Using jQuery to display text information about multiple images
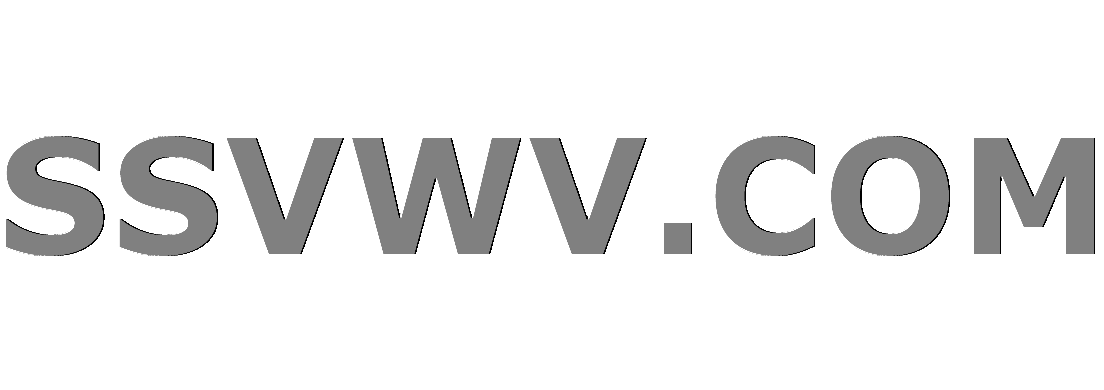
Multi tool use
I'm creating a self-contained sort of local web app using jQuery that
1) gets a list of image filenames from the user (assuming the images are all in the same folder as the .html file)
2) for each image, displays a thumbnail, the image file name, and the image's actual dimensions using image.naturalWidth and image.naturalHeight.
I managed to get it pretty much working the way I want - except that for each image displayed, the accompanying text info gets displayed after its respective the same number of times as the total number of images - i.e., the text is there once if there's a total of one images, twice if there's a total of 2 images, three times if there's 3 images, etc.
I think it has something to do with the use of $('img').each but I can't figure out how to structure it differently or even how to phrase a search for an answer.
I tried doing a JSfiddle but I can't get images to load from any remote servers for some reason. But that's fine for my purposes since I usually want to get a list of image dimensions locally.
The incremental number IDs for each image are sort of a vestigial part of an older version of this, but I'd like to keep it around in case I can think of another use for it - unless that's what's causing the problem.
Web boilerplate included just for context...
<!doctype html>
<html class="no-js" lang="">
<head>
<meta charset="utf-8">
<meta http-equiv="x-ua-compatible" content="ie=edge">
<title></title>
<meta name="description" content="">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
img {
width: 250px;
}
.inner {
font-size: 12px;
}
</style>
</head>
<body>
<textarea textarea id="image_list" rows="10" cols="70" /></textarea>
<input type='button' value='add to list' id='add' />
<table>
<div class="inner">
<ul id='list'></ul>
</div>
</table>
<script src="vendor/jquery.js"></script>
<script>
$(function() {
/* increase ID by 1 for each iteration */
var n = 0;
function increment() {
n++;
return n;
}
/* add image thumbnail and dimensions on click */
document.getElementById("add").onclick = function() {
var arrayOfLines = $('#image_list').val().split('n');
$.each(arrayOfLines, function(index, item) {
var text = arrayOfLines[n];
increment();
/* var text = arrayOfLines.value; //.value gets input values */
//Now construct a quick list element
var li = '<li class="test" id="' + n + '">' + '<img src="' + text + '">' + '</li>';
$('#list').append(li);
});
$('img').load(function() {
$('img').each(function() {
var image = this;
var theWidth = image.naturalWidth;
var theHeight = image.naturalHeight;
$(this).parent().append(' - ' + $(this).attr("src") + ' - ' + theWidth + 'x' + theHeight + ' ');
});
});
};
});
</script>
</body>
</html>
javascript jquery
add a comment |
I'm creating a self-contained sort of local web app using jQuery that
1) gets a list of image filenames from the user (assuming the images are all in the same folder as the .html file)
2) for each image, displays a thumbnail, the image file name, and the image's actual dimensions using image.naturalWidth and image.naturalHeight.
I managed to get it pretty much working the way I want - except that for each image displayed, the accompanying text info gets displayed after its respective the same number of times as the total number of images - i.e., the text is there once if there's a total of one images, twice if there's a total of 2 images, three times if there's 3 images, etc.
