How to read and update the same document using angularfire2?
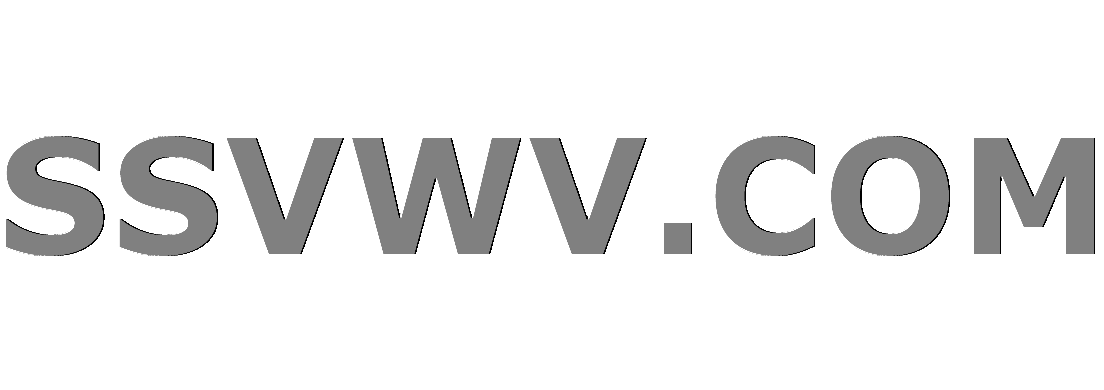
Multi tool use
I am using angularfire2
to try and update my Firestore document. I have a reference that I use valueChanges and then subscribe to get the data, but then since I'm updating the data inside the function it will keep calling it since the values change. I figured a 'hack' solution using first()
from rxjs/operators
, however, there has to be a better way to do this. Below is the code I am trying to implement.
this.storeCollectionRef = this.afs.collection('storeInfo');
this.storeDocumentRef= this.storeCollectionRef.doc<StoreInfo>(window.localStorage.getItem("uid"));
this.storeDocumentRef.valueChanges().subscribe(data=>{
console.log(data.itemcount);
var pictures = storage().ref(`${userid}/${data.itemcount+1}`);
pictures.putString(image, "data_url").then(()=>{
pictures.getDownloadURL().then(url => {
this.storeInfo.itemcount = data.itemcount+1;
this.storeInfo.image = url;
this.storeDocumentRef.update(this.storeInfo);
})
});
javascript firebase ionic3 google-cloud-firestore angularfire2
add a comment |
I am using angularfire2
to try and update my Firestore document. I have a reference that I use valueChanges and then subscribe to get the data, but then since I'm updating the data inside the function it will keep calling it since the values change. I figured a 'hack' solution using first()
from rxjs/operators
, however, there has to be a better way to do this. Below is the code I am trying to implement.
this.storeCollectionRef = this.afs.collection('storeInfo');
this.storeDocumentRef= this.storeCollectionRef.doc<StoreInfo>(window.localStorage.getItem("uid"));
this.storeDocumentRef.valueChanges().subscribe(data=>{
console.log(data.itemcount);
var pictures = storage().ref(`${userid}/${data.itemcount+1}`);
pictures.putString(image, "data_url").then(()=>{
pictures.getDownloadURL().then(url => {
this.storeInfo.itemcount = data.itemcount+1;
this.storeInfo.image = url;
this.storeDocumentRef.update(this.storeInfo);
})
});
javascript firebase ionic3 google-cloud-firestore angularfire2
add a comment |
I am using angularfire2
to try and update my Firestore document. I have a reference that I use valueChanges and then subscribe to get the data, but then since I'm updating the data inside the function it will keep calling it since the values change. I figured a 'hack' solution using first()
from rxjs/operators
, however, there has to be a better way to do this. Below is the code I am trying to implement.
this.storeCollectionRef = this.afs.collection('storeInfo');
this.storeDocumentRef= this.storeCollectionRef.doc<StoreInfo>(window.localStorage.getItem("uid"));
this.storeDocumentRef.valueChanges().subscribe(data=>{
console.log(data.itemcount);
var pictures = storage().ref(`${userid}/${data.itemcount+1}`);
pictures.putString(image, "data_url").then(()=>{
pictures.getDownloadURL().then(url => {
this.storeInfo.itemcount = data.itemcount+1;
this.storeInfo.image = url;
this.storeDocumentRef.update(this.storeInfo);
})
});
javascript firebase ionic3 google-cloud-firestore angularfire2
I am using angularfire2
to try and update my Firestore document. I have a reference that I use valueChanges and then subscribe to get the data, but then since I'm updating the data inside the function it will keep calling it since the values change. I figured a 'hack' solution using first()
from rxjs/operators
, however, there has to be a better way to do this. Below is the code I am trying to implement.
