assembler 8086 need help on clearing out a problem
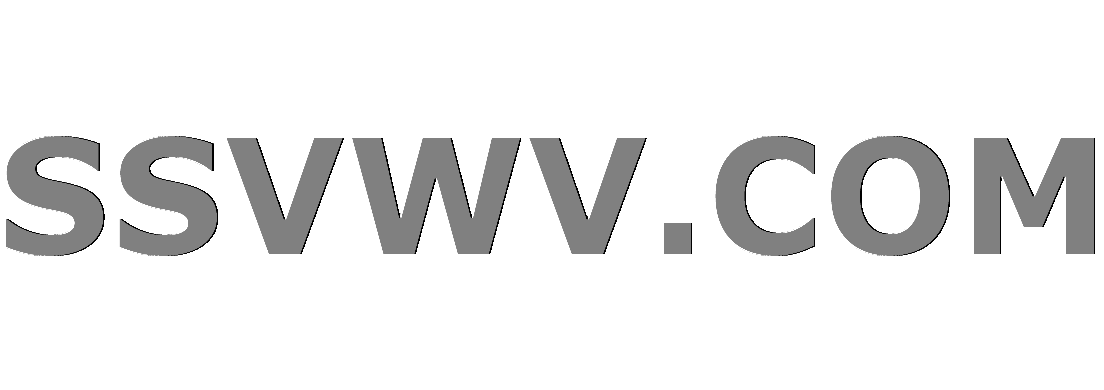
Multi tool use
I could use your help on a program that I'm writing as my school project. It has to open a file that contains about 3000 lines which contains of five fields that are separated by ";". First field is with letters (and it can be max 10 chars), other fields are numbers in range from -100 to 100. It should look something like that:
DSGHAA;12;24;55;-84
The assignment is that I have to open file that contains those lines, and check if line meets some requirements and if it meets them, write that line to another file, that will be created.
Requirements that are needed to be met:
1. It has to have two "A" letters in first field (not more, not less)(max 10 characters);
2. Second and third fields has positive numbers;
3. Fourth and fifth fields has to divide from 11;
Here's a part of my code:
-----------------Reading characters--------------------
.beginning
inc di
mov bx, [bp]
mov cx, 1
mov dx, character
mov ah, 0x3F
int 0x21
mov cl, [character]
jnc .tikrinimas
macPutString 'Error reading file', crlf, '$'
---------Checking if it isn't end of reading file------
.startChecking
cmp ax, 0
jne .ifLineEnd
jmp .closing
---------Checking if it is the end of line-------------
.ifLineEnds
cmp cl, 0x0A
je .splitLine
mov [line+di], cl
jmp .beginning
---------Startting to split my line into "fields"------
.splitLine
mov [line+di], cl
mov si, 0
mov di, 0
.firstField
mov al, byte [line+di]
mov byte [field1+si], al
cmp byte [line+di], ';'
je .splitLine2
inc di
inc si
loop .firstField
.splitLine2
mov si, 0
inc di
.secondField
mov al, byte [line+di]
mov byte [field2+si], al
cmp byte [line+di], ';'
je .splitLine3
inc di
inc si
loop .secondField
.splitLine3
mov si, 0
inc di
.thirdField
mov al, byte [line+di]
mov byte [field3+si], al
cmp byte [line+di], ';'
je .splitLine4
inc di
inc si
loop .thirdField
.splitLine4
mov si, 0
inc di
.fourthField
mov al, byte [line+di]
mov byte [field4+si], al
cmp byte [line+di], ';'
je .splitLine5
inc di
inc si
loop .fourthField
.splitLine5
mov si, 0
inc di
.fifthField
mov al, byte [line+di]
mov byte [field5+si], al
cmp byte [line+di], ';'
je .howManyA <----This is where I have a problem..
