Copying files using fgets instead of fgetc?
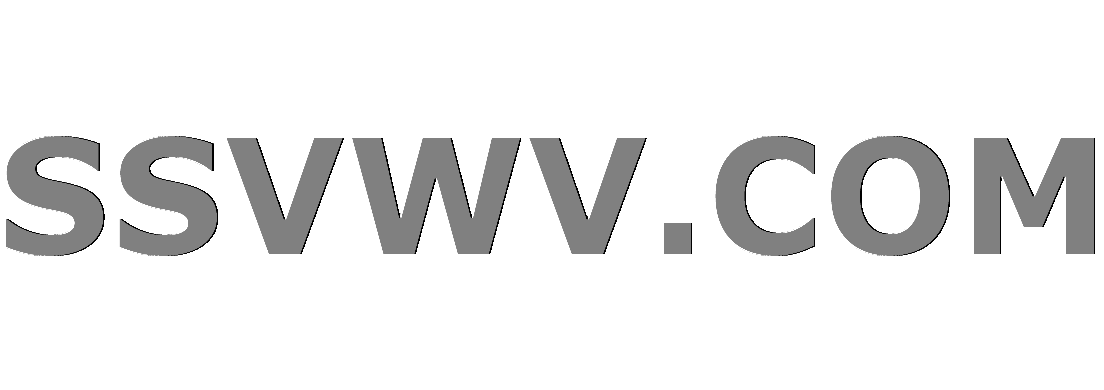
Multi tool use
I made a code:
#include <stdio.h>
#include <stdlib.h>
int main (int argc, char * argv) // taking files
{
FILE * fp;
FILE * fp2;
int c;
.
. // checking possible problems
.
while ( (c = fgetc(fp)) != EOF){ // copying one file to another one
fputc (c, stdout);
fputc (c, fp2);
}
fclose(fp);
fclose(fp2);
fp = NULL;
fp2 = NULL;
return EXIT_SUCCESS;
}
I would ask you, if is it possible to make the same while statement with use of fgets
function instead of fgetc
- like:
fgets(fp, sizeof(fp), stdin));
c file fgets fgetc
add a comment |
I made a code:
#include <stdio.h>
#include <stdlib.h>
int main (int argc, char * argv) // taking files
{
FILE * fp;
FILE * fp2;
int c;
.
. // checking possible problems
.
while ( (c = fgetc(fp)) != EOF){ // copying one file to another one
fputc (c, stdout);
fputc (c, fp2);
}
fclose(fp);
fclose(fp2);
fp = NULL;
fp2 = NULL;
return EXIT_SUCCESS;
}
I would ask you, if is it possible to make the same while statement with use of fgets
function instead of fgetc
- like:
fgets(fp, sizeof(fp), stdin));
c file fgets fgetc
Note that since you haveFILE *fp;
, your examplefgets(fp, sizeof(fp), stdin)
would be wholly inappropriate, even though it would compile — C is not a type-safe language at times. You wantchar buffer[4096];
andfgets(buffer, sizeof(buffer), stdin)
(or usefp
instead ofstdin
if you're reading from a file instead).
– Jonathan Leffler
Nov 20 at 22:41
Welcome to Stack Overflow. Please note that the preferred way of saying 'thanks' around here is by up-voting good questions and helpful answers (once you have enough reputation to do so), and by accepting the most helpful answer to any question you ask (which also gives you a small boost to your reputation). Please see the About page and also How do I ask questions here? and What do I do when someone answers my question?
– Jonathan Leffler
Nov 21 at 0:11
add a comment |
I made a code:
#include <stdio.h>
#include <stdlib.h>
int main (int argc, char * argv) // taking files
{
FILE * fp;
FILE * fp2;
int c;
.
