How do I tell if a key is contained in a non-named Hashmap within a Hashmap? Java, JavaFX
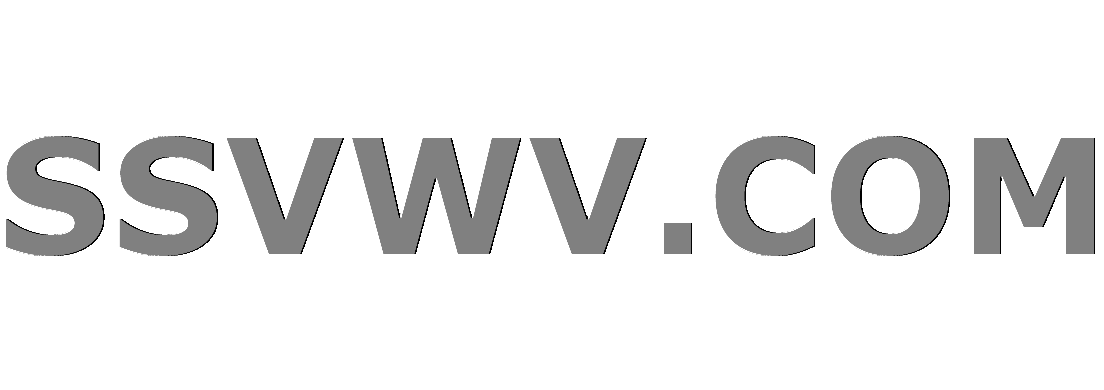
Multi tool use
I am currently working on an assignment for school where I am supposed to create a hashmap within a hashmap like this:
Map<String, Map<String, Integer>> girlsByYear = new HashMap<>();
Map<String, Map<String, Integer>> boysByYear = new HashMap<>();
According to the professor, there are not supposed to be any other maps needed to complete the assignment. The maps add elements to them by accessing files within a package that contain names, gender, and rank of baby names by year. When the program is compiled and finished, a JavaFX chart is created that requests a girl or boy's name to be entered. When entered, the chart shows by year the rank of how popular the name was.
Currently, I have figured most of it out, but I cannot understand how to access the Hashmap within the first Hashmap without a key for the first hashmap. By that, I mean that I am supposed to have a check performed by the textbox for the JavaFX class that reviews whether the name is in the Hashmap or not. Here is my code:
public class NameHelper {
// Declare the hash maps.
Map<String, Map<String, Integer>> girlsByYear = new HashMap<>();
Map<String, Map<String, Integer>> boysByYear = new HashMap<>();
// Declare addition variables.
String firstWord = "";
String secondWord = "";
String thirdWord = "";
Integer rank;
String fileName;
// This method will load the files from the data package, review the files,
// and add each item respectively to either map.
public void load() throws FileNotFoundException {
File dir = new File("src/data");
File files = dir.listFiles();
// for each file in the directory...
for (File f : files)
{
// Get the file name and split the year from it to add to each name.
String newFileName = f.getName();
fileName = newFileName.replaceAll("[yobtxt.]","");
Scanner scanner = new Scanner(f);
// While the files are not empty.
while(scanner.hasNextLine()) {
// If the second column split by a delimiter is M then add the information
// to the boys. Else girls.
String input = scanner.nextLine();
// Set the input to string values to enter into each hash map.
String initial = input.split(",")[1];
firstWord = fileName;
secondWord = (input.split(",")[0]).toLowerCase();
thirdWord = input.split(",")[2];
rank = Integer.parseInt(thirdWord);
// Use a switch statements since if statements aren't working.
switch(initial) {
case "M":
boysByYear.put(firstWord, new HashMap<String, Integer>());
boysByYear.get(firstWord).put(secondWord, rank);
break;
case "F":
girlsByYear.put(firstWord, new HashMap<String, Integer>());
girlsByYear.get(firstWord).put(secondWord, rank);
break;
default:
System.out.println("This is an issue");
break;
}
}
// Close the scanner.
scanner.close();
}
}
// This method will return a sorted set of years by getting the keyset from the hashmaps.
public Set<String> getYears() {
// Create the set.