inc di
inc si
loop .fifthField
-------counting how many "A" are in first field-------
.howManyA
mov si, 0
mov ch, 0
.check
cmp byte [field1+si], ';'
je .numberA
cmp byte [field1+si], 'A'
jne .next
inc ch
inc si
jmp .check
.next
inc si
jmp .check
.numberA
cmp ch, 2
je .isFirstPositive
jmp .toBeginning
--------checking if first number is positive----------
.isFirstPositive
mov ch, byte [field2]
cmp ch, 0x2D
jne .isSecondPositive
jmp .toBeginning
--------checking if second number is positive---------
.isSecondPositive
mov ch, byte [field3]
cmp ch, 0xD2
jmp .toBeginning
--------checking if third number divides from 11------
.firstDivide
mov ah, byte [field4]
cmp ax, 0
je .toBeginning
cmp ah, 0xD2
je .numbers
.numbers
mov ah, byte [laukas4+01]
mov al, byte [laukas4+02]
cmp ah, al
je .secondDivide
jmp .toBeginning
------checking if fourth number divides from 11------
.secondBeggining
mov ah, [field5+00]
cmp ax, 0
je .toBeginning
cmp ah, 0xD2
je .numbers2
.numbers2
mov ah, byte [field5+01]
mov al, byte [field5+02]
cmp ah, al
je .writeToFile
jmp .toBeginning
-----------jumping to beginning to read another line---------
.toBeginning
mov di, -1
jmp .beginning
---------------data----------------------------------
section .data
character: times 1 db 00
line: times 32 db 00
field1: times 11 db 00
field2: times 5 db 00
field3: times 5 db 00
field4: times 5 db 00
field5: times 5 db 00
I've omitted code parts where is opening, creating, writing and closing files. That works.
I've showed on my code where is a problem and I can't determine why..
I have a test file
input.txt that contains this line "AA;12;15;55;-77
Now, when I debug my program, everything works perfectly until it reaches first "7". Program compares "7" (ASCII 37) with ";" (ASCII 3B) and jumps to ".howManyA". I can't figure out why it does that and what to look for..
Any help would be appreciated.
assembly x86 x86-16
add a comment |
I could use your help on a program that I'm writing as my school project. It has to open a file that contains about 3000 lines which contains of five fields that are separated by ";". First field is with letters (and it can be max 10 chars), other fields are numbers in range from -100 to 100. It should look something like that:
DSGHAA;12;24;55;-84
The assignment is that I have to open file that contains those lines, and check if line meets some requirements and if it meets them, write that line to another file, that will be created.
Requirements that are needed to be met:
1. It has to have two "A" letters in first field (not more, not less)(max 10 characters);
2. Second and third fields has positive numbers;
3. Fourth and fifth fields has to divide from 11;
Here's a part of my code:
-----------------Reading characters--------------------
.beginning
inc di
mov bx, [bp]
mov cx, 1
mov dx, character
mov ah, 0x3F
int 0x21
mov cl, [character]
jnc .tikrinimas
macPutString 'Error reading file', crlf, '$'
---------Checking if it isn't end of reading file------
.startChecking
cmp ax, 0
jne .ifLineEnd
jmp .closing
---------Checking if it is the end of line-------------
.ifLineEnds
cmp cl, 0x0A
je .splitLine
mov [line+di], cl
jmp .beginning
---------Startting to split my line into "fields"------
.splitLine
mov [line+di], cl
mov si, 0
mov di, 0
.firstField
mov al, byte [line+di]
mov byte [field1+si], al
cmp byte [line+di], ';'
je .splitLine2
inc di
inc si
loop .firstField
.splitLine2
mov si, 0
inc di
.secondField
mov al, byte [line+di]
mov byte [field2+si], al
cmp byte [line+di], ';'
je .splitLine3
inc di
inc si
loop .secondField
.splitLine3
mov si, 0
inc di
.thirdField
mov al, byte [line+di]
mov byte [field3+si], al
cmp byte [line+di], ';'
je .splitLine4
inc di
inc si
loop .thirdField
.splitLine4
mov si, 0
inc di
.fourthField
mov al, byte [line+di]
mov byte [field4+si], al
cmp byte [line+di], ';'
je .splitLine5
inc di
inc si
loop .fourthField
.splitLine5
mov si, 0
inc di
.fifthField
mov al, byte [line+di]
mov byte [field5+si], al
cmp byte [line+di], ';'
je .howManyA <----This is where I have a problem..
