R How to make a POST request to MongoDB
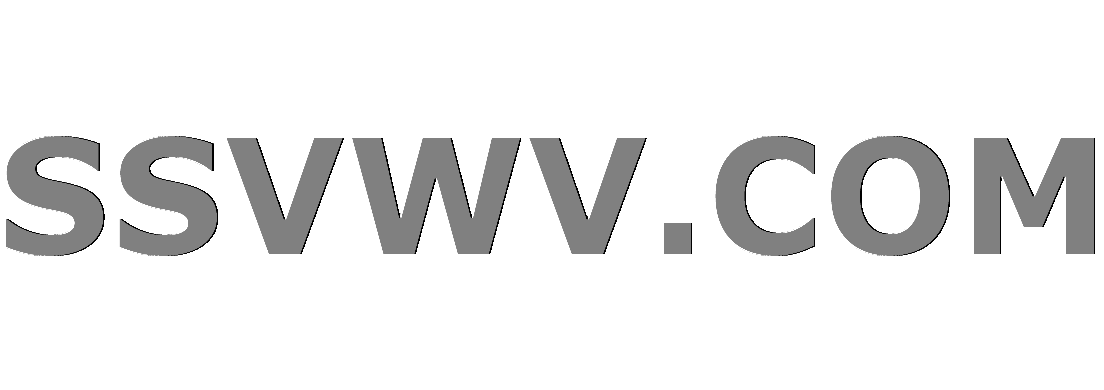
Multi tool use
I'm currently working with data from an online database. I access the data via API, which works when I retrieve all data at once. But this makes my system slow, so I want to make a request only for filtered data (which I did not make until now). This is the way to get the whole dataset:
#-------------------------------#
# packages #
#-------------------------------#
library(httr)
library(jsonlite)
#-------------------------------#
# API requests #
#-------------------------------#
## get all data at once ##
url <- "https://www.eter-project.com/api/3.0/HEIs/full"
raw_result <- GET(url)
#-------------------------------#
# data processing #
#-------------------------------#
# 'status_code' (if request worked) and 'content' (APIs answer) important
names(raw_result)
# '200' tells us that server received request
raw_result$status_code
# translate Unicode into text
this.raw.content <- rawToChar(raw_result$content)
# transform json into workable format for R
mydata <- fromJSON(this.raw.content, flatten = TRUE)
class(mydata)
dim(mydata)
According to the documentation (https://www.eter-project.com/api/doc/#/) I need a POST request using url https://www.eter-project.com/api/3.0/HEIs/query
and a filter embedded in the following structure:
{
"filter": {},
"fieldIds": {}
}
I want to filter for years and countries in order to only get the data I currently want to work with. The structure for the filter would be
{ "BAS.REFYEAR.v": 2011, "BAS.COUNTRY.v": "AT"}
.
Has anyone an idea, how I could implement this into a POST request?
Until now, I made some desperate attempts to include the filter into the POST requests (e.g. raw_result <- POST(url, body = list({
"filter": {"BAS.REFYEAR.v" = 2011}}), encode = "json")
and played around with the mongolite
package, which was not even close.
UPDATE: the filtering problem has been solved. I used the following solution:
myquery <- '{
"filter": {"BAS.REFYEAR.v": 2015, "BAS.COUNTRY.v": "LV"},
"fieldIds": {},
"searchTerms":
}'
url <- "https://www.eter-project.com/api/3.0/HEIs/query"
raw_result <- POST(url, body = myquery, content_type_json())
Now, I face another problem: the data include many special characters, which are not displayed properly in R (e.g. Alberta koledža
in the dataset is displayed as Alberta koledžain
R). Is there a way to solve this, for example by using UTF-8 in the request call?
