NestJS : Inject Service into Models / Entities
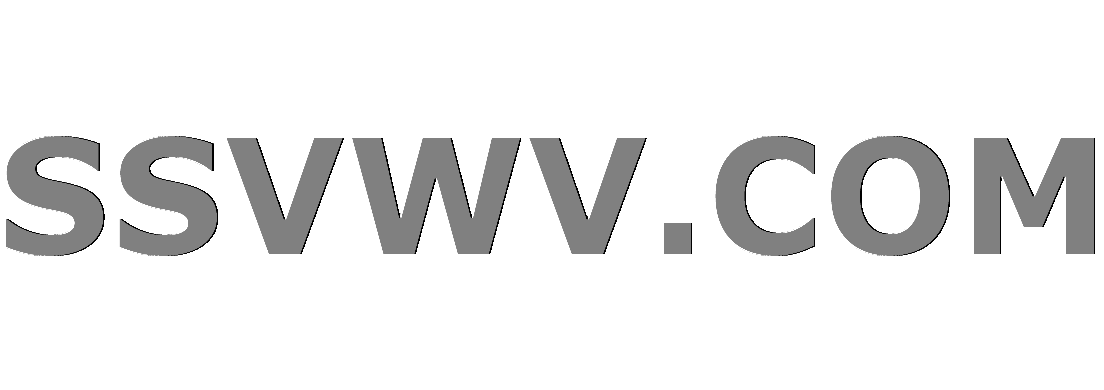
Multi tool use
i am currently stuck on a problem, which i don't know how to propery solve:
In my NestJS application, I would like to make all my TypeORM Entities
extend a BaseEntity
class that provide some general features. For example, i would like to provide an additional getHashedID()
method that hashed (and therefore hides) the internal ID from my API customers.
Hashing is done by a HashIdService
, which provides an encode()
and decode()
method.
My setup looks like this (removed the Decorators for readability!):
export class User extends BaseEntity {
id: int;
email: string;
name: string;
// ...
}
export class BaseEntity {
@Inject(HashIdService) private readonly hashids: HashIdService;
getHashedId() {
return this.hashids.encode(this.id);
}
}
However, if i call the this.hashids.encode()
method, it throws an exception with:
Cannot read property 'encode' of undefined
How can i inject
a service into a entity/model
class? Is this even possible?
UPDATE #1
In particular, i would like to "inject" the HashIdService
into my Entities
. Further, the Entities
should have a getHashedId()
method that returns their hashed ID.. As i don't want to do this "over and over again", i would like to "hide" this method in the BaseEntity
as described above..
My current NestJS version is as follows:
Nest version:
+-- @nestjs/common@5.4.0
+-- @nestjs/core@5.4.0
+-- @nestjs/microservices@5.4.0
+-- @nestjs/testing@5.4.0
+-- @nestjs/websockets@5.4.0
Thank you very much for your help!
dependency-injection entity nestjs
add a comment |
i am currently stuck on a problem, which i don't know how to propery solve:
In my NestJS application, I would like to make all my TypeORM Entities
extend a BaseEntity
class that provide some general features. For example, i would like to provide an additional getHashedID()
method that hashed (and therefore hides) the internal ID from my API customers.
Hashing is done by a HashIdService
, which provides an encode()
and decode()
method.
My setup looks like this (removed the Decorators for readability!):
export class User extends BaseEntity {
id: int;
email: string;
name: string;
// ...
}
export class BaseEntity {
@Inject(HashIdService) private readonly hashids: HashIdService;
getHashedId() {
return this.hashids.encode(this.id);
}
}
However, if i call the this.hashids.encode()
method, it throws an exception with:
Cannot read property 'encode' of undefined
How can i inject
a service into a entity/model
class? Is this even possible?
UPDATE #1
In particular, i would like to "inject" the HashIdService
into my Entities
. Further, the Entities
should have a getHashedId()
method that returns their hashed ID.. As i don't want to do this "over and over again", i would like to "hide" this method in the BaseEntity
as described above..
My current NestJS version is as follows:
Nest version:
+-- @nestjs/common@5.4.0
+-- @nestjs/core@5.4.0
+-- @nestjs/microservices@5.4.0
+-- @nestjs/testing@5.4.0
+-- @nestjs/websockets@5.4.0
Thank you very much for your help!
dependency-injection entity nestjs
Why is it necessary that this particular service gets injected as opposed to just referencing a utility function?
– Jesse Carter
Nov 23 '18 at 14:25
@JesseCarter Maybe it needs something like access to DB which is otherwise injected?
– Lazar Ljubenović
Nov 24 '18 at 17:02
@JesseCarter I just updated my initial question. I would like to inject theHashIdService
so my entites are able to hash their own ID.. How would you solve this issue? What do you mean with a "utility function"?
– zorn
Nov 25 '18 at 11:07
add a comment |
i am currently stuck on a problem, which i don't know how to propery solve:
In my NestJS application, I would like to make all my TypeORM Entities
extend a BaseEntity
class that provide some general features. For example, i would like to provide an additional getHashedID()
method that hashed (and therefore hides) the internal ID from my API customers.
