Send notification in Kaa platform by Java using RestAPI
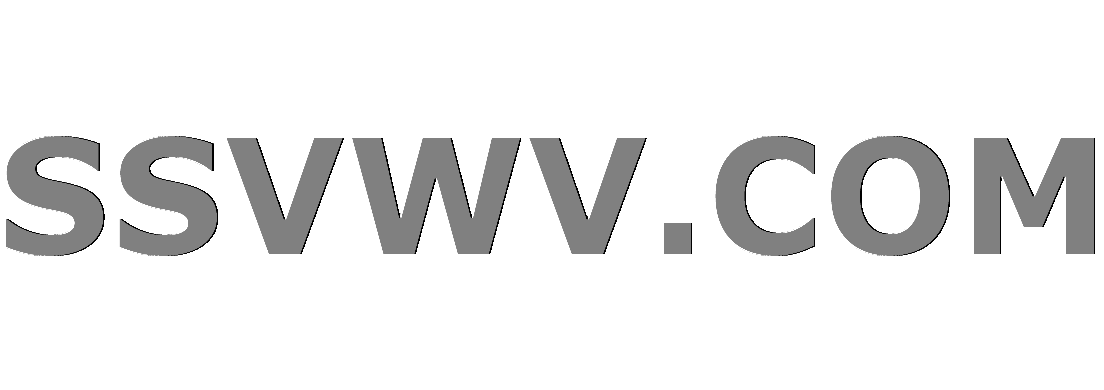
Multi tool use
In the Kaa document, here is the link rest api is provided to call if I want to send a notification: https://kaaproject.github.io/kaa/docs/v0.10.0/Programming-guide/Server-REST-APIs/#!/Notifications/sendNotification
When I used postman to call that api - everything is okay like this
https://ctninhkieu-my.sharepoint.com/:i:/g/personal/ltthanh_ctninhkieu_onmicrosoft_com/ERK5TH8cEE5Hn4hgZ0IHsqgBSzePovlDqD4eUD9q68MUrQ?e=fVlFec
But when I wrote java code to call it with glassfish jersey, it returned 415 code:
InboundJaxrsResponse{context=ClientResponse{method=POST, uri=http://localhost:8080/kaaAdmin/rest/api/sendNotification, status=415, reason=Unsupported Media Type}}
Here is my code:
String API_URI = "http://localhost:8080/kaaAdmin/rest/api/sendNotification";
Client client = ClientBuilder.newBuilder().register(MultiPartFeature.class).build();
MultiPart multiPart = new FormDataMultiPart()
.bodyPart(new FileDataBodyPart("notification", new File("files/notification.json")))
.bodyPart(new FileDataBodyPart("file", new File("files/file.json")));
Response response = client.target(API_URI)
.request()
.header("Authorization", "Basic AAAAAAAAAAAAAA")
.post(Entity.entity(multiPart, multiPart.getMediaType()));
System.out.println(response.toString());
And maven repository
<!-- https://mvnrepository.com/artifact/org.glassfish.jersey.core/jersey-client -->
<dependency>
<groupId>org.glassfish.jersey.core</groupId>
<artifactId>jersey-client</artifactId>
<version>2.27</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.glassfish.jersey.media/jersey-media-multipart -->
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-multipart</artifactId>
<version>2.27</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.glassfish.jersey.inject/jersey-hk2 -->
<dependency>
<groupId>org.glassfish.jersey.inject</groupId>
<artifactId>jersey-hk2</artifactId>
<version>2.27</version>
</dependency>
Thank you for your reading ^^
java jersey kaa
add a comment |
In the Kaa document, here is the link rest api is provided to call if I want to send a notification: https://kaaproject.github.io/kaa/docs/v0.10.0/Programming-guide/Server-REST-APIs/#!/Notifications/sendNotification
When I used postman to call that api - everything is okay like this
https://ctninhkieu-my.sharepoint.com/:i:/g/personal/ltthanh_ctninhkieu_onmicrosoft_com/ERK5TH8cEE5Hn4hgZ0IHsqgBSzePovlDqD4eUD9q68MUrQ?e=fVlFec
But when I wrote java code to call it with glassfish jersey, it returned 415 code:
InboundJaxrsResponse{context=ClientResponse{method=POST, uri=http://localhost:8080/kaaAdmin/rest/api/sendNotification, status=415, reason=Unsupported Media Type}}
Here is my code:
String API_URI = "http://localhost:8080/kaaAdmin/rest/api/sendNotification";
Client client = ClientBuilder.newBuilder().register(MultiPartFeature.class).build();
MultiPart multiPart = new FormDataMultiPart()
.bodyPart(new FileDataBodyPart("notification", new File("files/notification.json")))
.bodyPart(new FileDataBodyPart("file", new File("files/file.json")));
Response response = client.target(API_URI)
.request()
.header("Authorization", "Basic AAAAAAAAAAAAAA")
.post(Entity.entity(multiPart, multiPart.getMediaType()));
System.out.println(response.toString());
And maven repository
<!-- https://mvnrepository.com/artifact/org.glassfish.jersey.core/jersey-client -->
<dependency>
<groupId>org.glassfish.jersey.core</groupId>
<artifactId>jersey-client</artifactId>
<version>2.27</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.glassfish.jersey.media/jersey-media-multipart -->
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-multipart</artifactId>
<version>2.27</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.glassfish.jersey.inject/jersey-hk2 -->
<dependency>
<groupId>org.glassfish.jersey.inject</groupId>
<artifactId>jersey-hk2</artifactId>
<version>2.27</version>
</dependency>
Thank you for your reading ^^
java jersey kaa
add a comment |
