Snapshot test with Jest on nested react-navigation component - Object keys change so Snapshot match fails
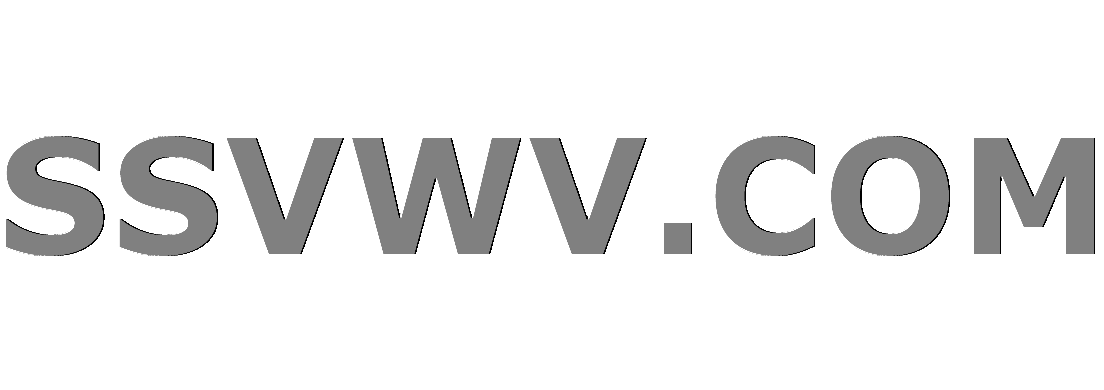
Multi tool use
When I run my jest test on my StackNavigator.test.js it always fails because of generated keys:
- Snapshot
+ Received
@@ -79,11 +79,11 @@
fromRoute={null}
index={0}
navigation={
Object {
"_childrenNavigation": Object {
- "**id-1542980055400-0**": Object {
+ "**id-1542980068677-0**": Object {
"actions": Object {
"dismiss": [Function],
"goBack": [Function],
"navigate": [Function],
"pop": [Function],
@@ -109,11 +109,11 @@
"replace": [Function],
"reset": [Function],
"router": undefined,
"setParams": [Function],
"state": Object {
- "key": "**id-1542980055400-0**",
+ "key": "**id-1542980068677-0**",
"routeName": "SignInOrRegister",
},
},
},
"actions": Object {
@@ -157,15 +157,15 @@
},
"setParams": [Function],
"state": Object {
"index": 0,
"isTransitioning": false,
- "key": "**id-1542980055400-1**",
+ "key": "**id-1542980068677-1**",
"routeName": "FluidTransitionNavigator",
"routes": Array [
Object {
- "key": "**id-1542980055400-0**",
+ "key": "**id-1542980068677-0**",
"routeName": "SignInOrRegister",
},
],
},
}
@@ -191,11 +191,11 @@
"overflow": "hidden",
},
undefined,
]
}
- toRoute="**id-1542980055400-0**"
+ toRoute="**id-1542980068677-0**"
>
<View
style={
Object {
"bottom": 0,
The StackNavigator component uses 'react-navigation' and has a nested FluidTransition component from 'react-navigation-fluid-transitions':
StackNavigator.js
import React, { Component } from 'react';
import { Platform, Animated, Easing } from 'react-native';
import { createStackNavigator } from 'react-navigation';
import { ThemeContext, getTheme } from 'react-native-material-ui';
import PropTypes from 'prop-types'
import FluidTransitionNavigator from './FluidTransitionNavigator';
import Dashboard from './../pages/Dashboard';
import Login from './../pages/Login';
import SignInOrRegister from './../pages/SignInOrRegister';
import UniToolbar from './UniToolbar';
const transitionConfig = () => {
return {
transitionSpec: {
duration: 1000,
easing: Easing.out(Easing.poly(4)),
timing: Animated.timing,
useNativeDriver: false,
},
screenInterpolator: sceneProps => {
const { layout, position, scene } = sceneProps
const thisSceneIndex = scene.index
const width = layout.initWidth
const translateX = position.interpolate({
inputRange: [thisSceneIndex - 1, thisSceneIndex],
outputRange: [width, 0],
})
return { transform: [ { translateX } ] }
},
}
}
const StackNavigator = createStackNavigator(
{
FluidTransitionNavigator: {
screen: FluidTransitionNavigator
},
Dashboard: {
screen: Dashboard
}
},
{
initialRouteName: 'FluidTransitionNavigator',
headerMode: 'float',
navigationOptions: (props) => ({
header: renderHeader(props)
}),
transitionConfig: transitionConfig
}
);
const renderHeader = (props) => {
let index = props.navigation.state.index;
const logout = (props.navigation.state.routeName === 'Dashboard');
let title = '';
switch (props.navigation.state.routeName) {
case 'Dashboard':
title = 'Dashboard';
break;
case 'FluidTransitionNavigator':
if (index !== undefined) {
switch (props.navigation.state.routes[index].routeName) {
case 'Login':
title = 'Sign In';
break;
case 'SignInOrRegister':
title = 'SignInOrRegister';
break;
default:
title = '';
}
}
break;
default:
title = '';
}
return (['SignInOrRegister', 'Sign In'].includes(title)) ? null : (
<ThemeContext.Provider value={getTheme(uiTheme)} >