inc di
inc si
loop .fifthField
-------counting how many "A" are in first field-------
.howManyA
mov si, 0
mov ch, 0
.check
cmp byte [field1+si], ';'
je .numberA
cmp byte [field1+si], 'A'
jne .next
inc ch
inc si
jmp .check
.next
inc si
jmp .check
.numberA
cmp ch, 2
je .isFirstPositive
jmp .toBeginning
--------checking if first number is positive----------
.isFirstPositive
mov ch, byte [field2]
cmp ch, 0x2D
jne .isSecondPositive
jmp .toBeginning
--------checking if second number is positive---------
.isSecondPositive
mov ch, byte [field3]
cmp ch, 0xD2
jmp .toBeginning
--------checking if third number divides from 11------
.firstDivide
mov ah, byte [field4]
cmp ax, 0
je .toBeginning
cmp ah, 0xD2
je .numbers
.numbers
mov ah, byte [laukas4+01]
mov al, byte [laukas4+02]
cmp ah, al
je .secondDivide
jmp .toBeginning
------checking if fourth number divides from 11------
.secondBeggining
mov ah, [field5+00]
cmp ax, 0
je .toBeginning
cmp ah, 0xD2
je .numbers2
.numbers2
mov ah, byte [field5+01]
mov al, byte [field5+02]
cmp ah, al
je .writeToFile
jmp .toBeginning
-----------jumping to beginning to read another line---------
.toBeginning
mov di, -1
jmp .beginning
---------------data----------------------------------
section .data
character: times 1 db 00
line: times 32 db 00
field1: times 11 db 00
field2: times 5 db 00
field3: times 5 db 00
field4: times 5 db 00
field5: times 5 db 00
I've omitted code parts where is opening, creating, writing and closing files. That works.
I've showed on my code where is a problem and I can't determine why..
I have a test file
input.txt that contains this line "AA;12;15;55;-77
Now, when I debug my program, everything works perfectly until it reaches first "7". Program compares "7" (ASCII 37) with ";" (ASCII 3B) and jumps to ".howManyA". I can't figure out why it does that and what to look for..
Any help would be appreciated.
assembly x86 x86-16
1
That should not happen. Verify you are using your debugger correctly and indeedal
equals'7'
at that point. I find it more likely that you don't understand howloop
works because you don't setcx
to a sensible counter value and it's actually that's falling through intohowManyA
not theje
. I guess you just wantjmp
instead of all yourloop
instructions.
– Jester
Nov 25 '18 at 13:20
I tested it and I can confirm the assumption of @Jester. It is theloop
that doesn't jump becauseCX
reaches 0.
– rkhb
Nov 25 '18 at 15:47
Jester, thank you a lot, you're right, problem was in loop, i've debugged my code and problem was in loop and in CX register. changed all loops in jmp and work like a charm. Thank you again
– Pooshkis
Nov 25 '18 at 16:40
add a comment |
I could use your help on a program that I'm writing as my school project. It has to open a file that contains about 3000 lines which contains of five fields that are separated by ";". First field is with letters (and it can be max 10 chars), other fields are numbers in range from -100 to 100. It should look something like that:
DSGHAA;12;24;55;-84
The assignment is that I have to open file that contains those lines, and check if line meets some requirements and if it meets them, write that line to another file, that will be created.
