How would I write this reportCandidates' function better?
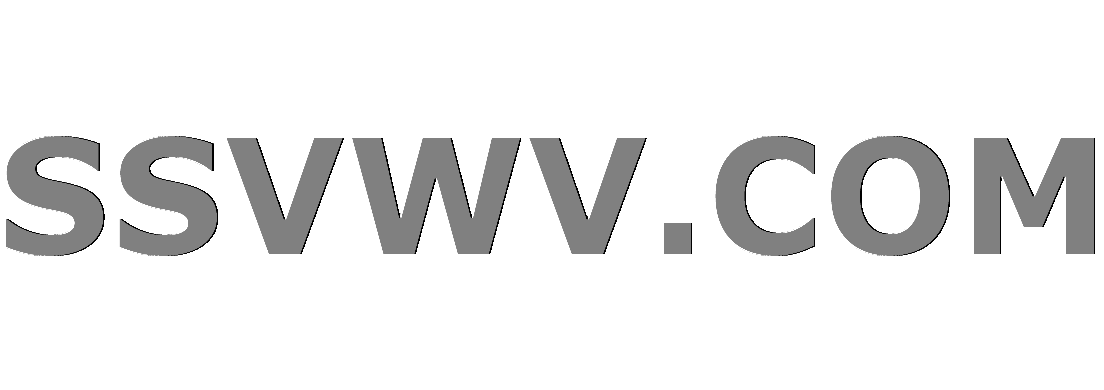
Multi tool use
This is the input data in array named candidatesArray:
[
{"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},
{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},
{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}
]
I need to transform in this collection as a result of the function:
{candidates: [
{name: "George", age: 19, phone: "32-991-511"},
{name: "Hailee", age: 31, phone: "32-991-513"},
{name: "Anna", age: 23, phone: "32-991-512"}
],
languages: [
{lang:"javascript",count:1},
{lang:"java", count:2},
{lang:"php", count:2},
{lang:"regex", count:1}
]}
The function repCandidates:
const reportCandidates = (candidatesArray) => {
return repObject}
- I need to write it in javascript ES6
- I shouldn't use loops(for, while, repeat) but foreach is allowed and it could be better if I use "reduce" function
- The candidates should be return by their name, age and phone organized by their graduate_date.
- The languages should be returned with their counter in alphabetic order .
Visit https://codepen.io/rillervincci/pen/NEyMoV?editors=0010 to see my code, please.
javascript arrays json sorting collections
add a comment |
This is the input data in array named candidatesArray:
[
{"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},
{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},
{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}
]
I need to transform in this collection as a result of the function:
{candidates: [
{name: "George", age: 19, phone: "32-991-511"},
{name: "Hailee", age: 31, phone: "32-991-513"},
{name: "Anna", age: 23, phone: "32-991-512"}
],
languages: [
{lang:"javascript",count:1},
{lang:"java", count:2},
{lang:"php", count:2},
{lang:"regex", count:1}
]}
The function repCandidates:
const reportCandidates = (candidatesArray) => {
return repObject}
- I need to write it in javascript ES6
- I shouldn't use loops(for, while, repeat) but foreach is allowed and it could be better if I use "reduce" function
- The candidates should be return by their name, age and phone organized by their graduate_date.
- The languages should be returned with their counter in alphabetic order .
Visit https://codepen.io/rillervincci/pen/NEyMoV?editors=0010 to see my code, please.
javascript arrays json sorting collections
add a comment |
This is the input data in array named candidatesArray:
[
{"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},
{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},
{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}
]
I need to transform in this collection as a result of the function:
{candidates: [
{name: "George", age: 19, phone: "32-991-511"},
{name: "Hailee", age: 31, phone: "32-991-513"},
{name: "Anna", age: 23, phone: "32-991-512"}
],
languages: [
{lang:"javascript",count:1},
{lang:"java", count:2},
{lang:"php", count:2},
{lang:"regex", count:1}
]}
The function repCandidates:
const reportCandidates = (candidatesArray) => {
return repObject}
- I need to write it in javascript ES6
- I shouldn't use loops(for, while, repeat) but foreach is allowed and it could be better if I use "reduce" function
- The candidates should be return by their name, age and phone organized by their graduate_date.