<UniToolbar navigation={props.navigation} toolbarTitle={title} logout={logout} />
</ThemeContext.Provider>
);
};
renderHeader.propTypes = {
navigation: PropTypes.object
};
const uiTheme = {
toolbar: {
container: {
...Platform.select({
ios: {
height: 70
},
android: {
height: 76
}
})
},
},
};
export default StackNavigator;
Below is my test:
StackNavigator.test.js
import React from "react";
import renderer from "react-test-renderer";
import StackNavigator from "../StackNavigator";
test("renders correctly", () => {
const tree = renderer.create(<StackNavigator />).toJSON();
expect(tree).toMatchSnapshot();
});
I have seen a similar almost identical question here: Jest snapshot test failing due to react navigation generated key
The accepted answer still does not answer my question. It did however lead me down another rabbit hole:
I tried to use inline matching: expect(tree).toMatchInlineSnapshot()
to generate the tree after running yarn run test:unit
and then tried to insert Any
in place of all the keys. That did not work unfortunately.<String>
I'm stumped. I don't know how to resolve this. I have searched and searched, tried multiple things to get around it, I just can't solve this.
Please can someone lend me a hand?
react-native jestjs react-navigation
add a comment |
When I run my jest test on my StackNavigator.test.js it always fails because of generated keys:
- Snapshot
+ Received
@@ -79,11 +79,11 @@
fromRoute={null}
index={0}
navigation={
Object {
"_childrenNavigation": Object {
- "**id-1542980055400-0**": Object {
+ "**id-1542980068677-0**": Object {
"actions": Object {
"dismiss": [Function],
"goBack": [Function],
"navigate": [Function],
"pop": [Function],
@@ -109,11 +109,11 @@
"replace": [Function],
"reset": [Function],
"router": undefined,
"setParams": [Function],
"state": Object {
- "key": "**id-1542980055400-0**",
+ "key": "**id-1542980068677-0**",
"routeName": "SignInOrRegister",
},
},
},
"actions": Object {
@@ -157,15 +157,15 @@
},
"setParams": [Function],
"state": Object {
"index": 0,
"isTransitioning": false,
- "key": "**id-1542980055400-1**",
+ "key": "**id-1542980068677-1**",
"routeName": "FluidTransitionNavigator",
"routes": Array [
Object {
- "key": "**id-1542980055400-0**",
+ "key": "**id-1542980068677-0**",
"routeName": "SignInOrRegister",
},
],
},
}
@@ -191,11 +191,11 @@
"overflow": "hidden",
},
undefined,
]
}
- toRoute="**id-1542980055400-0**"
+ toRoute="**id-1542980068677-0**"
>
<View
style={
Object {
"bottom": 0,
The StackNavigator component uses 'react-navigation' and has a nested FluidTransition component from 'react-navigation-fluid-transitions':
StackNavigator.js
import React, { Component } from 'react';
import { Platform, Animated, Easing } from 'react-native';
import { createStackNavigator } from 'react-navigation';
import { ThemeContext, getTheme } from 'react-native-material-ui';
import PropTypes from 'prop-types'
import FluidTransitionNavigator from './FluidTransitionNavigator';
import Dashboard from './../pages/Dashboard';
import Login from './../pages/Login';
import SignInOrRegister from './../pages/SignInOrRegister';
import UniToolbar from './UniToolbar';
const transitionConfig = () => {
return {
transitionSpec: {
duration: 1000,
easing: Easing.out(Easing.poly(4)),
timing: Animated.timing,
useNativeDriver: false,
},
screenInterpolator: sceneProps => {
const { layout, position, scene } = sceneProps
const thisSceneIndex = scene.index
const width = layout.initWidth
