ImageView conditionals alternatives (Android Studio)
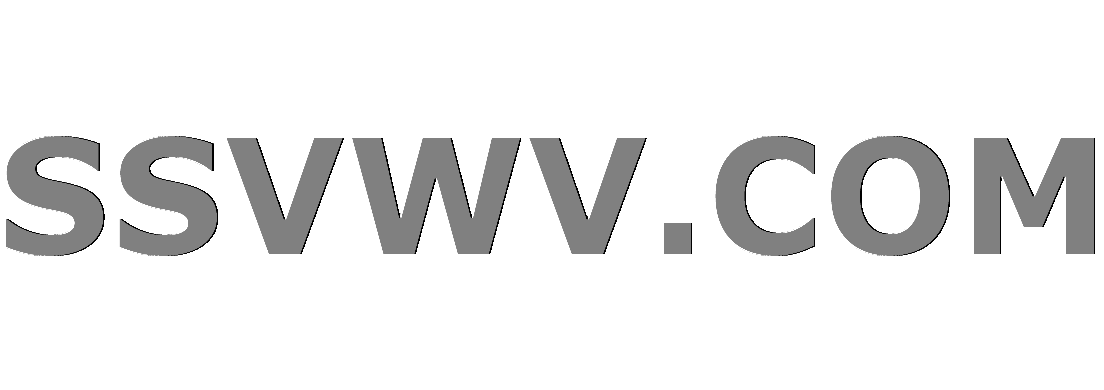
Multi tool use
I am a basic beginner at android studio and I am developing a weather app. I want to put conditionals for icon images to weather condition.
For example:
sun = sun image
rain = rain image
snow = snow image
I understand a switch case might be useful:
switch (id) {
case "Raining":
icon = "rainIcon";
break;
case "Snowing":
icon = "snowIcon";
break;
case "Drizzling":
icon = "drizzlingIcon";
break;
case "Foggy":
icon = "fogIcon";
break;
case "Thunderstorm":
icon = "thunderIcon;";
break;
case "Sunny":
icon = "sunIcon";
break;
}
However, I am confused how to implement it into my code as I am retrieving my data from my AsyncTask and need to implement the image change in that class but there is no proper method to change my ImageView variable there.
I understand there are ways to implement this on the onCreateView method but this is the way my code is implemented right now
java


add a comment |
I am a basic beginner at android studio and I am developing a weather app. I want to put conditionals for icon images to weather condition.
For example:
sun = sun image
rain = rain image
snow = snow image
I understand a switch case might be useful:
switch (id) {
case "Raining":
icon = "rainIcon";
break;
case "Snowing":
icon = "snowIcon";
break;
case "Drizzling":
icon = "drizzlingIcon";
break;
case "Foggy":
icon = "fogIcon";
break;
case "Thunderstorm":
icon = "thunderIcon;";
break;
case "Sunny":
icon = "sunIcon";
break;
}
However, I am confused how to implement it into my code as I am retrieving my data from my AsyncTask and need to implement the image change in that class but there is no proper method to change my ImageView variable there.
I understand there are ways to implement this on the onCreateView method but this is the way my code is implemented right now
java


add a comment |
I am a basic beginner at android studio and I am developing a weather app. I want to put conditionals for icon images to weather condition.
For example:
sun = sun image
rain = rain image
snow = snow image
I understand a switch case might be useful:
switch (id) {
case "Raining":
icon = "rainIcon";
break;
case "Snowing":
icon = "snowIcon";
break;
case "Drizzling":
icon = "drizzlingIcon";
break;
case "Foggy":
icon = "fogIcon";
break;
case "Thunderstorm":
icon = "thunderIcon;";
break;
case "Sunny":
icon = "sunIcon";
break;
}
However, I am confused how to implement it into my code as I am retrieving my data from my AsyncTask and need to implement the image change in that class but there is no proper method to change my ImageView variable there.
I understand there are ways to implement this on the onCreateView method but this is the way my code is implemented right now
java