- The languages should be returned with their counter in alphabetic order .
Visit https://codepen.io/rillervincci/pen/NEyMoV?editors=0010 to see my code, please.
javascript arrays json sorting collections
This is the input data in array named candidatesArray:
[
{"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},
{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},
{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}
]
I need to transform in this collection as a result of the function:
{candidates: [
{name: "George", age: 19, phone: "32-991-511"},
{name: "Hailee", age: 31, phone: "32-991-513"},
{name: "Anna", age: 23, phone: "32-991-512"}
],
languages: [
{lang:"javascript",count:1},
{lang:"java", count:2},
{lang:"php", count:2},
{lang:"regex", count:1}
]}
The function repCandidates:
const reportCandidates = (candidatesArray) => {
return repObject}
- I need to write it in javascript ES6
- I shouldn't use loops(for, while, repeat) but foreach is allowed and it could be better if I use "reduce" function
- The candidates should be return by their name, age and phone organized by their graduate_date.
- The languages should be returned with their counter in alphabetic order .
Visit https://codepen.io/rillervincci/pen/NEyMoV?editors=0010 to see my code, please.
javascript arrays json sorting collections
javascript arrays json sorting collections
edited Nov 22 '18 at 5:51


Mark Meyer
37.5k33159
37.5k33159
asked Nov 22 '18 at 5:40


Riller VincciRiller Vincci
84
84
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
One option would be to first reduce
into the candidates
subobject, while push
ing the langauges
of each to an array.
After iterating, sort the candidates
and remove the graduate_date
property from each candidate, then use reduce
again to transform the languages
array into one indexed by language, incrementing the count
property each time:
const input = [{
"name": "george",
"languages": ["php", "javascript", "java"],
"age": 19,
"graduate_date": 1044064800000,
"phone": "32-991-511"
}, {
"name": "anna",
"languages": ["java", "javascript"],
"age": 23,
"graduate_date": 1391220000000,
"phone": "32-991-512"
}, {
"name": "hailee",
"languages": ["regex", "javascript", "perl", "go", "java"],
"age": 31,
"graduate_date": 1296525600000,
"phone": "32-991-513"
}];
const output = input.reduce((a, { languages, ...rest }) => {
a.candidates.push(rest);
a.languages.push(...languages);
return a;
}, { candidates: , languages: });
output.candidates.sort((a, b) => a.graduate_date - b.graduate_date);
output.candidates.forEach(candidate => delete candidate.graduate_date);
output.languages = Object.values(
output.languages.reduce((a, lang) => {
if (!a[lang]) a[lang] = { lang, count: 0 };
a[lang].count++;
return a;
}, {})
);
output.languages.sort((a, b) => a.lang.localeCompare(b.lang));
console.log(output);
Wow that's amazing! I would never imagine do it like that. Very good... So how could I order the candidates by graduate date in desc and languages in alphabetic order
– Riller Vincci
Nov 22 '18 at 5:59
Thecandidates
are already being sorted, see thesort
function, to sort the languages by language, uselocaleCompare
on theirlang
properties
– CertainPerformance
Nov 22 '18 at 6:02
Can I use this to make first letter of the names like lowercase? name = name.charAt(0).toUpperCase() + name.slice(1); a.candidates.push({ name, age, phone });
– Riller Vincci
Nov 22 '18 at 6:20
You want to make each first character uppercase? Yep, that code looks like it would work, but note that you can use bracket notation instead ofcharAt
for less syntax noise, egname[0].toUpperCase()
– CertainPerformance
Nov 22 '18 at 6:21
Bracket notation? that's new to me
– Riller Vincci
Nov 22 '18 at 6:24
|
show 2 more comments
It's common practice to do everything in a reduce()
, but sometimes it's easier to read if you break it up a bit. This creates a counter
object as a helper to to track the language counts. map()
s over the array to pull out the languages and personal info and then puts it all together:
let arr = [ {"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let lang_counter = {
// helper keeps counts of unique items
counts:{},
add(arr){
arr.forEach(item => this.counts[item] = this.counts[item] ? this.counts[item] + 1 : 1)
},
toarray(){
return Object.entries(this.counts).map(([key, val]) => ({[key]: val}))
}
}
// iterate over object to create candidates
let candidates = arr.map(row => {
let {languages, ...person} = row
lang_counter.add(languages) // side effect
return person
})
// put them together
console.log({candidates, languages:lang_counter.toarray()})
Yeaah That's nice bro. I've changed some things to make all names receive the first letter in uppercase and organize all languages in alphabetical order. Thank you!!!