r json mongodb api post
add a comment |
I'm currently working with data from an online database. I access the data via API, which works when I retrieve all data at once. But this makes my system slow, so I want to make a request only for filtered data (which I did not make until now). This is the way to get the whole dataset:
#-------------------------------#
# packages #
#-------------------------------#
library(httr)
library(jsonlite)
#-------------------------------#
# API requests #
#-------------------------------#
## get all data at once ##
url <- "https://www.eter-project.com/api/3.0/HEIs/full"
raw_result <- GET(url)
#-------------------------------#
# data processing #
#-------------------------------#
# 'status_code' (if request worked) and 'content' (APIs answer) important
names(raw_result)
# '200' tells us that server received request
raw_result$status_code
# translate Unicode into text
this.raw.content <- rawToChar(raw_result$content)
# transform json into workable format for R
mydata <- fromJSON(this.raw.content, flatten = TRUE)
class(mydata)
dim(mydata)
According to the documentation (https://www.eter-project.com/api/doc/#/) I need a POST request using url https://www.eter-project.com/api/3.0/HEIs/query
and a filter embedded in the following structure:
{
"filter": {},
"fieldIds": {}
}
I want to filter for years and countries in order to only get the data I currently want to work with. The structure for the filter would be
{ "BAS.REFYEAR.v": 2011, "BAS.COUNTRY.v": "AT"}
.
Has anyone an idea, how I could implement this into a POST request?
Until now, I made some desperate attempts to include the filter into the POST requests (e.g. raw_result <- POST(url, body = list({
"filter": {"BAS.REFYEAR.v" = 2011}}), encode = "json")
and played around with the mongolite
package, which was not even close.
UPDATE: the filtering problem has been solved. I used the following solution:
myquery <- '{
"filter": {"BAS.REFYEAR.v": 2015, "BAS.COUNTRY.v": "LV"},
"fieldIds": {},
"searchTerms":
}'
url <- "https://www.eter-project.com/api/3.0/HEIs/query"
raw_result <- POST(url, body = myquery, content_type_json())
Now, I face another problem: the data include many special characters, which are not displayed properly in R (e.g. Alberta koledža
in the dataset is displayed as Alberta koledžain
R). Is there a way to solve this, for example by using UTF-8 in the request call?
r json mongodb api post
Have you triedhttr::POST()
? BTW, there are special packages on CRAN for interfacing with MongoDB.
– Ralf Stubner
Nov 23 '18 at 11:39
Yes, I triedPOST(url, _something_)
and also checked a lot of discussions, blog posts, documentations etc. But my problem is to technically implement the filter into the call.
– huan
Nov 23 '18 at 12:25
Please edit your question to include what you have tried and the relevant results of your attempts. BTW, did you read this: eter-project.com/#/info/api?
– Ralf Stubner
Nov 23 '18 at 12:27
add a comment |
I'm currently working with data from an online database. I access the data via API, which works when I retrieve all data at once. But this makes my system slow, so I want to make a request only for filtered data (which I did not make until now). This is the way to get the whole dataset:
#-------------------------------#
# packages #
#-------------------------------#
library(httr)
library(jsonlite)
#-------------------------------#
# API requests #
#-------------------------------#
## get all data at once ##
url <- "https://www.eter-project.com/api/3.0/HEIs/full"
raw_result <- GET(url)
#-------------------------------#
# data processing #
#-------------------------------#
# 'status_code' (if request worked) and 'content' (APIs answer) important
names(raw_result)
# '200' tells us that server received request
raw_result$status_code
# translate Unicode into text
this.raw.content <- rawToChar(raw_result$content)
# transform json into workable format for R
mydata <- fromJSON(this.raw.content, flatten = TRUE)
class(mydata)
dim(mydata)
According to the documentation (https://www.eter-project.com/api/doc/#/) I need a POST request using url https://www.eter-project.com/api/3.0/HEIs/query
and a filter embedded in the following structure:
{
"filter": {},
"fieldIds": {}
}
I want to filter for years and countries in order to only get the data I currently want to work with. The structure for the filter would be
{ "BAS.REFYEAR.v": 2011, "BAS.COUNTRY.v": "AT"}
.
Has anyone an idea, how I could implement this into a POST request?