. // checking possible problems
.
while ( (c = fgetc(fp)) != EOF){ // copying one file to another one
fputc (c, stdout);
fputc (c, fp2);
}
fclose(fp);
fclose(fp2);
fp = NULL;
fp2 = NULL;
return EXIT_SUCCESS;
}
I would ask you, if is it possible to make the same while statement with use of fgets
function instead of fgetc
- like:
fgets(fp, sizeof(fp), stdin));
c file fgets fgetc
I made a code:
#include <stdio.h>
#include <stdlib.h>
int main (int argc, char * argv) // taking files
{
FILE * fp;
FILE * fp2;
int c;
.
. // checking possible problems
.
while ( (c = fgetc(fp)) != EOF){ // copying one file to another one
fputc (c, stdout);
fputc (c, fp2);
}
fclose(fp);
fclose(fp2);
fp = NULL;
fp2 = NULL;
return EXIT_SUCCESS;
}
I would ask you, if is it possible to make the same while statement with use of fgets
function instead of fgetc
- like:
fgets(fp, sizeof(fp), stdin));
c file fgets fgetc
c file fgets fgetc
edited Nov 20 at 22:44


Jonathan Leffler
559k896651016
559k896651016
asked Nov 20 at 22:19


K. Jarek
32
32
Note that since you haveFILE *fp;
, your examplefgets(fp, sizeof(fp), stdin)
would be wholly inappropriate, even though it would compile — C is not a type-safe language at times. You wantchar buffer[4096];
andfgets(buffer, sizeof(buffer), stdin)
(or usefp
instead ofstdin
if you're reading from a file instead).
– Jonathan Leffler
Nov 20 at 22:41
Welcome to Stack Overflow. Please note that the preferred way of saying 'thanks' around here is by up-voting good questions and helpful answers (once you have enough reputation to do so), and by accepting the most helpful answer to any question you ask (which also gives you a small boost to your reputation). Please see the About page and also How do I ask questions here? and What do I do when someone answers my question?
– Jonathan Leffler
Nov 21 at 0:11
add a comment |
Note that since you haveFILE *fp;
, your examplefgets(fp, sizeof(fp), stdin)
would be wholly inappropriate, even though it would compile — C is not a type-safe language at times. You wantchar buffer[4096];
andfgets(buffer, sizeof(buffer), stdin)
(or usefp
instead ofstdin
if you're reading from a file instead).
– Jonathan Leffler
Nov 20 at 22:41
Welcome to Stack Overflow. Please note that the preferred way of saying 'thanks' around here is by up-voting good questions and helpful answers (once you have enough reputation to do so), and by accepting the most helpful answer to any question you ask (which also gives you a small boost to your reputation). Please see the About page and also How do I ask questions here? and What do I do when someone answers my question?
– Jonathan Leffler
Nov 21 at 0:11
Note that since you have
FILE *fp;
, your example fgets(fp, sizeof(fp), stdin)
would be wholly inappropriate, even though it would compile — C is not a type-safe language at times. You want char buffer[4096];
and fgets(buffer, sizeof(buffer), stdin)
(or use fp
instead of stdin
if you're reading from a file instead).– Jonathan Leffler
Nov 20 at 22:41
Note that since you have
FILE *fp;
, your example fgets(fp, sizeof(fp), stdin)
would be wholly inappropriate, even though it would compile — C is not a type-safe language at times. You want char buffer[4096];
and fgets(buffer, sizeof(buffer), stdin)
(or use fp
instead of stdin
if you're reading from a file instead).– Jonathan Leffler
Nov 20 at 22:41
Welcome to Stack Overflow. Please note that the preferred way of saying 'thanks' around here is by up-voting good questions and helpful answers (once you have enough reputation to do so), and by accepting the most helpful answer to any question you ask (which also gives you a small boost to your reputation). Please see the About page and also How do I ask questions here? and What do I do when someone answers my question?
– Jonathan Leffler
Nov 21 at 0:11
Welcome to Stack Overflow. Please note that the preferred way of saying 'thanks' around here is by up-voting good questions and helpful answers (once you have enough reputation to do so), and by accepting the most helpful answer to any question you ask (which also gives you a small boost to your reputation). Please see the About page and also How do I ask questions here? and What do I do when someone answers my question?