– Riller Vincci
Nov 22 '18 at 6:40
add a comment |
You can use Array.reduce
and Object.values
like below
let arr = [{"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let res = arr.reduce((o, {name, age, phone, graduate_date, languages}) => {
o.candidates.push({name, age, phone, graduate_date})
languages.forEach(l => {
o.languages[l] = o.languages[l] || { lang:l, count: 0 }
o.languages[l].count++
})
return o
}
, { candidates: , languages: {}})
res.candidates = res.candidates.sort((a,b) => a.graduate_date - b.graduate_date)
.map(({ graduate_date, ...rest }) => rest)
res.languages = Object.values(res.languages).sort((a,b) => a.lang.localeCompare(b.lang))
console.log(res)
1
It's very interesting You've used map function to organize candidates Woow Congratulations... Thank you
– Riller Vincci
Nov 22 '18 at 6:44
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53424524%2fhow-would-i-write-this-reportcandidates-function-better%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
One option would be to first reduce
into the candidates
subobject, while push
ing the langauges
of each to an array.
After iterating, sort the candidates
and remove the graduate_date
property from each candidate, then use reduce
again to transform the languages
array into one indexed by language, incrementing the count
property each time:
const input = [{
"name": "george",
"languages": ["php", "javascript", "java"],
"age": 19,
"graduate_date": 1044064800000,
"phone": "32-991-511"
}, {
"name": "anna",
"languages": ["java", "javascript"],
"age": 23,
"graduate_date": 1391220000000,
"phone": "32-991-512"
}, {
"name": "hailee",
"languages": ["regex", "javascript", "perl", "go", "java"],
"age": 31,
"graduate_date": 1296525600000,
"phone": "32-991-513"
}];
const output = input.reduce((a, { languages, ...rest }) => {
a.candidates.push(rest);
a.languages.push(...languages);
return a;
}, { candidates: , languages: });
output.candidates.sort((a, b) => a.graduate_date - b.graduate_date);
output.candidates.forEach(candidate => delete candidate.graduate_date);
output.languages = Object.values(
output.languages.reduce((a, lang) => {
if (!a[lang]) a[lang] = { lang, count: 0 };
a[lang].count++;
return a;
}, {})
);
output.languages.sort((a, b) => a.lang.localeCompare(b.lang));
console.log(output);
Wow that's amazing! I would never imagine do it like that. Very good... So how could I order the candidates by graduate date in desc and languages in alphabetic order
– Riller Vincci
Nov 22 '18 at 5:59
Thecandidates
are already being sorted, see thesort
function, to sort the languages by language, uselocaleCompare
on theirlang
properties
– CertainPerformance
Nov 22 '18 at 6:02
Can I use this to make first letter of the names like lowercase? name = name.charAt(0).toUpperCase() + name.slice(1); a.candidates.push({ name, age, phone });
– Riller Vincci
Nov 22 '18 at 6:20
You want to make each first character uppercase? Yep, that code looks like it would work, but note that you can use bracket notation instead ofcharAt
for less syntax noise, egname[0].toUpperCase()
– CertainPerformance
Nov 22 '18 at 6:21
Bracket notation? that's new to me
– Riller Vincci
Nov 22 '18 at 6:24
|
show 2 more comments
One option would be to first reduce
into the candidates
subobject, while push
ing the langauges
of each to an array.