Set<String> set = new HashSet<>();
// Add all the years of the listed by file name.
for(String key : girlsByYear.keySet()) {
set.add(key);
}
// Convert the set to a sorted set.
TreeSet<String> treeSet = new TreeSet<>(set);
return treeSet;
}
// This method will return true if the supplied name is found in the data structure.
// Use the gender input to determine which map to search by using "containsKey".
public boolean isNamePresent(String name, String gender) {
if(gender == "M") {
//Check if the name is within the map's map.
if(boysByYear.get(name).containsKey(name)) {
return true;
}
}
else if(gender == "F") {
if(girlsByYear.containsKey(name.toLowerCase())) {
return true;
}
}
return false;
}
The section that I need help with is the isNamePresent method. I need to check if the name is in the key of the second hashmap which is set up in this format (String year, HashMap(String name, Integer rank))
Any help or guidance would be greatly appreciated!
Additional notes: The JavaFx section for the chart was provided by the professor.
java javafx hashmap nested
add a comment |
I am currently working on an assignment for school where I am supposed to create a hashmap within a hashmap like this:
Map<String, Map<String, Integer>> girlsByYear = new HashMap<>();
Map<String, Map<String, Integer>> boysByYear = new HashMap<>();
According to the professor, there are not supposed to be any other maps needed to complete the assignment. The maps add elements to them by accessing files within a package that contain names, gender, and rank of baby names by year. When the program is compiled and finished, a JavaFX chart is created that requests a girl or boy's name to be entered. When entered, the chart shows by year the rank of how popular the name was.
Currently, I have figured most of it out, but I cannot understand how to access the Hashmap within the first Hashmap without a key for the first hashmap. By that, I mean that I am supposed to have a check performed by the textbox for the JavaFX class that reviews whether the name is in the Hashmap or not. Here is my code:
public class NameHelper {
// Declare the hash maps.
Map<String, Map<String, Integer>> girlsByYear = new HashMap<>();
Map<String, Map<String, Integer>> boysByYear = new HashMap<>();
// Declare addition variables.
String firstWord = "";
String secondWord = "";
String thirdWord = "";
Integer rank;
String fileName;
// This method will load the files from the data package, review the files,
// and add each item respectively to either map.
public void load() throws FileNotFoundException {
File dir = new File("src/data");
File files = dir.listFiles();
// for each file in the directory...
for (File f : files)
{
// Get the file name and split the year from it to add to each name.
String newFileName = f.getName();
fileName = newFileName.replaceAll("[yobtxt.]","");
Scanner scanner = new Scanner(f);
// While the files are not empty.
while(scanner.hasNextLine()) {
// If the second column split by a delimiter is M then add the information
// to the boys. Else girls.
String input = scanner.nextLine();
// Set the input to string values to enter into each hash map.
String initial = input.split(",")[1];
firstWord = fileName;
secondWord = (input.split(",")[0]).toLowerCase();
thirdWord = input.split(",")[2];
rank = Integer.parseInt(thirdWord);
// Use a switch statements since if statements aren't working.
switch(initial) {
case "M":
boysByYear.put(firstWord, new HashMap<String, Integer>());
boysByYear.get(firstWord).put(secondWord, rank);
break;
case "F":
girlsByYear.put(firstWord, new HashMap<String, Integer>());
girlsByYear.get(firstWord).put(secondWord, rank);
break;
default:
System.out.println("This is an issue");
break;
}
}
// Close the scanner.
scanner.close();
}
}
// This method will return a sorted set of years by getting the keyset from the hashmaps.
public Set<String> getYears() {
// Create the set.
Set<String> set = new HashSet<>();
// Add all the years of the listed by file name.
for(String key : girlsByYear.keySet()) {
set.add(key);
}
// Convert the set to a sorted set.