I think it has something to do with the use of $('img').each but I can't figure out how to structure it differently or even how to phrase a search for an answer.
I tried doing a JSfiddle but I can't get images to load from any remote servers for some reason. But that's fine for my purposes since I usually want to get a list of image dimensions locally.
The incremental number IDs for each image are sort of a vestigial part of an older version of this, but I'd like to keep it around in case I can think of another use for it - unless that's what's causing the problem.
Web boilerplate included just for context...
<!doctype html>
<html class="no-js" lang="">
<head>
<meta charset="utf-8">
<meta http-equiv="x-ua-compatible" content="ie=edge">
<title></title>
<meta name="description" content="">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
img {
width: 250px;
}
.inner {
font-size: 12px;
}
</style>
</head>
<body>
<textarea textarea id="image_list" rows="10" cols="70" /></textarea>
<input type='button' value='add to list' id='add' />
<table>
<div class="inner">
<ul id='list'></ul>
</div>
</table>
<script src="vendor/jquery.js"></script>
<script>
$(function() {
/* increase ID by 1 for each iteration */
var n = 0;
function increment() {
n++;
return n;
}
/* add image thumbnail and dimensions on click */
document.getElementById("add").onclick = function() {
var arrayOfLines = $('#image_list').val().split('n');
$.each(arrayOfLines, function(index, item) {
var text = arrayOfLines[n];
increment();
/* var text = arrayOfLines.value; //.value gets input values */
//Now construct a quick list element
var li = '<li class="test" id="' + n + '">' + '<img src="' + text + '">' + '</li>';
$('#list').append(li);
});
$('img').load(function() {
$('img').each(function() {
var image = this;
var theWidth = image.naturalWidth;
var theHeight = image.naturalHeight;
$(this).parent().append(' - ' + $(this).attr("src") + ' - ' + theWidth + 'x' + theHeight + ' ');
});
});
};
});
</script>
</body>
</html>
javascript jquery
1
Remove the$('img').each
completely as you are already in theload
event which is fired for each image.
– Gabriele Petrioli
Nov 21 '18 at 22:56
Brilliant! That did the trick. Thank you.
– moai-kun
Nov 21 '18 at 22:59
add a comment |
I'm creating a self-contained sort of local web app using jQuery that
1) gets a list of image filenames from the user (assuming the images are all in the same folder as the .html file)
2) for each image, displays a thumbnail, the image file name, and the image's actual dimensions using image.naturalWidth and image.naturalHeight.
I managed to get it pretty much working the way I want - except that for each image displayed, the accompanying text info gets displayed after its respective the same number of times as the total number of images - i.e., the text is there once if there's a total of one images, twice if there's a total of 2 images, three times if there's 3 images, etc.
I think it has something to do with the use of $('img').each but I can't figure out how to structure it differently or even how to phrase a search for an answer.
I tried doing a JSfiddle but I can't get images to load from any remote servers for some reason. But that's fine for my purposes since I usually want to get a list of image dimensions locally.
The incremental number IDs for each image are sort of a vestigial part of an older version of this, but I'd like to keep it around in case I can think of another use for it - unless that's what's causing the problem.
Web boilerplate included just for context...
<!doctype html>
<html class="no-js" lang="">
<head>
<meta charset="utf-8">
<meta http-equiv="x-ua-compatible" content="ie=edge">
<title></title>
<meta name="description" content="">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
img {
width: 250px;
}
.inner {
font-size: 12px;
}
</style>
</head>
<body>
<textarea textarea id="image_list" rows="10" cols="70" /></textarea>
<input type='button' value='add to list' id='add' />
<table>
<div class="inner">
<ul id='list'></ul>
</div>
</table>
<script src="vendor/jquery.js"></script>
<script>
$(function() {
/* increase ID by 1 for each iteration */
var n = 0;
function increment() {
n++;
return n;
}
/* add image thumbnail and dimensions on click */
document.getElementById("add").onclick = function() {
var arrayOfLines = $('#image_list').val().split('n');
$.each(arrayOfLines, function(index, item) {
var text = arrayOfLines[n];
increment();
/* var text = arrayOfLines.value; //.value gets input values */
//Now construct a quick list element
var li = '<li class="test" id="' + n + '">' + '<img src="' + text + '">' + '</li>';
$('#list').append(li);
});
$('img').load(function() {
$('img').each(function() {
var image = this;
var theWidth = image.naturalWidth;
var theHeight = image.naturalHeight;
$(this).parent().append(' - ' + $(this).attr("src") + ' - ' + theWidth + 'x' + theHeight + ' ');
});
});
};
});
</script>
</body>
</html>
javascript jquery
I'm creating a self-contained sort of local web app using jQuery that
1) gets a list of image filenames from the user (assuming the images are all in the same folder as the .html file)
2) for each image, displays a thumbnail, the image file name, and the image's actual dimensions using image.naturalWidth and image.naturalHeight.