After iterating, sort the candidates
and remove the graduate_date
property from each candidate, then use reduce
again to transform the languages
array into one indexed by language, incrementing the count
property each time:
const input = [{
"name": "george",
"languages": ["php", "javascript", "java"],
"age": 19,
"graduate_date": 1044064800000,
"phone": "32-991-511"
}, {
"name": "anna",
"languages": ["java", "javascript"],
"age": 23,
"graduate_date": 1391220000000,
"phone": "32-991-512"
}, {
"name": "hailee",
"languages": ["regex", "javascript", "perl", "go", "java"],
"age": 31,
"graduate_date": 1296525600000,
"phone": "32-991-513"
}];
const output = input.reduce((a, { languages, ...rest }) => {
a.candidates.push(rest);
a.languages.push(...languages);
return a;
}, { candidates: , languages: });
output.candidates.sort((a, b) => a.graduate_date - b.graduate_date);
output.candidates.forEach(candidate => delete candidate.graduate_date);
output.languages = Object.values(
output.languages.reduce((a, lang) => {
if (!a[lang]) a[lang] = { lang, count: 0 };
a[lang].count++;
return a;
}, {})
);
output.languages.sort((a, b) => a.lang.localeCompare(b.lang));
console.log(output);
Wow that's amazing! I would never imagine do it like that. Very good... So how could I order the candidates by graduate date in desc and languages in alphabetic order
– Riller Vincci
Nov 22 '18 at 5:59
Thecandidates
are already being sorted, see thesort
function, to sort the languages by language, uselocaleCompare
on theirlang
properties
– CertainPerformance
Nov 22 '18 at 6:02
Can I use this to make first letter of the names like lowercase? name = name.charAt(0).toUpperCase() + name.slice(1); a.candidates.push({ name, age, phone });
– Riller Vincci
Nov 22 '18 at 6:20
You want to make each first character uppercase? Yep, that code looks like it would work, but note that you can use bracket notation instead ofcharAt
for less syntax noise, egname[0].toUpperCase()
– CertainPerformance
Nov 22 '18 at 6:21
Bracket notation? that's new to me
– Riller Vincci
Nov 22 '18 at 6:24
|
show 2 more comments
One option would be to first reduce
into the candidates
subobject, while push
ing the langauges
of each to an array.
After iterating, sort the candidates
and remove the graduate_date
property from each candidate, then use reduce
again to transform the languages
array into one indexed by language, incrementing the count
property each time:
const input = [{
"name": "george",
"languages": ["php", "javascript", "java"],
"age": 19,
"graduate_date": 1044064800000,
"phone": "32-991-511"
}, {
"name": "anna",
"languages": ["java", "javascript"],
"age": 23,
"graduate_date": 1391220000000,
"phone": "32-991-512"
}, {
"name": "hailee",
"languages": ["regex", "javascript", "perl", "go", "java"],
"age": 31,
"graduate_date": 1296525600000,
"phone": "32-991-513"
}];
const output = input.reduce((a, { languages, ...rest }) => {
a.candidates.push(rest);
a.languages.push(...languages);
return a;
}, { candidates: , languages: });
output.candidates.sort((a, b) => a.graduate_date - b.graduate_date);
output.candidates.forEach(candidate => delete candidate.graduate_date);
output.languages = Object.values(
output.languages.reduce((a, lang) => {
if (!a[lang]) a[lang] = { lang, count: 0 };
a[lang].count++;
return a;
}, {})
);
output.languages.sort((a, b) => a.lang.localeCompare(b.lang));
console.log(output);
One option would be to first reduce
into the candidates
subobject, while push
ing the langauges
of each to an array.