I am a basic beginner at android studio and I am developing a weather app. I want to put conditionals for icon images to weather condition.
For example:
sun = sun image
rain = rain image
snow = snow image
I understand a switch case might be useful:
switch (id) {
case "Raining":
icon = "rainIcon";
break;
case "Snowing":
icon = "snowIcon";
break;
case "Drizzling":
icon = "drizzlingIcon";
break;
case "Foggy":
icon = "fogIcon";
break;
case "Thunderstorm":
icon = "thunderIcon;";
break;
case "Sunny":
icon = "sunIcon";
break;
}
However, I am confused how to implement it into my code as I am retrieving my data from my AsyncTask and need to implement the image change in that class but there is no proper method to change my ImageView variable there.
I understand there are ways to implement this on the onCreateView method but this is the way my code is implemented right now
java


java


edited Nov 27 '18 at 2:00
Pop Do
asked Nov 26 '18 at 2:26
Pop DoPop Do
133
133
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I suggest to create an enum of type Weathers.
public enum Weathers {
RAINING(R.drawable.ic_raining),
SNOWING(R.drawable.ic_snowing);
private int iconResId;
private Weathers(int iconResId) {
this.iconResId = iconResId;
}
public int getIconResId() {
return iconResId;
}
}
This might lessen the conditionals (The switch case above). To display into imageview, get an instance of the Weather instance by using Weathers.valuesOf(String)
where String
is upper-cased.
Weathers snowing = Weathers.valueOf("Snowing".toUpperCase(Locale.ENGLISH));
imageViewIcon.setImageResource(snowing.getIconResId());
I appreciate your help alot @Gero.
– Pop Do
Nov 26 '18 at 20:52
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474027%2fimageview-conditionals-alternatives-android-studio%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I suggest to create an enum of type Weathers.
public enum Weathers {
RAINING(R.drawable.ic_raining),
SNOWING(R.drawable.ic_snowing);
private int iconResId;
private Weathers(int iconResId) {
this.iconResId = iconResId;
}
public int getIconResId() {
return iconResId;
}
}
This might lessen the conditionals (The switch case above). To display into imageview, get an instance of the Weather instance by using Weathers.valuesOf(String)
where String
is upper-cased.
Weathers snowing = Weathers.valueOf("Snowing".toUpperCase(Locale.ENGLISH));
imageViewIcon.setImageResource(snowing.getIconResId());
I appreciate your help alot @Gero.
– Pop Do
Nov 26 '18 at 20:52
add a comment |
I suggest to create an enum of type Weathers.
public enum Weathers {
RAINING(R.drawable.ic_raining),
SNOWING(R.drawable.ic_snowing);
private int iconResId;
private Weathers(int iconResId) {
this.iconResId = iconResId;
}
public int getIconResId() {
return iconResId;
}
}
This might lessen the conditionals (The switch case above). To display into imageview, get an instance of the Weather instance by using Weathers.valuesOf(String)
where String
is upper-cased.
Weathers snowing = Weathers.valueOf("Snowing".toUpperCase(Locale.ENGLISH));
imageViewIcon.setImageResource(snowing.getIconResId());
I appreciate your help alot @Gero.
– Pop Do
Nov 26 '18 at 20:52
add a comment |
I suggest to create an enum of type Weathers.
public enum Weathers {
RAINING(R.drawable.ic_raining),
SNOWING(R.drawable.ic_snowing);
private int iconResId;
private Weathers(int iconResId) {
this.iconResId = iconResId;
}
public int getIconResId() {
return iconResId;
}
}
This might lessen the conditionals (The switch case above). To display into imageview, get an instance of the Weather instance by using Weathers.valuesOf(String)
where String
is upper-cased.
Weathers snowing = Weathers.valueOf("Snowing".toUpperCase(Locale.ENGLISH));
imageViewIcon.setImageResource(snowing.getIconResId());
I suggest to create an enum of type Weathers.
public enum Weathers {
RAINING(R.drawable.ic_raining),
SNOWING(R.drawable.ic_snowing);
private int iconResId;
private Weathers(int iconResId) {
this.iconResId = iconResId;
}
public int getIconResId() {
return iconResId;
}
}
This might lessen the conditionals (The switch case above). To display into imageview, get an instance of the Weather instance by using Weathers.valuesOf(String)
where String
is upper-cased.
Weathers snowing = Weathers.valueOf("Snowing".toUpperCase(Locale.ENGLISH));
imageViewIcon.setImageResource(snowing.getIconResId());
answered Nov 26 '18 at 3:00


GerosGeros
11.1k64145
11.1k64145
I appreciate your help alot @Gero.
– Pop Do
Nov 26 '18 at 20:52
add a comment |
I appreciate your help alot @Gero.
– Pop Do
Nov 26 '18 at 20:52
I appreciate your help alot @Gero.
– Pop Do
Nov 26 '18 at 20:52
I appreciate your help alot @Gero.
– Pop Do
Nov 26 '18 at 20:52
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474027%2fimageview-conditionals-alternatives-android-studio%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qVoP9R 9fZYdGs IVOckEvQ5xE1RJwly,R1aoLWPZ337qyv4Fs0Y