Visual Studio Code c++11 extension warning
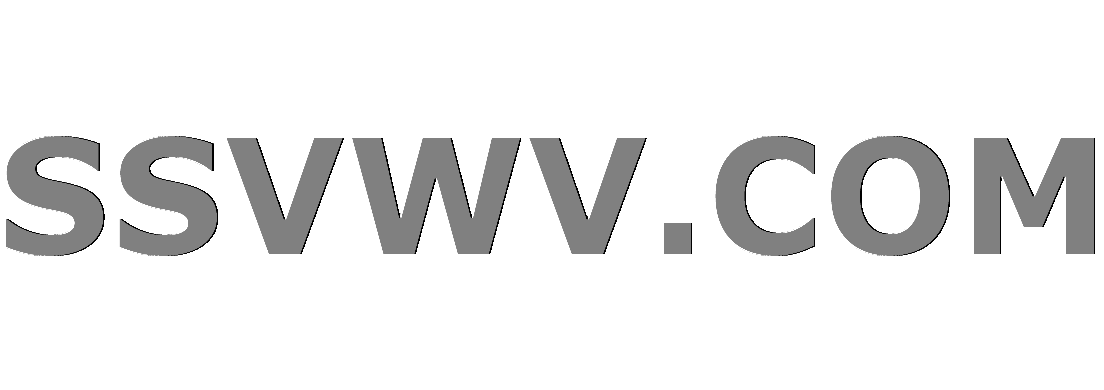
Multi tool use
I am in the process of learning c++ and I'm using visual studio code for Mac. I use Code Runner to run my program. My problem is that when I use something from c++11 like "auto" for variable declaration, visual studio code gives me a warning like this, but if I try running it on Xcode or Eclipse it doesn't:
warning: 'auto' type specifier is a C++11 extension [-Wc++11-extensions]
for(auto y: nstrVec)
This is the program if it's necessary:
#include <iostream>
#include <cstdlib>
#include <string>
#include <vector>
#include <numeric>
#include <sstream>
int main(){
std::vector<std::string> nstrVec(10);
std::string str("I'm a string");
nstrVec[0] = str;
std::cout << str.at(0) << "n";
std::cout << str.front() << " " << str.back() << "n";
std::cout << "Length " << str.length() << "n";
// copies all characters after the fourth
std::string str2(str, 4);
for(auto y: nstrVec)
if(y != "")
std::cout << y << "n";
return 0;
}
And this is the c_cpp_proprerties.json file:
{
"configurations": [
{
"name": "Mac",
"includePath": [
"${workspaceFolder}/**",
"/System/Library/Frameworks/Kernel.framework/Versions/A/Headers"
],
"defines": ,
"macFrameworkPath": [
"/System/Library/Frameworks",
"/Library/Frameworks"
],
"compilerPath": "/usr/bin/clang",
"cStandard": "c11",
"cppStandard": "c++17",
"intelliSenseMode": "clang-x64"
}
],
"version": 4
}
c++ macos visual-studio-code
|
show 10 more comments
I am in the process of learning c++ and I'm using visual studio code for Mac. I use Code Runner to run my program. My problem is that when I use something from c++11 like "auto" for variable declaration, visual studio code gives me a warning like this, but if I try running it on Xcode or Eclipse it doesn't:
warning: 'auto' type specifier is a C++11 extension [-Wc++11-extensions]
for(auto y: nstrVec)
This is the program if it's necessary:
#include <iostream>
#include <cstdlib>
#include <string>
#include <vector>
#include <numeric>
#include <sstream>
int main(){
std::vector<std::string> nstrVec(10);
std::string str("I'm a string");
nstrVec[0] = str;
std::cout << str.at(0) << "n";
std::cout << str.front() << " " << str.back() << "n";
std::cout << "Length " << str.length() << "n";
// copies all characters after the fourth
std::string str2(str, 4);
for(auto y: nstrVec)
if(y != "")
std::cout << y << "n";
return 0;
}
And this is the c_cpp_proprerties.json file:
{
"configurations": [
{
"name": "Mac",
"includePath": [
"${workspaceFolder}/**",
"/System/Library/Frameworks/Kernel.framework/Versions/A/Headers"
],
"defines": ,
"macFrameworkPath": [
"/System/Library/Frameworks",
"/Library/Frameworks"
],
"compilerPath": "/usr/bin/clang",
"cStandard": "c11",
"cppStandard": "c++17",
"intelliSenseMode": "clang-x64"
}
],
"version": 4
}
c++ macos visual-studio-code
Are you compiling for C++11? It doesn't sound like it. Theauto
keyword was introduced in C++11 so before that it was considered a language extension by Visual Studio.
