Unmarshalling LocalDate/LocalDateTime with MOXy
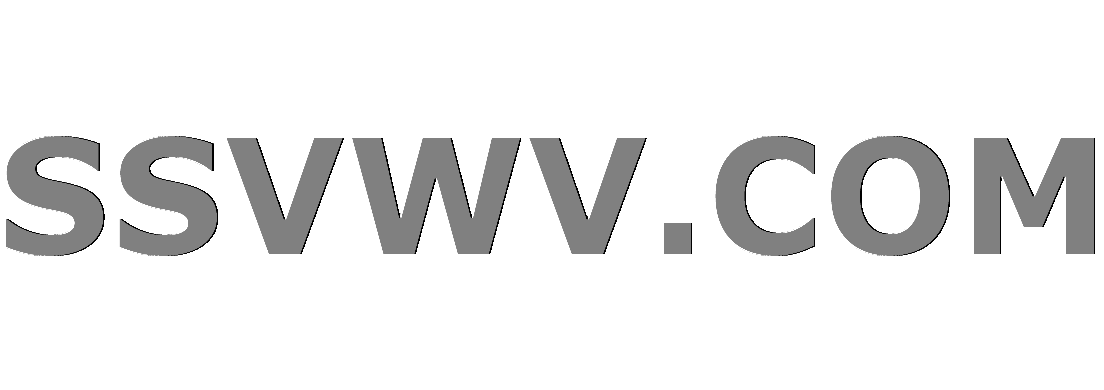
Multi tool use
How can I get MOXy to unmarshal JSON into LocalDate
and LocalDateTime
?
I've got an @GET
method which produces a sample instance with three fields of types LocalDate
, LocalDateTime
and Date
, respectively.
Hitting that endpoint, I get:
{
"localDate": "2017-07-11",
"localDateTime": "2017-07-11T10:11:10.817",
"date": "2017-07-11T10:11:10.817+02:00"
}
I then POST the above data to my @POST
method, which simply returns the data again:
{
"date": "2017-07-11T10:11:10.817+02:00"
}
As you can see, both localDate
and localDateTime
are lost in the process, because MOXy does not initialize those two fields.
What gives? MOXy seems to support serialization of these types, but not deserialization?
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.Date;
@Path("/test/date")
public class DateTest {
public static class Data {
public LocalDate localDate;
public LocalDateTime localDateTime;
public Date date;
}
@GET
@Path("roundtrip")
public Response roundtrip() {
Data sample = getSample();
return roundtrip(sample);
}
@POST
@Path("roundtrip")
@Consumes(MediaType.APPLICATION_JSON)
public Response roundtrip(Data t) {
return Response.status(Response.Status.OK).entity(t).build();
}
protected Data getSample() {
final Data data = new Data();
data.localDate = LocalDate.now();
data.localDateTime = LocalDateTime.now();
data.date = new Date();
return data;
}
}
Moxy version: jersey-media-moxy-2.25.1
java jaxb jersey jax-rs moxy
add a comment |
How can I get MOXy to unmarshal JSON into LocalDate
and LocalDateTime
?
I've got an @GET
method which produces a sample instance with three fields of types LocalDate
, LocalDateTime
and Date
, respectively.
Hitting that endpoint, I get:
{
"localDate": "2017-07-11",
"localDateTime": "2017-07-11T10:11:10.817",
"date": "2017-07-11T10:11:10.817+02:00"
}
I then POST the above data to my @POST
method, which simply returns the data again:
{
"date": "2017-07-11T10:11:10.817+02:00"
}
As you can see, both localDate
and localDateTime
are lost in the process, because MOXy does not initialize those two fields.
What gives? MOXy seems to support serialization of these types, but not deserialization?
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.Date;
@Path("/test/date")
public class DateTest {
public static class Data {
public LocalDate localDate;
public LocalDateTime localDateTime;
public Date date;
}
@GET
@Path("roundtrip")
public Response roundtrip() {
Data sample = getSample();
return roundtrip(sample);
}
@POST
@Path("roundtrip")
@Consumes(MediaType.APPLICATION_JSON)
public Response roundtrip(Data t) {
return Response.status(Response.Status.OK).entity(t).build();
}
protected Data getSample() {
final Data data = new Data();
data.localDate = LocalDate.now();
data.localDateTime = LocalDateTime.now();
data.date = new Date();
return data;
}
}
Moxy version: jersey-media-moxy-2.25.1
java jaxb jersey jax-rs moxy
1
Is it really "serializing" (in the more common sense) or is it simply calling toString()?. There's a difference. Until MOXy/JAXB actually supports Java8 time, you'll probably need to use an XMLAdapter. Or you can use Jackson instead of MOXy, and use its Java8 time support
– Paul Samsotha
Jul 11 '17 at 8:35
@peeskillet I didn't think of this possibility. I read your post and understood that to mean that it was supported.