Until now, I made some desperate attempts to include the filter into the POST requests (e.g. raw_result <- POST(url, body = list({
"filter": {"BAS.REFYEAR.v" = 2011}}), encode = "json")
and played around with the mongolite
package, which was not even close.
UPDATE: the filtering problem has been solved. I used the following solution:
myquery <- '{
"filter": {"BAS.REFYEAR.v": 2015, "BAS.COUNTRY.v": "LV"},
"fieldIds": {},
"searchTerms":
}'
url <- "https://www.eter-project.com/api/3.0/HEIs/query"
raw_result <- POST(url, body = myquery, content_type_json())
Now, I face another problem: the data include many special characters, which are not displayed properly in R (e.g. Alberta koledža
in the dataset is displayed as Alberta koledžain
R). Is there a way to solve this, for example by using UTF-8 in the request call?
r json mongodb api post
I'm currently working with data from an online database. I access the data via API, which works when I retrieve all data at once. But this makes my system slow, so I want to make a request only for filtered data (which I did not make until now). This is the way to get the whole dataset:
#-------------------------------#
# packages #
#-------------------------------#
library(httr)
library(jsonlite)
#-------------------------------#
# API requests #
#-------------------------------#
## get all data at once ##
url <- "https://www.eter-project.com/api/3.0/HEIs/full"
raw_result <- GET(url)
#-------------------------------#
# data processing #
#-------------------------------#
# 'status_code' (if request worked) and 'content' (APIs answer) important
names(raw_result)
# '200' tells us that server received request
raw_result$status_code
# translate Unicode into text
this.raw.content <- rawToChar(raw_result$content)
# transform json into workable format for R
mydata <- fromJSON(this.raw.content, flatten = TRUE)
class(mydata)
dim(mydata)
According to the documentation (https://www.eter-project.com/api/doc/#/) I need a POST request using url https://www.eter-project.com/api/3.0/HEIs/query
and a filter embedded in the following structure:
{
"filter": {},
"fieldIds": {}
}
I want to filter for years and countries in order to only get the data I currently want to work with. The structure for the filter would be
{ "BAS.REFYEAR.v": 2011, "BAS.COUNTRY.v": "AT"}
.
Has anyone an idea, how I could implement this into a POST request?
Until now, I made some desperate attempts to include the filter into the POST requests (e.g. raw_result <- POST(url, body = list({
"filter": {"BAS.REFYEAR.v" = 2011}}), encode = "json")
and played around with the mongolite
package, which was not even close.
UPDATE: the filtering problem has been solved. I used the following solution:
myquery <- '{
"filter": {"BAS.REFYEAR.v": 2015, "BAS.COUNTRY.v": "LV"},
"fieldIds": {},
"searchTerms":
}'
url <- "https://www.eter-project.com/api/3.0/HEIs/query"
raw_result <- POST(url, body = myquery, content_type_json())
Now, I face another problem: the data include many special characters, which are not displayed properly in R (e.g. Alberta koledža
in the dataset is displayed as Alberta koledžain
R). Is there a way to solve this, for example by using UTF-8 in the request call?
r json mongodb api post
r json mongodb api post
edited Nov 23 '18 at 13:50
huan
asked Nov 23 '18 at 11:35
huanhuan
6410
6410
Have you triedhttr::POST()
? BTW, there are special packages on CRAN for interfacing with MongoDB.
– Ralf Stubner
Nov 23 '18 at 11:39
Yes, I triedPOST(url, _something_)
and also checked a lot of discussions, blog posts, documentations etc. But my problem is to technically implement the filter into the call.
– huan
Nov 23 '18 at 12:25
Please edit your question to include what you have tried and the relevant results of your attempts. BTW, did you read this: eter-project.com/#/info/api?
– Ralf Stubner
Nov 23 '18 at 12:27
add a comment |
Have you triedhttr::POST()
? BTW, there are special packages on CRAN for interfacing with MongoDB.
– Ralf Stubner
Nov 23 '18 at 11:39
Yes, I triedPOST(url, _something_)
and also checked a lot of discussions, blog posts, documentations etc. But my problem is to technically implement the filter into the call.