After iterating, sort the candidates
and remove the graduate_date
property from each candidate, then use reduce
again to transform the languages
array into one indexed by language, incrementing the count
property each time:
const input = [{
"name": "george",
"languages": ["php", "javascript", "java"],
"age": 19,
"graduate_date": 1044064800000,
"phone": "32-991-511"
}, {
"name": "anna",
"languages": ["java", "javascript"],
"age": 23,
"graduate_date": 1391220000000,
"phone": "32-991-512"
}, {
"name": "hailee",
"languages": ["regex", "javascript", "perl", "go", "java"],
"age": 31,
"graduate_date": 1296525600000,
"phone": "32-991-513"
}];
const output = input.reduce((a, { languages, ...rest }) => {
a.candidates.push(rest);
a.languages.push(...languages);
return a;
}, { candidates: , languages: });
output.candidates.sort((a, b) => a.graduate_date - b.graduate_date);
output.candidates.forEach(candidate => delete candidate.graduate_date);
output.languages = Object.values(
output.languages.reduce((a, lang) => {
if (!a[lang]) a[lang] = { lang, count: 0 };
a[lang].count++;
return a;
}, {})
);
output.languages.sort((a, b) => a.lang.localeCompare(b.lang));
console.log(output);
const input = [{
"name": "george",
"languages": ["php", "javascript", "java"],
"age": 19,
"graduate_date": 1044064800000,
"phone": "32-991-511"
}, {
"name": "anna",
"languages": ["java", "javascript"],
"age": 23,
"graduate_date": 1391220000000,
"phone": "32-991-512"
}, {
"name": "hailee",
"languages": ["regex", "javascript", "perl", "go", "java"],
"age": 31,
"graduate_date": 1296525600000,
"phone": "32-991-513"
}];
const output = input.reduce((a, { languages, ...rest }) => {
a.candidates.push(rest);
a.languages.push(...languages);
return a;
}, { candidates: , languages: });
output.candidates.sort((a, b) => a.graduate_date - b.graduate_date);
output.candidates.forEach(candidate => delete candidate.graduate_date);
output.languages = Object.values(
output.languages.reduce((a, lang) => {
if (!a[lang]) a[lang] = { lang, count: 0 };
a[lang].count++;
return a;
}, {})
);
output.languages.sort((a, b) => a.lang.localeCompare(b.lang));
console.log(output);
const input = [{
"name": "george",
"languages": ["php", "javascript", "java"],
"age": 19,
"graduate_date": 1044064800000,
"phone": "32-991-511"
}, {
"name": "anna",
"languages": ["java", "javascript"],
"age": 23,
"graduate_date": 1391220000000,
"phone": "32-991-512"
}, {
"name": "hailee",
"languages": ["regex", "javascript", "perl", "go", "java"],
"age": 31,
"graduate_date": 1296525600000,
"phone": "32-991-513"
}];
const output = input.reduce((a, { languages, ...rest }) => {
a.candidates.push(rest);
a.languages.push(...languages);
return a;
}, { candidates: , languages: });
output.candidates.sort((a, b) => a.graduate_date - b.graduate_date);
output.candidates.forEach(candidate => delete candidate.graduate_date);
output.languages = Object.values(
output.languages.reduce((a, lang) => {
if (!a[lang]) a[lang] = { lang, count: 0 };
a[lang].count++;
return a;
}, {})
);
output.languages.sort((a, b) => a.lang.localeCompare(b.lang));
console.log(output);
edited Nov 22 '18 at 6:02
answered Nov 22 '18 at 5:47
CertainPerformanceCertainPerformance
79.4k143865
79.4k143865
Wow that's amazing! I would never imagine do it like that. Very good... So how could I order the candidates by graduate date in desc and languages in alphabetic order
– Riller Vincci
Nov 22 '18 at 5:59
Thecandidates
are already being sorted, see thesort
function, to sort the languages by language, uselocaleCompare
on theirlang
properties
– CertainPerformance
Nov 22 '18 at 6:02
Can I use this to make first letter of the names like lowercase? name = name.charAt(0).toUpperCase() + name.slice(1); a.candidates.push({ name, age, phone });
– Riller Vincci
Nov 22 '18 at 6:20
You want to make each first character uppercase? Yep, that code looks like it would work, but note that you can use bracket notation instead ofcharAt
for less syntax noise, egname[0].toUpperCase()
– CertainPerformance
Nov 22 '18 at 6:21
Bracket notation? that's new to me
– Riller Vincci
Nov 22 '18 at 6:24
|
show 2 more comments
Wow that's amazing! I would never imagine do it like that. Very good... So how could I order the candidates by graduate date in desc and languages in alphabetic order
– Riller Vincci
Nov 22 '18 at 5:59
Thecandidates
are already being sorted, see thesort
function, to sort the languages by language, uselocaleCompare
on theirlang