Requirements that are needed to be met:
1. It has to have two "A" letters in first field (not more, not less)(max 10 characters);
2. Second and third fields has positive numbers;
3. Fourth and fifth fields has to divide from 11;
Here's a part of my code:
-----------------Reading characters--------------------
.beginning
inc di
mov bx, [bp]
mov cx, 1
mov dx, character
mov ah, 0x3F
int 0x21
mov cl, [character]
jnc .tikrinimas
macPutString 'Error reading file', crlf, '$'
---------Checking if it isn't end of reading file------
.startChecking
cmp ax, 0
jne .ifLineEnd
jmp .closing
---------Checking if it is the end of line-------------
.ifLineEnds
cmp cl, 0x0A
je .splitLine
mov [line+di], cl
jmp .beginning
---------Startting to split my line into "fields"------
.splitLine
mov [line+di], cl
mov si, 0
mov di, 0
.firstField
mov al, byte [line+di]
mov byte [field1+si], al
cmp byte [line+di], ';'
je .splitLine2
inc di
inc si
loop .firstField
.splitLine2
mov si, 0
inc di
.secondField
mov al, byte [line+di]
mov byte [field2+si], al
cmp byte [line+di], ';'
je .splitLine3
inc di
inc si
loop .secondField
.splitLine3
mov si, 0
inc di
.thirdField
mov al, byte [line+di]
mov byte [field3+si], al
cmp byte [line+di], ';'
je .splitLine4
inc di
inc si
loop .thirdField
.splitLine4
mov si, 0
inc di
.fourthField
mov al, byte [line+di]
mov byte [field4+si], al
cmp byte [line+di], ';'
je .splitLine5
inc di
inc si
loop .fourthField
.splitLine5
mov si, 0
inc di
.fifthField
mov al, byte [line+di]
mov byte [field5+si], al
cmp byte [line+di], ';'
je .howManyA <----This is where I have a problem..
inc di
inc si
loop .fifthField
-------counting how many "A" are in first field-------
.howManyA
mov si, 0
mov ch, 0
.check
cmp byte [field1+si], ';'
je .numberA
cmp byte [field1+si], 'A'
jne .next
inc ch
inc si
jmp .check
.next
inc si
jmp .check
.numberA
cmp ch, 2
je .isFirstPositive
jmp .toBeginning
--------checking if first number is positive----------
.isFirstPositive
mov ch, byte [field2]
cmp ch, 0x2D
jne .isSecondPositive
jmp .toBeginning
--------checking if second number is positive---------
.isSecondPositive
mov ch, byte [field3]
cmp ch, 0xD2
jmp .toBeginning
--------checking if third number divides from 11------
.firstDivide
mov ah, byte [field4]
cmp ax, 0
je .toBeginning
cmp ah, 0xD2
je .numbers
.numbers
mov ah, byte [laukas4+01]
mov al, byte [laukas4+02]
cmp ah, al
je .secondDivide
jmp .toBeginning
------checking if fourth number divides from 11------
.secondBeggining
mov ah, [field5+00]
cmp ax, 0
je .toBeginning
cmp ah, 0xD2
je .numbers2
.numbers2
mov ah, byte [field5+01]
mov al, byte [field5+02]
cmp ah, al
je .writeToFile
jmp .toBeginning
-----------jumping to beginning to read another line---------
.toBeginning
mov di, -1
jmp .beginning
---------------data----------------------------------
section .data
character: times 1 db 00
line: times 32 db 00
field1: times 11 db 00
field2: times 5 db 00
field3: times 5 db 00
field4: times 5 db 00
field5: times 5 db 00
I've omitted code parts where is opening, creating, writing and closing files. That works.
I've showed on my code where is a problem and I can't determine why..
I have a test file
input.txt that contains this line "AA;12;15;55;-77
Now, when I debug my program, everything works perfectly until it reaches first "7". Program compares "7" (ASCII 37) with ";" (ASCII 3B) and jumps to ".howManyA". I can't figure out why it does that and what to look for..
Any help would be appreciated.
assembly x86 x86-16
I could use your help on a program that I'm writing as my school project. It has to open a file that contains about 3000 lines which contains of five fields that are separated by ";". First field is with letters (and it can be max 10 chars), other fields are numbers in range from -100 to 100. It should look something like that:
DSGHAA;12;24;55;-84
The assignment is that I have to open file that contains those lines, and check if line meets some requirements and if it meets them, write that line to another file, that will be created.