– phant0m
Jul 11 '17 at 8:40
In that post I actually gave both examples of what I mentioned as solutions in my previous comment (i.e. use an adapter or switch to Jackson)
– Paul Samsotha
Jul 11 '17 at 8:42
Actually, I think I need to update the post. I probably tested only using the serialization :-D
– Paul Samsotha
Jul 11 '17 at 8:44
@peeskillet the XMLAdapter really solves this problem. In addition you may then specify the format how the LocalDateTime will be marshalled/unmarshalled. +1
– gabriel
Sep 20 '17 at 13:11
add a comment |
How can I get MOXy to unmarshal JSON into LocalDate
and LocalDateTime
?
I've got an @GET
method which produces a sample instance with three fields of types LocalDate
, LocalDateTime
and Date
, respectively.
Hitting that endpoint, I get:
{
"localDate": "2017-07-11",
"localDateTime": "2017-07-11T10:11:10.817",
"date": "2017-07-11T10:11:10.817+02:00"
}
I then POST the above data to my @POST
method, which simply returns the data again:
{
"date": "2017-07-11T10:11:10.817+02:00"
}
As you can see, both localDate
and localDateTime
are lost in the process, because MOXy does not initialize those two fields.
What gives? MOXy seems to support serialization of these types, but not deserialization?
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.Date;
@Path("/test/date")
public class DateTest {
public static class Data {
public LocalDate localDate;
public LocalDateTime localDateTime;
public Date date;
}
@GET
@Path("roundtrip")
public Response roundtrip() {
Data sample = getSample();
return roundtrip(sample);
}
@POST
@Path("roundtrip")
@Consumes(MediaType.APPLICATION_JSON)
public Response roundtrip(Data t) {
return Response.status(Response.Status.OK).entity(t).build();
}
protected Data getSample() {
final Data data = new Data();
data.localDate = LocalDate.now();
data.localDateTime = LocalDateTime.now();
data.date = new Date();
return data;
}
}
Moxy version: jersey-media-moxy-2.25.1
java jaxb jersey jax-rs moxy
How can I get MOXy to unmarshal JSON into LocalDate
and LocalDateTime
?
I've got an @GET
method which produces a sample instance with three fields of types LocalDate
, LocalDateTime
and Date
, respectively.
Hitting that endpoint, I get:
{
"localDate": "2017-07-11",
"localDateTime": "2017-07-11T10:11:10.817",
"date": "2017-07-11T10:11:10.817+02:00"
}
I then POST the above data to my @POST
method, which simply returns the data again:
{
"date": "2017-07-11T10:11:10.817+02:00"
}
As you can see, both localDate
and localDateTime
are lost in the process, because MOXy does not initialize those two fields.
What gives? MOXy seems to support serialization of these types, but not deserialization?
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.Date;
@Path("/test/date")
public class DateTest {
public static class Data {
public LocalDate localDate;
public LocalDateTime localDateTime;
public Date date;
}
@GET
@Path("roundtrip")
public Response roundtrip() {
Data sample = getSample();
return roundtrip(sample);
}
@POST
@Path("roundtrip")
@Consumes(MediaType.APPLICATION_JSON)
public Response roundtrip(Data t) {
return Response.status(Response.Status.OK).entity(t).build();
}
protected Data getSample() {
final Data data = new Data();
data.localDate = LocalDate.now();
data.localDateTime = LocalDateTime.now();
data.date = new Date();
return data;
}
}
Moxy version: jersey-media-moxy-2.25.1
java jaxb jersey jax-rs moxy
java jaxb jersey jax-rs moxy
asked Jul 11 '17 at 8:25
phant0m
11.9k33268
11.9k33268
1
Is it really "serializing" (in the more common sense) or is it simply calling toString()?. There's a difference. Until MOXy/JAXB actually supports Java8 time, you'll probably need to use an XMLAdapter. Or you can use Jackson instead of MOXy, and use its Java8 time support
– Paul Samsotha
Jul 11 '17 at 8:35
@peeskillet I didn't think of this possibility. I read your post and understood that to mean that it was supported.