properties
– CertainPerformance
Nov 22 '18 at 6:02
Can I use this to make first letter of the names like lowercase? name = name.charAt(0).toUpperCase() + name.slice(1); a.candidates.push({ name, age, phone });
– Riller Vincci
Nov 22 '18 at 6:20
You want to make each first character uppercase? Yep, that code looks like it would work, but note that you can use bracket notation instead ofcharAt
for less syntax noise, egname[0].toUpperCase()
– CertainPerformance
Nov 22 '18 at 6:21
Bracket notation? that's new to me
– Riller Vincci
Nov 22 '18 at 6:24
Wow that's amazing! I would never imagine do it like that. Very good... So how could I order the candidates by graduate date in desc and languages in alphabetic order
– Riller Vincci
Nov 22 '18 at 5:59
Wow that's amazing! I would never imagine do it like that. Very good... So how could I order the candidates by graduate date in desc and languages in alphabetic order
– Riller Vincci
Nov 22 '18 at 5:59
The
candidates
are already being sorted, see the sort
function, to sort the languages by language, use localeCompare
on their lang
properties– CertainPerformance
Nov 22 '18 at 6:02
The
candidates
are already being sorted, see the sort
function, to sort the languages by language, use localeCompare
on their lang
properties– CertainPerformance
Nov 22 '18 at 6:02
Can I use this to make first letter of the names like lowercase? name = name.charAt(0).toUpperCase() + name.slice(1); a.candidates.push({ name, age, phone });
– Riller Vincci
Nov 22 '18 at 6:20
Can I use this to make first letter of the names like lowercase? name = name.charAt(0).toUpperCase() + name.slice(1); a.candidates.push({ name, age, phone });
– Riller Vincci
Nov 22 '18 at 6:20
You want to make each first character uppercase? Yep, that code looks like it would work, but note that you can use bracket notation instead of
charAt
for less syntax noise, eg name[0].toUpperCase()
– CertainPerformance
Nov 22 '18 at 6:21
You want to make each first character uppercase? Yep, that code looks like it would work, but note that you can use bracket notation instead of
charAt
for less syntax noise, eg name[0].toUpperCase()
– CertainPerformance
Nov 22 '18 at 6:21
Bracket notation? that's new to me
– Riller Vincci
Nov 22 '18 at 6:24
Bracket notation? that's new to me
– Riller Vincci
Nov 22 '18 at 6:24
|
show 2 more comments
It's common practice to do everything in a reduce()
, but sometimes it's easier to read if you break it up a bit. This creates a counter
object as a helper to to track the language counts. map()
s over the array to pull out the languages and personal info and then puts it all together:
let arr = [ {"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let lang_counter = {
// helper keeps counts of unique items
counts:{},
add(arr){
arr.forEach(item => this.counts[item] = this.counts[item] ? this.counts[item] + 1 : 1)
},
toarray(){
return Object.entries(this.counts).map(([key, val]) => ({[key]: val}))
}
}
// iterate over object to create candidates
let candidates = arr.map(row => {
let {languages, ...person} = row
lang_counter.add(languages) // side effect
return person
})
// put them together
console.log({candidates, languages:lang_counter.toarray()})
Yeaah That's nice bro. I've changed some things to make all names receive the first letter in uppercase and organize all languages in alphabetical order. Thank you!!!
– Riller Vincci
Nov 22 '18 at 6:40
add a comment |
It's common practice to do everything in a reduce()
, but sometimes it's easier to read if you break it up a bit. This creates a counter
object as a helper to to track the language counts. map()
s over the array to pull out the languages and personal info and then puts it all together:
let arr = [ {"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let lang_counter = {
// helper keeps counts of unique items
counts:{},
add(arr){
arr.forEach(item => this.counts[item] = this.counts[item] ? this.counts[item] + 1 : 1)
},
toarray(){
return Object.entries(this.counts).map(([key, val]) => ({[key]: val}))
}
}
// iterate over object to create candidates
let candidates = arr.map(row => {
let {languages, ...person} = row
lang_counter.add(languages) // side effect
return person
})
// put them together
console.log({candidates, languages:lang_counter.toarray()})
Yeaah That's nice bro. I've changed some things to make all names receive the first letter in uppercase and organize all languages in alphabetical order. Thank you!!!