TreeSet<String> treeSet = new TreeSet<>(set);
return treeSet;
}
// This method will return true if the supplied name is found in the data structure.
// Use the gender input to determine which map to search by using "containsKey".
public boolean isNamePresent(String name, String gender) {
if(gender == "M") {
//Check if the name is within the map's map.
if(boysByYear.get(name).containsKey(name)) {
return true;
}
}
else if(gender == "F") {
if(girlsByYear.containsKey(name.toLowerCase())) {
return true;
}
}
return false;
}
The section that I need help with is the isNamePresent method. I need to check if the name is in the key of the second hashmap which is set up in this format (String year, HashMap(String name, Integer rank))
Any help or guidance would be greatly appreciated!
Additional notes: The JavaFx section for the chart was provided by the professor.
java javafx hashmap nested
You should not create inner map every cycle - you should check if the inner map has been created, and create it when needed.
– Jai
Nov 26 '18 at 4:58
add a comment |
I am currently working on an assignment for school where I am supposed to create a hashmap within a hashmap like this:
Map<String, Map<String, Integer>> girlsByYear = new HashMap<>();
Map<String, Map<String, Integer>> boysByYear = new HashMap<>();
According to the professor, there are not supposed to be any other maps needed to complete the assignment. The maps add elements to them by accessing files within a package that contain names, gender, and rank of baby names by year. When the program is compiled and finished, a JavaFX chart is created that requests a girl or boy's name to be entered. When entered, the chart shows by year the rank of how popular the name was.
Currently, I have figured most of it out, but I cannot understand how to access the Hashmap within the first Hashmap without a key for the first hashmap. By that, I mean that I am supposed to have a check performed by the textbox for the JavaFX class that reviews whether the name is in the Hashmap or not. Here is my code:
public class NameHelper {
// Declare the hash maps.
Map<String, Map<String, Integer>> girlsByYear = new HashMap<>();
Map<String, Map<String, Integer>> boysByYear = new HashMap<>();
// Declare addition variables.
String firstWord = "";
String secondWord = "";
String thirdWord = "";
Integer rank;
String fileName;
// This method will load the files from the data package, review the files,
// and add each item respectively to either map.
public void load() throws FileNotFoundException {
File dir = new File("src/data");
File files = dir.listFiles();
// for each file in the directory...
for (File f : files)
{
// Get the file name and split the year from it to add to each name.
String newFileName = f.getName();
fileName = newFileName.replaceAll("[yobtxt.]","");
Scanner scanner = new Scanner(f);
// While the files are not empty.
while(scanner.hasNextLine()) {
// If the second column split by a delimiter is M then add the information
// to the boys. Else girls.
String input = scanner.nextLine();
// Set the input to string values to enter into each hash map.
String initial = input.split(",")[1];
firstWord = fileName;
secondWord = (input.split(",")[0]).toLowerCase();
thirdWord = input.split(",")[2];
rank = Integer.parseInt(thirdWord);
// Use a switch statements since if statements aren't working.
switch(initial) {
case "M":
boysByYear.put(firstWord, new HashMap<String, Integer>());
boysByYear.get(firstWord).put(secondWord, rank);
break;
case "F":
girlsByYear.put(firstWord, new HashMap<String, Integer>());
girlsByYear.get(firstWord).put(secondWord, rank);
break;
default:
System.out.println("This is an issue");
break;
}
}
// Close the scanner.
scanner.close();
}
}
// This method will return a sorted set of years by getting the keyset from the hashmaps.
public Set<String> getYears() {
// Create the set.
Set<String> set = new HashSet<>();
// Add all the years of the listed by file name.
for(String key : girlsByYear.keySet()) {
set.add(key);
}
// Convert the set to a sorted set.
TreeSet<String> treeSet = new TreeSet<>(set);
return treeSet;
}
// This method will return true if the supplied name is found in the data structure.
