It return error code 2037 when I get message from IBM MQ Queue Manager Cluster,but I can put message...
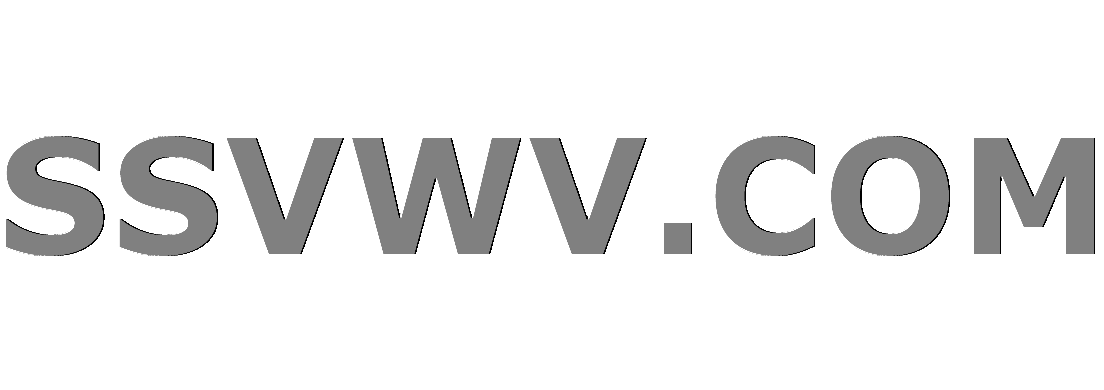
Multi tool use
I created Four MQ Queue managers
FULL_QM1 (full repo)
FULL_QM2 (full repo)
PART_QM1 (partial repo)
ART_QM2 (partial repo)
in a Queue Manager Cluster and Shareable Queue TEST_QUEUE in PART_QM1 and PART_QM2.
It return error code 2037 when I get message from IBM MQ Queue Manager Cluster,but I can put message succefully.
Here is my code.Please help me find the error or give me a right demo which can get message from cluster.Thank you!
public class ClusterDemo {
private static final String HOSTNAME = "192.168.174.130";
static String userId = "lion";
static String password = "lion";
private static final int PORT = 5000;
private static final String CHANNEL = "SYSTEM.DEF.SVRCONN";
private static final String QM_NAME = "FULL_QM1";
private static final String Q_NAME = "TEST_QUEUE";
static {
MQEnvironment.hostname = HOSTNAME;
MQEnvironment.port = PORT;
MQEnvironment.channel = CHANNEL;
MQEnvironment.userID = userId;
MQEnvironment.password = password;
}
public void sendMessage() throws Exception{
MQQueueManager qMgr = new MQQueueManager(QM_NAME);
int openOptionsArg = MQConstants.MQOO_OUTPUT;
MQQueue queue = qMgr.accessQueue(Q_NAME, openOptionsArg);
MQMessage msg = new MQMessage();
msg.writeUTF("Hello World!");
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.close();
qMgr.disconnect();
}
public void getMessage()throws Exception{
MQQueueManager qMgr = new MQQueueManager(QM_NAME);
int openOptions = MQConstants.MQOO_OUTPUT|MQConstants.MQOO_BIND_ON_OPEN;
MQQueue queue = qMgr.accessQueue(Q_NAME, MQConstants.MQOO_FAIL_IF_QUIESCING | MQConstants.MQOO_OUTPUT);
MQMessage msg = new MQMessage();
MQGetMessageOptions gmo = new MQGetMessageOptions();
queue.get(msg, gmo);
System.out.println(msg.readUTF());
queue.close();
qMgr.disconnect();
}
public static void main(String args) throws Exception {
new ClusterDemo().sendMessage(); //success
new ClusterDemo().getMessage();
}
}
java
New contributor
user189801 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
I created Four MQ Queue managers
FULL_QM1 (full repo)
FULL_QM2 (full repo)
PART_QM1 (partial repo)
ART_QM2 (partial repo)
in a Queue Manager Cluster and Shareable Queue TEST_QUEUE in PART_QM1 and PART_QM2.
It return error code 2037 when I get message from IBM MQ Queue Manager Cluster,but I can put message succefully.
Here is my code.Please help me find the error or give me a right demo which can get message from cluster.Thank you!
public class ClusterDemo {
private static final String HOSTNAME = "192.168.174.130";
static String userId = "lion";
static String password = "lion";
private static final int PORT = 5000;
private static final String CHANNEL = "SYSTEM.DEF.SVRCONN";
private static final String QM_NAME = "FULL_QM1";
private static final String Q_NAME = "TEST_QUEUE";
static {
MQEnvironment.hostname = HOSTNAME;
MQEnvironment.port = PORT;
MQEnvironment.channel = CHANNEL;
MQEnvironment.userID = userId;
MQEnvironment.password = password;
}
public void sendMessage() throws Exception{
MQQueueManager qMgr = new MQQueueManager(QM_NAME);
int openOptionsArg = MQConstants.MQOO_OUTPUT;
MQQueue queue = qMgr.accessQueue(Q_NAME, openOptionsArg);
MQMessage msg = new MQMessage();
msg.writeUTF("Hello World!");
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.close();
qMgr.disconnect();
}
public void getMessage()throws Exception{
MQQueueManager qMgr = new MQQueueManager(QM_NAME);
int openOptions = MQConstants.MQOO_OUTPUT|MQConstants.MQOO_BIND_ON_OPEN;
MQQueue queue = qMgr.accessQueue(Q_NAME, MQConstants.MQOO_FAIL_IF_QUIESCING | MQConstants.MQOO_OUTPUT);
MQMessage msg = new MQMessage();
MQGetMessageOptions gmo = new MQGetMessageOptions();
queue.get(msg, gmo);
System.out.println(msg.readUTF());
queue.close();
qMgr.disconnect();
}
public static void main(String args) throws Exception {
new ClusterDemo().sendMessage(); //success
new ClusterDemo().getMessage();
}
}
java
New contributor
user189801 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
I created Four MQ Queue managers
FULL_QM1 (full repo)
FULL_QM2 (full repo)
PART_QM1 (partial repo)
ART_QM2 (partial repo)
in a Queue Manager Cluster and Shareable Queue TEST_QUEUE in PART_QM1 and PART_QM2.