const translateX = position.interpolate({
inputRange: [thisSceneIndex - 1, thisSceneIndex],
outputRange: [width, 0],
})
return { transform: [ { translateX } ] }
},
}
}
const StackNavigator = createStackNavigator(
{
FluidTransitionNavigator: {
screen: FluidTransitionNavigator
},
Dashboard: {
screen: Dashboard
}
},
{
initialRouteName: 'FluidTransitionNavigator',
headerMode: 'float',
navigationOptions: (props) => ({
header: renderHeader(props)
}),
transitionConfig: transitionConfig
}
);
const renderHeader = (props) => {
let index = props.navigation.state.index;
const logout = (props.navigation.state.routeName === 'Dashboard');
let title = '';
switch (props.navigation.state.routeName) {
case 'Dashboard':
title = 'Dashboard';
break;
case 'FluidTransitionNavigator':
if (index !== undefined) {
switch (props.navigation.state.routes[index].routeName) {
case 'Login':
title = 'Sign In';
break;
case 'SignInOrRegister':
title = 'SignInOrRegister';
break;
default:
title = '';
}
}
break;
default:
title = '';
}
return (['SignInOrRegister', 'Sign In'].includes(title)) ? null : (
<ThemeContext.Provider value={getTheme(uiTheme)} >
<UniToolbar navigation={props.navigation} toolbarTitle={title} logout={logout} />
</ThemeContext.Provider>
);
};
renderHeader.propTypes = {
navigation: PropTypes.object
};
const uiTheme = {
toolbar: {
container: {
...Platform.select({
ios: {
height: 70
},
android: {
height: 76
}
})
},
},
};
export default StackNavigator;
Below is my test:
StackNavigator.test.js
import React from "react";
import renderer from "react-test-renderer";
import StackNavigator from "../StackNavigator";
test("renders correctly", () => {
const tree = renderer.create(<StackNavigator />).toJSON();
expect(tree).toMatchSnapshot();
});
I have seen a similar almost identical question here: Jest snapshot test failing due to react navigation generated key
The accepted answer still does not answer my question. It did however lead me down another rabbit hole:
I tried to use inline matching: expect(tree).toMatchInlineSnapshot()
to generate the tree after running yarn run test:unit
and then tried to insert Any
in place of all the keys. That did not work unfortunately.<String>
I'm stumped. I don't know how to resolve this. I have searched and searched, tried multiple things to get around it, I just can't solve this.
Please can someone lend me a hand?
react-native jestjs react-navigation
add a comment |
When I run my jest test on my StackNavigator.test.js it always fails because of generated keys:
- Snapshot
+ Received
@@ -79,11 +79,11 @@
fromRoute={null}
index={0}
navigation={
Object {
"_childrenNavigation": Object {
- "**id-1542980055400-0**": Object {
+ "**id-1542980068677-0**": Object {
"actions": Object {
"dismiss": [Function],
"goBack": [Function],
"navigate": [Function],
"pop": [Function],
@@ -109,11 +109,11 @@
"replace": [Function],
"reset": [Function],
"router": undefined,
"setParams": [Function],
"state": Object {
- "key": "**id-1542980055400-0**",
+ "key": "**id-1542980068677-0**",
"routeName": "SignInOrRegister",
},
},
},
"actions": Object {
@@ -157,15 +157,15 @@
},
"setParams": [Function],
"state": Object {
"index": 0,
"isTransitioning": false,
- "key": "**id-1542980055400-1**",
+ "key": "**id-1542980068677-1**",
"routeName": "FluidTransitionNavigator",
"routes": Array [
Object {
- "key": "**id-1542980055400-0**",
+ "key": "**id-1542980068677-0**",
"routeName": "SignInOrRegister",
},
],
},
}
@@ -191,11 +191,11 @@
"overflow": "hidden",