– Jonathan Leffler
Nov 21 at 0:11
add a comment |
1 Answer
1
active
oldest
votes
Yes, it's possible to use fgets()
and fputs()
.
If you're using POSIX systems, you might find getline()
better than fgets()
, but you'd still use fputs()
with it. Or you might find fread()
and fwrite()
appropriate instead. All of those work on arrays — you get to choose the size, you'd likely be using 4096 bytes as a buffer size.
For example:
char buffer[4096];
while (fgets(buffer, sizeof(buffer), fp) != NULL)
{
fputs(buffer, stdout);
fputs(buffer, fp2);
}
Or:
char buffer[4096];
size_t nbytes;
while ((nbytes = fread(buffer, sizeof(char), sizeof(buffer), fp)) > 0)
{
fwrite(buffer, sizeof(char), nbytes, stdout);
fwrite(buffer, sizeof(char), nbytes, fp2);
}
Warning: uncompiled code.
Note that in theory you should test that the fwrite()
operations worked OK (no short writes). Production quality code would do that. You should also check that the fputs()
operations succeed too.
Note that as hinted at by user2357112, the original code will work with arbitrary binary files (containing null bytes ''
) quite happily (though stdout
may be less happy with it). Using fread()
and fwrite()
will work perfectly with binary files, and the only issue with text files is that they'd ignore newlines and write blocks of text as they're read (which is not usually an actual problem).
Isn't thefgets
version also going to have issues with null bytes in the input?
– user2357112
Nov 20 at 22:46
@user2357112: yes — it is. If you copy binary data with text functions, you get what you get. If the copying is for arbitrary files, thenfread()
andfwrite()
are appropriate because they handle nulls fine. One problem withfgets()
is that it does not tell you how many bytes it read, so if you read a line and the newline is missing, it could be because there was a null byte in the input. But there's no way to know. Thegetline()
function reports how many bytes were read; you could usefwrite()
to ensure that those bytes are all written. There are issues withgetline()
, too.
– Jonathan Leffler
Nov 20 at 22:54
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402455%2fcopying-files-using-fgets-instead-of-fgetc%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Yes, it's possible to use fgets()
and fputs()
.
If you're using POSIX systems, you might find getline()
better than fgets()
, but you'd still use fputs()
with it. Or you might find fread()
and fwrite()
appropriate instead. All of those work on arrays — you get to choose the size, you'd likely be using 4096 bytes as a buffer size.
For example:
char buffer[4096];
while (fgets(buffer, sizeof(buffer), fp) != NULL)
{
fputs(buffer, stdout);
fputs(buffer, fp2);
}
Or:
char buffer[4096];
size_t nbytes;
while ((nbytes = fread(buffer, sizeof(char), sizeof(buffer), fp)) > 0)
{
fwrite(buffer, sizeof(char), nbytes, stdout);
fwrite(buffer, sizeof(char), nbytes, fp2);
}
Warning: uncompiled code.
Note that in theory you should test that the fwrite()
operations worked OK (no short writes). Production quality code would do that. You should also check that the fputs()
operations succeed too.
Note that as hinted at by user2357112, the original code will work with arbitrary binary files (containing null bytes ''
) quite happily (though stdout
may be less happy with it). Using fread()
and fwrite()
will work perfectly with binary files, and the only issue with text files is that they'd ignore newlines and write blocks of text as they're read (which is not usually an actual problem).
Isn't thefgets
version also going to have issues with null bytes in the input?
– user2357112
Nov 20 at 22:46
@user2357112: yes — it is. If you copy binary data with text functions, you get what you get. If the copying is for arbitrary files, thenfread()
andfwrite()
are appropriate because they handle nulls fine. One problem withfgets()
is that it does not tell you how many bytes it read, so if you read a line and the newline is missing, it could be because there was a null byte in the input. But there's no way to know. Thegetline()
function reports how many bytes were read; you could usefwrite()
to ensure that those bytes are all written. There are issues withgetline()
, too.
– Jonathan Leffler
Nov 20 at 22:54
add a comment |
Yes, it's possible to use fgets()
and fputs()
.
