How to write Multiple If-Else with Return Integer value
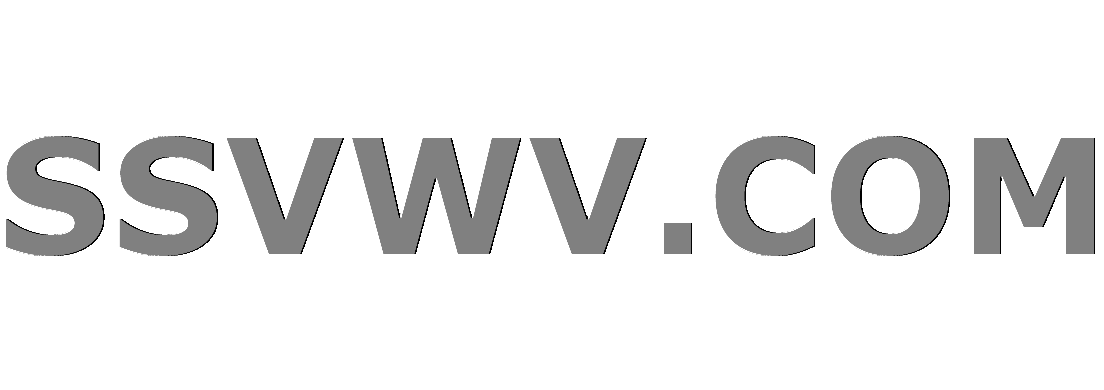
Multi tool use
def score():
edu = df.dummy
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
else: return score
Error Occured : The truth value of a Series is ambiguous. Use a.empty, a.bool(), a.item(), a.any() or a.all().
How to return a integer value with Multiple if else conditions
python-3.x
|
show 1 more comment
def score():
edu = df.dummy
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
else: return score
Error Occured : The truth value of a Series is ambiguous. Use a.empty, a.bool(), a.item(), a.any() or a.all().
How to return a integer value with Multiple if else conditions
python-3.x
What isedu
? What isdf
?
– Mureinik
Nov 22 '18 at 7:12
what's inside edu?
– Vikas Gautam
Nov 22 '18 at 7:13
Hi Mureinik, df is a Data Frame with dummy as a column of integer type and I am storing that value in edu(like 1,2,3,4,5,6,7,8)
– Kranthi Kumar Reddy
Nov 22 '18 at 7:15
You are comparing entirepandas.Series
with single int value that is why you are getting above error.
– Sociopath
Nov 22 '18 at 7:16
You want to return score right?
– Sandesh34
Nov 22 '18 at 7:22
|
show 1 more comment
def score():
edu = df.dummy
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
else: return score
Error Occured : The truth value of a Series is ambiguous. Use a.empty, a.bool(), a.item(), a.any() or a.all().
How to return a integer value with Multiple if else conditions
python-3.x
def score():
edu = df.dummy
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
else: return score
Error Occured : The truth value of a Series is ambiguous. Use a.empty, a.bool(), a.item(), a.any() or a.all().
How to return a integer value with Multiple if else conditions
python-3.x
python-3.x
edited Nov 22 '18 at 7:23


Sreeram TP
2,68921134
2,68921134
asked Nov 22 '18 at 7:08
Kranthi Kumar ReddyKranthi Kumar Reddy
102
102
What isedu
? What isdf
?
– Mureinik
Nov 22 '18 at 7:12
what's inside edu?
– Vikas Gautam
Nov 22 '18 at 7:13
Hi Mureinik, df is a Data Frame with dummy as a column of integer type and I am storing that value in edu(like 1,2,3,4,5,6,7,8)
– Kranthi Kumar Reddy
Nov 22 '18 at 7:15
You are comparing entirepandas.Series
with single int value that is why you are getting above error.
– Sociopath
Nov 22 '18 at 7:16
You want to return score right?
– Sandesh34
Nov 22 '18 at 7:22
|
show 1 more comment
What isedu
? What isdf
?
