I would iterate over a Map. Note the use of generics, the for-each loop and the LinkedHashMap so the entries appear in some kind of logical order. TreeMap would be another good choice.
Map<K,V> m=new LinkedHashMap<K,V>();
for(Map.Entry<K,V> entry: m.entrySet())
System.out.println(entry.getKey() + ": " + entry.getValue());
In Java 8 you can write
m.forEach((k, v) -> System.out.println(k + ": " + v));
So no need for the iterator?
– Jwan622
Jan 7 '16 at 17:13
1
@Jwan622 The for-each loop was added in Java 5.0 (2004) which would manage the Iterator for you. For collections there is still an Iterator but you don't need to use it directly (unless you want to remove entries)
– Peter Lawrey
Jan 7 '16 at 17:55
Ah so there's an iterator behind the scenes... got it. So generally speaking we take the map object, call entrySet on it to turn it into a set object, and then we take iterate over the set object by slicing it into Map.Entry's? How would you say it?
– Jwan622
Jan 7 '16 at 20:52
add a comment |
Because, logically, a map is a Set collection of key-value pairs - which is what a Map.Entry represents. Iteration is an operation on a collection generally, not a map specifically.
However, I've often wondered myself why Map
doesn't implement Iterable<Map.Entry<K,V>>
et al and provide an iterator()
method over the map entries directly instead of requiring an entry set (which it could certainly do also to provide a full Set
API.
add a comment |
Map is a collection of pairs of things, right (Entries). So you can iterate over entries, or iterate over the keys only (map.keySet()), or over the value only (map.values()). What else do you want to be able to iterate over?
add a comment |
Because Java doesn't have a better syntax to do it. (Yours can be improved)
It would be nice to
for(String key, Integer val : map)
print(key, val);
or
map.foreach (String key, Integer val) -> print(key, val);
but Java doesn't have these.
the problem is deeper than syntax. You can't do this using old-stylefor
loops, orwhile
loops either.
– Stephen C
Mar 25 '11 at 6:48
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f5428897%2fwhy-do-we-use-entryset-method-and-use-the-returned-set-to-iterate-a-map%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is as close to iterating over the map as we can because you have to say whether you want just the keys, just the values or the whole key/value entry. For Sets and Lists, there is only one option so, no need to have a separate method to do this.
BTW: This is how I would iterate over a Map. Note the use of generics, the for-each loop and the LinkedHashMap so the entries appear in some kind of logical order. TreeMap would be another good choice.
Map<K,V> m=new LinkedHashMap<K,V>();
for(Map.Entry<K,V> entry: m.entrySet())
System.out.println(entry.getKey() + ": " + entry.getValue());
In Java 8 you can write
m.forEach((k, v) -> System.out.println(k + ": " + v));
So no need for the iterator?
– Jwan622
Jan 7 '16 at 17:13
1
@Jwan622 The for-each loop was added in Java 5.0 (2004) which would manage the Iterator for you. For collections there is still an Iterator but you don't need to use it directly (unless you want to remove entries)
– Peter Lawrey
Jan 7 '16 at 17:55
Ah so there's an iterator behind the scenes... got it. So generally speaking we take the map object, call entrySet on it to turn it into a set object, and then we take iterate over the set object by slicing it into Map.Entry's? How would you say it?
– Jwan622
Jan 7 '16 at 20:52
add a comment |
This is as close to iterating over the map as we can because you have to say whether you want just the keys, just the values or the whole key/value entry. For Sets and Lists, there is only one option so, no need to have a separate method to do this.
BTW: This is how I would iterate over a Map. Note the use of generics, the for-each loop and the LinkedHashMap so the entries appear in some kind of logical order. TreeMap would be another good choice.
Map<K,V> m=new LinkedHashMap<K,V>();
for(Map.Entry<K,V> entry: m.entrySet())
System.out.println(entry.getKey() + ": " + entry.getValue());
In Java 8 you can write
m.forEach((k, v) -> System.out.println(k + ": " + v));
So no need for the iterator?
– Jwan622
Jan 7 '16 at 17:13
1
@Jwan622 The for-each loop was added in Java 5.0 (2004) which would manage the Iterator for you. For collections there is still an Iterator but you don't need to use it directly (unless you want to remove entries)
– Peter Lawrey
Jan 7 '16 at 17:55
Ah so there's an iterator behind the scenes... got it. So generally speaking we take the map object, call entrySet on it to turn it into a set object, and then we take iterate over the set object by slicing it into Map.Entry's? How would you say it?