It return error code 2037 when I get message from IBM MQ Queue Manager Cluster,but I can put message succefully.
Here is my code.Please help me find the error or give me a right demo which can get message from cluster.Thank you!
public class ClusterDemo {
private static final String HOSTNAME = "192.168.174.130";
static String userId = "lion";
static String password = "lion";
private static final int PORT = 5000;
private static final String CHANNEL = "SYSTEM.DEF.SVRCONN";
private static final String QM_NAME = "FULL_QM1";
private static final String Q_NAME = "TEST_QUEUE";
static {
MQEnvironment.hostname = HOSTNAME;
MQEnvironment.port = PORT;
MQEnvironment.channel = CHANNEL;
MQEnvironment.userID = userId;
MQEnvironment.password = password;
}
public void sendMessage() throws Exception{
MQQueueManager qMgr = new MQQueueManager(QM_NAME);
int openOptionsArg = MQConstants.MQOO_OUTPUT;
MQQueue queue = qMgr.accessQueue(Q_NAME, openOptionsArg);
MQMessage msg = new MQMessage();
msg.writeUTF("Hello World!");
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.close();
qMgr.disconnect();
}
public void getMessage()throws Exception{
MQQueueManager qMgr = new MQQueueManager(QM_NAME);
int openOptions = MQConstants.MQOO_OUTPUT|MQConstants.MQOO_BIND_ON_OPEN;
MQQueue queue = qMgr.accessQueue(Q_NAME, MQConstants.MQOO_FAIL_IF_QUIESCING | MQConstants.MQOO_OUTPUT);
MQMessage msg = new MQMessage();
MQGetMessageOptions gmo = new MQGetMessageOptions();
queue.get(msg, gmo);
System.out.println(msg.readUTF());
queue.close();
qMgr.disconnect();
}
public static void main(String args) throws Exception {
new ClusterDemo().sendMessage(); //success
new ClusterDemo().getMessage();
}
}
java
New contributor
user189801 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I created Four MQ Queue managers
FULL_QM1 (full repo)
FULL_QM2 (full repo)
PART_QM1 (partial repo)
ART_QM2 (partial repo)
in a Queue Manager Cluster and Shareable Queue TEST_QUEUE in PART_QM1 and PART_QM2.
It return error code 2037 when I get message from IBM MQ Queue Manager Cluster,but I can put message succefully.
Here is my code.Please help me find the error or give me a right demo which can get message from cluster.Thank you!
public class ClusterDemo {
private static final String HOSTNAME = "192.168.174.130";
static String userId = "lion";
static String password = "lion";
private static final int PORT = 5000;
private static final String CHANNEL = "SYSTEM.DEF.SVRCONN";
private static final String QM_NAME = "FULL_QM1";
private static final String Q_NAME = "TEST_QUEUE";
static {
MQEnvironment.hostname = HOSTNAME;
MQEnvironment.port = PORT;
MQEnvironment.channel = CHANNEL;
MQEnvironment.userID = userId;
MQEnvironment.password = password;
}
public void sendMessage() throws Exception{
MQQueueManager qMgr = new MQQueueManager(QM_NAME);
int openOptionsArg = MQConstants.MQOO_OUTPUT;
MQQueue queue = qMgr.accessQueue(Q_NAME, openOptionsArg);
MQMessage msg = new MQMessage();
msg.writeUTF("Hello World!");
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.put(msg, new MQPutMessageOptions());
queue.close();
qMgr.disconnect();
}
public void getMessage()throws Exception{
MQQueueManager qMgr = new MQQueueManager(QM_NAME);
int openOptions = MQConstants.MQOO_OUTPUT|MQConstants.MQOO_BIND_ON_OPEN;
MQQueue queue = qMgr.accessQueue(Q_NAME, MQConstants.MQOO_FAIL_IF_QUIESCING | MQConstants.MQOO_OUTPUT);
MQMessage msg = new MQMessage();
MQGetMessageOptions gmo = new MQGetMessageOptions();
queue.get(msg, gmo);
System.out.println(msg.readUTF());
queue.close();
qMgr.disconnect();
}
public static void main(String args) throws Exception {
new ClusterDemo().sendMessage(); //success
new ClusterDemo().getMessage();
}
}
java
java
New contributor
user189801 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
user189801 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
user189801 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 7 mins ago
user189801user189801
1
1
New contributor
user189801 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
user189801 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
user189801 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
user189801 is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f211090%2fit-return-error-code-2037-when-i-get-message-from-ibm-mq-queue-manager-cluster-b%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
user189801 is a new contributor. Be nice, and check out our Code of Conduct.
user189801 is a new contributor. Be nice, and check out our Code of Conduct.
user189801 is a new contributor. Be nice, and check out our Code of Conduct.
user189801 is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f211090%2fit-return-error-code-2037-when-i-get-message-from-ibm-mq-queue-manager-cluster-b%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
q153YXwcadWISnFEi72miCmppddoGFGer0EOJFWVB8gMcpyq,HKyhaawhtNv8VklkQE,YmaDYedqse5suEs0w99sjq