– Riller Vincci
Nov 22 '18 at 6:40
add a comment |
It's common practice to do everything in a reduce()
, but sometimes it's easier to read if you break it up a bit. This creates a counter
object as a helper to to track the language counts. map()
s over the array to pull out the languages and personal info and then puts it all together:
let arr = [ {"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let lang_counter = {
// helper keeps counts of unique items
counts:{},
add(arr){
arr.forEach(item => this.counts[item] = this.counts[item] ? this.counts[item] + 1 : 1)
},
toarray(){
return Object.entries(this.counts).map(([key, val]) => ({[key]: val}))
}
}
// iterate over object to create candidates
let candidates = arr.map(row => {
let {languages, ...person} = row
lang_counter.add(languages) // side effect
return person
})
// put them together
console.log({candidates, languages:lang_counter.toarray()})
It's common practice to do everything in a reduce()
, but sometimes it's easier to read if you break it up a bit. This creates a counter
object as a helper to to track the language counts. map()
s over the array to pull out the languages and personal info and then puts it all together:
let arr = [ {"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let lang_counter = {
// helper keeps counts of unique items
counts:{},
add(arr){
arr.forEach(item => this.counts[item] = this.counts[item] ? this.counts[item] + 1 : 1)
},
toarray(){
return Object.entries(this.counts).map(([key, val]) => ({[key]: val}))
}
}
// iterate over object to create candidates
let candidates = arr.map(row => {
let {languages, ...person} = row
lang_counter.add(languages) // side effect
return person
})
// put them together
console.log({candidates, languages:lang_counter.toarray()})
let arr = [ {"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let lang_counter = {
// helper keeps counts of unique items
counts:{},
add(arr){
arr.forEach(item => this.counts[item] = this.counts[item] ? this.counts[item] + 1 : 1)
},
toarray(){
return Object.entries(this.counts).map(([key, val]) => ({[key]: val}))
}
}
// iterate over object to create candidates
let candidates = arr.map(row => {
let {languages, ...person} = row
lang_counter.add(languages) // side effect
return person
})
// put them together
console.log({candidates, languages:lang_counter.toarray()})
let arr = [ {"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let lang_counter = {
// helper keeps counts of unique items
counts:{},
add(arr){
arr.forEach(item => this.counts[item] = this.counts[item] ? this.counts[item] + 1 : 1)
},
toarray(){
return Object.entries(this.counts).map(([key, val]) => ({[key]: val}))
}
}
// iterate over object to create candidates
let candidates = arr.map(row => {
let {languages, ...person} = row
lang_counter.add(languages) // side effect
return person
})
// put them together
console.log({candidates, languages:lang_counter.toarray()})
answered Nov 22 '18 at 6:14


Mark MeyerMark Meyer
37.5k33159
37.5k33159
Yeaah That's nice bro. I've changed some things to make all names receive the first letter in uppercase and organize all languages in alphabetical order. Thank you!!!
– Riller Vincci
Nov 22 '18 at 6:40
add a comment |
Yeaah That's nice bro. I've changed some things to make all names receive the first letter in uppercase and organize all languages in alphabetical order. Thank you!!!
– Riller Vincci
Nov 22 '18 at 6:40
Yeaah That's nice bro. I've changed some things to make all names receive the first letter in uppercase and organize all languages in alphabetical order. Thank you!!!
– Riller Vincci
Nov 22 '18 at 6:40
Yeaah That's nice bro. I've changed some things to make all names receive the first letter in uppercase and organize all languages in alphabetical order. Thank you!!!
