SnapKit Updating Constraint causes conflict
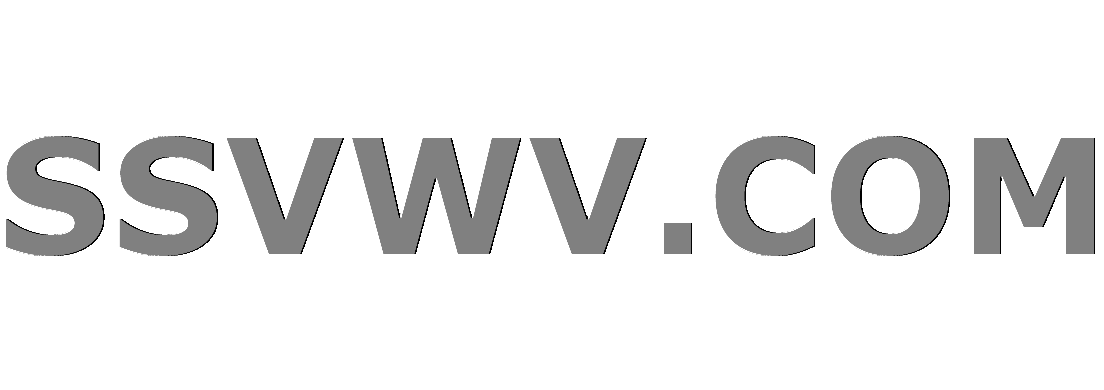
Multi tool use
I'm building an iOS app against iOS 12 SDK, Swift 4 and SnapKit 4.2
I want to update a constraint when I tap a button but it creates a conflict with the previous version of the constraint.
Here's my code:
private var menuConstraint: Constraint?
override func updateViewConstraints() {
super.updateViewConstraints()
menuVc.view.snp.makeConstraints { (make) in
self.menuConstraint = make.top.equalTo(view.snp.top).constraint
make.right.equalTo(view.snp.right)
make.width.equalTo(100)
make.height.equalTo(100)
}
}
@objc func onMenuTap() {
self.menuConstraint!.update(offset: 100)
}
When onMenuTap
is called I get the following error:
[LayoutConstraints] Unable to simultaneously satisfy constraints.
Probably at least one of the constraints in the following list is one you don't want.
Try this:
(1) look at each constraint and try to figure out which you don't expect;
(2) find the code that added the unwanted constraint or constraints and fix it.
(
"<SnapKit.LayoutConstraint:0xABC@MyViewController.swift#77 UIView:0xDEF.top == UIView:0xGHI.top>",
"<SnapKit.LayoutConstraint:0xABC@MyViewController.swift#77 UIView:0xDEF.top == UIView:0xGHI.top + 100.0>"
)
As you can see the previous version of the top constraint (without the offset) is conflicting with the new version. It's as if it didn't update the existing constraint but instead just created a new one.
I've tried a few variations:
- wrapping the update line in a
snp.updateConstraints
closure - setting an initial offset when first creating the constraint
- grabbing the underlying
LayoutConstraint
and updatingconstant
directly.
I always get the same error message.
Do I have something configured wrong?
ios swift autolayout uikit snapkit
add a comment |
I'm building an iOS app against iOS 12 SDK, Swift 4 and SnapKit 4.2
I want to update a constraint when I tap a button but it creates a conflict with the previous version of the constraint.
Here's my code:
private var menuConstraint: Constraint?
override func updateViewConstraints() {
super.updateViewConstraints()
menuVc.view.snp.makeConstraints { (make) in
self.menuConstraint = make.top.equalTo(view.snp.top).constraint
make.right.equalTo(view.snp.right)
make.width.equalTo(100)
make.height.equalTo(100)
}
}
@objc func onMenuTap() {
self.menuConstraint!.update(offset: 100)
}
When onMenuTap
is called I get the following error:
[LayoutConstraints] Unable to simultaneously satisfy constraints.
Probably at least one of the constraints in the following list is one you don't want.
Try this:
(1) look at each constraint and try to figure out which you don't expect;
(2) find the code that added the unwanted constraint or constraints and fix it.
(
"<SnapKit.LayoutConstraint:0xABC@MyViewController.swift#77 UIView:0xDEF.top == UIView:0xGHI.top>",
"<SnapKit.LayoutConstraint:0xABC@MyViewController.swift#77 UIView:0xDEF.top == UIView:0xGHI.top + 100.0>"
)
As you can see the previous version of the top constraint (without the offset) is conflicting with the new version. It's as if it didn't update the existing constraint but instead just created a new one.
I've tried a few variations:
- wrapping the update line in a
snp.updateConstraints
closure - setting an initial offset when first creating the constraint
- grabbing the underlying
LayoutConstraint
and updatingconstant
directly.
I always get the same error message.
Do I have something configured wrong?
ios swift autolayout uikit snapkit
add a comment |
I'm building an iOS app against iOS 12 SDK, Swift 4 and SnapKit 4.2
I want to update a constraint when I tap a button but it creates a conflict with the previous version of the constraint.
Here's my code:
private var menuConstraint: Constraint?
override func updateViewConstraints() {
super.updateViewConstraints()
menuVc.view.snp.makeConstraints { (make) in
self.menuConstraint = make.top.equalTo(view.snp.top).constraint
make.right.equalTo(view.snp.right)
make.width.equalTo(100)
make.height.equalTo(100)
}
}
@objc func onMenuTap() {
self.menuConstraint!.update(offset: 100)
}
When onMenuTap
is called I get the following error:
[LayoutConstraints] Unable to simultaneously satisfy constraints.