// Use the gender input to determine which map to search by using "containsKey".
public boolean isNamePresent(String name, String gender) {
if(gender == "M") {
//Check if the name is within the map's map.
if(boysByYear.get(name).containsKey(name)) {
return true;
}
}
else if(gender == "F") {
if(girlsByYear.containsKey(name.toLowerCase())) {
return true;
}
}
return false;
}
The section that I need help with is the isNamePresent method. I need to check if the name is in the key of the second hashmap which is set up in this format (String year, HashMap(String name, Integer rank))
Any help or guidance would be greatly appreciated!
Additional notes: The JavaFx section for the chart was provided by the professor.
java javafx hashmap nested
I am currently working on an assignment for school where I am supposed to create a hashmap within a hashmap like this:
Map<String, Map<String, Integer>> girlsByYear = new HashMap<>();
Map<String, Map<String, Integer>> boysByYear = new HashMap<>();
According to the professor, there are not supposed to be any other maps needed to complete the assignment. The maps add elements to them by accessing files within a package that contain names, gender, and rank of baby names by year. When the program is compiled and finished, a JavaFX chart is created that requests a girl or boy's name to be entered. When entered, the chart shows by year the rank of how popular the name was.
Currently, I have figured most of it out, but I cannot understand how to access the Hashmap within the first Hashmap without a key for the first hashmap. By that, I mean that I am supposed to have a check performed by the textbox for the JavaFX class that reviews whether the name is in the Hashmap or not. Here is my code:
public class NameHelper {
// Declare the hash maps.
Map<String, Map<String, Integer>> girlsByYear = new HashMap<>();
Map<String, Map<String, Integer>> boysByYear = new HashMap<>();
// Declare addition variables.
String firstWord = "";
String secondWord = "";
String thirdWord = "";
Integer rank;
String fileName;
// This method will load the files from the data package, review the files,
// and add each item respectively to either map.
public void load() throws FileNotFoundException {
File dir = new File("src/data");
File files = dir.listFiles();
// for each file in the directory...
for (File f : files)
{
// Get the file name and split the year from it to add to each name.
String newFileName = f.getName();
fileName = newFileName.replaceAll("[yobtxt.]","");
Scanner scanner = new Scanner(f);
// While the files are not empty.
while(scanner.hasNextLine()) {
// If the second column split by a delimiter is M then add the information
// to the boys. Else girls.
String input = scanner.nextLine();
// Set the input to string values to enter into each hash map.
String initial = input.split(",")[1];
firstWord = fileName;
secondWord = (input.split(",")[0]).toLowerCase();
thirdWord = input.split(",")[2];
rank = Integer.parseInt(thirdWord);
// Use a switch statements since if statements aren't working.
switch(initial) {
case "M":
boysByYear.put(firstWord, new HashMap<String, Integer>());
boysByYear.get(firstWord).put(secondWord, rank);
break;
case "F":
girlsByYear.put(firstWord, new HashMap<String, Integer>());
girlsByYear.get(firstWord).put(secondWord, rank);
break;
default:
System.out.println("This is an issue");
break;
}
}
// Close the scanner.
scanner.close();
}
}
// This method will return a sorted set of years by getting the keyset from the hashmaps.
public Set<String> getYears() {
// Create the set.
Set<String> set = new HashSet<>();
// Add all the years of the listed by file name.
for(String key : girlsByYear.keySet()) {
set.add(key);
}
// Convert the set to a sorted set.
TreeSet<String> treeSet = new TreeSet<>(set);
return treeSet;
}
// This method will return true if the supplied name is found in the data structure.
// Use the gender input to determine which map to search by using "containsKey".
public boolean isNamePresent(String name, String gender) {
if(gender == "M") {
//Check if the name is within the map's map.
if(boysByYear.get(name).containsKey(name)) {
return true;
}
}
else if(gender == "F") {
if(girlsByYear.containsKey(name.toLowerCase())) {
return true;
}
}
return false;
}
The section that I need help with is the isNamePresent method. I need to check if the name is in the key of the second hashmap which is set up in this format (String year, HashMap(String name, Integer rank))
Any help or guidance would be greatly appreciated!