– Mureinik
Nov 22 '18 at 7:12
what's inside edu?
– Vikas Gautam
Nov 22 '18 at 7:13
Hi Mureinik, df is a Data Frame with dummy as a column of integer type and I am storing that value in edu(like 1,2,3,4,5,6,7,8)
– Kranthi Kumar Reddy
Nov 22 '18 at 7:15
You are comparing entirepandas.Series
with single int value that is why you are getting above error.
– Sociopath
Nov 22 '18 at 7:16
You want to return score right?
– Sandesh34
Nov 22 '18 at 7:22
What is
edu
? What is df
?– Mureinik
Nov 22 '18 at 7:12
What is
edu
? What is df
?– Mureinik
Nov 22 '18 at 7:12
what's inside edu?
– Vikas Gautam
Nov 22 '18 at 7:13
what's inside edu?
– Vikas Gautam
Nov 22 '18 at 7:13
Hi Mureinik, df is a Data Frame with dummy as a column of integer type and I am storing that value in edu(like 1,2,3,4,5,6,7,8)
– Kranthi Kumar Reddy
Nov 22 '18 at 7:15
Hi Mureinik, df is a Data Frame with dummy as a column of integer type and I am storing that value in edu(like 1,2,3,4,5,6,7,8)
– Kranthi Kumar Reddy
Nov 22 '18 at 7:15
You are comparing entire
pandas.Series
with single int value that is why you are getting above error.– Sociopath
Nov 22 '18 at 7:16
You are comparing entire
pandas.Series
with single int value that is why you are getting above error.– Sociopath
Nov 22 '18 at 7:16
You want to return score right?
– Sandesh34
Nov 22 '18 at 7:22
You want to return score right?
– Sandesh34
Nov 22 '18 at 7:22
|
show 1 more comment
4 Answers
4
active
oldest
votes
def edu_score():
edu = df.dummy
edu_score=
for i in edu:
if(i == 1):
score = 10
elif(i == 2):
score = 30
elif(i == 3):
score = 80
elif(i == 4):
score = 100
elif(i == 5):
score = 125
elif(i == 6):
score = 150
elif(i == 7):
score = 200
edu_score.append(score)
return edu_score
Here is your answer
add a comment |
Firstly, if edu is bigger than 7, score is not assigned to any value. So you cannot return score.
And, you can try df = pd.get_dummies(df, columns=['type'])
.
edu consists of 9 values from 0 to 8
– Kranthi Kumar Reddy
Nov 22 '18 at 7:21
The problem is about score. Not edu. If edu is 0 or 8, you dont assign any value to "score" and you try to return score.
– tgbzkl
Nov 22 '18 at 7:27
add a comment |
def score(edu):
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
else:
score=0
return score
now, when you are calling the function, pass the dataframe as
score(df.dummy).
add a comment |
You just need to clear the else statement and you're done
def scores():
score=0
edu = df.dummy
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
return score
The code u gave works fine but it takes edu == 7 only
– Kranthi Kumar Reddy
Nov 22 '18 at 7:48
oh! I accidentally put the lineedu=7
in the function. Wait, I'll edit.