– CoryKramer
Jun 26 '18 at 15:46
@CoryKramer Question is about VS Code, not VS.
– Neil Butterworth
Jun 26 '18 at 15:47
You are telling the compiler to use 2 different standards: you have both "-std=c++17" and "-std=c++11".
– Fabio Turati
Jun 26 '18 at 15:47
2
Can you compile it from the command line? That is, open a shell, go to that directory, and typeg++ -std=c++17 -g helloworld.cpp -o helloworld
: does it work?
– Fabio Turati
Jun 26 '18 at 16:14
2
I'm glad that you could solve your problem, but please do not edit your post to add "SOLVED" in the title, Stack Overflow works differently. The correct way to indicate it is to accept an answer, once there is one. You could ask @Bob__ whether he is interested in posting one; if he isn't, please post it yourself and accept it. Thank you!
– Fabio Turati
Jun 26 '18 at 22:10
|
show 10 more comments
I am in the process of learning c++ and I'm using visual studio code for Mac. I use Code Runner to run my program. My problem is that when I use something from c++11 like "auto" for variable declaration, visual studio code gives me a warning like this, but if I try running it on Xcode or Eclipse it doesn't:
warning: 'auto' type specifier is a C++11 extension [-Wc++11-extensions]
for(auto y: nstrVec)
This is the program if it's necessary:
#include <iostream>
#include <cstdlib>
#include <string>
#include <vector>
#include <numeric>
#include <sstream>
int main(){
std::vector<std::string> nstrVec(10);
std::string str("I'm a string");
nstrVec[0] = str;
std::cout << str.at(0) << "n";
std::cout << str.front() << " " << str.back() << "n";
std::cout << "Length " << str.length() << "n";
// copies all characters after the fourth
std::string str2(str, 4);
for(auto y: nstrVec)
if(y != "")
std::cout << y << "n";
return 0;
}
And this is the c_cpp_proprerties.json file:
{
"configurations": [
{
"name": "Mac",
"includePath": [
"${workspaceFolder}/**",
"/System/Library/Frameworks/Kernel.framework/Versions/A/Headers"
],
"defines": ,
"macFrameworkPath": [
"/System/Library/Frameworks",
"/Library/Frameworks"
],
"compilerPath": "/usr/bin/clang",
"cStandard": "c11",
"cppStandard": "c++17",
"intelliSenseMode": "clang-x64"
}
],
"version": 4
}
c++ macos visual-studio-code
I am in the process of learning c++ and I'm using visual studio code for Mac. I use Code Runner to run my program. My problem is that when I use something from c++11 like "auto" for variable declaration, visual studio code gives me a warning like this, but if I try running it on Xcode or Eclipse it doesn't:
warning: 'auto' type specifier is a C++11 extension [-Wc++11-extensions]
for(auto y: nstrVec)
This is the program if it's necessary:
#include <iostream>
#include <cstdlib>
#include <string>
#include <vector>
#include <numeric>
#include <sstream>
int main(){
std::vector<std::string> nstrVec(10);
std::string str("I'm a string");
nstrVec[0] = str;
std::cout << str.at(0) << "n";
std::cout << str.front() << " " << str.back() << "n";
std::cout << "Length " << str.length() << "n";
// copies all characters after the fourth
std::string str2(str, 4);
for(auto y: nstrVec)
if(y != "")
std::cout << y << "n";
return 0;
}
And this is the c_cpp_proprerties.json file:
{
"configurations": [
{
"name": "Mac",
"includePath": [
"${workspaceFolder}/**",
"/System/Library/Frameworks/Kernel.framework/Versions/A/Headers"
],
"defines": ,
"macFrameworkPath": [
"/System/Library/Frameworks",
"/Library/Frameworks"
],
"compilerPath": "/usr/bin/clang",
"cStandard": "c11",
"cppStandard": "c++17",
"intelliSenseMode": "clang-x64"
}
],
"version": 4
}
c++ macos visual-studio-code
c++ macos visual-studio-code
edited Jul 8 '18 at 3:08
Cœur
17.6k9104145
17.6k9104145
asked Jun 26 '18 at 15:43


BONANDRINI CARLOBONANDRINI CARLO
306
306
Are you compiling for C++11? It doesn't sound like it. Theauto
keyword was introduced in C++11 so before that it was considered a language extension by Visual Studio.