Additional notes: The JavaFx section for the chart was provided by the professor.
java javafx hashmap nested
java javafx hashmap nested
asked Nov 26 '18 at 2:34


Josh GreenertJosh Greenert
1
1
You should not create inner map every cycle - you should check if the inner map has been created, and create it when needed.
– Jai
Nov 26 '18 at 4:58
add a comment |
You should not create inner map every cycle - you should check if the inner map has been created, and create it when needed.
– Jai
Nov 26 '18 at 4:58
You should not create inner map every cycle - you should check if the inner map has been created, and create it when needed.
– Jai
Nov 26 '18 at 4:58
You should not create inner map every cycle - you should check if the inner map has been created, and create it when needed.
– Jai
Nov 26 '18 at 4:58
add a comment |
2 Answers
2
active
oldest
votes
One thing you need to fix first is comparing the strings using ==
. This doesn't work unless both the string passed as gender
parameter is a string literal. You need to use equals
instead, see How do I compare strings in Java? (switch
does this automatically).
Furthermore you should avoid duplicating code by retrieving the map to a local variable:
Map<String, Map<String, Integer>> map;
switch (gender) {
case "M":
map = boysByYear;
break;
case "F":
map = girlsByYear;
break;
default:
return false; // alternatively throw new IllegalArgumentException();
}
To find out, if at least one of the maps contains name
as a key, go through all the values and check the maps:
final String nameLower = name.toLowerCase();
return map.values().stream().anyMatch(m -> m.containsKey(nameLower));
BTW: You need to fix the way you read the data. Otherwise you'll get at most one name per year&gender, since you replace the Map
. Furthermore I recommend storing the result of split
instead of invoking it 3 times. Also don't use fields as variables only needed in a loop and choose more discriptive variable names:
Map<String, Integer> boys = new HashMap<>();
Map<String, Integer> girls = new HashMap<>();
boysByYear.put(fileName, boys);
girlsByYear.put(fileName, girls);
while(scanner.hasNextLine()) {
// If the second column split by a delimiter is M then add the information
// to the boys. Else girls.
String input = scanner.nextLine();
String parts = input.split(",");
// Set the input to string values to enter into each hash map.
String gender = parts[1];
String name = parts[0].toLowerCase();
int rank = Integer.parseInt(parts[2]);
switch(gender) {
case "M":
boys.put(name, rank);
break;
case "F":
girls.put(name, rank);
break;
default:
System.out.println("This is an issue");
break;
}
}
add a comment |
To access the inner hash map without knowing the key of the outer map, you can iterate over each entry of the outer map.
for(Map.Entry<String, Integer> mapEntry: boysByYear.entrySet()){
// Get the innerMap and check if the name exists
Map<String, Integer> innerMap = mapEntry.getValue();
if(innerMap.containsKey(name)){
return true;
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474076%2fhow-do-i-tell-if-a-key-is-contained-in-a-non-named-hashmap-within-a-hashmap-jav%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
One thing you need to fix first is comparing the strings using ==
. This doesn't work unless both the string passed as gender
parameter is a string literal. You need to use equals
instead, see How do I compare strings in Java? (switch
does this automatically).