},
undefined,
]
}
- toRoute="**id-1542980055400-0**"
+ toRoute="**id-1542980068677-0**"
>
<View
style={
Object {
"bottom": 0,
The StackNavigator component uses 'react-navigation' and has a nested FluidTransition component from 'react-navigation-fluid-transitions':
StackNavigator.js
import React, { Component } from 'react';
import { Platform, Animated, Easing } from 'react-native';
import { createStackNavigator } from 'react-navigation';
import { ThemeContext, getTheme } from 'react-native-material-ui';
import PropTypes from 'prop-types'
import FluidTransitionNavigator from './FluidTransitionNavigator';
import Dashboard from './../pages/Dashboard';
import Login from './../pages/Login';
import SignInOrRegister from './../pages/SignInOrRegister';
import UniToolbar from './UniToolbar';
const transitionConfig = () => {
return {
transitionSpec: {
duration: 1000,
easing: Easing.out(Easing.poly(4)),
timing: Animated.timing,
useNativeDriver: false,
},
screenInterpolator: sceneProps => {
const { layout, position, scene } = sceneProps
const thisSceneIndex = scene.index
const width = layout.initWidth
const translateX = position.interpolate({
inputRange: [thisSceneIndex - 1, thisSceneIndex],
outputRange: [width, 0],
})
return { transform: [ { translateX } ] }
},
}
}
const StackNavigator = createStackNavigator(
{
FluidTransitionNavigator: {
screen: FluidTransitionNavigator
},
Dashboard: {
screen: Dashboard
}
},
{
initialRouteName: 'FluidTransitionNavigator',
headerMode: 'float',
navigationOptions: (props) => ({
header: renderHeader(props)
}),
transitionConfig: transitionConfig
}
);
const renderHeader = (props) => {
let index = props.navigation.state.index;
const logout = (props.navigation.state.routeName === 'Dashboard');
let title = '';
switch (props.navigation.state.routeName) {
case 'Dashboard':
title = 'Dashboard';
break;
case 'FluidTransitionNavigator':
if (index !== undefined) {
switch (props.navigation.state.routes[index].routeName) {
case 'Login':
title = 'Sign In';
break;
case 'SignInOrRegister':
title = 'SignInOrRegister';
break;
default:
title = '';
}
}
break;
default:
title = '';
}
return (['SignInOrRegister', 'Sign In'].includes(title)) ? null : (
<ThemeContext.Provider value={getTheme(uiTheme)} >
<UniToolbar navigation={props.navigation} toolbarTitle={title} logout={logout} />
</ThemeContext.Provider>
);
};
renderHeader.propTypes = {
navigation: PropTypes.object
};
const uiTheme = {
toolbar: {
container: {
...Platform.select({
ios: {
height: 70
},
android: {
height: 76
}
})
},
},
};
export default StackNavigator;
Below is my test:
StackNavigator.test.js
import React from "react";
import renderer from "react-test-renderer";
import StackNavigator from "../StackNavigator";
test("renders correctly", () => {
const tree = renderer.create(<StackNavigator />).toJSON();
expect(tree).toMatchSnapshot();
});
I have seen a similar almost identical question here: Jest snapshot test failing due to react navigation generated key
The accepted answer still does not answer my question. It did however lead me down another rabbit hole:
I tried to use inline matching: expect(tree).toMatchInlineSnapshot()
to generate the tree after running yarn run test:unit
and then tried to insert Any
in place of all the keys. That did not work unfortunately.<String>
I'm stumped. I don't know how to resolve this. I have searched and searched, tried multiple things to get around it, I just can't solve this.
Please can someone lend me a hand?