I managed to get it pretty much working the way I want - except that for each image displayed, the accompanying text info gets displayed after its respective the same number of times as the total number of images - i.e., the text is there once if there's a total of one images, twice if there's a total of 2 images, three times if there's 3 images, etc.
I think it has something to do with the use of $('img').each but I can't figure out how to structure it differently or even how to phrase a search for an answer.
I tried doing a JSfiddle but I can't get images to load from any remote servers for some reason. But that's fine for my purposes since I usually want to get a list of image dimensions locally.
The incremental number IDs for each image are sort of a vestigial part of an older version of this, but I'd like to keep it around in case I can think of another use for it - unless that's what's causing the problem.
Web boilerplate included just for context...
<!doctype html>
<html class="no-js" lang="">
<head>
<meta charset="utf-8">
<meta http-equiv="x-ua-compatible" content="ie=edge">
<title></title>
<meta name="description" content="">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
img {
width: 250px;
}
.inner {
font-size: 12px;
}
</style>
</head>
<body>
<textarea textarea id="image_list" rows="10" cols="70" /></textarea>
<input type='button' value='add to list' id='add' />
<table>
<div class="inner">
<ul id='list'></ul>
</div>
</table>
<script src="vendor/jquery.js"></script>
<script>
$(function() {
/* increase ID by 1 for each iteration */
var n = 0;
function increment() {
n++;
return n;
}
/* add image thumbnail and dimensions on click */
document.getElementById("add").onclick = function() {
var arrayOfLines = $('#image_list').val().split('n');
$.each(arrayOfLines, function(index, item) {
var text = arrayOfLines[n];
increment();
/* var text = arrayOfLines.value; //.value gets input values */
//Now construct a quick list element
var li = '<li class="test" id="' + n + '">' + '<img src="' + text + '">' + '</li>';
$('#list').append(li);
});
$('img').load(function() {
$('img').each(function() {
var image = this;
var theWidth = image.naturalWidth;
var theHeight = image.naturalHeight;
$(this).parent().append(' - ' + $(this).attr("src") + ' - ' + theWidth + 'x' + theHeight + ' ');
});
});
};
});
</script>
</body>
</html>
javascript jquery
javascript jquery
edited Nov 21 '18 at 22:58
moai-kun
asked Nov 21 '18 at 22:40
moai-kunmoai-kun
62
62
1
Remove the$('img').each
completely as you are already in theload
event which is fired for each image.
– Gabriele Petrioli
Nov 21 '18 at 22:56
Brilliant! That did the trick. Thank you.
– moai-kun
Nov 21 '18 at 22:59
add a comment |
1
Remove the$('img').each
completely as you are already in theload
event which is fired for each image.
– Gabriele Petrioli
Nov 21 '18 at 22:56
Brilliant! That did the trick. Thank you.
– moai-kun
Nov 21 '18 at 22:59
1
1
Remove the
$('img').each
completely as you are already in the load
event which is fired for each image.– Gabriele Petrioli
Nov 21 '18 at 22:56
Remove the
$('img').each
completely as you are already in the load
event which is fired for each image.– Gabriele Petrioli
Nov 21 '18 at 22:56
Brilliant! That did the trick. Thank you.
– moai-kun
Nov 21 '18 at 22:59
Brilliant! That did the trick. Thank you.
– moai-kun
Nov 21 '18 at 22:59
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53421432%2fusing-jquery-to-display-text-information-about-multiple-images%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53421432%2fusing-jquery-to-display-text-information-about-multiple-images%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DlIyuZe7Ga fttw5gtZX9ylv3 5tkv,3 BePxs
1
Remove the
$('img').each
completely as you are already in theload
event which is fired for each image.– Gabriele Petrioli
Nov 21 '18 at 22:56
Brilliant! That did the trick. Thank you.
– moai-kun
Nov 21 '18 at 22:59