In the Kaa document, here is the link rest api is provided to call if I want to send a notification: https://kaaproject.github.io/kaa/docs/v0.10.0/Programming-guide/Server-REST-APIs/#!/Notifications/sendNotification
When I used postman to call that api - everything is okay like this
https://ctninhkieu-my.sharepoint.com/:i:/g/personal/ltthanh_ctninhkieu_onmicrosoft_com/ERK5TH8cEE5Hn4hgZ0IHsqgBSzePovlDqD4eUD9q68MUrQ?e=fVlFec
But when I wrote java code to call it with glassfish jersey, it returned 415 code:
InboundJaxrsResponse{context=ClientResponse{method=POST, uri=http://localhost:8080/kaaAdmin/rest/api/sendNotification, status=415, reason=Unsupported Media Type}}
Here is my code:
String API_URI = "http://localhost:8080/kaaAdmin/rest/api/sendNotification";
Client client = ClientBuilder.newBuilder().register(MultiPartFeature.class).build();
MultiPart multiPart = new FormDataMultiPart()
.bodyPart(new FileDataBodyPart("notification", new File("files/notification.json")))
.bodyPart(new FileDataBodyPart("file", new File("files/file.json")));
Response response = client.target(API_URI)
.request()
.header("Authorization", "Basic AAAAAAAAAAAAAA")
.post(Entity.entity(multiPart, multiPart.getMediaType()));
System.out.println(response.toString());
And maven repository
<!-- https://mvnrepository.com/artifact/org.glassfish.jersey.core/jersey-client -->
<dependency>
<groupId>org.glassfish.jersey.core</groupId>
<artifactId>jersey-client</artifactId>
<version>2.27</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.glassfish.jersey.media/jersey-media-multipart -->
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-multipart</artifactId>
<version>2.27</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.glassfish.jersey.inject/jersey-hk2 -->
<dependency>
<groupId>org.glassfish.jersey.inject</groupId>
<artifactId>jersey-hk2</artifactId>
<version>2.27</version>
</dependency>
Thank you for your reading ^^
java jersey kaa
In the Kaa document, here is the link rest api is provided to call if I want to send a notification: https://kaaproject.github.io/kaa/docs/v0.10.0/Programming-guide/Server-REST-APIs/#!/Notifications/sendNotification
When I used postman to call that api - everything is okay like this
https://ctninhkieu-my.sharepoint.com/:i:/g/personal/ltthanh_ctninhkieu_onmicrosoft_com/ERK5TH8cEE5Hn4hgZ0IHsqgBSzePovlDqD4eUD9q68MUrQ?e=fVlFec
But when I wrote java code to call it with glassfish jersey, it returned 415 code:
InboundJaxrsResponse{context=ClientResponse{method=POST, uri=http://localhost:8080/kaaAdmin/rest/api/sendNotification, status=415, reason=Unsupported Media Type}}
Here is my code:
String API_URI = "http://localhost:8080/kaaAdmin/rest/api/sendNotification";
Client client = ClientBuilder.newBuilder().register(MultiPartFeature.class).build();
MultiPart multiPart = new FormDataMultiPart()
.bodyPart(new FileDataBodyPart("notification", new File("files/notification.json")))
.bodyPart(new FileDataBodyPart("file", new File("files/file.json")));
Response response = client.target(API_URI)
.request()
.header("Authorization", "Basic AAAAAAAAAAAAAA")
.post(Entity.entity(multiPart, multiPart.getMediaType()));
System.out.println(response.toString());
And maven repository
<!-- https://mvnrepository.com/artifact/org.glassfish.jersey.core/jersey-client -->
<dependency>
<groupId>org.glassfish.jersey.core</groupId>
<artifactId>jersey-client</artifactId>
<version>2.27</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.glassfish.jersey.media/jersey-media-multipart -->
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-multipart</artifactId>
<version>2.27</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.glassfish.jersey.inject/jersey-hk2 -->
<dependency>
<groupId>org.glassfish.jersey.inject</groupId>
<artifactId>jersey-hk2</artifactId>
<version>2.27</version>
</dependency>
Thank you for your reading ^^
java jersey kaa
java jersey kaa
asked Nov 26 '18 at 0:31
Lê Trí ThànhLê Trí Thành
113
113
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I solved this problem!!!