Probably at least one of the constraints in the following list is one you don't want.
Try this:
(1) look at each constraint and try to figure out which you don't expect;
(2) find the code that added the unwanted constraint or constraints and fix it.
(
"<SnapKit.LayoutConstraint:0xABC@MyViewController.swift#77 UIView:0xDEF.top == UIView:0xGHI.top>",
"<SnapKit.LayoutConstraint:0xABC@MyViewController.swift#77 UIView:0xDEF.top == UIView:0xGHI.top + 100.0>"
)
As you can see the previous version of the top constraint (without the offset) is conflicting with the new version. It's as if it didn't update the existing constraint but instead just created a new one.
I've tried a few variations:
- wrapping the update line in a
snp.updateConstraints
closure - setting an initial offset when first creating the constraint
- grabbing the underlying
LayoutConstraint
and updatingconstant
directly.
I always get the same error message.
Do I have something configured wrong?
ios swift autolayout uikit snapkit
I'm building an iOS app against iOS 12 SDK, Swift 4 and SnapKit 4.2
I want to update a constraint when I tap a button but it creates a conflict with the previous version of the constraint.
Here's my code:
private var menuConstraint: Constraint?
override func updateViewConstraints() {
super.updateViewConstraints()
menuVc.view.snp.makeConstraints { (make) in
self.menuConstraint = make.top.equalTo(view.snp.top).constraint
make.right.equalTo(view.snp.right)
make.width.equalTo(100)
make.height.equalTo(100)
}
}
@objc func onMenuTap() {
self.menuConstraint!.update(offset: 100)
}
When onMenuTap
is called I get the following error:
[LayoutConstraints] Unable to simultaneously satisfy constraints.
Probably at least one of the constraints in the following list is one you don't want.
Try this:
(1) look at each constraint and try to figure out which you don't expect;
(2) find the code that added the unwanted constraint or constraints and fix it.
(
"<SnapKit.LayoutConstraint:0xABC@MyViewController.swift#77 UIView:0xDEF.top == UIView:0xGHI.top>",
"<SnapKit.LayoutConstraint:0xABC@MyViewController.swift#77 UIView:0xDEF.top == UIView:0xGHI.top + 100.0>"
)
As you can see the previous version of the top constraint (without the offset) is conflicting with the new version. It's as if it didn't update the existing constraint but instead just created a new one.
I've tried a few variations:
- wrapping the update line in a
snp.updateConstraints
closure - setting an initial offset when first creating the constraint
- grabbing the underlying
LayoutConstraint
and updatingconstant
directly.
I always get the same error message.
Do I have something configured wrong?
ios swift autolayout uikit snapkit
ios swift autolayout uikit snapkit
asked Nov 25 '18 at 17:50
Adam LangsnerAdam Langsner
62111021
62111021
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Don't put constraints inside updateViewConstraints
as it'll recreate constraints as it's called multiple times so set the code inside viewDidLoad
1
That worked! thanks. I was callingupdateViewConstraints
from withinviewDidLoad
but I moved all the code directly insideviewDidLoad
and it fixed it. Thanks!
– Adam Langsner
Nov 25 '18 at 18:13
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53470239%2fsnapkit-updating-constraint-causes-conflict%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Don't put constraints inside updateViewConstraints
as it'll recreate constraints as it's called multiple times so set the code inside viewDidLoad
1
That worked! thanks. I was callingupdateViewConstraints
from withinviewDidLoad
but I moved all the code directly insideviewDidLoad
and it fixed it. Thanks!
– Adam Langsner
Nov 25 '18 at 18:13
add a comment |
Don't put constraints inside updateViewConstraints
as it'll recreate constraints as it's called multiple times so set the code inside viewDidLoad
1
That worked! thanks. I was callingupdateViewConstraints
from withinviewDidLoad
but I moved all the code directly insideviewDidLoad
and it fixed it. Thanks!
– Adam Langsner
Nov 25 '18 at 18:13
add a comment |
Don't put constraints inside updateViewConstraints
as it'll recreate constraints as it's called multiple times so set the code inside viewDidLoad
Don't put constraints inside updateViewConstraints
as it'll recreate constraints as it's called multiple times so set the code inside viewDidLoad
answered Nov 25 '18 at 18:09
Sh_KhanSh_Khan
44.4k51431
44.4k51431
1
That worked! thanks. I was callingupdateViewConstraints
from withinviewDidLoad
but I moved all the code directly insideviewDidLoad
and it fixed it. Thanks!
– Adam Langsner
Nov 25 '18 at 18:13
add a comment |
1
That worked! thanks. I was callingupdateViewConstraints
from withinviewDidLoad
but I moved all the code directly insideviewDidLoad
and it fixed it. Thanks!
– Adam Langsner
Nov 25 '18 at 18:13
1
1
That worked! thanks. I was calling
updateViewConstraints
from within viewDidLoad
but I moved all the code directly inside viewDidLoad
and it fixed it. Thanks!– Adam Langsner
Nov 25 '18 at 18:13
That worked! thanks. I was calling
updateViewConstraints
from within viewDidLoad
but I moved all the code directly inside viewDidLoad
and it fixed it. Thanks!– Adam Langsner
Nov 25 '18 at 18:13
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53470239%2fsnapkit-updating-constraint-causes-conflict%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
V8Z,a1AdMr 69ukLPF7bWNYWBVhLI31,M,g0RWkSRO91YO u,16PHL81O,e4V DBq55rJ,q90ndylvQWSZACEA1POAwxbTtsuXo