– Sandesh34
Nov 22 '18 at 7:49
@KranthiKumarReddy Try now
– Sandesh34
Nov 22 '18 at 7:50
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53425552%2fhow-to-write-multiple-if-else-with-return-integer-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
def edu_score():
edu = df.dummy
edu_score=
for i in edu:
if(i == 1):
score = 10
elif(i == 2):
score = 30
elif(i == 3):
score = 80
elif(i == 4):
score = 100
elif(i == 5):
score = 125
elif(i == 6):
score = 150
elif(i == 7):
score = 200
edu_score.append(score)
return edu_score
Here is your answer
add a comment |
def edu_score():
edu = df.dummy
edu_score=
for i in edu:
if(i == 1):
score = 10
elif(i == 2):
score = 30
elif(i == 3):
score = 80
elif(i == 4):
score = 100
elif(i == 5):
score = 125
elif(i == 6):
score = 150
elif(i == 7):
score = 200
edu_score.append(score)
return edu_score
Here is your answer
add a comment |
def edu_score():
edu = df.dummy
edu_score=
for i in edu:
if(i == 1):
score = 10
elif(i == 2):
score = 30
elif(i == 3):
score = 80
elif(i == 4):
score = 100
elif(i == 5):
score = 125
elif(i == 6):
score = 150
elif(i == 7):
score = 200
edu_score.append(score)
return edu_score
Here is your answer
def edu_score():
edu = df.dummy
edu_score=
for i in edu:
if(i == 1):
score = 10
elif(i == 2):
score = 30
elif(i == 3):
score = 80
elif(i == 4):
score = 100
elif(i == 5):
score = 125
elif(i == 6):
score = 150
elif(i == 7):
score = 200
edu_score.append(score)
return edu_score
Here is your answer
answered Nov 22 '18 at 8:44
Meghana ReddyMeghana Reddy
504
504
add a comment |
add a comment |
Firstly, if edu is bigger than 7, score is not assigned to any value. So you cannot return score.
And, you can try df = pd.get_dummies(df, columns=['type'])
.
edu consists of 9 values from 0 to 8
– Kranthi Kumar Reddy
Nov 22 '18 at 7:21
The problem is about score. Not edu. If edu is 0 or 8, you dont assign any value to "score" and you try to return score.
– tgbzkl
Nov 22 '18 at 7:27
add a comment |
Firstly, if edu is bigger than 7, score is not assigned to any value. So you cannot return score.
And, you can try df = pd.get_dummies(df, columns=['type'])
.
edu consists of 9 values from 0 to 8
– Kranthi Kumar Reddy
Nov 22 '18 at 7:21
The problem is about score. Not edu. If edu is 0 or 8, you dont assign any value to "score" and you try to return score.
– tgbzkl
Nov 22 '18 at 7:27
add a comment |
Firstly, if edu is bigger than 7, score is not assigned to any value. So you cannot return score.
And, you can try df = pd.get_dummies(df, columns=['type'])
.
Firstly, if edu is bigger than 7, score is not assigned to any value. So you cannot return score.
And, you can try df = pd.get_dummies(df, columns=['type'])
.
answered Nov 22 '18 at 7:18
tgbzkltgbzkl
1919
1919
edu consists of 9 values from 0 to 8
– Kranthi Kumar Reddy
Nov 22 '18 at 7:21
The problem is about score. Not edu. If edu is 0 or 8, you dont assign any value to "score" and you try to return score.
– tgbzkl
Nov 22 '18 at 7:27
add a comment |
edu consists of 9 values from 0 to 8
– Kranthi Kumar Reddy
Nov 22 '18 at 7:21
The problem is about score. Not edu. If edu is 0 or 8, you dont assign any value to "score" and you try to return score.
– tgbzkl
Nov 22 '18 at 7:27
edu consists of 9 values from 0 to 8
– Kranthi Kumar Reddy
Nov 22 '18 at 7:21
edu consists of 9 values from 0 to 8
– Kranthi Kumar Reddy
Nov 22 '18 at 7:21
The problem is about score. Not edu. If edu is 0 or 8, you dont assign any value to "score" and you try to return score.
– tgbzkl
Nov 22 '18 at 7:27
The problem is about score. Not edu. If edu is 0 or 8, you dont assign any value to "score" and you try to return score.
– tgbzkl
Nov 22 '18 at 7:27
add a comment |
def score(edu):
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
else:
score=0
return score
now, when you are calling the function, pass the dataframe as
score(df.dummy).
add a comment |
def score(edu):
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
else:
score=0
return score
now, when you are calling the function, pass the dataframe as
score(df.dummy).
add a comment |
def score(edu):
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
else:
score=0
return score
now, when you are calling the function, pass the dataframe as
score(df.dummy).
def score(edu):
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
else:
score=0
return score
now, when you are calling the function, pass the dataframe as
score(df.dummy).
answered Nov 22 '18 at 7:32
Meghana ReddyMeghana Reddy
504
504
add a comment |
add a comment |
You just need to clear the else statement and you're done
def scores():
score=0
edu = df.dummy
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
return score
The code u gave works fine but it takes edu == 7 only
– Kranthi Kumar Reddy
Nov 22 '18 at 7:48
oh! I accidentally put the lineedu=7
in the function. Wait, I'll edit.