Hashing is done by a HashIdService
, which provides an encode()
and decode()
method.
My setup looks like this (removed the Decorators for readability!):
export class User extends BaseEntity {
id: int;
email: string;
name: string;
// ...
}
export class BaseEntity {
@Inject(HashIdService) private readonly hashids: HashIdService;
getHashedId() {
return this.hashids.encode(this.id);
}
}
However, if i call the this.hashids.encode()
method, it throws an exception with:
Cannot read property 'encode' of undefined
How can i inject
a service into a entity/model
class? Is this even possible?
UPDATE #1
In particular, i would like to "inject" the HashIdService
into my Entities
. Further, the Entities
should have a getHashedId()
method that returns their hashed ID.. As i don't want to do this "over and over again", i would like to "hide" this method in the BaseEntity
as described above..
My current NestJS version is as follows:
Nest version:
+-- @nestjs/common@5.4.0
+-- @nestjs/core@5.4.0
+-- @nestjs/microservices@5.4.0
+-- @nestjs/testing@5.4.0
+-- @nestjs/websockets@5.4.0
Thank you very much for your help!
dependency-injection entity nestjs
i am currently stuck on a problem, which i don't know how to propery solve:
In my NestJS application, I would like to make all my TypeORM Entities
extend a BaseEntity
class that provide some general features. For example, i would like to provide an additional getHashedID()
method that hashed (and therefore hides) the internal ID from my API customers.
Hashing is done by a HashIdService
, which provides an encode()
and decode()
method.
My setup looks like this (removed the Decorators for readability!):
export class User extends BaseEntity {
id: int;
email: string;
name: string;
// ...
}
export class BaseEntity {
@Inject(HashIdService) private readonly hashids: HashIdService;
getHashedId() {
return this.hashids.encode(this.id);
}
}
However, if i call the this.hashids.encode()
method, it throws an exception with:
Cannot read property 'encode' of undefined
How can i inject
a service into a entity/model
class? Is this even possible?
UPDATE #1
In particular, i would like to "inject" the HashIdService
into my Entities
. Further, the Entities
should have a getHashedId()
method that returns their hashed ID.. As i don't want to do this "over and over again", i would like to "hide" this method in the BaseEntity
as described above..
My current NestJS version is as follows:
Nest version:
+-- @nestjs/common@5.4.0
+-- @nestjs/core@5.4.0
+-- @nestjs/microservices@5.4.0
+-- @nestjs/testing@5.4.0
+-- @nestjs/websockets@5.4.0
Thank you very much for your help!
dependency-injection entity nestjs
dependency-injection entity nestjs
edited Nov 25 '18 at 11:06
zorn
asked Nov 23 '18 at 9:10
zornzorn
11
11
Why is it necessary that this particular service gets injected as opposed to just referencing a utility function?
– Jesse Carter
Nov 23 '18 at 14:25
@JesseCarter Maybe it needs something like access to DB which is otherwise injected?
– Lazar Ljubenović
Nov 24 '18 at 17:02
@JesseCarter I just updated my initial question. I would like to inject theHashIdService
so my entites are able to hash their own ID.. How would you solve this issue? What do you mean with a "utility function"?
– zorn
Nov 25 '18 at 11:07
add a comment |
Why is it necessary that this particular service gets injected as opposed to just referencing a utility function?
– Jesse Carter
Nov 23 '18 at 14:25
@JesseCarter Maybe it needs something like access to DB which is otherwise injected?
– Lazar Ljubenović
Nov 24 '18 at 17:02
@JesseCarter I just updated my initial question. I would like to inject theHashIdService
so my entites are able to hash their own ID.. How would you solve this issue? What do you mean with a "utility function"?
– zorn
Nov 25 '18 at 11:07
Why is it necessary that this particular service gets injected as opposed to just referencing a utility function?
– Jesse Carter
Nov 23 '18 at 14:25
Why is it necessary that this particular service gets injected as opposed to just referencing a utility function?
– Jesse Carter
Nov 23 '18 at 14:25
@JesseCarter Maybe it needs something like access to DB which is otherwise injected?
– Lazar Ljubenović
Nov 24 '18 at 17:02
@JesseCarter Maybe it needs something like access to DB which is otherwise injected?
– Lazar Ljubenović
Nov 24 '18 at 17:02
@JesseCarter I just updated my initial question. I would like to inject the
HashIdService
so my entites are able to hash their own ID.. How would you solve this issue? What do you mean with a "utility function"?– zorn
Nov 25 '18 at 11:07
@JesseCarter I just updated my initial question. I would like to inject the
HashIdService
so my entites are able to hash their own ID.. How would you solve this issue? What do you mean with a "utility function"?– zorn
Nov 25 '18 at 11:07
add a comment |
1 Answer
1
active
oldest
votes
Dependency Injection can be a very useful technique especially when it comes to testing but for something simple like hasing an Id you could easily just export a function from somewhere and then simply import it in your BaseEntity. If it's only ever used by your BaseEntity you could even just have it in the same file. Trying to inject this behavior with a service seems like overkill.
