How to access/assert tuple key values in a python dictionary
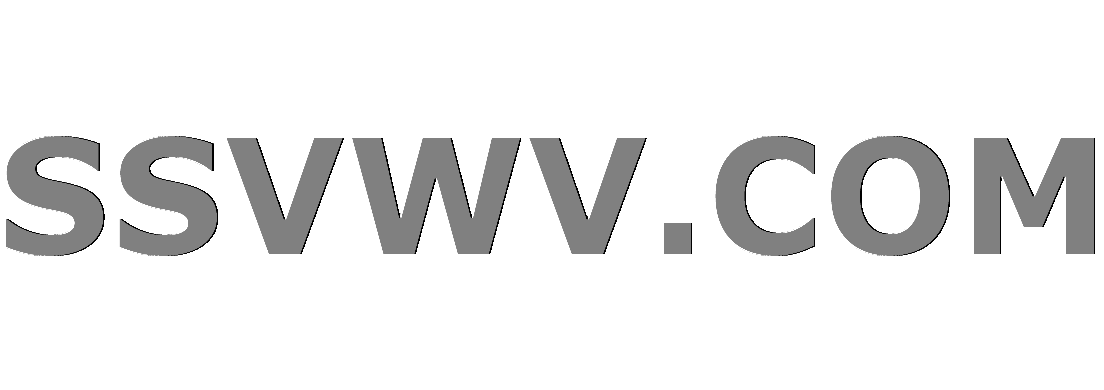
Multi tool use
How can I return "True" or "False" if 2 consecutive strings are in a dictionary key that is a tuple/triple?
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
I need an expression like:
return 'a', 'b' in d.keys()
python dictionary tuples
add a comment |
How can I return "True" or "False" if 2 consecutive strings are in a dictionary key that is a tuple/triple?
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
I need an expression like:
return 'a', 'b' in d.keys()
python dictionary tuples
It is supposed to check if 2 consecutive strings are in the key, that is - string 'a' followed by string 'b'
– user3383621
Nov 25 '18 at 18:23
add a comment |
How can I return "True" or "False" if 2 consecutive strings are in a dictionary key that is a tuple/triple?
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
I need an expression like:
return 'a', 'b' in d.keys()
python dictionary tuples
How can I return "True" or "False" if 2 consecutive strings are in a dictionary key that is a tuple/triple?
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
I need an expression like:
return 'a', 'b' in d.keys()
python dictionary tuples
python dictionary tuples
asked Nov 25 '18 at 18:02
user3383621user3383621
118112
118112
It is supposed to check if 2 consecutive strings are in the key, that is - string 'a' followed by string 'b'
– user3383621
Nov 25 '18 at 18:23
add a comment |
It is supposed to check if 2 consecutive strings are in the key, that is - string 'a' followed by string 'b'
– user3383621
Nov 25 '18 at 18:23
It is supposed to check if 2 consecutive strings are in the key, that is - string 'a' followed by string 'b'
– user3383621
Nov 25 '18 at 18:23
It is supposed to check if 2 consecutive strings are in the key, that is - string 'a' followed by string 'b'
– user3383621
Nov 25 '18 at 18:23
add a comment |
3 Answers
3
active
oldest
votes
You can do it with nested for loops:
def myFunc(myDict):
myKeys = list(myDict.keys())
for myList in myKeys:
myPreviousElement = None
for myElement in myList:
if myElement == myPreviousElement:
return True
myPreviousElement = myElement
return False
d = {(1, 'a', 'a') : 2, (4, 'c', 'd'):5}
print(myFunc(d)) # True
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
print(myFunc(d)) # False
Then you can customize return values how you prefer
I think there is a problem with this... if the triple is (‘a’, ‘b’, ‘a’) it give like they are consecutive
– Lorenzo Fiamingo
Nov 27 '18 at 9:16
add a comment |
You could pair elements for each key in the dictionary and then check if any of those pairs equals your desired result, eg:
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
# Check for existence of any key matching criteria
any(pair == ('a', 'b') for key in d for pair in zip(key, key[1:]))
# True
# Filter out keys/values matching criteria
{k: v for k, v in d.items() if any(p == ('a', 'b') for p in zip(k, k[1:]))}
# {(1, 'a', 'b'): 2}
add a comment |
this seems to work fine
for key in d:
return key[1] == string_1 and key[2] == string_2
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53470337%2fhow-to-access-assert-tuple-key-values-in-a-python-dictionary%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can do it with nested for loops:
def myFunc(myDict):
myKeys = list(myDict.keys())
for myList in myKeys:
myPreviousElement = None
for myElement in myList:
if myElement == myPreviousElement:
return True
myPreviousElement = myElement
return False
d = {(1, 'a', 'a') : 2, (4, 'c', 'd'):5}
print(myFunc(d)) # True
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
print(myFunc(d)) # False
Then you can customize return values how you prefer
I think there is a problem with this... if the triple is (‘a’, ‘b’, ‘a’) it give like they are consecutive
– Lorenzo Fiamingo
Nov 27 '18 at 9:16
add a comment |
You can do it with nested for loops:
def myFunc(myDict):
myKeys = list(myDict.keys())
for myList in myKeys:
myPreviousElement = None
for myElement in myList:
if myElement == myPreviousElement:
return True
myPreviousElement = myElement
return False
d = {(1, 'a', 'a') : 2, (4, 'c', 'd'):5}
print(myFunc(d)) # True
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
print(myFunc(d)) # False
Then you can customize return values how you prefer
I think there is a problem with this... if the triple is (‘a’, ‘b’, ‘a’) it give like they are consecutive