– phant0m
Jul 11 '17 at 8:40
In that post I actually gave both examples of what I mentioned as solutions in my previous comment (i.e. use an adapter or switch to Jackson)
– Paul Samsotha
Jul 11 '17 at 8:42
Actually, I think I need to update the post. I probably tested only using the serialization :-D
– Paul Samsotha
Jul 11 '17 at 8:44
@peeskillet the XMLAdapter really solves this problem. In addition you may then specify the format how the LocalDateTime will be marshalled/unmarshalled. +1
– gabriel
Sep 20 '17 at 13:11
add a comment |
1
Is it really "serializing" (in the more common sense) or is it simply calling toString()?. There's a difference. Until MOXy/JAXB actually supports Java8 time, you'll probably need to use an XMLAdapter. Or you can use Jackson instead of MOXy, and use its Java8 time support
– Paul Samsotha
Jul 11 '17 at 8:35
@peeskillet I didn't think of this possibility. I read your post and understood that to mean that it was supported.
– phant0m
Jul 11 '17 at 8:40
In that post I actually gave both examples of what I mentioned as solutions in my previous comment (i.e. use an adapter or switch to Jackson)
– Paul Samsotha
Jul 11 '17 at 8:42
Actually, I think I need to update the post. I probably tested only using the serialization :-D
– Paul Samsotha
Jul 11 '17 at 8:44
@peeskillet the XMLAdapter really solves this problem. In addition you may then specify the format how the LocalDateTime will be marshalled/unmarshalled. +1
– gabriel
Sep 20 '17 at 13:11
1
1
Is it really "serializing" (in the more common sense) or is it simply calling toString()?. There's a difference. Until MOXy/JAXB actually supports Java8 time, you'll probably need to use an XMLAdapter. Or you can use Jackson instead of MOXy, and use its Java8 time support
– Paul Samsotha
Jul 11 '17 at 8:35
Is it really "serializing" (in the more common sense) or is it simply calling toString()?. There's a difference. Until MOXy/JAXB actually supports Java8 time, you'll probably need to use an XMLAdapter. Or you can use Jackson instead of MOXy, and use its Java8 time support
– Paul Samsotha
Jul 11 '17 at 8:35
@peeskillet I didn't think of this possibility. I read your post and understood that to mean that it was supported.
– phant0m
Jul 11 '17 at 8:40
@peeskillet I didn't think of this possibility. I read your post and understood that to mean that it was supported.
– phant0m
Jul 11 '17 at 8:40
In that post I actually gave both examples of what I mentioned as solutions in my previous comment (i.e. use an adapter or switch to Jackson)
– Paul Samsotha
Jul 11 '17 at 8:42
In that post I actually gave both examples of what I mentioned as solutions in my previous comment (i.e. use an adapter or switch to Jackson)
– Paul Samsotha
Jul 11 '17 at 8:42
Actually, I think I need to update the post. I probably tested only using the serialization :-D
– Paul Samsotha
Jul 11 '17 at 8:44
Actually, I think I need to update the post. I probably tested only using the serialization :-D
– Paul Samsotha
Jul 11 '17 at 8:44
@peeskillet the XMLAdapter really solves this problem. In addition you may then specify the format how the LocalDateTime will be marshalled/unmarshalled. +1
– gabriel
Sep 20 '17 at 13:11
@peeskillet the XMLAdapter really solves this problem. In addition you may then specify the format how the LocalDateTime will be marshalled/unmarshalled. +1
– gabriel
Sep 20 '17 at 13:11
add a comment |
1 Answer
1
active
oldest
votes
According to peeskillet's suggestion I implemented the following adapter class:
public class LocalDateTimeAdapter extends XmlAdapter<String, LocalDateTime>{
private static final DateTimeFormatter DTF = DateTimeFormatter.ofPattern("yyyyMMddHHmmss");
@Override
public String marshal(LocalDateTime localDateTime) throws Exception {
return localDateTime.format(DTF);
}
@Override
public LocalDateTime unmarshal(String string) throws Exception {
return LocalDateTime.parse(string, DTF);
}
}
In addition, I created package-info.java
in the same package where my classes for MOXy and the adapter (in a subpackage) are located with the following content:
@XmlJavaTypeAdapters({
@XmlJavaTypeAdapter(type=LocalDateTime.class,
value=LocalDateTimeAdapter.class)
})
package api;
import java.time.LocalDateTime;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapters;
import api.adapter.LocalDateTimeAdapter;
Thus, marshalling and unmarshalling works without problems. And with DTF
you can specify the format that shall be applied.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f45029200%2funmarshalling-localdate-localdatetime-with-moxy%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
According to peeskillet's suggestion I implemented the following adapter class:
public class LocalDateTimeAdapter extends XmlAdapter<String, LocalDateTime>{
private static final DateTimeFormatter DTF = DateTimeFormatter.ofPattern("yyyyMMddHHmmss");
@Override
public String marshal(LocalDateTime localDateTime) throws Exception {
return localDateTime.format(DTF);
}
@Override
public LocalDateTime unmarshal(String string) throws Exception {
return LocalDateTime.parse(string, DTF);
}
}
In addition, I created package-info.java
in the same package where my classes for MOXy and the adapter (in a subpackage) are located with the following content:
@XmlJavaTypeAdapters({
@XmlJavaTypeAdapter(type=LocalDateTime.class,
value=LocalDateTimeAdapter.class)
})
package api;
import java.time.LocalDateTime;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapters;
import api.adapter.LocalDateTimeAdapter;
Thus, marshalling and unmarshalling works without problems. And with DTF
you can specify the format that shall be applied.