– Jwan622
Jan 7 '16 at 20:52
add a comment |
This is as close to iterating over the map as we can because you have to say whether you want just the keys, just the values or the whole key/value entry. For Sets and Lists, there is only one option so, no need to have a separate method to do this.
BTW: This is how I would iterate over a Map. Note the use of generics, the for-each loop and the LinkedHashMap so the entries appear in some kind of logical order. TreeMap would be another good choice.
Map<K,V> m=new LinkedHashMap<K,V>();
for(Map.Entry<K,V> entry: m.entrySet())
System.out.println(entry.getKey() + ": " + entry.getValue());
In Java 8 you can write
m.forEach((k, v) -> System.out.println(k + ": " + v));
This is as close to iterating over the map as we can because you have to say whether you want just the keys, just the values or the whole key/value entry. For Sets and Lists, there is only one option so, no need to have a separate method to do this.
BTW: This is how I would iterate over a Map. Note the use of generics, the for-each loop and the LinkedHashMap so the entries appear in some kind of logical order. TreeMap would be another good choice.
Map<K,V> m=new LinkedHashMap<K,V>();
for(Map.Entry<K,V> entry: m.entrySet())
System.out.println(entry.getKey() + ": " + entry.getValue());
In Java 8 you can write
m.forEach((k, v) -> System.out.println(k + ": " + v));
edited Jan 7 '16 at 17:56
answered Mar 25 '11 at 6:35
Peter LawreyPeter Lawrey
446k56568973
446k56568973
So no need for the iterator?
– Jwan622
Jan 7 '16 at 17:13
1
@Jwan622 The for-each loop was added in Java 5.0 (2004) which would manage the Iterator for you. For collections there is still an Iterator but you don't need to use it directly (unless you want to remove entries)
– Peter Lawrey
Jan 7 '16 at 17:55
Ah so there's an iterator behind the scenes... got it. So generally speaking we take the map object, call entrySet on it to turn it into a set object, and then we take iterate over the set object by slicing it into Map.Entry's? How would you say it?
– Jwan622
Jan 7 '16 at 20:52
add a comment |
So no need for the iterator?
– Jwan622
Jan 7 '16 at 17:13
1
@Jwan622 The for-each loop was added in Java 5.0 (2004) which would manage the Iterator for you. For collections there is still an Iterator but you don't need to use it directly (unless you want to remove entries)
– Peter Lawrey
Jan 7 '16 at 17:55
Ah so there's an iterator behind the scenes... got it. So generally speaking we take the map object, call entrySet on it to turn it into a set object, and then we take iterate over the set object by slicing it into Map.Entry's? How would you say it?
– Jwan622
Jan 7 '16 at 20:52
So no need for the iterator?
– Jwan622
Jan 7 '16 at 17:13
So no need for the iterator?
– Jwan622
Jan 7 '16 at 17:13
1
1
@Jwan622 The for-each loop was added in Java 5.0 (2004) which would manage the Iterator for you. For collections there is still an Iterator but you don't need to use it directly (unless you want to remove entries)
– Peter Lawrey
Jan 7 '16 at 17:55
@Jwan622 The for-each loop was added in Java 5.0 (2004) which would manage the Iterator for you. For collections there is still an Iterator but you don't need to use it directly (unless you want to remove entries)
– Peter Lawrey
Jan 7 '16 at 17:55
Ah so there's an iterator behind the scenes... got it. So generally speaking we take the map object, call entrySet on it to turn it into a set object, and then we take iterate over the set object by slicing it into Map.Entry's? How would you say it?
– Jwan622
Jan 7 '16 at 20:52
Ah so there's an iterator behind the scenes... got it. So generally speaking we take the map object, call entrySet on it to turn it into a set object, and then we take iterate over the set object by slicing it into Map.Entry's? How would you say it?
– Jwan622
Jan 7 '16 at 20:52
add a comment |
Because, logically, a map is a Set collection of key-value pairs - which is what a Map.Entry represents. Iteration is an operation on a collection generally, not a map specifically.
However, I've often wondered myself why Map
doesn't implement Iterable<Map.Entry<K,V>>
et al and provide an iterator()
method over the map entries directly instead of requiring an entry set (which it could certainly do also to provide a full Set
API.
add a comment |
Because, logically, a map is a Set collection of key-value pairs - which is what a Map.Entry represents. Iteration is an operation on a collection generally, not a map specifically.