react-native jestjs react-navigation
When I run my jest test on my StackNavigator.test.js it always fails because of generated keys:
- Snapshot
+ Received
@@ -79,11 +79,11 @@
fromRoute={null}
index={0}
navigation={
Object {
"_childrenNavigation": Object {
- "**id-1542980055400-0**": Object {
+ "**id-1542980068677-0**": Object {
"actions": Object {
"dismiss": [Function],
"goBack": [Function],
"navigate": [Function],
"pop": [Function],
@@ -109,11 +109,11 @@
"replace": [Function],
"reset": [Function],
"router": undefined,
"setParams": [Function],
"state": Object {
- "key": "**id-1542980055400-0**",
+ "key": "**id-1542980068677-0**",
"routeName": "SignInOrRegister",
},
},
},
"actions": Object {
@@ -157,15 +157,15 @@
},
"setParams": [Function],
"state": Object {
"index": 0,
"isTransitioning": false,
- "key": "**id-1542980055400-1**",
+ "key": "**id-1542980068677-1**",
"routeName": "FluidTransitionNavigator",
"routes": Array [
Object {
- "key": "**id-1542980055400-0**",
+ "key": "**id-1542980068677-0**",
"routeName": "SignInOrRegister",
},
],
},
}
@@ -191,11 +191,11 @@
"overflow": "hidden",
},
undefined,
]
}
- toRoute="**id-1542980055400-0**"
+ toRoute="**id-1542980068677-0**"
>
<View
style={
Object {
"bottom": 0,
The StackNavigator component uses 'react-navigation' and has a nested FluidTransition component from 'react-navigation-fluid-transitions':
StackNavigator.js
import React, { Component } from 'react';
import { Platform, Animated, Easing } from 'react-native';
import { createStackNavigator } from 'react-navigation';
import { ThemeContext, getTheme } from 'react-native-material-ui';
import PropTypes from 'prop-types'
import FluidTransitionNavigator from './FluidTransitionNavigator';
import Dashboard from './../pages/Dashboard';
import Login from './../pages/Login';
import SignInOrRegister from './../pages/SignInOrRegister';
import UniToolbar from './UniToolbar';
const transitionConfig = () => {
return {
transitionSpec: {
duration: 1000,
easing: Easing.out(Easing.poly(4)),
timing: Animated.timing,
useNativeDriver: false,
},
screenInterpolator: sceneProps => {
const { layout, position, scene } = sceneProps
const thisSceneIndex = scene.index
const width = layout.initWidth
const translateX = position.interpolate({
inputRange: [thisSceneIndex - 1, thisSceneIndex],
outputRange: [width, 0],
})
return { transform: [ { translateX } ] }
},
}
}
const StackNavigator = createStackNavigator(
{
FluidTransitionNavigator: {
screen: FluidTransitionNavigator
},
Dashboard: {
screen: Dashboard
}
},
{
initialRouteName: 'FluidTransitionNavigator',
headerMode: 'float',
navigationOptions: (props) => ({
header: renderHeader(props)
}),
transitionConfig: transitionConfig
}
);
const renderHeader = (props) => {
let index = props.navigation.state.index;
const logout = (props.navigation.state.routeName === 'Dashboard');
let title = '';
switch (props.navigation.state.routeName) {
case 'Dashboard':
title = 'Dashboard';
break;
case 'FluidTransitionNavigator':
if (index !== undefined) {
switch (props.navigation.state.routes[index].routeName) {
case 'Login':
title = 'Sign In';
break;
case 'SignInOrRegister':
title = 'SignInOrRegister';
break;
default:
title = '';
}
}
break;
default:
title = '';
}
return (['SignInOrRegister', 'Sign In'].includes(title)) ? null : (
<ThemeContext.Provider value={getTheme(uiTheme)} >
<UniToolbar navigation={props.navigation} toolbarTitle={title} logout={logout} />
</ThemeContext.Provider>
);
};
renderHeader.propTypes = {
navigation: PropTypes.object
};
const uiTheme = {
toolbar: {
container: {
...Platform.select({
ios: {
height: 70
},
android: {
height: 76
}
})
},
},
};
export default StackNavigator;
Below is my test:
StackNavigator.test.js
import React from "react";
import renderer from "react-test-renderer";
import StackNavigator from "../StackNavigator";
test("renders correctly", () => {
const tree = renderer.create(<StackNavigator />).toJSON();
expect(tree).toMatchSnapshot();
});
I have seen a similar almost identical question here: Jest snapshot test failing due to react navigation generated key
The accepted answer still does not answer my question. It did however lead me down another rabbit hole:
I tried to use inline matching: expect(tree).toMatchInlineSnapshot()
to generate the tree after running yarn run test:unit
and then tried to insert Any
in place of all the keys. That did not work unfortunately.<String>
I'm stumped. I don't know how to resolve this. I have searched and searched, tried multiple things to get around it, I just can't solve this.
Please can someone lend me a hand?
react-native jestjs react-navigation
react-native jestjs react-navigation
edited Nov 23 '18 at 15:21
skyboyer
3,61611128
3,61611128
asked Nov 23 '18 at 13:57


Kevin van ZylKevin van Zyl
107210
107210
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I solved my problem, but not by mocking Date.now which was suggested in many other cases.
Instead I adapted an answer I found on https://github.com/react-navigation/react-navigation/issues/2269#issuecomment-369318490 by a user named joeybaker.
The rationale for this was given as:
The keys aren't really important for your testing purposes. What you
really care about is the routes and the index.