– Lorenzo Fiamingo
Nov 27 '18 at 9:16
add a comment |
You can do it with nested for loops:
def myFunc(myDict):
myKeys = list(myDict.keys())
for myList in myKeys:
myPreviousElement = None
for myElement in myList:
if myElement == myPreviousElement:
return True
myPreviousElement = myElement
return False
d = {(1, 'a', 'a') : 2, (4, 'c', 'd'):5}
print(myFunc(d)) # True
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
print(myFunc(d)) # False
Then you can customize return values how you prefer
You can do it with nested for loops:
def myFunc(myDict):
myKeys = list(myDict.keys())
for myList in myKeys:
myPreviousElement = None
for myElement in myList:
if myElement == myPreviousElement:
return True
myPreviousElement = myElement
return False
d = {(1, 'a', 'a') : 2, (4, 'c', 'd'):5}
print(myFunc(d)) # True
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
print(myFunc(d)) # False
Then you can customize return values how you prefer
answered Nov 25 '18 at 18:22
Lorenzo FiamingoLorenzo Fiamingo
849
849
I think there is a problem with this... if the triple is (‘a’, ‘b’, ‘a’) it give like they are consecutive
– Lorenzo Fiamingo
Nov 27 '18 at 9:16
add a comment |
I think there is a problem with this... if the triple is (‘a’, ‘b’, ‘a’) it give like they are consecutive
– Lorenzo Fiamingo
Nov 27 '18 at 9:16
I think there is a problem with this... if the triple is (‘a’, ‘b’, ‘a’) it give like they are consecutive
– Lorenzo Fiamingo
Nov 27 '18 at 9:16
I think there is a problem with this... if the triple is (‘a’, ‘b’, ‘a’) it give like they are consecutive
– Lorenzo Fiamingo
Nov 27 '18 at 9:16
add a comment |
You could pair elements for each key in the dictionary and then check if any of those pairs equals your desired result, eg:
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
# Check for existence of any key matching criteria
any(pair == ('a', 'b') for key in d for pair in zip(key, key[1:]))
# True
# Filter out keys/values matching criteria
{k: v for k, v in d.items() if any(p == ('a', 'b') for p in zip(k, k[1:]))}
# {(1, 'a', 'b'): 2}
add a comment |
You could pair elements for each key in the dictionary and then check if any of those pairs equals your desired result, eg:
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
# Check for existence of any key matching criteria
any(pair == ('a', 'b') for key in d for pair in zip(key, key[1:]))
# True
# Filter out keys/values matching criteria
{k: v for k, v in d.items() if any(p == ('a', 'b') for p in zip(k, k[1:]))}
# {(1, 'a', 'b'): 2}
add a comment |
You could pair elements for each key in the dictionary and then check if any of those pairs equals your desired result, eg:
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
# Check for existence of any key matching criteria
any(pair == ('a', 'b') for key in d for pair in zip(key, key[1:]))
# True
# Filter out keys/values matching criteria
{k: v for k, v in d.items() if any(p == ('a', 'b') for p in zip(k, k[1:]))}
# {(1, 'a', 'b'): 2}
You could pair elements for each key in the dictionary and then check if any of those pairs equals your desired result, eg:
d = {(1, 'a', 'b') : 2, (4, 'c', 'd'):5}
# Check for existence of any key matching criteria
any(pair == ('a', 'b') for key in d for pair in zip(key, key[1:]))
# True
# Filter out keys/values matching criteria
{k: v for k, v in d.items() if any(p == ('a', 'b') for p in zip(k, k[1:]))}
# {(1, 'a', 'b'): 2}
answered Nov 25 '18 at 18:28


Jon Clements♦Jon Clements
100k19175219
100k19175219
add a comment |
add a comment |
this seems to work fine
for key in d:
return key[1] == string_1 and key[2] == string_2
add a comment |
this seems to work fine
for key in d:
return key[1] == string_1 and key[2] == string_2
add a comment |
this seems to work fine
for key in d:
return key[1] == string_1 and key[2] == string_2
this seems to work fine
for key in d:
return key[1] == string_1 and key[2] == string_2
answered Nov 25 '18 at 19:05
user3383621user3383621
118112
118112
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53470337%2fhow-to-access-assert-tuple-key-values-in-a-python-dictionary%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
AwAQxacviYb PDoqAqTzmQxp,oWXVm2I,TclZ U00tXLTGwA,f p19,tpwQrWH
It is supposed to check if 2 consecutive strings are in the key, that is - string 'a' followed by string 'b'
– user3383621
Nov 25 '18 at 18:23