However, I've often wondered myself why Map
doesn't implement Iterable<Map.Entry<K,V>>
et al and provide an iterator()
method over the map entries directly instead of requiring an entry set (which it could certainly do also to provide a full Set
API.
add a comment |
Because, logically, a map is a Set collection of key-value pairs - which is what a Map.Entry represents. Iteration is an operation on a collection generally, not a map specifically.
However, I've often wondered myself why Map
doesn't implement Iterable<Map.Entry<K,V>>
et al and provide an iterator()
method over the map entries directly instead of requiring an entry set (which it could certainly do also to provide a full Set
API.
Because, logically, a map is a Set collection of key-value pairs - which is what a Map.Entry represents. Iteration is an operation on a collection generally, not a map specifically.
However, I've often wondered myself why Map
doesn't implement Iterable<Map.Entry<K,V>>
et al and provide an iterator()
method over the map entries directly instead of requiring an entry set (which it could certainly do also to provide a full Set
API.
edited May 2 '14 at 17:52
answered Mar 25 '11 at 5:38
Lawrence DolLawrence Dol
46.9k23125176
46.9k23125176
add a comment |
add a comment |
Map is a collection of pairs of things, right (Entries). So you can iterate over entries, or iterate over the keys only (map.keySet()), or over the value only (map.values()). What else do you want to be able to iterate over?
add a comment |
Map is a collection of pairs of things, right (Entries). So you can iterate over entries, or iterate over the keys only (map.keySet()), or over the value only (map.values()). What else do you want to be able to iterate over?
add a comment |
Map is a collection of pairs of things, right (Entries). So you can iterate over entries, or iterate over the keys only (map.keySet()), or over the value only (map.values()). What else do you want to be able to iterate over?
Map is a collection of pairs of things, right (Entries). So you can iterate over entries, or iterate over the keys only (map.keySet()), or over the value only (map.values()). What else do you want to be able to iterate over?
answered Mar 25 '11 at 5:37


iluxailuxa
6,0781027
6,0781027
add a comment |
add a comment |
Because Java doesn't have a better syntax to do it. (Yours can be improved)
It would be nice to
for(String key, Integer val : map)
print(key, val);
or
map.foreach (String key, Integer val) -> print(key, val);
but Java doesn't have these.
the problem is deeper than syntax. You can't do this using old-stylefor
loops, orwhile
loops either.
– Stephen C
Mar 25 '11 at 6:48
add a comment |
Because Java doesn't have a better syntax to do it. (Yours can be improved)
It would be nice to
for(String key, Integer val : map)
print(key, val);
or
map.foreach (String key, Integer val) -> print(key, val);
but Java doesn't have these.
the problem is deeper than syntax. You can't do this using old-stylefor
loops, orwhile
loops either.
– Stephen C
Mar 25 '11 at 6:48
add a comment |
Because Java doesn't have a better syntax to do it. (Yours can be improved)
It would be nice to
for(String key, Integer val : map)
print(key, val);
or
map.foreach (String key, Integer val) -> print(key, val);
but Java doesn't have these.
Because Java doesn't have a better syntax to do it. (Yours can be improved)
It would be nice to
for(String key, Integer val : map)
print(key, val);
or
map.foreach (String key, Integer val) -> print(key, val);
but Java doesn't have these.
answered Mar 25 '11 at 5:52
irreputableirreputable
39.3k75485
39.3k75485
the problem is deeper than syntax. You can't do this using old-stylefor
loops, orwhile
loops either.
– Stephen C
Mar 25 '11 at 6:48
add a comment |
the problem is deeper than syntax. You can't do this using old-stylefor
loops, orwhile
loops either.
– Stephen C
Mar 25 '11 at 6:48
the problem is deeper than syntax. You can't do this using old-style
for
loops, or while
loops either.– Stephen C
Mar 25 '11 at 6:48
the problem is deeper than syntax. You can't do this using old-style
for
loops, or while
loops either.– Stephen C
Mar 25 '11 at 6:48
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f5428897%2fwhy-do-we-use-entryset-method-and-use-the-returned-set-to-iterate-a-map%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0 l v KIu 6kgIDwh,GpEp,ZfK1A VytRA,aC1