If you're using POSIX systems, you might find getline()
better than fgets()
, but you'd still use fputs()
with it. Or you might find fread()
and fwrite()
appropriate instead. All of those work on arrays — you get to choose the size, you'd likely be using 4096 bytes as a buffer size.
For example:
char buffer[4096];
while (fgets(buffer, sizeof(buffer), fp) != NULL)
{
fputs(buffer, stdout);
fputs(buffer, fp2);
}
Or:
char buffer[4096];
size_t nbytes;
while ((nbytes = fread(buffer, sizeof(char), sizeof(buffer), fp)) > 0)
{
fwrite(buffer, sizeof(char), nbytes, stdout);
fwrite(buffer, sizeof(char), nbytes, fp2);
}
Warning: uncompiled code.
Note that in theory you should test that the fwrite()
operations worked OK (no short writes). Production quality code would do that. You should also check that the fputs()
operations succeed too.
Note that as hinted at by user2357112, the original code will work with arbitrary binary files (containing null bytes ''
) quite happily (though stdout
may be less happy with it). Using fread()
and fwrite()
will work perfectly with binary files, and the only issue with text files is that they'd ignore newlines and write blocks of text as they're read (which is not usually an actual problem).
Isn't thefgets
version also going to have issues with null bytes in the input?
– user2357112
Nov 20 at 22:46
@user2357112: yes — it is. If you copy binary data with text functions, you get what you get. If the copying is for arbitrary files, thenfread()
andfwrite()
are appropriate because they handle nulls fine. One problem withfgets()
is that it does not tell you how many bytes it read, so if you read a line and the newline is missing, it could be because there was a null byte in the input. But there's no way to know. Thegetline()
function reports how many bytes were read; you could usefwrite()
to ensure that those bytes are all written. There are issues withgetline()
, too.
– Jonathan Leffler
Nov 20 at 22:54
add a comment |
Yes, it's possible to use fgets()
and fputs()
.
If you're using POSIX systems, you might find getline()
better than fgets()
, but you'd still use fputs()
with it. Or you might find fread()
and fwrite()
appropriate instead. All of those work on arrays — you get to choose the size, you'd likely be using 4096 bytes as a buffer size.
For example:
char buffer[4096];
while (fgets(buffer, sizeof(buffer), fp) != NULL)
{
fputs(buffer, stdout);
fputs(buffer, fp2);
}
Or:
char buffer[4096];
size_t nbytes;
while ((nbytes = fread(buffer, sizeof(char), sizeof(buffer), fp)) > 0)
{
fwrite(buffer, sizeof(char), nbytes, stdout);
fwrite(buffer, sizeof(char), nbytes, fp2);
}
Warning: uncompiled code.
Note that in theory you should test that the fwrite()
operations worked OK (no short writes). Production quality code would do that. You should also check that the fputs()
operations succeed too.
Note that as hinted at by user2357112, the original code will work with arbitrary binary files (containing null bytes ''
) quite happily (though stdout
may be less happy with it). Using fread()
and fwrite()
will work perfectly with binary files, and the only issue with text files is that they'd ignore newlines and write blocks of text as they're read (which is not usually an actual problem).
Yes, it's possible to use fgets()
and fputs()
.
If you're using POSIX systems, you might find getline()
better than fgets()
, but you'd still use fputs()
with it. Or you might find fread()
and fwrite()
appropriate instead. All of those work on arrays — you get to choose the size, you'd likely be using 4096 bytes as a buffer size.
For example:
char buffer[4096];
while (fgets(buffer, sizeof(buffer), fp) != NULL)
{
fputs(buffer, stdout);
fputs(buffer, fp2);
}
Or:
char buffer[4096];
size_t nbytes;
while ((nbytes = fread(buffer, sizeof(char), sizeof(buffer), fp)) > 0)
{
fwrite(buffer, sizeof(char), nbytes, stdout);
fwrite(buffer, sizeof(char), nbytes, fp2);
}
Warning: uncompiled code.