– CoryKramer
Jun 26 '18 at 15:46
@CoryKramer Question is about VS Code, not VS.
– Neil Butterworth
Jun 26 '18 at 15:47
You are telling the compiler to use 2 different standards: you have both "-std=c++17" and "-std=c++11".
– Fabio Turati
Jun 26 '18 at 15:47
2
Can you compile it from the command line? That is, open a shell, go to that directory, and typeg++ -std=c++17 -g helloworld.cpp -o helloworld
: does it work?
– Fabio Turati
Jun 26 '18 at 16:14
2
I'm glad that you could solve your problem, but please do not edit your post to add "SOLVED" in the title, Stack Overflow works differently. The correct way to indicate it is to accept an answer, once there is one. You could ask @Bob__ whether he is interested in posting one; if he isn't, please post it yourself and accept it. Thank you!
– Fabio Turati
Jun 26 '18 at 22:10
|
show 10 more comments
Are you compiling for C++11? It doesn't sound like it. Theauto
keyword was introduced in C++11 so before that it was considered a language extension by Visual Studio.
– CoryKramer
Jun 26 '18 at 15:46
@CoryKramer Question is about VS Code, not VS.
– Neil Butterworth
Jun 26 '18 at 15:47
You are telling the compiler to use 2 different standards: you have both "-std=c++17" and "-std=c++11".
– Fabio Turati
Jun 26 '18 at 15:47
2
Can you compile it from the command line? That is, open a shell, go to that directory, and typeg++ -std=c++17 -g helloworld.cpp -o helloworld
: does it work?
– Fabio Turati
Jun 26 '18 at 16:14
2
I'm glad that you could solve your problem, but please do not edit your post to add "SOLVED" in the title, Stack Overflow works differently. The correct way to indicate it is to accept an answer, once there is one. You could ask @Bob__ whether he is interested in posting one; if he isn't, please post it yourself and accept it. Thank you!
– Fabio Turati
Jun 26 '18 at 22:10
Are you compiling for C++11? It doesn't sound like it. The
auto
keyword was introduced in C++11 so before that it was considered a language extension by Visual Studio.– CoryKramer
Jun 26 '18 at 15:46
Are you compiling for C++11? It doesn't sound like it. The
auto
keyword was introduced in C++11 so before that it was considered a language extension by Visual Studio.– CoryKramer
Jun 26 '18 at 15:46
@CoryKramer Question is about VS Code, not VS.
– Neil Butterworth
Jun 26 '18 at 15:47
@CoryKramer Question is about VS Code, not VS.
– Neil Butterworth
Jun 26 '18 at 15:47
You are telling the compiler to use 2 different standards: you have both "-std=c++17" and "-std=c++11".
– Fabio Turati
Jun 26 '18 at 15:47
You are telling the compiler to use 2 different standards: you have both "-std=c++17" and "-std=c++11".
– Fabio Turati
Jun 26 '18 at 15:47
2
2
Can you compile it from the command line? That is, open a shell, go to that directory, and type
g++ -std=c++17 -g helloworld.cpp -o helloworld
: does it work?– Fabio Turati
Jun 26 '18 at 16:14
Can you compile it from the command line? That is, open a shell, go to that directory, and type
g++ -std=c++17 -g helloworld.cpp -o helloworld
: does it work?– Fabio Turati
Jun 26 '18 at 16:14
2
2
I'm glad that you could solve your problem, but please do not edit your post to add "SOLVED" in the title, Stack Overflow works differently. The correct way to indicate it is to accept an answer, once there is one. You could ask @Bob__ whether he is interested in posting one; if he isn't, please post it yourself and accept it. Thank you!