– huan
Nov 23 '18 at 12:25
Please edit your question to include what you have tried and the relevant results of your attempts. BTW, did you read this: eter-project.com/#/info/api?
– Ralf Stubner
Nov 23 '18 at 12:27
Have you tried
httr::POST()
? BTW, there are special packages on CRAN for interfacing with MongoDB.– Ralf Stubner
Nov 23 '18 at 11:39
Have you tried
httr::POST()
? BTW, there are special packages on CRAN for interfacing with MongoDB.– Ralf Stubner
Nov 23 '18 at 11:39
Yes, I tried
POST(url, _something_)
and also checked a lot of discussions, blog posts, documentations etc. But my problem is to technically implement the filter into the call.– huan
Nov 23 '18 at 12:25
Yes, I tried
POST(url, _something_)
and also checked a lot of discussions, blog posts, documentations etc. But my problem is to technically implement the filter into the call.– huan
Nov 23 '18 at 12:25
Please edit your question to include what you have tried and the relevant results of your attempts. BTW, did you read this: eter-project.com/#/info/api?
– Ralf Stubner
Nov 23 '18 at 12:27
Please edit your question to include what you have tried and the relevant results of your attempts. BTW, did you read this: eter-project.com/#/info/api?
– Ralf Stubner
Nov 23 '18 at 12:27
add a comment |
1 Answer
1
active
oldest
votes
You can try to build the required JSON as list of lists. However, I find it easier to supply the JSON explicitly and add the content type manually:
query <- '{
"filter": { "BAS.REFYEAR.v": 2011, "BAS.COUNTRY.v": "AT"},
"fieldIds": {},
"searchTerms":
}'
url <- "https://www.eter-project.com/api/3.0/HEIs/query"
raw_result <- httr::POST(url = url, body = query, content_type_json())
After this you can apply your processing as before.
Awesome, this works perfectly well. I still have a problem with the names, since there are many special characters in them (e.g.Alberta koledža
in the dataset is displayed asAlberta koledža
in R). Is there a way to solve this, e.g. by using UTF-8?
– huan
Nov 23 '18 at 13:48
@huan That looks like the UTF-8 representation of koledža interpreted with a a 8bit encoding like Latin-1. But please do not add additional issues to a solved question.
– Ralf Stubner
Nov 23 '18 at 14:28
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53445960%2fr-how-to-make-a-post-request-to-mongodb%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can try to build the required JSON as list of lists. However, I find it easier to supply the JSON explicitly and add the content type manually:
query <- '{
"filter": { "BAS.REFYEAR.v": 2011, "BAS.COUNTRY.v": "AT"},
"fieldIds": {},
"searchTerms":
}'
url <- "https://www.eter-project.com/api/3.0/HEIs/query"
raw_result <- httr::POST(url = url, body = query, content_type_json())
After this you can apply your processing as before.
Awesome, this works perfectly well. I still have a problem with the names, since there are many special characters in them (e.g.Alberta koledža
in the dataset is displayed asAlberta koledža
in R). Is there a way to solve this, e.g. by using UTF-8?
– huan
Nov 23 '18 at 13:48
@huan That looks like the UTF-8 representation of koledža interpreted with a a 8bit encoding like Latin-1. But please do not add additional issues to a solved question.
– Ralf Stubner
Nov 23 '18 at 14:28
add a comment |
You can try to build the required JSON as list of lists. However, I find it easier to supply the JSON explicitly and add the content type manually:
query <- '{
"filter": { "BAS.REFYEAR.v": 2011, "BAS.COUNTRY.v": "AT"},
"fieldIds": {},
"searchTerms":
}'
url <- "https://www.eter-project.com/api/3.0/HEIs/query"
raw_result <- httr::POST(url = url, body = query, content_type_json())
After this you can apply your processing as before.
Awesome, this works perfectly well. I still have a problem with the names, since there are many special characters in them (e.g.Alberta koledža
in the dataset is displayed asAlberta koledža
in R). Is there a way to solve this, e.g. by using UTF-8?