– Riller Vincci
Nov 22 '18 at 6:40
add a comment |
You can use Array.reduce
and Object.values
like below
let arr = [{"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let res = arr.reduce((o, {name, age, phone, graduate_date, languages}) => {
o.candidates.push({name, age, phone, graduate_date})
languages.forEach(l => {
o.languages[l] = o.languages[l] || { lang:l, count: 0 }
o.languages[l].count++
})
return o
}
, { candidates: , languages: {}})
res.candidates = res.candidates.sort((a,b) => a.graduate_date - b.graduate_date)
.map(({ graduate_date, ...rest }) => rest)
res.languages = Object.values(res.languages).sort((a,b) => a.lang.localeCompare(b.lang))
console.log(res)
1
It's very interesting You've used map function to organize candidates Woow Congratulations... Thank you
– Riller Vincci
Nov 22 '18 at 6:44
add a comment |
You can use Array.reduce
and Object.values
like below
let arr = [{"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let res = arr.reduce((o, {name, age, phone, graduate_date, languages}) => {
o.candidates.push({name, age, phone, graduate_date})
languages.forEach(l => {
o.languages[l] = o.languages[l] || { lang:l, count: 0 }
o.languages[l].count++
})
return o
}
, { candidates: , languages: {}})
res.candidates = res.candidates.sort((a,b) => a.graduate_date - b.graduate_date)
.map(({ graduate_date, ...rest }) => rest)
res.languages = Object.values(res.languages).sort((a,b) => a.lang.localeCompare(b.lang))
console.log(res)
1
It's very interesting You've used map function to organize candidates Woow Congratulations... Thank you
– Riller Vincci
Nov 22 '18 at 6:44
add a comment |
You can use Array.reduce
and Object.values
like below
let arr = [{"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let res = arr.reduce((o, {name, age, phone, graduate_date, languages}) => {
o.candidates.push({name, age, phone, graduate_date})
languages.forEach(l => {
o.languages[l] = o.languages[l] || { lang:l, count: 0 }
o.languages[l].count++
})
return o
}
, { candidates: , languages: {}})
res.candidates = res.candidates.sort((a,b) => a.graduate_date - b.graduate_date)
.map(({ graduate_date, ...rest }) => rest)
res.languages = Object.values(res.languages).sort((a,b) => a.lang.localeCompare(b.lang))
console.log(res)
You can use Array.reduce
and Object.values
like below
let arr = [{"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let res = arr.reduce((o, {name, age, phone, graduate_date, languages}) => {
o.candidates.push({name, age, phone, graduate_date})
languages.forEach(l => {
o.languages[l] = o.languages[l] || { lang:l, count: 0 }
o.languages[l].count++
})
return o
}
, { candidates: , languages: {}})
res.candidates = res.candidates.sort((a,b) => a.graduate_date - b.graduate_date)
.map(({ graduate_date, ...rest }) => rest)
res.languages = Object.values(res.languages).sort((a,b) => a.lang.localeCompare(b.lang))
console.log(res)
let arr = [{"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let res = arr.reduce((o, {name, age, phone, graduate_date, languages}) => {
o.candidates.push({name, age, phone, graduate_date})
languages.forEach(l => {
o.languages[l] = o.languages[l] || { lang:l, count: 0 }
o.languages[l].count++
})
return o
}
, { candidates: , languages: {}})
res.candidates = res.candidates.sort((a,b) => a.graduate_date - b.graduate_date)
.map(({ graduate_date, ...rest }) => rest)
res.languages = Object.values(res.languages).sort((a,b) => a.lang.localeCompare(b.lang))
console.log(res)
let arr = [{"name":"george","languages":["php","javascript","java"],"age":19,"graduate_date":1044064800000,"phone":"32-991-511"},{"name":"anna","languages":["java","javascript"],"age":23,"graduate_date":1391220000000,"phone":"32-991-512"},{"name":"hailee","languages":["regex","javascript","perl","go","java"],"age":31,"graduate_date":1296525600000,"phone":"32-991-513"}]
let res = arr.reduce((o, {name, age, phone, graduate_date, languages}) => {
o.candidates.push({name, age, phone, graduate_date})
languages.forEach(l => {
o.languages[l] = o.languages[l] || { lang:l, count: 0 }
o.languages[l].count++
})
return o
}
, { candidates: , languages: {}})
res.candidates = res.candidates.sort((a,b) => a.graduate_date - b.graduate_date)
.map(({ graduate_date, ...rest }) => rest)
res.languages = Object.values(res.languages).sort((a,b) => a.lang.localeCompare(b.lang))
console.log(res)
edited Nov 22 '18 at 6:40
answered Nov 22 '18 at 6:05


Nitish NarangNitish Narang
2,948815
2,948815
1
It's very interesting You've used map function to organize candidates Woow Congratulations... Thank you
– Riller Vincci
Nov 22 '18 at 6:44
add a comment |
1
It's very interesting You've used map function to organize candidates Woow Congratulations... Thank you
– Riller Vincci
Nov 22 '18 at 6:44
1
1
It's very interesting You've used map function to organize candidates Woow Congratulations... Thank you
– Riller Vincci
Nov 22 '18 at 6:44
It's very interesting You've used map function to organize candidates Woow Congratulations... Thank you
– Riller Vincci
Nov 22 '18 at 6:44
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53424524%2fhow-would-i-write-this-reportcandidates-function-better%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1Gr4tNGvu,kXbrELPTizw qyOW3w