Requirements that are needed to be met:
1. It has to have two "A" letters in first field (not more, not less)(max 10 characters);
2. Second and third fields has positive numbers;
3. Fourth and fifth fields has to divide from 11;
Here's a part of my code:
-----------------Reading characters--------------------
.beginning
inc di
mov bx, [bp]
mov cx, 1
mov dx, character
mov ah, 0x3F
int 0x21
mov cl, [character]
jnc .tikrinimas
macPutString 'Error reading file', crlf, '$'
---------Checking if it isn't end of reading file------
.startChecking
cmp ax, 0
jne .ifLineEnd
jmp .closing
---------Checking if it is the end of line-------------
.ifLineEnds
cmp cl, 0x0A
je .splitLine
mov [line+di], cl
jmp .beginning
---------Startting to split my line into "fields"------
.splitLine
mov [line+di], cl
mov si, 0
mov di, 0
.firstField
mov al, byte [line+di]
mov byte [field1+si], al
cmp byte [line+di], ';'
je .splitLine2
inc di
inc si
loop .firstField
.splitLine2
mov si, 0
inc di
.secondField
mov al, byte [line+di]
mov byte [field2+si], al
cmp byte [line+di], ';'
je .splitLine3
inc di
inc si
loop .secondField
.splitLine3
mov si, 0
inc di
.thirdField
mov al, byte [line+di]
mov byte [field3+si], al
cmp byte [line+di], ';'
je .splitLine4
inc di
inc si
loop .thirdField
.splitLine4
mov si, 0
inc di
.fourthField
mov al, byte [line+di]
mov byte [field4+si], al
cmp byte [line+di], ';'
je .splitLine5
inc di
inc si
loop .fourthField
.splitLine5
mov si, 0
inc di
.fifthField
mov al, byte [line+di]
mov byte [field5+si], al
cmp byte [line+di], ';'
je .howManyA <----This is where I have a problem..
inc di
inc si
loop .fifthField
-------counting how many "A" are in first field-------
.howManyA
mov si, 0
mov ch, 0
.check
cmp byte [field1+si], ';'
je .numberA
cmp byte [field1+si], 'A'
jne .next
inc ch
inc si
jmp .check
.next
inc si
jmp .check
.numberA
cmp ch, 2
je .isFirstPositive
jmp .toBeginning
--------checking if first number is positive----------
.isFirstPositive
mov ch, byte [field2]
cmp ch, 0x2D
jne .isSecondPositive
jmp .toBeginning
--------checking if second number is positive---------
.isSecondPositive
mov ch, byte [field3]
cmp ch, 0xD2
jmp .toBeginning
--------checking if third number divides from 11------
.firstDivide
mov ah, byte [field4]
cmp ax, 0
je .toBeginning
cmp ah, 0xD2
je .numbers
.numbers
mov ah, byte [laukas4+01]
mov al, byte [laukas4+02]
cmp ah, al
je .secondDivide
jmp .toBeginning
------checking if fourth number divides from 11------
.secondBeggining
mov ah, [field5+00]
cmp ax, 0
je .toBeginning
cmp ah, 0xD2
je .numbers2
.numbers2
mov ah, byte [field5+01]
mov al, byte [field5+02]
cmp ah, al
je .writeToFile
jmp .toBeginning
-----------jumping to beginning to read another line---------
.toBeginning
mov di, -1
jmp .beginning
---------------data----------------------------------
section .data
character: times 1 db 00
line: times 32 db 00
field1: times 11 db 00
field2: times 5 db 00
field3: times 5 db 00
field4: times 5 db 00
field5: times 5 db 00
I've omitted code parts where is opening, creating, writing and closing files. That works.
I've showed on my code where is a problem and I can't determine why..
I have a test file
input.txt that contains this line "AA;12;15;55;-77
Now, when I debug my program, everything works perfectly until it reaches first "7". Program compares "7" (ASCII 37) with ";" (ASCII 3B) and jumps to ".howManyA". I can't figure out why it does that and what to look for..