– huan
Nov 23 '18 at 13:48
@huan That looks like the UTF-8 representation of koledža interpreted with a a 8bit encoding like Latin-1. But please do not add additional issues to a solved question.
– Ralf Stubner
Nov 23 '18 at 14:28
add a comment |
You can try to build the required JSON as list of lists. However, I find it easier to supply the JSON explicitly and add the content type manually:
query <- '{
"filter": { "BAS.REFYEAR.v": 2011, "BAS.COUNTRY.v": "AT"},
"fieldIds": {},
"searchTerms":
}'
url <- "https://www.eter-project.com/api/3.0/HEIs/query"
raw_result <- httr::POST(url = url, body = query, content_type_json())
After this you can apply your processing as before.
You can try to build the required JSON as list of lists. However, I find it easier to supply the JSON explicitly and add the content type manually:
query <- '{
"filter": { "BAS.REFYEAR.v": 2011, "BAS.COUNTRY.v": "AT"},
"fieldIds": {},
"searchTerms":
}'
url <- "https://www.eter-project.com/api/3.0/HEIs/query"
raw_result <- httr::POST(url = url, body = query, content_type_json())
After this you can apply your processing as before.
answered Nov 23 '18 at 12:45


Ralf StubnerRalf Stubner
14.1k21537
14.1k21537
Awesome, this works perfectly well. I still have a problem with the names, since there are many special characters in them (e.g.Alberta koledža
in the dataset is displayed asAlberta koledža
in R). Is there a way to solve this, e.g. by using UTF-8?
– huan
Nov 23 '18 at 13:48
@huan That looks like the UTF-8 representation of koledža interpreted with a a 8bit encoding like Latin-1. But please do not add additional issues to a solved question.
– Ralf Stubner
Nov 23 '18 at 14:28
add a comment |
Awesome, this works perfectly well. I still have a problem with the names, since there are many special characters in them (e.g.Alberta koledža
in the dataset is displayed asAlberta koledža
in R). Is there a way to solve this, e.g. by using UTF-8?
– huan
Nov 23 '18 at 13:48
@huan That looks like the UTF-8 representation of koledža interpreted with a a 8bit encoding like Latin-1. But please do not add additional issues to a solved question.
– Ralf Stubner
Nov 23 '18 at 14:28
Awesome, this works perfectly well. I still have a problem with the names, since there are many special characters in them (e.g.
Alberta koledža
in the dataset is displayed as Alberta koledža
in R). Is there a way to solve this, e.g. by using UTF-8?– huan
Nov 23 '18 at 13:48
Awesome, this works perfectly well. I still have a problem with the names, since there are many special characters in them (e.g.
Alberta koledža
in the dataset is displayed as Alberta koledža
in R). Is there a way to solve this, e.g. by using UTF-8?– huan
Nov 23 '18 at 13:48
@huan That looks like the UTF-8 representation of koledža interpreted with a a 8bit encoding like Latin-1. But please do not add additional issues to a solved question.
– Ralf Stubner
Nov 23 '18 at 14:28
@huan That looks like the UTF-8 representation of koledža interpreted with a a 8bit encoding like Latin-1. But please do not add additional issues to a solved question.
– Ralf Stubner
Nov 23 '18 at 14:28
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53445960%2fr-how-to-make-a-post-request-to-mongodb%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
lG,ikkyRXqVvZYJjHQ64xtqxFSjHhSIg GO52 iy tIwMd a
Have you tried
httr::POST()
? BTW, there are special packages on CRAN for interfacing with MongoDB.– Ralf Stubner
Nov 23 '18 at 11:39
Yes, I tried
POST(url, _something_)
and also checked a lot of discussions, blog posts, documentations etc. But my problem is to technically implement the filter into the call.– huan
Nov 23 '18 at 12:25
Please edit your question to include what you have tried and the relevant results of your attempts. BTW, did you read this: eter-project.com/#/info/api?
– Ralf Stubner
Nov 23 '18 at 12:27