this.storeCollectionRef = this.afs.collection('storeInfo');
this.storeDocumentRef= this.storeCollectionRef.doc<StoreInfo>(window.localStorage.getItem("uid"));
this.storeDocumentRef.valueChanges().subscribe(data=>{
console.log(data.itemcount);
var pictures = storage().ref(`${userid}/${data.itemcount+1}`);
pictures.putString(image, "data_url").then(()=>{
pictures.getDownloadURL().then(url => {
this.storeInfo.itemcount = data.itemcount+1;
this.storeInfo.image = url;
this.storeDocumentRef.update(this.storeInfo);
})
});
this.storeCollectionRef = this.afs.collection('storeInfo');
this.storeDocumentRef= this.storeCollectionRef.doc<StoreInfo>(window.localStorage.getItem("uid"));
this.storeDocumentRef.valueChanges().subscribe(data=>{
console.log(data.itemcount);
var pictures = storage().ref(`${userid}/${data.itemcount+1}`);
pictures.putString(image, "data_url").then(()=>{
pictures.getDownloadURL().then(url => {
this.storeInfo.itemcount = data.itemcount+1;
this.storeInfo.image = url;
this.storeDocumentRef.update(this.storeInfo);
})
});
this.storeCollectionRef = this.afs.collection('storeInfo');
this.storeDocumentRef= this.storeCollectionRef.doc<StoreInfo>(window.localStorage.getItem("uid"));
this.storeDocumentRef.valueChanges().subscribe(data=>{
console.log(data.itemcount);
var pictures = storage().ref(`${userid}/${data.itemcount+1}`);
pictures.putString(image, "data_url").then(()=>{
pictures.getDownloadURL().then(url => {
this.storeInfo.itemcount = data.itemcount+1;
this.storeInfo.image = url;
this.storeDocumentRef.update(this.storeInfo);
})
});
javascript firebase ionic3 google-cloud-firestore angularfire2
javascript firebase ionic3 google-cloud-firestore angularfire2
edited Nov 26 '18 at 4:37
Rohan Dhar
1,1251318
1,1251318
asked Nov 26 '18 at 2:41
Tadas PetraitisTadas Petraitis
216
216
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Annoyingly, it's not included in the official AngularFire2 docs for working with Firestore documents. However, as you might know, AngularFire2 is just a wrapper around the original Firebase package - intended to make it easier to work with Firebase in an Angular environment. Checking out the docs for the original Firebase package provides this example of how to accomplish this goal with the basic Firebase package:
var docRef = db.collection("cities").doc("SF");
docRef.get().then(function(doc) {
if (doc.exists) {
console.log("Document data:", doc.data());
} else {
// doc.data() will be undefined in this case
console.log("No such document!");
}
}).catch(function(error) {
console.log("Error getting document:", error);
});
... which is pretty simple. If you were using the original Firebase package you could just get the reference to your document and call .get()
... if only AngularFire2 included that functionality! Digging a little deeper, it DOES appear to wrap that method too. Looking at the source code for AngularFire2 Firestore documents, waaayyyyyy at the bottom, the very last method, after valueChanges()
, is get()
! So it looks like it IS SUPPORTED just not documented.
/**
* Retrieve the document once.
* @param options
*/
get(options?: firestore.GetOptions) {
return from(this.ref.get(options)).pipe(
runInZone(this.afs.scheduler.zone)
);
}
I'm not in a position to test this myself, so I can't promise it will work. Try it and let me know. As a last resort if you can't get AF2 to behave, you could skip using the AngularFire2 package for that particular feature and also import the original Firebase package to that component, and just reference the document using the Firebase package's method instead.
EDIT:
Incase it wasn't clear, I'm suggesting you try something like:
this.storeCollectionRef = this.afs.collection('storeInfo');
this.storeDocumentRef= this.storeCollectionRef.doc<StoreInfo>(window.localStorage.getItem("uid"));
this.storeDocumentRef.get().then( data => {
console.log(data.itemcount);
// ... etc...
// continue doing your picture uploading stuff here
// ...