– Sandesh34
Nov 22 '18 at 7:49
@KranthiKumarReddy Try now
– Sandesh34
Nov 22 '18 at 7:50
add a comment |
You just need to clear the else statement and you're done
def scores():
score=0
edu = df.dummy
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
return score
The code u gave works fine but it takes edu == 7 only
– Kranthi Kumar Reddy
Nov 22 '18 at 7:48
oh! I accidentally put the lineedu=7
in the function. Wait, I'll edit.
– Sandesh34
Nov 22 '18 at 7:49
@KranthiKumarReddy Try now
– Sandesh34
Nov 22 '18 at 7:50
add a comment |
You just need to clear the else statement and you're done
def scores():
score=0
edu = df.dummy
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
return score
You just need to clear the else statement and you're done
def scores():
score=0
edu = df.dummy
if edu == 1:
score= 10
elif edu == 2:
score= 30
elif edu == 3:
score= 80
elif edu == 4:
score= 100
elif edu == 5:
score= 125
elif edu == 6:
score= 150
elif edu == 7:
score= 200
return score
edited Nov 22 '18 at 7:49
answered Nov 22 '18 at 7:27


Sandesh34Sandesh34
254112
254112
The code u gave works fine but it takes edu == 7 only
– Kranthi Kumar Reddy
Nov 22 '18 at 7:48
oh! I accidentally put the lineedu=7
in the function. Wait, I'll edit.
– Sandesh34
Nov 22 '18 at 7:49
@KranthiKumarReddy Try now
– Sandesh34
Nov 22 '18 at 7:50
add a comment |
The code u gave works fine but it takes edu == 7 only
– Kranthi Kumar Reddy
Nov 22 '18 at 7:48
oh! I accidentally put the lineedu=7
in the function. Wait, I'll edit.
– Sandesh34
Nov 22 '18 at 7:49
@KranthiKumarReddy Try now
– Sandesh34
Nov 22 '18 at 7:50
The code u gave works fine but it takes edu == 7 only
– Kranthi Kumar Reddy
Nov 22 '18 at 7:48
The code u gave works fine but it takes edu == 7 only
– Kranthi Kumar Reddy
Nov 22 '18 at 7:48
oh! I accidentally put the line
edu=7
in the function. Wait, I'll edit.– Sandesh34
Nov 22 '18 at 7:49
oh! I accidentally put the line
edu=7
in the function. Wait, I'll edit.– Sandesh34
Nov 22 '18 at 7:49
@KranthiKumarReddy Try now
– Sandesh34
Nov 22 '18 at 7:50
@KranthiKumarReddy Try now
– Sandesh34
Nov 22 '18 at 7:50
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53425552%2fhow-to-write-multiple-if-else-with-return-integer-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VlDgWxUkDhN4vIKwpBu3pzavoZJ MivkgjnZgRuBV Rm8DwOv,Vy0RCebD,Binh,0f 2 k0hlQlw7qc7Vxzk,2ban2R5n1JmK,VpR
What is
edu
? What isdf
?– Mureinik
Nov 22 '18 at 7:12
what's inside edu?
– Vikas Gautam
Nov 22 '18 at 7:13
Hi Mureinik, df is a Data Frame with dummy as a column of integer type and I am storing that value in edu(like 1,2,3,4,5,6,7,8)
– Kranthi Kumar Reddy
Nov 22 '18 at 7:15
You are comparing entire
pandas.Series
with single int value that is why you are getting above error.– Sociopath
Nov 22 '18 at 7:16
You want to return score right?
– Sandesh34
Nov 22 '18 at 7:22