Furthermore you should avoid duplicating code by retrieving the map to a local variable:
Map<String, Map<String, Integer>> map;
switch (gender) {
case "M":
map = boysByYear;
break;
case "F":
map = girlsByYear;
break;
default:
return false; // alternatively throw new IllegalArgumentException();
}
To find out, if at least one of the maps contains name
as a key, go through all the values and check the maps:
final String nameLower = name.toLowerCase();
return map.values().stream().anyMatch(m -> m.containsKey(nameLower));
BTW: You need to fix the way you read the data. Otherwise you'll get at most one name per year&gender, since you replace the Map
. Furthermore I recommend storing the result of split
instead of invoking it 3 times. Also don't use fields as variables only needed in a loop and choose more discriptive variable names:
Map<String, Integer> boys = new HashMap<>();
Map<String, Integer> girls = new HashMap<>();
boysByYear.put(fileName, boys);
girlsByYear.put(fileName, girls);
while(scanner.hasNextLine()) {
// If the second column split by a delimiter is M then add the information
// to the boys. Else girls.
String input = scanner.nextLine();
String parts = input.split(",");
// Set the input to string values to enter into each hash map.
String gender = parts[1];
String name = parts[0].toLowerCase();
int rank = Integer.parseInt(parts[2]);
switch(gender) {
case "M":
boys.put(name, rank);
break;
case "F":
girls.put(name, rank);
break;
default:
System.out.println("This is an issue");
break;
}
}
add a comment |
One thing you need to fix first is comparing the strings using ==
. This doesn't work unless both the string passed as gender
parameter is a string literal. You need to use equals
instead, see How do I compare strings in Java? (switch
does this automatically).
Furthermore you should avoid duplicating code by retrieving the map to a local variable:
Map<String, Map<String, Integer>> map;
switch (gender) {
case "M":
map = boysByYear;
break;
case "F":
map = girlsByYear;
break;
default:
return false; // alternatively throw new IllegalArgumentException();
}
To find out, if at least one of the maps contains name
as a key, go through all the values and check the maps:
final String nameLower = name.toLowerCase();
return map.values().stream().anyMatch(m -> m.containsKey(nameLower));
BTW: You need to fix the way you read the data. Otherwise you'll get at most one name per year&gender, since you replace the Map
. Furthermore I recommend storing the result of split
instead of invoking it 3 times. Also don't use fields as variables only needed in a loop and choose more discriptive variable names:
Map<String, Integer> boys = new HashMap<>();
Map<String, Integer> girls = new HashMap<>();
boysByYear.put(fileName, boys);
girlsByYear.put(fileName, girls);
while(scanner.hasNextLine()) {
// If the second column split by a delimiter is M then add the information
// to the boys. Else girls.
String input = scanner.nextLine();
String parts = input.split(",");
// Set the input to string values to enter into each hash map.
String gender = parts[1];
String name = parts[0].toLowerCase();
int rank = Integer.parseInt(parts[2]);
switch(gender) {
case "M":
boys.put(name, rank);
break;
case "F":
girls.put(name, rank);
break;
default:
System.out.println("This is an issue");
break;
}
}
add a comment |
One thing you need to fix first is comparing the strings using ==
. This doesn't work unless both the string passed as gender
parameter is a string literal. You need to use equals
instead, see How do I compare strings in Java? (switch
does this automatically).
Furthermore you should avoid duplicating code by retrieving the map to a local variable:
Map<String, Map<String, Integer>> map;
switch (gender) {
case "M":
map = boysByYear;
break;
case "F":
map = girlsByYear;
break;
default:
return false; // alternatively throw new IllegalArgumentException();
}
To find out, if at least one of the maps contains name
as a key, go through all the values and check the maps:
final String nameLower = name.toLowerCase();
return map.values().stream().anyMatch(m -> m.containsKey(nameLower));
BTW: You need to fix the way you read the data. Otherwise you'll get at most one name per year&gender, since you replace the Map
. Furthermore I recommend storing the result of split
instead of invoking it 3 times. Also don't use fields as variables only needed in a loop and choose more discriptive variable names:
Map<String, Integer> boys = new HashMap<>();
Map<String, Integer> girls = new HashMap<>();
boysByYear.put(fileName, boys);
girlsByYear.put(fileName, girls);
while(scanner.hasNextLine()) {
// If the second column split by a delimiter is M then add the information
// to the boys. Else girls.