// BaseEntity.ts
function encodeHash() {
// logic for hashing
}
class BaseEntity {
public getHashedId() {
return encodeHash(this.id).
}
}
1
Dear @JesseCarter , thank you very much for your response. However, how do you handle it, if theencodeHash
function depends on a 3rd-party service? E.g., hashing itself is provided byHashIds
package, and theHashIds
package is configured via config-params from aConfigService
..
– zorn
Nov 26 '18 at 16:29
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53443592%2fnestjs-inject-service-into-models-entities%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Dependency Injection can be a very useful technique especially when it comes to testing but for something simple like hasing an Id you could easily just export a function from somewhere and then simply import it in your BaseEntity. If it's only ever used by your BaseEntity you could even just have it in the same file. Trying to inject this behavior with a service seems like overkill.
// BaseEntity.ts
function encodeHash() {
// logic for hashing
}
class BaseEntity {
public getHashedId() {
return encodeHash(this.id).
}
}
1
Dear @JesseCarter , thank you very much for your response. However, how do you handle it, if theencodeHash
function depends on a 3rd-party service? E.g., hashing itself is provided byHashIds
package, and theHashIds
package is configured via config-params from aConfigService
..
– zorn
Nov 26 '18 at 16:29
add a comment |
Dependency Injection can be a very useful technique especially when it comes to testing but for something simple like hasing an Id you could easily just export a function from somewhere and then simply import it in your BaseEntity. If it's only ever used by your BaseEntity you could even just have it in the same file. Trying to inject this behavior with a service seems like overkill.
// BaseEntity.ts
function encodeHash() {
// logic for hashing
}
class BaseEntity {
public getHashedId() {
return encodeHash(this.id).
}
}
1
Dear @JesseCarter , thank you very much for your response. However, how do you handle it, if theencodeHash
function depends on a 3rd-party service? E.g., hashing itself is provided byHashIds
package, and theHashIds
package is configured via config-params from aConfigService
..
– zorn
Nov 26 '18 at 16:29
add a comment |
Dependency Injection can be a very useful technique especially when it comes to testing but for something simple like hasing an Id you could easily just export a function from somewhere and then simply import it in your BaseEntity. If it's only ever used by your BaseEntity you could even just have it in the same file. Trying to inject this behavior with a service seems like overkill.
// BaseEntity.ts
function encodeHash() {
// logic for hashing
}
class BaseEntity {
public getHashedId() {
return encodeHash(this.id).
}
}
Dependency Injection can be a very useful technique especially when it comes to testing but for something simple like hasing an Id you could easily just export a function from somewhere and then simply import it in your BaseEntity. If it's only ever used by your BaseEntity you could even just have it in the same file. Trying to inject this behavior with a service seems like overkill.
// BaseEntity.ts
function encodeHash() {
// logic for hashing
}
class BaseEntity {
public getHashedId() {
return encodeHash(this.id).
}
}
answered Nov 25 '18 at 14:43


Jesse CarterJesse Carter
9,25133560
9,25133560
1
Dear @JesseCarter , thank you very much for your response. However, how do you handle it, if theencodeHash
function depends on a 3rd-party service? E.g., hashing itself is provided byHashIds
package, and theHashIds
package is configured via config-params from aConfigService
..
– zorn
Nov 26 '18 at 16:29
add a comment |
1
Dear @JesseCarter , thank you very much for your response. However, how do you handle it, if theencodeHash
function depends on a 3rd-party service? E.g., hashing itself is provided byHashIds
package, and theHashIds
package is configured via config-params from aConfigService
..
– zorn
Nov 26 '18 at 16:29
1
1
Dear @JesseCarter , thank you very much for your response. However, how do you handle it, if the
encodeHash
function depends on a 3rd-party service? E.g., hashing itself is provided by HashIds
package, and the HashIds
package is configured via config-params from a ConfigService
..– zorn
Nov 26 '18 at 16:29
Dear @JesseCarter , thank you very much for your response. However, how do you handle it, if the
encodeHash
function depends on a 3rd-party service? E.g., hashing itself is provided by HashIds
package, and the HashIds
package is configured via config-params from a ConfigService
..– zorn
Nov 26 '18 at 16:29
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53443592%2fnestjs-inject-service-into-models-entities%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oIUii,nZvSQsQP WxUfGP
Why is it necessary that this particular service gets injected as opposed to just referencing a utility function?
– Jesse Carter
Nov 23 '18 at 14:25
@JesseCarter Maybe it needs something like access to DB which is otherwise injected?
– Lazar Ljubenović
Nov 24 '18 at 17:02
@JesseCarter I just updated my initial question. I would like to inject the
HashIdService
so my entites are able to hash their own ID.. How would you solve this issue? What do you mean with a "utility function"?– zorn
Nov 25 '18 at 11:07