});
I appreciate your response. This was already something that I had tried. If you try that you end up getting an error that Property 'then' does not exist on type 'Observable<DocumentSnapshot>'
– Tadas Petraitis
Nov 27 '18 at 0:45
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474127%2fhow-to-read-and-update-the-same-document-using-angularfire2%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Annoyingly, it's not included in the official AngularFire2 docs for working with Firestore documents. However, as you might know, AngularFire2 is just a wrapper around the original Firebase package - intended to make it easier to work with Firebase in an Angular environment. Checking out the docs for the original Firebase package provides this example of how to accomplish this goal with the basic Firebase package:
var docRef = db.collection("cities").doc("SF");
docRef.get().then(function(doc) {
if (doc.exists) {
console.log("Document data:", doc.data());
} else {
// doc.data() will be undefined in this case
console.log("No such document!");
}
}).catch(function(error) {
console.log("Error getting document:", error);
});
... which is pretty simple. If you were using the original Firebase package you could just get the reference to your document and call .get()
... if only AngularFire2 included that functionality! Digging a little deeper, it DOES appear to wrap that method too. Looking at the source code for AngularFire2 Firestore documents, waaayyyyyy at the bottom, the very last method, after valueChanges()
, is get()
! So it looks like it IS SUPPORTED just not documented.
/**
* Retrieve the document once.
* @param options
*/
get(options?: firestore.GetOptions) {
return from(this.ref.get(options)).pipe(
runInZone(this.afs.scheduler.zone)
);
}
I'm not in a position to test this myself, so I can't promise it will work. Try it and let me know. As a last resort if you can't get AF2 to behave, you could skip using the AngularFire2 package for that particular feature and also import the original Firebase package to that component, and just reference the document using the Firebase package's method instead.
EDIT:
Incase it wasn't clear, I'm suggesting you try something like:
this.storeCollectionRef = this.afs.collection('storeInfo');
this.storeDocumentRef= this.storeCollectionRef.doc<StoreInfo>(window.localStorage.getItem("uid"));
this.storeDocumentRef.get().then( data => {
console.log(data.itemcount);
// ... etc...
// continue doing your picture uploading stuff here
// ...
});
I appreciate your response. This was already something that I had tried. If you try that you end up getting an error that Property 'then' does not exist on type 'Observable<DocumentSnapshot>'
– Tadas Petraitis
Nov 27 '18 at 0:45
add a comment |
Annoyingly, it's not included in the official AngularFire2 docs for working with Firestore documents. However, as you might know, AngularFire2 is just a wrapper around the original Firebase package - intended to make it easier to work with Firebase in an Angular environment. Checking out the docs for the original Firebase package provides this example of how to accomplish this goal with the basic Firebase package:
var docRef = db.collection("cities").doc("SF");
docRef.get().then(function(doc) {
if (doc.exists) {
console.log("Document data:", doc.data());
} else {
// doc.data() will be undefined in this case
console.log("No such document!");
}
}).catch(function(error) {
console.log("Error getting document:", error);
});
... which is pretty simple. If you were using the original Firebase package you could just get the reference to your document and call .get()
... if only AngularFire2 included that functionality! Digging a little deeper, it DOES appear to wrap that method too. Looking at the source code for AngularFire2 Firestore documents, waaayyyyyy at the bottom, the very last method, after valueChanges()
, is get()
! So it looks like it IS SUPPORTED just not documented.
/**
* Retrieve the document once.
* @param options
*/
get(options?: firestore.GetOptions) {
return from(this.ref.get(options)).pipe(
runInZone(this.afs.scheduler.zone)
);
}
I'm not in a position to test this myself, so I can't promise it will work. Try it and let me know. As a last resort if you can't get AF2 to behave, you could skip using the AngularFire2 package for that particular feature and also import the original Firebase package to that component, and just reference the document using the Firebase package's method instead.
EDIT:
Incase it wasn't clear, I'm suggesting you try something like:
this.storeCollectionRef = this.afs.collection('storeInfo');
this.storeDocumentRef= this.storeCollectionRef.doc<StoreInfo>(window.localStorage.getItem("uid"));
this.storeDocumentRef.get().then( data => {
console.log(data.itemcount);
// ... etc...
// continue doing your picture uploading stuff here
// ...
});
I appreciate your response. This was already something that I had tried. If you try that you end up getting an error that Property 'then' does not exist on type 'Observable<DocumentSnapshot>'
– Tadas Petraitis
Nov 27 '18 at 0:45
add a comment |
Annoyingly, it's not included in the official AngularFire2 docs for working with Firestore documents. However, as you might know, AngularFire2 is just a wrapper around the original Firebase package - intended to make it easier to work with Firebase in an Angular environment. Checking out the docs for the original Firebase package provides this example of how to accomplish this goal with the basic Firebase package:
var docRef = db.collection("cities").doc("SF");
docRef.get().then(function(doc) {
if (doc.exists) {
console.log("Document data:", doc.data());
} else {
// doc.data() will be undefined in this case
console.log("No such document!");
}
}).catch(function(error) {
console.log("Error getting document:", error);
});
... which is pretty simple. If you were using the original Firebase package you could just get the reference to your document and call .get()
... if only AngularFire2 included that functionality! Digging a little deeper, it DOES appear to wrap that method too. Looking at the source code for AngularFire2 Firestore documents, waaayyyyyy at the bottom, the very last method, after valueChanges()
, is get()
! So it looks like it IS SUPPORTED just not documented.