Any help would be appreciated.
assembly x86 x86-16
assembly x86 x86-16
edited Nov 25 '18 at 13:37
Pooshkis
asked Nov 25 '18 at 13:12
PooshkisPooshkis
84
84
1
That should not happen. Verify you are using your debugger correctly and indeedal
equals'7'
at that point. I find it more likely that you don't understand howloop
works because you don't setcx
to a sensible counter value and it's actually that's falling through intohowManyA
not theje
. I guess you just wantjmp
instead of all yourloop
instructions.
– Jester
Nov 25 '18 at 13:20
I tested it and I can confirm the assumption of @Jester. It is theloop
that doesn't jump becauseCX
reaches 0.
– rkhb
Nov 25 '18 at 15:47
Jester, thank you a lot, you're right, problem was in loop, i've debugged my code and problem was in loop and in CX register. changed all loops in jmp and work like a charm. Thank you again
– Pooshkis
Nov 25 '18 at 16:40
add a comment |
1
That should not happen. Verify you are using your debugger correctly and indeedal
equals'7'
at that point. I find it more likely that you don't understand howloop
works because you don't setcx
to a sensible counter value and it's actually that's falling through intohowManyA
not theje
. I guess you just wantjmp
instead of all yourloop
instructions.
– Jester
Nov 25 '18 at 13:20
I tested it and I can confirm the assumption of @Jester. It is theloop
that doesn't jump becauseCX
reaches 0.
– rkhb
Nov 25 '18 at 15:47
Jester, thank you a lot, you're right, problem was in loop, i've debugged my code and problem was in loop and in CX register. changed all loops in jmp and work like a charm. Thank you again
– Pooshkis
Nov 25 '18 at 16:40
1
1
That should not happen. Verify you are using your debugger correctly and indeed
al
equals '7'
at that point. I find it more likely that you don't understand how loop
works because you don't set cx
to a sensible counter value and it's actually that's falling through into howManyA
not the je
. I guess you just want jmp
instead of all your loop
instructions.– Jester
Nov 25 '18 at 13:20
That should not happen. Verify you are using your debugger correctly and indeed
al
equals '7'
at that point. I find it more likely that you don't understand how loop
works because you don't set cx
to a sensible counter value and it's actually that's falling through into howManyA
not the je
. I guess you just want jmp
instead of all your loop
instructions.– Jester
Nov 25 '18 at 13:20
I tested it and I can confirm the assumption of @Jester. It is the
loop
that doesn't jump because CX
reaches 0.– rkhb
Nov 25 '18 at 15:47
I tested it and I can confirm the assumption of @Jester. It is the
loop
that doesn't jump because CX
reaches 0.– rkhb
Nov 25 '18 at 15:47
Jester, thank you a lot, you're right, problem was in loop, i've debugged my code and problem was in loop and in CX register. changed all loops in jmp and work like a charm. Thank you again
– Pooshkis
Nov 25 '18 at 16:40
Jester, thank you a lot, you're right, problem was in loop, i've debugged my code and problem was in loop and in CX register. changed all loops in jmp and work like a charm. Thank you again
– Pooshkis
Nov 25 '18 at 16:40
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53467814%2fassembler-8086-need-help-on-clearing-out-a-problem%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53467814%2fassembler-8086-need-help-on-clearing-out-a-problem%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
y9o15Ih
1
That should not happen. Verify you are using your debugger correctly and indeed
al
equals'7'
at that point. I find it more likely that you don't understand howloop
works because you don't setcx
to a sensible counter value and it's actually that's falling through intohowManyA
not theje
. I guess you just wantjmp
instead of all yourloop
instructions.– Jester
Nov 25 '18 at 13:20
I tested it and I can confirm the assumption of @Jester. It is the
loop
that doesn't jump becauseCX
reaches 0.– rkhb
Nov 25 '18 at 15:47
Jester, thank you a lot, you're right, problem was in loop, i've debugged my code and problem was in loop and in CX register. changed all loops in jmp and work like a charm. Thank you again
– Pooshkis
Nov 25 '18 at 16:40