Try another way to call this API:
CloseableHttpClient client = HttpClients.createDefault();
HttpPost httpPost = new HttpPost("http://localhost:8080/kaaAdmin/rest/api/sendNotification");
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addBinaryBody("notification", new File("files/notification.json"),
ContentType.APPLICATION_JSON, "notification.json");
builder.addBinaryBody("file", new File("files/file.json"),
ContentType.APPLICATION_OCTET_STREAM, "file.json");
HttpEntity multipart = builder.build();
httpPost.addHeader("Authorization", "Basic AAAAAAAAAAA");
httpPost.setEntity(multipart);
CloseableHttpResponse response = client.execute(httpPost);
System.out.println(response.getStatusLine().getStatusCode());
client.close();
with two dependencies
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpclient -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.6</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpmime -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpmime</artifactId>
<version>4.5.6</version>
</dependency>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53473409%2fsend-notification-in-kaa-platform-by-java-using-restapi%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I solved this problem!!!
Try another way to call this API:
CloseableHttpClient client = HttpClients.createDefault();
HttpPost httpPost = new HttpPost("http://localhost:8080/kaaAdmin/rest/api/sendNotification");
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addBinaryBody("notification", new File("files/notification.json"),
ContentType.APPLICATION_JSON, "notification.json");
builder.addBinaryBody("file", new File("files/file.json"),
ContentType.APPLICATION_OCTET_STREAM, "file.json");
HttpEntity multipart = builder.build();
httpPost.addHeader("Authorization", "Basic AAAAAAAAAAA");
httpPost.setEntity(multipart);
CloseableHttpResponse response = client.execute(httpPost);
System.out.println(response.getStatusLine().getStatusCode());
client.close();
with two dependencies
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpclient -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.6</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpmime -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpmime</artifactId>
<version>4.5.6</version>
</dependency>
add a comment |
I solved this problem!!!
Try another way to call this API:
CloseableHttpClient client = HttpClients.createDefault();
HttpPost httpPost = new HttpPost("http://localhost:8080/kaaAdmin/rest/api/sendNotification");
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addBinaryBody("notification", new File("files/notification.json"),
ContentType.APPLICATION_JSON, "notification.json");
builder.addBinaryBody("file", new File("files/file.json"),
ContentType.APPLICATION_OCTET_STREAM, "file.json");
HttpEntity multipart = builder.build();
httpPost.addHeader("Authorization", "Basic AAAAAAAAAAA");
httpPost.setEntity(multipart);
CloseableHttpResponse response = client.execute(httpPost);
System.out.println(response.getStatusLine().getStatusCode());
client.close();
with two dependencies
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpclient -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.6</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpmime -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpmime</artifactId>
<version>4.5.6</version>
</dependency>
add a comment |
I solved this problem!!!
Try another way to call this API:
CloseableHttpClient client = HttpClients.createDefault();
HttpPost httpPost = new HttpPost("http://localhost:8080/kaaAdmin/rest/api/sendNotification");
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addBinaryBody("notification", new File("files/notification.json"),
ContentType.APPLICATION_JSON, "notification.json");
builder.addBinaryBody("file", new File("files/file.json"),
ContentType.APPLICATION_OCTET_STREAM, "file.json");
HttpEntity multipart = builder.build();
httpPost.addHeader("Authorization", "Basic AAAAAAAAAAA");
httpPost.setEntity(multipart);
CloseableHttpResponse response = client.execute(httpPost);
System.out.println(response.getStatusLine().getStatusCode());
client.close();
with two dependencies
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpclient -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.6</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpmime -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpmime</artifactId>
<version>4.5.6</version>
</dependency>
I solved this problem!!!
Try another way to call this API:
CloseableHttpClient client = HttpClients.createDefault();
HttpPost httpPost = new HttpPost("http://localhost:8080/kaaAdmin/rest/api/sendNotification");
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addBinaryBody("notification", new File("files/notification.json"),
ContentType.APPLICATION_JSON, "notification.json");
builder.addBinaryBody("file", new File("files/file.json"),
ContentType.APPLICATION_OCTET_STREAM, "file.json");
HttpEntity multipart = builder.build();
httpPost.addHeader("Authorization", "Basic AAAAAAAAAAA");
httpPost.setEntity(multipart);
CloseableHttpResponse response = client.execute(httpPost);
System.out.println(response.getStatusLine().getStatusCode());
client.close();
with two dependencies
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpclient -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.6</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpmime -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpmime</artifactId>
<version>4.5.6</version>
</dependency>
answered Dec 1 '18 at 3:49
Lê Trí ThànhLê Trí Thành
113
113
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53473409%2fsend-notification-in-kaa-platform-by-java-using-restapi%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qG6Qc,sqIg6QUSZy1O,MgzN,t,T7D7Q0uhe4 mWe,R03zltoL,hz dUB