/**
* Retrieve the document once.
* @param options
*/
get(options?: firestore.GetOptions) {
return from(this.ref.get(options)).pipe(
runInZone(this.afs.scheduler.zone)
);
}
I'm not in a position to test this myself, so I can't promise it will work. Try it and let me know. As a last resort if you can't get AF2 to behave, you could skip using the AngularFire2 package for that particular feature and also import the original Firebase package to that component, and just reference the document using the Firebase package's method instead.
EDIT:
Incase it wasn't clear, I'm suggesting you try something like:
this.storeCollectionRef = this.afs.collection('storeInfo');
this.storeDocumentRef= this.storeCollectionRef.doc<StoreInfo>(window.localStorage.getItem("uid"));
this.storeDocumentRef.get().then( data => {
console.log(data.itemcount);
// ... etc...
// continue doing your picture uploading stuff here
// ...
});
Annoyingly, it's not included in the official AngularFire2 docs for working with Firestore documents. However, as you might know, AngularFire2 is just a wrapper around the original Firebase package - intended to make it easier to work with Firebase in an Angular environment. Checking out the docs for the original Firebase package provides this example of how to accomplish this goal with the basic Firebase package:
var docRef = db.collection("cities").doc("SF");
docRef.get().then(function(doc) {
if (doc.exists) {
console.log("Document data:", doc.data());
} else {
// doc.data() will be undefined in this case
console.log("No such document!");
}
}).catch(function(error) {
console.log("Error getting document:", error);
});
... which is pretty simple. If you were using the original Firebase package you could just get the reference to your document and call .get()
... if only AngularFire2 included that functionality! Digging a little deeper, it DOES appear to wrap that method too. Looking at the source code for AngularFire2 Firestore documents, waaayyyyyy at the bottom, the very last method, after valueChanges()
, is get()
! So it looks like it IS SUPPORTED just not documented.
/**
* Retrieve the document once.
* @param options
*/
get(options?: firestore.GetOptions) {
return from(this.ref.get(options)).pipe(
runInZone(this.afs.scheduler.zone)
);
}
I'm not in a position to test this myself, so I can't promise it will work. Try it and let me know. As a last resort if you can't get AF2 to behave, you could skip using the AngularFire2 package for that particular feature and also import the original Firebase package to that component, and just reference the document using the Firebase package's method instead.
EDIT:
Incase it wasn't clear, I'm suggesting you try something like:
this.storeCollectionRef = this.afs.collection('storeInfo');
this.storeDocumentRef= this.storeCollectionRef.doc<StoreInfo>(window.localStorage.getItem("uid"));
this.storeDocumentRef.get().then( data => {
console.log(data.itemcount);
// ... etc...
// continue doing your picture uploading stuff here
// ...
});
edited Nov 26 '18 at 15:52
answered Nov 26 '18 at 15:42
JeremyWJeremyW
1,903414
1,903414
I appreciate your response. This was already something that I had tried. If you try that you end up getting an error that Property 'then' does not exist on type 'Observable<DocumentSnapshot>'
– Tadas Petraitis
Nov 27 '18 at 0:45
add a comment |
I appreciate your response. This was already something that I had tried. If you try that you end up getting an error that Property 'then' does not exist on type 'Observable<DocumentSnapshot>'
– Tadas Petraitis
Nov 27 '18 at 0:45
I appreciate your response. This was already something that I had tried. If you try that you end up getting an error that Property 'then' does not exist on type 'Observable<DocumentSnapshot>'
– Tadas Petraitis
Nov 27 '18 at 0:45
I appreciate your response. This was already something that I had tried. If you try that you end up getting an error that Property 'then' does not exist on type 'Observable<DocumentSnapshot>'
– Tadas Petraitis
Nov 27 '18 at 0:45
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474127%2fhow-to-read-and-update-the-same-document-using-angularfire2%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
wNuopAq6G1t0ZHvMOOOp1C2GI7Ru PrDaFMv1837y20DlToz,4 ayjl7hltixTTEytit9P3fgN