add a comment |
According to peeskillet's suggestion I implemented the following adapter class:
public class LocalDateTimeAdapter extends XmlAdapter<String, LocalDateTime>{
private static final DateTimeFormatter DTF = DateTimeFormatter.ofPattern("yyyyMMddHHmmss");
@Override
public String marshal(LocalDateTime localDateTime) throws Exception {
return localDateTime.format(DTF);
}
@Override
public LocalDateTime unmarshal(String string) throws Exception {
return LocalDateTime.parse(string, DTF);
}
}
In addition, I created package-info.java
in the same package where my classes for MOXy and the adapter (in a subpackage) are located with the following content:
@XmlJavaTypeAdapters({
@XmlJavaTypeAdapter(type=LocalDateTime.class,
value=LocalDateTimeAdapter.class)
})
package api;
import java.time.LocalDateTime;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapters;
import api.adapter.LocalDateTimeAdapter;
Thus, marshalling and unmarshalling works without problems. And with DTF
you can specify the format that shall be applied.
add a comment |
According to peeskillet's suggestion I implemented the following adapter class:
public class LocalDateTimeAdapter extends XmlAdapter<String, LocalDateTime>{
private static final DateTimeFormatter DTF = DateTimeFormatter.ofPattern("yyyyMMddHHmmss");
@Override
public String marshal(LocalDateTime localDateTime) throws Exception {
return localDateTime.format(DTF);
}
@Override
public LocalDateTime unmarshal(String string) throws Exception {
return LocalDateTime.parse(string, DTF);
}
}
In addition, I created package-info.java
in the same package where my classes for MOXy and the adapter (in a subpackage) are located with the following content:
@XmlJavaTypeAdapters({
@XmlJavaTypeAdapter(type=LocalDateTime.class,
value=LocalDateTimeAdapter.class)
})
package api;
import java.time.LocalDateTime;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapters;
import api.adapter.LocalDateTimeAdapter;
Thus, marshalling and unmarshalling works without problems. And with DTF
you can specify the format that shall be applied.
According to peeskillet's suggestion I implemented the following adapter class:
public class LocalDateTimeAdapter extends XmlAdapter<String, LocalDateTime>{
private static final DateTimeFormatter DTF = DateTimeFormatter.ofPattern("yyyyMMddHHmmss");
@Override
public String marshal(LocalDateTime localDateTime) throws Exception {
return localDateTime.format(DTF);
}
@Override
public LocalDateTime unmarshal(String string) throws Exception {
return LocalDateTime.parse(string, DTF);
}
}
In addition, I created package-info.java
in the same package where my classes for MOXy and the adapter (in a subpackage) are located with the following content:
@XmlJavaTypeAdapters({
@XmlJavaTypeAdapter(type=LocalDateTime.class,
value=LocalDateTimeAdapter.class)
})
package api;
import java.time.LocalDateTime;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapters;
import api.adapter.LocalDateTimeAdapter;
Thus, marshalling and unmarshalling works without problems. And with DTF
you can specify the format that shall be applied.
edited Sep 20 '17 at 13:39
answered Sep 20 '17 at 13:27
gabriel
190216
190216
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f45029200%2funmarshalling-localdate-localdatetime-with-moxy%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8BZ 4 1SylNIGu,5Nc3g z0xnI
1
Is it really "serializing" (in the more common sense) or is it simply calling toString()?. There's a difference. Until MOXy/JAXB actually supports Java8 time, you'll probably need to use an XMLAdapter. Or you can use Jackson instead of MOXy, and use its Java8 time support
– Paul Samsotha
Jul 11 '17 at 8:35
@peeskillet I didn't think of this possibility. I read your post and understood that to mean that it was supported.
– phant0m
Jul 11 '17 at 8:40
In that post I actually gave both examples of what I mentioned as solutions in my previous comment (i.e. use an adapter or switch to Jackson)
– Paul Samsotha
Jul 11 '17 at 8:42
Actually, I think I need to update the post. I probably tested only using the serialization :-D
– Paul Samsotha
Jul 11 '17 at 8:44
@peeskillet the XMLAdapter really solves this problem. In addition you may then specify the format how the LocalDateTime will be marshalled/unmarshalled. +1
– gabriel
Sep 20 '17 at 13:11