Note that in theory you should test that the fwrite()
operations worked OK (no short writes). Production quality code would do that. You should also check that the fputs()
operations succeed too.
Note that as hinted at by user2357112, the original code will work with arbitrary binary files (containing null bytes ''
) quite happily (though stdout
may be less happy with it). Using fread()
and fwrite()
will work perfectly with binary files, and the only issue with text files is that they'd ignore newlines and write blocks of text as they're read (which is not usually an actual problem).
edited Nov 21 at 0:11
answered Nov 20 at 22:31


Jonathan Leffler
559k896651016
559k896651016
Isn't thefgets
version also going to have issues with null bytes in the input?
– user2357112
Nov 20 at 22:46
@user2357112: yes — it is. If you copy binary data with text functions, you get what you get. If the copying is for arbitrary files, thenfread()
andfwrite()
are appropriate because they handle nulls fine. One problem withfgets()
is that it does not tell you how many bytes it read, so if you read a line and the newline is missing, it could be because there was a null byte in the input. But there's no way to know. Thegetline()
function reports how many bytes were read; you could usefwrite()
to ensure that those bytes are all written. There are issues withgetline()
, too.
– Jonathan Leffler
Nov 20 at 22:54
add a comment |
Isn't thefgets
version also going to have issues with null bytes in the input?
– user2357112
Nov 20 at 22:46
@user2357112: yes — it is. If you copy binary data with text functions, you get what you get. If the copying is for arbitrary files, thenfread()
andfwrite()
are appropriate because they handle nulls fine. One problem withfgets()
is that it does not tell you how many bytes it read, so if you read a line and the newline is missing, it could be because there was a null byte in the input. But there's no way to know. Thegetline()
function reports how many bytes were read; you could usefwrite()
to ensure that those bytes are all written. There are issues withgetline()
, too.
– Jonathan Leffler
Nov 20 at 22:54
Isn't the
fgets
version also going to have issues with null bytes in the input?– user2357112
Nov 20 at 22:46
Isn't the
fgets
version also going to have issues with null bytes in the input?– user2357112
Nov 20 at 22:46
@user2357112: yes — it is. If you copy binary data with text functions, you get what you get. If the copying is for arbitrary files, then
fread()
and fwrite()
are appropriate because they handle nulls fine. One problem with fgets()
is that it does not tell you how many bytes it read, so if you read a line and the newline is missing, it could be because there was a null byte in the input. But there's no way to know. The getline()
function reports how many bytes were read; you could use fwrite()
to ensure that those bytes are all written. There are issues with getline()
, too.– Jonathan Leffler
Nov 20 at 22:54
@user2357112: yes — it is. If you copy binary data with text functions, you get what you get. If the copying is for arbitrary files, then
fread()
and fwrite()
are appropriate because they handle nulls fine. One problem with fgets()
is that it does not tell you how many bytes it read, so if you read a line and the newline is missing, it could be because there was a null byte in the input. But there's no way to know. The getline()
function reports how many bytes were read; you could use fwrite()
to ensure that those bytes are all written. There are issues with getline()
, too.– Jonathan Leffler
Nov 20 at 22:54
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402455%2fcopying-files-using-fgets-instead-of-fgetc%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
PNuEt 5NgWwdAqO4Br,dPSLj ViLwh9WJF5y Omx
Note that since you have
FILE *fp;
, your examplefgets(fp, sizeof(fp), stdin)
would be wholly inappropriate, even though it would compile — C is not a type-safe language at times. You wantchar buffer[4096];
andfgets(buffer, sizeof(buffer), stdin)
(or usefp
instead ofstdin
if you're reading from a file instead).– Jonathan Leffler
Nov 20 at 22:41
Welcome to Stack Overflow. Please note that the preferred way of saying 'thanks' around here is by up-voting good questions and helpful answers (once you have enough reputation to do so), and by accepting the most helpful answer to any question you ask (which also gives you a small boost to your reputation). Please see the About page and also How do I ask questions here? and What do I do when someone answers my question?
– Jonathan Leffler
Nov 21 at 0:11