His code is as follows and assumes the use of actions and reducers in Redux:
// keys are date and order-of-test based, so just removed them
const filterKeys = (state) => {
if (state.routes) {
return {
...state,
routes: state.routes.map((route) => {
const { key, ...others } = route
return filterKeys(others)
}),
}
}
return state
}
it('clears all other routes', () => {
const inputState = {}
const action = { type: AUTH_LOGOUT_SUCCESS }
const state = filterKeys(reducer(inputState, action))
expect(state.routes).toBe........
})
I have adapted this for my case (I do not use Redux yet) as follows:
test("renders correctly", () => {
const tree = renderer.create(<StackNavigator />);
const instance = tree.getInstance();
const state = filterKeys(instance.state.nav);
expect(state).toMatchSnapshot();
});
// keys are date and order-of-test based, so just removed them
const filterKeys = (state) => {
if (state.routes) {
return {
...state,
routes: state.routes.map((route) => {
const { key, ...others } = route
return filterKeys(others);
}),
}
}
return state;
};
The test that gets rendered looks like this:
// Jest Snapshot v1
exports[`renders correctly 1`] = `
Object {
"index": 0,
"isTransitioning": false,
"key": "StackRouterRoot",
"routes": Array [
Object {
"index": 0,
"isTransitioning": false,
"routeName": "FluidTransitionNavigator",
"routes": Array [
Object {
"routeName": "SignInOrRegister",
},
],
},
],
}
`;
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53448041%2fsnapshot-test-with-jest-on-nested-react-navigation-component-object-keys-chang%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I solved my problem, but not by mocking Date.now which was suggested in many other cases.
Instead I adapted an answer I found on https://github.com/react-navigation/react-navigation/issues/2269#issuecomment-369318490 by a user named joeybaker.
The rationale for this was given as:
The keys aren't really important for your testing purposes. What you
really care about is the routes and the index.
His code is as follows and assumes the use of actions and reducers in Redux:
// keys are date and order-of-test based, so just removed them
const filterKeys = (state) => {
if (state.routes) {
return {
...state,
routes: state.routes.map((route) => {
const { key, ...others } = route
return filterKeys(others)
}),
}
}
return state
}
it('clears all other routes', () => {
const inputState = {}
const action = { type: AUTH_LOGOUT_SUCCESS }
const state = filterKeys(reducer(inputState, action))
expect(state.routes).toBe........
})
I have adapted this for my case (I do not use Redux yet) as follows:
test("renders correctly", () => {
const tree = renderer.create(<StackNavigator />);
const instance = tree.getInstance();
const state = filterKeys(instance.state.nav);
expect(state).toMatchSnapshot();
});
// keys are date and order-of-test based, so just removed them
const filterKeys = (state) => {
if (state.routes) {
return {
...state,
routes: state.routes.map((route) => {
const { key, ...others } = route
return filterKeys(others);
}),
}
}
return state;
};
The test that gets rendered looks like this:
// Jest Snapshot v1
exports[`renders correctly 1`] = `
Object {
"index": 0,
"isTransitioning": false,
"key": "StackRouterRoot",
"routes": Array [
Object {
"index": 0,
"isTransitioning": false,
"routeName": "FluidTransitionNavigator",
"routes": Array [
Object {
"routeName": "SignInOrRegister",
},
],
},
],
}
`;
add a comment |
I solved my problem, but not by mocking Date.now which was suggested in many other cases.
Instead I adapted an answer I found on https://github.com/react-navigation/react-navigation/issues/2269#issuecomment-369318490 by a user named joeybaker.
The rationale for this was given as:
The keys aren't really important for your testing purposes. What you
really care about is the routes and the index.
His code is as follows and assumes the use of actions and reducers in Redux:
// keys are date and order-of-test based, so just removed them
const filterKeys = (state) => {
if (state.routes) {
return {
...state,
routes: state.routes.map((route) => {
const { key, ...others } = route
return filterKeys(others)
}),
}
}
return state
}
it('clears all other routes', () => {
const inputState = {}
const action = { type: AUTH_LOGOUT_SUCCESS }
const state = filterKeys(reducer(inputState, action))
expect(state.routes).toBe........