– Fabio Turati
Jun 26 '18 at 22:10
I'm glad that you could solve your problem, but please do not edit your post to add "SOLVED" in the title, Stack Overflow works differently. The correct way to indicate it is to accept an answer, once there is one. You could ask @Bob__ whether he is interested in posting one; if he isn't, please post it yourself and accept it. Thank you!
– Fabio Turati
Jun 26 '18 at 22:10
|
show 10 more comments
2 Answers
2
active
oldest
votes
I had the same problem, but solved it using set vscode-user-settings <>
"clang.cxxflags": ["-std=c++14"]
add a comment |
For everyone who comes to this question to find a quick answer (like I did):
The following compiler command should compile your program main.cpp
with the latest C++ standard (c++17) and should get rid of warning messages like the one described above:
g++ -std=c++17 -g main.cpp -o main
It is mentioned multiple times in the comments, but I think this question should have a regular answer.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f51046803%2fvisual-studio-code-c11-extension-warning%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I had the same problem, but solved it using set vscode-user-settings <>
"clang.cxxflags": ["-std=c++14"]
add a comment |
I had the same problem, but solved it using set vscode-user-settings <>
"clang.cxxflags": ["-std=c++14"]
add a comment |
I had the same problem, but solved it using set vscode-user-settings <>
"clang.cxxflags": ["-std=c++14"]
I had the same problem, but solved it using set vscode-user-settings <>
"clang.cxxflags": ["-std=c++14"]
edited Nov 22 '18 at 7:20


Filnor
1,15021624
1,15021624
answered Nov 22 '18 at 7:02


vic.zhangvic.zhang
214
214
add a comment |
add a comment |
For everyone who comes to this question to find a quick answer (like I did):
The following compiler command should compile your program main.cpp
with the latest C++ standard (c++17) and should get rid of warning messages like the one described above:
g++ -std=c++17 -g main.cpp -o main
It is mentioned multiple times in the comments, but I think this question should have a regular answer.
add a comment |
For everyone who comes to this question to find a quick answer (like I did):
The following compiler command should compile your program main.cpp
with the latest C++ standard (c++17) and should get rid of warning messages like the one described above:
g++ -std=c++17 -g main.cpp -o main
It is mentioned multiple times in the comments, but I think this question should have a regular answer.
add a comment |
For everyone who comes to this question to find a quick answer (like I did):
The following compiler command should compile your program main.cpp
with the latest C++ standard (c++17) and should get rid of warning messages like the one described above:
g++ -std=c++17 -g main.cpp -o main
It is mentioned multiple times in the comments, but I think this question should have a regular answer.
For everyone who comes to this question to find a quick answer (like I did):
The following compiler command should compile your program main.cpp
with the latest C++ standard (c++17) and should get rid of warning messages like the one described above:
g++ -std=c++17 -g main.cpp -o main
It is mentioned multiple times in the comments, but I think this question should have a regular answer.
answered Jul 15 '18 at 20:16


Daniel SchuetteDaniel Schuette
150112
150112
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f51046803%2fvisual-studio-code-c11-extension-warning%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ohpsM,pDvJR YJ peI70Zqk POwec5TLLZn s,UK7DsDSL0iF ix
Are you compiling for C++11? It doesn't sound like it. The
auto
keyword was introduced in C++11 so before that it was considered a language extension by Visual Studio.– CoryKramer
Jun 26 '18 at 15:46
@CoryKramer Question is about VS Code, not VS.
– Neil Butterworth
Jun 26 '18 at 15:47
You are telling the compiler to use 2 different standards: you have both "-std=c++17" and "-std=c++11".
– Fabio Turati
Jun 26 '18 at 15:47
2
Can you compile it from the command line? That is, open a shell, go to that directory, and type
g++ -std=c++17 -g helloworld.cpp -o helloworld
: does it work?– Fabio Turati
Jun 26 '18 at 16:14
2
I'm glad that you could solve your problem, but please do not edit your post to add "SOLVED" in the title, Stack Overflow works differently. The correct way to indicate it is to accept an answer, once there is one. You could ask @Bob__ whether he is interested in posting one; if he isn't, please post it yourself and accept it. Thank you!
– Fabio Turati
Jun 26 '18 at 22:10