String input = scanner.nextLine();
String parts = input.split(",");
// Set the input to string values to enter into each hash map.
String gender = parts[1];
String name = parts[0].toLowerCase();
int rank = Integer.parseInt(parts[2]);
switch(gender) {
case "M":
boys.put(name, rank);
break;
case "F":
girls.put(name, rank);
break;
default:
System.out.println("This is an issue");
break;
}
}
One thing you need to fix first is comparing the strings using ==
. This doesn't work unless both the string passed as gender
parameter is a string literal. You need to use equals
instead, see How do I compare strings in Java? (switch
does this automatically).
Furthermore you should avoid duplicating code by retrieving the map to a local variable:
Map<String, Map<String, Integer>> map;
switch (gender) {
case "M":
map = boysByYear;
break;
case "F":
map = girlsByYear;
break;
default:
return false; // alternatively throw new IllegalArgumentException();
}
To find out, if at least one of the maps contains name
as a key, go through all the values and check the maps:
final String nameLower = name.toLowerCase();
return map.values().stream().anyMatch(m -> m.containsKey(nameLower));
BTW: You need to fix the way you read the data. Otherwise you'll get at most one name per year&gender, since you replace the Map
. Furthermore I recommend storing the result of split
instead of invoking it 3 times. Also don't use fields as variables only needed in a loop and choose more discriptive variable names:
Map<String, Integer> boys = new HashMap<>();
Map<String, Integer> girls = new HashMap<>();
boysByYear.put(fileName, boys);
girlsByYear.put(fileName, girls);
while(scanner.hasNextLine()) {
// If the second column split by a delimiter is M then add the information
// to the boys. Else girls.
String input = scanner.nextLine();
String parts = input.split(",");
// Set the input to string values to enter into each hash map.
String gender = parts[1];
String name = parts[0].toLowerCase();
int rank = Integer.parseInt(parts[2]);
switch(gender) {
case "M":
boys.put(name, rank);
break;
case "F":
girls.put(name, rank);
break;
default:
System.out.println("This is an issue");
break;
}
}
answered Nov 26 '18 at 3:22
fabianfabian
52.6k115373
52.6k115373
add a comment |
add a comment |
To access the inner hash map without knowing the key of the outer map, you can iterate over each entry of the outer map.
for(Map.Entry<String, Integer> mapEntry: boysByYear.entrySet()){
// Get the innerMap and check if the name exists
Map<String, Integer> innerMap = mapEntry.getValue();
if(innerMap.containsKey(name)){
return true;
}
}
add a comment |
To access the inner hash map without knowing the key of the outer map, you can iterate over each entry of the outer map.
for(Map.Entry<String, Integer> mapEntry: boysByYear.entrySet()){
// Get the innerMap and check if the name exists
Map<String, Integer> innerMap = mapEntry.getValue();
if(innerMap.containsKey(name)){
return true;
}
}
add a comment |
To access the inner hash map without knowing the key of the outer map, you can iterate over each entry of the outer map.
for(Map.Entry<String, Integer> mapEntry: boysByYear.entrySet()){
// Get the innerMap and check if the name exists
Map<String, Integer> innerMap = mapEntry.getValue();
if(innerMap.containsKey(name)){
return true;
}
}
To access the inner hash map without knowing the key of the outer map, you can iterate over each entry of the outer map.
for(Map.Entry<String, Integer> mapEntry: boysByYear.entrySet()){
// Get the innerMap and check if the name exists
Map<String, Integer> innerMap = mapEntry.getValue();
if(innerMap.containsKey(name)){
return true;
}
}
answered Nov 26 '18 at 3:33
Aj05Aj05
413
413
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474076%2fhow-do-i-tell-if-a-key-is-contained-in-a-non-named-hashmap-within-a-hashmap-jav%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0rkasdUZRQAR
You should not create inner map every cycle - you should check if the inner map has been created, and create it when needed.
– Jai
Nov 26 '18 at 4:58