})
I have adapted this for my case (I do not use Redux yet) as follows:
test("renders correctly", () => {
const tree = renderer.create(<StackNavigator />);
const instance = tree.getInstance();
const state = filterKeys(instance.state.nav);
expect(state).toMatchSnapshot();
});
// keys are date and order-of-test based, so just removed them
const filterKeys = (state) => {
if (state.routes) {
return {
...state,
routes: state.routes.map((route) => {
const { key, ...others } = route
return filterKeys(others);
}),
}
}
return state;
};
The test that gets rendered looks like this:
// Jest Snapshot v1
exports[`renders correctly 1`] = `
Object {
"index": 0,
"isTransitioning": false,
"key": "StackRouterRoot",
"routes": Array [
Object {
"index": 0,
"isTransitioning": false,
"routeName": "FluidTransitionNavigator",
"routes": Array [
Object {
"routeName": "SignInOrRegister",
},
],
},
],
}
`;
add a comment |
I solved my problem, but not by mocking Date.now which was suggested in many other cases.
Instead I adapted an answer I found on https://github.com/react-navigation/react-navigation/issues/2269#issuecomment-369318490 by a user named joeybaker.
The rationale for this was given as:
The keys aren't really important for your testing purposes. What you
really care about is the routes and the index.
His code is as follows and assumes the use of actions and reducers in Redux:
// keys are date and order-of-test based, so just removed them
const filterKeys = (state) => {
if (state.routes) {
return {
...state,
routes: state.routes.map((route) => {
const { key, ...others } = route
return filterKeys(others)
}),
}
}
return state
}
it('clears all other routes', () => {
const inputState = {}
const action = { type: AUTH_LOGOUT_SUCCESS }
const state = filterKeys(reducer(inputState, action))
expect(state.routes).toBe........
})
I have adapted this for my case (I do not use Redux yet) as follows:
test("renders correctly", () => {
const tree = renderer.create(<StackNavigator />);
const instance = tree.getInstance();
const state = filterKeys(instance.state.nav);
expect(state).toMatchSnapshot();
});
// keys are date and order-of-test based, so just removed them
const filterKeys = (state) => {
if (state.routes) {
return {
...state,
routes: state.routes.map((route) => {
const { key, ...others } = route
return filterKeys(others);
}),
}
}
return state;
};
The test that gets rendered looks like this:
// Jest Snapshot v1
exports[`renders correctly 1`] = `
Object {
"index": 0,
"isTransitioning": false,
"key": "StackRouterRoot",
"routes": Array [
Object {
"index": 0,
"isTransitioning": false,
"routeName": "FluidTransitionNavigator",
"routes": Array [
Object {
"routeName": "SignInOrRegister",
},
],
},
],
}
`;
I solved my problem, but not by mocking Date.now which was suggested in many other cases.
Instead I adapted an answer I found on https://github.com/react-navigation/react-navigation/issues/2269#issuecomment-369318490 by a user named joeybaker.
The rationale for this was given as:
The keys aren't really important for your testing purposes. What you
really care about is the routes and the index.
His code is as follows and assumes the use of actions and reducers in Redux:
// keys are date and order-of-test based, so just removed them
const filterKeys = (state) => {
if (state.routes) {
return {
...state,
routes: state.routes.map((route) => {
const { key, ...others } = route
return filterKeys(others)
}),
}
}
return state
}
it('clears all other routes', () => {
const inputState = {}
const action = { type: AUTH_LOGOUT_SUCCESS }
const state = filterKeys(reducer(inputState, action))
expect(state.routes).toBe........
})
I have adapted this for my case (I do not use Redux yet) as follows:
test("renders correctly", () => {
const tree = renderer.create(<StackNavigator />);
const instance = tree.getInstance();
const state = filterKeys(instance.state.nav);
expect(state).toMatchSnapshot();
});
// keys are date and order-of-test based, so just removed them
const filterKeys = (state) => {
if (state.routes) {
return {
...state,
routes: state.routes.map((route) => {
const { key, ...others } = route
return filterKeys(others);
}),
}
}
return state;
};
The test that gets rendered looks like this:
// Jest Snapshot v1
exports[`renders correctly 1`] = `
Object {
"index": 0,
"isTransitioning": false,
"key": "StackRouterRoot",
"routes": Array [
Object {
"index": 0,
"isTransitioning": false,
"routeName": "FluidTransitionNavigator",
"routes": Array [
Object {
"routeName": "SignInOrRegister",
},
],
},
],
}
`;
answered Nov 24 '18 at 17:14


Kevin van ZylKevin van Zyl
107210
107210
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53448041%2fsnapshot-test-with-jest-on-nested-react-navigation-component-object-keys-chang%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8UMojv9UI7Z6w 8bdtbhC3oG6